Pull to Refresh with WKWebView
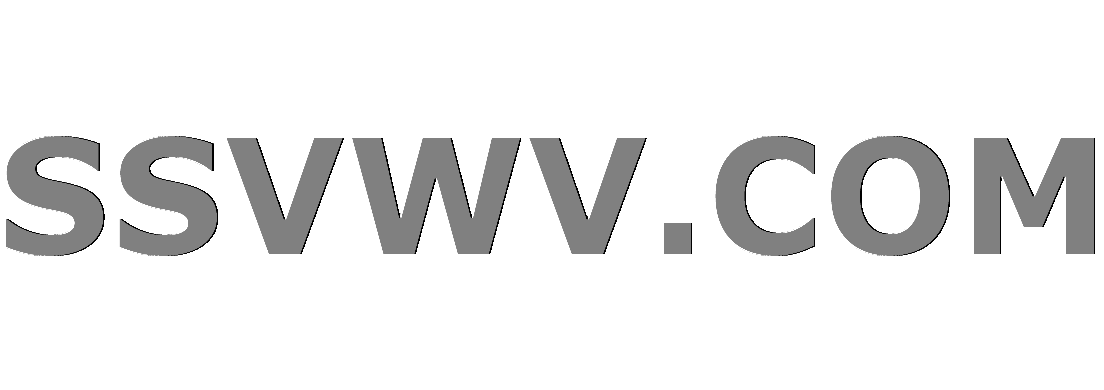
Multi tool use
I'm trying to add pull to Refresh to WKWebView. Where in this code would I add something that would allow me to trigger pull to refresh? I haven't found any viable ways to add it to my code so you won't find any attempts by me to accomplish this in the code below. Most answers that I've found are in Objective-C or a language unsupported by my project.
import UIKit
import WebKit
class CycleViewController: UIViewController, WKNavigationDelegate, WKUIDelegate {
var webView: WKWebView!
@IBOutlet weak var containerView: UIView!
@IBOutlet weak var progressView: UIProgressView!
override func loadView() {
super.loadView()
}
override func viewDidLoad() {
super.viewDidLoad()
progressView.setProgress(0.1, animated: true)
let webConfiguration = WKWebViewConfiguration()
webConfiguration.applicationNameForUserAgent = "Version/8.0.2 Safari/600.2.5"
let customFrame = CGRect.init(origin: CGPoint.zero, size: CGSize.init(width: 0.0, height: self.containerView.frame.size.height))
webView = WKWebView(frame: customFrame, configuration: webConfiguration)
webView.uiDelegate = self
webView.navigationDelegate = self
self.containerView.addSubview(webView)
self.webView.translatesAutoresizingMaskIntoConstraints = false
self.view.addConstraint(NSLayoutConstraint(item: webView, attribute: .trailing, relatedBy: .equal, toItem: self.containerView, attribute: .trailing, multiplier: 1, constant: 0))
self.view.addConstraint(NSLayoutConstraint(item: webView, attribute: .leading, relatedBy: .equal, toItem: self.containerView, attribute: .leading, multiplier: 1, constant: 0))
self.view.addConstraint(NSLayoutConstraint(item: webView, attribute: .top, relatedBy: .equal, toItem: self.containerView, attribute: .top, multiplier: 1, constant: 0))
self.view.addConstraint(NSLayoutConstraint(item: webView, attribute: .bottom, relatedBy: .equal, toItem: self.containerView, attribute: .bottom, multiplier: 1, constant: 0))
progressView.sizeToFit()
progressView.progressTintColor = UIColor(red: 255/255, green: 204/255, blue: 0/255, alpha: 1.0)
webView.backgroundColor = UIColor.clear
webView.backgroundColor = UIColor(red: 0.0196, green: 0.4, blue: 0.2902, alpha: 1.0)
webView.isOpaque = false
self.webView.load(NSURLRequest(url: URL(string: "https://jwelsh19.wixsite.com/countryday")!) as URLRequest)
self.webView.addObserver(self, forKeyPath: "estimatedProgress", options: .new, context: nil)
}
override func observeValue(forKeyPath keyPath: String?, of object: Any?, change: [NSKeyValueChangeKey : Any]?, context: UnsafeMutableRawPointer?) {
if keyPath == "estimatedProgress" {
print(self.webView.estimatedProgress);
self.progressView.progress = Float(self.webView.estimatedProgress); }
}
@IBAction func home(_ sender: UIButton) {
let myURL = URL(string: "https://jwelsh19.wixsite.com/countryday")
let myRequest = URLRequest(url: myURL!)
