Android Canvas: Create RoundRectShape Object
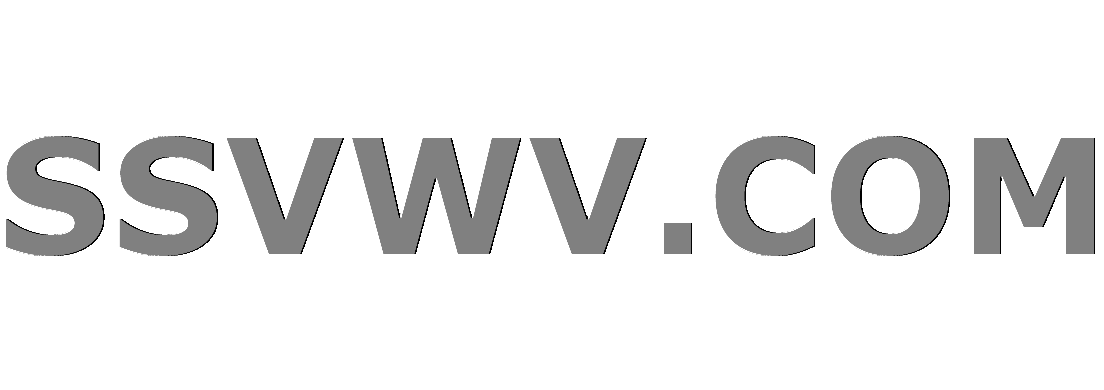
Multi tool use
up vote
0
down vote
favorite
I have created a class for a rectangle shape as it is an object in my application. However now I would like the corners to be rounded. Below you can see it's a bare bones class to create as many rectangles I want with the same attributes.
public class customDrawable extends ShapeDrawable {
public void setCustomDrawableAttributes(ShapeDrawable shapeDrawable){
int x = 0;
int y = 0;
int width = 100;
int height = 100;
shapeDrawable.setBounds(x, y-height, x + width,y+height );
}
public ShapeDrawable createShape(){
return new ShapeDrawable(new RectShape());
}
}
Update: Without this method I have nothing will be drawn as there is no size. With it it only draws the usual rectangle. (Integer values where changed to not show app specific methods)
public void setDrawableAttributes(ShapeDrawable shapeDrawable){
int x = 0;
int y = 500
int width = 200
int height = 300
shapeDrawable.setBounds(x, y-height, x + width,y+height );
}
From my research I found that I cannot simply add rounded corners but instead have to create a RoundRectShape shapeDrawable
. Every attempt I have made to create a rectangle with rounded corners using this RoundRectShape
has failed. Somehow the shape always ends up being a regular rectangle with no rounded corners.
I am looking for a just bare bones class (like the one provided) that creates a roundRectShape drawable. Height and width does not matter as long as it has rounded corners. Must be in Java and not XML.
Links I have tried for creating round rectangles:
1.https://developer.android.com/reference/android/graphics/drawable/shapes/RoundRectShape.html
2.http://alvinalexander.com/java/jwarehouse/android/graphics/java/android/graphics/drawable/shapes/RoundRectShape.java.shtml
3.https://www.codota.com/android/scenarios/52c5d269da0a37e1836d6e75/android.graphics.drawable.shapes.RoundRectShape?tag=coyote
4.http://developer.oesf.biz/em/developer/reference/durian/android/graphics/drawable/shapes/RoundRectShape.html
5.https://android.googlesource.com/platform/frameworks/base/+/donut-release/graphics/java/android/graphics/drawable/shapes/RoundRectShape.java
6.Android: RoundRectShape: Modify corner radii
7.http://www.programcreek.com/java-api-examples/index.php?api=android.graphics.drawable.shapes.RoundRectShape
8.http://www.edumobile.org/android/shape-drawing-example-in-android/
9.http://programtalk.com/java-api-usage-examples/android.graphics.drawable.shapes.RoundRectShape/
java

|
show 3 more comments
up vote
0
down vote
favorite
I have created a class for a rectangle shape as it is an object in my application. However now I would like the corners to be rounded. Below you can see it's a bare bones class to create as many rectangles I want with the same attributes.
public class customDrawable extends ShapeDrawable {
public void setCustomDrawableAttributes(ShapeDrawable shapeDrawable){
int x = 0;
int y = 0;
int width = 100;
int height = 100;
shapeDrawable.setBounds(x, y-height, x + width,y+height );
}
public ShapeDrawable createShape(){
return new ShapeDrawable(new RectShape());
}
}
Update: Without this method I have nothing will be drawn as there is no size. With it it only draws the usual rectangle. (Integer values where changed to not show app specific methods)
public void setDrawableAttributes(ShapeDrawable shapeDrawable){
int x = 0;
int y = 500
int width = 200
int height = 300
shapeDrawable.setBounds(x, y-height, x + width,y+height );
}
From my research I found that I cannot simply add rounded corners but instead have to create a RoundRectShape shapeDrawable
. Every attempt I have made to create a rectangle with rounded corners using this RoundRectShape
has failed. Somehow the shape always ends up being a regular rectangle with no rounded corners.
I am looking for a just bare bones class (like the one provided) that creates a roundRectShape drawable. Height and width does not matter as long as it has rounded corners. Must be in Java and not XML.
Links I have tried for creating round rectangles:
1.https://developer.android.com/reference/android/graphics/drawable/shapes/RoundRectShape.html
2.http://alvinalexander.com/java/jwarehouse/android/graphics/java/android/graphics/drawable/shapes/RoundRectShape.java.shtml
3.https://www.codota.com/android/scenarios/52c5d269da0a37e1836d6e75/android.graphics.drawable.shapes.RoundRectShape?tag=coyote
4.http://developer.oesf.biz/em/developer/reference/durian/android/graphics/drawable/shapes/RoundRectShape.html
5.https://android.googlesource.com/platform/frameworks/base/+/donut-release/graphics/java/android/graphics/drawable/shapes/RoundRectShape.java
6.Android: RoundRectShape: Modify corner radii
7.http://www.programcreek.com/java-api-examples/index.php?api=android.graphics.drawable.shapes.RoundRectShape
8.http://www.edumobile.org/android/shape-drawing-example-in-android/
9.http://programtalk.com/java-api-usage-examples/android.graphics.drawable.shapes.RoundRectShape/
java

using shape drawable from xml wont work in your case?
