LibGDX, GDX.files.internal() File not found
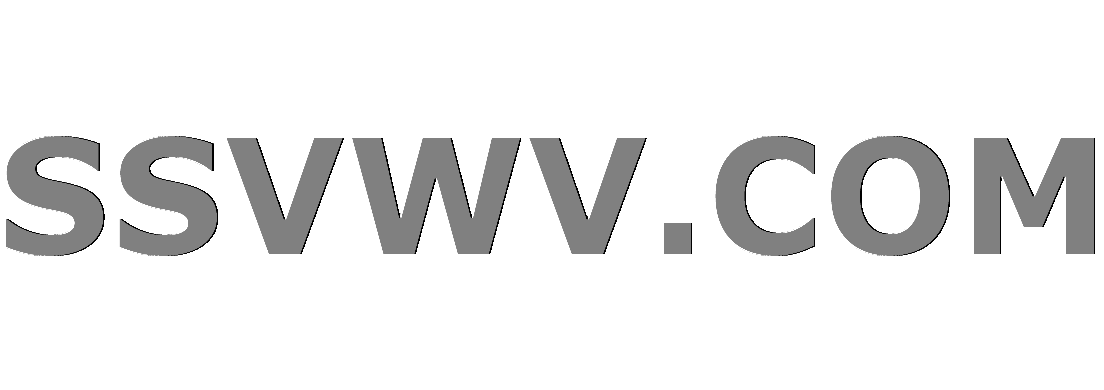
Multi tool use
up vote
0
down vote
favorite
I have an issue using LibGDX with assets directory. I actually build my project folder this way. I follow this tutorial to learn. (I work on Eclipse)
The code I use is :
package com.mygdx.game;
import com.badlogic.gdx.ApplicationListener;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.g2d.Sprite;
import com.badlogic.gdx.graphics.g2d.SpriteBatch;
import com.badlogic.gdx.graphics.g2d.TextureAtlas;
import com.badlogic.gdx.graphics.g2d.TextureAtlas.AtlasRegion;
import com.badlogic.gdx.utils.Timer;
import com.badlogic.gdx.utils.Timer.Task;
public class MyGdxGame implements ApplicationListener {
private SpriteBatch batch;
private TextureAtlas textureAtlas;
private Sprite sprite;
private int currentFrame = 1;
private String currentAtlasKey = new String("0001");
@Override
public void create() {
batch = new SpriteBatch();
// THE PROBLEM IS UNDER THIS LINE
textureAtlas = new TextureAtlas(Gdx.files.internal("spritesheet.atlas"));
AtlasRegion region = textureAtlas.findRegion("0001");
sprite = new Sprite(region);
sprite.setPosition(120, 100);
sprite.scale(2.5f);
Timer.schedule(new Task(){
@Override
public void run() {
currentFrame++;
if(currentFrame > 20)
currentFrame = 1;
// ATTENTION! String.format() doesnt work under GWT for god knows why...
currentAtlasKey = String.format("%04d", currentFrame);
sprite.setRegion(textureAtlas.findRegion(currentAtlasKey));
}
}
,0,1/30.0f);
}
@Override
public void dispose() {
batch.dispose();
textureAtlas.dispose();
}
@Override
public void render() {
Gdx.gl.glClearColor(0, 0, 0, 1);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
batch.begin();
sprite.draw(batch);
batch.end();
}
@Override
public void resize(int width, int height) {
}
@Override
public void pause() {
}
@Override
public void resume() {
}
(if the code is hard to read I use exactly the same code that is in the tutorial linked below)
My package explorer looks like this :
imgur link
And it returns me :
Exception in thread "LWJGL Application" com.badlogic.gdx.utils.GdxRuntimeException: File not found: spritesheet.atlas (Internal)
at com.badlogic.gdx.files.FileHandle.read(FileHandle.java:136)
at com.badlogic.gdx.graphics.g2d.TextureAtlas$TextureAtlasData.(TextureAtlas.java:103)
at com.badlogic.gdx.graphics.g2d.TextureAtlas.(TextureAtlas.java:231)
at com.badlogic.gdx.graphics.g2d.TextureAtlas.(TextureAtlas.java:226)
at com.badlogic.gdx.graphics.g2d.TextureAtlas.(TextureAtlas.java:216)
at com.mygdx.game.MyGdxGame.create(MyGdxGame.java:23)
at com.badlogic.gdx.backends.lwjgl.LwjglApplication.mainLoop(LwjglApplication.java:149)
at com.badlogic.gdx.backends.lwjgl.LwjglApplication$1.run(LwjglApplication.java:126)
I tried some trick like Project>Clean, refreshing, close and re-open Eclipse and even recreated a project. Any idea ?
