List of lists changes reflected across sublists unexpectedly
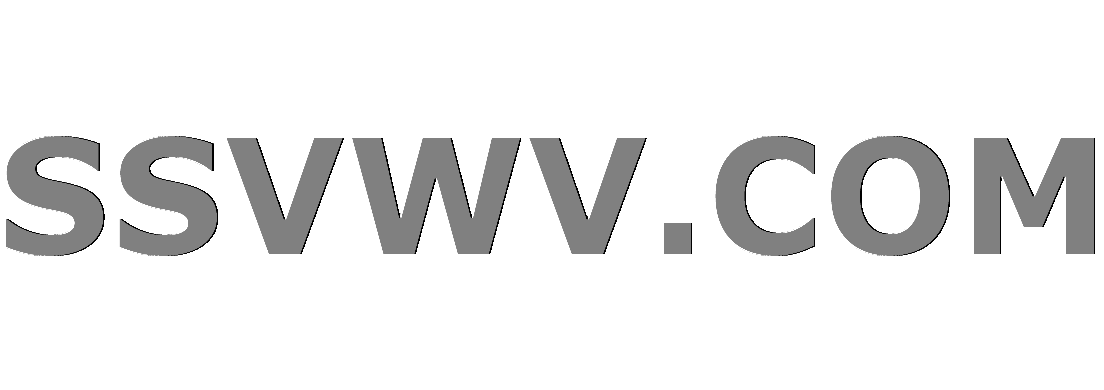
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I needed to create a list of lists in Python, so I typed the following:
myList = [[1] * 4] * 3
The list looked like this:
[[1, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
Then I changed one of the innermost values:
myList[0][0] = 5
Now my list looks like this:
[[5, 1, 1, 1], [5, 1, 1, 1], [5, 1, 1, 1]]
which is not what I wanted or expected. Can someone please explain what's going on, and how to get around it?
python list nested-lists mutable
add a comment |
I needed to create a list of lists in Python, so I typed the following:
myList = [[1] * 4] * 3
The list looked like this:
[[1, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
Then I changed one of the innermost values:
myList[0][0] = 5
Now my list looks like this:
[[5, 1, 1, 1], [5, 1, 1, 1], [5, 1, 1, 1]]
which is not what I wanted or expected. Can someone please explain what's going on, and how to get around it?
python list nested-lists mutable
add a comment |
I needed to create a list of lists in Python, so I typed the following:
myList = [[1] * 4] * 3
The list looked like this:
[[1, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
Then I changed one of the innermost values:
myList[0][0] = 5
Now my list looks like this:
[[5, 1, 1, 1], [5, 1, 1, 1], [5, 1, 1, 1]]
which is not what I wanted or expected. Can someone please explain what's going on, and how to get around it?
python list nested-lists mutable
I needed to create a list of lists in Python, so I typed the following:
myList = [[1] * 4] * 3
The list looked like this:
[[1, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
Then I changed one of the innermost values:
myList[0][0] = 5
Now my list looks like this:
[[5, 1, 1, 1], [5, 1, 1, 1], [5, 1, 1, 1]]
which is not what I wanted or expected. Can someone please explain what's going on, and how to get around it?
python list nested-lists mutable
python list nested-lists mutable
edited Mar 22 '17 at 16:14
Taryn♦
194k47295358
194k47295358
asked Oct 27 '08 at 14:57


Charles AndersonCharles Anderson
7,648124966
7,648124966
add a comment |
add a comment |
12 Answers
12
active
oldest
votes
When you write [x]*3
you get, essentially, the list [x, x, x]
. That is, a list with 3 references to the same x
. When you then modify this single x
it is visible via all three references to it.
To fix it, you need to make sure that you create a new list at each position. One way to do it is
[[1]*4 for _ in range(3)]
which will reevaluate [1]*4
each time instead of evaluating it once and making 3 references to 1 list.
You might wonder why *
can't make independent objects the way the list comprehension does. That's because the multiplication operator *
operates on objects, without seeing expressions. When you use *
to multiply [[1] * 4]
by 3, *
only sees the 1-element list [[1] * 4]
evaluates to, not the [[1] * 4
expression text. *
has no idea how to make copies of that element, no idea how to reevaluate [[1] * 4]
, and no idea you even want copies, and in general, there might not even be a way to copy the element.
The only option *
has is to make new references to the existing sublist instead of trying to make new sublists. Anything else would be inconsistent or require major redesigning of fundamental language design decisions.
In contrast, a list comprehension reevaluates the element expression on every iteration. [[1] * 4 for n in range(3)]
reevaluates [1] * 4
every time for the same reason [x**2 for x in range(3)]
reevaluates x**2
every time. Every evaluation of [1] * 4
generates a new list, so the list comprehension does what you wanted.
Incidentally, [1] * 4
also doesn't copy the elements of [1]
, but that doesn't matter, since integers are immutable. You can't do something like 1.value = 2
and turn a 1 into a 2.
2
Thanks for the explanation and the workaround. I am still surprised though that the outer list ends up holding three references to an anonymous inner list.
– Charles Anderson
Oct 27 '08 at 15:40
18
I am surprised that no body points out that, the answer here is misleading.[x]*3
store 3 references like[x, x, x]
is only right whenx
is mutable. This does't work for e.g.a=[4]*3
, where aftera[0]=5
,a=[5,4,4].
– Allanqunzi
May 22 '15 at 0:16
30
Technically, it's still correct.[4]*3
is essentially equivalent tox = 4; [x, x, x]
. It's true, though, that this will never cause any problem since4
is immutable. Also, your other example isn't really a different case.a = [x]*3; a[0] = 5
won't cause problems even ifx
is mutable, since you're not modifyingx
, only modifyinga
. I wouldn't describe my answer as misleading or incorrect - you just can't shoot yourself in the foot if you're dealing with immutable objects.
– CAdaker
May 22 '15 at 8:04
14
@Allanqunzi you are wrong. Dox = 1000; lst = [x]*2; lst[0] is lst[1]
->True
. Python does not distinguish between mutable and immutable objects here whatsoever.
– timgeb
Apr 17 '16 at 18:08
2
@ᴡʜᴀᴄᴋᴀᴍᴀᴅᴏᴏᴅʟᴇ3000import ctypes; ctypes.cast(id(1), ctypes.POINTER(ctypes.c_int))[6] = 2
, but prepare for weird behavior or a segfault.
– Zach Gates
Aug 4 '18 at 16:33
|
show 3 more comments
size = 3
matrix_surprise = [[0] * size] * size
matrix = [[0]*size for i in range(size)]
Live Python Tutor Visualize
4
I've put your image inline. For future reference, you really need to explain what you're linking to.
– Blckknght
Aug 27 '13 at 0:42
2
Great tool! Thanks for the reference
– Dennis
Feb 27 '16 at 15:43
So, why if we write matrix= [[x] * 2] doesn't make 2 elemnts for the same object like the example you describe, it seems to be the same concept, what am i missing?
– Ahmed Mohamed
Jul 1 '17 at 17:55
@AhmedMohamed Indeed it does make a list with two elements of the exact same object thatx
refers to. If you make a globally unique object withx = object()
and then makematrix = [[x] * 2]
these does come as true:matrix[0][0] is matrix[0][1]
– nadrimajstor
Jul 2 '17 at 13:13
@nadrimajstor so why the change in matrix[0] doesn't affect matrix[1] like the example above with 2d matrix.
– Ahmed Mohamed
Jul 2 '17 at 13:31
|
show 2 more comments
Actually, this is exactly what you would expect. Let's decompose what is happening here:
You write
lst = [[1] * 4] * 3
This is equivalent to:
lst1 = [1]*4
lst = [lst1]*3
This means lst
is a list with 3 elements all pointing to lst1
. This means the two following lines are equivalent:
lst[0][0] = 5
lst1[0] = 5
As lst[0]
is nothing but lst1
.
To obtain the desired behavior, you can use list comprehension:
lst = [ [1]*4 for n in xrange(3) ]
In this case, the expression is re-evaluated for each n, leading to a different list.
This is really clear for a beginner like me. Thank you!
– Petite Etincelle
Apr 29 '16 at 8:18
Just a small addition to the nice answer here: it's evident that you're dealing with same object if you doid(lst[0][0])
andid(lst[1][0])
or evenid(lst[0])
andid(lst[1])
– Sergiy Kolodyazhnyy
May 17 '17 at 7:08
add a comment |
[[1] * 4] * 3
or even:
[[1, 1, 1, 1]] * 3
Creates a list that references the internal [1,1,1,1]
3 times - not three copies of the inner list, so any time you modify the list (in any position), you'll see the change three times.
It's the same as this example:
>>> inner = [1,1,1,1]
>>> outer = [inner]*3
>>> outer
[[1, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
>>> inner[0] = 5
>>> outer
[[5, 1, 1, 1], [5, 1, 1, 1], [5, 1, 1, 1]]
where it's probably a little less surprising.
2
You can use the "is" operator to discover this. ls[0] is ls[1] returns True.
– mipadi
Oct 27 '08 at 15:03
add a comment |
Alongside the accepted answer that explained the problem correctly, within your list comprehension, if You are using python-2.x use xrange()
that returns a generator which is more efficient (range()
in python 3 does the same job) _
instead of the throwaway variable n
:
[[1]*4 for _ in xrange(3)] # and in python3 [[1]*4 for _ in range(3)]
Also, as a much more Pythonic way you can use itertools.repeat()
to create an iterator object of repeated elements :
>>> a=list(repeat(1,4))
[1, 1, 1, 1]
>>> a[0]=5
>>> a
[5, 1, 1, 1]
P.S. Using numpy, if you only want to create an array of ones or zeroes you can use np.ones
and np.zeros
and/or for other number use np.repeat()
:
In [1]: import numpy as np
In [2]:
In [2]: np.ones(4)
Out[2]: array([ 1., 1., 1., 1.])
In [3]: np.ones((4, 2))
Out[3]:
array([[ 1., 1.],
[ 1., 1.],
[ 1., 1.],
[ 1., 1.]])
