How to observe WorkManager during Configuration Change?
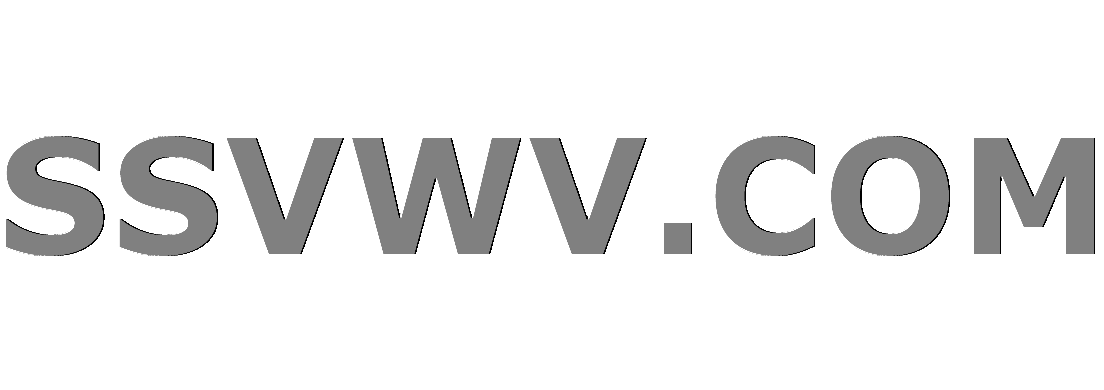
Multi tool use
I am investigating the use of androidx.work.WorkManager
for background work within my current Android application.
The versions I am employing are :-
compileSdkVersion = 27
targetSdkVersion = 27
minSdkVersion = 21
buildToolsVersion = "27.0.3"
supportLibVersion = "27.1.1"
archLifecycleVersion = "1.1.1"
archWorkerRuntimeVersion = "1.0.0-alpha11"
My application consists of An activity with a Fragment, the Fragment has an associated android.arch.lifecycle.AndroidViewModel
and the view model has a repository.
I control the WorkManager from within my repository class.
I set the fragment as the LifecycleOwner for the workManager Observer.
When I allow the workManager to complete its work without changing the orientation of my testing device it works as designed.
However when I start the workManager and then during its background processing I change the device orientation my observer is removed as the Fragment has been destroyed. The work manager still completes with success as I can see this in my application logs...
WorkerWrapper: Worker result SUCCESS for Work [ id=33058369-010d-4e07-ae94-fb04bcf4d805, tags={ com.sync.SyncWorker, SYNC-IN-PROGRESS-TAG } ]
I switched to using observeForever, however this approach gave worse results as my observer only received ENQUEUED
and RUNNING
, it never "saw" the SUCCEEDED
state, even though I still saw the above WorkerWrapper
log entry.
How can I observe WorkManager work before during and after a device configuration change?
My Code resembles this:-
private WorkManager mWorkManager;
/**
* SINGLETON
*/
private MyRepository() {
mWorkManager = WorkManager.getInstance();
}
private static final String WORK_IN_PROGRESS_TAG = "SYNC-IN-PROGRESS-TAG";
private static final String UNIQUE_WORK_NAME = "SYNC-UNIQUE_WORK_NAME";
private final Observer<WorkInfo> workerObserver = constructWorkStatusObserver();
private Observer<WorkInfo> constructWorkStatusObserver() {
return workInfo -> {
if (workInfo == null) {
return;
}
if (workInfo.getState().equals(WorkInfo.State.SUCCEEDED)) {
//DO SOMETHING
} else if (workInfo.getState().equals(WorkInfo.State.FAILED)) {
Log.e(TAG, "SyncWorker FAILED:");
}
};
}
private Action manageSuccess(final LifecycleOwner lifecycleOwner) {
return () -> {
final LiveData<WorkInfo> workStatus = constructWorkRequest();
workStatus.observe(lifecycleOwner, workerObserver);
};
}
private LiveData<WorkInfo> constructWorkRequest() {
final OneTimeWorkRequest refreshDatabaseWork = new OneTimeWorkRequest.Builder(SyncWorker.class).addTag(WORK_IN_PROGRESS_TAG).build();
mWorkManager.beginUniqueWork(UNIQUE_WORK_NAME, ExistingWorkPolicy.KEEP, refreshDatabaseWork).enqueue();
return mWorkManager.getWorkInfoByIdLiveData(refreshDatabaseWork.getId());
}

add a comment |
I am investigating the use of androidx.work.WorkManager
for background work within my current Android application.
