Java sort List of objects with a hierarchy alphabetically
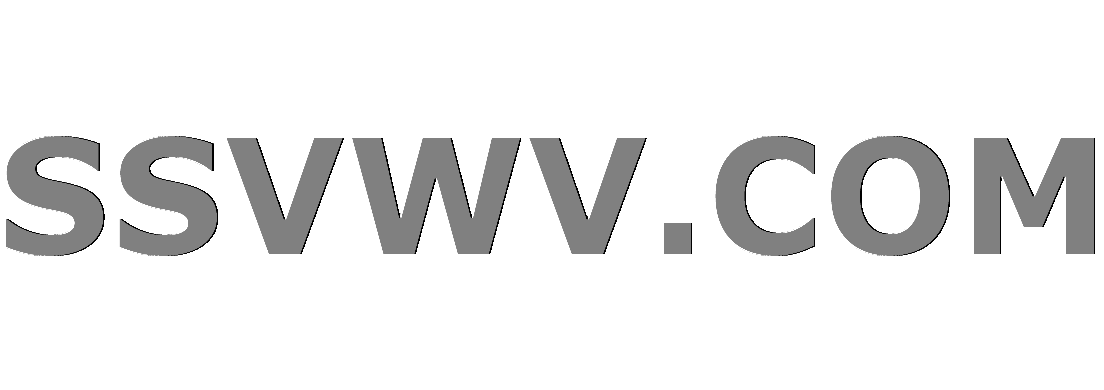
Multi tool use
I have an entity called Item
. Item
can have parents and children.
Each Item
has the following methods:
getParent()
--> returns anItem
getChildren()
--> returns aList<Item>
isLeaf()
--> returns aBoolean
getName()
--> returns aString
Each level in the hierarchy is a level in a construction site, for example level 1 is House 1, level 2 is Floor 1, level 3 is Room and level 4 is Window.
I have a List<Item
and I need to sort them like this:
- Item 1 (House 1 > Floor 1 > Room 1 > Bath)
- Item 2 (House 1 > Floor 1 > Room 2 > Basement)
- Item 3 (House 1 > Floor 1 > Room 2 > Door)
- Item 4 (House 1 > Floor 1 > Room 2 > Window)
- Item 5 (House 1 > Floor 2 > Room 1 > Door)
I think I need some kind of recursive function but I can't imagine how it has to be.
I've already researched for sorting objects with a hierarchy in Java but I don't find anything similar to my case.
I would appreciate any help and sorry if the question is not clear 100% but it is quite hard to describe.
Thanks.
java sorting recursion hierarchy comparator
add a comment |
I have an entity called Item
. Item
can have parents and children.
Each Item
has the following methods:
getParent()
--> returns anItem
getChildren()
--> returns aList<Item>
isLeaf()
--> returns aBoolean
getName()
--> returns aString
Each level in the hierarchy is a level in a construction site, for example level 1 is House 1, level 2 is Floor 1, level 3 is Room and level 4 is Window.
I have a List<Item
and I need to sort them like this:
- Item 1 (House 1 > Floor 1 > Room 1 > Bath)
- Item 2 (House 1 > Floor 1 > Room 2 > Basement)
- Item 3 (House 1 > Floor 1 > Room 2 > Door)
- Item 4 (House 1 > Floor 1 > Room 2 > Window)
- Item 5 (House 1 > Floor 2 > Room 1 > Door)
I think I need some kind of recursive function but I can't imagine how it has to be.
I've already researched for sorting objects with a hierarchy in Java but I don't find anything similar to my case.
I would appreciate any help and sorry if the question is not clear 100% but it is quite hard to describe.
Thanks.
java sorting recursion hierarchy comparator
Basically you want to sort the leaves then? I would imagine that you would sort when adding the elements to the house object and then do a depth first lookup of the leaves
– SBylemans
Nov 13 '18 at 11:00
What is an entity? You can't create a new class calledObject
. There is already an important class in Java calledObject
;)
– flakes
Nov 13 '18 at 11:45
@flakes thanks for your comment but I don't think that's important and it doesn't add anything to the question. The real name of the "Object" isObjekt
(Object in German). I omitted that detail to avoid confusing people with irrelevant information. If you feel happier I can renameObject
toItem
– Ale
Nov 13 '18 at 11:55
You need to provide some code. It is unclear to me what is your implementation. Are all entities of typeitem
(houses, floors, rooms) or are those different classes? Do they implement/extenditem
class?
