How is a CRC32 checksum calculated with FreePascal(Lazarus)?
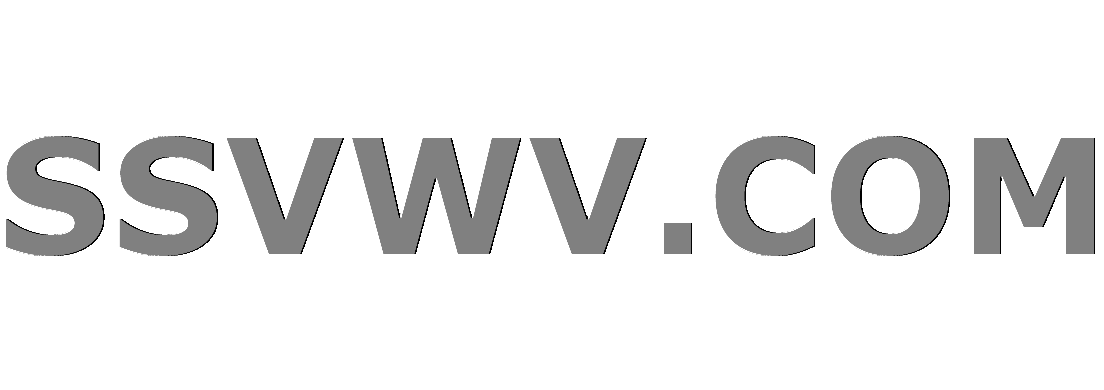
Multi tool use
I have to make some project for my college, and I need to calculate CRC32. But I almost didn't work with shifts before, so even after I read theory it's still hard to me. I found some CRC32 basic algorithm for C (not mine) and I tried to rewrite it for Lazarus(Delphi). But it doesn't work. I can't understand, what's wrong. Please, help (*_ _)人
Here my code:
procedure TMyFrame.CRC32_Checksum();
var
P : Pointer;
Size, i : Integer;
CRC, j : LongWord;
B : ^Byte;
flag : Boolean;
begin
AssignFile (f, FileName);
Reset(f, 1);
Size := FileSize(f);
GetMem(P, Size);
BlockRead(f, P^, Size);
B := P;
//
//
CRC := $FFFFFFFF;
for i := 1 to Size do
begin
CRC := CRC XOR B^;
Inc(B);
for j := 0 to 7 do
begin
flag := (CRC AND 1) > 0;
if flag then
CRC := (CRC SHR 1) XOR $04C11DB7
else
CRC := CRC SHR 1;
end;
end;
LabeledEdit1.Text := IntToHEX(CRC, 8);
//
//
Freemem(P);
CloseFile(f);
end;
lazarus crc32
|
show 2 more comments
I have to make some project for my college, and I need to calculate CRC32. But I almost didn't work with shifts before, so even after I read theory it's still hard to me. I found some CRC32 basic algorithm for C (not mine) and I tried to rewrite it for Lazarus(Delphi). But it doesn't work. I can't understand, what's wrong. Please, help (*_ _)人
Here my code:
procedure TMyFrame.CRC32_Checksum();
var
P : Pointer;
Size, i : Integer;
CRC, j : LongWord;
B : ^Byte;
flag : Boolean;
begin
AssignFile (f, FileName);
Reset(f, 1);
Size := FileSize(f);
GetMem(P, Size);
BlockRead(f, P^, Size);
B := P;
//
//
CRC := $FFFFFFFF;
for i := 1 to Size do
begin
CRC := CRC XOR B^;
Inc(B);
for j := 0 to 7 do
begin
flag := (CRC AND 1) > 0;
if flag then
CRC := (CRC SHR 1) XOR $04C11DB7
else
CRC := CRC SHR 1;
end;
end;
LabeledEdit1.Text := IntToHEX(CRC, 8);
//
//
Freemem(P);
CloseFile(f);
end;
lazarus crc32
We don't know what it is meant to do, and how it fails. You need to learn how to debug your program. That's your next task.
