c# xml missing element in nested classes
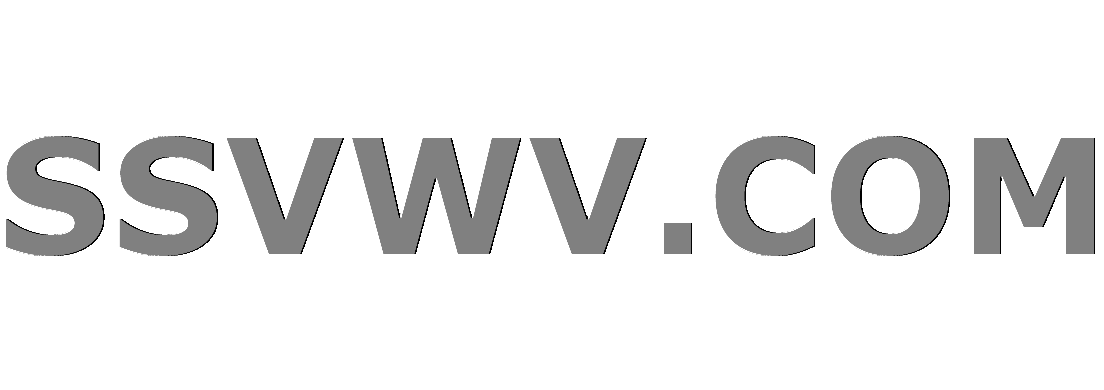
Multi tool use
I have a little problem that's been bugging my head for a few days. I am writing a kind of a manger tool for a virtual racing league.
I want to save all my data into a single xml-file so that it can easily be accessed from several parties.
However, because of the limitation of the xml-serializer, id have to make all my classes public. For that i have written an interface
through which i want to convert my original managing objects (with all the functionality) into raw data containing objects for xml-serialization.
To be able to track who has created/changed something every relevant needs to hold a "ChangeNotification" field.
For that i created a base class with these fields and from which my container classes are derived.
And now here comes the problem: If I serialize only a part of it it all works fine. But as soon as i serialize an Object which contains another Object derived from the same base,
my "CreateChangeNotification" will only be present in the top Object.
I don't see why it's doing that as there are clearly two instances of it saved in the Objects, holding different values at the same time.
Sorry for the long code snippet, but that is what i needed to recreate the problem and it seems to happen everytime with this structure.
[Serializable()]
public class DataManagerXmlContainer<DataContainerType> : IDataManagerContainer
{
[XmlElement("Created")]
public ChangeNotificationXmlContainer created;
[XmlIgnore()]
public IChangeNotificationDataContainer Created { get => created; set => created = new ChangeNotificationXmlContainer(value); }
//[XmlElement("Changed")]
//public ChangeNotificationXmlContainer changed;
//[XmlIgnore()]
//public IChangeNotificationDataContainer LastChange { get => changed; set => changed = new ChangeNotificationXmlContainer(value); }
public DataManagerXmlContainer() { }
}
[Serializable()]
public class ReviewDataXmlContainer : DataManagerXmlContainer<IReviewDataContainer>, IReviewDataContainer
{
[XmlArray("CommentList")]
[XmlArrayItem("Comment")]
public List<ReviewCommentXmlContainer> comments = new List<ReviewCommentXmlContainer>();
[XmlIgnore()]
public ICollection<IReviewCommentDataContainer> Comments
{
get => comments.Cast<IReviewCommentDataContainer>().ToList();
set => comments = value.ToList().ConvertAll(x => new ReviewCommentXmlContainer(x));
}
public ReviewDataXmlContainer() { }
public ReviewDataXmlContainer(string authorID)
{
created = new ChangeNotificationXmlContainer(authorID, DateTime.Now);
}
public void AddComment(IReviewCommentDataContainer comment)
{
comments.Add(new ReviewCommentXmlContainer(comment));
}
}
[Serializable()]
public class ReviewCommentXmlContainer : DataManagerXmlContainer<IReviewCommentDataContainer>, IReviewCommentDataContainer
{
[XmlAttribute("author_id")]
public string AuthorAdminID { get; set; }
public string Text { get; set; }
public ReviewCommentXmlContainer() { }
public ReviewCommentXmlContainer(string authorID, string text)
{
created = new ChangeNotificationXmlContainer(authorID, DateTime.Now);
AuthorAdminID = authorID;
Text = text;
}
public ReviewCommentXmlContainer(IReviewCommentDataContainer dataContainer)
{
AuthorAdminID = dataContainer.AuthorAdminID;
Text = dataContainer.Text;
