Looping tasks in Ansible
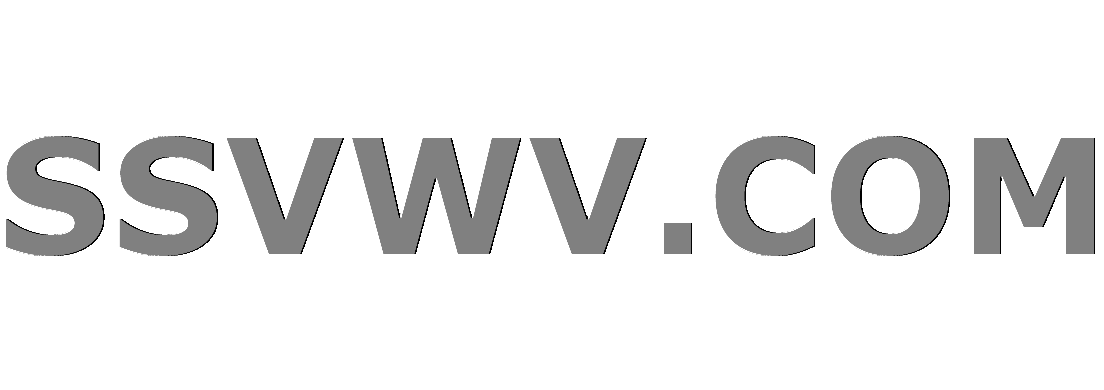
Multi tool use
I have the following tasks in Ansible:
- name: Getting Job Info
uri:
url: "https://{{ hostname }}/job/JobService"
method: POST
user: "{{ username }}"
password: "{{ password }}"
body: "{{ lookup( 'template' , 'jobInfo.xml.j2' ) }}"
status_code: 200
validate_certs: false
headers:
soapaction: "getJobInfoVO"
return_content: true
register: job_status_soap
- name: Converting Job Info response to JSON
set_fact:
job_status_json: "{{ job_status_soap.content | xml2json }}"
- name: Setting Job Status
set_fact:
job_status: "{{ job_status_json['soapenv:Envelope']['soapenv:Body']['multiRef'][0]['status']['#text'] }}"
- debug: msg="{{ job_status }}"
As you can see, it makes a web service call, converts the SOAP response to JSON and then extracts the relevant value (an integer) and sets it as a fact. I need to repeat this block of code until the aforementioned integer value is equal to certain integer.
Any ideas on how I might approach this task?
loops ansible uri
add a comment |
I have the following tasks in Ansible:
- name: Getting Job Info
uri:
url: "https://{{ hostname }}/job/JobService"
method: POST
user: "{{ username }}"
password: "{{ password }}"
body: "{{ lookup( 'template' , 'jobInfo.xml.j2' ) }}"
status_code: 200
validate_certs: false
headers:
soapaction: "getJobInfoVO"
return_content: true
register: job_status_soap
- name: Converting Job Info response to JSON
set_fact:
job_status_json: "{{ job_status_soap.content | xml2json }}"
- name: Setting Job Status
set_fact:
job_status: "{{ job_status_json['soapenv:Envelope']['soapenv:Body']['multiRef'][0]['status']['#text'] }}"
- debug: msg="{{ job_status }}"
As you can see, it makes a web service call, converts the SOAP response to JSON and then extracts the relevant value (an integer) and sets it as a fact. I need to repeat this block of code until the aforementioned integer value is equal to certain integer.
Any ideas on how I might approach this task?
loops ansible uri
Can you generate a list of the integers? Then you could use it as a dict, and loop through using with_items?
– Michael Gardner
Nov 16 '18 at 12:39
add a comment |
I have the following tasks in Ansible:
- name: Getting Job Info
uri:
url: "https://{{ hostname }}/job/JobService"
method: POST
user: "{{ username }}"
password: "{{ password }}"
body: "{{ lookup( 'template' , 'jobInfo.xml.j2' ) }}"
status_code: 200
validate_certs: false
headers:
soapaction: "getJobInfoVO"
return_content: true
register: job_status_soap
- name: Converting Job Info response to JSON
set_fact:
job_status_json: "{{ job_status_soap.content | xml2json }}"
- name: Setting Job Status
set_fact:
job_status: "{{ job_status_json['soapenv:Envelope']['soapenv:Body']['multiRef'][0]['status']['#text'] }}"
- debug: msg="{{ job_status }}"
As you can see, it makes a web service call, converts the SOAP response to JSON and then extracts the relevant value (an integer) and sets it as a fact. I need to repeat this block of code until the aforementioned integer value is equal to certain integer.
Any ideas on how I might approach this task?
loops ansible uri
I have the following tasks in Ansible:
- name: Getting Job Info
uri:
url: "https://{{ hostname }}/job/JobService"
method: POST
user: "{{ username }}"
password: "{{ password }}"
body: "{{ lookup( 'template' , 'jobInfo.xml.j2' ) }}"
status_code: 200
validate_certs: false
headers:
soapaction: "getJobInfoVO"
return_content: true
register: job_status_soap
- name: Converting Job Info response to JSON
set_fact:
job_status_json: "{{ job_status_soap.content | xml2json }}"
- name: Setting Job Status
set_fact:
job_status: "{{ job_status_json['soapenv:Envelope']['soapenv:Body']['multiRef'][0]['status']['#text'] }}"
- debug: msg="{{ job_status }}"
As you can see, it makes a web service call, converts the SOAP response to JSON and then extracts the relevant value (an integer) and sets it as a fact. I need to repeat this block of code until the aforementioned integer value is equal to certain integer.
