Vuex Modules Creating Nested Object
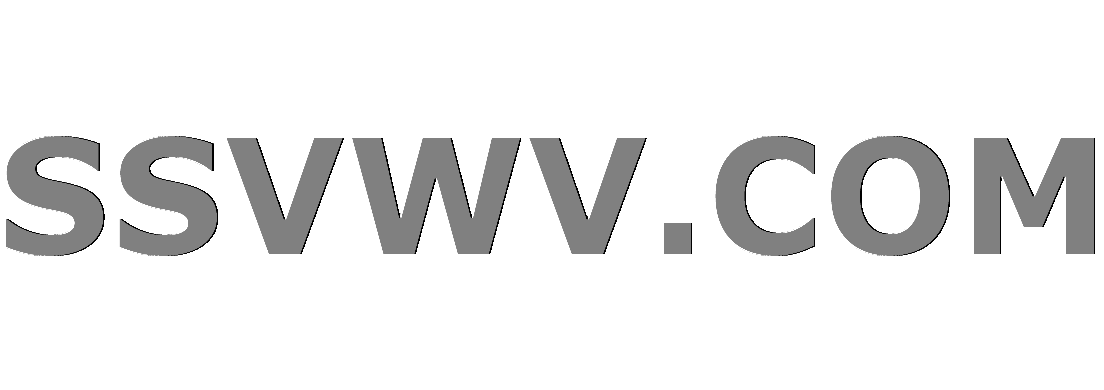
Multi tool use
I have been trying to separate my store.js file into multiple store modules. I have it working (somewhat), but it also created kind of a big issue.
In my initial one single file store, let's suppose this is my state object:
state: { user: '' }
And the output in the Vuex developer tools shows the user object as it should:
User: { name: "John", age: 33 }
But after splitting the the store into modules the user object in the Vuex developer tools is as follows:
User: { User: { name: "John", age: 33 } }
So it creates a new object using the name I give it in the modules object and import in the main store.js file, which then contains the "true" user object.
Is there any way to have it not create a new object and keep it the same as if the entire store lived inside the store.js file?
-Sergio
EDIT:
Here is the structure of my files:
store
store.js
modules
user.js
The code in the modules/user.js:
const state = { User: '' }
const mutations = { ... }
const actions = { ... }
export default { state, mutations, actions }
The code in my store.js
import User from './modules/user';
Vue.use(Vuex);
export default new Vuex.Store({
modules: {
User
}
});
Prior to externalizing the store I would get in Vuex dev tools:
User: { name: "John", age: 33 }
After externalizing the store I get:
User: { User: { name: "John", age: 33 } }
It's nesting User
from the modules/user
file inside the User
in the store.js file. I want to avoid that (if its possible) so that I don't have to change everything in my components/template files.
vue.js store vuex
|
show 1 more comment
I have been trying to separate my store.js file into multiple store modules. I have it working (somewhat), but it also created kind of a big issue.
In my initial one single file store, let's suppose this is my state object:
state: { user: '' }
And the output in the Vuex developer tools shows the user object as it should:
User: { name: "John", age: 33 }
But after splitting the the store into modules the user object in the Vuex developer tools is as follows:
User: { User: { name: "John", age: 33 } }
So it creates a new object using the name I give it in the modules object and import in the main store.js file, which then contains the "true" user object.
Is there any way to have it not create a new object and keep it the same as if the entire store lived inside the store.js file?
-Sergio
EDIT:
Here is the structure of my files:
store
store.js
modules
user.js
The code in the modules/user.js:
const state = { User: '' }
const mutations = { ... }
const actions = { ... }
export default { state, mutations, actions }
The code in my store.js
import User from './modules/user';
Vue.use(Vuex);
export default new Vuex.Store({
modules: {
User
}
});
Prior to externalizing the store I would get in Vuex dev tools:
User: { name: "John", age: 33 }
After externalizing the store I get:
User: { User: { name: "John", age: 33 } }
It's nesting User
from the modules/user
file inside the User
in the store.js file. I want to avoid that (if its possible) so that I don't have to change everything in my components/template files.
vue.js store vuex
But how are you doing this assignment?
– Hamilton Gabriel
Nov 10 '18 at 8:08
Hi Hamilton, I don't understand your question...can you please rephrase.
– Sergio
Nov 10 '18 at 8:11
At what point in time do you do this assignment? And how is this assignment being done? Are you calling this assignment more than once?
