Testing if a const modal component is called
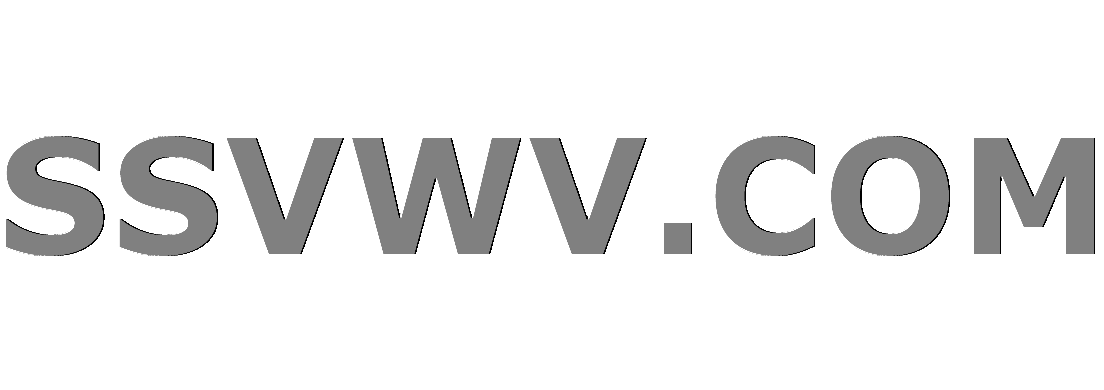
Multi tool use
I have a footer component which has several buttons on it. All of these buttons use the Message
const, which is an antd modal :
Message.jsx
import { Modal } from 'antd';
const { confirm } = Modal;
export const Message = (text, okayHandler, cancelHandler) => {
confirm({
title: text,
okText: 'Yes',
cancelText: 'No',
onOk: okayHandler,
onCancel: cancelHandler,
});
};
export default Message;
Footer.jsx
class Footer extends Component {
state = {
from: null,
redirectToReferrer: false,
};
cancelClicked = () => {
Message('Return to the previous screen?', () => {
this.setState({ redirectToReferrer: true, from: '/home' });
});
};
render() {
const { redirectToReferrer, from } = this.state;
if (redirectToReferrer) {
return <Redirect to={{ pathname: from }} />;
}
return (
<Card style={footerSyles}>
<Button
bounds={`${(3 * width) / 7 + (7 * width) / 84},5,${width / 7},30`}
text="CANCEL"
type="primary"
icon="close"
actionPerformed={this.cancelClicked}
/>
</Card>
//actionPerformed is actually onClick, it's taken as a prop from my <Button /> component. Card is an antd component while button is not.
I simply want to test when the Button is clicked, cancelClicked is called. If possible, I want to test if the state changes properly when 'OK' button is clicked on the Modal. My test is as follows, but the test fails because function is not called :
Footer.test
const defaultFooter = shallow(<Footer position="relative" bounds="10,0,775,100" />);
test('Footer should open a popup when cancel button is clicked, and redirect to home page', () => {
const instance = defaultFooter.instance();
const spy = jest.spyOn(instance, 'cancelClicked');
instance.forceUpdate();
const p = defaultFooter.find('Button[text="CANCEL"]');
p.simulate('click');
expect(spy).toHaveBeenCalled();
});
I've also tried with mount rather than shallow, but the spy is still not called.
reactjs jestjs enzyme antd
add a comment |
I have a footer component which has several buttons on it. All of these buttons use the Message
const, which is an antd modal :
Message.jsx
import { Modal } from 'antd';
const { confirm } = Modal;
export const Message = (text, okayHandler, cancelHandler) => {
confirm({
title: text,
okText: 'Yes',
cancelText: 'No',
onOk: okayHandler,
onCancel: cancelHandler,
});
};
export default Message;
Footer.jsx
class Footer extends Component {
state = {
from: null,
redirectToReferrer: false,
};
cancelClicked = () => {
Message('Return to the previous screen?', () => {
this.setState({ redirectToReferrer: true, from: '/home' });
});
};
render() {
const { redirectToReferrer, from } = this.state;
if (redirectToReferrer) {
return <Redirect to={{ pathname: from }} />;
}
return (
<Card style={footerSyles}>
<Button
bounds={`${(3 * width) / 7 + (7 * width) / 84},5,${width / 7},30`}
text="CANCEL"
type="primary"
icon="close"
actionPerformed={this.cancelClicked}
/>
</Card>
//actionPerformed is actually onClick, it's taken as a prop from my <Button /> component. Card is an antd component while button is not.