The versions I am employing are :-
compileSdkVersion = 27
targetSdkVersion = 27
minSdkVersion = 21
buildToolsVersion = "27.0.3"
supportLibVersion = "27.1.1"
archLifecycleVersion = "1.1.1"
archWorkerRuntimeVersion = "1.0.0-alpha11"
My application consists of An activity with a Fragment, the Fragment has an associated android.arch.lifecycle.AndroidViewModel
and the view model has a repository.
I control the WorkManager from within my repository class.
I set the fragment as the LifecycleOwner for the workManager Observer.
When I allow the workManager to complete its work without changing the orientation of my testing device it works as designed.
However when I start the workManager and then during its background processing I change the device orientation my observer is removed as the Fragment has been destroyed. The work manager still completes with success as I can see this in my application logs...
WorkerWrapper: Worker result SUCCESS for Work [ id=33058369-010d-4e07-ae94-fb04bcf4d805, tags={ com.sync.SyncWorker, SYNC-IN-PROGRESS-TAG } ]
I switched to using observeForever, however this approach gave worse results as my observer only received ENQUEUED
and RUNNING
, it never "saw" the SUCCEEDED
state, even though I still saw the above WorkerWrapper
log entry.
How can I observe WorkManager work before during and after a device configuration change?
My Code resembles this:-
private WorkManager mWorkManager;
/**
* SINGLETON
*/
private MyRepository() {
mWorkManager = WorkManager.getInstance();
}
private static final String WORK_IN_PROGRESS_TAG = "SYNC-IN-PROGRESS-TAG";
private static final String UNIQUE_WORK_NAME = "SYNC-UNIQUE_WORK_NAME";
private final Observer<WorkInfo> workerObserver = constructWorkStatusObserver();
private Observer<WorkInfo> constructWorkStatusObserver() {
return workInfo -> {
if (workInfo == null) {
return;
}
if (workInfo.getState().equals(WorkInfo.State.SUCCEEDED)) {
//DO SOMETHING
} else if (workInfo.getState().equals(WorkInfo.State.FAILED)) {
Log.e(TAG, "SyncWorker FAILED:");
}
};
}
private Action manageSuccess(final LifecycleOwner lifecycleOwner) {
return () -> {
final LiveData<WorkInfo> workStatus = constructWorkRequest();
workStatus.observe(lifecycleOwner, workerObserver);
};
}
private LiveData<WorkInfo> constructWorkRequest() {
final OneTimeWorkRequest refreshDatabaseWork = new OneTimeWorkRequest.Builder(SyncWorker.class).addTag(WORK_IN_PROGRESS_TAG).build();
mWorkManager.beginUniqueWork(UNIQUE_WORK_NAME, ExistingWorkPolicy.KEEP, refreshDatabaseWork).enqueue();
return mWorkManager.getWorkInfoByIdLiveData(refreshDatabaseWork.getId());
}

can you post code about how you observe result ?
– Jeel Vankhede
Nov 15 '18 at 14:14
@JeelVankhede yes, just added it, what mistake have I made?
– Hector
Nov 15 '18 at 14:26
You can try observing livedata by your unique work tag with this methodgetWorkInfosByTagLiveData
in yourconstructWorkRequest
. May be your observer getting null due to id change.
– Jeel Vankhede
Nov 15 '18 at 15:08
add a comment |
I am investigating the use of androidx.work.WorkManager
for background work within my current Android application.
The versions I am employing are :-
compileSdkVersion = 27
targetSdkVersion = 27
minSdkVersion = 21
buildToolsVersion = "27.0.3"
supportLibVersion = "27.1.1"
archLifecycleVersion = "1.1.1"
archWorkerRuntimeVersion = "1.0.0-alpha11"
My application consists of An activity with a Fragment, the Fragment has an associated android.arch.lifecycle.AndroidViewModel
and the view model has a repository.
