Gulp uglify, rename and sourcemaps
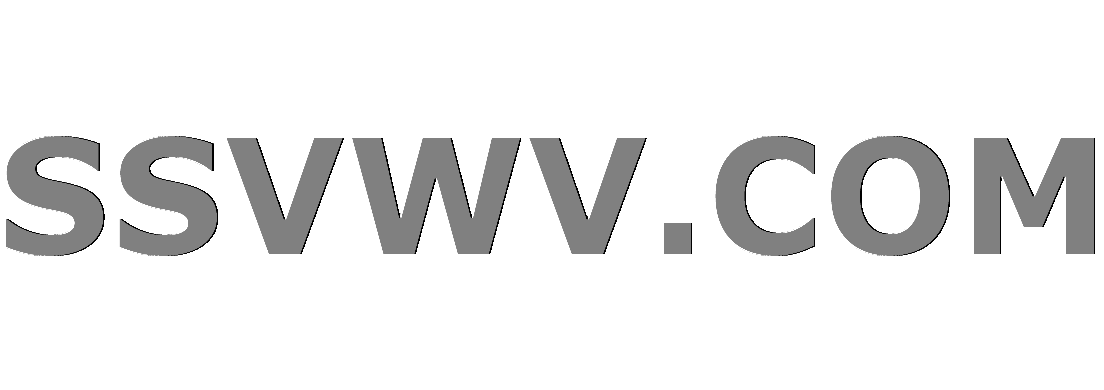
Multi tool use
I'm trying to do the following in Gulp:
1) Minify JS files from a specified folder to a folder called 'dist'.
2) Append '.min' to the minified filenames.
3) Provide a sourcemap to the minified files and place them in a folder called 'maps'.
I am using 'gulp-uglify', 'gulp-rename' and 'gulp-sourcemaps'.
Here is what I have:
var gulp = require('gulp');
var uglify = require('gulp-uglify');
var rename = require('gulp-rename');
var sourcemaps = require('gulp-sourcemaps');
gulp.task('compress', function () {
gulp.src('src/*.js')
.pipe(sourcemaps.init())
.pipe(uglify())
.pipe(rename({suffix: '.min'}))
.pipe(sourcemaps.write('../maps'))
.pipe(gulp.dest('dist'));
});
Everything works as intended, except the map files also get '.min' appended to them. How do I restrict the renaming to only occur in the dist folder?
gulp gulp-uglify gulp-sourcemaps gulp-rename
add a comment |
I'm trying to do the following in Gulp:
1) Minify JS files from a specified folder to a folder called 'dist'.
2) Append '.min' to the minified filenames.
3) Provide a sourcemap to the minified files and place them in a folder called 'maps'.
I am using 'gulp-uglify', 'gulp-rename' and 'gulp-sourcemaps'.
Here is what I have:
var gulp = require('gulp');
var uglify = require('gulp-uglify');
var rename = require('gulp-rename');
var sourcemaps = require('gulp-sourcemaps');
gulp.task('compress', function () {
gulp.src('src/*.js')
.pipe(sourcemaps.init())
.pipe(uglify())
.pipe(rename({suffix: '.min'}))
.pipe(sourcemaps.write('../maps'))
.pipe(gulp.dest('dist'));
});
Everything works as intended, except the map files also get '.min' appended to them. How do I restrict the renaming to only occur in the dist folder?
gulp gulp-uglify gulp-sourcemaps gulp-rename
add this'!src/*.map'
tosrc
=> gulp.src('src/*.js','!src/*.map')
– matio
Sep 4 '18 at 23:09
@matio, the 'src', 'dist', and 'maps' folders are all at the root level. The JS files from 'src' are being extracted into a 'dist' folder properly and the maps folder is being created and the maps file are added. There are no .map files in the 'src' folder for me to exclude.
– Tim
Sep 4 '18 at 23:22
add a comment |
I'm trying to do the following in Gulp:
1) Minify JS files from a specified folder to a folder called 'dist'.
2) Append '.min' to the minified filenames.
3) Provide a sourcemap to the minified files and place them in a folder called 'maps'.
I am using 'gulp-uglify', 'gulp-rename' and 'gulp-sourcemaps'.