I simply want to test when the Button is clicked, cancelClicked is called. If possible, I want to test if the state changes properly when 'OK' button is clicked on the Modal. My test is as follows, but the test fails because function is not called :
Footer.test
const defaultFooter = shallow(<Footer position="relative" bounds="10,0,775,100" />);
test('Footer should open a popup when cancel button is clicked, and redirect to home page', () => {
const instance = defaultFooter.instance();
const spy = jest.spyOn(instance, 'cancelClicked');
instance.forceUpdate();
const p = defaultFooter.find('Button[text="CANCEL"]');
p.simulate('click');
expect(spy).toHaveBeenCalled();
});
I've also tried with mount rather than shallow, but the spy is still not called.
reactjs jestjs enzyme antd
1
where isButton
component coming from? it's not native<button>
– skyboyer
Nov 14 '18 at 14:50
perhaps need to replaceactionPerformed
withonClick
.
– Alex
Nov 14 '18 at 18:01
add a comment |
I have a footer component which has several buttons on it. All of these buttons use the Message
const, which is an antd modal :
Message.jsx
import { Modal } from 'antd';
const { confirm } = Modal;
export const Message = (text, okayHandler, cancelHandler) => {
confirm({
title: text,
okText: 'Yes',
cancelText: 'No',
onOk: okayHandler,
onCancel: cancelHandler,
});
};
export default Message;
Footer.jsx
class Footer extends Component {
state = {
from: null,
redirectToReferrer: false,
};
cancelClicked = () => {
Message('Return to the previous screen?', () => {
this.setState({ redirectToReferrer: true, from: '/home' });
});
};
render() {
const { redirectToReferrer, from } = this.state;
if (redirectToReferrer) {
return <Redirect to={{ pathname: from }} />;
}
return (
<Card style={footerSyles}>
<Button
bounds={`${(3 * width) / 7 + (7 * width) / 84},5,${width / 7},30`}
text="CANCEL"
type="primary"
icon="close"
actionPerformed={this.cancelClicked}
/>
</Card>
//actionPerformed is actually onClick, it's taken as a prop from my <Button /> component. Card is an antd component while button is not.
I simply want to test when the Button is clicked, cancelClicked is called. If possible, I want to test if the state changes properly when 'OK' button is clicked on the Modal. My test is as follows, but the test fails because function is not called :
Footer.test
const defaultFooter = shallow(<Footer position="relative" bounds="10,0,775,100" />);
test('Footer should open a popup when cancel button is clicked, and redirect to home page', () => {
const instance = defaultFooter.instance();
const spy = jest.spyOn(instance, 'cancelClicked');
instance.forceUpdate();
const p = defaultFooter.find('Button[text="CANCEL"]');
p.simulate('click');
expect(spy).toHaveBeenCalled();
});
I've also tried with mount rather than shallow, but the spy is still not called.
reactjs jestjs enzyme antd
I have a footer component which has several buttons on it. All of these buttons use the Message
const, which is an antd modal :
Message.jsx
import { Modal } from 'antd';
const { confirm } = Modal;
export const Message = (text, okayHandler, cancelHandler) => {
confirm({
title: text,
okText: 'Yes',
cancelText: 'No',
onOk: okayHandler,
onCancel: cancelHandler,
});
};
export default Message;
Footer.jsx
class Footer extends Component {
state = {
from: null,
redirectToReferrer: false,
};
cancelClicked = () => {
Message('Return to the previous screen?', () => {
this.setState({ redirectToReferrer: true, from: '/home' });
});
};
render() {
const { redirectToReferrer, from } = this.state;
if (redirectToReferrer) {
return <Redirect to={{ pathname: from }} />;
}
return (
<Card style={footerSyles}>
<Button
bounds={`${(3 * width) / 7 + (7 * width) / 84},5,${width / 7},30`}
text="CANCEL"
type="primary"
icon="close"
actionPerformed={this.cancelClicked}
/>
</Card>
//actionPerformed is actually onClick, it's taken as a prop from my <Button /> component. Card is an antd component while button is not.
I simply want to test when the Button is clicked, cancelClicked is called. If possible, I want to test if the state changes properly when 'OK' button is clicked on the Modal. My test is as follows, but the test fails because function is not called :
Footer.test
const defaultFooter = shallow(<Footer position="relative" bounds="10,0,775,100" />);
test('Footer should open a popup when cancel button is clicked, and redirect to home page', () => {
const instance = defaultFooter.instance();
const spy = jest.spyOn(instance, 'cancelClicked');
instance.forceUpdate();
const p = defaultFooter.find('Button[text="CANCEL"]');
p.simulate('click');
expect(spy).toHaveBeenCalled();
});
I've also tried with mount rather than shallow, but the spy is still not called.
reactjs jestjs enzyme antd
reactjs jestjs enzyme antd
edited Nov 14 '18 at 14:47
skyboyer
3,57611128
3,57611128
asked Nov 14 '18 at 7:25


HarveyHarvey
959
959
1
where isButton
component coming from? it's not native<button>
– skyboyer
Nov 14 '18 at 14:50
perhaps need to replaceactionPerformed
withonClick
.
