Confused by difference between running R from batch file versus running it directly from shell
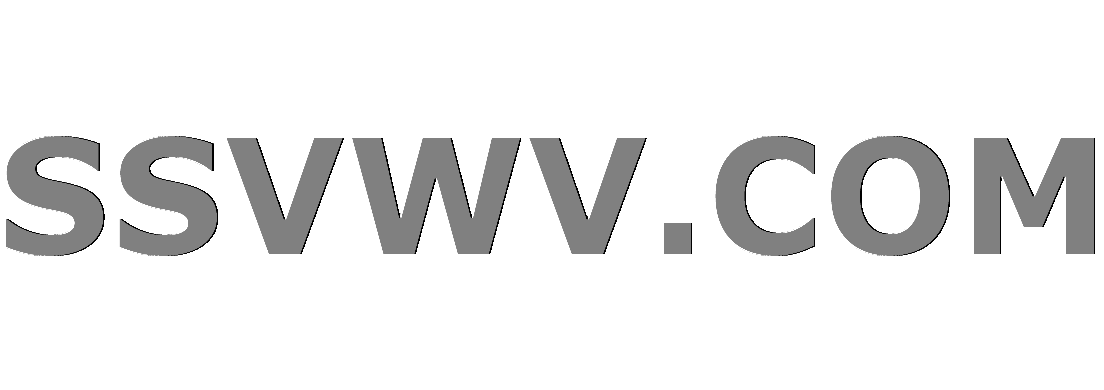
Multi tool use
I recently came across a strange error, and while I managed to fix it, I cannot for the life of me figure out what was going wrong behind the scenes and I would like to understand what was going on. I am currently writing a program that takes Excel inputs and uses them to direct a program written in R, that ultimately spits out results to be fed back into Excel for user review.
My original solution utilized a batch file that consisted of a single line, calling:
Rscript "Rfilepath.R"
that was called from VBA using the below:
Dim wsh As Object
Set wsh = VBA.CreateObject("WScript.Shell")
Dim waitOnReturn As Boolean: waitOnReturn = True
Dim windowstyle As Integer: windowstyle = 1
wsh.Run Chr(34) & BatchFilePath & Chr(34), windowstyle, waitOnReturn
However, this proved unable to run the below R code (extremely simplified):
library(ggplot2)
library(gridExtra)
library(ggpubr)
x <- seq(1,10)
y <- seq(11,20)
z <- seq(6,15)
a <- ggplot(data.frame(cbind(x,y)),aes(x=x,y=y))+geom_point()
b <- ggplot(data.frame(cbind(y,z)),aes(x=y,y=z))+geom_point()
c <- ggplot(data.frame(cbind(x,z)),aes(x=x,y=z))+geom_point()
test <- ggarrange(ggarrange(a,b,nrow=2),c,ncol=2)
ggsave(file="filepath.png",plot=test)
The problem resulted when the ggarrange function was called. However, I was able to get this situation working by doing the following in VBA (essentially cutting out the batch file step):
Set wsh = VBA.CreateObject("WScript.Shell")
Dim waitTillComplete As Boolean: waitTillComplete = True
Dim style As Integer: style = 1
Dim errorCode As Integer
errorCode = wsh.Run("Rscript " & Chr(34) & RPath & Chr(34), style, waitTillComplete)
What is the difference between these two approaches and why did one work while the other one did not? It was difficult to debug due to the batch terminal immediately closing on error (probably due to running it from VBA). Any tips or recommendations for debugging these types of issues would also be greatly appreciated. Thanks!
r excel vba excel-vba command-prompt
add a comment |
I recently came across a strange error, and while I managed to fix it, I cannot for the life of me figure out what was going wrong behind the scenes and I would like to understand what was going on. I am currently writing a program that takes Excel inputs and uses them to direct a program written in R, that ultimately spits out results to be fed back into Excel for user review.
