Converting string to Pascal Case using RegEx
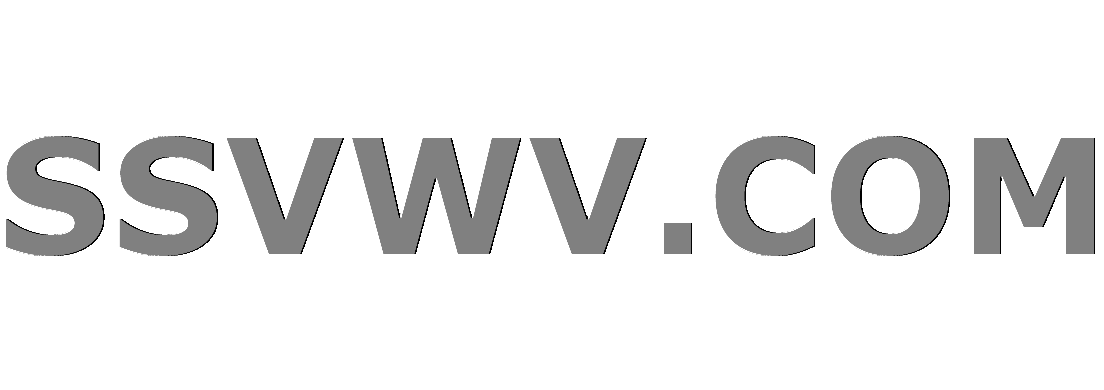
Multi tool use
I am trying to use RegEx.Replace to convert a string into Pascal case. RegEx is not necessary, but I thought that maybe it'll be easier. Here are some example test cases I'm trying to convert:
simple simon says => SimpleSimonSays
SIMPLE SIMON SaYs => SimpleSimonSays
simple_simon_says => SimpleSimonSays
simple simon says => SimpleSimonSays
simpleSimonSays => SimpleSimonSays
simple___simon___ says => SimpleSimonSays
The method I currently have doesn't use RegEx and it works correctly on 4 of the 5 examples above:
internal static string GetPascalCaseName(string name)
{
string s = System.Globalization.CultureInfo.CurrentCulture.
TextInfo.ToTitleCase(name.ToLower()).Replace(" ", "").Replace("_", "");
return s;
}
The one example that fails is simpleSimonSays
. It currently returns Simplesimonsays
instead of SimpleSimonSays
. How can I make this work on all 4 scenarios?
EDIT
So basically, words are distinguished if there are spaces seperating them, or underscores, or whenever an upper-case character is reached. Also, multiple spaces and/or multiple underscores should be treated as one. Basically spaces and underscores should just be ignored and used as a signal that the next letter should be a capital letter. Like this:
simple_____simon___ says => SimpleSimonSays
c# regex
|
show 6 more comments
I am trying to use RegEx.Replace to convert a string into Pascal case. RegEx is not necessary, but I thought that maybe it'll be easier. Here are some example test cases I'm trying to convert:
simple simon says => SimpleSimonSays
SIMPLE SIMON SaYs => SimpleSimonSays
simple_simon_says => SimpleSimonSays
simple simon says => SimpleSimonSays
simpleSimonSays => SimpleSimonSays
simple___simon___ says => SimpleSimonSays
The method I currently have doesn't use RegEx and it works correctly on 4 of the 5 examples above:
internal static string GetPascalCaseName(string name)
{
string s = System.Globalization.CultureInfo.CurrentCulture.
TextInfo.ToTitleCase(name.ToLower()).Replace(" ", "").Replace("_", "");
return s;
}
The one example that fails is simpleSimonSays
. It currently returns Simplesimonsays
instead of SimpleSimonSays
. How can I make this work on all 4 scenarios?
EDIT
So basically, words are distinguished if there are spaces seperating them, or underscores, or whenever an upper-case character is reached. Also, multiple spaces and/or multiple underscores should be treated as one. Basically spaces and underscores should just be ignored and used as a signal that the next letter should be a capital letter. Like this:
simple_____simon___ says => SimpleSimonSays
c# regex
3
How will you determine where 'simple' and 'simon', as well as 'simon' and 'says' starts and finishes? I think that is the real issue, how do you determine where one word ends and the other begins for casing if it is a single string with no determining start/finish between each word?
