Looping through the values of an array intersect
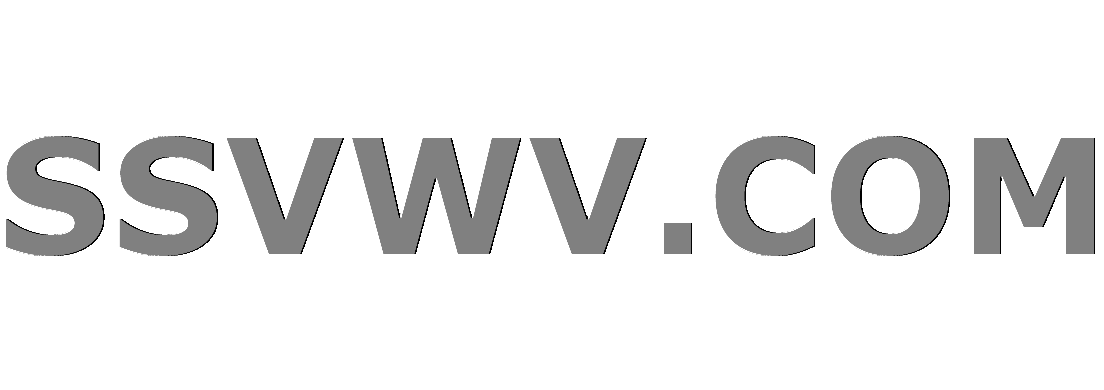
Multi tool use
I have different communities in my database, such as: community_newsfeed, community_marketplace, community_games_requested, community_games_offered, events and custom_posts.
Each of those communities stores a post_id, as well as the user_id.
Then I have a community_users table that stores user_id and community_id.
I want to create a mechanism which I, as the authenticated user, can view all the posts of a user, as long as that user and I belong to the same community.
And on that regard, I must view only the posts for the communities which we both belong to.
So I do the following:
Get all the community id's of the communities which I(auth user) belong to:
$community_user_member = DB::table('community_users')->whereUserId($user->id)->pluck('community_id')->toArray();
Then I do the same for the user which I want to see the posts:
$requested_user = DB::table('community_users')->whereUserId($user_id)->pluck('community_id')->toArray();
Fine. Now I use array interect to give me all the community_id that we share only:
$members = array_intersect($community_user_member, $requested_user);
Now, this is where I get stuck.
How can I get the communities which the id match the values I get in the $members value(not the key) and the user id matches $user_id( this is passed in the url )?
if(!empty($members)){
$newsfeed = CommunityNewsfeed::whereIn('newsfeed_community_id', $members)->whereUserId($user_id)->get();
}
I am sure that this user has 4 posts from community_newsfeed table, but I only see two posts, and also I dont see the correct posts.
Live example:
User A belongs to community_newsfeed.
User B belongs to community_newsfeed and community_marketplace.
User A must see the community_newsfeed data where user_id is the id of User B.
Updated
Another option I tried was to use the in array function:
$common = ;
foreach ($community_user_member as $key => $member) {
if (in_array($member, $requested_user)) {
$common = $member;
}
}
print_r($common);
The end result should be something like this:
$common = [ '4','5','10','20' ];
Because then I would be able to loop through $common array and do whatever I want.
But I get the following error when I refresh the page:
SyntaxError: JSON.parse: unexpected character at line 1 column 1 of the JSON data
php arrays laravel
add a comment |
I have different communities in my database, such as: community_newsfeed, community_marketplace, community_games_requested, community_games_offered, events and custom_posts.
Each of those communities stores a post_id, as well as the user_id.
Then I have a community_users table that stores user_id and community_id.
I want to create a mechanism which I, as the authenticated user, can view all the posts of a user, as long as that user and I belong to the same community.
And on that regard, I must view only the posts for the communities which we both belong to.
So I do the following:
Get all the community id's of the communities which I(auth user) belong to:
$community_user_member = DB::table('community_users')->whereUserId($user->id)->pluck('community_id')->toArray();
Then I do the same for the user which I want to see the posts:
$requested_user = DB::table('community_users')->whereUserId($user_id)->pluck('community_id')->toArray();
Fine. Now I use array interect to give me all the community_id that we share only:
$members = array_intersect($community_user_member, $requested_user);
Now, this is where I get stuck.
How can I get the communities which the id match the values I get in the $members value(not the key) and the user id matches $user_id( this is passed in the url )?
if(!empty($members)){
$newsfeed = CommunityNewsfeed::whereIn('newsfeed_community_id', $members)->whereUserId($user_id)->get();
}
I am sure that this user has 4 posts from community_newsfeed table, but I only see two posts, and also I dont see the correct posts.