}
}
[Serializable()]
public class ChangeNotificationXmlContainer : IChangeNotificationDataContainer
{
[XmlAttribute("author_id")]
public string AuthorID { get; set; }
[XmlAttribute("time")]
public DateTime Time { get; set; }
public ChangeNotificationXmlContainer() { }
public ChangeNotificationXmlContainer(string id, DateTime time)
{
AuthorID = id;
Time = time;
}
public ChangeNotificationXmlContainer(IChangeNotificationDataContainer dataContainer)
{
AuthorID = dataContainer.AuthorID;
Time = dataContainer.Time;
}
}
public interface IChangeNotificationDataContainer
{
string AuthorID { get; set; }
DateTime Time { get; set; }
}
public interface IDataManagerContainer
{
IChangeNotificationDataContainer Created { get; set; }
}
public interface IReviewDataContainer : IDataManagerContainer
{
ICollection<IReviewCommentDataContainer> Comments { get; set; }
void AddComment(IReviewCommentDataContainer comment);
}
public interface IReviewCommentDataContainer : IDataManagerContainer
{
string AuthorAdminID { get; set; }
string Text { get; set; }
}
And the Program code:
class Program
{
static void Main(string args)
{
IReviewCommentDataContainer comment = new ReviewCommentXmlContainer("12345", "This is a test");
Task.Delay(1000).Wait();
ReviewDataXmlContainer review = new ReviewDataXmlContainer("23456");
review.AddComment(comment);
XmlSerializer serializer = new XmlSerializer(typeof(ReviewDataXmlContainer));
TextWriter writer = new StreamWriter("Test.xml");
serializer.Serialize(writer, review);
//Task.Delay(-1).Wait();
}
}
This is how my xml looks like:
<?xml version="1.0" encoding="utf-8"?>
<ReviewDataXmlContainer xmlns:xsi="http://www.w3.org/2001/XMLSchema instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<Created author_id="23456" time="2018-11-16T06:56:16.5425819+01:00" />
<CommentList>
<Comment author_id="12345">
<Text>This is a test</Text>
</Comment>
</CommentList>
</ReviewDataXmlContainer>
As you can see the Create element of Comment is just missing.
c# xml serialization
|
show 1 more comment
I have a little problem that's been bugging my head for a few days. I am writing a kind of a manger tool for a virtual racing league.
I want to save all my data into a single xml-file so that it can easily be accessed from several parties.
However, because of the limitation of the xml-serializer, id have to make all my classes public. For that i have written an interface
through which i want to convert my original managing objects (with all the functionality) into raw data containing objects for xml-serialization.
To be able to track who has created/changed something every relevant needs to hold a "ChangeNotification" field.
For that i created a base class with these fields and from which my container classes are derived.
And now here comes the problem: If I serialize only a part of it it all works fine. But as soon as i serialize an Object which contains another Object derived from the same base,
my "CreateChangeNotification" will only be present in the top Object.
I don't see why it's doing that as there are clearly two instances of it saved in the Objects, holding different values at the same time.
Sorry for the long code snippet, but that is what i needed to recreate the problem and it seems to happen everytime with this structure.