– Hamilton Gabriel
Nov 10 '18 at 8:12
I have updated my initial question, hope it clarifies more.
– Sergio
Nov 10 '18 at 8:25
Are you assigning the user again in vuex, or are you doing this yourself?
– Hamilton Gabriel
Nov 10 '18 at 8:29
|
show 1 more comment
I have been trying to separate my store.js file into multiple store modules. I have it working (somewhat), but it also created kind of a big issue.
In my initial one single file store, let's suppose this is my state object:
state: { user: '' }
And the output in the Vuex developer tools shows the user object as it should:
User: { name: "John", age: 33 }
But after splitting the the store into modules the user object in the Vuex developer tools is as follows:
User: { User: { name: "John", age: 33 } }
So it creates a new object using the name I give it in the modules object and import in the main store.js file, which then contains the "true" user object.
Is there any way to have it not create a new object and keep it the same as if the entire store lived inside the store.js file?
-Sergio
EDIT:
Here is the structure of my files:
store
store.js
modules
user.js
The code in the modules/user.js:
const state = { User: '' }
const mutations = { ... }
const actions = { ... }
export default { state, mutations, actions }
The code in my store.js
import User from './modules/user';
Vue.use(Vuex);
export default new Vuex.Store({
modules: {
User
}
});
Prior to externalizing the store I would get in Vuex dev tools:
User: { name: "John", age: 33 }
After externalizing the store I get:
User: { User: { name: "John", age: 33 } }
It's nesting User
from the modules/user
file inside the User
in the store.js file. I want to avoid that (if its possible) so that I don't have to change everything in my components/template files.
vue.js store vuex
I have been trying to separate my store.js file into multiple store modules. I have it working (somewhat), but it also created kind of a big issue.
In my initial one single file store, let's suppose this is my state object:
state: { user: '' }
And the output in the Vuex developer tools shows the user object as it should:
User: { name: "John", age: 33 }
But after splitting the the store into modules the user object in the Vuex developer tools is as follows:
User: { User: { name: "John", age: 33 } }
So it creates a new object using the name I give it in the modules object and import in the main store.js file, which then contains the "true" user object.
Is there any way to have it not create a new object and keep it the same as if the entire store lived inside the store.js file?
-Sergio
EDIT:
Here is the structure of my files:
store
store.js
modules
user.js
The code in the modules/user.js:
const state = { User: '' }
const mutations = { ... }
const actions = { ... }
export default { state, mutations, actions }
The code in my store.js
import User from './modules/user';
Vue.use(Vuex);
export default new Vuex.Store({
modules: {
User
}
});
Prior to externalizing the store I would get in Vuex dev tools:
User: { name: "John", age: 33 }
After externalizing the store I get:
User: { User: { name: "John", age: 33 } }
It's nesting User
from the modules/user
file inside the User
in the store.js file. I want to avoid that (if its possible) so that I don't have to change everything in my components/template files.
vue.js store vuex
vue.js store vuex
edited Nov 10 '18 at 8:24
Sergio
asked Nov 10 '18 at 7:22


SergioSergio
387313
387313
But how are you doing this assignment?
– Hamilton Gabriel
Nov 10 '18 at 8:08
Hi Hamilton, I don't understand your question...can you please rephrase.
– Sergio
Nov 10 '18 at 8:11
At what point in time do you do this assignment? And how is this assignment being done? Are you calling this assignment more than once?
– Hamilton Gabriel
Nov 10 '18 at 8:12
I have updated my initial question, hope it clarifies more.
– Sergio
Nov 10 '18 at 8:25
Are you assigning the user again in vuex, or are you doing this yourself?
– Hamilton Gabriel
Nov 10 '18 at 8:29
|
show 1 more comment
But how are you doing this assignment?
– Hamilton Gabriel
Nov 10 '18 at 8:08
Hi Hamilton, I don't understand your question...can you please rephrase.
– Sergio
Nov 10 '18 at 8:11
At what point in time do you do this assignment? And how is this assignment being done? Are you calling this assignment more than once?
– Hamilton Gabriel
Nov 10 '18 at 8:12
I have updated my initial question, hope it clarifies more.
– Sergio
Nov 10 '18 at 8:25
Are you assigning the user again in vuex, or are you doing this yourself?
– Hamilton Gabriel
Nov 10 '18 at 8:29
But how are you doing this assignment?
