Program only prints out second last letter. want the whole word backwards instead
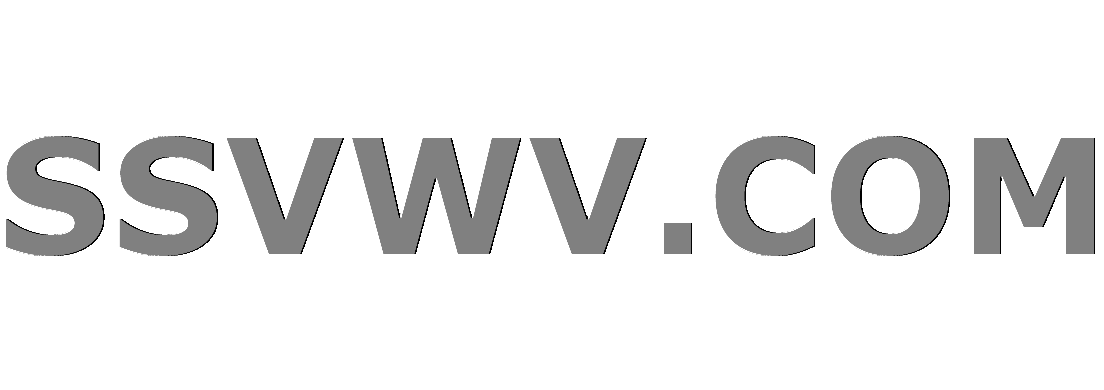
Multi tool use
import java.util.Scanner;
public class Project2 {
public static void main(String args) {
Scanner in = new Scanner(System.in);
String word;
String c;
int x, count, count1;
System.out.println("Please enter a word:");
word=in.nextLine();
x=word.length();
for(count=0;count<x;count++) {
count1=x;
count1--;
c=word.substring((count1)-1,count1);
System.out.println(c);
}
}
}
All this program does is print out the second to last character of the word that the user enters. I'm confused as to why it is doing this and want to know how to print out the whole word backwards. Someone help please.
java substring
add a comment |
import java.util.Scanner;
public class Project2 {
public static void main(String args) {
Scanner in = new Scanner(System.in);
String word;
String c;
int x, count, count1;
System.out.println("Please enter a word:");
word=in.nextLine();
x=word.length();
for(count=0;count<x;count++) {
count1=x;
count1--;
c=word.substring((count1)-1,count1);
System.out.println(c);
}
}
}
All this program does is print out the second to last character of the word that the user enters. I'm confused as to why it is doing this and want to know how to print out the whole word backwards. Someone help please.
java substring
When you need to traverse backward than traverse it backward.
– jack jay
Nov 14 '18 at 6:04
FWIW - ericlippert.com/2014/03/05/how-to-debug-small-programs
– cricket_007
Nov 14 '18 at 6:06
add a comment |
import java.util.Scanner;
public class Project2 {
public static void main(String args) {
Scanner in = new Scanner(System.in);
String word;
String c;
int x, count, count1;
System.out.println("Please enter a word:");
word=in.nextLine();
x=word.length();
for(count=0;count<x;count++) {
count1=x;
count1--;
c=word.substring((count1)-1,count1);
System.out.println(c);
}
}
}
All this program does is print out the second to last character of the word that the user enters. I'm confused as to why it is doing this and want to know how to print out the whole word backwards. Someone help please.
java substring
import java.util.Scanner;
public class Project2 {
public static void main(String args) {
Scanner in = new Scanner(System.in);
String word;
String c;
int x, count, count1;
System.out.println("Please enter a word:");
word=in.nextLine();
x=word.length();
for(count=0;count<x;count++) {
count1=x;
count1--;
c=word.substring((count1)-1,count1);
System.out.println(c);
}
}
}
All this program does is print out the second to last character of the word that the user enters. I'm confused as to why it is doing this and want to know how to print out the whole word backwards. Someone help please.
java substring
java substring
edited Nov 14 '18 at 7:12
Andreas
1,89421018
1,89421018
asked Nov 14 '18 at 6:01


Shrey VarmaShrey Varma
1
1
When you need to traverse backward than traverse it backward.