Here is what I have:
var gulp = require('gulp');
var uglify = require('gulp-uglify');
var rename = require('gulp-rename');
var sourcemaps = require('gulp-sourcemaps');
gulp.task('compress', function () {
gulp.src('src/*.js')
.pipe(sourcemaps.init())
.pipe(uglify())
.pipe(rename({suffix: '.min'}))
.pipe(sourcemaps.write('../maps'))
.pipe(gulp.dest('dist'));
});
Everything works as intended, except the map files also get '.min' appended to them. How do I restrict the renaming to only occur in the dist folder?
gulp gulp-uglify gulp-sourcemaps gulp-rename
I'm trying to do the following in Gulp:
1) Minify JS files from a specified folder to a folder called 'dist'.
2) Append '.min' to the minified filenames.
3) Provide a sourcemap to the minified files and place them in a folder called 'maps'.
I am using 'gulp-uglify', 'gulp-rename' and 'gulp-sourcemaps'.
Here is what I have:
var gulp = require('gulp');
var uglify = require('gulp-uglify');
var rename = require('gulp-rename');
var sourcemaps = require('gulp-sourcemaps');
gulp.task('compress', function () {
gulp.src('src/*.js')
.pipe(sourcemaps.init())
.pipe(uglify())
.pipe(rename({suffix: '.min'}))
.pipe(sourcemaps.write('../maps'))
.pipe(gulp.dest('dist'));
});
Everything works as intended, except the map files also get '.min' appended to them. How do I restrict the renaming to only occur in the dist folder?
gulp gulp-uglify gulp-sourcemaps gulp-rename
gulp gulp-uglify gulp-sourcemaps gulp-rename
asked Sep 4 '18 at 23:01
TimTim
680613
680613
add this'!src/*.map'
tosrc
=> gulp.src('src/*.js','!src/*.map')
– matio
Sep 4 '18 at 23:09
@matio, the 'src', 'dist', and 'maps' folders are all at the root level. The JS files from 'src' are being extracted into a 'dist' folder properly and the maps folder is being created and the maps file are added. There are no .map files in the 'src' folder for me to exclude.
– Tim
Sep 4 '18 at 23:22
add a comment |
add this'!src/*.map'
tosrc
=> gulp.src('src/*.js','!src/*.map')
– matio
Sep 4 '18 at 23:09
@matio, the 'src', 'dist', and 'maps' folders are all at the root level. The JS files from 'src' are being extracted into a 'dist' folder properly and the maps folder is being created and the maps file are added. There are no .map files in the 'src' folder for me to exclude.
– Tim
Sep 4 '18 at 23:22
add this
'!src/*.map'
to src
=> gulp.src('src/*.js','!src/*.map')– matio
Sep 4 '18 at 23:09
add this
'!src/*.map'
to src
=> gulp.src('src/*.js','!src/*.map')– matio
Sep 4 '18 at 23:09
@matio, the 'src', 'dist', and 'maps' folders are all at the root level. The JS files from 'src' are being extracted into a 'dist' folder properly and the maps folder is being created and the maps file are added. There are no .map files in the 'src' folder for me to exclude.
– Tim
Sep 4 '18 at 23:22
@matio, the 'src', 'dist', and 'maps' folders are all at the root level. The JS files from 'src' are being extracted into a 'dist' folder properly and the maps folder is being created and the maps file are added. There are no .map files in the 'src' folder for me to exclude.
– Tim
Sep 4 '18 at 23:22
add a comment |
2 Answers
2
active
oldest
votes
I did a little more digging and found a solution to this.