– Amongalen
Nov 13 '18 at 12:03
When discussing class design/method implementation, having a class name is pretty crucial for communication! Further, you should be providing some code in your questions to show us at what point your stuck. Define as much of the solution as you can and you'll find that people understand your problem better and provide answers closer to what you expect. As an aside, names likeObjekt
andItem
are pretty opaque names for a class.. try something more meaningful (makes it obvious as to the actual purpose,HouseNode
,FloorplanTree
, etc). Good naming is paramount to clean maintainable code
– flakes
Nov 13 '18 at 12:53
add a comment |
I have an entity called Item
. Item
can have parents and children.
Each Item
has the following methods:
getParent()
--> returns anItem
getChildren()
--> returns aList<Item>
isLeaf()
--> returns aBoolean
getName()
--> returns aString
Each level in the hierarchy is a level in a construction site, for example level 1 is House 1, level 2 is Floor 1, level 3 is Room and level 4 is Window.
I have a List<Item
and I need to sort them like this:
- Item 1 (House 1 > Floor 1 > Room 1 > Bath)
- Item 2 (House 1 > Floor 1 > Room 2 > Basement)
- Item 3 (House 1 > Floor 1 > Room 2 > Door)
- Item 4 (House 1 > Floor 1 > Room 2 > Window)
- Item 5 (House 1 > Floor 2 > Room 1 > Door)
I think I need some kind of recursive function but I can't imagine how it has to be.
I've already researched for sorting objects with a hierarchy in Java but I don't find anything similar to my case.
I would appreciate any help and sorry if the question is not clear 100% but it is quite hard to describe.
Thanks.
java sorting recursion hierarchy comparator
I have an entity called Item
. Item
can have parents and children.
Each Item
has the following methods:
getParent()
--> returns anItem
getChildren()
--> returns aList<Item>
isLeaf()
--> returns aBoolean
getName()
--> returns aString
Each level in the hierarchy is a level in a construction site, for example level 1 is House 1, level 2 is Floor 1, level 3 is Room and level 4 is Window.
I have a List<Item
and I need to sort them like this:
- Item 1 (House 1 > Floor 1 > Room 1 > Bath)
- Item 2 (House 1 > Floor 1 > Room 2 > Basement)
- Item 3 (House 1 > Floor 1 > Room 2 > Door)
- Item 4 (House 1 > Floor 1 > Room 2 > Window)
- Item 5 (House 1 > Floor 2 > Room 1 > Door)
I think I need some kind of recursive function but I can't imagine how it has to be.
I've already researched for sorting objects with a hierarchy in Java but I don't find anything similar to my case.
I would appreciate any help and sorry if the question is not clear 100% but it is quite hard to describe.
Thanks.
java sorting recursion hierarchy comparator
java sorting recursion hierarchy comparator
edited Nov 13 '18 at 13:46
rghome
4,69152540
4,69152540
asked Nov 13 '18 at 10:44


AleAle
82322144
82322144
Basically you want to sort the leaves then? I would imagine that you would sort when adding the elements to the house object and then do a depth first lookup of the leaves
– SBylemans
Nov 13 '18 at 11:00
What is an entity? You can't create a new class calledObject
. There is already an important class in Java calledObject
;)
– flakes
Nov 13 '18 at 11:45
@flakes thanks for your comment but I don't think that's important and it doesn't add anything to the question. The real name of the "Object" isObjekt
(Object in German). I omitted that detail to avoid confusing people with irrelevant information. If you feel happier I can renameObject
toItem
– Ale
Nov 13 '18 at 11:55
You need to provide some code. It is unclear to me what is your implementation. Are all entities of typeitem
(houses, floors, rooms) or are those different classes? Do they implement/extenditem
class?
