LINQ - Select Records with Max Property Value per Group
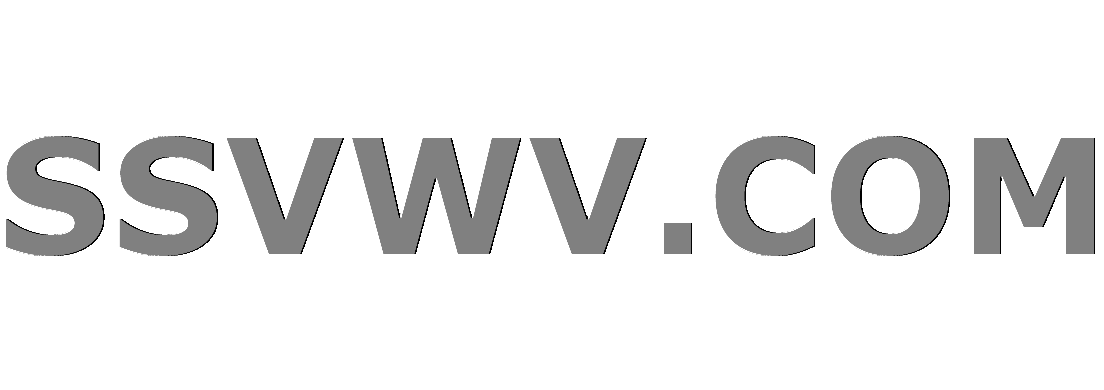
Multi tool use
up vote
2
down vote
favorite
I've got a data set like this:
GroupName GroupValue MemberName MemberValue
'Group1' 1 'Member1' 1
'Group1' 1 'Member2' 2
'Group2' 2 'Member3' 3
'Group2' 2 'Member4' 2
'Group3' 2 'Member5' 4
'Group3' 2 'Member6' 1
What I want to select is the rows that have the maximum MemberValue
per GroupName
, but only for those GroupName
s that have the largest GroupValue
, and pass them into a delegate function. Like this:
'Group2' 2 'Member3' 3
'Group3' 2 'Member5' 4
So far I've tried this format...
data.Where(maxGroupValue =>
maxGroupValue.GroupValue == data.Max(groupValue => groupValue.GroupValue))
.Select(FunctionThatTakesData)
...but that just gives me every member of Group2 and Group3. I've tried putting a GroupBy()
before the Select()
, but that turns the output into an IGrouping<string, DataType>
so FunctionThatTakesData()
doesn't know what to do with it, and I can't do another Where()
to filter out only the maximum MemberValue
s.
What can I do to get this data set properly filtered and passed into my function?
c# .net linq group-by
add a comment |
up vote
2
down vote
favorite
I've got a data set like this:
GroupName GroupValue MemberName MemberValue
'Group1' 1 'Member1' 1
'Group1' 1 'Member2' 2
'Group2' 2 'Member3' 3
'Group2' 2 'Member4' 2
'Group3' 2 'Member5' 4
'Group3' 2 'Member6' 1
What I want to select is the rows that have the maximum MemberValue
per GroupName
, but only for those GroupName
s that have the largest GroupValue
, and pass them into a delegate function. Like this:
'Group2' 2 'Member3' 3
'Group3' 2 'Member5' 4
So far I've tried this format...
data.Where(maxGroupValue =>
maxGroupValue.GroupValue == data.Max(groupValue => groupValue.GroupValue))
.Select(FunctionThatTakesData)
...but that just gives me every member of Group2 and Group3. I've tried putting a GroupBy()
before the Select()
, but that turns the output into an IGrouping<string, DataType>
so FunctionThatTakesData()
doesn't know what to do with it, and I can't do another Where()
to filter out only the maximum MemberValue
s.
