How can I capture the stdout output of a child process?
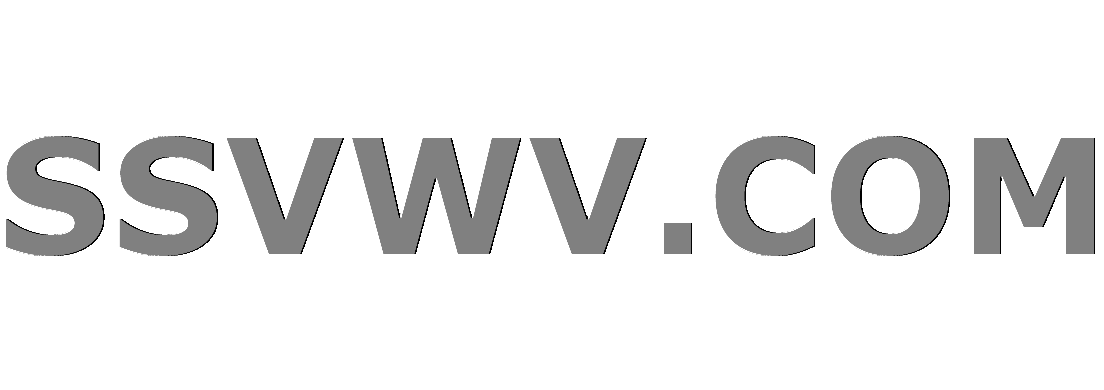
Multi tool use
I'm trying to write a program in Python and I'm told to run an .exe file. When this .exe file is run it spits out a lot of data and I need a certain line printed out to the screen. I'm pretty sure I need to use subprocess.popen
or something similar but I'm new to subprocess and have no clue. Anyone have an easy way for me to get this done?
python subprocess stdout
add a comment |
I'm trying to write a program in Python and I'm told to run an .exe file. When this .exe file is run it spits out a lot of data and I need a certain line printed out to the screen. I'm pretty sure I need to use subprocess.popen
or something similar but I'm new to subprocess and have no clue. Anyone have an easy way for me to get this done?
python subprocess stdout
add a comment |
I'm trying to write a program in Python and I'm told to run an .exe file. When this .exe file is run it spits out a lot of data and I need a certain line printed out to the screen. I'm pretty sure I need to use subprocess.popen
or something similar but I'm new to subprocess and have no clue. Anyone have an easy way for me to get this done?
python subprocess stdout
I'm trying to write a program in Python and I'm told to run an .exe file. When this .exe file is run it spits out a lot of data and I need a certain line printed out to the screen. I'm pretty sure I need to use subprocess.popen
or something similar but I'm new to subprocess and have no clue. Anyone have an easy way for me to get this done?
python subprocess stdout
python subprocess stdout
edited Nov 13 '18 at 20:00
Adam Stelmaszczyk
15.4k35497
15.4k35497
asked May 28 '09 at 20:45
TylerTyler
1,30271824
1,30271824
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Something like this:
import subprocess
process = subprocess.Popen(["yourcommand"], stdout=subprocess.PIPE)
result = process.communicate()[0]
How would i read a certain line out? is that where the 0 is? And yes I'm interested in printing output after process is finished. Also where you have "yourcommand" does the exe extension go there?
– Tyler
May 28 '09 at 20:57
1
"result" will be the entire output of "yourcommand". You can then process that string (or bytes object, in Py3.0) to find the line you're looking for.
– Dietrich Epp
May 28 '09 at 21:08
3
the [0] means the first element of the tuple returned (stdout, stderr); you may process result, which will be a string, to your liking, e.g. to find a particular line. "yourcommand" is your full command, eventually a full path to a command if the command ist not on path (at least on *nix).
– miku
May 28 '09 at 21:08
add a comment |
@Paolo's solution is perfect if you are interested in printing output after the process has finished executing. In case you want to poll output while the process is running you have to do it this way:
process = subprocess.Popen(cmd, stdout=subprocess.PIPE)
while True:
out = process.stdout.readline(1)
if out == '' and process.poll() != None:
break
if out.startswith('myline'):
sys.stdout.write(out)
sys.stdout.flush()
what ismyline
here?
– Hamza Rashid
May 10 '18 at 8:12
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f923079%2fhow-can-i-capture-the-stdout-output-of-a-child-process%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Something like this:
import subprocess
process = subprocess.Popen(["yourcommand"], stdout=subprocess.PIPE)
result = process.communicate()[0]
How would i read a certain line out? is that where the 0 is? And yes I'm interested in printing output after process is finished. Also where you have "yourcommand" does the exe extension go there?
– Tyler
May 28 '09 at 20:57
1
"result" will be the entire output of "yourcommand". You can then process that string (or bytes object, in Py3.0) to find the line you're looking for.
– Dietrich Epp
May 28 '09 at 21:08
3
the [0] means the first element of the tuple returned (stdout, stderr); you may process result, which will be a string, to your liking, e.g. to find a particular line. "yourcommand" is your full command, eventually a full path to a command if the command ist not on path (at least on *nix).
– miku
May 28 '09 at 21:08
add a comment |
Something like this:
import subprocess
process = subprocess.Popen(["yourcommand"], stdout=subprocess.PIPE)
result = process.communicate()[0]
How would i read a certain line out? is that where the 0 is? And yes I'm interested in printing output after process is finished. Also where you have "yourcommand" does the exe extension go there?
– Tyler
May 28 '09 at 20:57
1
"result" will be the entire output of "yourcommand". You can then process that string (or bytes object, in Py3.0) to find the line you're looking for.