I control the WorkManager from within my repository class.
I set the fragment as the LifecycleOwner for the workManager Observer.
When I allow the workManager to complete its work without changing the orientation of my testing device it works as designed.
However when I start the workManager and then during its background processing I change the device orientation my observer is removed as the Fragment has been destroyed. The work manager still completes with success as I can see this in my application logs...
WorkerWrapper: Worker result SUCCESS for Work [ id=33058369-010d-4e07-ae94-fb04bcf4d805, tags={ com.sync.SyncWorker, SYNC-IN-PROGRESS-TAG } ]
I switched to using observeForever, however this approach gave worse results as my observer only received ENQUEUED
and RUNNING
, it never "saw" the SUCCEEDED
state, even though I still saw the above WorkerWrapper
log entry.
How can I observe WorkManager work before during and after a device configuration change?
My Code resembles this:-
private WorkManager mWorkManager;
/**
* SINGLETON
*/
private MyRepository() {
mWorkManager = WorkManager.getInstance();
}
private static final String WORK_IN_PROGRESS_TAG = "SYNC-IN-PROGRESS-TAG";
private static final String UNIQUE_WORK_NAME = "SYNC-UNIQUE_WORK_NAME";
private final Observer<WorkInfo> workerObserver = constructWorkStatusObserver();
private Observer<WorkInfo> constructWorkStatusObserver() {
return workInfo -> {
if (workInfo == null) {
return;
}
if (workInfo.getState().equals(WorkInfo.State.SUCCEEDED)) {
//DO SOMETHING
} else if (workInfo.getState().equals(WorkInfo.State.FAILED)) {
Log.e(TAG, "SyncWorker FAILED:");
}
};
}
private Action manageSuccess(final LifecycleOwner lifecycleOwner) {
return () -> {
final LiveData<WorkInfo> workStatus = constructWorkRequest();
workStatus.observe(lifecycleOwner, workerObserver);
};
}
private LiveData<WorkInfo> constructWorkRequest() {
final OneTimeWorkRequest refreshDatabaseWork = new OneTimeWorkRequest.Builder(SyncWorker.class).addTag(WORK_IN_PROGRESS_TAG).build();
mWorkManager.beginUniqueWork(UNIQUE_WORK_NAME, ExistingWorkPolicy.KEEP, refreshDatabaseWork).enqueue();
return mWorkManager.getWorkInfoByIdLiveData(refreshDatabaseWork.getId());
}

I am investigating the use of androidx.work.WorkManager
for background work within my current Android application.
The versions I am employing are :-
compileSdkVersion = 27
targetSdkVersion = 27
minSdkVersion = 21
buildToolsVersion = "27.0.3"
supportLibVersion = "27.1.1"
archLifecycleVersion = "1.1.1"
archWorkerRuntimeVersion = "1.0.0-alpha11"
My application consists of An activity with a Fragment, the Fragment has an associated android.arch.lifecycle.AndroidViewModel
and the view model has a repository.
I control the WorkManager from within my repository class.
I set the fragment as the LifecycleOwner for the workManager Observer.
When I allow the workManager to complete its work without changing the orientation of my testing device it works as designed.
However when I start the workManager and then during its background processing I change the device orientation my observer is removed as the Fragment has been destroyed. The work manager still completes with success as I can see this in my application logs...
WorkerWrapper: Worker result SUCCESS for Work [ id=33058369-010d-4e07-ae94-fb04bcf4d805, tags={ com.sync.SyncWorker, SYNC-IN-PROGRESS-TAG } ]
I switched to using observeForever, however this approach gave worse results as my observer only received ENQUEUED
and RUNNING
, it never "saw" the SUCCEEDED
state, even though I still saw the above WorkerWrapper
log entry.
How can I observe WorkManager work before during and after a device configuration change?