– Mohammed Atif
May 14 '17 at 5:02
Maybe I suppose since they will be the same every time. Can you give an example?
– L1ghtk3ira
May 14 '17 at 5:08
I think programmatic is probably still better tho. I am assuming more options. Why xml instead?
– L1ghtk3ira
May 14 '17 at 5:12
You can customize xml shapes very efficiently. And yes, it will keep your code cleaner too.
– Mohammed Atif
May 14 '17 at 5:43
I have tried that. Does not work for me.
– L1ghtk3ira
May 14 '17 at 7:42
|
show 3 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have created a class for a rectangle shape as it is an object in my application. However now I would like the corners to be rounded. Below you can see it's a bare bones class to create as many rectangles I want with the same attributes.
public class customDrawable extends ShapeDrawable {
public void setCustomDrawableAttributes(ShapeDrawable shapeDrawable){
int x = 0;
int y = 0;
int width = 100;
int height = 100;
shapeDrawable.setBounds(x, y-height, x + width,y+height );
}
public ShapeDrawable createShape(){
return new ShapeDrawable(new RectShape());
}
}
Update: Without this method I have nothing will be drawn as there is no size. With it it only draws the usual rectangle. (Integer values where changed to not show app specific methods)
public void setDrawableAttributes(ShapeDrawable shapeDrawable){
int x = 0;
int y = 500
int width = 200
int height = 300
shapeDrawable.setBounds(x, y-height, x + width,y+height );
}
From my research I found that I cannot simply add rounded corners but instead have to create a RoundRectShape shapeDrawable
. Every attempt I have made to create a rectangle with rounded corners using this RoundRectShape
has failed. Somehow the shape always ends up being a regular rectangle with no rounded corners.
I am looking for a just bare bones class (like the one provided) that creates a roundRectShape drawable. Height and width does not matter as long as it has rounded corners. Must be in Java and not XML.
Links I have tried for creating round rectangles:
1.https://developer.android.com/reference/android/graphics/drawable/shapes/RoundRectShape.html
2.http://alvinalexander.com/java/jwarehouse/android/graphics/java/android/graphics/drawable/shapes/RoundRectShape.java.shtml
3.https://www.codota.com/android/scenarios/52c5d269da0a37e1836d6e75/android.graphics.drawable.shapes.RoundRectShape?tag=coyote
4.http://developer.oesf.biz/em/developer/reference/durian/android/graphics/drawable/shapes/RoundRectShape.html
5.https://android.googlesource.com/platform/frameworks/base/+/donut-release/graphics/java/android/graphics/drawable/shapes/RoundRectShape.java
6.Android: RoundRectShape: Modify corner radii
7.http://www.programcreek.com/java-api-examples/index.php?api=android.graphics.drawable.shapes.RoundRectShape
8.http://www.edumobile.org/android/shape-drawing-example-in-android/
9.http://programtalk.com/java-api-usage-examples/android.graphics.drawable.shapes.RoundRectShape/
java

I have created a class for a rectangle shape as it is an object in my application. However now I would like the corners to be rounded. Below you can see it's a bare bones class to create as many rectangles I want with the same attributes.
public class customDrawable extends ShapeDrawable {
public void setCustomDrawableAttributes(ShapeDrawable shapeDrawable){
int x = 0;
int y = 0;
int width = 100;
int height = 100;
shapeDrawable.setBounds(x, y-height, x + width,y+height );
}
public ShapeDrawable createShape(){
return new ShapeDrawable(new RectShape());
}
}
Update: Without this method I have nothing will be drawn as there is no size. With it it only draws the usual rectangle. (Integer values where changed to not show app specific methods)
public void setDrawableAttributes(ShapeDrawable shapeDrawable){
int x = 0;
int y = 500
int width = 200
int height = 300
shapeDrawable.setBounds(x, y-height, x + width,y+height );
}
From my research I found that I cannot simply add rounded corners but instead have to create a RoundRectShape shapeDrawable
. Every attempt I have made to create a rectangle with rounded corners using this RoundRectShape
has failed. Somehow the shape always ends up being a regular rectangle with no rounded corners.
I am looking for a just bare bones class (like the one provided) that creates a roundRectShape drawable. Height and width does not matter as long as it has rounded corners. Must be in Java and not XML.
Links I have tried for creating round rectangles:
1.https://developer.android.com/reference/android/graphics/drawable/shapes/RoundRectShape.html
2.http://alvinalexander.com/java/jwarehouse/android/graphics/java/android/graphics/drawable/shapes/RoundRectShape.java.shtml
3.https://www.codota.com/android/scenarios/52c5d269da0a37e1836d6e75/android.graphics.drawable.shapes.RoundRectShape?tag=coyote
4.http://developer.oesf.biz/em/developer/reference/durian/android/graphics/drawable/shapes/RoundRectShape.html
5.https://android.googlesource.com/platform/frameworks/base/+/donut-release/graphics/java/android/graphics/drawable/shapes/RoundRectShape.java
6.Android: RoundRectShape: Modify corner radii
7.http://www.programcreek.com/java-api-examples/index.php?api=android.graphics.drawable.shapes.RoundRectShape
8.http://www.edumobile.org/android/shape-drawing-example-in-android/
9.http://programtalk.com/java-api-usage-examples/android.graphics.drawable.shapes.RoundRectShape/
java

java

edited Nov 10 at 14:21


halfer
14.1k757104
14.1k757104
asked May 14 '17 at 4:47
L1ghtk3ira
60521026
60521026
using shape drawable from xml wont work in your case?
– Mohammed Atif
May 14 '17 at 5:02
Maybe I suppose since they will be the same every time. Can you give an example?
– L1ghtk3ira
May 14 '17 at 5:08
I think programmatic is probably still better tho. I am assuming more options. Why xml instead?
– L1ghtk3ira
May 14 '17 at 5:12
You can customize xml shapes very efficiently. And yes, it will keep your code cleaner too.