What can I do to get this data set properly filtered and passed into my function?
c# .net linq group-by
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I've got a data set like this:
GroupName GroupValue MemberName MemberValue
'Group1' 1 'Member1' 1
'Group1' 1 'Member2' 2
'Group2' 2 'Member3' 3
'Group2' 2 'Member4' 2
'Group3' 2 'Member5' 4
'Group3' 2 'Member6' 1
What I want to select is the rows that have the maximum MemberValue
per GroupName
, but only for those GroupName
s that have the largest GroupValue
, and pass them into a delegate function. Like this:
'Group2' 2 'Member3' 3
'Group3' 2 'Member5' 4
So far I've tried this format...
data.Where(maxGroupValue =>
maxGroupValue.GroupValue == data.Max(groupValue => groupValue.GroupValue))
.Select(FunctionThatTakesData)
...but that just gives me every member of Group2 and Group3. I've tried putting a GroupBy()
before the Select()
, but that turns the output into an IGrouping<string, DataType>
so FunctionThatTakesData()
doesn't know what to do with it, and I can't do another Where()
to filter out only the maximum MemberValue
s.
What can I do to get this data set properly filtered and passed into my function?
c# .net linq group-by
I've got a data set like this:
GroupName GroupValue MemberName MemberValue
'Group1' 1 'Member1' 1
'Group1' 1 'Member2' 2
'Group2' 2 'Member3' 3
'Group2' 2 'Member4' 2
'Group3' 2 'Member5' 4
'Group3' 2 'Member6' 1
What I want to select is the rows that have the maximum MemberValue
per GroupName
, but only for those GroupName
s that have the largest GroupValue
, and pass them into a delegate function. Like this:
'Group2' 2 'Member3' 3
'Group3' 2 'Member5' 4
So far I've tried this format...
data.Where(maxGroupValue =>
maxGroupValue.GroupValue == data.Max(groupValue => groupValue.GroupValue))
.Select(FunctionThatTakesData)
...but that just gives me every member of Group2 and Group3. I've tried putting a GroupBy()
before the Select()
, but that turns the output into an IGrouping<string, DataType>
so FunctionThatTakesData()
doesn't know what to do with it, and I can't do another Where()
to filter out only the maximum MemberValue
s.
What can I do to get this data set properly filtered and passed into my function?
c# .net linq group-by
c# .net linq group-by
asked May 5 '17 at 12:25
JAF
8510
8510
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
5
down vote
accepted
You can do that with the following Linq.
var results = data.GroupBy(r = r.GroupValue)
.OrderByDescending(g => g.Key)
.FirstOrDefault()
?.GroupBy(r => r.GroupName)
.Select(g => g.OrderByDescending(r => r.MemberValue).First());
First you have to group on the GroupValue
then order the groups in descending order by the Key
(which is the GroupValue
) and take the first one. Now you have all the rows with the max GroupValue
. Then you group those on the GroupName
and from those groups order the MemberValue
in descending order and take the First
row to get the row in each GroupName
group with the max MemberValue
. Also I'm using the C# 6 null conditional operator ?.
after FirstOrDefault
in case data
is empty. If you're not using C# 6 then you'll need to handle that case up front and you can just use First
instead.
Thank you! Now, suppose each grouping can have multiple members with the same value... should I just use aWhere()
in place of theFirst()
?
– JAF
May 5 '17 at 13:24
1
In that case you'd want.SelectMany(g => g.GroupBy(r => r.MemberValue).OrderByDescending(sg => sg.Key).First())
to replace the last line.
– juharr
May 5 '17 at 15:16
Genius! Thank you!
– JAF
May 5 '17 at 17:24
add a comment |
up vote
0
down vote
So basically what you want, is to divide your data elements into groups with the same value for GroupName
. From every group you want to take one element, namely the one with the largest value for property MemberValue
.
Whenever you have a sequence of items, and you want to divide this sequence into groups based on the value of one or more properties of the items in the sequence you use Enumerable.GroupBy
'GroupBy' takes your sequence as input and an extra input parameter: a function that selects which properties of your items you want to compare in your decision in which group you want the item to appear.
In your case, you want to divide your sequence into groups where all elements in a group have the same GroupName
.
var groups = mySequence.GroupBy(element => element.GroupName);
What it does, it takes from every element in mySequence the property GroupName, and puts this element into a group of elements that have this value of GroupName.