My Code resembles this:-
private WorkManager mWorkManager;
/**
* SINGLETON
*/
private MyRepository() {
mWorkManager = WorkManager.getInstance();
}
private static final String WORK_IN_PROGRESS_TAG = "SYNC-IN-PROGRESS-TAG";
private static final String UNIQUE_WORK_NAME = "SYNC-UNIQUE_WORK_NAME";
private final Observer<WorkInfo> workerObserver = constructWorkStatusObserver();
private Observer<WorkInfo> constructWorkStatusObserver() {
return workInfo -> {
if (workInfo == null) {
return;
}
if (workInfo.getState().equals(WorkInfo.State.SUCCEEDED)) {
//DO SOMETHING
} else if (workInfo.getState().equals(WorkInfo.State.FAILED)) {
Log.e(TAG, "SyncWorker FAILED:");
}
};
}
private Action manageSuccess(final LifecycleOwner lifecycleOwner) {
return () -> {
final LiveData<WorkInfo> workStatus = constructWorkRequest();
workStatus.observe(lifecycleOwner, workerObserver);
};
}
private LiveData<WorkInfo> constructWorkRequest() {
final OneTimeWorkRequest refreshDatabaseWork = new OneTimeWorkRequest.Builder(SyncWorker.class).addTag(WORK_IN_PROGRESS_TAG).build();
mWorkManager.beginUniqueWork(UNIQUE_WORK_NAME, ExistingWorkPolicy.KEEP, refreshDatabaseWork).enqueue();
return mWorkManager.getWorkInfoByIdLiveData(refreshDatabaseWork.getId());
}


edited Nov 15 '18 at 14:34


Fantômas
32.8k156390
32.8k156390
asked Nov 15 '18 at 14:07


HectorHector
87094596
87094596
can you post code about how you observe result ?
– Jeel Vankhede
Nov 15 '18 at 14:14
@JeelVankhede yes, just added it, what mistake have I made?
– Hector
Nov 15 '18 at 14:26
You can try observing livedata by your unique work tag with this methodgetWorkInfosByTagLiveData
in yourconstructWorkRequest
. May be your observer getting null due to id change.
– Jeel Vankhede
Nov 15 '18 at 15:08
add a comment |
can you post code about how you observe result ?
– Jeel Vankhede
Nov 15 '18 at 14:14
@JeelVankhede yes, just added it, what mistake have I made?
– Hector
Nov 15 '18 at 14:26
You can try observing livedata by your unique work tag with this methodgetWorkInfosByTagLiveData
in yourconstructWorkRequest
. May be your observer getting null due to id change.
– Jeel Vankhede
Nov 15 '18 at 15:08
can you post code about how you observe result ?
– Jeel Vankhede
Nov 15 '18 at 14:14
can you post code about how you observe result ?
– Jeel Vankhede
Nov 15 '18 at 14:14
@JeelVankhede yes, just added it, what mistake have I made?
– Hector
Nov 15 '18 at 14:26
@JeelVankhede yes, just added it, what mistake have I made?
– Hector
Nov 15 '18 at 14:26
You can try observing livedata by your unique work tag with this method
getWorkInfosByTagLiveData
in your constructWorkRequest
. May be your observer getting null due to id change.– Jeel Vankhede
Nov 15 '18 at 15:08
You can try observing livedata by your unique work tag with this method
getWorkInfosByTagLiveData
in your constructWorkRequest
. May be your observer getting null due to id change.– Jeel Vankhede
Nov 15 '18 at 15:08
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53321259%2fhow-to-observe-workmanager-during-configuration-change%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53321259%2fhow-to-observe-workmanager-during-configuration-change%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
2G,b1tVmrkIvoguXAfbkTiN9f,My9eJvOGAv8JyG9UrovTgMDNW6 AA4j18WgrtLGhQPlL7qltPDaa ARR72fG,X17V,zLmHG x9i
can you post code about how you observe result ?
– Jeel Vankhede
Nov 15 '18 at 14:14
@JeelVankhede yes, just added it, what mistake have I made?
– Hector
Nov 15 '18 at 14:26
You can try observing livedata by your unique work tag with this method
getWorkInfosByTagLiveData
in yourconstructWorkRequest
. May be your observer getting null due to id change.– Jeel Vankhede
Nov 15 '18 at 15:08