webView.load(myRequest)
}
@IBAction func refresh(_ sender: UIButton) {
webView.reload()
}
//This method will call when you press button.
@objc func acButtonPressed() {
print("button is pressed")
self.performSegue(withIdentifier: "Account", sender: self)
}
}
`
ios swift wkwebview pull-to-refresh
add a comment |
I'm trying to add pull to Refresh to WKWebView. Where in this code would I add something that would allow me to trigger pull to refresh? I haven't found any viable ways to add it to my code so you won't find any attempts by me to accomplish this in the code below. Most answers that I've found are in Objective-C or a language unsupported by my project.
import UIKit
import WebKit
class CycleViewController: UIViewController, WKNavigationDelegate, WKUIDelegate {
var webView: WKWebView!
@IBOutlet weak var containerView: UIView!
@IBOutlet weak var progressView: UIProgressView!
override func loadView() {
super.loadView()
}
override func viewDidLoad() {
super.viewDidLoad()
progressView.setProgress(0.1, animated: true)
let webConfiguration = WKWebViewConfiguration()
webConfiguration.applicationNameForUserAgent = "Version/8.0.2 Safari/600.2.5"
let customFrame = CGRect.init(origin: CGPoint.zero, size: CGSize.init(width: 0.0, height: self.containerView.frame.size.height))
webView = WKWebView(frame: customFrame, configuration: webConfiguration)
webView.uiDelegate = self
webView.navigationDelegate = self
self.containerView.addSubview(webView)
self.webView.translatesAutoresizingMaskIntoConstraints = false
self.view.addConstraint(NSLayoutConstraint(item: webView, attribute: .trailing, relatedBy: .equal, toItem: self.containerView, attribute: .trailing, multiplier: 1, constant: 0))
self.view.addConstraint(NSLayoutConstraint(item: webView, attribute: .leading, relatedBy: .equal, toItem: self.containerView, attribute: .leading, multiplier: 1, constant: 0))
self.view.addConstraint(NSLayoutConstraint(item: webView, attribute: .top, relatedBy: .equal, toItem: self.containerView, attribute: .top, multiplier: 1, constant: 0))
self.view.addConstraint(NSLayoutConstraint(item: webView, attribute: .bottom, relatedBy: .equal, toItem: self.containerView, attribute: .bottom, multiplier: 1, constant: 0))
progressView.sizeToFit()
progressView.progressTintColor = UIColor(red: 255/255, green: 204/255, blue: 0/255, alpha: 1.0)
webView.backgroundColor = UIColor.clear
webView.backgroundColor = UIColor(red: 0.0196, green: 0.4, blue: 0.2902, alpha: 1.0)
webView.isOpaque = false
self.webView.load(NSURLRequest(url: URL(string: "https://jwelsh19.wixsite.com/countryday")!) as URLRequest)
self.webView.addObserver(self, forKeyPath: "estimatedProgress", options: .new, context: nil)
}
override func observeValue(forKeyPath keyPath: String?, of object: Any?, change: [NSKeyValueChangeKey : Any]?, context: UnsafeMutableRawPointer?) {
if keyPath == "estimatedProgress" {
print(self.webView.estimatedProgress);
self.progressView.progress = Float(self.webView.estimatedProgress); }
}
@IBAction func home(_ sender: UIButton) {
let myURL = URL(string: "https://jwelsh19.wixsite.com/countryday")
let myRequest = URLRequest(url: myURL!)
webView.load(myRequest)
}
@IBAction func refresh(_ sender: UIButton) {
webView.reload()
}
//This method will call when you press button.