[Serializable()]
public class DataManagerXmlContainer<DataContainerType> : IDataManagerContainer
{
[XmlElement("Created")]
public ChangeNotificationXmlContainer created;
[XmlIgnore()]
public IChangeNotificationDataContainer Created { get => created; set => created = new ChangeNotificationXmlContainer(value); }
//[XmlElement("Changed")]
//public ChangeNotificationXmlContainer changed;
//[XmlIgnore()]
//public IChangeNotificationDataContainer LastChange { get => changed; set => changed = new ChangeNotificationXmlContainer(value); }
public DataManagerXmlContainer() { }
}
[Serializable()]
public class ReviewDataXmlContainer : DataManagerXmlContainer<IReviewDataContainer>, IReviewDataContainer
{
[XmlArray("CommentList")]
[XmlArrayItem("Comment")]
public List<ReviewCommentXmlContainer> comments = new List<ReviewCommentXmlContainer>();
[XmlIgnore()]
public ICollection<IReviewCommentDataContainer> Comments
{
get => comments.Cast<IReviewCommentDataContainer>().ToList();
set => comments = value.ToList().ConvertAll(x => new ReviewCommentXmlContainer(x));
}
public ReviewDataXmlContainer() { }
public ReviewDataXmlContainer(string authorID)
{
created = new ChangeNotificationXmlContainer(authorID, DateTime.Now);
}
public void AddComment(IReviewCommentDataContainer comment)
{
comments.Add(new ReviewCommentXmlContainer(comment));
}
}
[Serializable()]
public class ReviewCommentXmlContainer : DataManagerXmlContainer<IReviewCommentDataContainer>, IReviewCommentDataContainer
{
[XmlAttribute("author_id")]
public string AuthorAdminID { get; set; }
public string Text { get; set; }
public ReviewCommentXmlContainer() { }
public ReviewCommentXmlContainer(string authorID, string text)
{
created = new ChangeNotificationXmlContainer(authorID, DateTime.Now);
AuthorAdminID = authorID;
Text = text;
}
public ReviewCommentXmlContainer(IReviewCommentDataContainer dataContainer)
{
AuthorAdminID = dataContainer.AuthorAdminID;
Text = dataContainer.Text;
}
}
[Serializable()]
public class ChangeNotificationXmlContainer : IChangeNotificationDataContainer
{
[XmlAttribute("author_id")]
public string AuthorID { get; set; }
[XmlAttribute("time")]
public DateTime Time { get; set; }
public ChangeNotificationXmlContainer() { }
public ChangeNotificationXmlContainer(string id, DateTime time)
{
AuthorID = id;
Time = time;
}
public ChangeNotificationXmlContainer(IChangeNotificationDataContainer dataContainer)
{
AuthorID = dataContainer.AuthorID;
Time = dataContainer.Time;
}
}
public interface IChangeNotificationDataContainer
{
string AuthorID { get; set; }
DateTime Time { get; set; }
}
public interface IDataManagerContainer
{
IChangeNotificationDataContainer Created { get; set; }
}
public interface IReviewDataContainer : IDataManagerContainer
{
ICollection<IReviewCommentDataContainer> Comments { get; set; }
void AddComment(IReviewCommentDataContainer comment);
}
public interface IReviewCommentDataContainer : IDataManagerContainer
{
string AuthorAdminID { get; set; }
string Text { get; set; }
}
And the Program code:
class Program
{
static void Main(string args)
{
IReviewCommentDataContainer comment = new ReviewCommentXmlContainer("12345", "This is a test");
Task.Delay(1000).Wait();
ReviewDataXmlContainer review = new ReviewDataXmlContainer("23456");
review.AddComment(comment);
XmlSerializer serializer = new XmlSerializer(typeof(ReviewDataXmlContainer));
TextWriter writer = new StreamWriter("Test.xml");
serializer.Serialize(writer, review);
//Task.Delay(-1).Wait();
}
}
This is how my xml looks like:
<?xml version="1.0" encoding="utf-8"?>
<ReviewDataXmlContainer xmlns:xsi="http://www.w3.org/2001/XMLSchema instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<Created author_id="23456" time="2018-11-16T06:56:16.5425819+01:00" />
<CommentList>
<Comment author_id="12345">
<Text>This is a test</Text>
</Comment>
</CommentList>
</ReviewDataXmlContainer>
As you can see the Create element of Comment is just missing.
c# xml serialization
1
this is not actually answering your question but i have to ask - have you considered other options for persistence? if you like file-based why not sqlite.org/onefile.html. you may end up keeping your hair...
– JohnB
Nov 16 '18 at 6:24
Thx. I will take a look into it. However, the main goal of the project is to learn how to work with interfaces and serialization and such.
– S Schulze
Nov 16 '18 at 6:36
When serializing a class that inherits another class you need an include : [XmlInclude(type)]
– jdweng
Nov 16 '18 at 10:11
Thx i will give that a go later.
– S Schulze
Nov 16 '18 at 12:12
@jdweng: upon researching [xmlinclude] i only came across usage for serializing derived types. In this case i want it to correctly serialize members of the base type, which it should already know. How would i use [xmlinclude] in this context?