Any ideas on how I might approach this task?
loops ansible uri
loops ansible uri
edited Nov 14 '18 at 15:15
Anthon
30k1793147
30k1793147
asked Nov 14 '18 at 13:31
automation1002automation1002
61
61
Can you generate a list of the integers? Then you could use it as a dict, and loop through using with_items?
– Michael Gardner
Nov 16 '18 at 12:39
add a comment |
Can you generate a list of the integers? Then you could use it as a dict, and loop through using with_items?
– Michael Gardner
Nov 16 '18 at 12:39
Can you generate a list of the integers? Then you could use it as a dict, and loop through using with_items?
– Michael Gardner
Nov 16 '18 at 12:39
Can you generate a list of the integers? Then you could use it as a dict, and loop through using with_items?
– Michael Gardner
Nov 16 '18 at 12:39
add a comment |
1 Answer
1
active
oldest
votes
This would have been easy to implement using until
to execute ansible do-until loop if the response was in JSON format.
But here's a working example with xml response.
Pre-requisites: (Make sure you have the python dependencies installed on your ansible host)
1) Using custom xml_to_json filter.
2) Using json_query filter
P.S. Executing following play will not trigger retry as the until
contion is already met.
If you want to test if retry is working then change the until
condition or the value of tag in the request header.
---
- name: Retry url until xml content equals certain value
hosts: 127.0.0.1
connection: local
become_user: root
become: yes
tasks:
- name: Check job status
uri:
url: "https://httpbin.org/anything"
method: GET
timeout: 10
validate_certs: no
headers:
content: "<envelope><body><request><status>2</status></request></body></envelope>"
register: get_search_job_status_response
until: get_search_job_status_response.json.headers.Content|from_xml|json_query('envelope.body.request.status')|int == 2
retries: 2
delay: 2
...
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53301422%2flooping-tasks-in-ansible%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This would have been easy to implement using until
to execute ansible do-until loop if the response was in JSON format.
But here's a working example with xml response.
Pre-requisites: (Make sure you have the python dependencies installed on your ansible host)
1) Using custom xml_to_json filter.
2) Using json_query filter
P.S. Executing following play will not trigger retry as the until
contion is already met.
If you want to test if retry is working then change the until
condition or the value of tag in the request header.
---
- name: Retry url until xml content equals certain value
hosts: 127.0.0.1
connection: local
become_user: root
become: yes
tasks:
- name: Check job status
uri:
url: "https://httpbin.org/anything"
method: GET
timeout: 10
validate_certs: no
headers:
content: "<envelope><body><request><status>2</status></request></body></envelope>"
register: get_search_job_status_response
until: get_search_job_status_response.json.headers.Content|from_xml|json_query('envelope.body.request.status')|int == 2
retries: 2
delay: 2
...
add a comment |
This would have been easy to implement using until
to execute ansible do-until loop if the response was in JSON format.
But here's a working example with xml response.
Pre-requisites: (Make sure you have the python dependencies installed on your ansible host)
1) Using custom xml_to_json filter.
2) Using json_query filter
P.S. Executing following play will not trigger retry as the until
contion is already met.
If you want to test if retry is working then change the until
condition or the value of tag in the request header.
---
- name: Retry url until xml content equals certain value
hosts: 127.0.0.1
connection: local
become_user: root
become: yes
tasks:
- name: Check job status
uri:
url: "https://httpbin.org/anything"
method: GET
timeout: 10
validate_certs: no
headers:
content: "<envelope><body><request><status>2</status></request></body></envelope>"
register: get_search_job_status_response
until: get_search_job_status_response.json.headers.Content|from_xml|json_query('envelope.body.request.status')|int == 2
retries: 2
delay: 2
...
add a comment |
This would have been easy to implement using until
to execute ansible do-until loop if the response was in JSON format.
But here's a working example with xml response.
Pre-requisites: (Make sure you have the python dependencies installed on your ansible host)
1) Using custom xml_to_json filter.
2) Using json_query filter
P.S. Executing following play will not trigger retry as the until
contion is already met.
If you want to test if retry is working then change the until
condition or the value of tag in the request header.
---
- name: Retry url until xml content equals certain value
hosts: 127.0.0.1
connection: local
become_user: root
become: yes
tasks:
- name: Check job status
uri:
url: "https://httpbin.org/anything"
method: GET
timeout: 10
validate_certs: no
headers:
content: "<envelope><body><request><status>2</status></request></body></envelope>"
register: get_search_job_status_response
until: get_search_job_status_response.json.headers.Content|from_xml|json_query('envelope.body.request.status')|int == 2
retries: 2
delay: 2
...
This would have been easy to implement using until
to execute ansible do-until loop if the response was in JSON format.
But here's a working example with xml response.
Pre-requisites: (Make sure you have the python dependencies installed on your ansible host)
1) Using custom xml_to_json filter.
2) Using json_query filter
P.S. Executing following play will not trigger retry as the until
contion is already met.
If you want to test if retry is working then change the until
condition or the value of tag in the request header.
---
- name: Retry url until xml content equals certain value
hosts: 127.0.0.1
connection: local
become_user: root
become: yes
tasks:
- name: Check job status
uri:
url: "https://httpbin.org/anything"
method: GET
timeout: 10
validate_certs: no
headers:
content: "<envelope><body><request><status>2</status></request></body></envelope>"
register: get_search_job_status_response
until: get_search_job_status_response.json.headers.Content|from_xml|json_query('envelope.body.request.status')|int == 2
retries: 2
delay: 2
...
answered Nov 21 '18 at 5:31
Anant NaugaiAnant Naugai
19029
19029
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53301422%2flooping-tasks-in-ansible%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ZWYO5pbpgwwRM,li1Bxj69 yl kVlD SFeAcmbu
Can you generate a list of the integers? Then you could use it as a dict, and loop through using with_items?
– Michael Gardner
Nov 16 '18 at 12:39