– David Heffernan
Nov 14 '18 at 14:47
my program just looking for checksum and finds wrong numbers. It checks .txt file where "123456789" and it finds "0x340BC6D9", but it must be "0xCBF43926". I already tried to debug as you said, but I have no idea what's problem here. That's why I asking here about my problem
– Claire
Nov 14 '18 at 15:17
There are many variants of CRC32 algorithm. Who knows which one you want? Presumably you do. You have working C code, and now want Pascal code. Compare the execution of the two versions (Pascal against C). Find out where the two diverge. Fix, and repeat. When there is no more divergence, the task is done. Once again, your next task it to learn how to debug. This is the perfect opportunity. Would you like to learn how to debug? Or do you just want code that works?
– David Heffernan
Nov 14 '18 at 15:20
I'd like to learn how to debug, I guess... okay then, I'll try once again
– Claire
Nov 14 '18 at 15:29
Just do what I say. Compare the execution of the two programs, the C version and the Pascal version. Output (e.g. to console) the value ofCRC
each time around the loop and compare the outputs of those two programs. Take it from there.
– David Heffernan
Nov 14 '18 at 15:32
|
show 2 more comments
I have to make some project for my college, and I need to calculate CRC32. But I almost didn't work with shifts before, so even after I read theory it's still hard to me. I found some CRC32 basic algorithm for C (not mine) and I tried to rewrite it for Lazarus(Delphi). But it doesn't work. I can't understand, what's wrong. Please, help (*_ _)人
Here my code:
procedure TMyFrame.CRC32_Checksum();
var
P : Pointer;
Size, i : Integer;
CRC, j : LongWord;
B : ^Byte;
flag : Boolean;
begin
AssignFile (f, FileName);
Reset(f, 1);
Size := FileSize(f);
GetMem(P, Size);
BlockRead(f, P^, Size);
B := P;
//
//
CRC := $FFFFFFFF;
for i := 1 to Size do
begin
CRC := CRC XOR B^;
Inc(B);
for j := 0 to 7 do
begin
flag := (CRC AND 1) > 0;
if flag then
CRC := (CRC SHR 1) XOR $04C11DB7
else
CRC := CRC SHR 1;
end;
end;
LabeledEdit1.Text := IntToHEX(CRC, 8);
//
//
Freemem(P);
CloseFile(f);
end;
lazarus crc32
I have to make some project for my college, and I need to calculate CRC32. But I almost didn't work with shifts before, so even after I read theory it's still hard to me. I found some CRC32 basic algorithm for C (not mine) and I tried to rewrite it for Lazarus(Delphi). But it doesn't work. I can't understand, what's wrong. Please, help (*_ _)人
Here my code:
procedure TMyFrame.CRC32_Checksum();
var
P : Pointer;
Size, i : Integer;
CRC, j : LongWord;
B : ^Byte;
flag : Boolean;
begin
AssignFile (f, FileName);
Reset(f, 1);
Size := FileSize(f);
GetMem(P, Size);
BlockRead(f, P^, Size);
B := P;
//
//
CRC := $FFFFFFFF;
for i := 1 to Size do
begin
CRC := CRC XOR B^;
Inc(B);
for j := 0 to 7 do
begin
flag := (CRC AND 1) > 0;
if flag then
CRC := (CRC SHR 1) XOR $04C11DB7
else
CRC := CRC SHR 1;
end;
end;
LabeledEdit1.Text := IntToHEX(CRC, 8);
//
//
Freemem(P);
CloseFile(f);
end;
lazarus crc32
lazarus crc32
edited Nov 14 '18 at 15:19
Claire
asked Nov 14 '18 at 14:32


ClaireClaire
112
112
We don't know what it is meant to do, and how it fails. You need to learn how to debug your program. That's your next task.
– David Heffernan
Nov 14 '18 at 14:47
my program just looking for checksum and finds wrong numbers. It checks .txt file where "123456789" and it finds "0x340BC6D9", but it must be "0xCBF43926". I already tried to debug as you said, but I have no idea what's problem here. That's why I asking here about my problem
– Claire
Nov 14 '18 at 15:17
There are many variants of CRC32 algorithm. Who knows which one you want? Presumably you do. You have working C code, and now want Pascal code. Compare the execution of the two versions (Pascal against C). Find out where the two diverge. Fix, and repeat. When there is no more divergence, the task is done. Once again, your next task it to learn how to debug. This is the perfect opportunity. Would you like to learn how to debug? Or do you just want code that works?