In [4]: np.zeros((4, 2))
Out[4]:
array([[ 0., 0.],
[ 0., 0.],
[ 0., 0.],
[ 0., 0.]])
In [5]: np.repeat([7], 10)
Out[5]: array([7, 7, 7, 7, 7, 7, 7, 7, 7, 7])
add a comment |
In simple words this is happening because in python everything works by reference, so when you create a list of list that way you basically end up with such problems.
To solve your issue you can do either one of them:
1. Use numpy array documentation for numpy.empty
2. Append the list as you get to a list.
3. You can also use dictionary if you want
add a comment |
Python containers contain references to other objects. See this example:
>>> a =
>>> b = [a]
>>> b
[]
>>> a.append(1)
>>> b
[[1]]
In this b
is a list that contains one item that is a reference to list a
. The list a
is mutable.
The multiplication of a list by an integer is equivalent to adding the list to itself multiple times (see common sequence operations). So continuing with the example:
>>> c = b + b
>>> c
[[1], [1]]
>>>
>>> a[0] = 2
>>> c
[[2], [2]]
We can see that the list c
now contains two references to list a
which is equivalent to c = b * 2
.
Python FAQ also contains explanation of this behavior: How do I create a multidimensional list?
add a comment |
myList = [[1]*4] * 3
creates one list object [1,1,1,1]
in memory and copies its reference 3 times over. This is equivalent to obj = [1,1,1,1]; myList = [obj]*3
. Any modification to obj
will be reflected at three places, wherever obj
is referenced in the list.
The right statement would be:
myList = [[1]*4 for _ in range(3)]
or
myList = [[1 for __ in range(4)] for _ in range(3)]
Important thing to note here is that *
operator is mostly used to create a list of literals. Since 1
is a literal, hence obj =[1]*4
will create [1,1,1,1]
where each 1
is atomic and not a reference of 1
repeated 4 times. This means if we do obj[2]=42
, then obj
will become [1,1,42,1]
not as some may assume.[42,42,42,42]
2
It's not about literals.obj[2] = 42
replaces the reference at index2
, as opposed to mutating the object referenced by that index, which is whatmyList[2][0] = ...
does (myList[2]
is a list, and the assigment alters the reference at index 0 in tha list). Of course, integers are not mutable, but plenty of object types are. And note that the[....]
list display notation is also a form of literal syntax! Don't confuse compound (such as lists) and scalar objects (such as integers), with mutable vs. immutable objects.
– Martijn Pieters♦
Jul 25 '18 at 15:52
add a comment |
Let us rewrite your code in the following way:
x = 1
y = [x]
z = y * 4
myList = [z] * 3
Then having this, run the following code to make everything more clear. What the code does is basically print the id
s of the obtained objects, which
Return the “identity” of an object
and will help us identify them and analyse what happens:
print("myList:")
for i, subList in enumerate(myList):
print("t[{}]: {}".format(i, id(subList)))
for j, elem in enumerate(subList):
print("tt[{}]: {}".format(j, id(elem)))
And you will get the following output:
x: 1
y: [1]
z: [1, 1, 1, 1]
myList:
[0]: 4300763792
[0]: 4298171528
[1]: 4298171528
[2]: 4298171528
[3]: 4298171528
[1]: 4300763792
[0]: 4298171528
[1]: 4298171528
[2]: 4298171528
[3]: 4298171528
[2]: 4300763792
[0]: 4298171528
[1]: 4298171528
[2]: 4298171528
[3]: 4298171528
So now let us go step-by-step. You have x
which is 1
, and a single element list y
containing x
. Your first step is y * 4
which will get you a new list z
, which is basically [x, x, x, x]
, i.e. it creates a new list which will have 4 elements, which are references to the initial x
object. The net step is pretty similar. You basically do z * 3
, which is [[x, x, x, x]] * 3
and returns [[x, x, x, x], [x, x, x, x], [x, x, x, x]]
, for the same reason as for the first step.
Initially I was thinking, how is it possible to come up with these random numbers from your simple example. You really have to mention whatid
does before you throw this code at people.
– PascalVKooten
Jun 10 '15 at 14:56
@PascalvKooten thanks, done :)
– bagrat
Jun 10 '15 at 15:06
add a comment |
I guess everybody explain what is happening.
I suggest one way to solve it:
myList = [[1 for i in range(4)] for j in range(3)]
myList[0][0] = 5
print myList
And then you have:
[[5, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
add a comment |
Trying to explain it more descriptively,
Operation 1:
x = [[0, 0], [0, 0]]
print(type(x)) # <class 'list'>
print(x) # [[0, 0], [0, 0]]
x[0][0] = 1
print(x) # [[1, 0], [0, 0]]
Operation 2:
y = [[0] * 2] * 2
print(type(y)) # <class 'list'>
print(y) # [[0, 0], [0, 0]]
y[0][0] = 1
print(y) # [[1, 0], [1, 0]]
Noticed why doesn't modifying the first element of the first list didn't modify the second element of each list? That's because [0] * 2
really is a list of two numbers, and a reference to 0 cannot be modified.
If you want to create clone copies, try Operation 3:
import copy
y = [0] * 2
print(y) # [0, 0]
y = [y, copy.deepcopy(y)]
print(y) # [[0, 0], [0, 0]]
y[0][0] = 1
print(y) # [[1, 0], [0, 0]]
another interesting way to create clone copies, Operation 4:
import copy
y = [0] * 2
print(y) # [0, 0]
y = [copy.deepcopy(y) for num in range(1,5)]
print(y) # [[0, 0], [0, 0], [0, 0], [0, 0]]
y[0][0] = 5
print(y) # [[5, 0], [0, 0], [0, 0], [0, 0]]
add a comment |
By using the inbuilt list function you can do like this
a
out:[[1, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
#Displaying the list
a.remove(a[0])
out:[[1, 1, 1, 1], [1, 1, 1, 1]]
# Removed the first element of the list in which you want altered number
a.append([5,1,1,1])
out:[[1, 1, 1, 1], [1, 1, 1, 1], [5, 1, 1, 1]]
# append the element in the list but the appended element as you can see is appended in last but you want that in starting
a.reverse()
out:[[5, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
#So at last reverse the whole list to get the desired list
This works, but doesn't explain what's happening.
– Luigi Ballabio
Jul 15 '16 at 14:06
okay i am updating the code with comments
– anand tripathi
Jul 25 '16 at 9:06
1
Note, fourth step can be dropped if you make second step:a.insert(0,[5,1,1,1])
– U9-Forward
Oct 19 '18 at 5:29
yeah thanks @U9-Forward
– anand tripathi
Oct 20 '18 at 14:12
add a comment |
protected by styvane Apr 24 '16 at 15:14
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
12 Answers
12
active
oldest
votes
12 Answers
12
active
oldest
votes
active
oldest
votes
active
oldest
votes
When you write [x]*3
you get, essentially, the list [x, x, x]
. That is, a list with 3 references to the same x
. When you then modify this single x
it is visible via all three references to it.
To fix it, you need to make sure that you create a new list at each position. One way to do it is
[[1]*4 for _ in range(3)]
which will reevaluate [1]*4
each time instead of evaluating it once and making 3 references to 1 list.
You might wonder why *
can't make independent objects the way the list comprehension does. That's because the multiplication operator *
operates on objects, without seeing expressions. When you use *
to multiply [[1] * 4]
by 3, *
only sees the 1-element list [[1] * 4]
evaluates to, not the [[1] * 4
expression text. *
has no idea how to make copies of that element, no idea how to reevaluate [[1] * 4]
, and no idea you even want copies, and in general, there might not even be a way to copy the element.
The only option *
has is to make new references to the existing sublist instead of trying to make new sublists. Anything else would be inconsistent or require major redesigning of fundamental language design decisions.
In contrast, a list comprehension reevaluates the element expression on every iteration. [[1] * 4 for n in range(3)]
reevaluates [1] * 4
every time for the same reason [x**2 for x in range(3)]
reevaluates x**2
every time. Every evaluation of [1] * 4
generates a new list, so the list comprehension does what you wanted.
Incidentally, [1] * 4
also doesn't copy the elements of [1]
, but that doesn't matter, since integers are immutable. You can't do something like 1.value = 2
and turn a 1 into a 2.
2
Thanks for the explanation and the workaround. I am still surprised though that the outer list ends up holding three references to an anonymous inner list.
– Charles Anderson
Oct 27 '08 at 15:40
18
I am surprised that no body points out that, the answer here is misleading.[x]*3
store 3 references like[x, x, x]
is only right whenx
is mutable. This does't work for e.g.a=[4]*3
, where aftera[0]=5
,a=[5,4,4].
– Allanqunzi
May 22 '15 at 0:16
30
Technically, it's still correct.[4]*3
is essentially equivalent tox = 4; [x, x, x]
. It's true, though, that this will never cause any problem since4
is immutable. Also, your other example isn't really a different case.a = [x]*3; a[0] = 5
won't cause problems even ifx
is mutable, since you're not modifyingx
, only modifyinga
. I wouldn't describe my answer as misleading or incorrect - you just can't shoot yourself in the foot if you're dealing with immutable objects.
– CAdaker
May 22 '15 at 8:04
14
@Allanqunzi you are wrong. Dox = 1000; lst = [x]*2; lst[0] is lst[1]
->True
. Python does not distinguish between mutable and immutable objects here whatsoever.
– timgeb
Apr 17 '16 at 18:08
2
@ᴡʜᴀᴄᴋᴀᴍᴀᴅᴏᴏᴅʟᴇ3000import ctypes; ctypes.cast(id(1), ctypes.POINTER(ctypes.c_int))[6] = 2
, but prepare for weird behavior or a segfault.
– Zach Gates
Aug 4 '18 at 16:33
|
show 3 more comments
When you write [x]*3
you get, essentially, the list [x, x, x]
. That is, a list with 3 references to the same x
. When you then modify this single x
it is visible via all three references to it.
