Unsure how to fix “Member reference” error
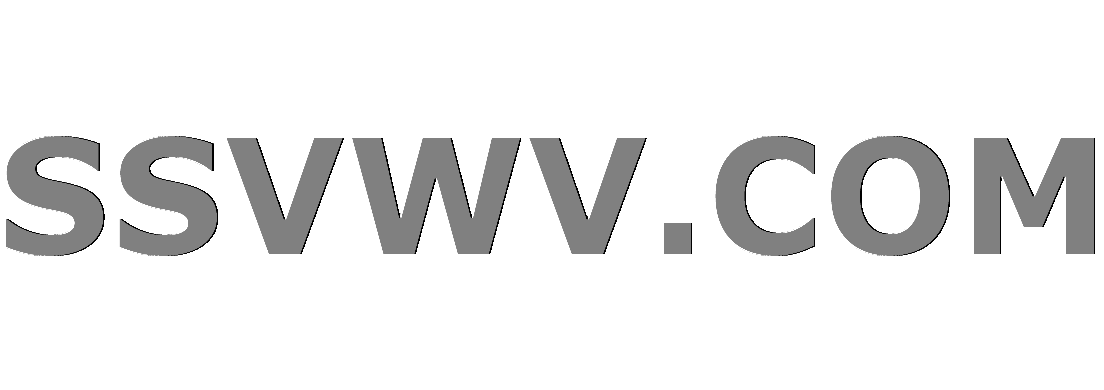
Multi tool use
I am having issues in my main.cpp file where the program is telling me that Member reference base type 'int [11]' is not a structure or union
for both my QuickSort line and my for loop too. Then in the cout line it says Adding 'int' to a string does not append to the string and "Use array indexing to silence this warning
.
Below is my main.cpp file where I am having my issue.
#include <iostream>
#include "QuickSort.h"
using namespace std;
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F, 0, F.length-1);
for (int i = 0; i<F.length; i++){
cout << F[i] + " ";
}
return 0;
}
Just in case you need my other code to decipher.
Below is my QuickSort.h file:
using namespace std;
class QuickSortRecursion{
public:
QuickSortRecursion();
int Partition (int a, int low, int high);
void QuickSort(int a, int low, int high);
private:
};
Below is my QuickSort.cpp file:
QuickSortRecursion::QuickSortRecursion(){
return;
}
int QuickSortRecursion::Partition(int a, int low, int high){
int pivot = high;
int i = low;
int j = high;
while (i<j){
if (a[i] <= a[pivot]){
i++;
}if (a[i] > a[pivot]){
if ((a[i] > a[pivot]) && (a[j] <= a[pivot])){
int temp = a[i];
a[i] = a[j];
a[j] = temp;
i++;
} if (a[j] > a[pivot]){
j--;
}
}
}
int temp = a[i];
a[i] = a[pivot];
a[pivot] = temp;
return i;
}
void QuickSortRecursion::QuickSort(int a, int low, int high){
if (low >= high){
return;
}
int split = Partition (a, low, high);
QuickSort(a, low, split-1);
QuickSort(a, split+1, high);
}
c++
add a comment |
I am having issues in my main.cpp file where the program is telling me that Member reference base type 'int [11]' is not a structure or union
for both my QuickSort line and my for loop too. Then in the cout line it says Adding 'int' to a string does not append to the string and "Use array indexing to silence this warning
.
Below is my main.cpp file where I am having my issue.
#include <iostream>
#include "QuickSort.h"
using namespace std;
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F, 0, F.length-1);
for (int i = 0; i<F.length; i++){
cout << F[i] + " ";
}
return 0;
}
Just in case you need my other code to decipher.
