Service stopForeground(false) remove notification when should not
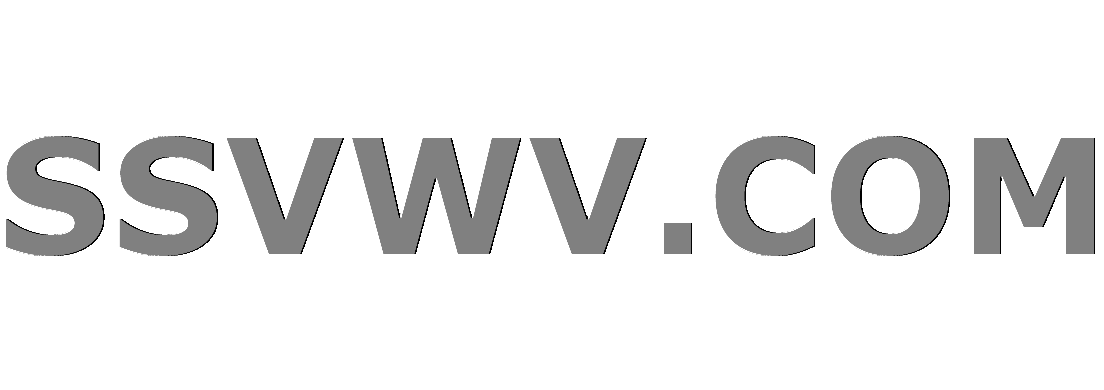
Multi tool use
Instead of
stopForeground(true)
calling,
stopForeground(false)
should retain the notification as it is (without ongoing state) unless it is dismissed by user/removed programmatically.
This also should prevents notification flashing since I am not recreating the notification.
But it does not work. stopForeground(false) has the same behavior of stopForeground(true).
This is a sample project:
public class AudioTestService extends Service {
private static final String CHANNEL_ID = "TestChannel";
private static final int NOTIFICATION_ID = 14;
Notification mBuilder;
public AudioTestService() {
}
@Override
public IBinder onBind(Intent intent) {
// TODO: Return the communication channel to the service.
// throw new UnsupportedOperationException("Not yet implemented");
return null;
}
@Override
public void onTaskRemoved(Intent rootIntent) {
stopForeground(true);
super.onTaskRemoved(rootIntent);
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
Intent intentA = new Intent(this, MainActivity.class);
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK | Intent.FLAG_ACTIVITY_CLEAR_TASK);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intentA, 0);
Notification mBuilder = new NotificationCompat.Builder(this, CHANNEL_ID)
.setSmallIcon(R.mipmap.ic_launcher)
.setContentTitle("Titolo")
.setContentText("Descrizione")
.setContentIntent(pendingIntent)
.setOngoing(false)
.setPriority(NotificationCompat.PRIORITY_DEFAULT)
.build();
this.mBuilder = mBuilder;
createNotificationChannel();
startForeground(NOTIFICATION_ID, mBuilder);
return START_STICKY;
}
private void createNotificationChannel() {
// Create the NotificationChannel, but only on API 26+ because
// the NotificationChannel class is new and not in the support library
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
CharSequence name = CHANNEL_ID;
String description = CHANNEL_ID + "Description ";
int importance = NotificationManager.IMPORTANCE_DEFAULT;
NotificationChannel channel = new NotificationChannel(CHANNEL_ID, name, importance);
channel.setDescription(description);
// Register the channel with the system; you can't change the importance
// or other notification behaviors after this
NotificationManager notificationManager = getSystemService(NotificationManager.class);
notificationManager.createNotificationChannel(channel);
}
}
@Override
public void onDestroy() {
stopForeground(false);
//NotificationManagerCompat.from(this).notify(NOTIFICATION_ID, mBuilder);
super.onDestroy();
} }
The activity, easily handle the button click event:
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button startService = findViewById(R.id.startService);
Button stopService = findViewById(R.id.stopService);
Button stopNotification = findViewById(R.id.stopWithNotification);
startService.setOnClickListener(this);
stopService.setOnClickListener(this);
stopNotification.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.startService:
ContextCompat.startForegroundService(this, new Intent(this, AudioTestService.class));
break;
case R.id.stopService:
finish();
break;
case R.id.stopWithNotification:
stopService(new Intent(this, AudioTestService.class));
break;
}
}}
If you look at the Service's onDestroy() method I set
stopForeground(false);
instead of the method onTaskRemoved() that should remove the notification when the app is cleaned from the task list.