– jack jay
Nov 14 '18 at 6:04
FWIW - ericlippert.com/2014/03/05/how-to-debug-small-programs
– cricket_007
Nov 14 '18 at 6:06
add a comment |
When you need to traverse backward than traverse it backward.
– jack jay
Nov 14 '18 at 6:04
FWIW - ericlippert.com/2014/03/05/how-to-debug-small-programs
– cricket_007
Nov 14 '18 at 6:06
When you need to traverse backward than traverse it backward.
– jack jay
Nov 14 '18 at 6:04
When you need to traverse backward than traverse it backward.
– jack jay
Nov 14 '18 at 6:04
FWIW - ericlippert.com/2014/03/05/how-to-debug-small-programs
– cricket_007
Nov 14 '18 at 6:06
FWIW - ericlippert.com/2014/03/05/how-to-debug-small-programs
– cricket_007
Nov 14 '18 at 6:06
add a comment |
6 Answers
6
active
oldest
votes
You don't need a loop to reverse a string.
Ref - StringBuilder#reverse
Scanner in = new Scanner(System.in);
System.out.println(new StringBuilder(in.nextLine()).reverse());
If you want to print characters in reverse, then forget the substring-ing.
String word = in.nextLine();
int x = word.length();
for(count = x - 1; count >= 0; count--) {
System.out.println(word.charAt(count));
}
1
this is not an answer to the question.. if an alternative was to be provided, we could direct the OP to Reverse a string in Java
– Kartik
Nov 14 '18 at 6:10
Perhaps, but they did ask ultimately say want to know how to print out the whole word backwards, so your choice...
– cricket_007
Nov 14 '18 at 6:11
add a comment |
Take count1=x;
assignment out of the loop. Also make count--;
after printing the letter.
But then what do i make the starting value for count1?
– Shrey Varma
Nov 14 '18 at 6:04
I doubt this is the only fix becausec
is getting reassigned within the loop over and over again, never making a reversed string
– cricket_007
Nov 14 '18 at 6:08
It will print each letter of string in new line.
– Jignesh M. Khatri
Nov 14 '18 at 6:17
add a comment |
You are correct up until x = word.length()
. It is printing the second from last character because you keep setting the value of count1 to length of word
and you substract it by 1. Therefore, it keeps referring to the second last character. To fix that, do the following instead:
count1=x;
for(count=0;count<x;count++) {
c=word.substring((count1)-1,count1);
System.out.println(c);
count1--;
}
Please remember to accept the answer that suits your question the most, so StackOverflow can archive it properly.
– Andreas
Nov 14 '18 at 6:11
add a comment |
Every time the loop is running, you are resetting the count1 value to x (count1=x). So c will always be the same value.
To make this work, try taking count1 = x out of the loop so that every time the loop is running, count1 value will be reduced as expected providing the required sub-string.
add a comment |
Into the loop for(count=0;count<x;count++)
Every loop you did the same thing
count1=x;
count1--;
c=word.substring((count1)-1,count1);
System.out.println(c);
This block has no relation with the loop!
Thats why you are getting the second last character!
To fix this:
Solution 1: (Just reverse the String)
word=in.nextLine();
System.out.println(new StringBuilder(word).reverse());
or Solution 2: (Using loop using your code)
x=word.length();
for(count= x-1; count >= 0; count--) {
c = word.substring((count)-1, count);
System.out.print(c);
}
add a comment |
If at all you want to do it the hard way by traversing, do the following changes.
for(count=x;count>=0;count--) {
System.out.println(word.substring(count - 1,count));
}
Update: You can use charAt#String
to easily get the character at some position.
for(count=x-1;count>=0;count--) {
System.out.println(word.charAt(count));
}
substring(count - 1,count)
is the same effect ascharAt(count)
– cricket_007
Nov 14 '18 at 6:14
@cricket_007 yeah. Thanks for mentioning it.