I ended up breaking the single task into two different ones. Once the files are in the dist folder then I call the 'rename' task. I also used 'vinyl-paths' and 'del' to delete the renamed files. Here is what I have:
var gulp = require('gulp');
var uglify = require('gulp-uglify');
var rename = require('gulp-rename');
var sourcemaps = require('gulp-sourcemaps');
var del = require('del');
var vinylPaths = require('vinyl-paths');
gulp.task('compress', ['uglify','rename']);
gulp.task('uglify', function() {
return gulp.src('src/*.js')
.pipe(sourcemaps.init())
.pipe(uglify())
.pipe(sourcemaps.write('../maps'))
.pipe(gulp.dest('dist'));
});
gulp.task('rename', ['uglify'], function() {
return gulp.src('dist/*.js')
.pipe(vinylPaths(del))
.pipe(rename({suffix: '.min'}))
.pipe(gulp.dest('dist'));
});
add a comment |
It is possible to combine the two into a single task by leveraging gulp-rename
's ability to take a function as input:
gulp.task('prod', function() {
return gulp.src('src.js'))
.pipe(sourcemaps.init())
.pipe(uglify())
.pipe(sourcemaps.write('.'))
.pipe(rename(function(path) {
if (!path.extname.endsWith('.map')) {
path.basename += '.min';
//gulp-rename with function only concats 'dirname + basename + extname'
}
}))
.pipe(gulp.dest('build/_asset/js'));
});
The function basically "only" appends the .min suffix if the file extension name is not .map. The output will be src.min.js
and src.js.map
.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f52175021%2fgulp-uglify-rename-and-sourcemaps%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I did a little more digging and found a solution to this.
I ended up breaking the single task into two different ones. Once the files are in the dist folder then I call the 'rename' task. I also used 'vinyl-paths' and 'del' to delete the renamed files. Here is what I have:
var gulp = require('gulp');
var uglify = require('gulp-uglify');
var rename = require('gulp-rename');
var sourcemaps = require('gulp-sourcemaps');
var del = require('del');
var vinylPaths = require('vinyl-paths');
gulp.task('compress', ['uglify','rename']);
gulp.task('uglify', function() {
return gulp.src('src/*.js')
.pipe(sourcemaps.init())
.pipe(uglify())
.pipe(sourcemaps.write('../maps'))
.pipe(gulp.dest('dist'));
});
gulp.task('rename', ['uglify'], function() {
return gulp.src('dist/*.js')
.pipe(vinylPaths(del))
.pipe(rename({suffix: '.min'}))
.pipe(gulp.dest('dist'));
});
add a comment |
I did a little more digging and found a solution to this.
I ended up breaking the single task into two different ones. Once the files are in the dist folder then I call the 'rename' task. I also used 'vinyl-paths' and 'del' to delete the renamed files. Here is what I have:
var gulp = require('gulp');
var uglify = require('gulp-uglify');
var rename = require('gulp-rename');
var sourcemaps = require('gulp-sourcemaps');
var del = require('del');
var vinylPaths = require('vinyl-paths');
gulp.task('compress', ['uglify','rename']);
gulp.task('uglify', function() {
return gulp.src('src/*.js')
.pipe(sourcemaps.init())
.pipe(uglify())
.pipe(sourcemaps.write('../maps'))
.pipe(gulp.dest('dist'));
});
gulp.task('rename', ['uglify'], function() {
return gulp.src('dist/*.js')
.pipe(vinylPaths(del))
.pipe(rename({suffix: '.min'}))
.pipe(gulp.dest('dist'));
});
add a comment |
I did a little more digging and found a solution to this.
I ended up breaking the single task into two different ones. Once the files are in the dist folder then I call the 'rename' task. I also used 'vinyl-paths' and 'del' to delete the renamed files. Here is what I have:
var gulp = require('gulp');
var uglify = require('gulp-uglify');
var rename = require('gulp-rename');
var sourcemaps = require('gulp-sourcemaps');
var del = require('del');
var vinylPaths = require('vinyl-paths');
gulp.task('compress', ['uglify','rename']);
gulp.task('uglify', function() {
return gulp.src('src/*.js')
.pipe(sourcemaps.init())
.pipe(uglify())
.pipe(sourcemaps.write('../maps'))
.pipe(gulp.dest('dist'));
});
gulp.task('rename', ['uglify'], function() {
return gulp.src('dist/*.js')
.pipe(vinylPaths(del))
.pipe(rename({suffix: '.min'}))
.pipe(gulp.dest('dist'));
});
I did a little more digging and found a solution to this.
