Setting a JLabel visible on Jradiobuttons click
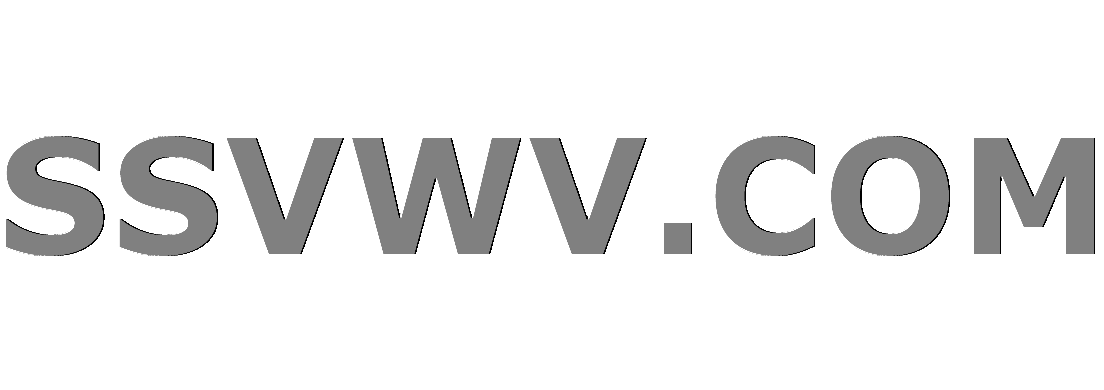
Multi tool use
up vote
0
down vote
favorite
I tried setting JLabel on being visible only after I select a certain RadioButton. The programm is getting an error if I set lblNewLabel_1.setVisible(true) in the action performed of a radiobutton. It worked with the textField, but with this not. What can I do? Is it different with the JLabel? Is there any advice someone can offer me?
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.Window;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import javax.swing.JTextArea;
import javax.swing.JRadioButton;
import javax.swing.JLabel;
import java.awt.Font;
import javax.swing.JTextField;
import javax.swing.ButtonGroup;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class Fereastra extends JFrame {
private JPanel contentPane;
private JTextField textField;
private JTextField textField_1;
private final ButtonGroup buttonGroup = new ButtonGroup();
/**
* Launch the application.
*/
public static void main(String args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Fereastra frame = new Fereastra();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public Fereastra() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 751, 565);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
contentPane.setLayout(null);
setContentPane(contentPane);
JTextArea textArea = new JTextArea();
textArea.setBounds(12, 13, 447, 251);
contentPane.add(textArea);
JRadioButton rdbtnNewRadioButton = new JRadioButton("Cautarea pe nivel");
rdbtnNewRadioButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton);
rdbtnNewRadioButton.setBounds(488, 55, 185, 25);
contentPane.add(rdbtnNewRadioButton);
JRadioButton rdbtnNewRadioButton_1 = new JRadioButton("Cautarea in adancime");
rdbtnNewRadioButton_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
if(rdbtnNewRadioButton_1.isSelected())
{
textField.setVisible(true);
textField_1.setVisible(true);
}
else
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_1);
rdbtnNewRadioButton_1.setBounds(488, 96, 237, 25);
contentPane.add(rdbtnNewRadioButton_1);
JRadioButton rdbtnNewRadioButton_2 = new JRadioButton("Costul uniform");
rdbtnNewRadioButton_2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_2.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_2);
rdbtnNewRadioButton_2.setBounds(488, 138, 127, 25);
contentPane.add(rdbtnNewRadioButton_2);
JRadioButton rdbtnNewRadioButton_3 = new JRadioButton("Adancime iterativa");
rdbtnNewRadioButton_3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_3.isSelected())
{
textField_1.setVisible(true);
textField.setVisible(true);
}
else
{
textField_1.setVisible(false);
textField.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_3);
rdbtnNewRadioButton_3.setBounds(488, 179, 237, 25);
contentPane.add(rdbtnNewRadioButton_3);
JRadioButton rdbtnNewRadioButton_4 = new JRadioButton("Greedy");
rdbtnNewRadioButton_4.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_4.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_4);
rdbtnNewRadioButton_4.setBounds(488, 221, 127, 25);
contentPane.add(rdbtnNewRadioButton_4);
JLabel lblNewLabel = new JLabel("Alegeti:");
lblNewLabel.setFont(new Font("Tahoma", Font.PLAIN, 16));
lblNewLabel.setBounds(488, 13, 84, 33);
contentPane.add(lblNewLabel);
textField = new JTextField();
textField.setBounds(402, 277, 116, 22);
contentPane.add(textField);
textField.setColumns(10);
textField.setVisible(false);
textField_1 = new JTextField();
textField_1.setBounds(246, 277, 116, 22);
contentPane.add(textField_1);
textField_1.setColumns(10);
textField_1.setVisible(false);
JLabel lblNewLabel_1 = new JLabel("Introduceti valorile dorite:");
lblNewLabel_1.setFont(new Font("Tahoma", Font.PLAIN, 16));
lblNewLabel_1.setBounds(22, 277, 212, 21);
contentPane.add(lblNewLabel_1);
lblNewLabel_1.setVisible(false);
}
}
eclipse jlabel
New contributor
piglet is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
I tried setting JLabel on being visible only after I select a certain RadioButton. The programm is getting an error if I set lblNewLabel_1.setVisible(true) in the action performed of a radiobutton. It worked with the textField, but with this not. What can I do? Is it different with the JLabel? Is there any advice someone can offer me?