What am I doing wrong?
Please do not mark this as duplicated, I am looking for a solution for days...

add a comment |
Instead of
stopForeground(true)
calling,
stopForeground(false)
should retain the notification as it is (without ongoing state) unless it is dismissed by user/removed programmatically.
This also should prevents notification flashing since I am not recreating the notification.
But it does not work. stopForeground(false) has the same behavior of stopForeground(true).
This is a sample project:
public class AudioTestService extends Service {
private static final String CHANNEL_ID = "TestChannel";
private static final int NOTIFICATION_ID = 14;
Notification mBuilder;
public AudioTestService() {
}
@Override
public IBinder onBind(Intent intent) {
// TODO: Return the communication channel to the service.
// throw new UnsupportedOperationException("Not yet implemented");
return null;
}
@Override
public void onTaskRemoved(Intent rootIntent) {
stopForeground(true);
super.onTaskRemoved(rootIntent);
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
Intent intentA = new Intent(this, MainActivity.class);
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK | Intent.FLAG_ACTIVITY_CLEAR_TASK);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intentA, 0);
Notification mBuilder = new NotificationCompat.Builder(this, CHANNEL_ID)
.setSmallIcon(R.mipmap.ic_launcher)
.setContentTitle("Titolo")
.setContentText("Descrizione")
.setContentIntent(pendingIntent)
.setOngoing(false)
.setPriority(NotificationCompat.PRIORITY_DEFAULT)
.build();
this.mBuilder = mBuilder;
createNotificationChannel();
startForeground(NOTIFICATION_ID, mBuilder);
return START_STICKY;
}
private void createNotificationChannel() {
// Create the NotificationChannel, but only on API 26+ because
// the NotificationChannel class is new and not in the support library
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
CharSequence name = CHANNEL_ID;
String description = CHANNEL_ID + "Description ";
int importance = NotificationManager.IMPORTANCE_DEFAULT;
NotificationChannel channel = new NotificationChannel(CHANNEL_ID, name, importance);
channel.setDescription(description);
// Register the channel with the system; you can't change the importance
// or other notification behaviors after this
NotificationManager notificationManager = getSystemService(NotificationManager.class);
notificationManager.createNotificationChannel(channel);
}
}
@Override
public void onDestroy() {
stopForeground(false);
//NotificationManagerCompat.from(this).notify(NOTIFICATION_ID, mBuilder);
super.onDestroy();
} }
The activity, easily handle the button click event:
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button startService = findViewById(R.id.startService);
Button stopService = findViewById(R.id.stopService);
Button stopNotification = findViewById(R.id.stopWithNotification);
startService.setOnClickListener(this);
stopService.setOnClickListener(this);
stopNotification.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.startService:
ContextCompat.startForegroundService(this, new Intent(this, AudioTestService.class));
break;
case R.id.stopService:
finish();
break;
case R.id.stopWithNotification:
stopService(new Intent(this, AudioTestService.class));
break;
}
}}
If you look at the Service's onDestroy() method I set
stopForeground(false);
instead of the method onTaskRemoved() that should remove the notification when the app is cleaned from the task list.
What am I doing wrong?
Please do not mark this as duplicated, I am looking for a solution for days...

developer.android.com/reference/android/app/…
– Jonathan I
Nov 14 '18 at 14:45
add a comment |
Instead of
stopForeground(true)
calling,
stopForeground(false)
should retain the notification as it is (without ongoing state) unless it is dismissed by user/removed programmatically.
This also should prevents notification flashing since I am not recreating the notification.
But it does not work. stopForeground(false) has the same behavior of stopForeground(true).
This is a sample project:
public class AudioTestService extends Service {
private static final String CHANNEL_ID = "TestChannel";
private static final int NOTIFICATION_ID = 14;
Notification mBuilder;
public AudioTestService() {
}
@Override
public IBinder onBind(Intent intent) {
// TODO: Return the communication channel to the service.