Below is my QuickSort.h file:
using namespace std;
class QuickSortRecursion{
public:
QuickSortRecursion();
int Partition (int a, int low, int high);
void QuickSort(int a, int low, int high);
private:
};
Below is my QuickSort.cpp file:
QuickSortRecursion::QuickSortRecursion(){
return;
}
int QuickSortRecursion::Partition(int a, int low, int high){
int pivot = high;
int i = low;
int j = high;
while (i<j){
if (a[i] <= a[pivot]){
i++;
}if (a[i] > a[pivot]){
if ((a[i] > a[pivot]) && (a[j] <= a[pivot])){
int temp = a[i];
a[i] = a[j];
a[j] = temp;
i++;
} if (a[j] > a[pivot]){
j--;
}
}
}
int temp = a[i];
a[i] = a[pivot];
a[pivot] = temp;
return i;
}
void QuickSortRecursion::QuickSort(int a, int low, int high){
if (low >= high){
return;
}
int split = Partition (a, low, high);
QuickSort(a, low, split-1);
QuickSort(a, split+1, high);
}
c++
Side note: Your partitioning doesn't look quite right. You've got something that looks like a Lomuto Partition, but shouldn't need anything that looks likeif ((a[i] > a[pivot]) && (a[j] <= a[pivot]))
.
– user4581301
Nov 13 '18 at 3:33
add a comment |
I am having issues in my main.cpp file where the program is telling me that Member reference base type 'int [11]' is not a structure or union
for both my QuickSort line and my for loop too. Then in the cout line it says Adding 'int' to a string does not append to the string and "Use array indexing to silence this warning
.
Below is my main.cpp file where I am having my issue.
#include <iostream>
#include "QuickSort.h"
using namespace std;
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F, 0, F.length-1);
for (int i = 0; i<F.length; i++){
cout << F[i] + " ";
}
return 0;
}
Just in case you need my other code to decipher.
Below is my QuickSort.h file:
using namespace std;
class QuickSortRecursion{
public:
QuickSortRecursion();
int Partition (int a, int low, int high);
void QuickSort(int a, int low, int high);
private:
};
Below is my QuickSort.cpp file:
QuickSortRecursion::QuickSortRecursion(){
return;
}
int QuickSortRecursion::Partition(int a, int low, int high){
int pivot = high;
int i = low;
int j = high;
while (i<j){
if (a[i] <= a[pivot]){
i++;
}if (a[i] > a[pivot]){
if ((a[i] > a[pivot]) && (a[j] <= a[pivot])){
int temp = a[i];
a[i] = a[j];
a[j] = temp;
i++;
} if (a[j] > a[pivot]){
j--;
}
}
}
int temp = a[i];
a[i] = a[pivot];
a[pivot] = temp;
return i;
}
void QuickSortRecursion::QuickSort(int a, int low, int high){
if (low >= high){
return;
}
int split = Partition (a, low, high);
QuickSort(a, low, split-1);
QuickSort(a, split+1, high);
}
c++
I am having issues in my main.cpp file where the program is telling me that Member reference base type 'int [11]' is not a structure or union
for both my QuickSort line and my for loop too. Then in the cout line it says Adding 'int' to a string does not append to the string and "Use array indexing to silence this warning
.
Below is my main.cpp file where I am having my issue.
#include <iostream>
#include "QuickSort.h"
using namespace std;
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F, 0, F.length-1);
for (int i = 0; i<F.length; i++){
cout << F[i] + " ";
}
return 0;
}
Just in case you need my other code to decipher.
Below is my QuickSort.h file:
using namespace std;
class QuickSortRecursion{
public:
QuickSortRecursion();
int Partition (int a, int low, int high);
void QuickSort(int a, int low, int high);
private:
};
Below is my QuickSort.cpp file:
QuickSortRecursion::QuickSortRecursion(){
return;
}
int QuickSortRecursion::Partition(int a, int low, int high){
int pivot = high;
int i = low;
int j = high;
while (i<j){
if (a[i] <= a[pivot]){
i++;
}if (a[i] > a[pivot]){
if ((a[i] > a[pivot]) && (a[j] <= a[pivot])){
int temp = a[i];
a[i] = a[j];
a[j] = temp;
i++;
} if (a[j] > a[pivot]){
j--;
}
}
}
int temp = a[i];
a[i] = a[pivot];
a[pivot] = temp;
return i;
}
void QuickSortRecursion::QuickSort(int a, int low, int high){
if (low >= high){
return;
}
int split = Partition (a, low, high);
QuickSort(a, low, split-1);
QuickSort(a, split+1, high);
}
c++
c++
edited Nov 13 '18 at 5:24


Busy Bee
9591619
9591619
asked Nov 13 '18 at 3:23
coder6720
111
111
Side note: Your partitioning doesn't look quite right. You've got something that looks like a Lomuto Partition, but shouldn't need anything that looks likeif ((a[i] > a[pivot]) && (a[j] <= a[pivot]))
.