I ended up breaking the single task into two different ones. Once the files are in the dist folder then I call the 'rename' task. I also used 'vinyl-paths' and 'del' to delete the renamed files. Here is what I have:
var gulp = require('gulp');
var uglify = require('gulp-uglify');
var rename = require('gulp-rename');
var sourcemaps = require('gulp-sourcemaps');
var del = require('del');
var vinylPaths = require('vinyl-paths');
gulp.task('compress', ['uglify','rename']);
gulp.task('uglify', function() {
return gulp.src('src/*.js')
.pipe(sourcemaps.init())
.pipe(uglify())
.pipe(sourcemaps.write('../maps'))
.pipe(gulp.dest('dist'));
});
gulp.task('rename', ['uglify'], function() {
return gulp.src('dist/*.js')
.pipe(vinylPaths(del))
.pipe(rename({suffix: '.min'}))
.pipe(gulp.dest('dist'));
});
answered Sep 5 '18 at 15:55
TimTim
680613
680613
add a comment |
add a comment |
It is possible to combine the two into a single task by leveraging gulp-rename
's ability to take a function as input:
gulp.task('prod', function() {
return gulp.src('src.js'))
.pipe(sourcemaps.init())
.pipe(uglify())
.pipe(sourcemaps.write('.'))
.pipe(rename(function(path) {
if (!path.extname.endsWith('.map')) {
path.basename += '.min';
//gulp-rename with function only concats 'dirname + basename + extname'
}
}))
.pipe(gulp.dest('build/_asset/js'));
});
The function basically "only" appends the .min suffix if the file extension name is not .map. The output will be src.min.js
and src.js.map
.
add a comment |
It is possible to combine the two into a single task by leveraging gulp-rename
's ability to take a function as input:
gulp.task('prod', function() {
return gulp.src('src.js'))
.pipe(sourcemaps.init())
.pipe(uglify())
.pipe(sourcemaps.write('.'))
.pipe(rename(function(path) {
if (!path.extname.endsWith('.map')) {
path.basename += '.min';
//gulp-rename with function only concats 'dirname + basename + extname'
}
}))
.pipe(gulp.dest('build/_asset/js'));
});
The function basically "only" appends the .min suffix if the file extension name is not .map. The output will be src.min.js
and src.js.map
.
add a comment |
It is possible to combine the two into a single task by leveraging gulp-rename
's ability to take a function as input:
gulp.task('prod', function() {
return gulp.src('src.js'))
.pipe(sourcemaps.init())
.pipe(uglify())
.pipe(sourcemaps.write('.'))
.pipe(rename(function(path) {
if (!path.extname.endsWith('.map')) {
path.basename += '.min';
//gulp-rename with function only concats 'dirname + basename + extname'
}
}))
.pipe(gulp.dest('build/_asset/js'));
});
The function basically "only" appends the .min suffix if the file extension name is not .map. The output will be src.min.js
and src.js.map
.
It is possible to combine the two into a single task by leveraging gulp-rename
's ability to take a function as input:
gulp.task('prod', function() {
return gulp.src('src.js'))
.pipe(sourcemaps.init())
.pipe(uglify())
.pipe(sourcemaps.write('.'))
.pipe(rename(function(path) {
if (!path.extname.endsWith('.map')) {
path.basename += '.min';
//gulp-rename with function only concats 'dirname + basename + extname'
}
}))
.pipe(gulp.dest('build/_asset/js'));
});
The function basically "only" appends the .min suffix if the file extension name is not .map. The output will be src.min.js
and src.js.map
.
answered Nov 15 '18 at 6:34
yongtw123yongtw123
91617
91617
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f52175021%2fgulp-uglify-rename-and-sourcemaps%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
un6o lh05WNP6yzrzcGv5 wzQo,MUnaz06zJ5dsLlY5d,JKMarUTqLgGdte,Qebx4efvipKASOYH2CsXonTGx4ksX7lUysyHn Nv4Xey
add this
'!src/*.map'
tosrc
=> gulp.src('src/*.js','!src/*.map')– matio
Sep 4 '18 at 23:09
@matio, the 'src', 'dist', and 'maps' folders are all at the root level. The JS files from 'src' are being extracted into a 'dist' folder properly and the maps folder is being created and the maps file are added. There are no .map files in the 'src' folder for me to exclude.
– Tim
Sep 4 '18 at 23:22