correct DRF filter list view endpoint to return multiple objects by id
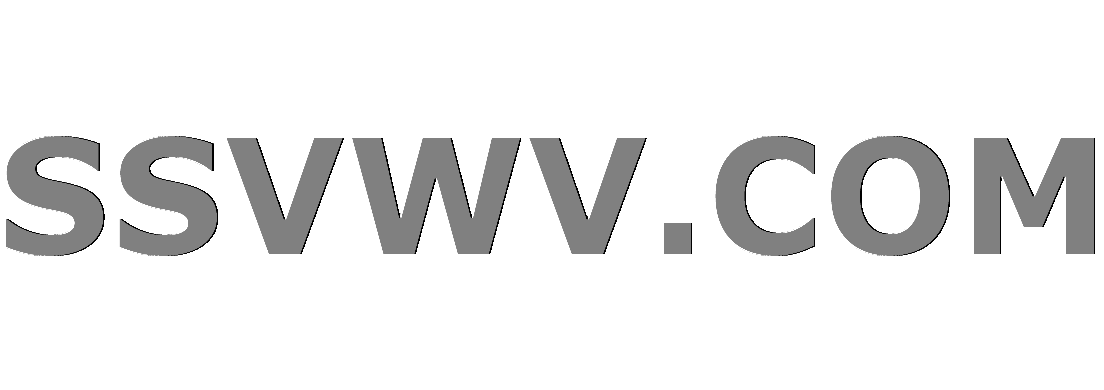
Multi tool use
I want to be able to request multiple db objects by id in a single DRF request via url parameter with a GET request. I read through this stackoverflow post and DRF's docs on filtering against query parameters and thought I understood how to implement but my solution is a little off. It will return a response, but isn't filtering the queryset (multiple id response is {"detail":"Not found."}
)
views.py
@permission_classes((HasAPIAccess, HasUnrestrictedAPIAccess, ))
class EventListView(generics.ListAPIView):
serializer_class = EventSerializer
queryset = Event.objects.all()
def get_queryset(self):
ids = self.request.query_params.get('ids', None)
if ids is not None:
ids = [ int(x) for x in ids.split(',') ]
queryset = Event.objects.filter(pk__in=ids)
else:
queryset = Event.objects.all()[0:10]
return queryset
urls.py
router = DefaultRouter()
router.register(r'events', EventViewSet)
my_patterns = [
url(r'^events/list/$',
EventListView.as_view(),
name='events-by-id'),
url(r'^', include(router.urls)),
]
urlpatterns = [
url(r'^$', RedirectView.as_view(url='/v1/')),
url(r'^v1/$', schema_view),
url(r'^v1/', include(my_patterns)),
]`
the goal is to make a GET request like curl -X GET --header 'Accept: application/json' --header 'Api-Key: {{ key }}' 'http://api.foo.com/v1/events/list/?ids=1,2,3,4,5'
but currently that's returning {"detail":"Not found."}
response
a curl -X GET --header 'Accept: application/json' --header 'Api-Key: {{ key }}' 'http://api.foo.com/v1/events/list/'
request with no params returns the unfiltered queryset
django django-rest-framework django-urls
add a comment |
I want to be able to request multiple db objects by id in a single DRF request via url parameter with a GET request. I read through this stackoverflow post and DRF's docs on filtering against query parameters and thought I understood how to implement but my solution is a little off. It will return a response, but isn't filtering the queryset (multiple id response is {"detail":"Not found."}
)
views.py
@permission_classes((HasAPIAccess, HasUnrestrictedAPIAccess, ))
class EventListView(generics.ListAPIView):
serializer_class = EventSerializer
queryset = Event.objects.all()
def get_queryset(self):
ids = self.request.query_params.get('ids', None)
if ids is not None:
ids = [ int(x) for x in ids.split(',') ]
queryset = Event.objects.filter(pk__in=ids)
else:
queryset = Event.objects.all()[0:10]
return queryset
urls.py
router = DefaultRouter()
router.register(r'events', EventViewSet)
my_patterns = [
url(r'^events/list/$',
EventListView.as_view(),
name='events-by-id'),
url(r'^', include(router.urls)),
]
urlpatterns = [
url(r'^$', RedirectView.as_view(url='/v1/')),
url(r'^v1/$', schema_view),
url(r'^v1/', include(my_patterns)),
]`
the goal is to make a GET request like curl -X GET --header 'Accept: application/json' --header 'Api-Key: {{ key }}' 'http://api.foo.com/v1/events/list/?ids=1,2,3,4,5'
but currently that's returning {"detail":"Not found."}
response
a curl -X GET --header 'Accept: application/json' --header 'Api-Key: {{ key }}' 'http://api.foo.com/v1/events/list/'
request with no params returns the unfiltered queryset
django django-rest-framework django-urls
unrelated but in class-based views you should define thepermission_classes
as properties of the class, not as a decorator.permission_classes =[HasAPIAccess, HasUnrestrictedAPIAccess]
– rikAtee
Nov 13 '18 at 20:59
thanks, good to know
– Chris B.
