How to deal with nulls in GraphQL schema
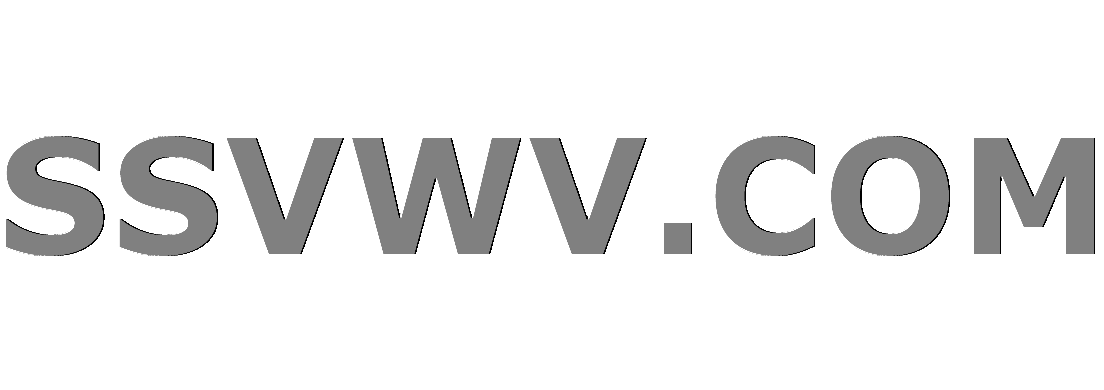
Multi tool use
up vote
2
down vote
favorite
I keep getting the "Cannot return null for non-nullable field Airline.id." error when FlightSchedule.operatingAirline is null (perfectly valid as per schema) and client queries for FlightSchedule.operatingAirline.id. How to fix this? Making Airline.id, Airline.code and Airline.name as nullable fixes this but is not the right way to solve this problem because if an Airline exist, these 3 fields will always exist too. Below is my schema:
type Airline {
id: String!,
code: String!,
name: String!
}
type FlightSchedule {
airline: Airline!
operatingAirline: Airline
}
And below is my query:
getFlightSchedules {
airline
{
id
code
name
}
operatingAirline
{
id
code
name
}
}
graphql apollo-server
add a comment |
up vote
2
down vote
favorite
I keep getting the "Cannot return null for non-nullable field Airline.id." error when FlightSchedule.operatingAirline is null (perfectly valid as per schema) and client queries for FlightSchedule.operatingAirline.id. How to fix this? Making Airline.id, Airline.code and Airline.name as nullable fixes this but is not the right way to solve this problem because if an Airline exist, these 3 fields will always exist too. Below is my schema:
type Airline {
id: String!,
code: String!,
name: String!
}
type FlightSchedule {
airline: Airline!
operatingAirline: Airline
}
And below is my query:
getFlightSchedules {
airline
{
id
code
name
}
operatingAirline
{
id
code
name
}
}
graphql apollo-server
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I keep getting the "Cannot return null for non-nullable field Airline.id." error when FlightSchedule.operatingAirline is null (perfectly valid as per schema) and client queries for FlightSchedule.operatingAirline.id. How to fix this? Making Airline.id, Airline.code and Airline.name as nullable fixes this but is not the right way to solve this problem because if an Airline exist, these 3 fields will always exist too. Below is my schema:
type Airline {
id: String!,
code: String!,
name: String!
}
type FlightSchedule {
airline: Airline!
operatingAirline: Airline
}
And below is my query:
getFlightSchedules {
airline
{
id
code
name
}
operatingAirline
{
id
code
name
}
}
graphql apollo-server
I keep getting the "Cannot return null for non-nullable field Airline.id." error when FlightSchedule.operatingAirline is null (perfectly valid as per schema) and client queries for FlightSchedule.operatingAirline.id. How to fix this? Making Airline.id, Airline.code and Airline.name as nullable fixes this but is not the right way to solve this problem because if an Airline exist, these 3 fields will always exist too. Below is my schema:
type Airline {
id: String!,
code: String!,
name: String!
}
type FlightSchedule {
airline: Airline!
operatingAirline: Airline
}
And below is my query:
getFlightSchedules {
airline
{
id
code
name
}
operatingAirline
{
id
code
name
}
}
graphql apollo-server
graphql apollo-server
asked Nov 10 at 14:35
Raj Chaudhary
8619
8619
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
A field will resolve to null when an error is encountered while resolving it. This includes validation errors like the one you're encountering. From the spec:
If during ExecuteSelectionSet() a field with a non‐null fieldType throws a field error then that error must propagate to this entire selection set, either resolving to null if allowed or further propagated to a parent field.
If this occurs, any sibling fields which have not yet executed or have not yet yielded a value may be cancelled to avoid unnecessary work.
In other words, if a parent field is of a particular object type, and that type has a non-nullable field, and that field resolves to null, that parent field will also resolve to null. The parent field cannot return an object that is invalid (in this case because it had a non-null field return null), so the only thing it can do is return null. Of course, if the parent field itself is non-null, this behavior is propagated up the tree until a nullable field is finally encountered.