// throw new UnsupportedOperationException("Not yet implemented");
return null;
}
@Override
public void onTaskRemoved(Intent rootIntent) {
stopForeground(true);
super.onTaskRemoved(rootIntent);
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
Intent intentA = new Intent(this, MainActivity.class);
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK | Intent.FLAG_ACTIVITY_CLEAR_TASK);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intentA, 0);
Notification mBuilder = new NotificationCompat.Builder(this, CHANNEL_ID)
.setSmallIcon(R.mipmap.ic_launcher)
.setContentTitle("Titolo")
.setContentText("Descrizione")
.setContentIntent(pendingIntent)
.setOngoing(false)
.setPriority(NotificationCompat.PRIORITY_DEFAULT)
.build();
this.mBuilder = mBuilder;
createNotificationChannel();
startForeground(NOTIFICATION_ID, mBuilder);
return START_STICKY;
}
private void createNotificationChannel() {
// Create the NotificationChannel, but only on API 26+ because
// the NotificationChannel class is new and not in the support library
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
CharSequence name = CHANNEL_ID;
String description = CHANNEL_ID + "Description ";
int importance = NotificationManager.IMPORTANCE_DEFAULT;
NotificationChannel channel = new NotificationChannel(CHANNEL_ID, name, importance);
channel.setDescription(description);
// Register the channel with the system; you can't change the importance
// or other notification behaviors after this
NotificationManager notificationManager = getSystemService(NotificationManager.class);
notificationManager.createNotificationChannel(channel);
}
}
@Override
public void onDestroy() {
stopForeground(false);
//NotificationManagerCompat.from(this).notify(NOTIFICATION_ID, mBuilder);
super.onDestroy();
} }
The activity, easily handle the button click event:
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button startService = findViewById(R.id.startService);
Button stopService = findViewById(R.id.stopService);
Button stopNotification = findViewById(R.id.stopWithNotification);
startService.setOnClickListener(this);
stopService.setOnClickListener(this);
stopNotification.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.startService:
ContextCompat.startForegroundService(this, new Intent(this, AudioTestService.class));
break;
case R.id.stopService:
finish();
break;
case R.id.stopWithNotification:
stopService(new Intent(this, AudioTestService.class));
break;
}
}}
If you look at the Service's onDestroy() method I set
stopForeground(false);
instead of the method onTaskRemoved() that should remove the notification when the app is cleaned from the task list.
What am I doing wrong?
Please do not mark this as duplicated, I am looking for a solution for days...

Instead of
stopForeground(true)
calling,
stopForeground(false)
should retain the notification as it is (without ongoing state) unless it is dismissed by user/removed programmatically.
This also should prevents notification flashing since I am not recreating the notification.
But it does not work. stopForeground(false) has the same behavior of stopForeground(true).
This is a sample project:
public class AudioTestService extends Service {
private static final String CHANNEL_ID = "TestChannel";
private static final int NOTIFICATION_ID = 14;
Notification mBuilder;
public AudioTestService() {
}
@Override
public IBinder onBind(Intent intent) {
// TODO: Return the communication channel to the service.
// throw new UnsupportedOperationException("Not yet implemented");
return null;
}
@Override
public void onTaskRemoved(Intent rootIntent) {
stopForeground(true);
super.onTaskRemoved(rootIntent);
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
Intent intentA = new Intent(this, MainActivity.class);
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK | Intent.FLAG_ACTIVITY_CLEAR_TASK);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intentA, 0);
Notification mBuilder = new NotificationCompat.Builder(this, CHANNEL_ID)
.setSmallIcon(R.mipmap.ic_launcher)
.setContentTitle("Titolo")
.setContentText("Descrizione")
.setContentIntent(pendingIntent)
.setOngoing(false)
.setPriority(NotificationCompat.PRIORITY_DEFAULT)
.build();
this.mBuilder = mBuilder;
createNotificationChannel();
startForeground(NOTIFICATION_ID, mBuilder);
return START_STICKY;
}
private void createNotificationChannel() {
// Create the NotificationChannel, but only on API 26+ because
// the NotificationChannel class is new and not in the support library
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
CharSequence name = CHANNEL_ID;
String description = CHANNEL_ID + "Description ";
int importance = NotificationManager.IMPORTANCE_DEFAULT;
NotificationChannel channel = new NotificationChannel(CHANNEL_ID, name, importance);
channel.setDescription(description);
// Register the channel with the system; you can't change the importance
// or other notification behaviors after this
NotificationManager notificationManager = getSystemService(NotificationManager.class);
notificationManager.createNotificationChannel(channel);
}
}
@Override
public void onDestroy() {
stopForeground(false);
//NotificationManagerCompat.from(this).notify(NOTIFICATION_ID, mBuilder);
super.onDestroy();
} }
The activity, easily handle the button click event:
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button startService = findViewById(R.id.startService);
Button stopService = findViewById(R.id.stopService);
Button stopNotification = findViewById(R.id.stopWithNotification);
startService.setOnClickListener(this);
stopService.setOnClickListener(this);
stopNotification.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.startService:
ContextCompat.startForegroundService(this, new Intent(this, AudioTestService.class));
break;
case R.id.stopService:
finish();
break;
case R.id.stopWithNotification:
stopService(new Intent(this, AudioTestService.class));
break;
}
}}
If you look at the Service's onDestroy() method I set
stopForeground(false);
instead of the method onTaskRemoved() that should remove the notification when the app is cleaned from the task list.