– S Schulze
Nov 16 '18 at 13:03
|
show 1 more comment
I have a little problem that's been bugging my head for a few days. I am writing a kind of a manger tool for a virtual racing league.
I want to save all my data into a single xml-file so that it can easily be accessed from several parties.
However, because of the limitation of the xml-serializer, id have to make all my classes public. For that i have written an interface
through which i want to convert my original managing objects (with all the functionality) into raw data containing objects for xml-serialization.
To be able to track who has created/changed something every relevant needs to hold a "ChangeNotification" field.
For that i created a base class with these fields and from which my container classes are derived.
And now here comes the problem: If I serialize only a part of it it all works fine. But as soon as i serialize an Object which contains another Object derived from the same base,
my "CreateChangeNotification" will only be present in the top Object.
I don't see why it's doing that as there are clearly two instances of it saved in the Objects, holding different values at the same time.
Sorry for the long code snippet, but that is what i needed to recreate the problem and it seems to happen everytime with this structure.
[Serializable()]
public class DataManagerXmlContainer<DataContainerType> : IDataManagerContainer
{
[XmlElement("Created")]
public ChangeNotificationXmlContainer created;
[XmlIgnore()]
public IChangeNotificationDataContainer Created { get => created; set => created = new ChangeNotificationXmlContainer(value); }
//[XmlElement("Changed")]
//public ChangeNotificationXmlContainer changed;
//[XmlIgnore()]
//public IChangeNotificationDataContainer LastChange { get => changed; set => changed = new ChangeNotificationXmlContainer(value); }
public DataManagerXmlContainer() { }
}
[Serializable()]
public class ReviewDataXmlContainer : DataManagerXmlContainer<IReviewDataContainer>, IReviewDataContainer
{
[XmlArray("CommentList")]
[XmlArrayItem("Comment")]
public List<ReviewCommentXmlContainer> comments = new List<ReviewCommentXmlContainer>();
[XmlIgnore()]
public ICollection<IReviewCommentDataContainer> Comments
{
get => comments.Cast<IReviewCommentDataContainer>().ToList();
set => comments = value.ToList().ConvertAll(x => new ReviewCommentXmlContainer(x));
}
public ReviewDataXmlContainer() { }
public ReviewDataXmlContainer(string authorID)
{
created = new ChangeNotificationXmlContainer(authorID, DateTime.Now);
}
public void AddComment(IReviewCommentDataContainer comment)
{
comments.Add(new ReviewCommentXmlContainer(comment));
}
}
[Serializable()]
public class ReviewCommentXmlContainer : DataManagerXmlContainer<IReviewCommentDataContainer>, IReviewCommentDataContainer
{
[XmlAttribute("author_id")]
public string AuthorAdminID { get; set; }
public string Text { get; set; }
public ReviewCommentXmlContainer() { }
public ReviewCommentXmlContainer(string authorID, string text)
{
created = new ChangeNotificationXmlContainer(authorID, DateTime.Now);
AuthorAdminID = authorID;
Text = text;
}
public ReviewCommentXmlContainer(IReviewCommentDataContainer dataContainer)
{
AuthorAdminID = dataContainer.AuthorAdminID;
Text = dataContainer.Text;
}
}
[Serializable()]
public class ChangeNotificationXmlContainer : IChangeNotificationDataContainer
{
[XmlAttribute("author_id")]
public string AuthorID { get; set; }
[XmlAttribute("time")]
public DateTime Time { get; set; }
public ChangeNotificationXmlContainer() { }
public ChangeNotificationXmlContainer(string id, DateTime time)
{
AuthorID = id;
Time = time;
}
public ChangeNotificationXmlContainer(IChangeNotificationDataContainer dataContainer)
{
AuthorID = dataContainer.AuthorID;
Time = dataContainer.Time;
}
}
public interface IChangeNotificationDataContainer
{
string AuthorID { get; set; }
DateTime Time { get; set; }
}
public interface IDataManagerContainer
{
IChangeNotificationDataContainer Created { get; set; }
}
public interface IReviewDataContainer : IDataManagerContainer
{
ICollection<IReviewCommentDataContainer> Comments { get; set; }
void AddComment(IReviewCommentDataContainer comment);
}
public interface IReviewCommentDataContainer : IDataManagerContainer
{
string AuthorAdminID { get; set; }
string Text { get; set; }
}
And the Program code:
class Program
{
static void Main(string args)
{
IReviewCommentDataContainer comment = new ReviewCommentXmlContainer("12345", "This is a test");
Task.Delay(1000).Wait();
ReviewDataXmlContainer review = new ReviewDataXmlContainer("23456");
review.AddComment(comment);
XmlSerializer serializer = new XmlSerializer(typeof(ReviewDataXmlContainer));
TextWriter writer = new StreamWriter("Test.xml");
serializer.Serialize(writer, review);
//Task.Delay(-1).Wait();
}
}
This is how my xml looks like:
<?xml version="1.0" encoding="utf-8"?>
<ReviewDataXmlContainer xmlns:xsi="http://www.w3.org/2001/XMLSchema instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<Created author_id="23456" time="2018-11-16T06:56:16.5425819+01:00" />
<CommentList>
<Comment author_id="12345">
<Text>This is a test</Text>
</Comment>
</CommentList>
</ReviewDataXmlContainer>
As you can see the Create element of Comment is just missing.