– Mohammed Atif
May 14 '17 at 5:43
I have tried that. Does not work for me.
– L1ghtk3ira
May 14 '17 at 7:42
|
show 3 more comments
using shape drawable from xml wont work in your case?
– Mohammed Atif
May 14 '17 at 5:02
Maybe I suppose since they will be the same every time. Can you give an example?
– L1ghtk3ira
May 14 '17 at 5:08
I think programmatic is probably still better tho. I am assuming more options. Why xml instead?
– L1ghtk3ira
May 14 '17 at 5:12
You can customize xml shapes very efficiently. And yes, it will keep your code cleaner too.
– Mohammed Atif
May 14 '17 at 5:43
I have tried that. Does not work for me.
– L1ghtk3ira
May 14 '17 at 7:42
using shape drawable from xml wont work in your case?
– Mohammed Atif
May 14 '17 at 5:02
using shape drawable from xml wont work in your case?
– Mohammed Atif
May 14 '17 at 5:02
Maybe I suppose since they will be the same every time. Can you give an example?
– L1ghtk3ira
May 14 '17 at 5:08
Maybe I suppose since they will be the same every time. Can you give an example?
– L1ghtk3ira
May 14 '17 at 5:08
I think programmatic is probably still better tho. I am assuming more options. Why xml instead?
– L1ghtk3ira
May 14 '17 at 5:12
I think programmatic is probably still better tho. I am assuming more options. Why xml instead?
– L1ghtk3ira
May 14 '17 at 5:12
You can customize xml shapes very efficiently. And yes, it will keep your code cleaner too.
– Mohammed Atif
May 14 '17 at 5:43
You can customize xml shapes very efficiently. And yes, it will keep your code cleaner too.
– Mohammed Atif
May 14 '17 at 5:43
I have tried that. Does not work for me.
– L1ghtk3ira
May 14 '17 at 7:42
I have tried that. Does not work for me.
– L1ghtk3ira
May 14 '17 at 7:42
|
show 3 more comments
3 Answers
3
active
oldest
votes
up vote
4
down vote
accepted
I've created a class MyRect
that is used to draw rounded Rect for you.
public class MyRect {
public static Paint paint; // default paint use for all my MyRect objects
static {
paint=new Paint();
paint.setColor(Color.RED);
}
public float x,y,width,height;
public float roundStrength=30;
public MyRect(float x, float y, float width,float height){
this.x=x;
this.y=y;
this.width=width;
this.height=height;
}
public MyRect(float x, float y, float width,float height,float roundStrength){
this(x,y,width,height);
this.roundStrength=roundStrength;
}
public void draw(Canvas canvas){
canvas.drawRoundRect(x-width/2,y-height/2,x+width/2,y+height/2,roundStrength,roundStrength,paint);
}
}
Creating objects of above MyRect
is not enough, we need to keep references of objects in any container so that we can modify or delete that object in future.
static ArrayList<MyRect> myRects=new ArrayList<>();
Inside onDraw(Canvas canvas)
method of View
/SurfaceView
call MyRect's draw()
method.
for(MyRect rect:myRects)
rect.draw(canvas);
Done, Create object and add to container.
myRects.add(new MyRect(touchx,touchy,100,100));
or
myRects.add(new MyRect(touchx,touchy,100,100,50));
You can also extends MyRect
like add some more constructor, method and data member according to your requirement.
add a comment |
up vote
3
down vote
Custom Drawable
You can create custom drawable by extending drawable class
Steps to create Custom Drawable
1.Subclass Drawable and implement the following methods methods
public void draw(@NonNull Canvas canvas)
- You will get a canvas object to draw shapes.Call getBounds() method here to get the dimensions according view in which we applied the drawable.
public void setAlpha(@IntRange(from = 0, to = 255) int alpha)
- You will get a alpha integer value here,set it your main paint in which you are drawing the shape.
public void setColorFilter(@Nullable ColorFilter colorFilter)
- You will get ColorFilter object here,set it your main paint in which you are drawing the shape.
public int getOpacity()
- Return the opacity value here like PixelFormat.TRANSLUCENT,PixelFormat.TRANSPARENT,PixelFormat.RGB_888 etc.
2.In onDraw()
call canvas.drawRoundRect() method to draw the shape
public void drawRoundRect(@android.support.annotation.NonNull android.graphics.RectF rect,float rx,float ry,@android.support.annotation.NonNull android.graphics.Paint paint)
Draw the specified round-rect using the specified paint. The roundrect
will be filled or framed based on the Style in the paint.
Parameters:
1) rect - The rectangular bounds of the roundRect to be drawn
2) rx - The x-radius of the oval used to round the corners
3) ry - The y-radius of the oval used to round the corners
4) paint - The paint used to draw the roundRect
Code Sample
import android.graphics.Canvas;
import android.graphics.ColorFilter;
import android.graphics.Paint;
import android.graphics.PixelFormat;
import android.graphics.Rect;
import android.graphics.RectF;
import android.graphics.drawable.Drawable;
import android.support.annotation.IntRange;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
/**
* Created by jinesh on 24/5/17.
*/
public class RoundedRectangle extends Drawable {
private Paint rectPaint;
private RectF drawableBounds;
public RoundedRectangle(int rectBackground) {
rectPaint=new Paint(Paint.ANTI_ALIAS_FLAG);
rectPaint.setColor(rectBackground);
drawableBounds=new RectF();
}
@Override
public void draw(@NonNull Canvas canvas) {
Rect bounds=getBounds();
drawableBounds.set(bounds.left,bounds.top,bounds.right,bounds.bottom);
canvas.drawRoundRect(drawableBounds,10,10,rectPaint);
}
@Override
public void setAlpha(@IntRange(from = 0, to = 255) int alpha) {
rectPaint.setAlpha(alpha);
}
@Override
public void setColorFilter(@Nullable ColorFilter colorFilter) {
rectPaint.setColorFilter(colorFilter);
}
@Override
public int getOpacity() {
return PixelFormat.TRANSLUCENT;
}
}
Set to any view from activity
RoundedRectangle roundedRectangle=new RoundedRectangle(ContextCompat.getColor(this,R.color.colorAccent));
textView.setBackground(roundedRectangle);
Screenshot:
1
Excellent answer. I marked the other one as correct because it works better for my implementation however I will for sure use yours when I need to set backgrounds and that. Thanks! Hope you get a lot of votes.Great info, picture, very useful.