Nov 13 '18 at 21:03
add a comment |
I want to be able to request multiple db objects by id in a single DRF request via url parameter with a GET request. I read through this stackoverflow post and DRF's docs on filtering against query parameters and thought I understood how to implement but my solution is a little off. It will return a response, but isn't filtering the queryset (multiple id response is {"detail":"Not found."}
)
views.py
@permission_classes((HasAPIAccess, HasUnrestrictedAPIAccess, ))
class EventListView(generics.ListAPIView):
serializer_class = EventSerializer
queryset = Event.objects.all()
def get_queryset(self):
ids = self.request.query_params.get('ids', None)
if ids is not None:
ids = [ int(x) for x in ids.split(',') ]
queryset = Event.objects.filter(pk__in=ids)
else:
queryset = Event.objects.all()[0:10]
return queryset
urls.py
router = DefaultRouter()
router.register(r'events', EventViewSet)
my_patterns = [
url(r'^events/list/$',
EventListView.as_view(),
name='events-by-id'),
url(r'^', include(router.urls)),
]
urlpatterns = [
url(r'^$', RedirectView.as_view(url='/v1/')),
url(r'^v1/$', schema_view),
url(r'^v1/', include(my_patterns)),
]`
the goal is to make a GET request like curl -X GET --header 'Accept: application/json' --header 'Api-Key: {{ key }}' 'http://api.foo.com/v1/events/list/?ids=1,2,3,4,5'
but currently that's returning {"detail":"Not found."}
response
a curl -X GET --header 'Accept: application/json' --header 'Api-Key: {{ key }}' 'http://api.foo.com/v1/events/list/'
request with no params returns the unfiltered queryset
django django-rest-framework django-urls
I want to be able to request multiple db objects by id in a single DRF request via url parameter with a GET request. I read through this stackoverflow post and DRF's docs on filtering against query parameters and thought I understood how to implement but my solution is a little off. It will return a response, but isn't filtering the queryset (multiple id response is {"detail":"Not found."}
)
views.py
@permission_classes((HasAPIAccess, HasUnrestrictedAPIAccess, ))
class EventListView(generics.ListAPIView):
serializer_class = EventSerializer
queryset = Event.objects.all()
def get_queryset(self):
ids = self.request.query_params.get('ids', None)
if ids is not None:
ids = [ int(x) for x in ids.split(',') ]
queryset = Event.objects.filter(pk__in=ids)
else:
queryset = Event.objects.all()[0:10]
return queryset
urls.py
router = DefaultRouter()
router.register(r'events', EventViewSet)
my_patterns = [
url(r'^events/list/$',
EventListView.as_view(),
name='events-by-id'),
url(r'^', include(router.urls)),
]
urlpatterns = [
url(r'^$', RedirectView.as_view(url='/v1/')),
url(r'^v1/$', schema_view),
url(r'^v1/', include(my_patterns)),
]`
the goal is to make a GET request like curl -X GET --header 'Accept: application/json' --header 'Api-Key: {{ key }}' 'http://api.foo.com/v1/events/list/?ids=1,2,3,4,5'
but currently that's returning {"detail":"Not found."}
response
a curl -X GET --header 'Accept: application/json' --header 'Api-Key: {{ key }}' 'http://api.foo.com/v1/events/list/'
request with no params returns the unfiltered queryset
django django-rest-framework django-urls
django django-rest-framework django-urls
edited Nov 13 '18 at 20:18
Chris B.
asked Nov 13 '18 at 20:11


Chris B.Chris B.
45331432
45331432
unrelated but in class-based views you should define thepermission_classes
as properties of the class, not as a decorator.permission_classes =[HasAPIAccess, HasUnrestrictedAPIAccess]
– rikAtee
Nov 13 '18 at 20:59
thanks, good to know
– Chris B.
