OpenGL Move camera around point
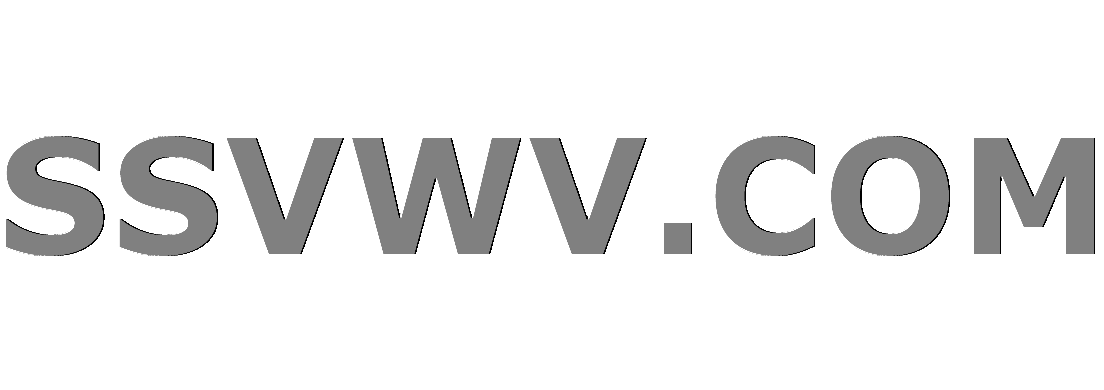
Multi tool use
I initialize the camera class from the eye, target and up vectors with glm::lookAt
function, then I get Yaw, Pitch and Roll values from glm::yaw
and so on.
I made a rotate method that takes xoffset
and yoffset
to rotate the camera around its target point.
void ThirdPersonCamera::Rotate(float _yaw, float _pitch) {
view.yaw += _yaw;
view.pitch += _pitch;
float radius = glm::length(view.GetEye() - view.GetTarget());
view.GetEye().x = glm::cos(glm::radians(view.yaw)) * glm::cos(glm::radians(view.pitch)) * radius;
view.GetEye().y = glm::sin(glm::radians(view.pitch)) * radius;
view.GetEye().z = glm::sin(glm::radians(view.yaw)) * glm::cos(glm::radians(view.pitch)) * radius;
view.init();
}
where view.init()
creates the view matrix from lookAt
.
the problem is that for the first rotation the eye X and Z values are exchanged so the camera jumps from its place to another one for example if the camera initialized at (0,10,10) then after first movement the eye became at (10,10,0) then it works fine.
c++ opengl rotation glm-math euler-angles
add a comment |
I initialize the camera class from the eye, target and up vectors with glm::lookAt
function, then I get Yaw, Pitch and Roll values from glm::yaw
and so on.
I made a rotate method that takes xoffset
and yoffset
to rotate the camera around its target point.
void ThirdPersonCamera::Rotate(float _yaw, float _pitch) {
view.yaw += _yaw;
view.pitch += _pitch;
float radius = glm::length(view.GetEye() - view.GetTarget());
view.GetEye().x = glm::cos(glm::radians(view.yaw)) * glm::cos(glm::radians(view.pitch)) * radius;
view.GetEye().y = glm::sin(glm::radians(view.pitch)) * radius;
view.GetEye().z = glm::sin(glm::radians(view.yaw)) * glm::cos(glm::radians(view.pitch)) * radius;
view.init();
}
where view.init()
creates the view matrix from lookAt
.
the problem is that for the first rotation the eye X and Z values are exchanged so the camera jumps from its place to another one for example if the camera initialized at (0,10,10) then after first movement the eye became at (10,10,0) then it works fine.
c++ opengl rotation glm-math euler-angles
Yes I know, so what is the wrong in calculations? I tried get the Yaw and Pitch from the inverse of the view matrix after initializing but I got the Y in negative .
– Mohamed Moussa
Nov 13 '18 at 13:44
add a comment |
I initialize the camera class from the eye, target and up vectors with glm::lookAt
function, then I get Yaw, Pitch and Roll values from glm::yaw
and so on.
I made a rotate method that takes xoffset
and yoffset
to rotate the camera around its target point.
void ThirdPersonCamera::Rotate(float _yaw, float _pitch) {
view.yaw += _yaw;
view.pitch += _pitch;
float radius = glm::length(view.GetEye() - view.GetTarget());
view.GetEye().x = glm::cos(glm::radians(view.yaw)) * glm::cos(glm::radians(view.pitch)) * radius;
view.GetEye().y = glm::sin(glm::radians(view.pitch)) * radius;
view.GetEye().z = glm::sin(glm::radians(view.yaw)) * glm::cos(glm::radians(view.pitch)) * radius;
view.init();
}
where view.init()
creates the view matrix from lookAt
.
the problem is that for the first rotation the eye X and Z values are exchanged so the camera jumps from its place to another one for example if the camera initialized at (0,10,10) then after first movement the eye became at (10,10,0) then it works fine.
c++ opengl rotation glm-math euler-angles
I initialize the camera class from the eye, target and up vectors with glm::lookAt
function, then I get Yaw, Pitch and Roll values from glm::yaw
and so on.