– Dietrich Epp
May 28 '09 at 21:08
3
the [0] means the first element of the tuple returned (stdout, stderr); you may process result, which will be a string, to your liking, e.g. to find a particular line. "yourcommand" is your full command, eventually a full path to a command if the command ist not on path (at least on *nix).
– miku
May 28 '09 at 21:08
add a comment |
Something like this:
import subprocess
process = subprocess.Popen(["yourcommand"], stdout=subprocess.PIPE)
result = process.communicate()[0]
Something like this:
import subprocess
process = subprocess.Popen(["yourcommand"], stdout=subprocess.PIPE)
result = process.communicate()[0]
answered May 28 '09 at 20:49
Paolo BergantinoPaolo Bergantino
378k73486424
378k73486424
How would i read a certain line out? is that where the 0 is? And yes I'm interested in printing output after process is finished. Also where you have "yourcommand" does the exe extension go there?
– Tyler
May 28 '09 at 20:57
1
"result" will be the entire output of "yourcommand". You can then process that string (or bytes object, in Py3.0) to find the line you're looking for.
– Dietrich Epp
May 28 '09 at 21:08
3
the [0] means the first element of the tuple returned (stdout, stderr); you may process result, which will be a string, to your liking, e.g. to find a particular line. "yourcommand" is your full command, eventually a full path to a command if the command ist not on path (at least on *nix).
– miku
May 28 '09 at 21:08
add a comment |
How would i read a certain line out? is that where the 0 is? And yes I'm interested in printing output after process is finished. Also where you have "yourcommand" does the exe extension go there?
– Tyler
May 28 '09 at 20:57
1
"result" will be the entire output of "yourcommand". You can then process that string (or bytes object, in Py3.0) to find the line you're looking for.
– Dietrich Epp
May 28 '09 at 21:08
3
the [0] means the first element of the tuple returned (stdout, stderr); you may process result, which will be a string, to your liking, e.g. to find a particular line. "yourcommand" is your full command, eventually a full path to a command if the command ist not on path (at least on *nix).
– miku
May 28 '09 at 21:08
How would i read a certain line out? is that where the 0 is? And yes I'm interested in printing output after process is finished. Also where you have "yourcommand" does the exe extension go there?
– Tyler
May 28 '09 at 20:57
How would i read a certain line out? is that where the 0 is? And yes I'm interested in printing output after process is finished. Also where you have "yourcommand" does the exe extension go there?
– Tyler
May 28 '09 at 20:57
1
1
"result" will be the entire output of "yourcommand". You can then process that string (or bytes object, in Py3.0) to find the line you're looking for.
– Dietrich Epp
May 28 '09 at 21:08
"result" will be the entire output of "yourcommand". You can then process that string (or bytes object, in Py3.0) to find the line you're looking for.
– Dietrich Epp
May 28 '09 at 21:08
3
3
the [0] means the first element of the tuple returned (stdout, stderr); you may process result, which will be a string, to your liking, e.g. to find a particular line. "yourcommand" is your full command, eventually a full path to a command if the command ist not on path (at least on *nix).
– miku
May 28 '09 at 21:08
the [0] means the first element of the tuple returned (stdout, stderr); you may process result, which will be a string, to your liking, e.g. to find a particular line. "yourcommand" is your full command, eventually a full path to a command if the command ist not on path (at least on *nix).
– miku
May 28 '09 at 21:08
add a comment |
@Paolo's solution is perfect if you are interested in printing output after the process has finished executing. In case you want to poll output while the process is running you have to do it this way:
process = subprocess.Popen(cmd, stdout=subprocess.PIPE)
while True:
out = process.stdout.readline(1)
if out == '' and process.poll() != None:
break
if out.startswith('myline'):
sys.stdout.write(out)
sys.stdout.flush()
what ismyline
here?
– Hamza Rashid
May 10 '18 at 8:12
add a comment |
@Paolo's solution is perfect if you are interested in printing output after the process has finished executing. In case you want to poll output while the process is running you have to do it this way:
process = subprocess.Popen(cmd, stdout=subprocess.PIPE)
while True:
out = process.stdout.readline(1)
if out == '' and process.poll() != None:
break
if out.startswith('myline'):
sys.stdout.write(out)
sys.stdout.flush()
what ismyline
here?
– Hamza Rashid
May 10 '18 at 8:12
add a comment |
@Paolo's solution is perfect if you are interested in printing output after the process has finished executing. In case you want to poll output while the process is running you have to do it this way:
process = subprocess.Popen(cmd, stdout=subprocess.PIPE)
while True:
out = process.stdout.readline(1)
if out == '' and process.poll() != None:
break
if out.startswith('myline'):
sys.stdout.write(out)
sys.stdout.flush()
@Paolo's solution is perfect if you are interested in printing output after the process has finished executing. In case you want to poll output while the process is running you have to do it this way:
process = subprocess.Popen(cmd, stdout=subprocess.PIPE)
while True:
out = process.stdout.readline(1)
if out == '' and process.poll() != None:
break
if out.startswith('myline'):
sys.stdout.write(out)
sys.stdout.flush()
answered May 28 '09 at 20:52
Nadia AlramliNadia Alramli
78.7k24153147
78.7k24153147
what ismyline
here?
– Hamza Rashid
May 10 '18 at 8:12
add a comment |
what ismyline
here?
– Hamza Rashid
May 10 '18 at 8:12
what is
myline
here?– Hamza Rashid
May 10 '18 at 8:12
what is
myline
here?– Hamza Rashid
May 10 '18 at 8:12
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f923079%2fhow-can-i-capture-the-stdout-output-of-a-child-process%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
yH59afAUpBNT2,FS1tnxgDnAAMmbmu Bwm8aG8c o