java eclipse libgdx
add a comment |
up vote
0
down vote
favorite
I have an issue using LibGDX with assets directory. I actually build my project folder this way. I follow this tutorial to learn. (I work on Eclipse)
The code I use is :
package com.mygdx.game;
import com.badlogic.gdx.ApplicationListener;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.g2d.Sprite;
import com.badlogic.gdx.graphics.g2d.SpriteBatch;
import com.badlogic.gdx.graphics.g2d.TextureAtlas;
import com.badlogic.gdx.graphics.g2d.TextureAtlas.AtlasRegion;
import com.badlogic.gdx.utils.Timer;
import com.badlogic.gdx.utils.Timer.Task;
public class MyGdxGame implements ApplicationListener {
private SpriteBatch batch;
private TextureAtlas textureAtlas;
private Sprite sprite;
private int currentFrame = 1;
private String currentAtlasKey = new String("0001");
@Override
public void create() {
batch = new SpriteBatch();
// THE PROBLEM IS UNDER THIS LINE
textureAtlas = new TextureAtlas(Gdx.files.internal("spritesheet.atlas"));
AtlasRegion region = textureAtlas.findRegion("0001");
sprite = new Sprite(region);
sprite.setPosition(120, 100);
sprite.scale(2.5f);
Timer.schedule(new Task(){
@Override
public void run() {
currentFrame++;
if(currentFrame > 20)
currentFrame = 1;
// ATTENTION! String.format() doesnt work under GWT for god knows why...
currentAtlasKey = String.format("%04d", currentFrame);
sprite.setRegion(textureAtlas.findRegion(currentAtlasKey));
}
}
,0,1/30.0f);
}
@Override
public void dispose() {
batch.dispose();
textureAtlas.dispose();
}
@Override
public void render() {
Gdx.gl.glClearColor(0, 0, 0, 1);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
batch.begin();
sprite.draw(batch);
batch.end();
}
@Override
public void resize(int width, int height) {
}
@Override
public void pause() {
}
@Override
public void resume() {
}
(if the code is hard to read I use exactly the same code that is in the tutorial linked below)
My package explorer looks like this :
imgur link
And it returns me :
Exception in thread "LWJGL Application" com.badlogic.gdx.utils.GdxRuntimeException: File not found: spritesheet.atlas (Internal)
at com.badlogic.gdx.files.FileHandle.read(FileHandle.java:136)
at com.badlogic.gdx.graphics.g2d.TextureAtlas$TextureAtlasData.(TextureAtlas.java:103)
at com.badlogic.gdx.graphics.g2d.TextureAtlas.(TextureAtlas.java:231)
at com.badlogic.gdx.graphics.g2d.TextureAtlas.(TextureAtlas.java:226)
at com.badlogic.gdx.graphics.g2d.TextureAtlas.(TextureAtlas.java:216)
at com.mygdx.game.MyGdxGame.create(MyGdxGame.java:23)
at com.badlogic.gdx.backends.lwjgl.LwjglApplication.mainLoop(LwjglApplication.java:149)
at com.badlogic.gdx.backends.lwjgl.LwjglApplication$1.run(LwjglApplication.java:126)
I tried some trick like Project>Clean, refreshing, close and re-open Eclipse and even recreated a project. Any idea ?