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.Window;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import javax.swing.JTextArea;
import javax.swing.JRadioButton;
import javax.swing.JLabel;
import java.awt.Font;
import javax.swing.JTextField;
import javax.swing.ButtonGroup;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class Fereastra extends JFrame {
private JPanel contentPane;
private JTextField textField;
private JTextField textField_1;
private final ButtonGroup buttonGroup = new ButtonGroup();
/**
* Launch the application.
*/
public static void main(String args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Fereastra frame = new Fereastra();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public Fereastra() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 751, 565);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
contentPane.setLayout(null);
setContentPane(contentPane);
JTextArea textArea = new JTextArea();
textArea.setBounds(12, 13, 447, 251);
contentPane.add(textArea);
JRadioButton rdbtnNewRadioButton = new JRadioButton("Cautarea pe nivel");
rdbtnNewRadioButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton);
rdbtnNewRadioButton.setBounds(488, 55, 185, 25);
contentPane.add(rdbtnNewRadioButton);
JRadioButton rdbtnNewRadioButton_1 = new JRadioButton("Cautarea in adancime");
rdbtnNewRadioButton_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
if(rdbtnNewRadioButton_1.isSelected())
{
textField.setVisible(true);
textField_1.setVisible(true);
}
else
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_1);
rdbtnNewRadioButton_1.setBounds(488, 96, 237, 25);
contentPane.add(rdbtnNewRadioButton_1);
JRadioButton rdbtnNewRadioButton_2 = new JRadioButton("Costul uniform");
rdbtnNewRadioButton_2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_2.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_2);
rdbtnNewRadioButton_2.setBounds(488, 138, 127, 25);
contentPane.add(rdbtnNewRadioButton_2);
JRadioButton rdbtnNewRadioButton_3 = new JRadioButton("Adancime iterativa");
rdbtnNewRadioButton_3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_3.isSelected())
{
textField_1.setVisible(true);
textField.setVisible(true);
}
else
{
textField_1.setVisible(false);
textField.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_3);
rdbtnNewRadioButton_3.setBounds(488, 179, 237, 25);
contentPane.add(rdbtnNewRadioButton_3);
JRadioButton rdbtnNewRadioButton_4 = new JRadioButton("Greedy");
rdbtnNewRadioButton_4.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_4.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_4);
rdbtnNewRadioButton_4.setBounds(488, 221, 127, 25);
contentPane.add(rdbtnNewRadioButton_4);
JLabel lblNewLabel = new JLabel("Alegeti:");
lblNewLabel.setFont(new Font("Tahoma", Font.PLAIN, 16));
lblNewLabel.setBounds(488, 13, 84, 33);
contentPane.add(lblNewLabel);
textField = new JTextField();
textField.setBounds(402, 277, 116, 22);
contentPane.add(textField);
textField.setColumns(10);
textField.setVisible(false);
textField_1 = new JTextField();
textField_1.setBounds(246, 277, 116, 22);
contentPane.add(textField_1);
textField_1.setColumns(10);
textField_1.setVisible(false);
JLabel lblNewLabel_1 = new JLabel("Introduceti valorile dorite:");
lblNewLabel_1.setFont(new Font("Tahoma", Font.PLAIN, 16));
lblNewLabel_1.setBounds(22, 277, 212, 21);
contentPane.add(lblNewLabel_1);
lblNewLabel_1.setVisible(false);
}
}
eclipse jlabel
New contributor
piglet is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I tried setting JLabel on being visible only after I select a certain RadioButton. The programm is getting an error if I set lblNewLabel_1.setVisible(true) in the action performed of a radiobutton. It worked with the textField, but with this not. What can I do? Is it different with the JLabel? Is there any advice someone can offer me?