To fix it, you need to make sure that you create a new list at each position. One way to do it is
[[1]*4 for _ in range(3)]
which will reevaluate [1]*4
each time instead of evaluating it once and making 3 references to 1 list.
You might wonder why *
can't make independent objects the way the list comprehension does. That's because the multiplication operator *
operates on objects, without seeing expressions. When you use *
to multiply [[1] * 4]
by 3, *
only sees the 1-element list [[1] * 4]
evaluates to, not the [[1] * 4
expression text. *
has no idea how to make copies of that element, no idea how to reevaluate [[1] * 4]
, and no idea you even want copies, and in general, there might not even be a way to copy the element.
The only option *
has is to make new references to the existing sublist instead of trying to make new sublists. Anything else would be inconsistent or require major redesigning of fundamental language design decisions.
In contrast, a list comprehension reevaluates the element expression on every iteration. [[1] * 4 for n in range(3)]
reevaluates [1] * 4
every time for the same reason [x**2 for x in range(3)]
reevaluates x**2
every time. Every evaluation of [1] * 4
generates a new list, so the list comprehension does what you wanted.
Incidentally, [1] * 4
also doesn't copy the elements of [1]
, but that doesn't matter, since integers are immutable. You can't do something like 1.value = 2
and turn a 1 into a 2.
2
Thanks for the explanation and the workaround. I am still surprised though that the outer list ends up holding three references to an anonymous inner list.
– Charles Anderson
Oct 27 '08 at 15:40
18
I am surprised that no body points out that, the answer here is misleading.[x]*3
store 3 references like[x, x, x]
is only right whenx
is mutable. This does't work for e.g.a=[4]*3
, where aftera[0]=5
,a=[5,4,4].
– Allanqunzi
May 22 '15 at 0:16
30
Technically, it's still correct.[4]*3
is essentially equivalent tox = 4; [x, x, x]
. It's true, though, that this will never cause any problem since4
is immutable. Also, your other example isn't really a different case.a = [x]*3; a[0] = 5
won't cause problems even ifx
is mutable, since you're not modifyingx
, only modifyinga
. I wouldn't describe my answer as misleading or incorrect - you just can't shoot yourself in the foot if you're dealing with immutable objects.
– CAdaker
May 22 '15 at 8:04
14
@Allanqunzi you are wrong. Dox = 1000; lst = [x]*2; lst[0] is lst[1]
->True
. Python does not distinguish between mutable and immutable objects here whatsoever.
– timgeb
Apr 17 '16 at 18:08
2
@ᴡʜᴀᴄᴋᴀᴍᴀᴅᴏᴏᴅʟᴇ3000import ctypes; ctypes.cast(id(1), ctypes.POINTER(ctypes.c_int))[6] = 2
, but prepare for weird behavior or a segfault.
– Zach Gates
Aug 4 '18 at 16:33
|
show 3 more comments
When you write [x]*3
you get, essentially, the list [x, x, x]
. That is, a list with 3 references to the same x
. When you then modify this single x
it is visible via all three references to it.
To fix it, you need to make sure that you create a new list at each position. One way to do it is
[[1]*4 for _ in range(3)]
which will reevaluate [1]*4
each time instead of evaluating it once and making 3 references to 1 list.
You might wonder why *
can't make independent objects the way the list comprehension does. That's because the multiplication operator *
operates on objects, without seeing expressions. When you use *
to multiply [[1] * 4]
by 3, *
only sees the 1-element list [[1] * 4]
evaluates to, not the [[1] * 4
expression text. *
has no idea how to make copies of that element, no idea how to reevaluate [[1] * 4]
, and no idea you even want copies, and in general, there might not even be a way to copy the element.
The only option *
has is to make new references to the existing sublist instead of trying to make new sublists. Anything else would be inconsistent or require major redesigning of fundamental language design decisions.
In contrast, a list comprehension reevaluates the element expression on every iteration. [[1] * 4 for n in range(3)]
reevaluates [1] * 4
every time for the same reason [x**2 for x in range(3)]
reevaluates x**2
every time. Every evaluation of [1] * 4
generates a new list, so the list comprehension does what you wanted.
Incidentally, [1] * 4
also doesn't copy the elements of [1]
, but that doesn't matter, since integers are immutable. You can't do something like 1.value = 2
and turn a 1 into a 2.
When you write [x]*3
you get, essentially, the list [x, x, x]
. That is, a list with 3 references to the same x
. When you then modify this single x
it is visible via all three references to it.
To fix it, you need to make sure that you create a new list at each position. One way to do it is
[[1]*4 for _ in range(3)]
which will reevaluate [1]*4
each time instead of evaluating it once and making 3 references to 1 list.
You might wonder why *
can't make independent objects the way the list comprehension does. That's because the multiplication operator *
operates on objects, without seeing expressions. When you use *
to multiply [[1] * 4]
by 3, *
only sees the 1-element list [[1] * 4]
evaluates to, not the [[1] * 4
expression text. *
has no idea how to make copies of that element, no idea how to reevaluate [[1] * 4]
, and no idea you even want copies, and in general, there might not even be a way to copy the element.
The only option *
has is to make new references to the existing sublist instead of trying to make new sublists. Anything else would be inconsistent or require major redesigning of fundamental language design decisions.
In contrast, a list comprehension reevaluates the element expression on every iteration. [[1] * 4 for n in range(3)]
reevaluates [1] * 4
every time for the same reason [x**2 for x in range(3)]
reevaluates x**2
every time. Every evaluation of [1] * 4
generates a new list, so the list comprehension does what you wanted.
Incidentally, [1] * 4
also doesn't copy the elements of [1]
, but that doesn't matter, since integers are immutable. You can't do something like 1.value = 2
and turn a 1 into a 2.
edited yesterday


Jean-François Fabre♦
107k1058116
107k1058116
answered Oct 27 '08 at 15:03
CAdakerCAdaker
9,67522329
9,67522329
2
Thanks for the explanation and the workaround. I am still surprised though that the outer list ends up holding three references to an anonymous inner list.
– Charles Anderson
Oct 27 '08 at 15:40
18
I am surprised that no body points out that, the answer here is misleading.[x]*3
store 3 references like[x, x, x]
is only right whenx
is mutable. This does't work for e.g.a=[4]*3
, where aftera[0]=5
,a=[5,4,4].
– Allanqunzi
May 22 '15 at 0:16
30
Technically, it's still correct.[4]*3
is essentially equivalent tox = 4; [x, x, x]
. It's true, though, that this will never cause any problem since4
is immutable. Also, your other example isn't really a different case.a = [x]*3; a[0] = 5
won't cause problems even ifx
is mutable, since you're not modifyingx
, only modifyinga
. I wouldn't describe my answer as misleading or incorrect - you just can't shoot yourself in the foot if you're dealing with immutable objects.
– CAdaker
May 22 '15 at 8:04
14
@Allanqunzi you are wrong. Dox = 1000; lst = [x]*2; lst[0] is lst[1]
->True
. Python does not distinguish between mutable and immutable objects here whatsoever.
– timgeb
Apr 17 '16 at 18:08
2
@ᴡʜᴀᴄᴋᴀᴍᴀᴅᴏᴏᴅʟᴇ3000import ctypes; ctypes.cast(id(1), ctypes.POINTER(ctypes.c_int))[6] = 2
, but prepare for weird behavior or a segfault.
– Zach Gates
Aug 4 '18 at 16:33
|
show 3 more comments
2
Thanks for the explanation and the workaround. I am still surprised though that the outer list ends up holding three references to an anonymous inner list.
– Charles Anderson
Oct 27 '08 at 15:40
18
I am surprised that no body points out that, the answer here is misleading.[x]*3
store 3 references like[x, x, x]
is only right whenx
is mutable. This does't work for e.g.a=[4]*3
, where aftera[0]=5
,a=[5,4,4].
– Allanqunzi
May 22 '15 at 0:16
30
Technically, it's still correct.[4]*3
is essentially equivalent tox = 4; [x, x, x]
. It's true, though, that this will never cause any problem since4
is immutable. Also, your other example isn't really a different case.a = [x]*3; a[0] = 5
won't cause problems even ifx
is mutable, since you're not modifyingx
, only modifyinga
. I wouldn't describe my answer as misleading or incorrect - you just can't shoot yourself in the foot if you're dealing with immutable objects.
– CAdaker
May 22 '15 at 8:04
14
@Allanqunzi you are wrong. Dox = 1000; lst = [x]*2; lst[0] is lst[1]
->True
. Python does not distinguish between mutable and immutable objects here whatsoever.
– timgeb
Apr 17 '16 at 18:08
2
@ᴡʜᴀᴄᴋᴀᴍᴀᴅᴏᴏᴅʟᴇ3000import ctypes; ctypes.cast(id(1), ctypes.POINTER(ctypes.c_int))[6] = 2
, but prepare for weird behavior or a segfault.
– Zach Gates
Aug 4 '18 at 16:33
2
2
Thanks for the explanation and the workaround. I am still surprised though that the outer list ends up holding three references to an anonymous inner list.
– Charles Anderson
Oct 27 '08 at 15:40
Thanks for the explanation and the workaround. I am still surprised though that the outer list ends up holding three references to an anonymous inner list.
– Charles Anderson
Oct 27 '08 at 15:40
18
18
I am surprised that no body points out that, the answer here is misleading.
[x]*3
store 3 references like [x, x, x]
is only right when x
is mutable. This does't work for e.g. a=[4]*3
, where after a[0]=5
, a=[5,4,4].
– Allanqunzi
May 22 '15 at 0:16
I am surprised that no body points out that, the answer here is misleading.
[x]*3
store 3 references like [x, x, x]
is only right when x
is mutable. This does't work for e.g. a=[4]*3
, where after a[0]=5
, a=[5,4,4].
– Allanqunzi
May 22 '15 at 0:16
30
30
Technically, it's still correct.
