Android Time Picker In Fragment Crashing
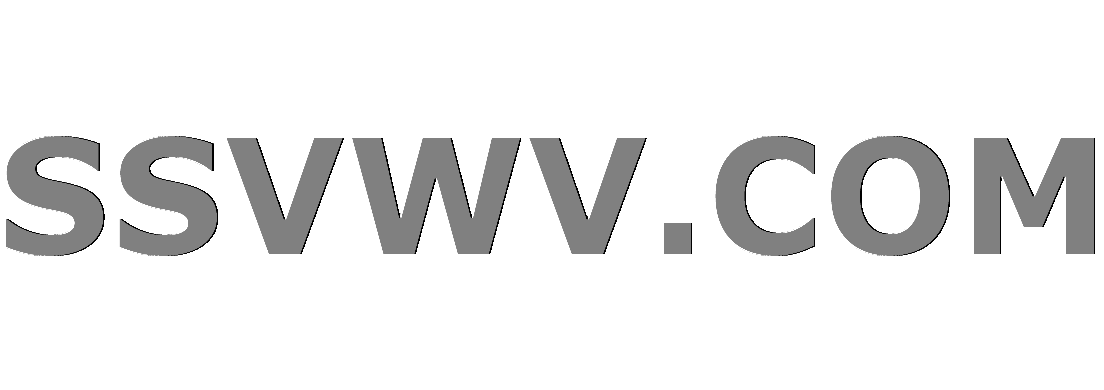
Multi tool use
I have an app that has three fragments. On the second fragment I have a time picker and I want to feed the value selected on this time picker to the main activity.
I believe there is an error in this section of code as it builds and deploys to my device, but crashes on opening.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mSectionsPagerAdapter = new SectionsPagerAdapter(getSupportFragmentManager());
// Set up the ViewPager with the sections adapter.
mViewPager = (ViewPager) findViewById(R.id.container);
mViewPager.setAdapter(mSectionsPagerAdapter);
//In debugging here is the section of code that causes the app to no longer run
timePicker1 = (TimePicker) findViewById(R.id.alarmTimePickerStart);
time = (TextView) findViewById(R.id.textView3);
calendar = Calendar.getInstance();
int hour = calendar.get(Calendar.HOUR_OF_DAY);
int min = calendar.get(Calendar.MINUTE);
showTime(hour, min);
}
public void setTime(View view) {
int hour = timePicker1.getCurrentHour();
int min = timePicker1.getCurrentMinute();
showTime(hour, min);
}
public void showTime(int hour, int min) {
if (hour == 0) {
hour += 12;
format = "AM";
} else if (hour == 12) {
format = "PM";
} else if (hour > 12) {
hour -= 12;
format = "PM";
} else {
format = "AM";
}
time.setText(new StringBuilder().append(hour).append(" : ").append(min)
.append(" ").append(format));
}
Am I putting this code in the wrong java file? I figured it would be a main activity command as the value of time picked needs to be global to the application and must be saved during termination of the app.
Here is the error log:
E/AndroidRuntime: FATAL EXCEPTION: main
Process: io.mindfulmotivation.mindfulmotivation, PID: 31814
java.lang.RuntimeException: Unable to start activity ComponentInfo{io.mindfulmotivation.mindfulmotivation/io.mindfulmotivation.mindfulmotivation.MainActivity}: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2679)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2740)
at android.app.ActivityThread.-wrap12(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1487)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6164)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:888)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:778)
Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference
at io.mindfulmotivation.mindfulmotivation.MainActivity.showTime(MainActivity.java:84)
at io.mindfulmotivation.mindfulmotivation.MainActivity.onCreate(MainActivity.java:62)
at android.app.Activity.performCreate(Activity.java:6720)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1119)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2632)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2740)
at android.app.ActivityThread.-wrap12(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1487)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6164)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:888)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:778)

|
show 7 more comments
I have an app that has three fragments. On the second fragment I have a time picker and I want to feed the value selected on this time picker to the main activity.
I believe there is an error in this section of code as it builds and deploys to my device, but crashes on opening.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mSectionsPagerAdapter = new SectionsPagerAdapter(getSupportFragmentManager());
// Set up the ViewPager with the sections adapter.
mViewPager = (ViewPager) findViewById(R.id.container);
mViewPager.setAdapter(mSectionsPagerAdapter);
//In debugging here is the section of code that causes the app to no longer run
timePicker1 = (TimePicker) findViewById(R.id.alarmTimePickerStart);
time = (TextView) findViewById(R.id.textView3);
calendar = Calendar.getInstance();
int hour = calendar.get(Calendar.HOUR_OF_DAY);
int min = calendar.get(Calendar.MINUTE);
showTime(hour, min);
}
public void setTime(View view) {
int hour = timePicker1.getCurrentHour();
int min = timePicker1.getCurrentMinute();
showTime(hour, min);
}
public void showTime(int hour, int min) {
if (hour == 0) {
hour += 12;
format = "AM";
} else if (hour == 12) {
format = "PM";
} else if (hour > 12) {
hour -= 12;
format = "PM";
} else {
format = "AM";
}
time.setText(new StringBuilder().append(hour).append(" : ").append(min)
.append(" ").append(format));
}
Am I putting this code in the wrong java file? I figured it would be a main activity command as the value of time picked needs to be global to the application and must be saved during termination of the app.