– Ingenioushax
Nov 12 at 19:00
As far as the single words likesimpleSimonSays
, there is no boundary to extract a case separation. So, unless you're using natural language processing, regex isn't going to ever do that.
– sln
Nov 12 at 19:15
Otherwise,b([^W_]+)(?:[ _]*([^W_]+))*b
and use Capture Collections within a delegate callback.
– sln
Nov 12 at 19:20
Think of the single word scenario like this, instead of using simpleSimonSays usepkrltUdrXywaT
– sln
Nov 12 at 19:23
@Ingenioushax - I Updated my question. Words are distinguished if there are spaces separating them, or underscores, or whenever an upper-case character is reached (assume it's the start of a new word)
– Icemanind
Nov 12 at 21:33
|
show 6 more comments
I am trying to use RegEx.Replace to convert a string into Pascal case. RegEx is not necessary, but I thought that maybe it'll be easier. Here are some example test cases I'm trying to convert:
simple simon says => SimpleSimonSays
SIMPLE SIMON SaYs => SimpleSimonSays
simple_simon_says => SimpleSimonSays
simple simon says => SimpleSimonSays
simpleSimonSays => SimpleSimonSays
simple___simon___ says => SimpleSimonSays
The method I currently have doesn't use RegEx and it works correctly on 4 of the 5 examples above:
internal static string GetPascalCaseName(string name)
{
string s = System.Globalization.CultureInfo.CurrentCulture.
TextInfo.ToTitleCase(name.ToLower()).Replace(" ", "").Replace("_", "");
return s;
}
The one example that fails is simpleSimonSays
. It currently returns Simplesimonsays
instead of SimpleSimonSays
. How can I make this work on all 4 scenarios?
EDIT
So basically, words are distinguished if there are spaces seperating them, or underscores, or whenever an upper-case character is reached. Also, multiple spaces and/or multiple underscores should be treated as one. Basically spaces and underscores should just be ignored and used as a signal that the next letter should be a capital letter. Like this:
simple_____simon___ says => SimpleSimonSays
c# regex
I am trying to use RegEx.Replace to convert a string into Pascal case. RegEx is not necessary, but I thought that maybe it'll be easier. Here are some example test cases I'm trying to convert:
simple simon says => SimpleSimonSays
SIMPLE SIMON SaYs => SimpleSimonSays
simple_simon_says => SimpleSimonSays
simple simon says => SimpleSimonSays
simpleSimonSays => SimpleSimonSays
simple___simon___ says => SimpleSimonSays
The method I currently have doesn't use RegEx and it works correctly on 4 of the 5 examples above:
internal static string GetPascalCaseName(string name)
{
string s = System.Globalization.CultureInfo.CurrentCulture.
TextInfo.ToTitleCase(name.ToLower()).Replace(" ", "").Replace("_", "");
return s;
}
The one example that fails is simpleSimonSays
. It currently returns Simplesimonsays
instead of SimpleSimonSays
. How can I make this work on all 4 scenarios?
EDIT
So basically, words are distinguished if there are spaces seperating them, or underscores, or whenever an upper-case character is reached. Also, multiple spaces and/or multiple underscores should be treated as one. Basically spaces and underscores should just be ignored and used as a signal that the next letter should be a capital letter. Like this:
simple_____simon___ says => SimpleSimonSays
c# regex
c# regex
edited Nov 12 at 21:42
asked Nov 12 at 18:49


Icemanind
31.1k38133247
31.1k38133247
3
How will you determine where 'simple' and 'simon', as well as 'simon' and 'says' starts and finishes? I think that is the real issue, how do you determine where one word ends and the other begins for casing if it is a single string with no determining start/finish between each word?
– Ingenioushax
Nov 12 at 19:00
As far as the single words likesimpleSimonSays
, there is no boundary to extract a case separation. So, unless you're using natural language processing, regex isn't going to ever do that.
– sln
Nov 12 at 19:15
Otherwise,b([^W_]+)(?:[ _]*([^W_]+))*b
and use Capture Collections within a delegate callback.