– David Heffernan
Nov 14 '18 at 15:20
I'd like to learn how to debug, I guess... okay then, I'll try once again
– Claire
Nov 14 '18 at 15:29
Just do what I say. Compare the execution of the two programs, the C version and the Pascal version. Output (e.g. to console) the value ofCRC
each time around the loop and compare the outputs of those two programs. Take it from there.
– David Heffernan
Nov 14 '18 at 15:32
|
show 2 more comments
We don't know what it is meant to do, and how it fails. You need to learn how to debug your program. That's your next task.
– David Heffernan
Nov 14 '18 at 14:47
my program just looking for checksum and finds wrong numbers. It checks .txt file where "123456789" and it finds "0x340BC6D9", but it must be "0xCBF43926". I already tried to debug as you said, but I have no idea what's problem here. That's why I asking here about my problem
– Claire
Nov 14 '18 at 15:17
There are many variants of CRC32 algorithm. Who knows which one you want? Presumably you do. You have working C code, and now want Pascal code. Compare the execution of the two versions (Pascal against C). Find out where the two diverge. Fix, and repeat. When there is no more divergence, the task is done. Once again, your next task it to learn how to debug. This is the perfect opportunity. Would you like to learn how to debug? Or do you just want code that works?
– David Heffernan
Nov 14 '18 at 15:20
I'd like to learn how to debug, I guess... okay then, I'll try once again
– Claire
Nov 14 '18 at 15:29
Just do what I say. Compare the execution of the two programs, the C version and the Pascal version. Output (e.g. to console) the value ofCRC
each time around the loop and compare the outputs of those two programs. Take it from there.
– David Heffernan
Nov 14 '18 at 15:32
We don't know what it is meant to do, and how it fails. You need to learn how to debug your program. That's your next task.
– David Heffernan
Nov 14 '18 at 14:47
We don't know what it is meant to do, and how it fails. You need to learn how to debug your program. That's your next task.
– David Heffernan
Nov 14 '18 at 14:47
my program just looking for checksum and finds wrong numbers. It checks .txt file where "123456789" and it finds "0x340BC6D9", but it must be "0xCBF43926". I already tried to debug as you said, but I have no idea what's problem here. That's why I asking here about my problem
– Claire
Nov 14 '18 at 15:17
my program just looking for checksum and finds wrong numbers. It checks .txt file where "123456789" and it finds "0x340BC6D9", but it must be "0xCBF43926". I already tried to debug as you said, but I have no idea what's problem here. That's why I asking here about my problem
– Claire
Nov 14 '18 at 15:17
There are many variants of CRC32 algorithm. Who knows which one you want? Presumably you do. You have working C code, and now want Pascal code. Compare the execution of the two versions (Pascal against C). Find out where the two diverge. Fix, and repeat. When there is no more divergence, the task is done. Once again, your next task it to learn how to debug. This is the perfect opportunity. Would you like to learn how to debug? Or do you just want code that works?
– David Heffernan
Nov 14 '18 at 15:20
There are many variants of CRC32 algorithm. Who knows which one you want? Presumably you do. You have working C code, and now want Pascal code. Compare the execution of the two versions (Pascal against C). Find out where the two diverge. Fix, and repeat. When there is no more divergence, the task is done. Once again, your next task it to learn how to debug. This is the perfect opportunity. Would you like to learn how to debug? Or do you just want code that works?
– David Heffernan
Nov 14 '18 at 15:20
I'd like to learn how to debug, I guess... okay then, I'll try once again
– Claire
Nov 14 '18 at 15:29
I'd like to learn how to debug, I guess... okay then, I'll try once again
– Claire
Nov 14 '18 at 15:29
Just do what I say. Compare the execution of the two programs, the C version and the Pascal version. Output (e.g. to console) the value of
CRC
each time around the loop and compare the outputs of those two programs. Take it from there.– David Heffernan
Nov 14 '18 at 15:32
Just do what I say. Compare the execution of the two programs, the C version and the Pascal version. Output (e.g. to console) the value of
CRC
each time around the loop and compare the outputs of those two programs. Take it from there.– David Heffernan
Nov 14 '18 at 15:32
|
show 2 more comments
2 Answers
2
active
oldest
votes
0xCBF43926
is the bit-wise inverse ("not") of 0x340BC6D9
. You just need to use not
on the result, or exclusive or with $FFFFFFFF
.