– Amongalen
Nov 13 '18 at 12:03
When discussing class design/method implementation, having a class name is pretty crucial for communication! Further, you should be providing some code in your questions to show us at what point your stuck. Define as much of the solution as you can and you'll find that people understand your problem better and provide answers closer to what you expect. As an aside, names likeObjekt
andItem
are pretty opaque names for a class.. try something more meaningful (makes it obvious as to the actual purpose,HouseNode
,FloorplanTree
, etc). Good naming is paramount to clean maintainable code
– flakes
Nov 13 '18 at 12:53
add a comment |
Basically you want to sort the leaves then? I would imagine that you would sort when adding the elements to the house object and then do a depth first lookup of the leaves
– SBylemans
Nov 13 '18 at 11:00
What is an entity? You can't create a new class calledObject
. There is already an important class in Java calledObject
;)
– flakes
Nov 13 '18 at 11:45
@flakes thanks for your comment but I don't think that's important and it doesn't add anything to the question. The real name of the "Object" isObjekt
(Object in German). I omitted that detail to avoid confusing people with irrelevant information. If you feel happier I can renameObject
toItem
– Ale
Nov 13 '18 at 11:55
You need to provide some code. It is unclear to me what is your implementation. Are all entities of typeitem
(houses, floors, rooms) or are those different classes? Do they implement/extenditem
class?
– Amongalen
Nov 13 '18 at 12:03
When discussing class design/method implementation, having a class name is pretty crucial for communication! Further, you should be providing some code in your questions to show us at what point your stuck. Define as much of the solution as you can and you'll find that people understand your problem better and provide answers closer to what you expect. As an aside, names likeObjekt
andItem
are pretty opaque names for a class.. try something more meaningful (makes it obvious as to the actual purpose,HouseNode
,FloorplanTree
, etc). Good naming is paramount to clean maintainable code
– flakes
Nov 13 '18 at 12:53
Basically you want to sort the leaves then? I would imagine that you would sort when adding the elements to the house object and then do a depth first lookup of the leaves
– SBylemans
Nov 13 '18 at 11:00
Basically you want to sort the leaves then? I would imagine that you would sort when adding the elements to the house object and then do a depth first lookup of the leaves
– SBylemans
Nov 13 '18 at 11:00
What is an entity? You can't create a new class called
Object
. There is already an important class in Java called Object
;)– flakes
Nov 13 '18 at 11:45
What is an entity? You can't create a new class called
Object
. There is already an important class in Java called Object
;)– flakes
Nov 13 '18 at 11:45
@flakes thanks for your comment but I don't think that's important and it doesn't add anything to the question. The real name of the "Object" is
Objekt
(Object in German). I omitted that detail to avoid confusing people with irrelevant information. If you feel happier I can rename Object
to Item
– Ale
Nov 13 '18 at 11:55
@flakes thanks for your comment but I don't think that's important and it doesn't add anything to the question. The real name of the "Object" is
Objekt
(Object in German). I omitted that detail to avoid confusing people with irrelevant information. If you feel happier I can rename Object
to Item
– Ale
Nov 13 '18 at 11:55
You need to provide some code. It is unclear to me what is your implementation. Are all entities of type
item
(houses, floors, rooms) or are those different classes? Do they implement/extend item
class?– Amongalen
Nov 13 '18 at 12:03
You need to provide some code. It is unclear to me what is your implementation. Are all entities of type
item
(houses, floors, rooms) or are those different classes? Do they implement/extend item
class?– Amongalen
Nov 13 '18 at 12:03
When discussing class design/method implementation, having a class name is pretty crucial for communication! Further, you should be providing some code in your questions to show us at what point your stuck. Define as much of the solution as you can and you'll find that people understand your problem better and provide answers closer to what you expect. As an aside, names like
Objekt
and Item
are pretty opaque names for a class.. try something more meaningful (makes it obvious as to the actual purpose, HouseNode
, FloorplanTree
, etc). Good naming is paramount to clean maintainable code– flakes
Nov 13 '18 at 12:53
When discussing class design/method implementation, having a class name is pretty crucial for communication! Further, you should be providing some code in your questions to show us at what point your stuck. Define as much of the solution as you can and you'll find that people understand your problem better and provide answers closer to what you expect. As an aside, names like
Objekt
and Item
are pretty opaque names for a class.. try something more meaningful (makes it obvious as to the actual purpose, HouseNode
, FloorplanTree
, etc). Good naming is paramount to clean maintainable code– flakes
Nov 13 '18 at 12:53
add a comment |
2 Answers
2
active
oldest
votes
It would indeed be recursive. The trouble is that a simple recursive compare would only work if the two nodes were on the same level.