– sln
Nov 12 at 19:20
Think of the single word scenario like this, instead of using simpleSimonSays usepkrltUdrXywaT
– sln
Nov 12 at 19:23
@Ingenioushax - I Updated my question. Words are distinguished if there are spaces separating them, or underscores, or whenever an upper-case character is reached (assume it's the start of a new word)
– Icemanind
Nov 12 at 21:33
|
show 6 more comments
3
How will you determine where 'simple' and 'simon', as well as 'simon' and 'says' starts and finishes? I think that is the real issue, how do you determine where one word ends and the other begins for casing if it is a single string with no determining start/finish between each word?
– Ingenioushax
Nov 12 at 19:00
As far as the single words likesimpleSimonSays
, there is no boundary to extract a case separation. So, unless you're using natural language processing, regex isn't going to ever do that.
– sln
Nov 12 at 19:15
Otherwise,b([^W_]+)(?:[ _]*([^W_]+))*b
and use Capture Collections within a delegate callback.
– sln
Nov 12 at 19:20
Think of the single word scenario like this, instead of using simpleSimonSays usepkrltUdrXywaT
– sln
Nov 12 at 19:23
@Ingenioushax - I Updated my question. Words are distinguished if there are spaces separating them, or underscores, or whenever an upper-case character is reached (assume it's the start of a new word)
– Icemanind
Nov 12 at 21:33
3
3
How will you determine where 'simple' and 'simon', as well as 'simon' and 'says' starts and finishes? I think that is the real issue, how do you determine where one word ends and the other begins for casing if it is a single string with no determining start/finish between each word?
– Ingenioushax
Nov 12 at 19:00
How will you determine where 'simple' and 'simon', as well as 'simon' and 'says' starts and finishes? I think that is the real issue, how do you determine where one word ends and the other begins for casing if it is a single string with no determining start/finish between each word?
– Ingenioushax
Nov 12 at 19:00
As far as the single words like
simpleSimonSays
, there is no boundary to extract a case separation. So, unless you're using natural language processing, regex isn't going to ever do that.– sln
Nov 12 at 19:15
As far as the single words like
simpleSimonSays
, there is no boundary to extract a case separation. So, unless you're using natural language processing, regex isn't going to ever do that.– sln
Nov 12 at 19:15
Otherwise,
b([^W_]+)(?:[ _]*([^W_]+))*b
and use Capture Collections within a delegate callback.– sln
Nov 12 at 19:20
Otherwise,
b([^W_]+)(?:[ _]*([^W_]+))*b
and use Capture Collections within a delegate callback.– sln
Nov 12 at 19:20
Think of the single word scenario like this, instead of using simpleSimonSays use
pkrltUdrXywaT
– sln
Nov 12 at 19:23
Think of the single word scenario like this, instead of using simpleSimonSays use
pkrltUdrXywaT
– sln
Nov 12 at 19:23
@Ingenioushax - I Updated my question. Words are distinguished if there are spaces separating them, or underscores, or whenever an upper-case character is reached (assume it's the start of a new word)
– Icemanind
Nov 12 at 21:33
@Ingenioushax - I Updated my question. Words are distinguished if there are spaces separating them, or underscores, or whenever an upper-case character is reached (assume it's the start of a new word)
– Icemanind
Nov 12 at 21:33
|
show 6 more comments
3 Answers
3
active
oldest
votes
I have a trick for solving your problem. Using regex, split the word and introduce a space within word for words where there is no space or underscore, that are camel case (like this simpleSimonSays). Modify your method to this,
internal static string GetPascalCaseName(string name)
{
if (!name.Contains(" ")) {
name = Regex.Replace(name, "(?<=[a-z])(?=[A-Z])", " ");
}
string s = System.Globalization.CultureInfo.CurrentCulture.
TextInfo.ToTitleCase(name.ToLower()).Replace(" ", "").Replace("_", "");
return s;
}
This new line in your method,
name = Regex.Replace(name, "(?<=[a-z])(?=[A-Z])", " ");
splits the camel case word by introducing a space between them, making them like others where you had no difficultly.
For this input,
simpleSimonSays
It outputs this,
SimpleSimonSays
And for rest of the input, it works anyway. This strategy will work even for words where you have partially camel case and partially space or underscore too.
How shouldpkrltUdrXywaT
be capitalized ?