My original solution utilized a batch file that consisted of a single line, calling:
Rscript "Rfilepath.R"
that was called from VBA using the below:
Dim wsh As Object
Set wsh = VBA.CreateObject("WScript.Shell")
Dim waitOnReturn As Boolean: waitOnReturn = True
Dim windowstyle As Integer: windowstyle = 1
wsh.Run Chr(34) & BatchFilePath & Chr(34), windowstyle, waitOnReturn
However, this proved unable to run the below R code (extremely simplified):
library(ggplot2)
library(gridExtra)
library(ggpubr)
x <- seq(1,10)
y <- seq(11,20)
z <- seq(6,15)
a <- ggplot(data.frame(cbind(x,y)),aes(x=x,y=y))+geom_point()
b <- ggplot(data.frame(cbind(y,z)),aes(x=y,y=z))+geom_point()
c <- ggplot(data.frame(cbind(x,z)),aes(x=x,y=z))+geom_point()
test <- ggarrange(ggarrange(a,b,nrow=2),c,ncol=2)
ggsave(file="filepath.png",plot=test)
The problem resulted when the ggarrange function was called. However, I was able to get this situation working by doing the following in VBA (essentially cutting out the batch file step):
Set wsh = VBA.CreateObject("WScript.Shell")
Dim waitTillComplete As Boolean: waitTillComplete = True
Dim style As Integer: style = 1
Dim errorCode As Integer
errorCode = wsh.Run("Rscript " & Chr(34) & RPath & Chr(34), style, waitTillComplete)
What is the difference between these two approaches and why did one work while the other one did not? It was difficult to debug due to the batch terminal immediately closing on error (probably due to running it from VBA). Any tips or recommendations for debugging these types of issues would also be greatly appreciated. Thanks!
r excel vba excel-vba command-prompt
You're only using filenames in your calls, which means you're making assumptions about what the current working directory is - it would maybe be better to either set that explicitly, or use full paths in your scripts.
– Tim Williams
Nov 13 '18 at 23:35
The issue is not the filepath, I merely replaced actual wd with a simplified version, the R script is definitely getting opened up and running and fails at that specific line of code. I have already identified that it works with the ggarrange call commented out, but doesn't work with it not commented out.
– actuary_meets_data
Nov 14 '18 at 15:37
You can keep the cmd window open - itechtics.com/…
– Tim Williams
Nov 14 '18 at 17:26
add a comment |
I recently came across a strange error, and while I managed to fix it, I cannot for the life of me figure out what was going wrong behind the scenes and I would like to understand what was going on. I am currently writing a program that takes Excel inputs and uses them to direct a program written in R, that ultimately spits out results to be fed back into Excel for user review.
My original solution utilized a batch file that consisted of a single line, calling:
Rscript "Rfilepath.R"
that was called from VBA using the below:
Dim wsh As Object
Set wsh = VBA.CreateObject("WScript.Shell")
Dim waitOnReturn As Boolean: waitOnReturn = True
Dim windowstyle As Integer: windowstyle = 1
wsh.Run Chr(34) & BatchFilePath & Chr(34), windowstyle, waitOnReturn
However, this proved unable to run the below R code (extremely simplified):
library(ggplot2)
library(gridExtra)
library(ggpubr)
x <- seq(1,10)
y <- seq(11,20)
z <- seq(6,15)
a <- ggplot(data.frame(cbind(x,y)),aes(x=x,y=y))+geom_point()
b <- ggplot(data.frame(cbind(y,z)),aes(x=y,y=z))+geom_point()
c <- ggplot(data.frame(cbind(x,z)),aes(x=x,y=z))+geom_point()
test <- ggarrange(ggarrange(a,b,nrow=2),c,ncol=2)
ggsave(file="filepath.png",plot=test)
The problem resulted when the ggarrange function was called. However, I was able to get this situation working by doing the following in VBA (essentially cutting out the batch file step):
Set wsh = VBA.CreateObject("WScript.Shell")
Dim waitTillComplete As Boolean: waitTillComplete = True
Dim style As Integer: style = 1
Dim errorCode As Integer
errorCode = wsh.Run("Rscript " & Chr(34) & RPath & Chr(34), style, waitTillComplete)
What is the difference between these two approaches and why did one work while the other one did not? It was difficult to debug due to the batch terminal immediately closing on error (probably due to running it from VBA). Any tips or recommendations for debugging these types of issues would also be greatly appreciated. Thanks!
r excel vba excel-vba command-prompt
I recently came across a strange error, and while I managed to fix it, I cannot for the life of me figure out what was going wrong behind the scenes and I would like to understand what was going on. I am currently writing a program that takes Excel inputs and uses them to direct a program written in R, that ultimately spits out results to be fed back into Excel for user review.
