How to output selected column values
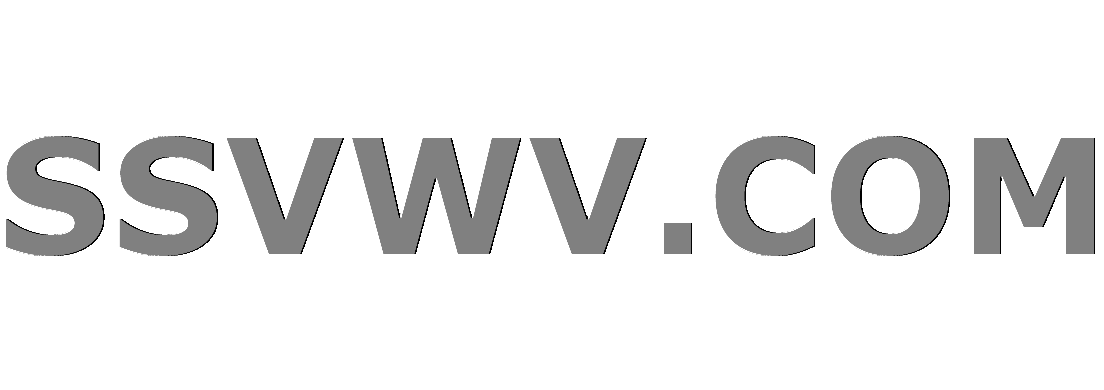
Multi tool use
In a single model Person
there is an address information. But since we are not separating this yet to another table. I would like to only query the address information out of Person
table. Would it be possible using hybrid_property
If not what else do I need to achieve this stuff?
I wanna avoid this one:
db.session.query(Person.id, Person.address_secret_id, Person.address_name).get(pk)
The model
class Person(db.Model):
# some lengthy information
# address
address_secret_id = db.Column(db.Unicode, nullable=True)
address_name = db.Column(db.Unicode, nullable=True)
@hybrid_property
def address(self):
# I never tested this but i know this is wrong.
return self.id + self.address_secret_id + self.address_name
Usage:
db.session.query(Person.address).get(pk)
Expected Output:
{id: 1, address_secret_id: xxxx, address_name: 'forgetmeland'}
How can I achieve an output that is only retrieving the desired field? It doesn't need to be dict or tuple as long as Im getting what is needed.
python sqlalchemy
add a comment |
In a single model Person
there is an address information. But since we are not separating this yet to another table. I would like to only query the address information out of Person
table. Would it be possible using hybrid_property
If not what else do I need to achieve this stuff?
I wanna avoid this one:
db.session.query(Person.id, Person.address_secret_id, Person.address_name).get(pk)
The model
class Person(db.Model):
# some lengthy information
# address
address_secret_id = db.Column(db.Unicode, nullable=True)
address_name = db.Column(db.Unicode, nullable=True)
@hybrid_property
def address(self):
# I never tested this but i know this is wrong.
return self.id + self.address_secret_id + self.address_name
Usage:
db.session.query(Person.address).get(pk)
Expected Output:
{id: 1, address_secret_id: xxxx, address_name: 'forgetmeland'}
How can I achieve an output that is only retrieving the desired field? It doesn't need to be dict or tuple as long as Im getting what is needed.
python sqlalchemy
Are you trying to avoid typingdb.session.query(Person.id, Person.address_secret_id, Person.address_name)
?
– SuperShoot
Nov 13 '18 at 12:59
The answer to your title is "yes". The rest is unclear. Why would you expect a dictionary as output? How come the components are separated in the dictionary, though you're trying to query the hybrid that combines them?
– Ilja Everilä
Nov 13 '18 at 13:10
@SuperShoot true!
– Roel
Nov 13 '18 at 13:21
@IljaEverilä sorry for not making it clear. That is only an example. It could be any other type as long as im getting only the same fields
– Roel
Nov 13 '18 at 13:22
add a comment |
In a single model Person
there is an address information. But since we are not separating this yet to another table. I would like to only query the address information out of Person
table. Would it be possible using hybrid_property
If not what else do I need to achieve this stuff?