– sln
Nov 12 at 21:16
This almost works. It does not work for my second example though.SIMPLE SIMON SaYs
becomesSimpleSimonSaYs
instead ofSimpleSimonSays
– Icemanind
Nov 12 at 21:31
@sln: I don't see any trouble there. pkrltUdrXywaT would simply become PkrltUdrXywaT where only the first letter changes and becomes capital letter. Did you expect something else?
– Pushpesh Kumar Rajwanshi
Nov 13 at 6:29
@Icemanind: Sorry I missed to see your one case that wasn't working. I've taken care for your 'SIMPLE SIMON SaYs' input and now it would give you your desired result 'SimpleSimonSays'. Please check my updated code. Also, if you have any other case, then let me know. I will further tweak the code and make it work.
– Pushpesh Kumar Rajwanshi
Nov 13 at 6:39
add a comment |
Here is solution without Regex. The last one cannot be done.
string input = {
"simple simon says",
"SIMPLE SIMON SaYs",
"simple_simon_says",
"simple simon says",
"simpleSimonSays"
};
var temp = input.Select(x => x.Split(new char {' ', '_'}, StringSplitOptions.RemoveEmptyEntries).Select(y => y.Select((z,i) => (i == 0) ? z.ToString().ToUpper() : z.ToString().ToLower()))).ToArray();
string output = temp.Select(x => string.Join("", x.Select(y => string.Join("",y)))).ToArray();
add a comment |
If can be version like "abc simpleSimonSays" then it's impossible. Or need to add more rules. Or things like deep learning :)
EDIT:
possible code (but without "abc simpleSimonSays"):
var s = "simple__simon_says __ Hi _ _,,, __coolWa";
var s1 = Regex.Replace(s, "[ _,]+", " ");
var s2 = CultureInfo.CurrentCulture.TextInfo.ToTitleCase(s1);
var s3 = s2.Replace(" ","");
// s1 = "simple simon says Hi coolWa"
// s2 = "Simple Simon Says Hi Coolwa"
// s3 = "SimpleSimonSaysHiCoolwa"
Words are distinguished if there are spaces separating them, or underscores, or whenever an upper-case character is reached (assume it's the start of a new word). So in your example, it should be"AbcSimpleSimonSays"
.
– Icemanind
Nov 12 at 21:34
I mean difference a)SIMPLE SIMON SaYs => SimpleSimonSays b)abc simpleSimonSays => AbcSimpleSimonSays program can't know why a)"Y" should become "y" but b)"S" should left big. Human understands, but for program - just big letter after small
– AndrewF
Nov 12 at 22:15
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53268333%2fconverting-string-to-pascal-case-using-regex%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
I have a trick for solving your problem. Using regex, split the word and introduce a space within word for words where there is no space or underscore, that are camel case (like this simpleSimonSays). Modify your method to this,
internal static string GetPascalCaseName(string name)
{
if (!name.Contains(" ")) {
name = Regex.Replace(name, "(?<=[a-z])(?=[A-Z])", " ");
}
string s = System.Globalization.CultureInfo.CurrentCulture.
TextInfo.ToTitleCase(name.ToLower()).Replace(" ", "").Replace("_", "");
return s;
}
This new line in your method,
name = Regex.Replace(name, "(?<=[a-z])(?=[A-Z])", " ");
splits the camel case word by introducing a space between them, making them like others where you had no difficultly.
For this input,
simpleSimonSays
It outputs this,
SimpleSimonSays
And for rest of the input, it works anyway. This strategy will work even for words where you have partially camel case and partially space or underscore too.
How shouldpkrltUdrXywaT
be capitalized ?
– sln
Nov 12 at 21:16
This almost works. It does not work for my second example though.SIMPLE SIMON SaYs
becomesSimpleSimonSaYs
instead ofSimpleSimonSays
– Icemanind
Nov 12 at 21:31
@sln: I don't see any trouble there. pkrltUdrXywaT would simply become PkrltUdrXywaT where only the first letter changes and becomes capital letter. Did you expect something else?
– Pushpesh Kumar Rajwanshi
Nov 13 at 6:29
@Icemanind: Sorry I missed to see your one case that wasn't working. I've taken care for your 'SIMPLE SIMON SaYs' input and now it would give you your desired result 'SimpleSimonSays'. Please check my updated code. Also, if you have any other case, then let me know. I will further tweak the code and make it work.