– user4581301
Nov 13 '18 at 3:33
add a comment |
Side note: Your partitioning doesn't look quite right. You've got something that looks like a Lomuto Partition, but shouldn't need anything that looks likeif ((a[i] > a[pivot]) && (a[j] <= a[pivot]))
.
– user4581301
Nov 13 '18 at 3:33
Side note: Your partitioning doesn't look quite right. You've got something that looks like a Lomuto Partition, but shouldn't need anything that looks like
if ((a[i] > a[pivot]) && (a[j] <= a[pivot]))
.– user4581301
Nov 13 '18 at 3:33
Side note: Your partitioning doesn't look quite right. You've got something that looks like a Lomuto Partition, but shouldn't need anything that looks like
if ((a[i] > a[pivot]) && (a[j] <= a[pivot]))
.– user4581301
Nov 13 '18 at 3:33
add a comment |
1 Answer
1
active
oldest
votes
In
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F, 0, F.length-1);
for (int i = 0; i<F.length; i++){
cout << F[i] + " ";
}
return 0;
}
F
is an array and arrays do not have any members so there is no F.length
.
Solutions (in order of personal preference)
Use std::size
if it is available (New in C++17).
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F, 0, std::size(F)-1);
for (int i = 0; i<std::size(F); i++){
cout << F[i] + " ";
}
return 0;
}
Use std::vector
instead of a raw array. std::array is more fitting, but I don't have a good formula to make
std::array<int> F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
^
Need to specify array size here, and if you knew that you there
wouldn't be a problem.
Example with std::vector
int main() {
std::vector<int> F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F.data(), 0, F.size()-1);
for (int i = 0; i<F.size(); i++){
cout << F[i] + " ";
}
return 0;
}
Use sizeof
to get the length in bytes and then divide it by the sizeof
an element to get the number of elements in the array
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
const int length = sizeof(F) / sizeof(F[0]);
QuickSort(F, 0, length -1);
for (int i = 0; i<length ; i++){
cout << F[i] + " ";
}
return 0;
}
Side Notes
If you have a constructor that does nothing, let the compiler generate it.
If you have a class with no state (member variables) consider making it a namespace
instead.
The partitioning doesn't look right. It looks like you're trying for Lomuto Partitioning, but are a little bit off.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53273326%2funsure-how-to-fix-member-reference-error%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
In
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F, 0, F.length-1);
for (int i = 0; i<F.length; i++){
cout << F[i] + " ";
}
return 0;
}
F
is an array and arrays do not have any members so there is no F.length
.
Solutions (in order of personal preference)
Use std::size
if it is available (New in C++17).
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F, 0, std::size(F)-1);
for (int i = 0; i<std::size(F); i++){
cout << F[i] + " ";
}
return 0;
}
Use std::vector
instead of a raw array. std::array is more fitting, but I don't have a good formula to make
std::array<int> F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
^
Need to specify array size here, and if you knew that you there
wouldn't be a problem.
Example with std::vector
int main() {
std::vector<int> F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F.data(), 0, F.size()-1);
for (int i = 0; i<F.size(); i++){
cout << F[i] + " ";
}
return 0;
}
Use sizeof
to get the length in bytes and then divide it by the sizeof
an element to get the number of elements in the array
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
const int length = sizeof(F) / sizeof(F[0]);
QuickSort(F, 0, length -1);
for (int i = 0; i<length ; i++){
cout << F[i] + " ";
}
return 0;
}
Side Notes
If you have a constructor that does nothing, let the compiler generate it.
If you have a class with no state (member variables) consider making it a namespace
instead.
The partitioning doesn't look right. It looks like you're trying for Lomuto Partitioning, but are a little bit off.
add a comment |
In
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F, 0, F.length-1);
for (int i = 0; i<F.length; i++){
cout << F[i] + " ";
}
return 0;
}
F
is an array and arrays do not have any members so there is no F.length
.
Solutions (in order of personal preference)
Use std::size
if it is available (New in C++17).
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F, 0, std::size(F)-1);
for (int i = 0; i<std::size(F); i++){
cout << F[i] + " ";
}
return 0;
}
Use std::vector
instead of a raw array. std::array is more fitting, but I don't have a good formula to make
std::array<int> F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
^
Need to specify array size here, and if you knew that you there
wouldn't be a problem.