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.Window;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import javax.swing.JTextArea;
import javax.swing.JRadioButton;
import javax.swing.JLabel;
import java.awt.Font;
import javax.swing.JTextField;
import javax.swing.ButtonGroup;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class Fereastra extends JFrame {
private JPanel contentPane;
private JTextField textField;
private JTextField textField_1;
private final ButtonGroup buttonGroup = new ButtonGroup();
/**
* Launch the application.
*/
public static void main(String args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Fereastra frame = new Fereastra();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public Fereastra() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 751, 565);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
contentPane.setLayout(null);
setContentPane(contentPane);
JTextArea textArea = new JTextArea();
textArea.setBounds(12, 13, 447, 251);
contentPane.add(textArea);
JRadioButton rdbtnNewRadioButton = new JRadioButton("Cautarea pe nivel");
rdbtnNewRadioButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton);
rdbtnNewRadioButton.setBounds(488, 55, 185, 25);
contentPane.add(rdbtnNewRadioButton);
JRadioButton rdbtnNewRadioButton_1 = new JRadioButton("Cautarea in adancime");
rdbtnNewRadioButton_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
if(rdbtnNewRadioButton_1.isSelected())
{
textField.setVisible(true);
textField_1.setVisible(true);
}
else
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_1);
rdbtnNewRadioButton_1.setBounds(488, 96, 237, 25);
contentPane.add(rdbtnNewRadioButton_1);
JRadioButton rdbtnNewRadioButton_2 = new JRadioButton("Costul uniform");
rdbtnNewRadioButton_2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_2.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_2);
rdbtnNewRadioButton_2.setBounds(488, 138, 127, 25);
contentPane.add(rdbtnNewRadioButton_2);
JRadioButton rdbtnNewRadioButton_3 = new JRadioButton("Adancime iterativa");
rdbtnNewRadioButton_3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_3.isSelected())
{
textField_1.setVisible(true);
textField.setVisible(true);
}
else
{
textField_1.setVisible(false);
textField.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_3);
rdbtnNewRadioButton_3.setBounds(488, 179, 237, 25);
contentPane.add(rdbtnNewRadioButton_3);
JRadioButton rdbtnNewRadioButton_4 = new JRadioButton("Greedy");
rdbtnNewRadioButton_4.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_4.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_4);
rdbtnNewRadioButton_4.setBounds(488, 221, 127, 25);
contentPane.add(rdbtnNewRadioButton_4);
JLabel lblNewLabel = new JLabel("Alegeti:");
lblNewLabel.setFont(new Font("Tahoma", Font.PLAIN, 16));
lblNewLabel.setBounds(488, 13, 84, 33);
contentPane.add(lblNewLabel);
textField = new JTextField();
textField.setBounds(402, 277, 116, 22);
contentPane.add(textField);
textField.setColumns(10);
textField.setVisible(false);
textField_1 = new JTextField();
textField_1.setBounds(246, 277, 116, 22);
contentPane.add(textField_1);
textField_1.setColumns(10);
textField_1.setVisible(false);
JLabel lblNewLabel_1 = new JLabel("Introduceti valorile dorite:");
lblNewLabel_1.setFont(new Font("Tahoma", Font.PLAIN, 16));
lblNewLabel_1.setBounds(22, 277, 212, 21);
contentPane.add(lblNewLabel_1);
lblNewLabel_1.setVisible(false);
}
}
eclipse jlabel
New contributor
piglet is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I tried setting JLabel on being visible only after I select a certain RadioButton. The programm is getting an error if I set lblNewLabel_1.setVisible(true) in the action performed of a radiobutton. It worked with the textField, but with this not. What can I do? Is it different with the JLabel? Is there any advice someone can offer me?
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.Window;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import javax.swing.JTextArea;
import javax.swing.JRadioButton;
import javax.swing.JLabel;
import java.awt.Font;
import javax.swing.JTextField;
import javax.swing.ButtonGroup;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class Fereastra extends JFrame {
private JPanel contentPane;
private JTextField textField;
private JTextField textField_1;
private final ButtonGroup buttonGroup = new ButtonGroup();
/**
* Launch the application.