– L1ghtk3ira
May 26 '17 at 2:24
add a comment |
up vote
2
down vote
Why don't you use the drawRoundRect
function of the Canvas
class?
public class RoundRect{
int l,r,t,b,rx,ry;
Paint paint;
public RoundRect(int l,int r,int t,int b,int rx,int ry,Paint paint){
this.l=l;
this.r=r;
this.t=t;
this.b=b;
this.paint=paint;
}
public void draw(Canvas c,Paint paint){
c.drawRoundRect(l,t,r,b,rx,ry,paint);
}
}`
Because I have many objects. For better coding I should have my objects as classes. Not just canvas.drawSomething. The application will get bigger anyways which will make that kind of coding hard to manage.
– L1ghtk3ira
May 14 '17 at 5:07
Don't understand what you mean... It is possible to define a class for your rounded rectangle with it's parameters (position, size...) and usedrawRoundRect
when you need to draw it.
– Zelig63
May 14 '17 at 8:33
Can you provide a java class example? It is not good to draw directly onto the canvas viacanvas.drawRect
for example. It is better to create objects that you can control all parameters in methods and just create a new object every time.
– L1ghtk3ira
May 14 '17 at 8:35
You can try this : public class RoundRect{ int l,r,t,b,rx,ry; Paint paint; public RoundRect(int l,int r,int t,int b,int rx,int ry,Paint paint){ this.l=l; this.r=r; this.t=t; this.b=b; this.paint=paint; } public void draw(Canvas c,Paint paint){ c.drawRoundRect(l,t,r,b,rx,ry,paint); } }
– Zelig63
May 14 '17 at 13:12
Can you add this as an answer? And i will try it
– L1ghtk3ira
May 14 '17 at 18:16
|
show 1 more comment
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
4
down vote
accepted
I've created a class MyRect
that is used to draw rounded Rect for you.
public class MyRect {
public static Paint paint; // default paint use for all my MyRect objects
static {
paint=new Paint();
paint.setColor(Color.RED);
}
public float x,y,width,height;
public float roundStrength=30;
public MyRect(float x, float y, float width,float height){
this.x=x;
this.y=y;
this.width=width;
this.height=height;
}
public MyRect(float x, float y, float width,float height,float roundStrength){
this(x,y,width,height);
this.roundStrength=roundStrength;
}
public void draw(Canvas canvas){
canvas.drawRoundRect(x-width/2,y-height/2,x+width/2,y+height/2,roundStrength,roundStrength,paint);
}
}
Creating objects of above MyRect
is not enough, we need to keep references of objects in any container so that we can modify or delete that object in future.
static ArrayList<MyRect> myRects=new ArrayList<>();
Inside onDraw(Canvas canvas)
method of View
/SurfaceView
call MyRect's draw()
method.
for(MyRect rect:myRects)
rect.draw(canvas);
Done, Create object and add to container.
myRects.add(new MyRect(touchx,touchy,100,100));
or
myRects.add(new MyRect(touchx,touchy,100,100,50));
You can also extends MyRect
like add some more constructor, method and data member according to your requirement.
add a comment |
up vote
4
down vote
accepted
I've created a class MyRect
that is used to draw rounded Rect for you.
public class MyRect {
public static Paint paint; // default paint use for all my MyRect objects
static {
paint=new Paint();
paint.setColor(Color.RED);
}
public float x,y,width,height;
public float roundStrength=30;
public MyRect(float x, float y, float width,float height){
this.x=x;
this.y=y;
this.width=width;
this.height=height;
}
public MyRect(float x, float y, float width,float height,float roundStrength){
this(x,y,width,height);
this.roundStrength=roundStrength;
}
public void draw(Canvas canvas){
canvas.drawRoundRect(x-width/2,y-height/2,x+width/2,y+height/2,roundStrength,roundStrength,paint);
}
}
Creating objects of above MyRect
is not enough, we need to keep references of objects in any container so that we can modify or delete that object in future.
static ArrayList<MyRect> myRects=new ArrayList<>();
Inside onDraw(Canvas canvas)
method of View
/SurfaceView
call MyRect's draw()
method.
for(MyRect rect:myRects)
rect.draw(canvas);
Done, Create object and add to container.
myRects.add(new MyRect(touchx,touchy,100,100));
or
myRects.add(new MyRect(touchx,touchy,100,100,50));
You can also extends MyRect
like add some more constructor, method and data member according to your requirement.
add a comment |
up vote
4
down vote
accepted
up vote
4
down vote
accepted
I've created a class MyRect
that is used to draw rounded Rect for you.
public class MyRect {
public static Paint paint; // default paint use for all my MyRect objects
static {
paint=new Paint();
paint.setColor(Color.RED);
}
public float x,y,width,height;
public float roundStrength=30;
public MyRect(float x, float y, float width,float height){
this.x=x;
this.y=y;
this.width=width;
this.height=height;
}
public MyRect(float x, float y, float width,float height,float roundStrength){
this(x,y,width,height);
this.roundStrength=roundStrength;
}
public void draw(Canvas canvas){
canvas.drawRoundRect(x-width/2,y-height/2,x+width/2,y+height/2,roundStrength,roundStrength,paint);
}
}
Creating objects of above MyRect
is not enough, we need to keep references of objects in any container so that we can modify or delete that object in future.
static ArrayList<MyRect> myRects=new ArrayList<>();
Inside onDraw(Canvas canvas)
method of View
/SurfaceView
call MyRect's draw()
method.
for(MyRect rect:myRects)
rect.draw(canvas);
Done, Create object and add to container.
myRects.add(new MyRect(touchx,touchy,100,100));
or
myRects.add(new MyRect(touchx,touchy,100,100,50));
You can also extends MyRect
like add some more constructor, method and data member according to your requirement.