– jack jay
Nov 14 '18 at 6:20
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53294022%2fprogram-only-prints-out-second-last-letter-want-the-whole-word-backwards-instea%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
You don't need a loop to reverse a string.
Ref - StringBuilder#reverse
Scanner in = new Scanner(System.in);
System.out.println(new StringBuilder(in.nextLine()).reverse());
If you want to print characters in reverse, then forget the substring-ing.
String word = in.nextLine();
int x = word.length();
for(count = x - 1; count >= 0; count--) {
System.out.println(word.charAt(count));
}
1
this is not an answer to the question.. if an alternative was to be provided, we could direct the OP to Reverse a string in Java
– Kartik
Nov 14 '18 at 6:10
Perhaps, but they did ask ultimately say want to know how to print out the whole word backwards, so your choice...
– cricket_007
Nov 14 '18 at 6:11
add a comment |
You don't need a loop to reverse a string.
Ref - StringBuilder#reverse
Scanner in = new Scanner(System.in);
System.out.println(new StringBuilder(in.nextLine()).reverse());
If you want to print characters in reverse, then forget the substring-ing.
String word = in.nextLine();
int x = word.length();
for(count = x - 1; count >= 0; count--) {
System.out.println(word.charAt(count));
}
1
this is not an answer to the question.. if an alternative was to be provided, we could direct the OP to Reverse a string in Java
– Kartik
Nov 14 '18 at 6:10
Perhaps, but they did ask ultimately say want to know how to print out the whole word backwards, so your choice...
– cricket_007
Nov 14 '18 at 6:11
add a comment |
You don't need a loop to reverse a string.
Ref - StringBuilder#reverse
Scanner in = new Scanner(System.in);
System.out.println(new StringBuilder(in.nextLine()).reverse());
If you want to print characters in reverse, then forget the substring-ing.
String word = in.nextLine();
int x = word.length();
for(count = x - 1; count >= 0; count--) {
System.out.println(word.charAt(count));
}
You don't need a loop to reverse a string.
Ref - StringBuilder#reverse
Scanner in = new Scanner(System.in);
System.out.println(new StringBuilder(in.nextLine()).reverse());
If you want to print characters in reverse, then forget the substring-ing.
String word = in.nextLine();
int x = word.length();
for(count = x - 1; count >= 0; count--) {
System.out.println(word.charAt(count));
}
edited Nov 14 '18 at 6:10
answered Nov 14 '18 at 6:04
cricket_007cricket_007
81.2k1142111
81.2k1142111
1
this is not an answer to the question.. if an alternative was to be provided, we could direct the OP to Reverse a string in Java
– Kartik
Nov 14 '18 at 6:10
Perhaps, but they did ask ultimately say want to know how to print out the whole word backwards, so your choice...
– cricket_007
Nov 14 '18 at 6:11
add a comment |
1
this is not an answer to the question.. if an alternative was to be provided, we could direct the OP to Reverse a string in Java
– Kartik
Nov 14 '18 at 6:10
Perhaps, but they did ask ultimately say want to know how to print out the whole word backwards, so your choice...
– cricket_007
Nov 14 '18 at 6:11
1
1
this is not an answer to the question.. if an alternative was to be provided, we could direct the OP to Reverse a string in Java
– Kartik
Nov 14 '18 at 6:10
this is not an answer to the question.. if an alternative was to be provided, we could direct the OP to Reverse a string in Java
– Kartik
Nov 14 '18 at 6:10
Perhaps, but they did ask ultimately say want to know how to print out the whole word backwards, so your choice...