– Pushpesh Kumar Rajwanshi
Nov 13 at 6:39
add a comment |
I have a trick for solving your problem. Using regex, split the word and introduce a space within word for words where there is no space or underscore, that are camel case (like this simpleSimonSays). Modify your method to this,
internal static string GetPascalCaseName(string name)
{
if (!name.Contains(" ")) {
name = Regex.Replace(name, "(?<=[a-z])(?=[A-Z])", " ");
}
string s = System.Globalization.CultureInfo.CurrentCulture.
TextInfo.ToTitleCase(name.ToLower()).Replace(" ", "").Replace("_", "");
return s;
}
This new line in your method,
name = Regex.Replace(name, "(?<=[a-z])(?=[A-Z])", " ");
splits the camel case word by introducing a space between them, making them like others where you had no difficultly.
For this input,
simpleSimonSays
It outputs this,
SimpleSimonSays
And for rest of the input, it works anyway. This strategy will work even for words where you have partially camel case and partially space or underscore too.
How shouldpkrltUdrXywaT
be capitalized ?
– sln
Nov 12 at 21:16
This almost works. It does not work for my second example though.SIMPLE SIMON SaYs
becomesSimpleSimonSaYs
instead ofSimpleSimonSays
– Icemanind
Nov 12 at 21:31
@sln: I don't see any trouble there. pkrltUdrXywaT would simply become PkrltUdrXywaT where only the first letter changes and becomes capital letter. Did you expect something else?
– Pushpesh Kumar Rajwanshi
Nov 13 at 6:29
@Icemanind: Sorry I missed to see your one case that wasn't working. I've taken care for your 'SIMPLE SIMON SaYs' input and now it would give you your desired result 'SimpleSimonSays'. Please check my updated code. Also, if you have any other case, then let me know. I will further tweak the code and make it work.
– Pushpesh Kumar Rajwanshi
Nov 13 at 6:39
add a comment |
I have a trick for solving your problem. Using regex, split the word and introduce a space within word for words where there is no space or underscore, that are camel case (like this simpleSimonSays). Modify your method to this,
internal static string GetPascalCaseName(string name)
{
if (!name.Contains(" ")) {
name = Regex.Replace(name, "(?<=[a-z])(?=[A-Z])", " ");
}
string s = System.Globalization.CultureInfo.CurrentCulture.
TextInfo.ToTitleCase(name.ToLower()).Replace(" ", "").Replace("_", "");
return s;
}
This new line in your method,
name = Regex.Replace(name, "(?<=[a-z])(?=[A-Z])", " ");
splits the camel case word by introducing a space between them, making them like others where you had no difficultly.
For this input,
simpleSimonSays
It outputs this,
SimpleSimonSays
And for rest of the input, it works anyway. This strategy will work even for words where you have partially camel case and partially space or underscore too.
I have a trick for solving your problem. Using regex, split the word and introduce a space within word for words where there is no space or underscore, that are camel case (like this simpleSimonSays). Modify your method to this,
internal static string GetPascalCaseName(string name)
{
if (!name.Contains(" ")) {
name = Regex.Replace(name, "(?<=[a-z])(?=[A-Z])", " ");
}
string s = System.Globalization.CultureInfo.CurrentCulture.
TextInfo.ToTitleCase(name.ToLower()).Replace(" ", "").Replace("_", "");
return s;
}
This new line in your method,
name = Regex.Replace(name, "(?<=[a-z])(?=[A-Z])", " ");
splits the camel case word by introducing a space between them, making them like others where you had no difficultly.
For this input,
simpleSimonSays
It outputs this,
SimpleSimonSays
And for rest of the input, it works anyway. This strategy will work even for words where you have partially camel case and partially space or underscore too.
edited Nov 13 at 6:40
answered Nov 12 at 19:56
Pushpesh Kumar Rajwanshi
5,1561827
5,1561827
How shouldpkrltUdrXywaT
be capitalized ?