[4]*3
is essentially equivalent to x = 4; [x, x, x]
. It's true, though, that this will never cause any problem since 4
is immutable. Also, your other example isn't really a different case. a = [x]*3; a[0] = 5
won't cause problems even if x
is mutable, since you're not modifying x
, only modifying a
. I wouldn't describe my answer as misleading or incorrect - you just can't shoot yourself in the foot if you're dealing with immutable objects.– CAdaker
May 22 '15 at 8:04
Technically, it's still correct.
[4]*3
is essentially equivalent to x = 4; [x, x, x]
. It's true, though, that this will never cause any problem since 4
is immutable. Also, your other example isn't really a different case. a = [x]*3; a[0] = 5
won't cause problems even if x
is mutable, since you're not modifying x
, only modifying a
. I wouldn't describe my answer as misleading or incorrect - you just can't shoot yourself in the foot if you're dealing with immutable objects.– CAdaker
May 22 '15 at 8:04
14
14
@Allanqunzi you are wrong. Do
x = 1000; lst = [x]*2; lst[0] is lst[1]
-> True
. Python does not distinguish between mutable and immutable objects here whatsoever.– timgeb
Apr 17 '16 at 18:08
@Allanqunzi you are wrong. Do
x = 1000; lst = [x]*2; lst[0] is lst[1]
-> True
. Python does not distinguish between mutable and immutable objects here whatsoever.– timgeb
Apr 17 '16 at 18:08
2
2
@ᴡʜᴀᴄᴋᴀᴍᴀᴅᴏᴏᴅʟᴇ3000
import ctypes; ctypes.cast(id(1), ctypes.POINTER(ctypes.c_int))[6] = 2
, but prepare for weird behavior or a segfault.– Zach Gates
Aug 4 '18 at 16:33
@ᴡʜᴀᴄᴋᴀᴍᴀᴅᴏᴏᴅʟᴇ3000
import ctypes; ctypes.cast(id(1), ctypes.POINTER(ctypes.c_int))[6] = 2
, but prepare for weird behavior or a segfault.– Zach Gates
Aug 4 '18 at 16:33
|
show 3 more comments
size = 3
matrix_surprise = [[0] * size] * size
matrix = [[0]*size for i in range(size)]
Live Python Tutor Visualize
4
I've put your image inline. For future reference, you really need to explain what you're linking to.
– Blckknght
Aug 27 '13 at 0:42
2
Great tool! Thanks for the reference
– Dennis
Feb 27 '16 at 15:43
So, why if we write matrix= [[x] * 2] doesn't make 2 elemnts for the same object like the example you describe, it seems to be the same concept, what am i missing?
– Ahmed Mohamed
Jul 1 '17 at 17:55
@AhmedMohamed Indeed it does make a list with two elements of the exact same object thatx
refers to. If you make a globally unique object withx = object()
and then makematrix = [[x] * 2]
these does come as true:matrix[0][0] is matrix[0][1]
– nadrimajstor
Jul 2 '17 at 13:13
@nadrimajstor so why the change in matrix[0] doesn't affect matrix[1] like the example above with 2d matrix.
– Ahmed Mohamed
Jul 2 '17 at 13:31
|
show 2 more comments
size = 3
matrix_surprise = [[0] * size] * size
matrix = [[0]*size for i in range(size)]
Live Python Tutor Visualize
4
I've put your image inline. For future reference, you really need to explain what you're linking to.
– Blckknght
Aug 27 '13 at 0:42
2
Great tool! Thanks for the reference
– Dennis
Feb 27 '16 at 15:43
So, why if we write matrix= [[x] * 2] doesn't make 2 elemnts for the same object like the example you describe, it seems to be the same concept, what am i missing?
– Ahmed Mohamed
Jul 1 '17 at 17:55
@AhmedMohamed Indeed it does make a list with two elements of the exact same object thatx
refers to. If you make a globally unique object withx = object()
and then makematrix = [[x] * 2]
these does come as true:matrix[0][0] is matrix[0][1]
– nadrimajstor
Jul 2 '17 at 13:13
@nadrimajstor so why the change in matrix[0] doesn't affect matrix[1] like the example above with 2d matrix.
– Ahmed Mohamed
Jul 2 '17 at 13:31
|
show 2 more comments
size = 3
matrix_surprise = [[0] * size] * size
matrix = [[0]*size for i in range(size)]
Live Python Tutor Visualize
size = 3
matrix_surprise = [[0] * size] * size
matrix = [[0]*size for i in range(size)]
Live Python Tutor Visualize
edited Dec 27 '14 at 12:44


Boann
37.5k1291123
37.5k1291123
answered Aug 26 '13 at 23:17
nadrimajstornadrimajstor
1,178189
1,178189
4
I've put your image inline. For future reference, you really need to explain what you're linking to.
– Blckknght
Aug 27 '13 at 0:42
2
Great tool! Thanks for the reference
– Dennis
Feb 27 '16 at 15:43
So, why if we write matrix= [[x] * 2] doesn't make 2 elemnts for the same object like the example you describe, it seems to be the same concept, what am i missing?
– Ahmed Mohamed
Jul 1 '17 at 17:55
@AhmedMohamed Indeed it does make a list with two elements of the exact same object thatx
refers to. If you make a globally unique object withx = object()
and then makematrix = [[x] * 2]
these does come as true:matrix[0][0] is matrix[0][1]
– nadrimajstor
Jul 2 '17 at 13:13
@nadrimajstor so why the change in matrix[0] doesn't affect matrix[1] like the example above with 2d matrix.
– Ahmed Mohamed
Jul 2 '17 at 13:31
|
show 2 more comments
4
I've put your image inline. For future reference, you really need to explain what you're linking to.
– Blckknght
Aug 27 '13 at 0:42
2
Great tool! Thanks for the reference
– Dennis
Feb 27 '16 at 15:43
So, why if we write matrix= [[x] * 2] doesn't make 2 elemnts for the same object like the example you describe, it seems to be the same concept, what am i missing?
– Ahmed Mohamed
Jul 1 '17 at 17:55
@AhmedMohamed Indeed it does make a list with two elements of the exact same object thatx
refers to. If you make a globally unique object withx = object()
and then makematrix = [[x] * 2]
these does come as true:matrix[0][0] is matrix[0][1]
– nadrimajstor
Jul 2 '17 at 13:13
@nadrimajstor so why the change in matrix[0] doesn't affect matrix[1] like the example above with 2d matrix.
– Ahmed Mohamed
Jul 2 '17 at 13:31
4
4
I've put your image inline. For future reference, you really need to explain what you're linking to.
– Blckknght
Aug 27 '13 at 0:42
I've put your image inline. For future reference, you really need to explain what you're linking to.
– Blckknght
Aug 27 '13 at 0:42
2
2
Great tool! Thanks for the reference
– Dennis
Feb 27 '16 at 15:43
Great tool! Thanks for the reference
– Dennis
Feb 27 '16 at 15:43
So, why if we write matrix= [[x] * 2] doesn't make 2 elemnts for the same object like the example you describe, it seems to be the same concept, what am i missing?
– Ahmed Mohamed
Jul 1 '17 at 17:55
So, why if we write matrix= [[x] * 2] doesn't make 2 elemnts for the same object like the example you describe, it seems to be the same concept, what am i missing?
– Ahmed Mohamed
Jul 1 '17 at 17:55
@AhmedMohamed Indeed it does make a list with two elements of the exact same object that
x
refers to. If you make a globally unique object with x = object()
and then make matrix = [[x] * 2]
these does come as true: matrix[0][0] is matrix[0][1]
– nadrimajstor
Jul 2 '17 at 13:13
@AhmedMohamed Indeed it does make a list with two elements of the exact same object that
x
refers to. If you make a globally unique object with x = object()
and then make matrix = [[x] * 2]
these does come as true: matrix[0][0] is matrix[0][1]
– nadrimajstor
Jul 2 '17 at 13:13
@nadrimajstor so why the change in matrix[0] doesn't affect matrix[1] like the example above with 2d matrix.
– Ahmed Mohamed
Jul 2 '17 at 13:31
@nadrimajstor so why the change in matrix[0] doesn't affect matrix[1] like the example above with 2d matrix.
– Ahmed Mohamed
Jul 2 '17 at 13:31
|
show 2 more comments
Actually, this is exactly what you would expect. Let's decompose what is happening here:
You write
lst = [[1] * 4] * 3
This is equivalent to:
lst1 = [1]*4
lst = [lst1]*3
This means lst
is a list with 3 elements all pointing to lst1
. This means the two following lines are equivalent:
lst[0][0] = 5
lst1[0] = 5
As lst[0]
is nothing but lst1
.
To obtain the desired behavior, you can use list comprehension:
lst = [ [1]*4 for n in xrange(3) ]
In this case, the expression is re-evaluated for each n, leading to a different list.
This is really clear for a beginner like me. Thank you!
– Petite Etincelle
Apr 29 '16 at 8:18
Just a small addition to the nice answer here: it's evident that you're dealing with same object if you doid(lst[0][0])
andid(lst[1][0])
or evenid(lst[0])
andid(lst[1])
– Sergiy Kolodyazhnyy
May 17 '17 at 7:08
add a comment |
Actually, this is exactly what you would expect. Let's decompose what is happening here:
You write
lst = [[1] * 4] * 3
This is equivalent to:
lst1 = [1]*4
lst = [lst1]*3
This means lst
is a list with 3 elements all pointing to lst1
. This means the two following lines are equivalent:
lst[0][0] = 5
lst1[0] = 5
As lst[0]
is nothing but lst1
.
To obtain the desired behavior, you can use list comprehension:
lst = [ [1]*4 for n in xrange(3) ]
In this case, the expression is re-evaluated for each n, leading to a different list.
This is really clear for a beginner like me. Thank you!
– Petite Etincelle
Apr 29 '16 at 8:18
Just a small addition to the nice answer here: it's evident that you're dealing with same object if you doid(lst[0][0])
andid(lst[1][0])
or evenid(lst[0])
andid(lst[1])
– Sergiy Kolodyazhnyy
May 17 '17 at 7:08
add a comment |
Actually, this is exactly what you would expect. Let's decompose what is happening here:
You write
lst = [[1] * 4] * 3
This is equivalent to:
lst1 = [1]*4
lst = [lst1]*3
This means lst
is a list with 3 elements all pointing to lst1
. This means the two following lines are equivalent:
lst[0][0] = 5
lst1[0] = 5
As lst[0]
is nothing but lst1
.