– cricket_007
Nov 14 '18 at 6:11
Perhaps, but they did ask ultimately say want to know how to print out the whole word backwards, so your choice...
– cricket_007
Nov 14 '18 at 6:11
add a comment |
Take count1=x;
assignment out of the loop. Also make count--;
after printing the letter.
But then what do i make the starting value for count1?
– Shrey Varma
Nov 14 '18 at 6:04
I doubt this is the only fix becausec
is getting reassigned within the loop over and over again, never making a reversed string
– cricket_007
Nov 14 '18 at 6:08
It will print each letter of string in new line.
– Jignesh M. Khatri
Nov 14 '18 at 6:17
add a comment |
Take count1=x;
assignment out of the loop. Also make count--;
after printing the letter.
But then what do i make the starting value for count1?
– Shrey Varma
Nov 14 '18 at 6:04
I doubt this is the only fix becausec
is getting reassigned within the loop over and over again, never making a reversed string
– cricket_007
Nov 14 '18 at 6:08
It will print each letter of string in new line.
– Jignesh M. Khatri
Nov 14 '18 at 6:17
add a comment |
Take count1=x;
assignment out of the loop. Also make count--;
after printing the letter.
Take count1=x;
assignment out of the loop. Also make count--;
after printing the letter.
answered Nov 14 '18 at 6:02
Jignesh M. KhatriJignesh M. Khatri
501411
501411
But then what do i make the starting value for count1?
– Shrey Varma
Nov 14 '18 at 6:04
I doubt this is the only fix becausec
is getting reassigned within the loop over and over again, never making a reversed string
– cricket_007
Nov 14 '18 at 6:08
It will print each letter of string in new line.
– Jignesh M. Khatri
Nov 14 '18 at 6:17
add a comment |
But then what do i make the starting value for count1?
– Shrey Varma
Nov 14 '18 at 6:04
I doubt this is the only fix becausec
is getting reassigned within the loop over and over again, never making a reversed string
– cricket_007
Nov 14 '18 at 6:08
It will print each letter of string in new line.
– Jignesh M. Khatri
Nov 14 '18 at 6:17
But then what do i make the starting value for count1?
– Shrey Varma
Nov 14 '18 at 6:04
But then what do i make the starting value for count1?
– Shrey Varma
Nov 14 '18 at 6:04
I doubt this is the only fix because
c
is getting reassigned within the loop over and over again, never making a reversed string– cricket_007
Nov 14 '18 at 6:08
I doubt this is the only fix because
c
is getting reassigned within the loop over and over again, never making a reversed string– cricket_007
Nov 14 '18 at 6:08
It will print each letter of string in new line.
– Jignesh M. Khatri
Nov 14 '18 at 6:17
It will print each letter of string in new line.
– Jignesh M. Khatri
Nov 14 '18 at 6:17
add a comment |
You are correct up until x = word.length()
. It is printing the second from last character because you keep setting the value of count1 to length of word
and you substract it by 1. Therefore, it keeps referring to the second last character. To fix that, do the following instead:
count1=x;
for(count=0;count<x;count++) {
c=word.substring((count1)-1,count1);
System.out.println(c);
count1--;
}
Please remember to accept the answer that suits your question the most, so StackOverflow can archive it properly.
– Andreas
Nov 14 '18 at 6:11
add a comment |
You are correct up until x = word.length()
. It is printing the second from last character because you keep setting the value of count1 to length of word
and you substract it by 1. Therefore, it keeps referring to the second last character. To fix that, do the following instead:
count1=x;
for(count=0;count<x;count++) {
c=word.substring((count1)-1,count1);
System.out.println(c);
count1--;
}
Please remember to accept the answer that suits your question the most, so StackOverflow can archive it properly.