*/
public static void main(String args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Fereastra frame = new Fereastra();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public Fereastra() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 751, 565);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
contentPane.setLayout(null);
setContentPane(contentPane);
JTextArea textArea = new JTextArea();
textArea.setBounds(12, 13, 447, 251);
contentPane.add(textArea);
JRadioButton rdbtnNewRadioButton = new JRadioButton("Cautarea pe nivel");
rdbtnNewRadioButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton);
rdbtnNewRadioButton.setBounds(488, 55, 185, 25);
contentPane.add(rdbtnNewRadioButton);
JRadioButton rdbtnNewRadioButton_1 = new JRadioButton("Cautarea in adancime");
rdbtnNewRadioButton_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
if(rdbtnNewRadioButton_1.isSelected())
{
textField.setVisible(true);
textField_1.setVisible(true);
}
else
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_1);
rdbtnNewRadioButton_1.setBounds(488, 96, 237, 25);
contentPane.add(rdbtnNewRadioButton_1);
JRadioButton rdbtnNewRadioButton_2 = new JRadioButton("Costul uniform");
rdbtnNewRadioButton_2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_2.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_2);
rdbtnNewRadioButton_2.setBounds(488, 138, 127, 25);
contentPane.add(rdbtnNewRadioButton_2);
JRadioButton rdbtnNewRadioButton_3 = new JRadioButton("Adancime iterativa");
rdbtnNewRadioButton_3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_3.isSelected())
{
textField_1.setVisible(true);
textField.setVisible(true);
}
else
{
textField_1.setVisible(false);
textField.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_3);
rdbtnNewRadioButton_3.setBounds(488, 179, 237, 25);
contentPane.add(rdbtnNewRadioButton_3);
JRadioButton rdbtnNewRadioButton_4 = new JRadioButton("Greedy");
rdbtnNewRadioButton_4.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_4.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_4);
rdbtnNewRadioButton_4.setBounds(488, 221, 127, 25);
contentPane.add(rdbtnNewRadioButton_4);
JLabel lblNewLabel = new JLabel("Alegeti:");
lblNewLabel.setFont(new Font("Tahoma", Font.PLAIN, 16));
lblNewLabel.setBounds(488, 13, 84, 33);
contentPane.add(lblNewLabel);
textField = new JTextField();
textField.setBounds(402, 277, 116, 22);
contentPane.add(textField);
textField.setColumns(10);
textField.setVisible(false);
textField_1 = new JTextField();
textField_1.setBounds(246, 277, 116, 22);
contentPane.add(textField_1);
textField_1.setColumns(10);
textField_1.setVisible(false);
JLabel lblNewLabel_1 = new JLabel("Introduceti valorile dorite:");
lblNewLabel_1.setFont(new Font("Tahoma", Font.PLAIN, 16));
lblNewLabel_1.setBounds(22, 277, 212, 21);
contentPane.add(lblNewLabel_1);
lblNewLabel_1.setVisible(false);
}
}
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.Window;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import javax.swing.JTextArea;
import javax.swing.JRadioButton;
import javax.swing.JLabel;
import java.awt.Font;
import javax.swing.JTextField;
import javax.swing.ButtonGroup;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class Fereastra extends JFrame {
private JPanel contentPane;
private JTextField textField;
private JTextField textField_1;
private final ButtonGroup buttonGroup = new ButtonGroup();
/**
* Launch the application.