To obtain the desired behavior, you can use list comprehension:
lst = [ [1]*4 for n in xrange(3) ]
In this case, the expression is re-evaluated for each n, leading to a different list.
Actually, this is exactly what you would expect. Let's decompose what is happening here:
You write
lst = [[1] * 4] * 3
This is equivalent to:
lst1 = [1]*4
lst = [lst1]*3
This means lst
is a list with 3 elements all pointing to lst1
. This means the two following lines are equivalent:
lst[0][0] = 5
lst1[0] = 5
As lst[0]
is nothing but lst1
.
To obtain the desired behavior, you can use list comprehension:
lst = [ [1]*4 for n in xrange(3) ]
In this case, the expression is re-evaluated for each n, leading to a different list.
answered Oct 27 '08 at 15:07
PierreBdRPierreBdR
31.7k93757
31.7k93757
This is really clear for a beginner like me. Thank you!
– Petite Etincelle
Apr 29 '16 at 8:18
Just a small addition to the nice answer here: it's evident that you're dealing with same object if you doid(lst[0][0])
andid(lst[1][0])
or evenid(lst[0])
andid(lst[1])
– Sergiy Kolodyazhnyy
May 17 '17 at 7:08
add a comment |
This is really clear for a beginner like me. Thank you!
– Petite Etincelle
Apr 29 '16 at 8:18
Just a small addition to the nice answer here: it's evident that you're dealing with same object if you doid(lst[0][0])
andid(lst[1][0])
or evenid(lst[0])
andid(lst[1])
– Sergiy Kolodyazhnyy
May 17 '17 at 7:08
This is really clear for a beginner like me. Thank you!
– Petite Etincelle
Apr 29 '16 at 8:18
This is really clear for a beginner like me. Thank you!
– Petite Etincelle
Apr 29 '16 at 8:18
Just a small addition to the nice answer here: it's evident that you're dealing with same object if you do
id(lst[0][0])
and id(lst[1][0])
or even id(lst[0])
and id(lst[1])
– Sergiy Kolodyazhnyy
May 17 '17 at 7:08
Just a small addition to the nice answer here: it's evident that you're dealing with same object if you do
id(lst[0][0])
and id(lst[1][0])
or even id(lst[0])
and id(lst[1])
– Sergiy Kolodyazhnyy
May 17 '17 at 7:08
add a comment |
[[1] * 4] * 3
or even:
[[1, 1, 1, 1]] * 3
Creates a list that references the internal [1,1,1,1]
3 times - not three copies of the inner list, so any time you modify the list (in any position), you'll see the change three times.
It's the same as this example:
>>> inner = [1,1,1,1]
>>> outer = [inner]*3
>>> outer
[[1, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
>>> inner[0] = 5
>>> outer
[[5, 1, 1, 1], [5, 1, 1, 1], [5, 1, 1, 1]]
where it's probably a little less surprising.
2
You can use the "is" operator to discover this. ls[0] is ls[1] returns True.
– mipadi
Oct 27 '08 at 15:03
add a comment |
[[1] * 4] * 3
or even:
[[1, 1, 1, 1]] * 3
Creates a list that references the internal [1,1,1,1]
3 times - not three copies of the inner list, so any time you modify the list (in any position), you'll see the change three times.
It's the same as this example:
>>> inner = [1,1,1,1]
>>> outer = [inner]*3
>>> outer
[[1, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
>>> inner[0] = 5
>>> outer
[[5, 1, 1, 1], [5, 1, 1, 1], [5, 1, 1, 1]]
where it's probably a little less surprising.
2
You can use the "is" operator to discover this. ls[0] is ls[1] returns True.
– mipadi
Oct 27 '08 at 15:03
add a comment |
[[1] * 4] * 3
or even:
[[1, 1, 1, 1]] * 3
Creates a list that references the internal [1,1,1,1]
3 times - not three copies of the inner list, so any time you modify the list (in any position), you'll see the change three times.
It's the same as this example:
>>> inner = [1,1,1,1]
>>> outer = [inner]*3
>>> outer
[[1, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
>>> inner[0] = 5
>>> outer
[[5, 1, 1, 1], [5, 1, 1, 1], [5, 1, 1, 1]]
where it's probably a little less surprising.
[[1] * 4] * 3
or even:
[[1, 1, 1, 1]] * 3
Creates a list that references the internal [1,1,1,1]
3 times - not three copies of the inner list, so any time you modify the list (in any position), you'll see the change three times.
It's the same as this example:
>>> inner = [1,1,1,1]
>>> outer = [inner]*3
>>> outer
[[1, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
>>> inner[0] = 5
>>> outer
[[5, 1, 1, 1], [5, 1, 1, 1], [5, 1, 1, 1]]
where it's probably a little less surprising.
edited Jan 14 '17 at 7:54


Jim Fasarakis Hilliard
80k18163185
80k18163185
answered Oct 27 '08 at 15:02
Blair ConradBlair Conrad
158k24120105
158k24120105
2
You can use the "is" operator to discover this. ls[0] is ls[1] returns True.
– mipadi
Oct 27 '08 at 15:03
add a comment |
2
You can use the "is" operator to discover this. ls[0] is ls[1] returns True.
– mipadi
Oct 27 '08 at 15:03
2
2
You can use the "is" operator to discover this. ls[0] is ls[1] returns True.
– mipadi
Oct 27 '08 at 15:03
You can use the "is" operator to discover this. ls[0] is ls[1] returns True.
– mipadi
Oct 27 '08 at 15:03
add a comment |
Alongside the accepted answer that explained the problem correctly, within your list comprehension, if You are using python-2.x use xrange()
that returns a generator which is more efficient (range()
in python 3 does the same job) _
instead of the throwaway variable n
:
[[1]*4 for _ in xrange(3)] # and in python3 [[1]*4 for _ in range(3)]
Also, as a much more Pythonic way you can use itertools.repeat()
to create an iterator object of repeated elements :
>>> a=list(repeat(1,4))
[1, 1, 1, 1]
>>> a[0]=5
>>> a
[5, 1, 1, 1]
P.S. Using numpy, if you only want to create an array of ones or zeroes you can use np.ones
and np.zeros
and/or for other number use np.repeat()
:
In [1]: import numpy as np
In [2]:
In [2]: np.ones(4)
Out[2]: array([ 1., 1., 1., 1.])
In [3]: np.ones((4, 2))
Out[3]:
array([[ 1., 1.],
[ 1., 1.],
[ 1., 1.],
[ 1., 1.]])
In [4]: np.zeros((4, 2))
Out[4]:
array([[ 0., 0.],
[ 0., 0.],
[ 0., 0.],
[ 0., 0.]])
In [5]: np.repeat([7], 10)
Out[5]: array([7, 7, 7, 7, 7, 7, 7, 7, 7, 7])
add a comment |
Alongside the accepted answer that explained the problem correctly, within your list comprehension, if You are using python-2.x use xrange()
that returns a generator which is more efficient (range()
in python 3 does the same job) _
instead of the throwaway variable n
:
[[1]*4 for _ in xrange(3)] # and in python3 [[1]*4 for _ in range(3)]
Also, as a much more Pythonic way you can use itertools.repeat()
to create an iterator object of repeated elements :
>>> a=list(repeat(1,4))
[1, 1, 1, 1]
>>> a[0]=5
>>> a
[5, 1, 1, 1]
P.S. Using numpy, if you only want to create an array of ones or zeroes you can use np.ones
and np.zeros
and/or for other number use np.repeat()
:
In [1]: import numpy as np
In [2]:
In [2]: np.ones(4)
Out[2]: array([ 1., 1., 1., 1.])
In [3]: np.ones((4, 2))
Out[3]:
array([[ 1., 1.],
[ 1., 1.],
[ 1., 1.],
[ 1., 1.]])
In [4]: np.zeros((4, 2))
Out[4]:
array([[ 0., 0.],
[ 0., 0.],
[ 0., 0.],
[ 0., 0.]])
In [5]: np.repeat([7], 10)
Out[5]: array([7, 7, 7, 7, 7, 7, 7, 7, 7, 7])
add a comment |
Alongside the accepted answer that explained the problem correctly, within your list comprehension, if You are using python-2.x use xrange()
that returns a generator which is more efficient (range()
in python 3 does the same job) _
instead of the throwaway variable n
:
[[1]*4 for _ in xrange(3)] # and in python3 [[1]*4 for _ in range(3)]
Also, as a much more Pythonic way you can use itertools.repeat()
to create an iterator object of repeated elements :
>>> a=list(repeat(1,4))
[1, 1, 1, 1]
>>> a[0]=5
>>> a
[5, 1, 1, 1]
P.S. Using numpy, if you only want to create an array of ones or zeroes you can use np.ones
and np.zeros
and/or for other number use np.repeat()
:
In [1]: import numpy as np
In [2]:
In [2]: np.ones(4)
Out[2]: array([ 1., 1., 1., 1.])
In [3]: np.ones((4, 2))
Out[3]:
array([[ 1., 1.],
[ 1., 1.],
[ 1., 1.],
[ 1., 1.]])
In [4]: np.zeros((4, 2))
Out[4]:
array([[ 0., 0.],
[ 0., 0.],
[ 0., 0.],
[ 0., 0.]])