c# xml serialization
I have a little problem that's been bugging my head for a few days. I am writing a kind of a manger tool for a virtual racing league.
I want to save all my data into a single xml-file so that it can easily be accessed from several parties.
However, because of the limitation of the xml-serializer, id have to make all my classes public. For that i have written an interface
through which i want to convert my original managing objects (with all the functionality) into raw data containing objects for xml-serialization.
To be able to track who has created/changed something every relevant needs to hold a "ChangeNotification" field.
For that i created a base class with these fields and from which my container classes are derived.
And now here comes the problem: If I serialize only a part of it it all works fine. But as soon as i serialize an Object which contains another Object derived from the same base,
my "CreateChangeNotification" will only be present in the top Object.
I don't see why it's doing that as there are clearly two instances of it saved in the Objects, holding different values at the same time.
Sorry for the long code snippet, but that is what i needed to recreate the problem and it seems to happen everytime with this structure.
[Serializable()]
public class DataManagerXmlContainer<DataContainerType> : IDataManagerContainer
{
[XmlElement("Created")]
public ChangeNotificationXmlContainer created;
[XmlIgnore()]
public IChangeNotificationDataContainer Created { get => created; set => created = new ChangeNotificationXmlContainer(value); }
//[XmlElement("Changed")]
//public ChangeNotificationXmlContainer changed;
//[XmlIgnore()]
//public IChangeNotificationDataContainer LastChange { get => changed; set => changed = new ChangeNotificationXmlContainer(value); }
public DataManagerXmlContainer() { }
}
[Serializable()]
public class ReviewDataXmlContainer : DataManagerXmlContainer<IReviewDataContainer>, IReviewDataContainer
{
[XmlArray("CommentList")]
[XmlArrayItem("Comment")]
public List<ReviewCommentXmlContainer> comments = new List<ReviewCommentXmlContainer>();
[XmlIgnore()]
public ICollection<IReviewCommentDataContainer> Comments
{
get => comments.Cast<IReviewCommentDataContainer>().ToList();
set => comments = value.ToList().ConvertAll(x => new ReviewCommentXmlContainer(x));
}
public ReviewDataXmlContainer() { }
public ReviewDataXmlContainer(string authorID)
{
created = new ChangeNotificationXmlContainer(authorID, DateTime.Now);
}
public void AddComment(IReviewCommentDataContainer comment)
{
comments.Add(new ReviewCommentXmlContainer(comment));
}
}
[Serializable()]
public class ReviewCommentXmlContainer : DataManagerXmlContainer<IReviewCommentDataContainer>, IReviewCommentDataContainer
{
[XmlAttribute("author_id")]
public string AuthorAdminID { get; set; }
public string Text { get; set; }
public ReviewCommentXmlContainer() { }
public ReviewCommentXmlContainer(string authorID, string text)
{
created = new ChangeNotificationXmlContainer(authorID, DateTime.Now);
AuthorAdminID = authorID;
Text = text;
}
public ReviewCommentXmlContainer(IReviewCommentDataContainer dataContainer)
{
AuthorAdminID = dataContainer.AuthorAdminID;
Text = dataContainer.Text;
}
}
[Serializable()]
public class ChangeNotificationXmlContainer : IChangeNotificationDataContainer
{
[XmlAttribute("author_id")]
public string AuthorID { get; set; }
[XmlAttribute("time")]
public DateTime Time { get; set; }
public ChangeNotificationXmlContainer() { }
public ChangeNotificationXmlContainer(string id, DateTime time)
{
AuthorID = id;
Time = time;
}
public ChangeNotificationXmlContainer(IChangeNotificationDataContainer dataContainer)