@objc func acButtonPressed() {
print("button is pressed")
self.performSegue(withIdentifier: "Account", sender: self)
}
}
`
ios swift wkwebview pull-to-refresh
What's wrong with the Objective-C code you've found? It's the same APIs used by Swift. Just translate the code as needed using the APIs as a guideline.
– rmaddy
Nov 12 at 18:55
add a comment |
I'm trying to add pull to Refresh to WKWebView. Where in this code would I add something that would allow me to trigger pull to refresh? I haven't found any viable ways to add it to my code so you won't find any attempts by me to accomplish this in the code below. Most answers that I've found are in Objective-C or a language unsupported by my project.
import UIKit
import WebKit
class CycleViewController: UIViewController, WKNavigationDelegate, WKUIDelegate {
var webView: WKWebView!
@IBOutlet weak var containerView: UIView!
@IBOutlet weak var progressView: UIProgressView!
override func loadView() {
super.loadView()
}
override func viewDidLoad() {
super.viewDidLoad()
progressView.setProgress(0.1, animated: true)
let webConfiguration = WKWebViewConfiguration()
webConfiguration.applicationNameForUserAgent = "Version/8.0.2 Safari/600.2.5"
let customFrame = CGRect.init(origin: CGPoint.zero, size: CGSize.init(width: 0.0, height: self.containerView.frame.size.height))
webView = WKWebView(frame: customFrame, configuration: webConfiguration)
webView.uiDelegate = self
webView.navigationDelegate = self
self.containerView.addSubview(webView)
self.webView.translatesAutoresizingMaskIntoConstraints = false
self.view.addConstraint(NSLayoutConstraint(item: webView, attribute: .trailing, relatedBy: .equal, toItem: self.containerView, attribute: .trailing, multiplier: 1, constant: 0))
self.view.addConstraint(NSLayoutConstraint(item: webView, attribute: .leading, relatedBy: .equal, toItem: self.containerView, attribute: .leading, multiplier: 1, constant: 0))
self.view.addConstraint(NSLayoutConstraint(item: webView, attribute: .top, relatedBy: .equal, toItem: self.containerView, attribute: .top, multiplier: 1, constant: 0))
self.view.addConstraint(NSLayoutConstraint(item: webView, attribute: .bottom, relatedBy: .equal, toItem: self.containerView, attribute: .bottom, multiplier: 1, constant: 0))
progressView.sizeToFit()
progressView.progressTintColor = UIColor(red: 255/255, green: 204/255, blue: 0/255, alpha: 1.0)
webView.backgroundColor = UIColor.clear
webView.backgroundColor = UIColor(red: 0.0196, green: 0.4, blue: 0.2902, alpha: 1.0)
webView.isOpaque = false
self.webView.load(NSURLRequest(url: URL(string: "https://jwelsh19.wixsite.com/countryday")!) as URLRequest)
self.webView.addObserver(self, forKeyPath: "estimatedProgress", options: .new, context: nil)
}
override func observeValue(forKeyPath keyPath: String?, of object: Any?, change: [NSKeyValueChangeKey : Any]?, context: UnsafeMutableRawPointer?) {
if keyPath == "estimatedProgress" {
print(self.webView.estimatedProgress);
self.progressView.progress = Float(self.webView.estimatedProgress); }
}
@IBAction func home(_ sender: UIButton) {
let myURL = URL(string: "https://jwelsh19.wixsite.com/countryday")
let myRequest = URLRequest(url: myURL!)
webView.load(myRequest)
}
@IBAction func refresh(_ sender: UIButton) {
webView.reload()
}
//This method will call when you press button.
