Computed property not updating
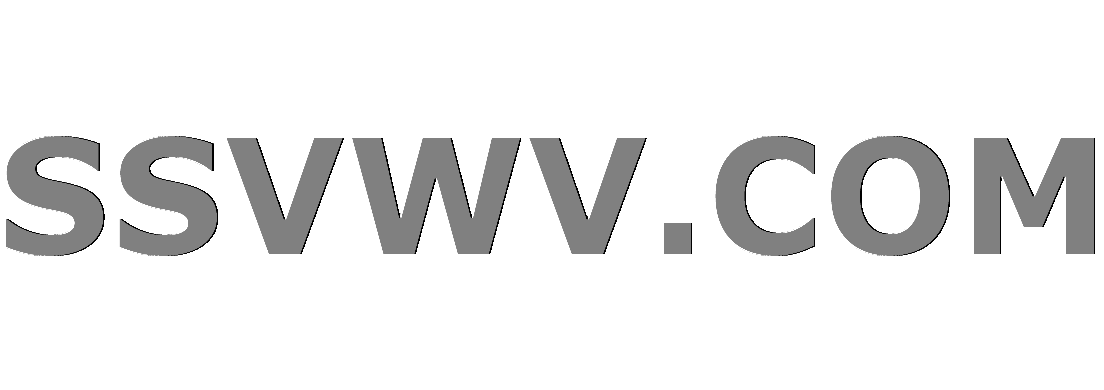
Multi tool use
I have a weird problem. I'm trying do to a simple time tracking app.
So I have this form.
<form class="form" @submit.prevent="saveHours">
<div class="status">
<div class="selector" v-for="(select, index) in select_all">
<component :is="select" :id="index" @percentage="trackTime"></component>
</div>
</div><!-- /.status -->
<div class="form-submit">
<button type="submit" class="form__submit">
<span v-if="loading">Guardando...</span>
<span v-else>Guardar</span>
</button>
</div>
</form>
And this is my vue code
export default {
name: 'home',
data() {
return {
select_all: [Selector],
loading: false,
allTimes: ,
saveForm:
}
},
components: {
Selector
},
computed: {
calculateTotal() {
return this.allTimes.reduce((accumulator, currentValue) => parseInt(accumulator) + parseInt(currentValue), 0);
}
},
methods: {
addNewSelector() {
this.calcTotal();
this.select_all.push(Selector)
},
trackTime(time, index, proyecto) {
this.currentTime = time;
this.allTimes[index] = time;
const data = {
time,
proyecto
}
this.saveForm[index] = data;
},
saveHours() {
const currentWeek = moment(new Date()).format('w');
const diverRef = db.collection('divers').doc(firebaseAuth.currentUser.email);
const currentWeekRef = diverRef.collection('reportes').doc(`semana_${currentWeek}`);
var self = this;
currentWeekRef.get().then(function(doc) {
if ( doc.exists ) {
console.log('Ya registraste tus horas');
} else {
currentWeekRef.set({
data: self.saveForm
})
}
});
},
}
}
I have a component called , what I do is that when someone types the number of hours, I emit that time back to the parent and use the trackTime
function to add the time of each project to the allTimes
array.
I'm trying to use a computed property calculateTotal
to add the times together so I know when a user completed the 100% of hours. But is not updating.
Is really weird, if I use it as a method it works like a charm, but it doesn't work for me because i want it to update as the user is typing and since I'm using a component for the input I can't use keyup
events.
I've been trying to figure this out for hours now and nothing. Thanks!
javascript vue.js computed-properties
add a comment |
I have a weird problem. I'm trying do to a simple time tracking app.
So I have this form.
<form class="form" @submit.prevent="saveHours">
<div class="status">
<div class="selector" v-for="(select, index) in select_all">
<component :is="select" :id="index" @percentage="trackTime"></component>
</div>
</div><!-- /.status -->
<div class="form-submit">
<button type="submit" class="form__submit">
<span v-if="loading">Guardando...</span>
<span v-else>Guardar</span>
</button>
</div>
</form>
And this is my vue code
export default {
name: 'home',
data() {
return {
select_all: [Selector],
loading: false,
allTimes: ,
saveForm:
}
},
components: {
Selector
},
computed: {
calculateTotal() {
return this.allTimes.reduce((accumulator, currentValue) => parseInt(accumulator) + parseInt(currentValue), 0);
}
},
methods: {
addNewSelector() {
this.calcTotal();
this.select_all.push(Selector)
},
trackTime(time, index, proyecto) {
this.currentTime = time;
this.allTimes[index] = time;
const data = {
time,
proyecto
}
this.saveForm[index] = data;
},
saveHours() {
const currentWeek = moment(new Date()).format('w');
const diverRef = db.collection('divers').doc(firebaseAuth.currentUser.email);
const currentWeekRef = diverRef.collection('reportes').doc(`semana_${currentWeek}`);
var self = this;
currentWeekRef.get().then(function(doc) {
if ( doc.exists ) {
console.log('Ya registraste tus horas');
} else {
currentWeekRef.set({
data: self.saveForm
})
}
});
},
}
}
I have a component called , what I do is that when someone types the number of hours, I emit that time back to the parent and use the trackTime
function to add the time of each project to the allTimes
array.