Here is the error log:
E/AndroidRuntime: FATAL EXCEPTION: main
Process: io.mindfulmotivation.mindfulmotivation, PID: 31814
java.lang.RuntimeException: Unable to start activity ComponentInfo{io.mindfulmotivation.mindfulmotivation/io.mindfulmotivation.mindfulmotivation.MainActivity}: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2679)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2740)
at android.app.ActivityThread.-wrap12(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1487)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6164)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:888)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:778)
Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference
at io.mindfulmotivation.mindfulmotivation.MainActivity.showTime(MainActivity.java:84)
at io.mindfulmotivation.mindfulmotivation.MainActivity.onCreate(MainActivity.java:62)
at android.app.Activity.performCreate(Activity.java:6720)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1119)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2632)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2740)
at android.app.ActivityThread.-wrap12(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1487)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6164)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:888)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:778)

2
add logcat pls..
– Basi
Nov 16 '18 at 5:05
Post your error log
– Piyush
Nov 16 '18 at 5:06
1
If theTimePicker
is in aFragment
, you should only be directly handling it in theFragment
, and delivering its data to theActivity
through some other mechanism; e.g., aninterface
. Since not only is it in aFragment
, but also thatFragment
is inside aViewPager
, theTimePicker
is definitely not going to created and attached to theActivity
's hierarchy by the time theActivity
'sonCreate()
finishes, and thefindViewById()
for it will return null there.
– Mike M.
Nov 16 '18 at 5:08
If your time picker is in fragment then you need to changefindViewById
withview.findViewById
.
– Piyush
Nov 16 '18 at 5:08
1
The Exception is happening at thetime.setText()
call. If thatTextView
is in the sameFragment
as theTimePicker
, the same thing as I mentioned above applies: you should be handling it in theFragment
, not directly in theActivity
.
– Mike M.
Nov 16 '18 at 5:21
|
show 7 more comments
I have an app that has three fragments. On the second fragment I have a time picker and I want to feed the value selected on this time picker to the main activity.
I believe there is an error in this section of code as it builds and deploys to my device, but crashes on opening.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mSectionsPagerAdapter = new SectionsPagerAdapter(getSupportFragmentManager());
// Set up the ViewPager with the sections adapter.
mViewPager = (ViewPager) findViewById(R.id.container);
mViewPager.setAdapter(mSectionsPagerAdapter);
//In debugging here is the section of code that causes the app to no longer run
timePicker1 = (TimePicker) findViewById(R.id.alarmTimePickerStart);
time = (TextView) findViewById(R.id.textView3);
calendar = Calendar.getInstance();
int hour = calendar.get(Calendar.HOUR_OF_DAY);
int min = calendar.get(Calendar.MINUTE);
showTime(hour, min);
}
public void setTime(View view) {
int hour = timePicker1.getCurrentHour();
int min = timePicker1.getCurrentMinute();
showTime(hour, min);
}
public void showTime(int hour, int min) {
if (hour == 0) {
hour += 12;
format = "AM";
} else if (hour == 12) {
format = "PM";
} else if (hour > 12) {
hour -= 12;
format = "PM";
} else {
format = "AM";
}
time.setText(new StringBuilder().append(hour).append(" : ").append(min)
.append(" ").append(format));
}
Am I putting this code in the wrong java file? I figured it would be a main activity command as the value of time picked needs to be global to the application and must be saved during termination of the app.
Here is the error log:
E/AndroidRuntime: FATAL EXCEPTION: main
Process: io.mindfulmotivation.mindfulmotivation, PID: 31814
java.lang.RuntimeException: Unable to start activity ComponentInfo{io.mindfulmotivation.mindfulmotivation/io.mindfulmotivation.mindfulmotivation.MainActivity}: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2679)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2740)
at android.app.ActivityThread.-wrap12(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1487)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6164)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:888)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:778)
Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference
at io.mindfulmotivation.mindfulmotivation.MainActivity.showTime(MainActivity.java:84)
at io.mindfulmotivation.mindfulmotivation.MainActivity.onCreate(MainActivity.java:62)
at android.app.Activity.performCreate(Activity.java:6720)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1119)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2632)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2740)
at android.app.ActivityThread.-wrap12(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1487)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6164)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:888)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:778)

I have an app that has three fragments. On the second fragment I have a time picker and I want to feed the value selected on this time picker to the main activity.