Example with std::vector
int main() {
std::vector<int> F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F.data(), 0, F.size()-1);
for (int i = 0; i<F.size(); i++){
cout << F[i] + " ";
}
return 0;
}
Use sizeof
to get the length in bytes and then divide it by the sizeof
an element to get the number of elements in the array
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
const int length = sizeof(F) / sizeof(F[0]);
QuickSort(F, 0, length -1);
for (int i = 0; i<length ; i++){
cout << F[i] + " ";
}
return 0;
}
Side Notes
If you have a constructor that does nothing, let the compiler generate it.
If you have a class with no state (member variables) consider making it a namespace
instead.
The partitioning doesn't look right. It looks like you're trying for Lomuto Partitioning, but are a little bit off.
add a comment |
In
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F, 0, F.length-1);
for (int i = 0; i<F.length; i++){
cout << F[i] + " ";
}
return 0;
}
F
is an array and arrays do not have any members so there is no F.length
.
Solutions (in order of personal preference)
Use std::size
if it is available (New in C++17).
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F, 0, std::size(F)-1);
for (int i = 0; i<std::size(F); i++){
cout << F[i] + " ";
}
return 0;
}
Use std::vector
instead of a raw array. std::array is more fitting, but I don't have a good formula to make
std::array<int> F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
^
Need to specify array size here, and if you knew that you there
wouldn't be a problem.
Example with std::vector
int main() {
std::vector<int> F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F.data(), 0, F.size()-1);
for (int i = 0; i<F.size(); i++){
cout << F[i] + " ";
}
return 0;
}
Use sizeof
to get the length in bytes and then divide it by the sizeof
an element to get the number of elements in the array
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
const int length = sizeof(F) / sizeof(F[0]);
QuickSort(F, 0, length -1);
for (int i = 0; i<length ; i++){
cout << F[i] + " ";
}
return 0;
}
Side Notes
If you have a constructor that does nothing, let the compiler generate it.
If you have a class with no state (member variables) consider making it a namespace
instead.
The partitioning doesn't look right. It looks like you're trying for Lomuto Partitioning, but are a little bit off.
In
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F, 0, F.length-1);
for (int i = 0; i<F.length; i++){
cout << F[i] + " ";
}
return 0;
}
F
is an array and arrays do not have any members so there is no F.length
.
Solutions (in order of personal preference)
Use std::size
if it is available (New in C++17).
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F, 0, std::size(F)-1);
for (int i = 0; i<std::size(F); i++){
cout << F[i] + " ";
}
return 0;
}
Use std::vector
instead of a raw array. std::array is more fitting, but I don't have a good formula to make
std::array<int> F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
^
Need to specify array size here, and if you knew that you there
wouldn't be a problem.
Example with std::vector
int main() {
std::vector<int> F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
QuickSort(F.data(), 0, F.size()-1);
for (int i = 0; i<F.size(); i++){
cout << F[i] + " ";
}
return 0;
}
Use sizeof
to get the length in bytes and then divide it by the sizeof
an element to get the number of elements in the array
int main() {
int F = {12, 2, 16, 30, 8, 28, 4, 10, 20, 6, 18};
const int length = sizeof(F) / sizeof(F[0]);
QuickSort(F, 0, length -1);
for (int i = 0; i<length ; i++){
cout << F[i] + " ";
}
return 0;
}
Side Notes
If you have a constructor that does nothing, let the compiler generate it.
If you have a class with no state (member variables) consider making it a namespace
instead.
The partitioning doesn't look right. It looks like you're trying for Lomuto Partitioning, but are a little bit off.
answered Nov 13 '18 at 4:01
user4581301
19.5k51831
19.5k51831
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53273326%2funsure-how-to-fix-member-reference-error%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
U3FUsPpVM7TkWHo9OjTHyjJj91K6gh3N9bfqU,KzoMQOwA70MQaJIZIzl,x,dhYdbMiRx2hlRkpET524x6Hc3uXzTi kKPPPPWUn
Side note: Your partitioning doesn't look quite right. You've got something that looks like a Lomuto Partition, but shouldn't need anything that looks like
if ((a[i] > a[pivot]) && (a[j] <= a[pivot]))
.– user4581301
Nov 13 '18 at 3:33