– sln
Nov 12 at 21:16
This almost works. It does not work for my second example though.SIMPLE SIMON SaYs
becomesSimpleSimonSaYs
instead ofSimpleSimonSays
– Icemanind
Nov 12 at 21:31
@sln: I don't see any trouble there. pkrltUdrXywaT would simply become PkrltUdrXywaT where only the first letter changes and becomes capital letter. Did you expect something else?
– Pushpesh Kumar Rajwanshi
Nov 13 at 6:29
@Icemanind: Sorry I missed to see your one case that wasn't working. I've taken care for your 'SIMPLE SIMON SaYs' input and now it would give you your desired result 'SimpleSimonSays'. Please check my updated code. Also, if you have any other case, then let me know. I will further tweak the code and make it work.
– Pushpesh Kumar Rajwanshi
Nov 13 at 6:39
add a comment |
How shouldpkrltUdrXywaT
be capitalized ?
– sln
Nov 12 at 21:16
This almost works. It does not work for my second example though.SIMPLE SIMON SaYs
becomesSimpleSimonSaYs
instead ofSimpleSimonSays
– Icemanind
Nov 12 at 21:31
@sln: I don't see any trouble there. pkrltUdrXywaT would simply become PkrltUdrXywaT where only the first letter changes and becomes capital letter. Did you expect something else?
– Pushpesh Kumar Rajwanshi
Nov 13 at 6:29
@Icemanind: Sorry I missed to see your one case that wasn't working. I've taken care for your 'SIMPLE SIMON SaYs' input and now it would give you your desired result 'SimpleSimonSays'. Please check my updated code. Also, if you have any other case, then let me know. I will further tweak the code and make it work.
– Pushpesh Kumar Rajwanshi
Nov 13 at 6:39
How should
pkrltUdrXywaT
be capitalized ?– sln
Nov 12 at 21:16
How should
pkrltUdrXywaT
be capitalized ?– sln
Nov 12 at 21:16
This almost works. It does not work for my second example though.
SIMPLE SIMON SaYs
becomes SimpleSimonSaYs
instead of SimpleSimonSays
– Icemanind
Nov 12 at 21:31
This almost works. It does not work for my second example though.
SIMPLE SIMON SaYs
becomes SimpleSimonSaYs
instead of SimpleSimonSays
– Icemanind
Nov 12 at 21:31
@sln: I don't see any trouble there. pkrltUdrXywaT would simply become PkrltUdrXywaT where only the first letter changes and becomes capital letter. Did you expect something else?
– Pushpesh Kumar Rajwanshi
Nov 13 at 6:29
@sln: I don't see any trouble there. pkrltUdrXywaT would simply become PkrltUdrXywaT where only the first letter changes and becomes capital letter. Did you expect something else?
– Pushpesh Kumar Rajwanshi
Nov 13 at 6:29
@Icemanind: Sorry I missed to see your one case that wasn't working. I've taken care for your 'SIMPLE SIMON SaYs' input and now it would give you your desired result 'SimpleSimonSays'. Please check my updated code. Also, if you have any other case, then let me know. I will further tweak the code and make it work.
– Pushpesh Kumar Rajwanshi
Nov 13 at 6:39
@Icemanind: Sorry I missed to see your one case that wasn't working. I've taken care for your 'SIMPLE SIMON SaYs' input and now it would give you your desired result 'SimpleSimonSays'. Please check my updated code. Also, if you have any other case, then let me know. I will further tweak the code and make it work.
– Pushpesh Kumar Rajwanshi
Nov 13 at 6:39
add a comment |
Here is solution without Regex. The last one cannot be done.
string input = {
"simple simon says",
"SIMPLE SIMON SaYs",
"simple_simon_says",
"simple simon says",
"simpleSimonSays"
};
var temp = input.Select(x => x.Split(new char {' ', '_'}, StringSplitOptions.RemoveEmptyEntries).Select(y => y.Select((z,i) => (i == 0) ? z.ToString().ToUpper() : z.ToString().ToLower()))).ToArray();
string output = temp.Select(x => string.Join("", x.Select(y => string.Join("",y)))).ToArray();
add a comment |
Here is solution without Regex. The last one cannot be done.