I'm trying to use a computed property calculateTotal
to add the times together so I know when a user completed the 100% of hours. But is not updating.
Is really weird, if I use it as a method it works like a charm, but it doesn't work for me because i want it to update as the user is typing and since I'm using a component for the input I can't use keyup
events.
I've been trying to figure this out for hours now and nothing. Thanks!
javascript vue.js computed-properties
1
Your computed depends onallTimes
. You're modifying it bythis.allTimes[index] = time;
. But you cannot do that, vue reactivity breaks if you modify an array by index: vuejs.org/2016/02/06/common-gotchas/…
– Sergeon
Nov 15 '18 at 14:18
vuejs.org/v2/guide/reactivity.html#Change-Detection-Caveats
– Roy J
Nov 15 '18 at 14:47
Thank you so much!
– Saúl Solórzano
Nov 15 '18 at 15:16
add a comment |
I have a weird problem. I'm trying do to a simple time tracking app.
So I have this form.
<form class="form" @submit.prevent="saveHours">
<div class="status">
<div class="selector" v-for="(select, index) in select_all">
<component :is="select" :id="index" @percentage="trackTime"></component>
</div>
</div><!-- /.status -->
<div class="form-submit">
<button type="submit" class="form__submit">
<span v-if="loading">Guardando...</span>
<span v-else>Guardar</span>
</button>
</div>
</form>
And this is my vue code
export default {
name: 'home',
data() {
return {
select_all: [Selector],
loading: false,
allTimes: ,
saveForm:
}
},
components: {
Selector
},
computed: {
calculateTotal() {
return this.allTimes.reduce((accumulator, currentValue) => parseInt(accumulator) + parseInt(currentValue), 0);
}
},
methods: {
addNewSelector() {
this.calcTotal();
this.select_all.push(Selector)
},
trackTime(time, index, proyecto) {
this.currentTime = time;
this.allTimes[index] = time;
const data = {
time,
proyecto
}
this.saveForm[index] = data;
},
saveHours() {
const currentWeek = moment(new Date()).format('w');
const diverRef = db.collection('divers').doc(firebaseAuth.currentUser.email);
const currentWeekRef = diverRef.collection('reportes').doc(`semana_${currentWeek}`);
var self = this;
currentWeekRef.get().then(function(doc) {
if ( doc.exists ) {
console.log('Ya registraste tus horas');
} else {
currentWeekRef.set({
data: self.saveForm
})
}
});
},
}
}
I have a component called , what I do is that when someone types the number of hours, I emit that time back to the parent and use the trackTime
function to add the time of each project to the allTimes
array.
I'm trying to use a computed property calculateTotal
to add the times together so I know when a user completed the 100% of hours. But is not updating.
Is really weird, if I use it as a method it works like a charm, but it doesn't work for me because i want it to update as the user is typing and since I'm using a component for the input I can't use keyup
events.
I've been trying to figure this out for hours now and nothing. Thanks!
javascript vue.js computed-properties
I have a weird problem. I'm trying do to a simple time tracking app.
So I have this form.
<form class="form" @submit.prevent="saveHours">
<div class="status">
<div class="selector" v-for="(select, index) in select_all">
<component :is="select" :id="index" @percentage="trackTime"></component>
</div>
</div><!-- /.status -->
<div class="form-submit">
<button type="submit" class="form__submit">
<span v-if="loading">Guardando...</span>
<span v-else>Guardar</span>
</button>
</div>
</form>
And this is my vue code
export default {
name: 'home',
data() {
return {
select_all: [Selector],
loading: false,
allTimes: ,
saveForm:
}
},
components: {
Selector
},
computed: {
calculateTotal() {
return this.allTimes.reduce((accumulator, currentValue) => parseInt(accumulator) + parseInt(currentValue), 0);
}
},
methods: {
addNewSelector() {
this.calcTotal();
this.select_all.push(Selector)
},
trackTime(time, index, proyecto) {
this.currentTime = time;
this.allTimes[index] = time;
const data = {
time,
proyecto
}
this.saveForm[index] = data;
},
saveHours() {
const currentWeek = moment(new Date()).format('w');
const diverRef = db.collection('divers').doc(firebaseAuth.currentUser.email);
const currentWeekRef = diverRef.collection('reportes').doc(`semana_${currentWeek}`);
var self = this;
currentWeekRef.get().then(function(doc) {
if ( doc.exists ) {
console.log('Ya registraste tus horas');
} else {
currentWeekRef.set({
data: self.saveForm
})
}
});
},
}
}
I have a component called , what I do is that when someone types the number of hours, I emit that time back to the parent and use the trackTime
function to add the time of each project to the allTimes
array.