@objc func acButtonPressed() {
print("button is pressed")
self.performSegue(withIdentifier: "Account", sender: self)
}
}
`
ios swift wkwebview pull-to-refresh
I'm trying to add pull to Refresh to WKWebView. Where in this code would I add something that would allow me to trigger pull to refresh? I haven't found any viable ways to add it to my code so you won't find any attempts by me to accomplish this in the code below. Most answers that I've found are in Objective-C or a language unsupported by my project.
import UIKit
import WebKit
class CycleViewController: UIViewController, WKNavigationDelegate, WKUIDelegate {
var webView: WKWebView!
@IBOutlet weak var containerView: UIView!
@IBOutlet weak var progressView: UIProgressView!
override func loadView() {
super.loadView()
}
override func viewDidLoad() {
super.viewDidLoad()
progressView.setProgress(0.1, animated: true)
let webConfiguration = WKWebViewConfiguration()
webConfiguration.applicationNameForUserAgent = "Version/8.0.2 Safari/600.2.5"
let customFrame = CGRect.init(origin: CGPoint.zero, size: CGSize.init(width: 0.0, height: self.containerView.frame.size.height))
webView = WKWebView(frame: customFrame, configuration: webConfiguration)
webView.uiDelegate = self
webView.navigationDelegate = self
self.containerView.addSubview(webView)
self.webView.translatesAutoresizingMaskIntoConstraints = false
self.view.addConstraint(NSLayoutConstraint(item: webView, attribute: .trailing, relatedBy: .equal, toItem: self.containerView, attribute: .trailing, multiplier: 1, constant: 0))
self.view.addConstraint(NSLayoutConstraint(item: webView, attribute: .leading, relatedBy: .equal, toItem: self.containerView, attribute: .leading, multiplier: 1, constant: 0))
self.view.addConstraint(NSLayoutConstraint(item: webView, attribute: .top, relatedBy: .equal, toItem: self.containerView, attribute: .top, multiplier: 1, constant: 0))
self.view.addConstraint(NSLayoutConstraint(item: webView, attribute: .bottom, relatedBy: .equal, toItem: self.containerView, attribute: .bottom, multiplier: 1, constant: 0))
progressView.sizeToFit()
progressView.progressTintColor = UIColor(red: 255/255, green: 204/255, blue: 0/255, alpha: 1.0)
webView.backgroundColor = UIColor.clear
webView.backgroundColor = UIColor(red: 0.0196, green: 0.4, blue: 0.2902, alpha: 1.0)
webView.isOpaque = false
self.webView.load(NSURLRequest(url: URL(string: "https://jwelsh19.wixsite.com/countryday")!) as URLRequest)
self.webView.addObserver(self, forKeyPath: "estimatedProgress", options: .new, context: nil)
}
override func observeValue(forKeyPath keyPath: String?, of object: Any?, change: [NSKeyValueChangeKey : Any]?, context: UnsafeMutableRawPointer?) {
if keyPath == "estimatedProgress" {
print(self.webView.estimatedProgress);
self.progressView.progress = Float(self.webView.estimatedProgress); }
}
@IBAction func home(_ sender: UIButton) {
let myURL = URL(string: "https://jwelsh19.wixsite.com/countryday")
let myRequest = URLRequest(url: myURL!)
webView.load(myRequest)
}
@IBAction func refresh(_ sender: UIButton) {
webView.reload()
}
//This method will call when you press button.
@objc func acButtonPressed() {
print("button is pressed")
self.performSegue(withIdentifier: "Account", sender: self)
}
}
`
ios swift wkwebview pull-to-refresh
ios swift wkwebview pull-to-refresh
edited Nov 12 at 18:54


rmaddy
238k27309375
238k27309375
asked Nov 12 at 18:54


Nigel
182
182
What's wrong with the Objective-C code you've found? It's the same APIs used by Swift. Just translate the code as needed using the APIs as a guideline.
– rmaddy
Nov 12 at 18:55
add a comment |
What's wrong with the Objective-C code you've found? It's the same APIs used by Swift. Just translate the code as needed using the APIs as a guideline.
– rmaddy
Nov 12 at 18:55
What's wrong with the Objective-C code you've found? It's the same APIs used by Swift. Just translate the code as needed using the APIs as a guideline.