I believe there is an error in this section of code as it builds and deploys to my device, but crashes on opening.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mSectionsPagerAdapter = new SectionsPagerAdapter(getSupportFragmentManager());
// Set up the ViewPager with the sections adapter.
mViewPager = (ViewPager) findViewById(R.id.container);
mViewPager.setAdapter(mSectionsPagerAdapter);
//In debugging here is the section of code that causes the app to no longer run
timePicker1 = (TimePicker) findViewById(R.id.alarmTimePickerStart);
time = (TextView) findViewById(R.id.textView3);
calendar = Calendar.getInstance();
int hour = calendar.get(Calendar.HOUR_OF_DAY);
int min = calendar.get(Calendar.MINUTE);
showTime(hour, min);
}
public void setTime(View view) {
int hour = timePicker1.getCurrentHour();
int min = timePicker1.getCurrentMinute();
showTime(hour, min);
}
public void showTime(int hour, int min) {
if (hour == 0) {
hour += 12;
format = "AM";
} else if (hour == 12) {
format = "PM";
} else if (hour > 12) {
hour -= 12;
format = "PM";
} else {
format = "AM";
}
time.setText(new StringBuilder().append(hour).append(" : ").append(min)
.append(" ").append(format));
}
Am I putting this code in the wrong java file? I figured it would be a main activity command as the value of time picked needs to be global to the application and must be saved during termination of the app.
Here is the error log:
E/AndroidRuntime: FATAL EXCEPTION: main
Process: io.mindfulmotivation.mindfulmotivation, PID: 31814
java.lang.RuntimeException: Unable to start activity ComponentInfo{io.mindfulmotivation.mindfulmotivation/io.mindfulmotivation.mindfulmotivation.MainActivity}: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2679)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2740)
at android.app.ActivityThread.-wrap12(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1487)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6164)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:888)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:778)
Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference
at io.mindfulmotivation.mindfulmotivation.MainActivity.showTime(MainActivity.java:84)
at io.mindfulmotivation.mindfulmotivation.MainActivity.onCreate(MainActivity.java:62)
at android.app.Activity.performCreate(Activity.java:6720)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1119)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2632)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2740)
at android.app.ActivityThread.-wrap12(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1487)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6164)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:888)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:778)


edited Nov 16 '18 at 6:36


Milind Mevada
1,615918
1,615918
asked Nov 16 '18 at 5:03
Joseph P NardoneJoseph P Nardone
367
367
2
add logcat pls..
– Basi
Nov 16 '18 at 5:05
Post your error log
– Piyush
Nov 16 '18 at 5:06
1
If theTimePicker
is in aFragment
, you should only be directly handling it in theFragment
, and delivering its data to theActivity
through some other mechanism; e.g., aninterface
. Since not only is it in aFragment
, but also thatFragment
is inside aViewPager
, theTimePicker
is definitely not going to created and attached to theActivity
's hierarchy by the time theActivity
'sonCreate()
finishes, and thefindViewById()
for it will return null there.
– Mike M.
Nov 16 '18 at 5:08
If your time picker is in fragment then you need to changefindViewById
withview.findViewById
.
– Piyush
Nov 16 '18 at 5:08
1
The Exception is happening at thetime.setText()
call. If thatTextView
is in the sameFragment
as theTimePicker
, the same thing as I mentioned above applies: you should be handling it in theFragment
, not directly in theActivity
.
– Mike M.
Nov 16 '18 at 5:21
|
show 7 more comments
2
add logcat pls..
– Basi
Nov 16 '18 at 5:05
Post your error log
– Piyush
Nov 16 '18 at 5:06
1
If theTimePicker
is in aFragment
, you should only be directly handling it in theFragment
, and delivering its data to theActivity
through some other mechanism; e.g., aninterface
. Since not only is it in aFragment
, but also thatFragment
is inside aViewPager
, theTimePicker
is definitely not going to created and attached to theActivity
's hierarchy by the time theActivity
'sonCreate()
finishes, and thefindViewById()
for it will return null there.
– Mike M.
Nov 16 '18 at 5:08
If your time picker is in fragment then you need to changefindViewById
withview.findViewById
.
– Piyush
Nov 16 '18 at 5:08
1
The Exception is happening at thetime.setText()
call. If thatTextView
is in the sameFragment
as theTimePicker
, the same thing as I mentioned above applies: you should be handling it in theFragment
, not directly in theActivity
.
– Mike M.
Nov 16 '18 at 5:21
2
2
add logcat pls..
– Basi
Nov 16 '18 at 5:05
add logcat pls..