In [5]: np.repeat([7], 10)
Out[5]: array([7, 7, 7, 7, 7, 7, 7, 7, 7, 7])
Alongside the accepted answer that explained the problem correctly, within your list comprehension, if You are using python-2.x use xrange()
that returns a generator which is more efficient (range()
in python 3 does the same job) _
instead of the throwaway variable n
:
[[1]*4 for _ in xrange(3)] # and in python3 [[1]*4 for _ in range(3)]
Also, as a much more Pythonic way you can use itertools.repeat()
to create an iterator object of repeated elements :
>>> a=list(repeat(1,4))
[1, 1, 1, 1]
>>> a[0]=5
>>> a
[5, 1, 1, 1]
P.S. Using numpy, if you only want to create an array of ones or zeroes you can use np.ones
and np.zeros
and/or for other number use np.repeat()
:
In [1]: import numpy as np
In [2]:
In [2]: np.ones(4)
Out[2]: array([ 1., 1., 1., 1.])
In [3]: np.ones((4, 2))
Out[3]:
array([[ 1., 1.],
[ 1., 1.],
[ 1., 1.],
[ 1., 1.]])
In [4]: np.zeros((4, 2))
Out[4]:
array([[ 0., 0.],
[ 0., 0.],
[ 0., 0.],
[ 0., 0.]])
In [5]: np.repeat([7], 10)
Out[5]: array([7, 7, 7, 7, 7, 7, 7, 7, 7, 7])
edited Sep 15 '17 at 15:19
answered Jun 17 '15 at 17:08


KasrâmvdKasrâmvd
79.9k1092132
79.9k1092132
add a comment |
add a comment |
In simple words this is happening because in python everything works by reference, so when you create a list of list that way you basically end up with such problems.
To solve your issue you can do either one of them:
1. Use numpy array documentation for numpy.empty
2. Append the list as you get to a list.
3. You can also use dictionary if you want
add a comment |
In simple words this is happening because in python everything works by reference, so when you create a list of list that way you basically end up with such problems.
To solve your issue you can do either one of them:
1. Use numpy array documentation for numpy.empty
2. Append the list as you get to a list.
3. You can also use dictionary if you want
add a comment |
In simple words this is happening because in python everything works by reference, so when you create a list of list that way you basically end up with such problems.
To solve your issue you can do either one of them:
1. Use numpy array documentation for numpy.empty
2. Append the list as you get to a list.
3. You can also use dictionary if you want
In simple words this is happening because in python everything works by reference, so when you create a list of list that way you basically end up with such problems.
To solve your issue you can do either one of them:
1. Use numpy array documentation for numpy.empty
2. Append the list as you get to a list.
3. You can also use dictionary if you want
answered Jun 14 '16 at 6:36
Neeraj KomuravalliNeeraj Komuravalli
1418
1418
add a comment |
add a comment |
Python containers contain references to other objects. See this example:
>>> a =
>>> b = [a]
>>> b
[]
>>> a.append(1)
>>> b
[[1]]
In this b
is a list that contains one item that is a reference to list a
. The list a
is mutable.
The multiplication of a list by an integer is equivalent to adding the list to itself multiple times (see common sequence operations). So continuing with the example:
>>> c = b + b
>>> c
[[1], [1]]
>>>
>>> a[0] = 2
>>> c
[[2], [2]]
We can see that the list c
now contains two references to list a
which is equivalent to c = b * 2
.
Python FAQ also contains explanation of this behavior: How do I create a multidimensional list?
add a comment |
Python containers contain references to other objects. See this example:
>>> a =
>>> b = [a]
>>> b
[]
>>> a.append(1)
>>> b
[[1]]
In this b
is a list that contains one item that is a reference to list a
. The list a
is mutable.
The multiplication of a list by an integer is equivalent to adding the list to itself multiple times (see common sequence operations). So continuing with the example:
>>> c = b + b
>>> c
[[1], [1]]
>>>
>>> a[0] = 2
>>> c
[[2], [2]]
We can see that the list c
now contains two references to list a
which is equivalent to c = b * 2
.
Python FAQ also contains explanation of this behavior: How do I create a multidimensional list?
add a comment |
Python containers contain references to other objects. See this example:
>>> a =
>>> b = [a]
>>> b
[]
>>> a.append(1)
>>> b
[[1]]
In this b
is a list that contains one item that is a reference to list a
. The list a
is mutable.
The multiplication of a list by an integer is equivalent to adding the list to itself multiple times (see common sequence operations). So continuing with the example:
>>> c = b + b
>>> c
[[1], [1]]
>>>
>>> a[0] = 2
>>> c
[[2], [2]]
We can see that the list c
now contains two references to list a
which is equivalent to c = b * 2
.
Python FAQ also contains explanation of this behavior: How do I create a multidimensional list?
Python containers contain references to other objects. See this example:
>>> a =
>>> b = [a]
>>> b
[]
>>> a.append(1)
>>> b
[[1]]
In this b
is a list that contains one item that is a reference to list a
. The list a
is mutable.
The multiplication of a list by an integer is equivalent to adding the list to itself multiple times (see common sequence operations). So continuing with the example:
>>> c = b + b
>>> c
[[1], [1]]
>>>
>>> a[0] = 2
>>> c
[[2], [2]]
We can see that the list c
now contains two references to list a
which is equivalent to c = b * 2
.
Python FAQ also contains explanation of this behavior: How do I create a multidimensional list?
answered Apr 6 '16 at 13:40
Zbyněk WinklerZbyněk Winkler
38238
38238
add a comment |
add a comment |
myList = [[1]*4] * 3
creates one list object [1,1,1,1]
in memory and copies its reference 3 times over. This is equivalent to obj = [1,1,1,1]; myList = [obj]*3
. Any modification to obj
will be reflected at three places, wherever obj
is referenced in the list.
The right statement would be:
myList = [[1]*4 for _ in range(3)]
or
myList = [[1 for __ in range(4)] for _ in range(3)]
Important thing to note here is that *
operator is mostly used to create a list of literals. Since 1
is a literal, hence obj =[1]*4
will create [1,1,1,1]
where each 1
is atomic and not a reference of 1
repeated 4 times. This means if we do obj[2]=42
, then obj
will become [1,1,42,1]
not as some may assume.[42,42,42,42]
2
It's not about literals.obj[2] = 42
replaces the reference at index2
, as opposed to mutating the object referenced by that index, which is whatmyList[2][0] = ...
does (myList[2]
is a list, and the assigment alters the reference at index 0 in tha list). Of course, integers are not mutable, but plenty of object types are. And note that the[....]
list display notation is also a form of literal syntax! Don't confuse compound (such as lists) and scalar objects (such as integers), with mutable vs. immutable objects.
– Martijn Pieters♦
Jul 25 '18 at 15:52
add a comment |
myList = [[1]*4] * 3
creates one list object [1,1,1,1]
in memory and copies its reference 3 times over. This is equivalent to obj = [1,1,1,1]; myList = [obj]*3
. Any modification to obj
will be reflected at three places, wherever obj
is referenced in the list.
The right statement would be:
myList = [[1]*4 for _ in range(3)]
or
myList = [[1 for __ in range(4)] for _ in range(3)]
Important thing to note here is that *
operator is mostly used to create a list of literals. Since 1
is a literal, hence obj =[1]*4
will create [1,1,1,1]
where each 1
is atomic and not a reference of 1
repeated 4 times. This means if we do obj[2]=42
, then obj
will become [1,1,42,1]
not as some may assume.[42,42,42,42]
2
It's not about literals.obj[2] = 42
replaces the reference at index2
, as opposed to mutating the object referenced by that index, which is whatmyList[2][0] = ...
does (myList[2]
is a list, and the assigment alters the reference at index 0 in tha list). Of course, integers are not mutable, but plenty of object types are. And note that the[....]
list display notation is also a form of literal syntax! Don't confuse compound (such as lists) and scalar objects (such as integers), with mutable vs. immutable objects.
– Martijn Pieters♦
Jul 25 '18 at 15:52
add a comment |
myList = [[1]*4] * 3
creates one list object [1,1,1,1]
in memory and copies its reference 3 times over. This is equivalent to obj = [1,1,1,1]; myList = [obj]*3
. Any modification to obj
will be reflected at three places, wherever obj
is referenced in the list.
The right statement would be:
myList = [[1]*4 for _ in range(3)]
or
myList = [[1 for __ in range(4)] for _ in range(3)]
Important thing to note here is that *
operator is mostly used to create a list of literals. Since 1
is a literal, hence obj =[1]*4
will create [1,1,1,1]
where each 1
is atomic and not a reference of 1
repeated 4 times. This means if we do obj[2]=42
, then obj
will become [1,1,42,1]
not as some may assume.[42,42,42,42]
myList = [[1]*4] * 3
creates one list object [1,1,1,1]
in memory and copies its reference 3 times over. This is equivalent to obj = [1,1,1,1]; myList = [obj]*3
. Any modification to obj
will be reflected at three places, wherever obj
is referenced in the list.
The right statement would be:
myList = [[1]*4 for _ in range(3)]
or
myList = [[1 for __ in range(4)] for _ in range(3)]
Important thing to note here is that *
operator is mostly used to create a list of literals. Since 1
is a literal, hence obj =[1]*4
will create [1,1,1,1]
where each 1
is atomic and not a reference of 1
repeated 4 times. This means if we do obj[2]=42
, then obj
will become [1,1,42,1]
not as some may assume.[42,42,42,42]
edited Apr 6 '17 at 5:48
answered Apr 6 '17 at 5:36
jerrymousejerrymouse
8,847105068
8,847105068
2
It's not about literals.obj[2] = 42
replaces the reference at index2
, as opposed to mutating the object referenced by that index, which is whatmyList[2][0] = ...
does (myList[2]
is a list, and the assigment alters the reference at index 0 in tha list). Of course, integers are not mutable, but plenty of object types are. And note that the[....]
list display notation is also a form of literal syntax! Don't confuse compound (such as lists) and scalar objects (such as integers), with mutable vs. immutable objects.
– Martijn Pieters♦
Jul 25 '18 at 15:52
add a comment |
2
It's not about literals.obj[2] = 42
replaces the reference at index2
, as opposed to mutating the object referenced by that index, which is whatmyList[2][0] = ...
does (myList[2]
is a list, and the assigment alters the reference at index 0 in tha list). Of course, integers are not mutable, but plenty of object types are. And note that the[....]
list display notation is also a form of literal syntax! Don't confuse compound (such as lists) and scalar objects (such as integers), with mutable vs. immutable objects.