{
AuthorID = dataContainer.AuthorID;
Time = dataContainer.Time;
}
}
public interface IChangeNotificationDataContainer
{
string AuthorID { get; set; }
DateTime Time { get; set; }
}
public interface IDataManagerContainer
{
IChangeNotificationDataContainer Created { get; set; }
}
public interface IReviewDataContainer : IDataManagerContainer
{
ICollection<IReviewCommentDataContainer> Comments { get; set; }
void AddComment(IReviewCommentDataContainer comment);
}
public interface IReviewCommentDataContainer : IDataManagerContainer
{
string AuthorAdminID { get; set; }
string Text { get; set; }
}
And the Program code:
class Program
{
static void Main(string args)
{
IReviewCommentDataContainer comment = new ReviewCommentXmlContainer("12345", "This is a test");
Task.Delay(1000).Wait();
ReviewDataXmlContainer review = new ReviewDataXmlContainer("23456");
review.AddComment(comment);
XmlSerializer serializer = new XmlSerializer(typeof(ReviewDataXmlContainer));
TextWriter writer = new StreamWriter("Test.xml");
serializer.Serialize(writer, review);
//Task.Delay(-1).Wait();
}
}
This is how my xml looks like:
<?xml version="1.0" encoding="utf-8"?>
<ReviewDataXmlContainer xmlns:xsi="http://www.w3.org/2001/XMLSchema instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<Created author_id="23456" time="2018-11-16T06:56:16.5425819+01:00" />
<CommentList>
<Comment author_id="12345">
<Text>This is a test</Text>
</Comment>
</CommentList>
</ReviewDataXmlContainer>
As you can see the Create element of Comment is just missing.
c# xml serialization
c# xml serialization
asked Nov 16 '18 at 6:17


S SchulzeS Schulze
11
11
1
this is not actually answering your question but i have to ask - have you considered other options for persistence? if you like file-based why not sqlite.org/onefile.html. you may end up keeping your hair...
– JohnB
Nov 16 '18 at 6:24
Thx. I will take a look into it. However, the main goal of the project is to learn how to work with interfaces and serialization and such.
– S Schulze
Nov 16 '18 at 6:36
When serializing a class that inherits another class you need an include : [XmlInclude(type)]
– jdweng
Nov 16 '18 at 10:11
Thx i will give that a go later.
– S Schulze
Nov 16 '18 at 12:12
@jdweng: upon researching [xmlinclude] i only came across usage for serializing derived types. In this case i want it to correctly serialize members of the base type, which it should already know. How would i use [xmlinclude] in this context?
– S Schulze
Nov 16 '18 at 13:03
|
show 1 more comment
1
this is not actually answering your question but i have to ask - have you considered other options for persistence? if you like file-based why not sqlite.org/onefile.html. you may end up keeping your hair...
– JohnB
Nov 16 '18 at 6:24
Thx. I will take a look into it. However, the main goal of the project is to learn how to work with interfaces and serialization and such.
– S Schulze
Nov 16 '18 at 6:36
When serializing a class that inherits another class you need an include : [XmlInclude(type)]
– jdweng
Nov 16 '18 at 10:11
Thx i will give that a go later.
– S Schulze
Nov 16 '18 at 12:12
@jdweng: upon researching [xmlinclude] i only came across usage for serializing derived types. In this case i want it to correctly serialize members of the base type, which it should already know. How would i use [xmlinclude] in this context?
– S Schulze
Nov 16 '18 at 13:03
1
1
this is not actually answering your question but i have to ask - have you considered other options for persistence? if you like file-based why not sqlite.org/onefile.html. you may end up keeping your hair...