– rmaddy
Nov 12 at 18:55
What's wrong with the Objective-C code you've found? It's the same APIs used by Swift. Just translate the code as needed using the APIs as a guideline.
– rmaddy
Nov 12 at 18:55
add a comment |
2 Answers
2
active
oldest
votes
Nothing special about applying pull to refresh behavior to a web view, however keep in mind that you would need to add the UIRefreshControl
as a subview into the web view scrollView
:
override func viewDidLoad() {
// after done with setup the `webView`:
let refreshControl = UIRefreshControl()
refreshControl.addTarget(self, action: #selector(reloadWebView(_:)), for: .valueChanged)
webView.scrollView.addSubview(refreshControl)
}
reloadWebView
method:
@objc func reloadWebView(_ sender: UIRefreshControl) {
webView.reload()
sender.endRefreshing()
}
Tip: instead of using NSURLRequest
and casting it to URLRequest
you could use URLRequest
directly, so instead of:
self.webView.load(NSURLRequest(url: URL(string: "https://jwelsh19.wixsite.com/countryday")!) as URLRequest)
do it as:
webView.load(URLRequest(url: URL(string: "https://jwelsh19.wixsite.com/countryday")!))
add a comment |
Add refreshControl
to webView.scrollView
under didSet
callback to webView
var webView: WKWebView! {
didSet {
let refreshControl = UIRefreshControl()
refreshControl.addTarget(self, action: #selector(reload(_:)), for: .valueChanged)
webView.scrollView.refreshControl = refreshControl
}
}
And add this to reload webView
@objc private func reload(_ refreshControl: UIRefreshControl) {
refreshControl.endRefreshing()
webView.reload()
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53268384%2fpull-to-refresh-with-wkwebview%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Nothing special about applying pull to refresh behavior to a web view, however keep in mind that you would need to add the UIRefreshControl
as a subview into the web view scrollView
:
override func viewDidLoad() {
// after done with setup the `webView`:
let refreshControl = UIRefreshControl()
refreshControl.addTarget(self, action: #selector(reloadWebView(_:)), for: .valueChanged)
webView.scrollView.addSubview(refreshControl)
}
reloadWebView
method:
@objc func reloadWebView(_ sender: UIRefreshControl) {
webView.reload()
sender.endRefreshing()
}
Tip: instead of using NSURLRequest
and casting it to URLRequest
you could use URLRequest
directly, so instead of:
self.webView.load(NSURLRequest(url: URL(string: "https://jwelsh19.wixsite.com/countryday")!) as URLRequest)
do it as:
webView.load(URLRequest(url: URL(string: "https://jwelsh19.wixsite.com/countryday")!))
add a comment |
Nothing special about applying pull to refresh behavior to a web view, however keep in mind that you would need to add the UIRefreshControl
as a subview into the web view scrollView
:
override func viewDidLoad() {
// after done with setup the `webView`:
let refreshControl = UIRefreshControl()
refreshControl.addTarget(self, action: #selector(reloadWebView(_:)), for: .valueChanged)
webView.scrollView.addSubview(refreshControl)
}
reloadWebView
method:
@objc func reloadWebView(_ sender: UIRefreshControl) {
webView.reload()
sender.endRefreshing()
}
Tip: instead of using NSURLRequest
and casting it to URLRequest
you could use URLRequest
directly, so instead of:
self.webView.load(NSURLRequest(url: URL(string: "https://jwelsh19.wixsite.com/countryday")!) as URLRequest)
do it as:
webView.load(URLRequest(url: URL(string: "https://jwelsh19.wixsite.com/countryday")!))
add a comment |
Nothing special about applying pull to refresh behavior to a web view, however keep in mind that you would need to add the UIRefreshControl
as a subview into the web view scrollView
:
override func viewDidLoad() {
// after done with setup the `webView`:
let refreshControl = UIRefreshControl()
refreshControl.addTarget(self, action: #selector(reloadWebView(_:)), for: .valueChanged)
webView.scrollView.addSubview(refreshControl)
}
reloadWebView
method:
@objc func reloadWebView(_ sender: UIRefreshControl) {
webView.reload()
sender.endRefreshing()
}
Tip: instead of using NSURLRequest
and casting it to URLRequest
you could use URLRequest
directly, so instead of:
self.webView.load(NSURLRequest(url: URL(string: "https://jwelsh19.wixsite.com/countryday")!) as URLRequest)
do it as:
webView.load(URLRequest(url: URL(string: "https://jwelsh19.wixsite.com/countryday")!))