– Alex
Nov 14 '18 at 18:01
add a comment |
1
where isButton
component coming from? it's not native<button>
– skyboyer
Nov 14 '18 at 14:50
perhaps need to replaceactionPerformed
withonClick
.
– Alex
Nov 14 '18 at 18:01
1
1
where is
Button
component coming from? it's not native <button>
– skyboyer
Nov 14 '18 at 14:50
where is
Button
component coming from? it's not native <button>
– skyboyer
Nov 14 '18 at 14:50
perhaps need to replace
actionPerformed
with onClick
.– Alex
Nov 14 '18 at 18:01
perhaps need to replace
actionPerformed
with onClick
.– Alex
Nov 14 '18 at 18:01
add a comment |
1 Answer
1
active
oldest
votes
The "Common Gotchas" section for simulate
says that "even though the name would imply this simulates an actual event, .simulate() will in fact target the component's prop based on the event you give it. For example, .simulate('click') will actually get the onClick prop and call it."
That behavior can be seen in the Enzyme
source code here and here.
So the line p.simulate('click');
ends up trying to call the onClick
prop of the Button
which doesn't exist so it essentially does nothing.
This post from an Airbnb dev recommends invoking props directly and avoiding simulate
.
In this case you would call the actionPerformed
property directly like this:
p.props().actionPerformed();
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53295027%2ftesting-if-a-const-modal-component-is-called%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The "Common Gotchas" section for simulate
says that "even though the name would imply this simulates an actual event, .simulate() will in fact target the component's prop based on the event you give it. For example, .simulate('click') will actually get the onClick prop and call it."
That behavior can be seen in the Enzyme
source code here and here.
So the line p.simulate('click');
ends up trying to call the onClick
prop of the Button
which doesn't exist so it essentially does nothing.
This post from an Airbnb dev recommends invoking props directly and avoiding simulate
.
In this case you would call the actionPerformed
property directly like this:
p.props().actionPerformed();
add a comment |
The "Common Gotchas" section for simulate
says that "even though the name would imply this simulates an actual event, .simulate() will in fact target the component's prop based on the event you give it. For example, .simulate('click') will actually get the onClick prop and call it."
That behavior can be seen in the Enzyme
source code here and here.
So the line p.simulate('click');
ends up trying to call the onClick
prop of the Button
which doesn't exist so it essentially does nothing.
This post from an Airbnb dev recommends invoking props directly and avoiding simulate
.
In this case you would call the actionPerformed
property directly like this:
p.props().actionPerformed();
add a comment |
The "Common Gotchas" section for simulate
says that "even though the name would imply this simulates an actual event, .simulate() will in fact target the component's prop based on the event you give it. For example, .simulate('click') will actually get the onClick prop and call it."
That behavior can be seen in the Enzyme
source code here and here.
So the line p.simulate('click');
ends up trying to call the onClick
prop of the Button
which doesn't exist so it essentially does nothing.
This post from an Airbnb dev recommends invoking props directly and avoiding simulate
.
In this case you would call the actionPerformed
property directly like this:
p.props().actionPerformed();
The "Common Gotchas" section for simulate
says that "even though the name would imply this simulates an actual event, .simulate() will in fact target the component's prop based on the event you give it. For example, .simulate('click') will actually get the onClick prop and call it."
That behavior can be seen in the Enzyme
source code here and here.
So the line p.simulate('click');
ends up trying to call the onClick
prop of the Button
which doesn't exist so it essentially does nothing.
This post from an Airbnb dev recommends invoking props directly and avoiding simulate
.
In this case you would call the actionPerformed
property directly like this:
p.props().actionPerformed();
answered Nov 15 '18 at 3:24


brian-lives-outdoorsbrian-lives-outdoors
5,6651322
5,6651322
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53295027%2ftesting-if-a-const-modal-component-is-called%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
p ur OXy
1
where is
Button
component coming from? it's not native<button>
– skyboyer
Nov 14 '18 at 14:50
perhaps need to replace
actionPerformed
withonClick
.– Alex
Nov 14 '18 at 18:01