Using your example data, you'll have three groups:
- The group with all elements with GroupName == "Group1". The first two elements of your sequence will be in this group
- The group with all elements with GroupName == "Group2". The third and fourth element of your sequence will be in this group
- The group with all elements with GroupName == "Group3". The last two elements of your sequence will be in this group
Each group has a property Key, containing your selection value. This key identifies the group and is guaranteed to be unique within your collection of groups. So you'll have a group with Key == "Group1", a group with Key == "Group2", etc.
Besides the Key
, every group is a sequence of the elements in the group (note: the group IS an enumerable sequence, not: it HAS an enumerable sequence.
Your second step would be to take from every group the element in the group with the largest value for MemberValue
. For this you would order the elements in the group by descending value for property MemberValue and take the first one.
var myResult = mySequence.GroupBy(element => element.GroupName)
// intermediate result: groups where all elements have the same GroupName
.Select(group => group.OrderByDescending(groupElement => groupElement.MemberValue)
// intermediate result: groups where all elements are ordered in descending memberValue
.First();
Result: from every group ordered by descending memberValue, take the first element, which should be the largest one.
It is not very efficient to order the complete group, if you only want the element with the largest value for memberValue. The answer for this can be found
here on StackOverflow
That gives you eachGroupName
with the maxMemberValue
. The OP wants only the rows with the maxGroupValue
and then eachMemberName
with the maxMemberValue
.
– juharr
May 5 '17 at 13:00
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f43804896%2flinq-select-records-with-max-property-value-per-group%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
5
down vote
accepted
You can do that with the following Linq.
var results = data.GroupBy(r = r.GroupValue)
.OrderByDescending(g => g.Key)
.FirstOrDefault()
?.GroupBy(r => r.GroupName)
.Select(g => g.OrderByDescending(r => r.MemberValue).First());
First you have to group on the GroupValue
then order the groups in descending order by the Key
(which is the GroupValue
) and take the first one. Now you have all the rows with the max GroupValue
. Then you group those on the GroupName
and from those groups order the MemberValue
in descending order and take the First
row to get the row in each GroupName
group with the max MemberValue
. Also I'm using the C# 6 null conditional operator ?.
after FirstOrDefault
in case data
is empty. If you're not using C# 6 then you'll need to handle that case up front and you can just use First
instead.
Thank you! Now, suppose each grouping can have multiple members with the same value... should I just use aWhere()
in place of theFirst()
?
– JAF
May 5 '17 at 13:24
1
In that case you'd want.SelectMany(g => g.GroupBy(r => r.MemberValue).OrderByDescending(sg => sg.Key).First())
to replace the last line.
– juharr
May 5 '17 at 15:16
Genius! Thank you!
– JAF
May 5 '17 at 17:24
add a comment |
up vote
5
down vote
accepted
You can do that with the following Linq.
var results = data.GroupBy(r = r.GroupValue)
.OrderByDescending(g => g.Key)
.FirstOrDefault()
?.GroupBy(r => r.GroupName)
.Select(g => g.OrderByDescending(r => r.MemberValue).First());
First you have to group on the GroupValue
then order the groups in descending order by the Key
(which is the GroupValue
) and take the first one. Now you have all the rows with the max GroupValue
. Then you group those on the GroupName
and from those groups order the MemberValue
in descending order and take the First
row to get the row in each GroupName
group with the max MemberValue
. Also I'm using the C# 6 null conditional operator ?.
after FirstOrDefault
in case data
is empty. If you're not using C# 6 then you'll need to handle that case up front and you can just use First
instead.
Thank you! Now, suppose each grouping can have multiple members with the same value... should I just use aWhere()
in place of theFirst()
?
– JAF
May 5 '17 at 13:24
1
In that case you'd want.SelectMany(g => g.GroupBy(r => r.MemberValue).OrderByDescending(sg => sg.Key).First())
to replace the last line.
– juharr
May 5 '17 at 15:16
Genius! Thank you!