My original solution utilized a batch file that consisted of a single line, calling:
Rscript "Rfilepath.R"
that was called from VBA using the below:
Dim wsh As Object
Set wsh = VBA.CreateObject("WScript.Shell")
Dim waitOnReturn As Boolean: waitOnReturn = True
Dim windowstyle As Integer: windowstyle = 1
wsh.Run Chr(34) & BatchFilePath & Chr(34), windowstyle, waitOnReturn
However, this proved unable to run the below R code (extremely simplified):
library(ggplot2)
library(gridExtra)
library(ggpubr)
x <- seq(1,10)
y <- seq(11,20)
z <- seq(6,15)
a <- ggplot(data.frame(cbind(x,y)),aes(x=x,y=y))+geom_point()
b <- ggplot(data.frame(cbind(y,z)),aes(x=y,y=z))+geom_point()
c <- ggplot(data.frame(cbind(x,z)),aes(x=x,y=z))+geom_point()
test <- ggarrange(ggarrange(a,b,nrow=2),c,ncol=2)
ggsave(file="filepath.png",plot=test)
The problem resulted when the ggarrange function was called. However, I was able to get this situation working by doing the following in VBA (essentially cutting out the batch file step):
Set wsh = VBA.CreateObject("WScript.Shell")
Dim waitTillComplete As Boolean: waitTillComplete = True
Dim style As Integer: style = 1
Dim errorCode As Integer
errorCode = wsh.Run("Rscript " & Chr(34) & RPath & Chr(34), style, waitTillComplete)
What is the difference between these two approaches and why did one work while the other one did not? It was difficult to debug due to the batch terminal immediately closing on error (probably due to running it from VBA). Any tips or recommendations for debugging these types of issues would also be greatly appreciated. Thanks!
r excel vba excel-vba command-prompt
r excel vba excel-vba command-prompt
edited Nov 14 '18 at 7:55
Pᴇʜ
21.1k42750
21.1k42750
asked Nov 13 '18 at 20:17
actuary_meets_dataactuary_meets_data
235
235
You're only using filenames in your calls, which means you're making assumptions about what the current working directory is - it would maybe be better to either set that explicitly, or use full paths in your scripts.
– Tim Williams
Nov 13 '18 at 23:35
The issue is not the filepath, I merely replaced actual wd with a simplified version, the R script is definitely getting opened up and running and fails at that specific line of code. I have already identified that it works with the ggarrange call commented out, but doesn't work with it not commented out.
– actuary_meets_data
Nov 14 '18 at 15:37
You can keep the cmd window open - itechtics.com/…
– Tim Williams
Nov 14 '18 at 17:26
add a comment |
You're only using filenames in your calls, which means you're making assumptions about what the current working directory is - it would maybe be better to either set that explicitly, or use full paths in your scripts.
– Tim Williams
Nov 13 '18 at 23:35
The issue is not the filepath, I merely replaced actual wd with a simplified version, the R script is definitely getting opened up and running and fails at that specific line of code. I have already identified that it works with the ggarrange call commented out, but doesn't work with it not commented out.
– actuary_meets_data
Nov 14 '18 at 15:37
You can keep the cmd window open - itechtics.com/…
– Tim Williams
Nov 14 '18 at 17:26
You're only using filenames in your calls, which means you're making assumptions about what the current working directory is - it would maybe be better to either set that explicitly, or use full paths in your scripts.
– Tim Williams
Nov 13 '18 at 23:35
You're only using filenames in your calls, which means you're making assumptions about what the current working directory is - it would maybe be better to either set that explicitly, or use full paths in your scripts.
– Tim Williams
Nov 13 '18 at 23:35
The issue is not the filepath, I merely replaced actual wd with a simplified version, the R script is definitely getting opened up and running and fails at that specific line of code. I have already identified that it works with the ggarrange call commented out, but doesn't work with it not commented out.
– actuary_meets_data
Nov 14 '18 at 15:37
The issue is not the filepath, I merely replaced actual wd with a simplified version, the R script is definitely getting opened up and running and fails at that specific line of code. I have already identified that it works with the ggarrange call commented out, but doesn't work with it not commented out.