Nothing special about applying pull to refresh behavior to a web view, however keep in mind that you would need to add the UIRefreshControl
as a subview into the web view scrollView
:
override func viewDidLoad() {
// after done with setup the `webView`:
let refreshControl = UIRefreshControl()
refreshControl.addTarget(self, action: #selector(reloadWebView(_:)), for: .valueChanged)
webView.scrollView.addSubview(refreshControl)
}
reloadWebView
method:
@objc func reloadWebView(_ sender: UIRefreshControl) {
webView.reload()
sender.endRefreshing()
}
Tip: instead of using NSURLRequest
and casting it to URLRequest
you could use URLRequest
directly, so instead of:
self.webView.load(NSURLRequest(url: URL(string: "https://jwelsh19.wixsite.com/countryday")!) as URLRequest)
do it as:
webView.load(URLRequest(url: URL(string: "https://jwelsh19.wixsite.com/countryday")!))
edited Nov 12 at 19:42
answered Nov 12 at 19:27


Ahmad F
15.9k104080
15.9k104080
add a comment |
add a comment |
Add refreshControl
to webView.scrollView
under didSet
callback to webView
var webView: WKWebView! {
didSet {
let refreshControl = UIRefreshControl()
refreshControl.addTarget(self, action: #selector(reload(_:)), for: .valueChanged)
webView.scrollView.refreshControl = refreshControl
}
}
And add this to reload webView
@objc private func reload(_ refreshControl: UIRefreshControl) {
refreshControl.endRefreshing()
webView.reload()
}
add a comment |
Add refreshControl
to webView.scrollView
under didSet
callback to webView
var webView: WKWebView! {
didSet {
let refreshControl = UIRefreshControl()
refreshControl.addTarget(self, action: #selector(reload(_:)), for: .valueChanged)
webView.scrollView.refreshControl = refreshControl
}
}
And add this to reload webView
@objc private func reload(_ refreshControl: UIRefreshControl) {
refreshControl.endRefreshing()
webView.reload()
}
add a comment |
Add refreshControl
to webView.scrollView
under didSet
callback to webView
var webView: WKWebView! {
didSet {
let refreshControl = UIRefreshControl()
refreshControl.addTarget(self, action: #selector(reload(_:)), for: .valueChanged)
webView.scrollView.refreshControl = refreshControl
}
}
And add this to reload webView
@objc private func reload(_ refreshControl: UIRefreshControl) {
refreshControl.endRefreshing()
webView.reload()
}
Add refreshControl
to webView.scrollView
under didSet
callback to webView
var webView: WKWebView! {
didSet {
let refreshControl = UIRefreshControl()
refreshControl.addTarget(self, action: #selector(reload(_:)), for: .valueChanged)
webView.scrollView.refreshControl = refreshControl
}
}
And add this to reload webView
@objc private func reload(_ refreshControl: UIRefreshControl) {
refreshControl.endRefreshing()
webView.reload()
}
answered Nov 12 at 20:22
Satish
1,016615
1,016615
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53268384%2fpull-to-refresh-with-wkwebview%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9ekj6A,VPLG8LM,d5o,YgcqG aFCmh5,N3AQaAl Tu8,kKeyFuP,So3EID,BdrWnaKup,Bz,Rx8FEwX7wjMSCGbIHc OPD oYXs9ylPDN0,s06cVZ
What's wrong with the Objective-C code you've found? It's the same APIs used by Swift. Just translate the code as needed using the APIs as a guideline.
– rmaddy
Nov 12 at 18:55