– Martijn Pieters♦
Jul 25 '18 at 15:52
2
2
It's not about literals.
obj[2] = 42
replaces the reference at index 2
, as opposed to mutating the object referenced by that index, which is what myList[2][0] = ...
does (myList[2]
is a list, and the assigment alters the reference at index 0 in tha list). Of course, integers are not mutable, but plenty of object types are. And note that the [....]
list display notation is also a form of literal syntax! Don't confuse compound (such as lists) and scalar objects (such as integers), with mutable vs. immutable objects.– Martijn Pieters♦
Jul 25 '18 at 15:52
It's not about literals.
obj[2] = 42
replaces the reference at index 2
, as opposed to mutating the object referenced by that index, which is what myList[2][0] = ...
does (myList[2]
is a list, and the assigment alters the reference at index 0 in tha list). Of course, integers are not mutable, but plenty of object types are. And note that the [....]
list display notation is also a form of literal syntax! Don't confuse compound (such as lists) and scalar objects (such as integers), with mutable vs. immutable objects.– Martijn Pieters♦
Jul 25 '18 at 15:52
add a comment |
Let us rewrite your code in the following way:
x = 1
y = [x]
z = y * 4
myList = [z] * 3
Then having this, run the following code to make everything more clear. What the code does is basically print the id
s of the obtained objects, which
Return the “identity” of an object
and will help us identify them and analyse what happens:
print("myList:")
for i, subList in enumerate(myList):
print("t[{}]: {}".format(i, id(subList)))
for j, elem in enumerate(subList):
print("tt[{}]: {}".format(j, id(elem)))
And you will get the following output:
x: 1
y: [1]
z: [1, 1, 1, 1]
myList:
[0]: 4300763792
[0]: 4298171528
[1]: 4298171528
[2]: 4298171528
[3]: 4298171528
[1]: 4300763792
[0]: 4298171528
[1]: 4298171528
[2]: 4298171528
[3]: 4298171528
[2]: 4300763792
[0]: 4298171528
[1]: 4298171528
[2]: 4298171528
[3]: 4298171528
So now let us go step-by-step. You have x
which is 1
, and a single element list y
containing x
. Your first step is y * 4
which will get you a new list z
, which is basically [x, x, x, x]
, i.e. it creates a new list which will have 4 elements, which are references to the initial x
object. The net step is pretty similar. You basically do z * 3
, which is [[x, x, x, x]] * 3
and returns [[x, x, x, x], [x, x, x, x], [x, x, x, x]]
, for the same reason as for the first step.
Initially I was thinking, how is it possible to come up with these random numbers from your simple example. You really have to mention whatid
does before you throw this code at people.
– PascalVKooten
Jun 10 '15 at 14:56
@PascalvKooten thanks, done :)
– bagrat
Jun 10 '15 at 15:06
add a comment |
Let us rewrite your code in the following way:
x = 1
y = [x]
z = y * 4
myList = [z] * 3
Then having this, run the following code to make everything more clear. What the code does is basically print the id
s of the obtained objects, which
Return the “identity” of an object
and will help us identify them and analyse what happens:
print("myList:")
for i, subList in enumerate(myList):
print("t[{}]: {}".format(i, id(subList)))
for j, elem in enumerate(subList):
print("tt[{}]: {}".format(j, id(elem)))
And you will get the following output:
x: 1
y: [1]
z: [1, 1, 1, 1]
myList:
[0]: 4300763792
[0]: 4298171528
[1]: 4298171528
[2]: 4298171528
[3]: 4298171528
[1]: 4300763792
[0]: 4298171528
[1]: 4298171528
[2]: 4298171528
[3]: 4298171528
[2]: 4300763792
[0]: 4298171528
[1]: 4298171528
[2]: 4298171528
[3]: 4298171528
So now let us go step-by-step. You have x
which is 1
, and a single element list y
containing x
. Your first step is y * 4
which will get you a new list z
, which is basically [x, x, x, x]
, i.e. it creates a new list which will have 4 elements, which are references to the initial x
object. The net step is pretty similar. You basically do z * 3
, which is [[x, x, x, x]] * 3
and returns [[x, x, x, x], [x, x, x, x], [x, x, x, x]]
, for the same reason as for the first step.
Initially I was thinking, how is it possible to come up with these random numbers from your simple example. You really have to mention whatid
does before you throw this code at people.
– PascalVKooten
Jun 10 '15 at 14:56
@PascalvKooten thanks, done :)
– bagrat
Jun 10 '15 at 15:06
add a comment |
Let us rewrite your code in the following way:
x = 1
y = [x]
z = y * 4
myList = [z] * 3
Then having this, run the following code to make everything more clear. What the code does is basically print the id
s of the obtained objects, which
Return the “identity” of an object
and will help us identify them and analyse what happens:
print("myList:")
for i, subList in enumerate(myList):
print("t[{}]: {}".format(i, id(subList)))
for j, elem in enumerate(subList):
print("tt[{}]: {}".format(j, id(elem)))
And you will get the following output:
x: 1
y: [1]
z: [1, 1, 1, 1]
myList:
[0]: 4300763792
[0]: 4298171528
[1]: 4298171528
[2]: 4298171528
[3]: 4298171528
[1]: 4300763792
[0]: 4298171528
[1]: 4298171528
[2]: 4298171528
[3]: 4298171528
[2]: 4300763792
[0]: 4298171528
[1]: 4298171528
[2]: 4298171528
[3]: 4298171528
So now let us go step-by-step. You have x
which is 1
, and a single element list y
containing x
. Your first step is y * 4
which will get you a new list z
, which is basically [x, x, x, x]
, i.e. it creates a new list which will have 4 elements, which are references to the initial x
object. The net step is pretty similar. You basically do z * 3
, which is [[x, x, x, x]] * 3
and returns [[x, x, x, x], [x, x, x, x], [x, x, x, x]]
, for the same reason as for the first step.
Let us rewrite your code in the following way:
x = 1
y = [x]
z = y * 4
myList = [z] * 3
Then having this, run the following code to make everything more clear. What the code does is basically print the id
s of the obtained objects, which
Return the “identity” of an object
and will help us identify them and analyse what happens:
print("myList:")
for i, subList in enumerate(myList):
print("t[{}]: {}".format(i, id(subList)))
for j, elem in enumerate(subList):
print("tt[{}]: {}".format(j, id(elem)))
And you will get the following output:
x: 1
y: [1]
z: [1, 1, 1, 1]
myList:
[0]: 4300763792
[0]: 4298171528
[1]: 4298171528
[2]: 4298171528
[3]: 4298171528
[1]: 4300763792
[0]: 4298171528
[1]: 4298171528
[2]: 4298171528
[3]: 4298171528
[2]: 4300763792
[0]: 4298171528
[1]: 4298171528
[2]: 4298171528
[3]: 4298171528
So now let us go step-by-step. You have x
which is 1
, and a single element list y
containing x
. Your first step is y * 4
which will get you a new list z
, which is basically [x, x, x, x]
, i.e. it creates a new list which will have 4 elements, which are references to the initial x
object. The net step is pretty similar. You basically do z * 3
, which is [[x, x, x, x]] * 3
and returns [[x, x, x, x], [x, x, x, x], [x, x, x, x]]
, for the same reason as for the first step.
edited Jun 10 '15 at 15:06
answered Jun 10 '15 at 14:38


bagratbagrat
4,67941745
4,67941745
Initially I was thinking, how is it possible to come up with these random numbers from your simple example. You really have to mention whatid
does before you throw this code at people.
– PascalVKooten
Jun 10 '15 at 14:56
@PascalvKooten thanks, done :)
– bagrat
Jun 10 '15 at 15:06
add a comment |
Initially I was thinking, how is it possible to come up with these random numbers from your simple example. You really have to mention whatid
does before you throw this code at people.
– PascalVKooten
Jun 10 '15 at 14:56
@PascalvKooten thanks, done :)
– bagrat
Jun 10 '15 at 15:06
Initially I was thinking, how is it possible to come up with these random numbers from your simple example. You really have to mention what
id
does before you throw this code at people.– PascalVKooten
Jun 10 '15 at 14:56
Initially I was thinking, how is it possible to come up with these random numbers from your simple example. You really have to mention what
id
does before you throw this code at people.– PascalVKooten
Jun 10 '15 at 14:56
@PascalvKooten thanks, done :)
– bagrat
Jun 10 '15 at 15:06
@PascalvKooten thanks, done :)
– bagrat
Jun 10 '15 at 15:06
add a comment |
I guess everybody explain what is happening.
I suggest one way to solve it:
myList = [[1 for i in range(4)] for j in range(3)]
myList[0][0] = 5
print myList
And then you have:
[[5, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
add a comment |
I guess everybody explain what is happening.
I suggest one way to solve it:
myList = [[1 for i in range(4)] for j in range(3)]
myList[0][0] = 5
print myList
And then you have:
[[5, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
add a comment |
I guess everybody explain what is happening.
I suggest one way to solve it:
myList = [[1 for i in range(4)] for j in range(3)]
myList[0][0] = 5
print myList
And then you have:
[[5, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
I guess everybody explain what is happening.
I suggest one way to solve it:
myList = [[1 for i in range(4)] for j in range(3)]
myList[0][0] = 5
print myList
And then you have:
[[5, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
answered Apr 24 '16 at 13:31


awulllawulll
1018
1018
add a comment |
add a comment |
Trying to explain it more descriptively,
Operation 1:
x = [[0, 0], [0, 0]]
print(type(x)) # <class 'list'>
print(x) # [[0, 0], [0, 0]]
x[0][0] = 1
print(x) # [[1, 0], [0, 0]]
Operation 2:
y = [[0] * 2] * 2
print(type(y)) # <class 'list'>
print(y) # [[0, 0], [0, 0]]
y[0][0] = 1
print(y) # [[1, 0], [1, 0]]
Noticed why doesn't modifying the first element of the first list didn't modify the second element of each list? That's because [0] * 2
really is a list of two numbers, and a reference to 0 cannot be modified.