string input = {
"simple simon says",
"SIMPLE SIMON SaYs",
"simple_simon_says",
"simple simon says",
"simpleSimonSays"
};
var temp = input.Select(x => x.Split(new char {' ', '_'}, StringSplitOptions.RemoveEmptyEntries).Select(y => y.Select((z,i) => (i == 0) ? z.ToString().ToUpper() : z.ToString().ToLower()))).ToArray();
string output = temp.Select(x => string.Join("", x.Select(y => string.Join("",y)))).ToArray();
add a comment |
Here is solution without Regex. The last one cannot be done.
string input = {
"simple simon says",
"SIMPLE SIMON SaYs",
"simple_simon_says",
"simple simon says",
"simpleSimonSays"
};
var temp = input.Select(x => x.Split(new char {' ', '_'}, StringSplitOptions.RemoveEmptyEntries).Select(y => y.Select((z,i) => (i == 0) ? z.ToString().ToUpper() : z.ToString().ToLower()))).ToArray();
string output = temp.Select(x => string.Join("", x.Select(y => string.Join("",y)))).ToArray();
Here is solution without Regex. The last one cannot be done.
string input = {
"simple simon says",
"SIMPLE SIMON SaYs",
"simple_simon_says",
"simple simon says",
"simpleSimonSays"
};
var temp = input.Select(x => x.Split(new char {' ', '_'}, StringSplitOptions.RemoveEmptyEntries).Select(y => y.Select((z,i) => (i == 0) ? z.ToString().ToUpper() : z.ToString().ToLower()))).ToArray();
string output = temp.Select(x => string.Join("", x.Select(y => string.Join("",y)))).ToArray();
answered Nov 12 at 20:05
jdweng
16.8k2717
16.8k2717
add a comment |
add a comment |
If can be version like "abc simpleSimonSays" then it's impossible. Or need to add more rules. Or things like deep learning :)
EDIT:
possible code (but without "abc simpleSimonSays"):
var s = "simple__simon_says __ Hi _ _,,, __coolWa";
var s1 = Regex.Replace(s, "[ _,]+", " ");
var s2 = CultureInfo.CurrentCulture.TextInfo.ToTitleCase(s1);
var s3 = s2.Replace(" ","");
// s1 = "simple simon says Hi coolWa"
// s2 = "Simple Simon Says Hi Coolwa"
// s3 = "SimpleSimonSaysHiCoolwa"
Words are distinguished if there are spaces separating them, or underscores, or whenever an upper-case character is reached (assume it's the start of a new word). So in your example, it should be"AbcSimpleSimonSays"
.
– Icemanind
Nov 12 at 21:34
I mean difference a)SIMPLE SIMON SaYs => SimpleSimonSays b)abc simpleSimonSays => AbcSimpleSimonSays program can't know why a)"Y" should become "y" but b)"S" should left big. Human understands, but for program - just big letter after small
– AndrewF
Nov 12 at 22:15
add a comment |
If can be version like "abc simpleSimonSays" then it's impossible. Or need to add more rules. Or things like deep learning :)
EDIT:
possible code (but without "abc simpleSimonSays"):
var s = "simple__simon_says __ Hi _ _,,, __coolWa";
var s1 = Regex.Replace(s, "[ _,]+", " ");
var s2 = CultureInfo.CurrentCulture.TextInfo.ToTitleCase(s1);
var s3 = s2.Replace(" ","");
// s1 = "simple simon says Hi coolWa"
// s2 = "Simple Simon Says Hi Coolwa"
// s3 = "SimpleSimonSaysHiCoolwa"
Words are distinguished if there are spaces separating them, or underscores, or whenever an upper-case character is reached (assume it's the start of a new word). So in your example, it should be"AbcSimpleSimonSays"
.