– Andreas
Nov 14 '18 at 6:11
add a comment |
You are correct up until x = word.length()
. It is printing the second from last character because you keep setting the value of count1 to length of word
and you substract it by 1. Therefore, it keeps referring to the second last character. To fix that, do the following instead:
count1=x;
for(count=0;count<x;count++) {
c=word.substring((count1)-1,count1);
System.out.println(c);
count1--;
}
You are correct up until x = word.length()
. It is printing the second from last character because you keep setting the value of count1 to length of word
and you substract it by 1. Therefore, it keeps referring to the second last character. To fix that, do the following instead:
count1=x;
for(count=0;count<x;count++) {
c=word.substring((count1)-1,count1);
System.out.println(c);
count1--;
}
answered Nov 14 '18 at 6:06
AndreasAndreas
1,89421018
1,89421018
Please remember to accept the answer that suits your question the most, so StackOverflow can archive it properly.
– Andreas
Nov 14 '18 at 6:11
add a comment |
Please remember to accept the answer that suits your question the most, so StackOverflow can archive it properly.
– Andreas
Nov 14 '18 at 6:11
Please remember to accept the answer that suits your question the most, so StackOverflow can archive it properly.
– Andreas
Nov 14 '18 at 6:11
Please remember to accept the answer that suits your question the most, so StackOverflow can archive it properly.
– Andreas
Nov 14 '18 at 6:11
add a comment |
Every time the loop is running, you are resetting the count1 value to x (count1=x). So c will always be the same value.
To make this work, try taking count1 = x out of the loop so that every time the loop is running, count1 value will be reduced as expected providing the required sub-string.
add a comment |
Every time the loop is running, you are resetting the count1 value to x (count1=x). So c will always be the same value.
To make this work, try taking count1 = x out of the loop so that every time the loop is running, count1 value will be reduced as expected providing the required sub-string.
add a comment |
Every time the loop is running, you are resetting the count1 value to x (count1=x). So c will always be the same value.
To make this work, try taking count1 = x out of the loop so that every time the loop is running, count1 value will be reduced as expected providing the required sub-string.
Every time the loop is running, you are resetting the count1 value to x (count1=x). So c will always be the same value.
To make this work, try taking count1 = x out of the loop so that every time the loop is running, count1 value will be reduced as expected providing the required sub-string.
answered Nov 14 '18 at 6:08


RakihthaRRRakihthaRR
185111
185111
add a comment |
add a comment |
Into the loop for(count=0;count<x;count++)
Every loop you did the same thing
count1=x;
count1--;
c=word.substring((count1)-1,count1);
System.out.println(c);
This block has no relation with the loop!
Thats why you are getting the second last character!
To fix this:
Solution 1: (Just reverse the String)
word=in.nextLine();
System.out.println(new StringBuilder(word).reverse());
or Solution 2: (Using loop using your code)
x=word.length();
for(count= x-1; count >= 0; count--) {
c = word.substring((count)-1, count);
System.out.print(c);
}
add a comment |
Into the loop for(count=0;count<x;count++)
Every loop you did the same thing
count1=x;
count1--;
c=word.substring((count1)-1,count1);
System.out.println(c);
This block has no relation with the loop!
Thats why you are getting the second last character!
To fix this:
Solution 1: (Just reverse the String)
word=in.nextLine();
System.out.println(new StringBuilder(word).reverse());
or Solution 2: (Using loop using your code)
x=word.length();
for(count= x-1; count >= 0; count--) {
c = word.substring((count)-1, count);
System.out.print(c);
}
add a comment |
Into the loop for(count=0;count<x;count++)
Every loop you did the same thing
count1=x;
count1--;
c=word.substring((count1)-1,count1);
System.out.println(c);
This block has no relation with the loop!
Thats why you are getting the second last character!
To fix this:
Solution 1: (Just reverse the String)
word=in.nextLine();
System.out.println(new StringBuilder(word).reverse());
or Solution 2: (Using loop using your code)
x=word.length();
for(count= x-1; count >= 0; count--) {
c = word.substring((count)-1, count);
System.out.print(c);
}
Into the loop for(count=0;count<x;count++)
Every loop you did the same thing
count1=x;
count1--;
c=word.substring((count1)-1,count1);
System.out.println(c);
This block has no relation with the loop!