– Hamilton Gabriel
Nov 10 '18 at 8:08
But how are you doing this assignment?
– Hamilton Gabriel
Nov 10 '18 at 8:08
Hi Hamilton, I don't understand your question...can you please rephrase.
– Sergio
Nov 10 '18 at 8:11
Hi Hamilton, I don't understand your question...can you please rephrase.
– Sergio
Nov 10 '18 at 8:11
At what point in time do you do this assignment? And how is this assignment being done? Are you calling this assignment more than once?
– Hamilton Gabriel
Nov 10 '18 at 8:12
At what point in time do you do this assignment? And how is this assignment being done? Are you calling this assignment more than once?
– Hamilton Gabriel
Nov 10 '18 at 8:12
I have updated my initial question, hope it clarifies more.
– Sergio
Nov 10 '18 at 8:25
I have updated my initial question, hope it clarifies more.
– Sergio
Nov 10 '18 at 8:25
Are you assigning the user again in vuex, or are you doing this yourself?
– Hamilton Gabriel
Nov 10 '18 at 8:29
Are you assigning the user again in vuex, or are you doing this yourself?
– Hamilton Gabriel
Nov 10 '18 at 8:29
|
show 1 more comment
1 Answer
1
active
oldest
votes
The 'extra parent object' shown in the Vue Devtools is actually the namespaced module. If you set the User module's namespace
attribute to false
, the User state will no longer be placed within a namespaced object and will instead be
registered under the global namespace. (Vuex Namespacing)
Setting namespace: false
is totally fine if you don't expect User function names to conflict with other modular state function names.
const store = new Vuex.Store({
modules: {
User: {
namespaced: false,
state: { user: '' },
...
}
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53236866%2fvuex-modules-creating-nested-object%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The 'extra parent object' shown in the Vue Devtools is actually the namespaced module. If you set the User module's namespace
attribute to false
, the User state will no longer be placed within a namespaced object and will instead be
registered under the global namespace. (Vuex Namespacing)
Setting namespace: false
is totally fine if you don't expect User function names to conflict with other modular state function names.
const store = new Vuex.Store({
modules: {
User: {
namespaced: false,
state: { user: '' },
...
}
}
}
add a comment |
The 'extra parent object' shown in the Vue Devtools is actually the namespaced module. If you set the User module's namespace
attribute to false
, the User state will no longer be placed within a namespaced object and will instead be
registered under the global namespace. (Vuex Namespacing)
Setting namespace: false
is totally fine if you don't expect User function names to conflict with other modular state function names.
const store = new Vuex.Store({
modules: {
User: {
namespaced: false,
state: { user: '' },
...
}
}
}
add a comment |
The 'extra parent object' shown in the Vue Devtools is actually the namespaced module. If you set the User module's namespace
attribute to false
, the User state will no longer be placed within a namespaced object and will instead be
registered under the global namespace. (Vuex Namespacing)
Setting namespace: false
is totally fine if you don't expect User function names to conflict with other modular state function names.
const store = new Vuex.Store({
modules: {
User: {
namespaced: false,
state: { user: '' },
...
}
}
}
The 'extra parent object' shown in the Vue Devtools is actually the namespaced module. If you set the User module's namespace
attribute to false
, the User state will no longer be placed within a namespaced object and will instead be
registered under the global namespace. (Vuex Namespacing)
Setting namespace: false
is totally fine if you don't expect User function names to conflict with other modular state function names.
const store = new Vuex.Store({
modules: {
User: {
namespaced: false,
state: { user: '' },
...
}
}
}
answered Nov 16 '18 at 6:07


Jackson RyanJackson Ryan
163
163
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53236866%2fvuex-modules-creating-nested-object%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ASJBTUCA,p,U 43G8ic,d,mAGmQGbI8xlBlz 4eoYD R,g7ycAbR3 4M904
But how are you doing this assignment?
– Hamilton Gabriel
Nov 10 '18 at 8:08
Hi Hamilton, I don't understand your question...can you please rephrase.
– Sergio
Nov 10 '18 at 8:11
At what point in time do you do this assignment? And how is this assignment being done? Are you calling this assignment more than once?
– Hamilton Gabriel
Nov 10 '18 at 8:12
I have updated my initial question, hope it clarifies more.
– Sergio
Nov 10 '18 at 8:25
Are you assigning the user again in vuex, or are you doing this yourself?
– Hamilton Gabriel
Nov 10 '18 at 8:29