I made a rotate method that takes xoffset
and yoffset
to rotate the camera around its target point.
void ThirdPersonCamera::Rotate(float _yaw, float _pitch) {
view.yaw += _yaw;
view.pitch += _pitch;
float radius = glm::length(view.GetEye() - view.GetTarget());
view.GetEye().x = glm::cos(glm::radians(view.yaw)) * glm::cos(glm::radians(view.pitch)) * radius;
view.GetEye().y = glm::sin(glm::radians(view.pitch)) * radius;
view.GetEye().z = glm::sin(glm::radians(view.yaw)) * glm::cos(glm::radians(view.pitch)) * radius;
view.init();
}
where view.init()
creates the view matrix from lookAt
.
the problem is that for the first rotation the eye X and Z values are exchanged so the camera jumps from its place to another one for example if the camera initialized at (0,10,10) then after first movement the eye became at (10,10,0) then it works fine.
c++ opengl rotation glm-math euler-angles
c++ opengl rotation glm-math euler-angles
edited Nov 13 '18 at 14:32
Nicol Bolas
283k33468643
283k33468643
asked Nov 13 '18 at 12:52


Mohamed MoussaMohamed Moussa
378
378
Yes I know, so what is the wrong in calculations? I tried get the Yaw and Pitch from the inverse of the view matrix after initializing but I got the Y in negative .
– Mohamed Moussa
Nov 13 '18 at 13:44
add a comment |
Yes I know, so what is the wrong in calculations? I tried get the Yaw and Pitch from the inverse of the view matrix after initializing but I got the Y in negative .
– Mohamed Moussa
Nov 13 '18 at 13:44
Yes I know, so what is the wrong in calculations? I tried get the Yaw and Pitch from the inverse of the view matrix after initializing but I got the Y in negative .
– Mohamed Moussa
Nov 13 '18 at 13:44
Yes I know, so what is the wrong in calculations? I tried get the Yaw and Pitch from the inverse of the view matrix after initializing but I got the Y in negative .
– Mohamed Moussa
Nov 13 '18 at 13:44
add a comment |
1 Answer
1
active
oldest
votes
Obviously, glm::yaw()
and so on do not do what you need. You need the inverse of your calculations for the eye. That is:
auto d = eye - target;
yaw = std::atan2(d.z, d.x);
pitch = std::asin(d.y / glm::length(d));
For the last line, make sure that the argument to asin
stays within [-1, 1]
. Floating-point inaccuracies might produce an argument outside of this range.
Solved, Thank you
– Mohamed Moussa
Nov 13 '18 at 16:54
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53281436%2fopengl-move-camera-around-point%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Obviously, glm::yaw()
and so on do not do what you need. You need the inverse of your calculations for the eye. That is:
auto d = eye - target;
yaw = std::atan2(d.z, d.x);
pitch = std::asin(d.y / glm::length(d));
For the last line, make sure that the argument to asin
stays within [-1, 1]
. Floating-point inaccuracies might produce an argument outside of this range.
Solved, Thank you
– Mohamed Moussa
Nov 13 '18 at 16:54
add a comment |
Obviously, glm::yaw()
and so on do not do what you need. You need the inverse of your calculations for the eye. That is:
auto d = eye - target;
yaw = std::atan2(d.z, d.x);
pitch = std::asin(d.y / glm::length(d));
For the last line, make sure that the argument to asin
stays within [-1, 1]
. Floating-point inaccuracies might produce an argument outside of this range.
Solved, Thank you
– Mohamed Moussa
Nov 13 '18 at 16:54
add a comment |
Obviously, glm::yaw()
and so on do not do what you need. You need the inverse of your calculations for the eye. That is:
auto d = eye - target;
yaw = std::atan2(d.z, d.x);
pitch = std::asin(d.y / glm::length(d));
For the last line, make sure that the argument to asin
stays within [-1, 1]
. Floating-point inaccuracies might produce an argument outside of this range.
Obviously, glm::yaw()
and so on do not do what you need. You need the inverse of your calculations for the eye. That is:
auto d = eye - target;
yaw = std::atan2(d.z, d.x);
pitch = std::asin(d.y / glm::length(d));
For the last line, make sure that the argument to asin
stays within [-1, 1]
. Floating-point inaccuracies might produce an argument outside of this range.
answered Nov 13 '18 at 16:42
Nico SchertlerNico Schertler
25.1k42350
25.1k42350
Solved, Thank you
– Mohamed Moussa
Nov 13 '18 at 16:54
add a comment |
Solved, Thank you
– Mohamed Moussa
Nov 13 '18 at 16:54
Solved, Thank you
– Mohamed Moussa
Nov 13 '18 at 16:54
Solved, Thank you
– Mohamed Moussa
Nov 13 '18 at 16:54
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53281436%2fopengl-move-camera-around-point%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KZTkX6aKzGod4ES w
Yes I know, so what is the wrong in calculations? I tried get the Yaw and Pitch from the inverse of the view matrix after initializing but I got the Y in negative .
– Mohamed Moussa
Nov 13 '18 at 13:44