I wanna avoid this one:
db.session.query(Person.id, Person.address_secret_id, Person.address_name).get(pk)
The model
class Person(db.Model):
# some lengthy information
# address
address_secret_id = db.Column(db.Unicode, nullable=True)
address_name = db.Column(db.Unicode, nullable=True)
@hybrid_property
def address(self):
# I never tested this but i know this is wrong.
return self.id + self.address_secret_id + self.address_name
Usage:
db.session.query(Person.address).get(pk)
Expected Output:
{id: 1, address_secret_id: xxxx, address_name: 'forgetmeland'}
How can I achieve an output that is only retrieving the desired field? It doesn't need to be dict or tuple as long as Im getting what is needed.
python sqlalchemy
In a single model Person
there is an address information. But since we are not separating this yet to another table. I would like to only query the address information out of Person
table. Would it be possible using hybrid_property
If not what else do I need to achieve this stuff?
I wanna avoid this one:
db.session.query(Person.id, Person.address_secret_id, Person.address_name).get(pk)
The model
class Person(db.Model):
# some lengthy information
# address
address_secret_id = db.Column(db.Unicode, nullable=True)
address_name = db.Column(db.Unicode, nullable=True)
@hybrid_property
def address(self):
# I never tested this but i know this is wrong.
return self.id + self.address_secret_id + self.address_name
Usage:
db.session.query(Person.address).get(pk)
Expected Output:
{id: 1, address_secret_id: xxxx, address_name: 'forgetmeland'}
How can I achieve an output that is only retrieving the desired field? It doesn't need to be dict or tuple as long as Im getting what is needed.
python sqlalchemy
python sqlalchemy
edited Nov 13 '18 at 15:20
SuperShoot
1,679619
1,679619
asked Nov 13 '18 at 12:48


RoelRoel
2,00122058
2,00122058
Are you trying to avoid typingdb.session.query(Person.id, Person.address_secret_id, Person.address_name)
?
– SuperShoot
Nov 13 '18 at 12:59
The answer to your title is "yes". The rest is unclear. Why would you expect a dictionary as output? How come the components are separated in the dictionary, though you're trying to query the hybrid that combines them?
– Ilja Everilä
Nov 13 '18 at 13:10
@SuperShoot true!
– Roel
Nov 13 '18 at 13:21
@IljaEverilä sorry for not making it clear. That is only an example. It could be any other type as long as im getting only the same fields
– Roel
Nov 13 '18 at 13:22
add a comment |
Are you trying to avoid typingdb.session.query(Person.id, Person.address_secret_id, Person.address_name)
?
– SuperShoot
Nov 13 '18 at 12:59
The answer to your title is "yes". The rest is unclear. Why would you expect a dictionary as output? How come the components are separated in the dictionary, though you're trying to query the hybrid that combines them?
– Ilja Everilä
Nov 13 '18 at 13:10
@SuperShoot true!
– Roel
Nov 13 '18 at 13:21
@IljaEverilä sorry for not making it clear. That is only an example. It could be any other type as long as im getting only the same fields
– Roel
Nov 13 '18 at 13:22
Are you trying to avoid typing
db.session.query(Person.id, Person.address_secret_id, Person.address_name)
?– SuperShoot
Nov 13 '18 at 12:59
Are you trying to avoid typing
db.session.query(Person.id, Person.address_secret_id, Person.address_name)
?– SuperShoot
Nov 13 '18 at 12:59
The answer to your title is "yes". The rest is unclear. Why would you expect a dictionary as output? How come the components are separated in the dictionary, though you're trying to query the hybrid that combines them?
– Ilja Everilä
Nov 13 '18 at 13:10
The answer to your title is "yes". The rest is unclear. Why would you expect a dictionary as output? How come the components are separated in the dictionary, though you're trying to query the hybrid that combines them?
– Ilja Everilä
Nov 13 '18 at 13:10
@SuperShoot true!
– Roel
Nov 13 '18 at 13:21
@SuperShoot true!