Live example:
User A belongs to community_newsfeed.
User B belongs to community_newsfeed and community_marketplace.
User A must see the community_newsfeed data where user_id is the id of User B.
Updated
Another option I tried was to use the in array function:
$common = ;
foreach ($community_user_member as $key => $member) {
if (in_array($member, $requested_user)) {
$common = $member;
}
}
print_r($common);
The end result should be something like this:
$common = [ '4','5','10','20' ];
Because then I would be able to loop through $common array and do whatever I want.
But I get the following error when I refresh the page:
SyntaxError: JSON.parse: unexpected character at line 1 column 1 of the JSON data
php arrays laravel
Tryarray_values($members)
but I don't think that's the issue check if$user_id
is correct or not
– Viney
Nov 14 '18 at 15:43
@Vineyif I get at array of key value pairs. Something like this: 0: 229 1: 246 2: 248 3: 234 Now, Is there a way I can get an array of values only? Not sure if am confusing myself
– deSousa
Nov 14 '18 at 15:59
Php array will always have a key bound with the values so there is not such thing as "array of values".So even when declaring array like$a = array(1,2,3);
php internally creates it as$a = array(0=>1,1=>2,2=>3);
, yes it's one of those quirky parts
– Viney
Nov 14 '18 at 16:05
add a comment |
I have different communities in my database, such as: community_newsfeed, community_marketplace, community_games_requested, community_games_offered, events and custom_posts.
Each of those communities stores a post_id, as well as the user_id.
Then I have a community_users table that stores user_id and community_id.
I want to create a mechanism which I, as the authenticated user, can view all the posts of a user, as long as that user and I belong to the same community.
And on that regard, I must view only the posts for the communities which we both belong to.
So I do the following:
Get all the community id's of the communities which I(auth user) belong to:
$community_user_member = DB::table('community_users')->whereUserId($user->id)->pluck('community_id')->toArray();
Then I do the same for the user which I want to see the posts:
$requested_user = DB::table('community_users')->whereUserId($user_id)->pluck('community_id')->toArray();
Fine. Now I use array interect to give me all the community_id that we share only:
$members = array_intersect($community_user_member, $requested_user);
Now, this is where I get stuck.
How can I get the communities which the id match the values I get in the $members value(not the key) and the user id matches $user_id( this is passed in the url )?
if(!empty($members)){
$newsfeed = CommunityNewsfeed::whereIn('newsfeed_community_id', $members)->whereUserId($user_id)->get();
}
I am sure that this user has 4 posts from community_newsfeed table, but I only see two posts, and also I dont see the correct posts.
Live example:
User A belongs to community_newsfeed.
User B belongs to community_newsfeed and community_marketplace.
User A must see the community_newsfeed data where user_id is the id of User B.
Updated
Another option I tried was to use the in array function:
$common = ;
foreach ($community_user_member as $key => $member) {
if (in_array($member, $requested_user)) {
$common = $member;
}
}
print_r($common);
The end result should be something like this:
$common = [ '4','5','10','20' ];
Because then I would be able to loop through $common array and do whatever I want.
But I get the following error when I refresh the page:
SyntaxError: JSON.parse: unexpected character at line 1 column 1 of the JSON data
php arrays laravel
I have different communities in my database, such as: community_newsfeed, community_marketplace, community_games_requested, community_games_offered, events and custom_posts.
Each of those communities stores a post_id, as well as the user_id.
Then I have a community_users table that stores user_id and community_id.
I want to create a mechanism which I, as the authenticated user, can view all the posts of a user, as long as that user and I belong to the same community.
And on that regard, I must view only the posts for the communities which we both belong to.
So I do the following:
Get all the community id's of the communities which I(auth user) belong to:
$community_user_member = DB::table('community_users')->whereUserId($user->id)->pluck('community_id')->toArray();
Then I do the same for the user which I want to see the posts:
$requested_user = DB::table('community_users')->whereUserId($user_id)->pluck('community_id')->toArray();
Fine. Now I use array interect to give me all the community_id that we share only:
$members = array_intersect($community_user_member, $requested_user);
Now, this is where I get stuck.
How can I get the communities which the id match the values I get in the $members value(not the key) and the user id matches $user_id( this is passed in the url )?
if(!empty($members)){
$newsfeed = CommunityNewsfeed::whereIn('newsfeed_community_id', $members)->whereUserId($user_id)->get();
}
I am sure that this user has 4 posts from community_newsfeed table, but I only see two posts, and also I dont see the correct posts.