– Icemanind
Nov 12 at 21:34
I mean difference a)SIMPLE SIMON SaYs => SimpleSimonSays b)abc simpleSimonSays => AbcSimpleSimonSays program can't know why a)"Y" should become "y" but b)"S" should left big. Human understands, but for program - just big letter after small
– AndrewF
Nov 12 at 22:15
add a comment |
If can be version like "abc simpleSimonSays" then it's impossible. Or need to add more rules. Or things like deep learning :)
EDIT:
possible code (but without "abc simpleSimonSays"):
var s = "simple__simon_says __ Hi _ _,,, __coolWa";
var s1 = Regex.Replace(s, "[ _,]+", " ");
var s2 = CultureInfo.CurrentCulture.TextInfo.ToTitleCase(s1);
var s3 = s2.Replace(" ","");
// s1 = "simple simon says Hi coolWa"
// s2 = "Simple Simon Says Hi Coolwa"
// s3 = "SimpleSimonSaysHiCoolwa"
If can be version like "abc simpleSimonSays" then it's impossible. Or need to add more rules. Or things like deep learning :)
EDIT:
possible code (but without "abc simpleSimonSays"):
var s = "simple__simon_says __ Hi _ _,,, __coolWa";
var s1 = Regex.Replace(s, "[ _,]+", " ");
var s2 = CultureInfo.CurrentCulture.TextInfo.ToTitleCase(s1);
var s3 = s2.Replace(" ","");
// s1 = "simple simon says Hi coolWa"
// s2 = "Simple Simon Says Hi Coolwa"
// s3 = "SimpleSimonSaysHiCoolwa"
edited Nov 13 at 11:40
answered Nov 12 at 20:04
AndrewF
333
333
Words are distinguished if there are spaces separating them, or underscores, or whenever an upper-case character is reached (assume it's the start of a new word). So in your example, it should be"AbcSimpleSimonSays"
.
– Icemanind
Nov 12 at 21:34
I mean difference a)SIMPLE SIMON SaYs => SimpleSimonSays b)abc simpleSimonSays => AbcSimpleSimonSays program can't know why a)"Y" should become "y" but b)"S" should left big. Human understands, but for program - just big letter after small
– AndrewF
Nov 12 at 22:15
add a comment |
Words are distinguished if there are spaces separating them, or underscores, or whenever an upper-case character is reached (assume it's the start of a new word). So in your example, it should be"AbcSimpleSimonSays"
.
– Icemanind
Nov 12 at 21:34
I mean difference a)SIMPLE SIMON SaYs => SimpleSimonSays b)abc simpleSimonSays => AbcSimpleSimonSays program can't know why a)"Y" should become "y" but b)"S" should left big. Human understands, but for program - just big letter after small
– AndrewF
Nov 12 at 22:15
Words are distinguished if there are spaces separating them, or underscores, or whenever an upper-case character is reached (assume it's the start of a new word). So in your example, it should be
"AbcSimpleSimonSays"
.– Icemanind
Nov 12 at 21:34
Words are distinguished if there are spaces separating them, or underscores, or whenever an upper-case character is reached (assume it's the start of a new word). So in your example, it should be
"AbcSimpleSimonSays"
.– Icemanind
Nov 12 at 21:34
I mean difference a)SIMPLE SIMON SaYs => SimpleSimonSays b)abc simpleSimonSays => AbcSimpleSimonSays program can't know why a)"Y" should become "y" but b)"S" should left big. Human understands, but for program - just big letter after small
– AndrewF
Nov 12 at 22:15
I mean difference a)SIMPLE SIMON SaYs => SimpleSimonSays b)abc simpleSimonSays => AbcSimpleSimonSays program can't know why a)"Y" should become "y" but b)"S" should left big. Human understands, but for program - just big letter after small
– AndrewF
Nov 12 at 22:15
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53268333%2fconverting-string-to-pascal-case-using-regex%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uvweWjuKlpwARwG,4TTo M
3
How will you determine where 'simple' and 'simon', as well as 'simon' and 'says' starts and finishes? I think that is the real issue, how do you determine where one word ends and the other begins for casing if it is a single string with no determining start/finish between each word?
– Ingenioushax
Nov 12 at 19:00
As far as the single words like
simpleSimonSays
, there is no boundary to extract a case separation. So, unless you're using natural language processing, regex isn't going to ever do that.– sln
Nov 12 at 19:15
Otherwise,
b([^W_]+)(?:[ _]*([^W_]+))*b
and use Capture Collections within a delegate callback.– sln
Nov 12 at 19:20
Think of the single word scenario like this, instead of using simpleSimonSays use
pkrltUdrXywaT
– sln
Nov 12 at 19:23
@Ingenioushax - I Updated my question. Words are distinguished if there are spaces separating them, or underscores, or whenever an upper-case character is reached (assume it's the start of a new word)
– Icemanind
Nov 12 at 21:33