– actuary_meets_data
Nov 14 '18 at 15:37
You can keep the cmd window open - itechtics.com/…
– Tim Williams
Nov 14 '18 at 17:26
You can keep the cmd window open - itechtics.com/…
– Tim Williams
Nov 14 '18 at 17:26
add a comment |
1 Answer
1
active
oldest
votes
I think you have a problem with PATH of the RScript in case one : it is lost in the double batch call.
Are you implying that the code wouldn't run in the first place? I pasted a simplified version of my code to hide filepaths, but the script definitely runs when running it from the batch file. In fact that is the method I have been using for awhile (something I pulled off of stack a little while ago actually). It just isn't able to get past any step that involves ggarrange or arrangeGrob in R for some reason.
– actuary_meets_data
Nov 13 '18 at 21:01
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53288856%2fconfused-by-difference-between-running-r-from-batch-file-versus-running-it-direc%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think you have a problem with PATH of the RScript in case one : it is lost in the double batch call.
Are you implying that the code wouldn't run in the first place? I pasted a simplified version of my code to hide filepaths, but the script definitely runs when running it from the batch file. In fact that is the method I have been using for awhile (something I pulled off of stack a little while ago actually). It just isn't able to get past any step that involves ggarrange or arrangeGrob in R for some reason.
– actuary_meets_data
Nov 13 '18 at 21:01
add a comment |
I think you have a problem with PATH of the RScript in case one : it is lost in the double batch call.
Are you implying that the code wouldn't run in the first place? I pasted a simplified version of my code to hide filepaths, but the script definitely runs when running it from the batch file. In fact that is the method I have been using for awhile (something I pulled off of stack a little while ago actually). It just isn't able to get past any step that involves ggarrange or arrangeGrob in R for some reason.
– actuary_meets_data
Nov 13 '18 at 21:01
add a comment |
I think you have a problem with PATH of the RScript in case one : it is lost in the double batch call.
I think you have a problem with PATH of the RScript in case one : it is lost in the double batch call.
answered Nov 13 '18 at 20:50


phili_bphili_b
8611
8611
Are you implying that the code wouldn't run in the first place? I pasted a simplified version of my code to hide filepaths, but the script definitely runs when running it from the batch file. In fact that is the method I have been using for awhile (something I pulled off of stack a little while ago actually). It just isn't able to get past any step that involves ggarrange or arrangeGrob in R for some reason.
– actuary_meets_data
Nov 13 '18 at 21:01
add a comment |
Are you implying that the code wouldn't run in the first place? I pasted a simplified version of my code to hide filepaths, but the script definitely runs when running it from the batch file. In fact that is the method I have been using for awhile (something I pulled off of stack a little while ago actually). It just isn't able to get past any step that involves ggarrange or arrangeGrob in R for some reason.
– actuary_meets_data
Nov 13 '18 at 21:01
Are you implying that the code wouldn't run in the first place? I pasted a simplified version of my code to hide filepaths, but the script definitely runs when running it from the batch file. In fact that is the method I have been using for awhile (something I pulled off of stack a little while ago actually). It just isn't able to get past any step that involves ggarrange or arrangeGrob in R for some reason.
– actuary_meets_data
Nov 13 '18 at 21:01
Are you implying that the code wouldn't run in the first place? I pasted a simplified version of my code to hide filepaths, but the script definitely runs when running it from the batch file. In fact that is the method I have been using for awhile (something I pulled off of stack a little while ago actually). It just isn't able to get past any step that involves ggarrange or arrangeGrob in R for some reason.
– actuary_meets_data
Nov 13 '18 at 21:01
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53288856%2fconfused-by-difference-between-running-r-from-batch-file-versus-running-it-direc%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
oF8hJtcP6mWXPjC,CDEqk,5W 1UKGqVb7mnvTEShaWjvd,vqGr JKge,WEvr4NCIPc3
You're only using filenames in your calls, which means you're making assumptions about what the current working directory is - it would maybe be better to either set that explicitly, or use full paths in your scripts.
– Tim Williams
Nov 13 '18 at 23:35
The issue is not the filepath, I merely replaced actual wd with a simplified version, the R script is definitely getting opened up and running and fails at that specific line of code. I have already identified that it works with the ggarrange call commented out, but doesn't work with it not commented out.
– actuary_meets_data
Nov 14 '18 at 15:37
You can keep the cmd window open - itechtics.com/…
– Tim Williams
Nov 14 '18 at 17:26