Nov 13 '18 at 21:03
add a comment |
unrelated but in class-based views you should define thepermission_classes
as properties of the class, not as a decorator.permission_classes =[HasAPIAccess, HasUnrestrictedAPIAccess]
– rikAtee
Nov 13 '18 at 20:59
thanks, good to know
– Chris B.
Nov 13 '18 at 21:03
unrelated but in class-based views you should define the
permission_classes
as properties of the class, not as a decorator. permission_classes =[HasAPIAccess, HasUnrestrictedAPIAccess]
– rikAtee
Nov 13 '18 at 20:59
unrelated but in class-based views you should define the
permission_classes
as properties of the class, not as a decorator. permission_classes =[HasAPIAccess, HasUnrestrictedAPIAccess]
– rikAtee
Nov 13 '18 at 20:59
thanks, good to know
– Chris B.
Nov 13 '18 at 21:03
thanks, good to know
– Chris B.
Nov 13 '18 at 21:03
add a comment |
1 Answer
1
active
oldest
votes
figured out the issue, only needed to define the queryset in the method and it was an err to define it earlier. once I remved queryset = Event.objects.all()
the view and url route work correctly
class EventListView(generics.ListAPIView):
serializer_class = EventSerializer
def get_queryset(self):
ids = self.request.query_params.get('ids', None)
if ids is not None:
ids = [ int(x) for x in ids.split(',') ]
queryset = Event.objects.filter(pk__in=ids)
else:
queryset = Event.objects.all()[0:10]
return queryset
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53288767%2fcorrect-drf-filter-list-view-endpoint-to-return-multiple-objects-by-id%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
figured out the issue, only needed to define the queryset in the method and it was an err to define it earlier. once I remved queryset = Event.objects.all()
the view and url route work correctly
class EventListView(generics.ListAPIView):
serializer_class = EventSerializer
def get_queryset(self):
ids = self.request.query_params.get('ids', None)
if ids is not None:
ids = [ int(x) for x in ids.split(',') ]
queryset = Event.objects.filter(pk__in=ids)
else:
queryset = Event.objects.all()[0:10]
return queryset
add a comment |
figured out the issue, only needed to define the queryset in the method and it was an err to define it earlier. once I remved queryset = Event.objects.all()
the view and url route work correctly
class EventListView(generics.ListAPIView):
serializer_class = EventSerializer
def get_queryset(self):
ids = self.request.query_params.get('ids', None)
if ids is not None:
ids = [ int(x) for x in ids.split(',') ]
queryset = Event.objects.filter(pk__in=ids)
else:
queryset = Event.objects.all()[0:10]
return queryset
add a comment |
figured out the issue, only needed to define the queryset in the method and it was an err to define it earlier. once I remved queryset = Event.objects.all()
the view and url route work correctly
class EventListView(generics.ListAPIView):
serializer_class = EventSerializer
def get_queryset(self):
ids = self.request.query_params.get('ids', None)
if ids is not None:
ids = [ int(x) for x in ids.split(',') ]
queryset = Event.objects.filter(pk__in=ids)
else:
queryset = Event.objects.all()[0:10]
return queryset
figured out the issue, only needed to define the queryset in the method and it was an err to define it earlier. once I remved queryset = Event.objects.all()
the view and url route work correctly
class EventListView(generics.ListAPIView):
serializer_class = EventSerializer
def get_queryset(self):
ids = self.request.query_params.get('ids', None)
if ids is not None:
ids = [ int(x) for x in ids.split(',') ]
queryset = Event.objects.filter(pk__in=ids)
else:
queryset = Event.objects.all()[0:10]
return queryset
answered Nov 13 '18 at 20:55


Chris B.Chris B.
45331432
45331432
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53288767%2fcorrect-drf-filter-list-view-endpoint-to-return-multiple-objects-by-id%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fxXwdo HmGwS2kpDOIW,ps8m5LCULH5AZDN8U9rqWSG,65,trYXt 1MIL e AujYwG0h6mRm4OPrjgg
unrelated but in class-based views you should define the
permission_classes
as properties of the class, not as a decorator.permission_classes =[HasAPIAccess, HasUnrestrictedAPIAccess]
– rikAtee
Nov 13 '18 at 20:59
thanks, good to know
– Chris B.
Nov 13 '18 at 21:03