// Same level compare
int compareSameLevel(Item item) {
int c = 0;
if (this.getParent != null) {
c = compare(this.getParent(), item.getParent());
}
return (c != 0) ? c : getName().compare(item.getName());
}
But you could adjust the levels to find a common level and assume that the children go after the parent:
// Compare on any level
int compare(Item item) {
Item thisItem = this;
int thisLevel = thisItem.level();
int itemLevel = item.level();
for (int i = thisLevel; i > itemLevel; i--) {
thisItem = thisItem.getParent();
}
for (int j = itemLevel; j > thisLevel; j--) {
item = item.getParent();
}
int c = compareSameLevel(thisItem, item);
return c != 0 ? c : (thisLevel > itemLevel ? -1 : 1);
}
This is just to give you an idea. It is not tested or compiled.
add a comment |
Pseudo code: Try like this
private static void printChildElements(List<Object> childNodes) {
for(Object childNode : childNodes) {
List<Object > childElements = childNode .....;
if(childElements.size() > 0) {
printChildElements( childElements);
}
}
The question is about sorting, not about printing the whole tree.
– rghome
Nov 13 '18 at 12:41
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53279254%2fjava-sort-list-of-objects-with-a-hierarchy-alphabetically%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
It would indeed be recursive. The trouble is that a simple recursive compare would only work if the two nodes were on the same level.
// Same level compare
int compareSameLevel(Item item) {
int c = 0;
if (this.getParent != null) {
c = compare(this.getParent(), item.getParent());
}
return (c != 0) ? c : getName().compare(item.getName());
}
But you could adjust the levels to find a common level and assume that the children go after the parent:
// Compare on any level
int compare(Item item) {
Item thisItem = this;
int thisLevel = thisItem.level();
int itemLevel = item.level();
for (int i = thisLevel; i > itemLevel; i--) {
thisItem = thisItem.getParent();
}
for (int j = itemLevel; j > thisLevel; j--) {
item = item.getParent();
}
int c = compareSameLevel(thisItem, item);
return c != 0 ? c : (thisLevel > itemLevel ? -1 : 1);
}
This is just to give you an idea. It is not tested or compiled.
add a comment |
It would indeed be recursive. The trouble is that a simple recursive compare would only work if the two nodes were on the same level.
// Same level compare
int compareSameLevel(Item item) {
int c = 0;
if (this.getParent != null) {
c = compare(this.getParent(), item.getParent());
}
return (c != 0) ? c : getName().compare(item.getName());
}
But you could adjust the levels to find a common level and assume that the children go after the parent:
// Compare on any level
int compare(Item item) {
Item thisItem = this;
int thisLevel = thisItem.level();
int itemLevel = item.level();
for (int i = thisLevel; i > itemLevel; i--) {
thisItem = thisItem.getParent();
}
for (int j = itemLevel; j > thisLevel; j--) {
item = item.getParent();
}
int c = compareSameLevel(thisItem, item);
return c != 0 ? c : (thisLevel > itemLevel ? -1 : 1);
}
This is just to give you an idea. It is not tested or compiled.
add a comment |
It would indeed be recursive. The trouble is that a simple recursive compare would only work if the two nodes were on the same level.
// Same level compare
int compareSameLevel(Item item) {
int c = 0;
if (this.getParent != null) {
c = compare(this.getParent(), item.getParent());
}
return (c != 0) ? c : getName().compare(item.getName());
}
But you could adjust the levels to find a common level and assume that the children go after the parent:
// Compare on any level
int compare(Item item) {
Item thisItem = this;
int thisLevel = thisItem.level();
int itemLevel = item.level();
for (int i = thisLevel; i > itemLevel; i--) {
thisItem = thisItem.getParent();
}
for (int j = itemLevel; j > thisLevel; j--) {
item = item.getParent();
}
int c = compareSameLevel(thisItem, item);
return c != 0 ? c : (thisLevel > itemLevel ? -1 : 1);
}
This is just to give you an idea. It is not tested or compiled.