I've created a class MyRect
that is used to draw rounded Rect for you.
public class MyRect {
public static Paint paint; // default paint use for all my MyRect objects
static {
paint=new Paint();
paint.setColor(Color.RED);
}
public float x,y,width,height;
public float roundStrength=30;
public MyRect(float x, float y, float width,float height){
this.x=x;
this.y=y;
this.width=width;
this.height=height;
}
public MyRect(float x, float y, float width,float height,float roundStrength){
this(x,y,width,height);
this.roundStrength=roundStrength;
}
public void draw(Canvas canvas){
canvas.drawRoundRect(x-width/2,y-height/2,x+width/2,y+height/2,roundStrength,roundStrength,paint);
}
}
Creating objects of above MyRect
is not enough, we need to keep references of objects in any container so that we can modify or delete that object in future.
static ArrayList<MyRect> myRects=new ArrayList<>();
Inside onDraw(Canvas canvas)
method of View
/SurfaceView
call MyRect's draw()
method.
for(MyRect rect:myRects)
rect.draw(canvas);
Done, Create object and add to container.
myRects.add(new MyRect(touchx,touchy,100,100));
or
myRects.add(new MyRect(touchx,touchy,100,100,50));
You can also extends MyRect
like add some more constructor, method and data member according to your requirement.
answered May 21 '17 at 22:10


Aryan
14.7k73248
14.7k73248
add a comment |
add a comment |
up vote
3
down vote
Custom Drawable
You can create custom drawable by extending drawable class
Steps to create Custom Drawable
1.Subclass Drawable and implement the following methods methods
public void draw(@NonNull Canvas canvas)
- You will get a canvas object to draw shapes.Call getBounds() method here to get the dimensions according view in which we applied the drawable.
public void setAlpha(@IntRange(from = 0, to = 255) int alpha)
- You will get a alpha integer value here,set it your main paint in which you are drawing the shape.
public void setColorFilter(@Nullable ColorFilter colorFilter)
- You will get ColorFilter object here,set it your main paint in which you are drawing the shape.
public int getOpacity()
- Return the opacity value here like PixelFormat.TRANSLUCENT,PixelFormat.TRANSPARENT,PixelFormat.RGB_888 etc.
2.In onDraw()
call canvas.drawRoundRect() method to draw the shape
public void drawRoundRect(@android.support.annotation.NonNull android.graphics.RectF rect,float rx,float ry,@android.support.annotation.NonNull android.graphics.Paint paint)
Draw the specified round-rect using the specified paint. The roundrect
will be filled or framed based on the Style in the paint.
Parameters:
1) rect - The rectangular bounds of the roundRect to be drawn
2) rx - The x-radius of the oval used to round the corners
3) ry - The y-radius of the oval used to round the corners
4) paint - The paint used to draw the roundRect
Code Sample
import android.graphics.Canvas;
import android.graphics.ColorFilter;
import android.graphics.Paint;
import android.graphics.PixelFormat;
import android.graphics.Rect;
import android.graphics.RectF;
import android.graphics.drawable.Drawable;
import android.support.annotation.IntRange;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
/**
* Created by jinesh on 24/5/17.
*/
public class RoundedRectangle extends Drawable {
private Paint rectPaint;
private RectF drawableBounds;
public RoundedRectangle(int rectBackground) {
rectPaint=new Paint(Paint.ANTI_ALIAS_FLAG);
rectPaint.setColor(rectBackground);
drawableBounds=new RectF();
}
@Override
public void draw(@NonNull Canvas canvas) {
Rect bounds=getBounds();
drawableBounds.set(bounds.left,bounds.top,bounds.right,bounds.bottom);
canvas.drawRoundRect(drawableBounds,10,10,rectPaint);
}
@Override
public void setAlpha(@IntRange(from = 0, to = 255) int alpha) {
rectPaint.setAlpha(alpha);
}
@Override
public void setColorFilter(@Nullable ColorFilter colorFilter) {
rectPaint.setColorFilter(colorFilter);
}
@Override
public int getOpacity() {
return PixelFormat.TRANSLUCENT;
}
}
Set to any view from activity
RoundedRectangle roundedRectangle=new RoundedRectangle(ContextCompat.getColor(this,R.color.colorAccent));
textView.setBackground(roundedRectangle);
Screenshot:
1
Excellent answer. I marked the other one as correct because it works better for my implementation however I will for sure use yours when I need to set backgrounds and that. Thanks! Hope you get a lot of votes.Great info, picture, very useful.
– L1ghtk3ira
May 26 '17 at 2:24
add a comment |
up vote
3
down vote
Custom Drawable
You can create custom drawable by extending drawable class
Steps to create Custom Drawable
1.Subclass Drawable and implement the following methods methods
public void draw(@NonNull Canvas canvas)
- You will get a canvas object to draw shapes.Call getBounds() method here to get the dimensions according view in which we applied the drawable.
public void setAlpha(@IntRange(from = 0, to = 255) int alpha)
- You will get a alpha integer value here,set it your main paint in which you are drawing the shape.
public void setColorFilter(@Nullable ColorFilter colorFilter)
- You will get ColorFilter object here,set it your main paint in which you are drawing the shape.
public int getOpacity()
- Return the opacity value here like PixelFormat.TRANSLUCENT,PixelFormat.TRANSPARENT,PixelFormat.RGB_888 etc.
2.In onDraw()
call canvas.drawRoundRect() method to draw the shape
public void drawRoundRect(@android.support.annotation.NonNull android.graphics.RectF rect,float rx,float ry,@android.support.annotation.NonNull android.graphics.Paint paint)
Draw the specified round-rect using the specified paint. The roundrect
will be filled or framed based on the Style in the paint.
Parameters:
1) rect - The rectangular bounds of the roundRect to be drawn
2) rx - The x-radius of the oval used to round the corners
3) ry - The y-radius of the oval used to round the corners
4) paint - The paint used to draw the roundRect
Code Sample
import android.graphics.Canvas;
import android.graphics.ColorFilter;
import android.graphics.Paint;
import android.graphics.PixelFormat;
import android.graphics.Rect;
import android.graphics.RectF;
import android.graphics.drawable.Drawable;
import android.support.annotation.IntRange;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
/**
* Created by jinesh on 24/5/17.