*/
public static void main(String args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Fereastra frame = new Fereastra();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public Fereastra() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 751, 565);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
contentPane.setLayout(null);
setContentPane(contentPane);
JTextArea textArea = new JTextArea();
textArea.setBounds(12, 13, 447, 251);
contentPane.add(textArea);
JRadioButton rdbtnNewRadioButton = new JRadioButton("Cautarea pe nivel");
rdbtnNewRadioButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton);
rdbtnNewRadioButton.setBounds(488, 55, 185, 25);
contentPane.add(rdbtnNewRadioButton);
JRadioButton rdbtnNewRadioButton_1 = new JRadioButton("Cautarea in adancime");
rdbtnNewRadioButton_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
if(rdbtnNewRadioButton_1.isSelected())
{
textField.setVisible(true);
textField_1.setVisible(true);
}
else
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_1);
rdbtnNewRadioButton_1.setBounds(488, 96, 237, 25);
contentPane.add(rdbtnNewRadioButton_1);
JRadioButton rdbtnNewRadioButton_2 = new JRadioButton("Costul uniform");
rdbtnNewRadioButton_2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_2.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_2);
rdbtnNewRadioButton_2.setBounds(488, 138, 127, 25);
contentPane.add(rdbtnNewRadioButton_2);
JRadioButton rdbtnNewRadioButton_3 = new JRadioButton("Adancime iterativa");
rdbtnNewRadioButton_3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_3.isSelected())
{
textField_1.setVisible(true);
textField.setVisible(true);
}
else
{
textField_1.setVisible(false);
textField.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_3);
rdbtnNewRadioButton_3.setBounds(488, 179, 237, 25);
contentPane.add(rdbtnNewRadioButton_3);
JRadioButton rdbtnNewRadioButton_4 = new JRadioButton("Greedy");
rdbtnNewRadioButton_4.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_4.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_4);
rdbtnNewRadioButton_4.setBounds(488, 221, 127, 25);
contentPane.add(rdbtnNewRadioButton_4);
JLabel lblNewLabel = new JLabel("Alegeti:");
lblNewLabel.setFont(new Font("Tahoma", Font.PLAIN, 16));
lblNewLabel.setBounds(488, 13, 84, 33);
contentPane.add(lblNewLabel);
textField = new JTextField();
textField.setBounds(402, 277, 116, 22);
contentPane.add(textField);
textField.setColumns(10);
textField.setVisible(false);
textField_1 = new JTextField();
textField_1.setBounds(246, 277, 116, 22);
contentPane.add(textField_1);
textField_1.setColumns(10);
textField_1.setVisible(false);
JLabel lblNewLabel_1 = new JLabel("Introduceti valorile dorite:");
lblNewLabel_1.setFont(new Font("Tahoma", Font.PLAIN, 16));
lblNewLabel_1.setBounds(22, 277, 212, 21);
contentPane.add(lblNewLabel_1);
lblNewLabel_1.setVisible(false);
}
}
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.Window;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import javax.swing.JTextArea;
import javax.swing.JRadioButton;
import javax.swing.JLabel;
import java.awt.Font;
import javax.swing.JTextField;
import javax.swing.ButtonGroup;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class Fereastra extends JFrame {
private JPanel contentPane;
private JTextField textField;
private JTextField textField_1;
private final ButtonGroup buttonGroup = new ButtonGroup();
/**
* Launch the application.
*/
public static void main(String args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Fereastra frame = new Fereastra();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public Fereastra() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 751, 565);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
contentPane.setLayout(null);
setContentPane(contentPane);
JTextArea textArea = new JTextArea();
textArea.setBounds(12, 13, 447, 251);
contentPane.add(textArea);
JRadioButton rdbtnNewRadioButton = new JRadioButton("Cautarea pe nivel");
rdbtnNewRadioButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton);
rdbtnNewRadioButton.setBounds(488, 55, 185, 25);
contentPane.add(rdbtnNewRadioButton);
JRadioButton rdbtnNewRadioButton_1 = new JRadioButton("Cautarea in adancime");
rdbtnNewRadioButton_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
if(rdbtnNewRadioButton_1.isSelected())
{
textField.setVisible(true);
textField_1.setVisible(true);
}
else
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_1);
rdbtnNewRadioButton_1.setBounds(488, 96, 237, 25);
contentPane.add(rdbtnNewRadioButton_1);
JRadioButton rdbtnNewRadioButton_2 = new JRadioButton("Costul uniform");
rdbtnNewRadioButton_2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_2.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_2);
rdbtnNewRadioButton_2.setBounds(488, 138, 127, 25);
contentPane.add(rdbtnNewRadioButton_2);
JRadioButton rdbtnNewRadioButton_3 = new JRadioButton("Adancime iterativa");
rdbtnNewRadioButton_3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_3.isSelected())
{
textField_1.setVisible(true);
textField.setVisible(true);
}
else
{
textField_1.setVisible(false);
textField.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_3);