– Roel
Nov 13 '18 at 13:21
@IljaEverilä sorry for not making it clear. That is only an example. It could be any other type as long as im getting only the same fields
– Roel
Nov 13 '18 at 13:22
@IljaEverilä sorry for not making it clear. That is only an example. It could be any other type as long as im getting only the same fields
– Roel
Nov 13 '18 at 13:22
add a comment |
1 Answer
1
active
oldest
votes
If you are trying to avoid having to type db.session.query(Person.id, Person.address_secret_id, Person.address_name)
, just add an address_details
property on the person model.
class Person(db.Model):
# some lengthy information
# address
address_secret_id = db.Column(db.Unicode, nullable=True)
address_name = db.Column(db.Unicode, nullable=True)
@property
def address_details(self):
keys = ('id', 'address_secret_id', 'address_name')
return {k: getattr(self, k) for k in in keys}
Probably less lines of code than trying to use some sort of hybrid query, and still just the one trip to the database.
Query would be:
Person.query.get(1).address_details
Is there no other way in query() level?
– Roel
Nov 13 '18 at 14:06
Yes, but you are trying to avoid it.
– SuperShoot
Nov 13 '18 at 14:10
I see so there is no otherway in doing this using hybrid_property
– Roel
Nov 13 '18 at 14:13
1
There might be, but what would be the advantage of adding the complexity?
– SuperShoot
Nov 13 '18 at 14:16
actually in the real model there are a lot of field that is need to be refactored. So for the time being we need to come up with a temporary solution. and that is not costly in sql level
– Roel
Nov 13 '18 at 14:40
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53281365%2fhow-to-output-selected-column-values%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you are trying to avoid having to type db.session.query(Person.id, Person.address_secret_id, Person.address_name)
, just add an address_details
property on the person model.
class Person(db.Model):
# some lengthy information
# address
address_secret_id = db.Column(db.Unicode, nullable=True)
address_name = db.Column(db.Unicode, nullable=True)
@property
def address_details(self):
keys = ('id', 'address_secret_id', 'address_name')
return {k: getattr(self, k) for k in in keys}
Probably less lines of code than trying to use some sort of hybrid query, and still just the one trip to the database.
Query would be:
Person.query.get(1).address_details
Is there no other way in query() level?
– Roel
Nov 13 '18 at 14:06
Yes, but you are trying to avoid it.
– SuperShoot
Nov 13 '18 at 14:10
I see so there is no otherway in doing this using hybrid_property
– Roel
Nov 13 '18 at 14:13
1
There might be, but what would be the advantage of adding the complexity?
– SuperShoot
Nov 13 '18 at 14:16
actually in the real model there are a lot of field that is need to be refactored. So for the time being we need to come up with a temporary solution. and that is not costly in sql level
– Roel
Nov 13 '18 at 14:40
add a comment |
If you are trying to avoid having to type db.session.query(Person.id, Person.address_secret_id, Person.address_name)
, just add an address_details
property on the person model.
class Person(db.Model):
# some lengthy information
# address
address_secret_id = db.Column(db.Unicode, nullable=True)
address_name = db.Column(db.Unicode, nullable=True)
@property
def address_details(self):
keys = ('id', 'address_secret_id', 'address_name')
return {k: getattr(self, k) for k in in keys}
Probably less lines of code than trying to use some sort of hybrid query, and still just the one trip to the database.
Query would be:
Person.query.get(1).address_details
Is there no other way in query() level?
– Roel
Nov 13 '18 at 14:06
Yes, but you are trying to avoid it.
– SuperShoot
Nov 13 '18 at 14:10
I see so there is no otherway in doing this using hybrid_property
– Roel
Nov 13 '18 at 14:13
1
There might be, but what would be the advantage of adding the complexity?
– SuperShoot
Nov 13 '18 at 14:16
actually in the real model there are a lot of field that is need to be refactored. So for the time being we need to come up with a temporary solution. and that is not costly in sql level
– Roel
Nov 13 '18 at 14:40
add a comment |
If you are trying to avoid having to type db.session.query(Person.id, Person.address_secret_id, Person.address_name)
, just add an address_details
property on the person model.
class Person(db.Model):
# some lengthy information
# address
address_secret_id = db.Column(db.Unicode, nullable=True)
address_name = db.Column(db.Unicode, nullable=True)
@property
def address_details(self):
keys = ('id', 'address_secret_id', 'address_name')
return {k: getattr(self, k) for k in in keys}
Probably less lines of code than trying to use some sort of hybrid query, and still just the one trip to the database.