*/
public class RoundedRectangle extends Drawable {
private Paint rectPaint;
private RectF drawableBounds;
public RoundedRectangle(int rectBackground) {
rectPaint=new Paint(Paint.ANTI_ALIAS_FLAG);
rectPaint.setColor(rectBackground);
drawableBounds=new RectF();
}
@Override
public void draw(@NonNull Canvas canvas) {
Rect bounds=getBounds();
drawableBounds.set(bounds.left,bounds.top,bounds.right,bounds.bottom);
canvas.drawRoundRect(drawableBounds,10,10,rectPaint);
}
@Override
public void setAlpha(@IntRange(from = 0, to = 255) int alpha) {
rectPaint.setAlpha(alpha);
}
@Override
public void setColorFilter(@Nullable ColorFilter colorFilter) {
rectPaint.setColorFilter(colorFilter);
}
@Override
public int getOpacity() {
return PixelFormat.TRANSLUCENT;
}
}
Set to any view from activity
RoundedRectangle roundedRectangle=new RoundedRectangle(ContextCompat.getColor(this,R.color.colorAccent));
textView.setBackground(roundedRectangle);
Screenshot:
1
Excellent answer. I marked the other one as correct because it works better for my implementation however I will for sure use yours when I need to set backgrounds and that. Thanks! Hope you get a lot of votes.Great info, picture, very useful.
– L1ghtk3ira
May 26 '17 at 2:24
add a comment |
up vote
3
down vote
up vote
3
down vote
Custom Drawable
You can create custom drawable by extending drawable class
Steps to create Custom Drawable
1.Subclass Drawable and implement the following methods methods
public void draw(@NonNull Canvas canvas)
- You will get a canvas object to draw shapes.Call getBounds() method here to get the dimensions according view in which we applied the drawable.
public void setAlpha(@IntRange(from = 0, to = 255) int alpha)
- You will get a alpha integer value here,set it your main paint in which you are drawing the shape.
public void setColorFilter(@Nullable ColorFilter colorFilter)
- You will get ColorFilter object here,set it your main paint in which you are drawing the shape.
public int getOpacity()
- Return the opacity value here like PixelFormat.TRANSLUCENT,PixelFormat.TRANSPARENT,PixelFormat.RGB_888 etc.
2.In onDraw()
call canvas.drawRoundRect() method to draw the shape
public void drawRoundRect(@android.support.annotation.NonNull android.graphics.RectF rect,float rx,float ry,@android.support.annotation.NonNull android.graphics.Paint paint)
Draw the specified round-rect using the specified paint. The roundrect
will be filled or framed based on the Style in the paint.
Parameters:
1) rect - The rectangular bounds of the roundRect to be drawn
2) rx - The x-radius of the oval used to round the corners
3) ry - The y-radius of the oval used to round the corners
4) paint - The paint used to draw the roundRect
Code Sample
import android.graphics.Canvas;
import android.graphics.ColorFilter;
import android.graphics.Paint;
import android.graphics.PixelFormat;
import android.graphics.Rect;
import android.graphics.RectF;
import android.graphics.drawable.Drawable;
import android.support.annotation.IntRange;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
/**
* Created by jinesh on 24/5/17.
*/
public class RoundedRectangle extends Drawable {
private Paint rectPaint;
private RectF drawableBounds;
public RoundedRectangle(int rectBackground) {
rectPaint=new Paint(Paint.ANTI_ALIAS_FLAG);
rectPaint.setColor(rectBackground);
drawableBounds=new RectF();
}
@Override
public void draw(@NonNull Canvas canvas) {
Rect bounds=getBounds();
drawableBounds.set(bounds.left,bounds.top,bounds.right,bounds.bottom);
canvas.drawRoundRect(drawableBounds,10,10,rectPaint);
}
@Override
public void setAlpha(@IntRange(from = 0, to = 255) int alpha) {
rectPaint.setAlpha(alpha);
}
@Override
public void setColorFilter(@Nullable ColorFilter colorFilter) {
rectPaint.setColorFilter(colorFilter);
}
@Override
public int getOpacity() {
return PixelFormat.TRANSLUCENT;
}
}
Set to any view from activity
RoundedRectangle roundedRectangle=new RoundedRectangle(ContextCompat.getColor(this,R.color.colorAccent));
textView.setBackground(roundedRectangle);
Screenshot:
Custom Drawable
You can create custom drawable by extending drawable class
Steps to create Custom Drawable
1.Subclass Drawable and implement the following methods methods
public void draw(@NonNull Canvas canvas)
- You will get a canvas object to draw shapes.Call getBounds() method here to get the dimensions according view in which we applied the drawable.
public void setAlpha(@IntRange(from = 0, to = 255) int alpha)
- You will get a alpha integer value here,set it your main paint in which you are drawing the shape.
public void setColorFilter(@Nullable ColorFilter colorFilter)
- You will get ColorFilter object here,set it your main paint in which you are drawing the shape.
public int getOpacity()
- Return the opacity value here like PixelFormat.TRANSLUCENT,PixelFormat.TRANSPARENT,PixelFormat.RGB_888 etc.
2.In onDraw()
call canvas.drawRoundRect() method to draw the shape
public void drawRoundRect(@android.support.annotation.NonNull android.graphics.RectF rect,float rx,float ry,@android.support.annotation.NonNull android.graphics.Paint paint)
Draw the specified round-rect using the specified paint. The roundrect
will be filled or framed based on the Style in the paint.
Parameters:
1) rect - The rectangular bounds of the roundRect to be drawn
2) rx - The x-radius of the oval used to round the corners
3) ry - The y-radius of the oval used to round the corners
4) paint - The paint used to draw the roundRect
Code Sample
import android.graphics.Canvas;
import android.graphics.ColorFilter;
import android.graphics.Paint;
import android.graphics.PixelFormat;
import android.graphics.Rect;
import android.graphics.RectF;
import android.graphics.drawable.Drawable;
import android.support.annotation.IntRange;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
/**
* Created by jinesh on 24/5/17.