So, why are you getting that error? Because your resolver for operatingAirline
is not returning null. It is returning some kind of object (either an incomplete airline object, an array, a string or something else) that GraphQL then effectively tries to coerce into the Airline
type. The id field was requested, but it resolves to null based on the object returned by operatingAirline
's resolver. Since the id
was requested and returned null, the entire operatingAirline
field fails validation and returns null.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
A field will resolve to null when an error is encountered while resolving it. This includes validation errors like the one you're encountering. From the spec:
If during ExecuteSelectionSet() a field with a non‐null fieldType throws a field error then that error must propagate to this entire selection set, either resolving to null if allowed or further propagated to a parent field.
If this occurs, any sibling fields which have not yet executed or have not yet yielded a value may be cancelled to avoid unnecessary work.
In other words, if a parent field is of a particular object type, and that type has a non-nullable field, and that field resolves to null, that parent field will also resolve to null. The parent field cannot return an object that is invalid (in this case because it had a non-null field return null), so the only thing it can do is return null. Of course, if the parent field itself is non-null, this behavior is propagated up the tree until a nullable field is finally encountered.
So, why are you getting that error? Because your resolver for operatingAirline
is not returning null. It is returning some kind of object (either an incomplete airline object, an array, a string or something else) that GraphQL then effectively tries to coerce into the Airline
type. The id field was requested, but it resolves to null based on the object returned by operatingAirline
's resolver. Since the id
was requested and returned null, the entire operatingAirline
field fails validation and returns null.
add a comment |
up vote
1
down vote
A field will resolve to null when an error is encountered while resolving it. This includes validation errors like the one you're encountering. From the spec:
If during ExecuteSelectionSet() a field with a non‐null fieldType throws a field error then that error must propagate to this entire selection set, either resolving to null if allowed or further propagated to a parent field.
If this occurs, any sibling fields which have not yet executed or have not yet yielded a value may be cancelled to avoid unnecessary work.
In other words, if a parent field is of a particular object type, and that type has a non-nullable field, and that field resolves to null, that parent field will also resolve to null. The parent field cannot return an object that is invalid (in this case because it had a non-null field return null), so the only thing it can do is return null. Of course, if the parent field itself is non-null, this behavior is propagated up the tree until a nullable field is finally encountered.
So, why are you getting that error? Because your resolver for operatingAirline
is not returning null. It is returning some kind of object (either an incomplete airline object, an array, a string or something else) that GraphQL then effectively tries to coerce into the Airline
type. The id field was requested, but it resolves to null based on the object returned by operatingAirline
's resolver. Since the id
was requested and returned null, the entire operatingAirline
field fails validation and returns null.
add a comment |
up vote
1
down vote
up vote
1
down vote
A field will resolve to null when an error is encountered while resolving it. This includes validation errors like the one you're encountering. From the spec:
If during ExecuteSelectionSet() a field with a non‐null fieldType throws a field error then that error must propagate to this entire selection set, either resolving to null if allowed or further propagated to a parent field.
If this occurs, any sibling fields which have not yet executed or have not yet yielded a value may be cancelled to avoid unnecessary work.
In other words, if a parent field is of a particular object type, and that type has a non-nullable field, and that field resolves to null, that parent field will also resolve to null. The parent field cannot return an object that is invalid (in this case because it had a non-null field return null), so the only thing it can do is return null. Of course, if the parent field itself is non-null, this behavior is propagated up the tree until a nullable field is finally encountered.
So, why are you getting that error? Because your resolver for operatingAirline
is not returning null. It is returning some kind of object (either an incomplete airline object, an array, a string or something else) that GraphQL then effectively tries to coerce into the Airline
type. The id field was requested, but it resolves to null based on the object returned by operatingAirline
's resolver. Since the id
was requested and returned null, the entire operatingAirline
field fails validation and returns null.
A field will resolve to null when an error is encountered while resolving it. This includes validation errors like the one you're encountering. From the spec:
If during ExecuteSelectionSet() a field with a non‐null fieldType throws a field error then that error must propagate to this entire selection set, either resolving to null if allowed or further propagated to a parent field.
If this occurs, any sibling fields which have not yet executed or have not yet yielded a value may be cancelled to avoid unnecessary work.
In other words, if a parent field is of a particular object type, and that type has a non-nullable field, and that field resolves to null, that parent field will also resolve to null. The parent field cannot return an object that is invalid (in this case because it had a non-null field return null), so the only thing it can do is return null. Of course, if the parent field itself is non-null, this behavior is propagated up the tree until a nullable field is finally encountered.
So, why are you getting that error? Because your resolver for operatingAirline
is not returning null. It is returning some kind of object (either an incomplete airline object, an array, a string or something else) that GraphQL then effectively tries to coerce into the Airline
type. The id field was requested, but it resolves to null based on the object returned by operatingAirline
's resolver. Since the id
was requested and returned null, the entire operatingAirline
field fails validation and returns null.
answered Nov 10 at 16:16


Daniel Rearden
11.1k1934
11.1k1934
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53239983%2fhow-to-deal-with-nulls-in-graphql-schema%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
09LVKcbVtmojozHPuL8rOA,yIksrLYtffjedKX63f 3hh2AzUVmVAUlAb7BUSq,CYRIAQFs3QP1KsYjfN 5,wSjc2P6cb