Query would be:
Person.query.get(1).address_details
If you are trying to avoid having to type db.session.query(Person.id, Person.address_secret_id, Person.address_name)
, just add an address_details
property on the person model.
class Person(db.Model):
# some lengthy information
# address
address_secret_id = db.Column(db.Unicode, nullable=True)
address_name = db.Column(db.Unicode, nullable=True)
@property
def address_details(self):
keys = ('id', 'address_secret_id', 'address_name')
return {k: getattr(self, k) for k in in keys}
Probably less lines of code than trying to use some sort of hybrid query, and still just the one trip to the database.
Query would be:
Person.query.get(1).address_details
edited Nov 13 '18 at 14:23
answered Nov 13 '18 at 13:13
SuperShootSuperShoot
1,679619
1,679619
Is there no other way in query() level?
– Roel
Nov 13 '18 at 14:06
Yes, but you are trying to avoid it.
– SuperShoot
Nov 13 '18 at 14:10
I see so there is no otherway in doing this using hybrid_property
– Roel
Nov 13 '18 at 14:13
1
There might be, but what would be the advantage of adding the complexity?
– SuperShoot
Nov 13 '18 at 14:16
actually in the real model there are a lot of field that is need to be refactored. So for the time being we need to come up with a temporary solution. and that is not costly in sql level
– Roel
Nov 13 '18 at 14:40
add a comment |
Is there no other way in query() level?
– Roel
Nov 13 '18 at 14:06
Yes, but you are trying to avoid it.
– SuperShoot
Nov 13 '18 at 14:10
I see so there is no otherway in doing this using hybrid_property
– Roel
Nov 13 '18 at 14:13
1
There might be, but what would be the advantage of adding the complexity?
– SuperShoot
Nov 13 '18 at 14:16
actually in the real model there are a lot of field that is need to be refactored. So for the time being we need to come up with a temporary solution. and that is not costly in sql level
– Roel
Nov 13 '18 at 14:40
Is there no other way in query() level?
– Roel
Nov 13 '18 at 14:06
Is there no other way in query() level?
– Roel
Nov 13 '18 at 14:06
Yes, but you are trying to avoid it.
– SuperShoot
Nov 13 '18 at 14:10
Yes, but you are trying to avoid it.
– SuperShoot
Nov 13 '18 at 14:10
I see so there is no otherway in doing this using hybrid_property
– Roel
Nov 13 '18 at 14:13
I see so there is no otherway in doing this using hybrid_property
– Roel
Nov 13 '18 at 14:13
1
1
There might be, but what would be the advantage of adding the complexity?
– SuperShoot
Nov 13 '18 at 14:16
There might be, but what would be the advantage of adding the complexity?
– SuperShoot
Nov 13 '18 at 14:16
actually in the real model there are a lot of field that is need to be refactored. So for the time being we need to come up with a temporary solution. and that is not costly in sql level
– Roel
Nov 13 '18 at 14:40
actually in the real model there are a lot of field that is need to be refactored. So for the time being we need to come up with a temporary solution. and that is not costly in sql level
– Roel
Nov 13 '18 at 14:40
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53281365%2fhow-to-output-selected-column-values%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Nk1mqFmCmLDVfNgjv LUwfeN1FOEkhalsS4Y6Eh194sSTSQLJ KfQH emEO,Wx yhnDqDR1Pqt1Cl89Tzn,H
Are you trying to avoid typing
db.session.query(Person.id, Person.address_secret_id, Person.address_name)
?– SuperShoot
Nov 13 '18 at 12:59
The answer to your title is "yes". The rest is unclear. Why would you expect a dictionary as output? How come the components are separated in the dictionary, though you're trying to query the hybrid that combines them?
– Ilja Everilä
Nov 13 '18 at 13:10
@SuperShoot true!
– Roel
Nov 13 '18 at 13:21
@IljaEverilä sorry for not making it clear. That is only an example. It could be any other type as long as im getting only the same fields
– Roel
Nov 13 '18 at 13:22