rdbtnNewRadioButton_3.setBounds(488, 179, 237, 25);
contentPane.add(rdbtnNewRadioButton_3);
JRadioButton rdbtnNewRadioButton_4 = new JRadioButton("Greedy");
rdbtnNewRadioButton_4.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(rdbtnNewRadioButton_4.isSelected())
{
textField.setVisible(false);
textField_1.setVisible(false);
}
}
});
buttonGroup.add(rdbtnNewRadioButton_4);
rdbtnNewRadioButton_4.setBounds(488, 221, 127, 25);
contentPane.add(rdbtnNewRadioButton_4);
JLabel lblNewLabel = new JLabel("Alegeti:");
lblNewLabel.setFont(new Font("Tahoma", Font.PLAIN, 16));
lblNewLabel.setBounds(488, 13, 84, 33);
contentPane.add(lblNewLabel);
textField = new JTextField();
textField.setBounds(402, 277, 116, 22);
contentPane.add(textField);
textField.setColumns(10);
textField.setVisible(false);
textField_1 = new JTextField();
textField_1.setBounds(246, 277, 116, 22);
contentPane.add(textField_1);
textField_1.setColumns(10);
textField_1.setVisible(false);
JLabel lblNewLabel_1 = new JLabel("Introduceti valorile dorite:");
lblNewLabel_1.setFont(new Font("Tahoma", Font.PLAIN, 16));
lblNewLabel_1.setBounds(22, 277, 212, 21);
contentPane.add(lblNewLabel_1);
lblNewLabel_1.setVisible(false);
}
}
eclipse jlabel
eclipse jlabel
New contributor
piglet is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
piglet is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
piglet is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Nov 10 at 14:36
piglet
33
33
New contributor
piglet is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
piglet is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
piglet is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
It's because your label is declared in function context, put the declaration next the textbox declaration and it should work fine
I did not manage to do that. I declared JLabel lblNewLabel_1 at the top of the declarations and I still get the error. Can you be more specific please? It would be very helpful. Thank you
– piglet
Nov 10 at 14:53
You have solved your problem?
– Vince
Nov 10 at 18:15
Yes, thank you very much
– piglet
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
It's because your label is declared in function context, put the declaration next the textbox declaration and it should work fine
I did not manage to do that. I declared JLabel lblNewLabel_1 at the top of the declarations and I still get the error. Can you be more specific please? It would be very helpful. Thank you
– piglet
Nov 10 at 14:53
You have solved your problem?
– Vince
Nov 10 at 18:15
Yes, thank you very much
– piglet
2 days ago
add a comment |
up vote
0
down vote
accepted
It's because your label is declared in function context, put the declaration next the textbox declaration and it should work fine
I did not manage to do that. I declared JLabel lblNewLabel_1 at the top of the declarations and I still get the error. Can you be more specific please? It would be very helpful. Thank you
– piglet
Nov 10 at 14:53
You have solved your problem?
– Vince
Nov 10 at 18:15
Yes, thank you very much
– piglet
2 days ago
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
It's because your label is declared in function context, put the declaration next the textbox declaration and it should work fine
It's because your label is declared in function context, put the declaration next the textbox declaration and it should work fine
edited Nov 10 at 14:47
answered Nov 10 at 14:44


Vince
362
362
I did not manage to do that. I declared JLabel lblNewLabel_1 at the top of the declarations and I still get the error. Can you be more specific please? It would be very helpful. Thank you
– piglet
Nov 10 at 14:53
You have solved your problem?
– Vince
Nov 10 at 18:15
Yes, thank you very much
– piglet
2 days ago
add a comment |
I did not manage to do that. I declared JLabel lblNewLabel_1 at the top of the declarations and I still get the error. Can you be more specific please? It would be very helpful. Thank you
– piglet
Nov 10 at 14:53
You have solved your problem?
– Vince
Nov 10 at 18:15
Yes, thank you very much
– piglet
2 days ago
I did not manage to do that. I declared JLabel lblNewLabel_1 at the top of the declarations and I still get the error. Can you be more specific please? It would be very helpful. Thank you
– piglet
Nov 10 at 14:53
I did not manage to do that. I declared JLabel lblNewLabel_1 at the top of the declarations and I still get the error. Can you be more specific please? It would be very helpful. Thank you
– piglet
Nov 10 at 14:53
You have solved your problem?
– Vince
Nov 10 at 18:15
You have solved your problem?
– Vince
Nov 10 at 18:15
Yes, thank you very much
– piglet
2 days ago
Yes, thank you very much
– piglet
2 days ago
add a comment |
piglet is a new contributor. Be nice, and check out our Code of Conduct.
piglet is a new contributor. Be nice, and check out our Code of Conduct.
piglet is a new contributor. Be nice, and check out our Code of Conduct.
piglet is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53239993%2fsetting-a-jlabel-visible-on-jradiobuttons-click%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
F8yQvhVP96