add a comment |
Note that FPC comes with a CRC32 unit. (derived from crc32.c by Mark Adler, above )
This unit has a function to calculate the CRC for a block called crc32()
function crc32 (crc : cardinal; buf : Pbyte; len : cardinal): cardinal;
The XOR is included in this crc32.crc32() function.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53302601%2fhow-is-a-crc32-checksum-calculated-with-freepascallazarus%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
0xCBF43926
is the bit-wise inverse ("not") of 0x340BC6D9
. You just need to use not
on the result, or exclusive or with $FFFFFFFF
.
add a comment |
0xCBF43926
is the bit-wise inverse ("not") of 0x340BC6D9
. You just need to use not
on the result, or exclusive or with $FFFFFFFF
.
add a comment |
0xCBF43926
is the bit-wise inverse ("not") of 0x340BC6D9
. You just need to use not
on the result, or exclusive or with $FFFFFFFF
.
0xCBF43926
is the bit-wise inverse ("not") of 0x340BC6D9
. You just need to use not
on the result, or exclusive or with $FFFFFFFF
.
answered Nov 14 '18 at 16:26


Mark AdlerMark Adler
58.3k864111
58.3k864111
add a comment |
add a comment |
Note that FPC comes with a CRC32 unit. (derived from crc32.c by Mark Adler, above )
This unit has a function to calculate the CRC for a block called crc32()
function crc32 (crc : cardinal; buf : Pbyte; len : cardinal): cardinal;
The XOR is included in this crc32.crc32() function.
add a comment |
Note that FPC comes with a CRC32 unit. (derived from crc32.c by Mark Adler, above )
This unit has a function to calculate the CRC for a block called crc32()
function crc32 (crc : cardinal; buf : Pbyte; len : cardinal): cardinal;
The XOR is included in this crc32.crc32() function.
add a comment |
Note that FPC comes with a CRC32 unit. (derived from crc32.c by Mark Adler, above )
This unit has a function to calculate the CRC for a block called crc32()
function crc32 (crc : cardinal; buf : Pbyte; len : cardinal): cardinal;
The XOR is included in this crc32.crc32() function.
Note that FPC comes with a CRC32 unit. (derived from crc32.c by Mark Adler, above )
This unit has a function to calculate the CRC for a block called crc32()
function crc32 (crc : cardinal; buf : Pbyte; len : cardinal): cardinal;
The XOR is included in this crc32.crc32() function.
answered Nov 15 '18 at 10:32
Marco van de VoortMarco van de Voort
22.2k44079
22.2k44079
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53302601%2fhow-is-a-crc32-checksum-calculated-with-freepascallazarus%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
dAgR7xTPXo xo0D1i CHl7JOY,2kD GQLelk5yfo605 VS7w,7,clWuz,OJUR Pj
We don't know what it is meant to do, and how it fails. You need to learn how to debug your program. That's your next task.
– David Heffernan
Nov 14 '18 at 14:47
my program just looking for checksum and finds wrong numbers. It checks .txt file where "123456789" and it finds "0x340BC6D9", but it must be "0xCBF43926". I already tried to debug as you said, but I have no idea what's problem here. That's why I asking here about my problem
– Claire
Nov 14 '18 at 15:17
There are many variants of CRC32 algorithm. Who knows which one you want? Presumably you do. You have working C code, and now want Pascal code. Compare the execution of the two versions (Pascal against C). Find out where the two diverge. Fix, and repeat. When there is no more divergence, the task is done. Once again, your next task it to learn how to debug. This is the perfect opportunity. Would you like to learn how to debug? Or do you just want code that works?
– David Heffernan
Nov 14 '18 at 15:20
I'd like to learn how to debug, I guess... okay then, I'll try once again
– Claire
Nov 14 '18 at 15:29
Just do what I say. Compare the execution of the two programs, the C version and the Pascal version. Output (e.g. to console) the value of
CRC
each time around the loop and compare the outputs of those two programs. Take it from there.– David Heffernan
Nov 14 '18 at 15:32