If you want to create clone copies, try Operation 3:
import copy
y = [0] * 2
print(y) # [0, 0]
y = [y, copy.deepcopy(y)]
print(y) # [[0, 0], [0, 0]]
y[0][0] = 1
print(y) # [[1, 0], [0, 0]]
another interesting way to create clone copies, Operation 4:
import copy
y = [0] * 2
print(y) # [0, 0]
y = [copy.deepcopy(y) for num in range(1,5)]
print(y) # [[0, 0], [0, 0], [0, 0], [0, 0]]
y[0][0] = 5
print(y) # [[5, 0], [0, 0], [0, 0], [0, 0]]
add a comment |
Trying to explain it more descriptively,
Operation 1:
x = [[0, 0], [0, 0]]
print(type(x)) # <class 'list'>
print(x) # [[0, 0], [0, 0]]
x[0][0] = 1
print(x) # [[1, 0], [0, 0]]
Operation 2:
y = [[0] * 2] * 2
print(type(y)) # <class 'list'>
print(y) # [[0, 0], [0, 0]]
y[0][0] = 1
print(y) # [[1, 0], [1, 0]]
Noticed why doesn't modifying the first element of the first list didn't modify the second element of each list? That's because [0] * 2
really is a list of two numbers, and a reference to 0 cannot be modified.
If you want to create clone copies, try Operation 3:
import copy
y = [0] * 2
print(y) # [0, 0]
y = [y, copy.deepcopy(y)]
print(y) # [[0, 0], [0, 0]]
y[0][0] = 1
print(y) # [[1, 0], [0, 0]]
another interesting way to create clone copies, Operation 4:
import copy
y = [0] * 2
print(y) # [0, 0]
y = [copy.deepcopy(y) for num in range(1,5)]
print(y) # [[0, 0], [0, 0], [0, 0], [0, 0]]
y[0][0] = 5
print(y) # [[5, 0], [0, 0], [0, 0], [0, 0]]
add a comment |
Trying to explain it more descriptively,
Operation 1:
x = [[0, 0], [0, 0]]
print(type(x)) # <class 'list'>
print(x) # [[0, 0], [0, 0]]
x[0][0] = 1
print(x) # [[1, 0], [0, 0]]
Operation 2:
y = [[0] * 2] * 2
print(type(y)) # <class 'list'>
print(y) # [[0, 0], [0, 0]]
y[0][0] = 1
print(y) # [[1, 0], [1, 0]]
Noticed why doesn't modifying the first element of the first list didn't modify the second element of each list? That's because [0] * 2
really is a list of two numbers, and a reference to 0 cannot be modified.
If you want to create clone copies, try Operation 3:
import copy
y = [0] * 2
print(y) # [0, 0]
y = [y, copy.deepcopy(y)]
print(y) # [[0, 0], [0, 0]]
y[0][0] = 1
print(y) # [[1, 0], [0, 0]]
another interesting way to create clone copies, Operation 4:
import copy
y = [0] * 2
print(y) # [0, 0]
y = [copy.deepcopy(y) for num in range(1,5)]
print(y) # [[0, 0], [0, 0], [0, 0], [0, 0]]
y[0][0] = 5
print(y) # [[5, 0], [0, 0], [0, 0], [0, 0]]
Trying to explain it more descriptively,
Operation 1:
x = [[0, 0], [0, 0]]
print(type(x)) # <class 'list'>
print(x) # [[0, 0], [0, 0]]
x[0][0] = 1
print(x) # [[1, 0], [0, 0]]
Operation 2:
y = [[0] * 2] * 2
print(type(y)) # <class 'list'>
print(y) # [[0, 0], [0, 0]]
y[0][0] = 1
print(y) # [[1, 0], [1, 0]]
Noticed why doesn't modifying the first element of the first list didn't modify the second element of each list? That's because [0] * 2
really is a list of two numbers, and a reference to 0 cannot be modified.
If you want to create clone copies, try Operation 3:
import copy
y = [0] * 2
print(y) # [0, 0]
y = [y, copy.deepcopy(y)]
print(y) # [[0, 0], [0, 0]]
y[0][0] = 1
print(y) # [[1, 0], [0, 0]]
another interesting way to create clone copies, Operation 4:
import copy
y = [0] * 2
print(y) # [0, 0]
y = [copy.deepcopy(y) for num in range(1,5)]
print(y) # [[0, 0], [0, 0], [0, 0], [0, 0]]
y[0][0] = 5
print(y) # [[5, 0], [0, 0], [0, 0], [0, 0]]
edited Aug 10 '16 at 7:29
answered Aug 10 '16 at 7:09


Adil AbbasiAdil Abbasi
2,29913029
2,29913029
add a comment |
add a comment |
By using the inbuilt list function you can do like this
a
out:[[1, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
#Displaying the list
a.remove(a[0])
out:[[1, 1, 1, 1], [1, 1, 1, 1]]
# Removed the first element of the list in which you want altered number
a.append([5,1,1,1])
out:[[1, 1, 1, 1], [1, 1, 1, 1], [5, 1, 1, 1]]
# append the element in the list but the appended element as you can see is appended in last but you want that in starting
a.reverse()
out:[[5, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
#So at last reverse the whole list to get the desired list
This works, but doesn't explain what's happening.
– Luigi Ballabio
Jul 15 '16 at 14:06
okay i am updating the code with comments
– anand tripathi
Jul 25 '16 at 9:06
1
Note, fourth step can be dropped if you make second step:a.insert(0,[5,1,1,1])
– U9-Forward
Oct 19 '18 at 5:29
yeah thanks @U9-Forward
– anand tripathi
Oct 20 '18 at 14:12
add a comment |
By using the inbuilt list function you can do like this
a
out:[[1, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
#Displaying the list
a.remove(a[0])
out:[[1, 1, 1, 1], [1, 1, 1, 1]]
# Removed the first element of the list in which you want altered number
a.append([5,1,1,1])
out:[[1, 1, 1, 1], [1, 1, 1, 1], [5, 1, 1, 1]]
# append the element in the list but the appended element as you can see is appended in last but you want that in starting
a.reverse()
out:[[5, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
#So at last reverse the whole list to get the desired list
This works, but doesn't explain what's happening.
– Luigi Ballabio
Jul 15 '16 at 14:06
okay i am updating the code with comments
– anand tripathi
Jul 25 '16 at 9:06
1
Note, fourth step can be dropped if you make second step:a.insert(0,[5,1,1,1])
– U9-Forward
Oct 19 '18 at 5:29
yeah thanks @U9-Forward
– anand tripathi
Oct 20 '18 at 14:12
add a comment |
By using the inbuilt list function you can do like this
a
out:[[1, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
#Displaying the list
a.remove(a[0])
out:[[1, 1, 1, 1], [1, 1, 1, 1]]
# Removed the first element of the list in which you want altered number
a.append([5,1,1,1])
out:[[1, 1, 1, 1], [1, 1, 1, 1], [5, 1, 1, 1]]
# append the element in the list but the appended element as you can see is appended in last but you want that in starting
a.reverse()
out:[[5, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
#So at last reverse the whole list to get the desired list
By using the inbuilt list function you can do like this
a
out:[[1, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
#Displaying the list
a.remove(a[0])
out:[[1, 1, 1, 1], [1, 1, 1, 1]]
# Removed the first element of the list in which you want altered number
a.append([5,1,1,1])
out:[[1, 1, 1, 1], [1, 1, 1, 1], [5, 1, 1, 1]]
# append the element in the list but the appended element as you can see is appended in last but you want that in starting
a.reverse()
out:[[5, 1, 1, 1], [1, 1, 1, 1], [1, 1, 1, 1]]
#So at last reverse the whole list to get the desired list
edited Jul 25 '16 at 9:09
answered Jul 15 '16 at 13:48


anand tripathianand tripathi
3,48811528
3,48811528
This works, but doesn't explain what's happening.
– Luigi Ballabio
Jul 15 '16 at 14:06
okay i am updating the code with comments
– anand tripathi
Jul 25 '16 at 9:06
1
Note, fourth step can be dropped if you make second step:a.insert(0,[5,1,1,1])
– U9-Forward
Oct 19 '18 at 5:29
yeah thanks @U9-Forward
– anand tripathi
Oct 20 '18 at 14:12
add a comment |
This works, but doesn't explain what's happening.
– Luigi Ballabio
Jul 15 '16 at 14:06
okay i am updating the code with comments
– anand tripathi
Jul 25 '16 at 9:06
1
Note, fourth step can be dropped if you make second step:a.insert(0,[5,1,1,1])
– U9-Forward
Oct 19 '18 at 5:29
yeah thanks @U9-Forward
– anand tripathi
Oct 20 '18 at 14:12
This works, but doesn't explain what's happening.
– Luigi Ballabio
Jul 15 '16 at 14:06
This works, but doesn't explain what's happening.
– Luigi Ballabio
Jul 15 '16 at 14:06
okay i am updating the code with comments
– anand tripathi
Jul 25 '16 at 9:06
okay i am updating the code with comments
– anand tripathi
Jul 25 '16 at 9:06
1
1
Note, fourth step can be dropped if you make second step:
a.insert(0,[5,1,1,1])
– U9-Forward
Oct 19 '18 at 5:29
Note, fourth step can be dropped if you make second step:
a.insert(0,[5,1,1,1])
– U9-Forward
Oct 19 '18 at 5:29
yeah thanks @U9-Forward
– anand tripathi
Oct 20 '18 at 14:12
yeah thanks @U9-Forward
– anand tripathi
Oct 20 '18 at 14:12
add a comment |
protected by styvane Apr 24 '16 at 15:14
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
Hk66n E,u5o nRkbLYvxk0E85M7Y5lfvja,dhCNibpricnUMUdkMRgs 3f6AY WmUyvE,zMCDXHe0jiB,MVPFCoeNeU,wT5dQ R486BADqB