– JohnB
Nov 16 '18 at 6:24
this is not actually answering your question but i have to ask - have you considered other options for persistence? if you like file-based why not sqlite.org/onefile.html. you may end up keeping your hair...
– JohnB
Nov 16 '18 at 6:24
Thx. I will take a look into it. However, the main goal of the project is to learn how to work with interfaces and serialization and such.
– S Schulze
Nov 16 '18 at 6:36
Thx. I will take a look into it. However, the main goal of the project is to learn how to work with interfaces and serialization and such.
– S Schulze
Nov 16 '18 at 6:36
When serializing a class that inherits another class you need an include : [XmlInclude(type)]
– jdweng
Nov 16 '18 at 10:11
When serializing a class that inherits another class you need an include : [XmlInclude(type)]
– jdweng
Nov 16 '18 at 10:11
Thx i will give that a go later.
– S Schulze
Nov 16 '18 at 12:12
Thx i will give that a go later.
– S Schulze
Nov 16 '18 at 12:12
@jdweng: upon researching [xmlinclude] i only came across usage for serializing derived types. In this case i want it to correctly serialize members of the base type, which it should already know. How would i use [xmlinclude] in this context?
– S Schulze
Nov 16 '18 at 13:03
@jdweng: upon researching [xmlinclude] i only came across usage for serializing derived types. In this case i want it to correctly serialize members of the base type, which it should already know. How would i use [xmlinclude] in this context?
– S Schulze
Nov 16 '18 at 13:03
|
show 1 more comment
1 Answer
1
active
oldest
votes
I found the problem. When adding the comment object through the interface the changenotification is not taken with and gets lost.
In my complete code the problem was a bit more masked becase i used reflection to automatically set all properties.
However, i was only looking at the main interface-type and not all implemented ones.
I changed the code and now it works fine.
Thanks for the support though.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53332444%2fc-sharp-xml-missing-element-in-nested-classes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I found the problem. When adding the comment object through the interface the changenotification is not taken with and gets lost.
In my complete code the problem was a bit more masked becase i used reflection to automatically set all properties.
However, i was only looking at the main interface-type and not all implemented ones.
I changed the code and now it works fine.
Thanks for the support though.
add a comment |
I found the problem. When adding the comment object through the interface the changenotification is not taken with and gets lost.
In my complete code the problem was a bit more masked becase i used reflection to automatically set all properties.
However, i was only looking at the main interface-type and not all implemented ones.
I changed the code and now it works fine.
Thanks for the support though.
add a comment |
I found the problem. When adding the comment object through the interface the changenotification is not taken with and gets lost.
In my complete code the problem was a bit more masked becase i used reflection to automatically set all properties.
However, i was only looking at the main interface-type and not all implemented ones.
I changed the code and now it works fine.
Thanks for the support though.
I found the problem. When adding the comment object through the interface the changenotification is not taken with and gets lost.
In my complete code the problem was a bit more masked becase i used reflection to automatically set all properties.
However, i was only looking at the main interface-type and not all implemented ones.
I changed the code and now it works fine.
Thanks for the support though.
answered Nov 17 '18 at 7:49


S SchulzeS Schulze
11
11
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53332444%2fc-sharp-xml-missing-element-in-nested-classes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cP9FXpYPPYVXmZh8RKfHW8r GSSy,k01veHPYXoE bGrGAYFbM,pg,OgxBm8Ts4y yC5WK25u cGzw,Hv8u6DTfxSMHVusx9 k 5fKzGF,Z
1
this is not actually answering your question but i have to ask - have you considered other options for persistence? if you like file-based why not sqlite.org/onefile.html. you may end up keeping your hair...
– JohnB
Nov 16 '18 at 6:24
Thx. I will take a look into it. However, the main goal of the project is to learn how to work with interfaces and serialization and such.
– S Schulze
Nov 16 '18 at 6:36
When serializing a class that inherits another class you need an include : [XmlInclude(type)]
– jdweng
Nov 16 '18 at 10:11
Thx i will give that a go later.
– S Schulze
Nov 16 '18 at 12:12
@jdweng: upon researching [xmlinclude] i only came across usage for serializing derived types. In this case i want it to correctly serialize members of the base type, which it should already know. How would i use [xmlinclude] in this context?
– S Schulze
Nov 16 '18 at 13:03