It would indeed be recursive. The trouble is that a simple recursive compare would only work if the two nodes were on the same level.
// Same level compare
int compareSameLevel(Item item) {
int c = 0;
if (this.getParent != null) {
c = compare(this.getParent(), item.getParent());
}
return (c != 0) ? c : getName().compare(item.getName());
}
But you could adjust the levels to find a common level and assume that the children go after the parent:
// Compare on any level
int compare(Item item) {
Item thisItem = this;
int thisLevel = thisItem.level();
int itemLevel = item.level();
for (int i = thisLevel; i > itemLevel; i--) {
thisItem = thisItem.getParent();
}
for (int j = itemLevel; j > thisLevel; j--) {
item = item.getParent();
}
int c = compareSameLevel(thisItem, item);
return c != 0 ? c : (thisLevel > itemLevel ? -1 : 1);
}
This is just to give you an idea. It is not tested or compiled.
answered Nov 13 '18 at 12:37
rghomerghome
4,69152540
4,69152540
add a comment |
add a comment |
Pseudo code: Try like this
private static void printChildElements(List<Object> childNodes) {
for(Object childNode : childNodes) {
List<Object > childElements = childNode .....;
if(childElements.size() > 0) {
printChildElements( childElements);
}
}
The question is about sorting, not about printing the whole tree.
– rghome
Nov 13 '18 at 12:41
add a comment |
Pseudo code: Try like this
private static void printChildElements(List<Object> childNodes) {
for(Object childNode : childNodes) {
List<Object > childElements = childNode .....;
if(childElements.size() > 0) {
printChildElements( childElements);
}
}
The question is about sorting, not about printing the whole tree.
– rghome
Nov 13 '18 at 12:41
add a comment |
Pseudo code: Try like this
private static void printChildElements(List<Object> childNodes) {
for(Object childNode : childNodes) {
List<Object > childElements = childNode .....;
if(childElements.size() > 0) {
printChildElements( childElements);
}
}
Pseudo code: Try like this
private static void printChildElements(List<Object> childNodes) {
for(Object childNode : childNodes) {
List<Object > childElements = childNode .....;
if(childElements.size() > 0) {
printChildElements( childElements);
}
}
answered Nov 13 '18 at 12:23


Prakash NPrakash N
347
347
The question is about sorting, not about printing the whole tree.
– rghome
Nov 13 '18 at 12:41
add a comment |
The question is about sorting, not about printing the whole tree.
– rghome
Nov 13 '18 at 12:41
The question is about sorting, not about printing the whole tree.
– rghome
Nov 13 '18 at 12:41
The question is about sorting, not about printing the whole tree.
– rghome
Nov 13 '18 at 12:41
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53279254%2fjava-sort-list-of-objects-with-a-hierarchy-alphabetically%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
mJYpWt7QrleB,ur8acWqB,X2
Basically you want to sort the leaves then? I would imagine that you would sort when adding the elements to the house object and then do a depth first lookup of the leaves
– SBylemans
Nov 13 '18 at 11:00
What is an entity? You can't create a new class called
Object
. There is already an important class in Java calledObject
;)– flakes
Nov 13 '18 at 11:45
@flakes thanks for your comment but I don't think that's important and it doesn't add anything to the question. The real name of the "Object" is
Objekt
(Object in German). I omitted that detail to avoid confusing people with irrelevant information. If you feel happier I can renameObject
toItem
– Ale
Nov 13 '18 at 11:55
You need to provide some code. It is unclear to me what is your implementation. Are all entities of type
item
(houses, floors, rooms) or are those different classes? Do they implement/extenditem
class?– Amongalen
Nov 13 '18 at 12:03
When discussing class design/method implementation, having a class name is pretty crucial for communication! Further, you should be providing some code in your questions to show us at what point your stuck. Define as much of the solution as you can and you'll find that people understand your problem better and provide answers closer to what you expect. As an aside, names like
Objekt
andItem
are pretty opaque names for a class.. try something more meaningful (makes it obvious as to the actual purpose,HouseNode
,FloorplanTree
, etc). Good naming is paramount to clean maintainable code– flakes
Nov 13 '18 at 12:53