– JAF
May 5 '17 at 17:24
add a comment |
up vote
5
down vote
accepted
up vote
5
down vote
accepted
You can do that with the following Linq.
var results = data.GroupBy(r = r.GroupValue)
.OrderByDescending(g => g.Key)
.FirstOrDefault()
?.GroupBy(r => r.GroupName)
.Select(g => g.OrderByDescending(r => r.MemberValue).First());
First you have to group on the GroupValue
then order the groups in descending order by the Key
(which is the GroupValue
) and take the first one. Now you have all the rows with the max GroupValue
. Then you group those on the GroupName
and from those groups order the MemberValue
in descending order and take the First
row to get the row in each GroupName
group with the max MemberValue
. Also I'm using the C# 6 null conditional operator ?.
after FirstOrDefault
in case data
is empty. If you're not using C# 6 then you'll need to handle that case up front and you can just use First
instead.
You can do that with the following Linq.
var results = data.GroupBy(r = r.GroupValue)
.OrderByDescending(g => g.Key)
.FirstOrDefault()
?.GroupBy(r => r.GroupName)
.Select(g => g.OrderByDescending(r => r.MemberValue).First());
First you have to group on the GroupValue
then order the groups in descending order by the Key
(which is the GroupValue
) and take the first one. Now you have all the rows with the max GroupValue
. Then you group those on the GroupName
and from those groups order the MemberValue
in descending order and take the First
row to get the row in each GroupName
group with the max MemberValue
. Also I'm using the C# 6 null conditional operator ?.
after FirstOrDefault
in case data
is empty. If you're not using C# 6 then you'll need to handle that case up front and you can just use First
instead.
edited May 5 '17 at 15:18
answered May 5 '17 at 12:46
juharr
25.2k33575
25.2k33575
Thank you! Now, suppose each grouping can have multiple members with the same value... should I just use aWhere()
in place of theFirst()
?
– JAF
May 5 '17 at 13:24
1
In that case you'd want.SelectMany(g => g.GroupBy(r => r.MemberValue).OrderByDescending(sg => sg.Key).First())
to replace the last line.
– juharr
May 5 '17 at 15:16
Genius! Thank you!
– JAF
May 5 '17 at 17:24
add a comment |
Thank you! Now, suppose each grouping can have multiple members with the same value... should I just use aWhere()
in place of theFirst()
?
– JAF
May 5 '17 at 13:24
1
In that case you'd want.SelectMany(g => g.GroupBy(r => r.MemberValue).OrderByDescending(sg => sg.Key).First())
to replace the last line.
– juharr
May 5 '17 at 15:16
Genius! Thank you!
– JAF
May 5 '17 at 17:24
Thank you! Now, suppose each grouping can have multiple members with the same value... should I just use a
Where()
in place of the First()
?– JAF
May 5 '17 at 13:24
Thank you! Now, suppose each grouping can have multiple members with the same value... should I just use a
Where()
in place of the First()
?– JAF
May 5 '17 at 13:24
1
1
In that case you'd want
.SelectMany(g => g.GroupBy(r => r.MemberValue).OrderByDescending(sg => sg.Key).First())
to replace the last line.– juharr
May 5 '17 at 15:16
In that case you'd want
.SelectMany(g => g.GroupBy(r => r.MemberValue).OrderByDescending(sg => sg.Key).First())
to replace the last line.– juharr
May 5 '17 at 15:16
Genius! Thank you!
– JAF
May 5 '17 at 17:24
Genius! Thank you!
– JAF
May 5 '17 at 17:24
add a comment |
up vote
0
down vote
So basically what you want, is to divide your data elements into groups with the same value for GroupName
. From every group you want to take one element, namely the one with the largest value for property MemberValue
.
Whenever you have a sequence of items, and you want to divide this sequence into groups based on the value of one or more properties of the items in the sequence you use Enumerable.GroupBy
'GroupBy' takes your sequence as input and an extra input parameter: a function that selects which properties of your items you want to compare in your decision in which group you want the item to appear.
In your case, you want to divide your sequence into groups where all elements in a group have the same GroupName
.
var groups = mySequence.GroupBy(element => element.GroupName);
What it does, it takes from every element in mySequence the property GroupName, and puts this element into a group of elements that have this value of GroupName.
Using your example data, you'll have three groups:
- The group with all elements with GroupName == "Group1". The first two elements of your sequence will be in this group
- The group with all elements with GroupName == "Group2". The third and fourth element of your sequence will be in this group
- The group with all elements with GroupName == "Group3". The last two elements of your sequence will be in this group
Each group has a property Key, containing your selection value. This key identifies the group and is guaranteed to be unique within your collection of groups. So you'll have a group with Key == "Group1", a group with Key == "Group2", etc.