*/
public class RoundedRectangle extends Drawable {
private Paint rectPaint;
private RectF drawableBounds;
public RoundedRectangle(int rectBackground) {
rectPaint=new Paint(Paint.ANTI_ALIAS_FLAG);
rectPaint.setColor(rectBackground);
drawableBounds=new RectF();
}
@Override
public void draw(@NonNull Canvas canvas) {
Rect bounds=getBounds();
drawableBounds.set(bounds.left,bounds.top,bounds.right,bounds.bottom);
canvas.drawRoundRect(drawableBounds,10,10,rectPaint);
}
@Override
public void setAlpha(@IntRange(from = 0, to = 255) int alpha) {
rectPaint.setAlpha(alpha);
}
@Override
public void setColorFilter(@Nullable ColorFilter colorFilter) {
rectPaint.setColorFilter(colorFilter);
}
@Override
public int getOpacity() {
return PixelFormat.TRANSLUCENT;
}
}
Set to any view from activity
RoundedRectangle roundedRectangle=new RoundedRectangle(ContextCompat.getColor(this,R.color.colorAccent));
textView.setBackground(roundedRectangle);
Screenshot:
answered May 24 '17 at 13:04


Jinesh Francis
1,2821926
1,2821926
1
Excellent answer. I marked the other one as correct because it works better for my implementation however I will for sure use yours when I need to set backgrounds and that. Thanks! Hope you get a lot of votes.Great info, picture, very useful.
– L1ghtk3ira
May 26 '17 at 2:24
add a comment |
1
Excellent answer. I marked the other one as correct because it works better for my implementation however I will for sure use yours when I need to set backgrounds and that. Thanks! Hope you get a lot of votes.Great info, picture, very useful.
– L1ghtk3ira
May 26 '17 at 2:24
1
1
Excellent answer. I marked the other one as correct because it works better for my implementation however I will for sure use yours when I need to set backgrounds and that. Thanks! Hope you get a lot of votes.Great info, picture, very useful.
– L1ghtk3ira
May 26 '17 at 2:24
Excellent answer. I marked the other one as correct because it works better for my implementation however I will for sure use yours when I need to set backgrounds and that. Thanks! Hope you get a lot of votes.Great info, picture, very useful.
– L1ghtk3ira
May 26 '17 at 2:24
add a comment |
up vote
2
down vote
Why don't you use the drawRoundRect
function of the Canvas
class?
public class RoundRect{
int l,r,t,b,rx,ry;
Paint paint;
public RoundRect(int l,int r,int t,int b,int rx,int ry,Paint paint){
this.l=l;
this.r=r;
this.t=t;
this.b=b;
this.paint=paint;
}
public void draw(Canvas c,Paint paint){
c.drawRoundRect(l,t,r,b,rx,ry,paint);
}
}`
Because I have many objects. For better coding I should have my objects as classes. Not just canvas.drawSomething. The application will get bigger anyways which will make that kind of coding hard to manage.
– L1ghtk3ira
May 14 '17 at 5:07
Don't understand what you mean... It is possible to define a class for your rounded rectangle with it's parameters (position, size...) and usedrawRoundRect
when you need to draw it.
– Zelig63
May 14 '17 at 8:33
Can you provide a java class example? It is not good to draw directly onto the canvas viacanvas.drawRect
for example. It is better to create objects that you can control all parameters in methods and just create a new object every time.
– L1ghtk3ira
May 14 '17 at 8:35
You can try this : public class RoundRect{ int l,r,t,b,rx,ry; Paint paint; public RoundRect(int l,int r,int t,int b,int rx,int ry,Paint paint){ this.l=l; this.r=r; this.t=t; this.b=b; this.paint=paint; } public void draw(Canvas c,Paint paint){ c.drawRoundRect(l,t,r,b,rx,ry,paint); } }
– Zelig63
May 14 '17 at 13:12
Can you add this as an answer? And i will try it
– L1ghtk3ira
May 14 '17 at 18:16
|
show 1 more comment
up vote
2
down vote
Why don't you use the drawRoundRect
function of the Canvas
class?
public class RoundRect{
int l,r,t,b,rx,ry;
Paint paint;
public RoundRect(int l,int r,int t,int b,int rx,int ry,Paint paint){
this.l=l;
this.r=r;
this.t=t;
this.b=b;
this.paint=paint;
}
public void draw(Canvas c,Paint paint){
c.drawRoundRect(l,t,r,b,rx,ry,paint);
}
}`
Because I have many objects. For better coding I should have my objects as classes. Not just canvas.drawSomething. The application will get bigger anyways which will make that kind of coding hard to manage.
– L1ghtk3ira
May 14 '17 at 5:07
Don't understand what you mean... It is possible to define a class for your rounded rectangle with it's parameters (position, size...) and usedrawRoundRect
when you need to draw it.
– Zelig63
May 14 '17 at 8:33
Can you provide a java class example? It is not good to draw directly onto the canvas viacanvas.drawRect
for example. It is better to create objects that you can control all parameters in methods and just create a new object every time.
– L1ghtk3ira
May 14 '17 at 8:35
You can try this : public class RoundRect{ int l,r,t,b,rx,ry; Paint paint; public RoundRect(int l,int r,int t,int b,int rx,int ry,Paint paint){ this.l=l; this.r=r; this.t=t; this.b=b; this.paint=paint; } public void draw(Canvas c,Paint paint){ c.drawRoundRect(l,t,r,b,rx,ry,paint); } }
– Zelig63
May 14 '17 at 13:12
Can you add this as an answer? And i will try it
– L1ghtk3ira
May 14 '17 at 18:16
|
show 1 more comment
up vote
2
down vote
up vote
2
down vote
Why don't you use the drawRoundRect
function of the Canvas
class?
public class RoundRect{
int l,r,t,b,rx,ry;
Paint paint;
public RoundRect(int l,int r,int t,int b,int rx,int ry,Paint paint){
this.l=l;
this.r=r;
this.t=t;
this.b=b;
this.paint=paint;
}
public void draw(Canvas c,Paint paint){
c.drawRoundRect(l,t,r,b,rx,ry,paint);
}
}`
Why don't you use the drawRoundRect
function of the Canvas
class?
public class RoundRect{
int l,r,t,b,rx,ry;
Paint paint;
public RoundRect(int l,int r,int t,int b,int rx,int ry,Paint paint){
this.l=l;
this.r=r;
this.t=t;
this.b=b;
this.paint=paint;
}
public void draw(Canvas c,Paint paint){
c.drawRoundRect(l,t,r,b,rx,ry,paint);
}
}`
edited May 15 '17 at 16:22
answered May 14 '17 at 4:51
Zelig63
52311021
52311021
Because I have many objects. For better coding I should have my objects as classes. Not just canvas.drawSomething. The application will get bigger anyways which will make that kind of coding hard to manage.