I'm trying to use a computed property calculateTotal
to add the times together so I know when a user completed the 100% of hours. But is not updating.
Is really weird, if I use it as a method it works like a charm, but it doesn't work for me because i want it to update as the user is typing and since I'm using a component for the input I can't use keyup
events.
I've been trying to figure this out for hours now and nothing. Thanks!
javascript vue.js computed-properties
javascript vue.js computed-properties
asked Nov 15 '18 at 14:12
Saúl SolórzanoSaúl Solórzano
53
53
1
Your computed depends onallTimes
. You're modifying it bythis.allTimes[index] = time;
. But you cannot do that, vue reactivity breaks if you modify an array by index: vuejs.org/2016/02/06/common-gotchas/…
– Sergeon
Nov 15 '18 at 14:18
vuejs.org/v2/guide/reactivity.html#Change-Detection-Caveats
– Roy J
Nov 15 '18 at 14:47
Thank you so much!
– Saúl Solórzano
Nov 15 '18 at 15:16
add a comment |
1
Your computed depends onallTimes
. You're modifying it bythis.allTimes[index] = time;
. But you cannot do that, vue reactivity breaks if you modify an array by index: vuejs.org/2016/02/06/common-gotchas/…
– Sergeon
Nov 15 '18 at 14:18
vuejs.org/v2/guide/reactivity.html#Change-Detection-Caveats
– Roy J
Nov 15 '18 at 14:47
Thank you so much!
– Saúl Solórzano
Nov 15 '18 at 15:16
1
1
Your computed depends on
allTimes
. You're modifying it by this.allTimes[index] = time;
. But you cannot do that, vue reactivity breaks if you modify an array by index: vuejs.org/2016/02/06/common-gotchas/…– Sergeon
Nov 15 '18 at 14:18
Your computed depends on
allTimes
. You're modifying it by this.allTimes[index] = time;
. But you cannot do that, vue reactivity breaks if you modify an array by index: vuejs.org/2016/02/06/common-gotchas/…– Sergeon
Nov 15 '18 at 14:18
vuejs.org/v2/guide/reactivity.html#Change-Detection-Caveats
– Roy J
Nov 15 '18 at 14:47
vuejs.org/v2/guide/reactivity.html#Change-Detection-Caveats
– Roy J
Nov 15 '18 at 14:47
Thank you so much!
– Saúl Solórzano
Nov 15 '18 at 15:16
Thank you so much!
– Saúl Solórzano
Nov 15 '18 at 15:16
add a comment |
1 Answer
1
active
oldest
votes
If anyone is having the same problem Sergeon and Roy J where right.
I was doing this
this.allTimes[index] = time;
And that was messing up Vue. I changed the code to
this.allTimes.splice(index, 1, time)
And it works perfect now.
Thanks
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53321351%2fcomputed-property-not-updating%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If anyone is having the same problem Sergeon and Roy J where right.
I was doing this
this.allTimes[index] = time;
And that was messing up Vue. I changed the code to
this.allTimes.splice(index, 1, time)
And it works perfect now.
Thanks
add a comment |
If anyone is having the same problem Sergeon and Roy J where right.
I was doing this
this.allTimes[index] = time;
And that was messing up Vue. I changed the code to
this.allTimes.splice(index, 1, time)
And it works perfect now.
Thanks
add a comment |
If anyone is having the same problem Sergeon and Roy J where right.
I was doing this
this.allTimes[index] = time;
And that was messing up Vue. I changed the code to
this.allTimes.splice(index, 1, time)
And it works perfect now.
Thanks
If anyone is having the same problem Sergeon and Roy J where right.
I was doing this
this.allTimes[index] = time;
And that was messing up Vue. I changed the code to
this.allTimes.splice(index, 1, time)
And it works perfect now.
Thanks
answered Nov 15 '18 at 15:19
Saúl SolórzanoSaúl Solórzano
53
53
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53321351%2fcomputed-property-not-updating%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
F5o kMGV,neaKaUqJE
1
Your computed depends on
allTimes
. You're modifying it bythis.allTimes[index] = time;
. But you cannot do that, vue reactivity breaks if you modify an array by index: vuejs.org/2016/02/06/common-gotchas/…– Sergeon
Nov 15 '18 at 14:18
vuejs.org/v2/guide/reactivity.html#Change-Detection-Caveats
– Roy J
Nov 15 '18 at 14:47
Thank you so much!
– Saúl Solórzano
Nov 15 '18 at 15:16