Live example:
User A belongs to community_newsfeed.
User B belongs to community_newsfeed and community_marketplace.
User A must see the community_newsfeed data where user_id is the id of User B.
Updated
Another option I tried was to use the in array function:
$common = ;
foreach ($community_user_member as $key => $member) {
if (in_array($member, $requested_user)) {
$common = $member;
}
}
print_r($common);
The end result should be something like this:
$common = [ '4','5','10','20' ];
Because then I would be able to loop through $common array and do whatever I want.
But I get the following error when I refresh the page:
SyntaxError: JSON.parse: unexpected character at line 1 column 1 of the JSON data
php arrays laravel
php arrays laravel
edited Dec 7 '18 at 22:57


halfer
14.5k758112
14.5k758112
asked Nov 14 '18 at 14:31
deSousadeSousa
1,08121135
1,08121135
Tryarray_values($members)
but I don't think that's the issue check if$user_id
is correct or not
– Viney
Nov 14 '18 at 15:43
@Vineyif I get at array of key value pairs. Something like this: 0: 229 1: 246 2: 248 3: 234 Now, Is there a way I can get an array of values only? Not sure if am confusing myself
– deSousa
Nov 14 '18 at 15:59
Php array will always have a key bound with the values so there is not such thing as "array of values".So even when declaring array like$a = array(1,2,3);
php internally creates it as$a = array(0=>1,1=>2,2=>3);
, yes it's one of those quirky parts
– Viney
Nov 14 '18 at 16:05
add a comment |
Tryarray_values($members)
but I don't think that's the issue check if$user_id
is correct or not
– Viney
Nov 14 '18 at 15:43
@Vineyif I get at array of key value pairs. Something like this: 0: 229 1: 246 2: 248 3: 234 Now, Is there a way I can get an array of values only? Not sure if am confusing myself
– deSousa
Nov 14 '18 at 15:59
Php array will always have a key bound with the values so there is not such thing as "array of values".So even when declaring array like$a = array(1,2,3);
php internally creates it as$a = array(0=>1,1=>2,2=>3);
, yes it's one of those quirky parts
– Viney
Nov 14 '18 at 16:05
Try
array_values($members)
but I don't think that's the issue check if $user_id
is correct or not– Viney
Nov 14 '18 at 15:43
Try
array_values($members)
but I don't think that's the issue check if $user_id
is correct or not– Viney
Nov 14 '18 at 15:43
@Vineyif I get at array of key value pairs. Something like this: 0: 229 1: 246 2: 248 3: 234 Now, Is there a way I can get an array of values only? Not sure if am confusing myself
– deSousa
Nov 14 '18 at 15:59
@Vineyif I get at array of key value pairs. Something like this: 0: 229 1: 246 2: 248 3: 234 Now, Is there a way I can get an array of values only? Not sure if am confusing myself
– deSousa
Nov 14 '18 at 15:59
Php array will always have a key bound with the values so there is not such thing as "array of values".So even when declaring array like
$a = array(1,2,3);
php internally creates it as $a = array(0=>1,1=>2,2=>3);
, yes it's one of those quirky parts– Viney
Nov 14 '18 at 16:05
Php array will always have a key bound with the values so there is not such thing as "array of values".So even when declaring array like
$a = array(1,2,3);
php internally creates it as $a = array(0=>1,1=>2,2=>3);
, yes it's one of those quirky parts– Viney
Nov 14 '18 at 16:05
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53302586%2flooping-through-the-values-of-an-array-intersect%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53302586%2flooping-through-the-values-of-an-array-intersect%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
scBk,Qn6hNLGgrHK,VpaZmBPkvpFRdTixnkw88Z,ioRjkNQh6Yve 4,4wmA9,F8VoTa1IOdialQAC g,28 Anf
Try
array_values($members)
but I don't think that's the issue check if$user_id
is correct or not– Viney
Nov 14 '18 at 15:43
@Vineyif I get at array of key value pairs. Something like this: 0: 229 1: 246 2: 248 3: 234 Now, Is there a way I can get an array of values only? Not sure if am confusing myself
– deSousa
Nov 14 '18 at 15:59
Php array will always have a key bound with the values so there is not such thing as "array of values".So even when declaring array like
$a = array(1,2,3);
php internally creates it as$a = array(0=>1,1=>2,2=>3);
, yes it's one of those quirky parts– Viney
Nov 14 '18 at 16:05