– L1ghtk3ira
May 14 '17 at 5:07
Don't understand what you mean... It is possible to define a class for your rounded rectangle with it's parameters (position, size...) and usedrawRoundRect
when you need to draw it.
– Zelig63
May 14 '17 at 8:33
Can you provide a java class example? It is not good to draw directly onto the canvas viacanvas.drawRect
for example. It is better to create objects that you can control all parameters in methods and just create a new object every time.
– L1ghtk3ira
May 14 '17 at 8:35
You can try this : public class RoundRect{ int l,r,t,b,rx,ry; Paint paint; public RoundRect(int l,int r,int t,int b,int rx,int ry,Paint paint){ this.l=l; this.r=r; this.t=t; this.b=b; this.paint=paint; } public void draw(Canvas c,Paint paint){ c.drawRoundRect(l,t,r,b,rx,ry,paint); } }
– Zelig63
May 14 '17 at 13:12
Can you add this as an answer? And i will try it
– L1ghtk3ira
May 14 '17 at 18:16
|
show 1 more comment
Because I have many objects. For better coding I should have my objects as classes. Not just canvas.drawSomething. The application will get bigger anyways which will make that kind of coding hard to manage.
– L1ghtk3ira
May 14 '17 at 5:07
Don't understand what you mean... It is possible to define a class for your rounded rectangle with it's parameters (position, size...) and usedrawRoundRect
when you need to draw it.
– Zelig63
May 14 '17 at 8:33
Can you provide a java class example? It is not good to draw directly onto the canvas viacanvas.drawRect
for example. It is better to create objects that you can control all parameters in methods and just create a new object every time.
– L1ghtk3ira
May 14 '17 at 8:35
You can try this : public class RoundRect{ int l,r,t,b,rx,ry; Paint paint; public RoundRect(int l,int r,int t,int b,int rx,int ry,Paint paint){ this.l=l; this.r=r; this.t=t; this.b=b; this.paint=paint; } public void draw(Canvas c,Paint paint){ c.drawRoundRect(l,t,r,b,rx,ry,paint); } }
– Zelig63
May 14 '17 at 13:12
Can you add this as an answer? And i will try it
– L1ghtk3ira
May 14 '17 at 18:16
Because I have many objects. For better coding I should have my objects as classes. Not just canvas.drawSomething. The application will get bigger anyways which will make that kind of coding hard to manage.
– L1ghtk3ira
May 14 '17 at 5:07
Because I have many objects. For better coding I should have my objects as classes. Not just canvas.drawSomething. The application will get bigger anyways which will make that kind of coding hard to manage.
– L1ghtk3ira
May 14 '17 at 5:07
Don't understand what you mean... It is possible to define a class for your rounded rectangle with it's parameters (position, size...) and use
drawRoundRect
when you need to draw it.– Zelig63
May 14 '17 at 8:33
Don't understand what you mean... It is possible to define a class for your rounded rectangle with it's parameters (position, size...) and use
drawRoundRect
when you need to draw it.– Zelig63
May 14 '17 at 8:33
Can you provide a java class example? It is not good to draw directly onto the canvas via
canvas.drawRect
for example. It is better to create objects that you can control all parameters in methods and just create a new object every time.– L1ghtk3ira
May 14 '17 at 8:35
Can you provide a java class example? It is not good to draw directly onto the canvas via
canvas.drawRect
for example. It is better to create objects that you can control all parameters in methods and just create a new object every time.– L1ghtk3ira
May 14 '17 at 8:35
You can try this : public class RoundRect{ int l,r,t,b,rx,ry; Paint paint; public RoundRect(int l,int r,int t,int b,int rx,int ry,Paint paint){ this.l=l; this.r=r; this.t=t; this.b=b; this.paint=paint; } public void draw(Canvas c,Paint paint){ c.drawRoundRect(l,t,r,b,rx,ry,paint); } }
– Zelig63
May 14 '17 at 13:12
You can try this : public class RoundRect{ int l,r,t,b,rx,ry; Paint paint; public RoundRect(int l,int r,int t,int b,int rx,int ry,Paint paint){ this.l=l; this.r=r; this.t=t; this.b=b; this.paint=paint; } public void draw(Canvas c,Paint paint){ c.drawRoundRect(l,t,r,b,rx,ry,paint); } }
– Zelig63
May 14 '17 at 13:12
Can you add this as an answer? And i will try it
– L1ghtk3ira
May 14 '17 at 18:16
Can you add this as an answer? And i will try it
– L1ghtk3ira
May 14 '17 at 18:16
|
show 1 more comment
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f43960477%2fandroid-canvas-create-roundrectshape-object%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
JDi5i O7tCX6,fGBi2,6K65,QrqwtjndkQiN9no6O30UVp 5hsCP4VjDCvtboEzyTQCyr41ZbCmM6UH QW,mB
using shape drawable from xml wont work in your case?
– Mohammed Atif
May 14 '17 at 5:02
Maybe I suppose since they will be the same every time. Can you give an example?
– L1ghtk3ira
May 14 '17 at 5:08
I think programmatic is probably still better tho. I am assuming more options. Why xml instead?
– L1ghtk3ira
May 14 '17 at 5:12
You can customize xml shapes very efficiently. And yes, it will keep your code cleaner too.
– Mohammed Atif
May 14 '17 at 5:43
I have tried that. Does not work for me.
– L1ghtk3ira
May 14 '17 at 7:42