Besides the Key
, every group is a sequence of the elements in the group (note: the group IS an enumerable sequence, not: it HAS an enumerable sequence.
Your second step would be to take from every group the element in the group with the largest value for MemberValue
. For this you would order the elements in the group by descending value for property MemberValue and take the first one.
var myResult = mySequence.GroupBy(element => element.GroupName)
// intermediate result: groups where all elements have the same GroupName
.Select(group => group.OrderByDescending(groupElement => groupElement.MemberValue)
// intermediate result: groups where all elements are ordered in descending memberValue
.First();
Result: from every group ordered by descending memberValue, take the first element, which should be the largest one.
It is not very efficient to order the complete group, if you only want the element with the largest value for memberValue. The answer for this can be found
here on StackOverflow
That gives you eachGroupName
with the maxMemberValue
. The OP wants only the rows with the maxGroupValue
and then eachMemberName
with the maxMemberValue
.
– juharr
May 5 '17 at 13:00
add a comment |
up vote
0
down vote
So basically what you want, is to divide your data elements into groups with the same value for GroupName
. From every group you want to take one element, namely the one with the largest value for property MemberValue
.
Whenever you have a sequence of items, and you want to divide this sequence into groups based on the value of one or more properties of the items in the sequence you use Enumerable.GroupBy
'GroupBy' takes your sequence as input and an extra input parameter: a function that selects which properties of your items you want to compare in your decision in which group you want the item to appear.
In your case, you want to divide your sequence into groups where all elements in a group have the same GroupName
.
var groups = mySequence.GroupBy(element => element.GroupName);
What it does, it takes from every element in mySequence the property GroupName, and puts this element into a group of elements that have this value of GroupName.
Using your example data, you'll have three groups:
- The group with all elements with GroupName == "Group1". The first two elements of your sequence will be in this group
- The group with all elements with GroupName == "Group2". The third and fourth element of your sequence will be in this group
- The group with all elements with GroupName == "Group3". The last two elements of your sequence will be in this group
Each group has a property Key, containing your selection value. This key identifies the group and is guaranteed to be unique within your collection of groups. So you'll have a group with Key == "Group1", a group with Key == "Group2", etc.
Besides the Key
, every group is a sequence of the elements in the group (note: the group IS an enumerable sequence, not: it HAS an enumerable sequence.
Your second step would be to take from every group the element in the group with the largest value for MemberValue
. For this you would order the elements in the group by descending value for property MemberValue and take the first one.
var myResult = mySequence.GroupBy(element => element.GroupName)
// intermediate result: groups where all elements have the same GroupName
.Select(group => group.OrderByDescending(groupElement => groupElement.MemberValue)
// intermediate result: groups where all elements are ordered in descending memberValue
.First();
Result: from every group ordered by descending memberValue, take the first element, which should be the largest one.
It is not very efficient to order the complete group, if you only want the element with the largest value for memberValue. The answer for this can be found
here on StackOverflow
That gives you eachGroupName
with the maxMemberValue
. The OP wants only the rows with the maxGroupValue
and then eachMemberName
with the maxMemberValue
.
– juharr
May 5 '17 at 13:00
add a comment |
up vote
0
down vote
up vote
0
down vote
So basically what you want, is to divide your data elements into groups with the same value for GroupName
. From every group you want to take one element, namely the one with the largest value for property MemberValue
.
Whenever you have a sequence of items, and you want to divide this sequence into groups based on the value of one or more properties of the items in the sequence you use Enumerable.GroupBy
'GroupBy' takes your sequence as input and an extra input parameter: a function that selects which properties of your items you want to compare in your decision in which group you want the item to appear.
In your case, you want to divide your sequence into groups where all elements in a group have the same GroupName
.
var groups = mySequence.GroupBy(element => element.GroupName);
What it does, it takes from every element in mySequence the property GroupName, and puts this element into a group of elements that have this value of GroupName.