What am I doing wrong?
Please do not mark this as duplicated, I am looking for a solution for days...


asked Nov 14 '18 at 14:27


Jonathan IJonathan I
861213
861213
developer.android.com/reference/android/app/…
– Jonathan I
Nov 14 '18 at 14:45
add a comment |
developer.android.com/reference/android/app/…
– Jonathan I
Nov 14 '18 at 14:45
developer.android.com/reference/android/app/…
– Jonathan I
Nov 14 '18 at 14:45
developer.android.com/reference/android/app/…
– Jonathan I
Nov 14 '18 at 14:45
add a comment |
1 Answer
1
active
oldest
votes
Instead of calling stopForeground(false);
from onDestroy()
, send a broadcast from activity (with action) for stop service. Change your onStartCommand
code to check action in intent
and do startForeground
or stopForeground(false);
...Thanks for your advice.
– Jonathan I
Nov 15 '18 at 21:38
It seems that your solution works. Thanks!
– Jonathan I
Nov 17 '18 at 12:42
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53302511%2fservice-stopforegroundfalse-remove-notification-when-should-not%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Instead of calling stopForeground(false);
from onDestroy()
, send a broadcast from activity (with action) for stop service. Change your onStartCommand
code to check action in intent
and do startForeground
or stopForeground(false);
...Thanks for your advice.
– Jonathan I
Nov 15 '18 at 21:38
It seems that your solution works. Thanks!
– Jonathan I
Nov 17 '18 at 12:42
add a comment |
Instead of calling stopForeground(false);
from onDestroy()
, send a broadcast from activity (with action) for stop service. Change your onStartCommand
code to check action in intent
and do startForeground
or stopForeground(false);
...Thanks for your advice.
– Jonathan I
Nov 15 '18 at 21:38
It seems that your solution works. Thanks!
– Jonathan I
Nov 17 '18 at 12:42
add a comment |
Instead of calling stopForeground(false);
from onDestroy()
, send a broadcast from activity (with action) for stop service. Change your onStartCommand
code to check action in intent
and do startForeground
or stopForeground(false);
Instead of calling stopForeground(false);
from onDestroy()
, send a broadcast from activity (with action) for stop service. Change your onStartCommand
code to check action in intent
and do startForeground
or stopForeground(false);
answered Nov 14 '18 at 18:27
VichitraVijVichitraVij
32028
32028
...Thanks for your advice.
– Jonathan I
Nov 15 '18 at 21:38
It seems that your solution works. Thanks!
– Jonathan I
Nov 17 '18 at 12:42
add a comment |
...Thanks for your advice.
– Jonathan I
Nov 15 '18 at 21:38
It seems that your solution works. Thanks!
– Jonathan I
Nov 17 '18 at 12:42
...Thanks for your advice.
– Jonathan I
Nov 15 '18 at 21:38
...Thanks for your advice.
– Jonathan I
Nov 15 '18 at 21:38
It seems that your solution works. Thanks!
– Jonathan I
Nov 17 '18 at 12:42
It seems that your solution works. Thanks!
– Jonathan I
Nov 17 '18 at 12:42
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53302511%2fservice-stopforegroundfalse-remove-notification-when-should-not%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
k O7CeYT uwHurmcWdpkrlvM,L9SWa7ZQ64h8pXeg
developer.android.com/reference/android/app/…
– Jonathan I
Nov 14 '18 at 14:45