Thats why you are getting the second last character!
To fix this:
Solution 1: (Just reverse the String)
word=in.nextLine();
System.out.println(new StringBuilder(word).reverse());
or Solution 2: (Using loop using your code)
x=word.length();
for(count= x-1; count >= 0; count--) {
c = word.substring((count)-1, count);
System.out.print(c);
}
edited Nov 14 '18 at 6:18
answered Nov 14 '18 at 6:12


ZicoZico
1,74411620
1,74411620
add a comment |
add a comment |
If at all you want to do it the hard way by traversing, do the following changes.
for(count=x;count>=0;count--) {
System.out.println(word.substring(count - 1,count));
}
Update: You can use charAt#String
to easily get the character at some position.
for(count=x-1;count>=0;count--) {
System.out.println(word.charAt(count));
}
substring(count - 1,count)
is the same effect ascharAt(count)
– cricket_007
Nov 14 '18 at 6:14
@cricket_007 yeah. Thanks for mentioning it.
– jack jay
Nov 14 '18 at 6:20
add a comment |
If at all you want to do it the hard way by traversing, do the following changes.
for(count=x;count>=0;count--) {
System.out.println(word.substring(count - 1,count));
}
Update: You can use charAt#String
to easily get the character at some position.
for(count=x-1;count>=0;count--) {
System.out.println(word.charAt(count));
}
substring(count - 1,count)
is the same effect ascharAt(count)
– cricket_007
Nov 14 '18 at 6:14
@cricket_007 yeah. Thanks for mentioning it.
– jack jay
Nov 14 '18 at 6:20
add a comment |
If at all you want to do it the hard way by traversing, do the following changes.
for(count=x;count>=0;count--) {
System.out.println(word.substring(count - 1,count));
}
Update: You can use charAt#String
to easily get the character at some position.
for(count=x-1;count>=0;count--) {
System.out.println(word.charAt(count));
}
If at all you want to do it the hard way by traversing, do the following changes.
for(count=x;count>=0;count--) {
System.out.println(word.substring(count - 1,count));
}
Update: You can use charAt#String
to easily get the character at some position.
for(count=x-1;count>=0;count--) {
System.out.println(word.charAt(count));
}
edited Nov 14 '18 at 6:21
answered Nov 14 '18 at 6:06


jack jayjack jay
2,2121824
2,2121824
substring(count - 1,count)
is the same effect ascharAt(count)
– cricket_007
Nov 14 '18 at 6:14
@cricket_007 yeah. Thanks for mentioning it.
– jack jay
Nov 14 '18 at 6:20
add a comment |
substring(count - 1,count)
is the same effect ascharAt(count)
– cricket_007
Nov 14 '18 at 6:14
@cricket_007 yeah. Thanks for mentioning it.
– jack jay
Nov 14 '18 at 6:20
substring(count - 1,count)
is the same effect as charAt(count)
– cricket_007
Nov 14 '18 at 6:14
substring(count - 1,count)
is the same effect as charAt(count)
– cricket_007
Nov 14 '18 at 6:14
@cricket_007 yeah. Thanks for mentioning it.
– jack jay
Nov 14 '18 at 6:20
@cricket_007 yeah. Thanks for mentioning it.
– jack jay
Nov 14 '18 at 6:20
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53294022%2fprogram-only-prints-out-second-last-letter-want-the-whole-word-backwards-instea%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
owifV cUyv7ey2Zki,T5n A
When you need to traverse backward than traverse it backward.
– jack jay
Nov 14 '18 at 6:04
FWIW - ericlippert.com/2014/03/05/how-to-debug-small-programs
– cricket_007
Nov 14 '18 at 6:06