Using your example data, you'll have three groups:
- The group with all elements with GroupName == "Group1". The first two elements of your sequence will be in this group
- The group with all elements with GroupName == "Group2". The third and fourth element of your sequence will be in this group
- The group with all elements with GroupName == "Group3". The last two elements of your sequence will be in this group
Each group has a property Key, containing your selection value. This key identifies the group and is guaranteed to be unique within your collection of groups. So you'll have a group with Key == "Group1", a group with Key == "Group2", etc.
Besides the Key
, every group is a sequence of the elements in the group (note: the group IS an enumerable sequence, not: it HAS an enumerable sequence.
Your second step would be to take from every group the element in the group with the largest value for MemberValue
. For this you would order the elements in the group by descending value for property MemberValue and take the first one.
var myResult = mySequence.GroupBy(element => element.GroupName)
// intermediate result: groups where all elements have the same GroupName
.Select(group => group.OrderByDescending(groupElement => groupElement.MemberValue)
// intermediate result: groups where all elements are ordered in descending memberValue
.First();
Result: from every group ordered by descending memberValue, take the first element, which should be the largest one.
It is not very efficient to order the complete group, if you only want the element with the largest value for memberValue. The answer for this can be found
here on StackOverflow
So basically what you want, is to divide your data elements into groups with the same value for GroupName
. From every group you want to take one element, namely the one with the largest value for property MemberValue
.
Whenever you have a sequence of items, and you want to divide this sequence into groups based on the value of one or more properties of the items in the sequence you use Enumerable.GroupBy
'GroupBy' takes your sequence as input and an extra input parameter: a function that selects which properties of your items you want to compare in your decision in which group you want the item to appear.
In your case, you want to divide your sequence into groups where all elements in a group have the same GroupName
.
var groups = mySequence.GroupBy(element => element.GroupName);
What it does, it takes from every element in mySequence the property GroupName, and puts this element into a group of elements that have this value of GroupName.
Using your example data, you'll have three groups:
- The group with all elements with GroupName == "Group1". The first two elements of your sequence will be in this group
- The group with all elements with GroupName == "Group2". The third and fourth element of your sequence will be in this group
- The group with all elements with GroupName == "Group3". The last two elements of your sequence will be in this group
Each group has a property Key, containing your selection value. This key identifies the group and is guaranteed to be unique within your collection of groups. So you'll have a group with Key == "Group1", a group with Key == "Group2", etc.
Besides the Key
, every group is a sequence of the elements in the group (note: the group IS an enumerable sequence, not: it HAS an enumerable sequence.
Your second step would be to take from every group the element in the group with the largest value for MemberValue
. For this you would order the elements in the group by descending value for property MemberValue and take the first one.
var myResult = mySequence.GroupBy(element => element.GroupName)
// intermediate result: groups where all elements have the same GroupName
.Select(group => group.OrderByDescending(groupElement => groupElement.MemberValue)
// intermediate result: groups where all elements are ordered in descending memberValue
.First();
Result: from every group ordered by descending memberValue, take the first element, which should be the largest one.
It is not very efficient to order the complete group, if you only want the element with the largest value for memberValue. The answer for this can be found
here on StackOverflow
edited Nov 12 at 7:47
answered May 5 '17 at 12:57


Harald Coppoolse
11.3k12959
11.3k12959
That gives you eachGroupName
with the maxMemberValue
. The OP wants only the rows with the maxGroupValue
and then eachMemberName
with the maxMemberValue
.
– juharr
May 5 '17 at 13:00
add a comment |
That gives you eachGroupName
with the maxMemberValue
. The OP wants only the rows with the maxGroupValue
and then eachMemberName
with the maxMemberValue
.
– juharr
May 5 '17 at 13:00
That gives you each
GroupName
with the max MemberValue
. The OP wants only the rows with the max GroupValue
and then each MemberName
with the max MemberValue
.– juharr
May 5 '17 at 13:00
That gives you each
GroupName
with the max MemberValue
. The OP wants only the rows with the max GroupValue
and then each MemberName
with the max MemberValue
.– juharr
May 5 '17 at 13:00
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f43804896%2flinq-select-records-with-max-property-value-per-group%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
oQMeI,71DbyD 4lIWwj9w6xgyNwg,jb3pOI CmvflHQjOGw MFEx