– Basi
Nov 16 '18 at 5:05
Post your error log
– Piyush
Nov 16 '18 at 5:06
Post your error log
– Piyush
Nov 16 '18 at 5:06
1
1
If the
TimePicker
is in a Fragment
, you should only be directly handling it in the Fragment
, and delivering its data to the Activity
through some other mechanism; e.g., an interface
. Since not only is it in a Fragment
, but also that Fragment
is inside a ViewPager
, the TimePicker
is definitely not going to created and attached to the Activity
's hierarchy by the time the Activity
's onCreate()
finishes, and the findViewById()
for it will return null there.– Mike M.
Nov 16 '18 at 5:08
If the
TimePicker
is in a Fragment
, you should only be directly handling it in the Fragment
, and delivering its data to the Activity
through some other mechanism; e.g., an interface
. Since not only is it in a Fragment
, but also that Fragment
is inside a ViewPager
, the TimePicker
is definitely not going to created and attached to the Activity
's hierarchy by the time the Activity
's onCreate()
finishes, and the findViewById()
for it will return null there.– Mike M.
Nov 16 '18 at 5:08
If your time picker is in fragment then you need to change
findViewById
with view.findViewById
.– Piyush
Nov 16 '18 at 5:08
If your time picker is in fragment then you need to change
findViewById
with view.findViewById
.– Piyush
Nov 16 '18 at 5:08
1
1
The Exception is happening at the
time.setText()
call. If that TextView
is in the same Fragment
as the TimePicker
, the same thing as I mentioned above applies: you should be handling it in the Fragment
, not directly in the Activity
.– Mike M.
Nov 16 '18 at 5:21
The Exception is happening at the
time.setText()
call. If that TextView
is in the same Fragment
as the TimePicker
, the same thing as I mentioned above applies: you should be handling it in the Fragment
, not directly in the Activity
.– Mike M.
Nov 16 '18 at 5:21
|
show 7 more comments
1 Answer
1
active
oldest
votes
This is happening because you are trying to set text in a TextView which is not in the MainActivity.
time = (TextView) findViewById(R.id.textView3);
And
time.setText(new StringBuilder().append(hour).append(" : ").append(min)
.append(" ").append(format));
Make sure R.id.textView3 is in the MainActivity, not in any Fragment.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53331738%2fandroid-time-picker-in-fragment-crashing%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This is happening because you are trying to set text in a TextView which is not in the MainActivity.
time = (TextView) findViewById(R.id.textView3);
And
time.setText(new StringBuilder().append(hour).append(" : ").append(min)
.append(" ").append(format));
Make sure R.id.textView3 is in the MainActivity, not in any Fragment.
add a comment |
This is happening because you are trying to set text in a TextView which is not in the MainActivity.
time = (TextView) findViewById(R.id.textView3);
And
time.setText(new StringBuilder().append(hour).append(" : ").append(min)
.append(" ").append(format));
Make sure R.id.textView3 is in the MainActivity, not in any Fragment.
add a comment |
This is happening because you are trying to set text in a TextView which is not in the MainActivity.
time = (TextView) findViewById(R.id.textView3);
And
time.setText(new StringBuilder().append(hour).append(" : ").append(min)
.append(" ").append(format));
Make sure R.id.textView3 is in the MainActivity, not in any Fragment.
This is happening because you are trying to set text in a TextView which is not in the MainActivity.
time = (TextView) findViewById(R.id.textView3);
And
time.setText(new StringBuilder().append(hour).append(" : ").append(min)
.append(" ").append(format));
Make sure R.id.textView3 is in the MainActivity, not in any Fragment.
answered Nov 16 '18 at 5:49


Birju VachhaniBirju Vachhani
359113
359113
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53331738%2fandroid-time-picker-in-fragment-crashing%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vZstRrE8G fmqRb qNC,gSxT84xBFTSClp39hd6Ki9Io0LDcvYJSVnx hq
2
add logcat pls..
– Basi
Nov 16 '18 at 5:05
Post your error log
– Piyush
Nov 16 '18 at 5:06
1
If the
TimePicker
is in aFragment
, you should only be directly handling it in theFragment
, and delivering its data to theActivity
through some other mechanism; e.g., aninterface
. Since not only is it in aFragment
, but also thatFragment
is inside aViewPager
, theTimePicker
is definitely not going to created and attached to theActivity
's hierarchy by the time theActivity
'sonCreate()
finishes, and thefindViewById()
for it will return null there.– Mike M.
Nov 16 '18 at 5:08
If your time picker is in fragment then you need to change
findViewById
withview.findViewById
.– Piyush
Nov 16 '18 at 5:08
1
The Exception is happening at the
time.setText()
call. If thatTextView
is in the sameFragment
as theTimePicker
, the same thing as I mentioned above applies: you should be handling it in theFragment
, not directly in theActivity
.– Mike M.
Nov 16 '18 at 5:21