java eclipse libgdx
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have an issue using LibGDX with assets directory. I actually build my project folder this way. I follow this tutorial to learn. (I work on Eclipse)
The code I use is :
package com.mygdx.game;
import com.badlogic.gdx.ApplicationListener;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.g2d.Sprite;
import com.badlogic.gdx.graphics.g2d.SpriteBatch;
import com.badlogic.gdx.graphics.g2d.TextureAtlas;
import com.badlogic.gdx.graphics.g2d.TextureAtlas.AtlasRegion;
import com.badlogic.gdx.utils.Timer;
import com.badlogic.gdx.utils.Timer.Task;
public class MyGdxGame implements ApplicationListener {
private SpriteBatch batch;
private TextureAtlas textureAtlas;
private Sprite sprite;
private int currentFrame = 1;
private String currentAtlasKey = new String("0001");
@Override
public void create() {
batch = new SpriteBatch();
// THE PROBLEM IS UNDER THIS LINE
textureAtlas = new TextureAtlas(Gdx.files.internal("spritesheet.atlas"));
AtlasRegion region = textureAtlas.findRegion("0001");
sprite = new Sprite(region);
sprite.setPosition(120, 100);
sprite.scale(2.5f);
Timer.schedule(new Task(){
@Override
public void run() {
currentFrame++;
if(currentFrame > 20)
currentFrame = 1;
// ATTENTION! String.format() doesnt work under GWT for god knows why...
currentAtlasKey = String.format("%04d", currentFrame);
sprite.setRegion(textureAtlas.findRegion(currentAtlasKey));
}
}
,0,1/30.0f);
}
@Override
public void dispose() {
batch.dispose();
textureAtlas.dispose();
}
@Override
public void render() {
Gdx.gl.glClearColor(0, 0, 0, 1);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
batch.begin();
sprite.draw(batch);
batch.end();
}
@Override
public void resize(int width, int height) {
}
@Override
public void pause() {
}
@Override
public void resume() {
}
(if the code is hard to read I use exactly the same code that is in the tutorial linked below)
My package explorer looks like this :
imgur link
And it returns me :
Exception in thread "LWJGL Application" com.badlogic.gdx.utils.GdxRuntimeException: File not found: spritesheet.atlas (Internal)
at com.badlogic.gdx.files.FileHandle.read(FileHandle.java:136)
at com.badlogic.gdx.graphics.g2d.TextureAtlas$TextureAtlasData.(TextureAtlas.java:103)
at com.badlogic.gdx.graphics.g2d.TextureAtlas.(TextureAtlas.java:231)
at com.badlogic.gdx.graphics.g2d.TextureAtlas.(TextureAtlas.java:226)
at com.badlogic.gdx.graphics.g2d.TextureAtlas.(TextureAtlas.java:216)
at com.mygdx.game.MyGdxGame.create(MyGdxGame.java:23)
at com.badlogic.gdx.backends.lwjgl.LwjglApplication.mainLoop(LwjglApplication.java:149)
at com.badlogic.gdx.backends.lwjgl.LwjglApplication$1.run(LwjglApplication.java:126)
I tried some trick like Project>Clean, refreshing, close and re-open Eclipse and even recreated a project. Any idea ?
java eclipse libgdx
I have an issue using LibGDX with assets directory. I actually build my project folder this way. I follow this tutorial to learn. (I work on Eclipse)
The code I use is :
package com.mygdx.game;
import com.badlogic.gdx.ApplicationListener;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.g2d.Sprite;
import com.badlogic.gdx.graphics.g2d.SpriteBatch;
import com.badlogic.gdx.graphics.g2d.TextureAtlas;
import com.badlogic.gdx.graphics.g2d.TextureAtlas.AtlasRegion;
import com.badlogic.gdx.utils.Timer;
import com.badlogic.gdx.utils.Timer.Task;
public class MyGdxGame implements ApplicationListener {
private SpriteBatch batch;
private TextureAtlas textureAtlas;
private Sprite sprite;
private int currentFrame = 1;
private String currentAtlasKey = new String("0001");
@Override
public void create() {
batch = new SpriteBatch();
// THE PROBLEM IS UNDER THIS LINE
textureAtlas = new TextureAtlas(Gdx.files.internal("spritesheet.atlas"));
AtlasRegion region = textureAtlas.findRegion("0001");
sprite = new Sprite(region);
sprite.setPosition(120, 100);
sprite.scale(2.5f);
Timer.schedule(new Task(){
@Override
public void run() {
currentFrame++;
if(currentFrame > 20)
currentFrame = 1;
// ATTENTION! String.format() doesnt work under GWT for god knows why...
currentAtlasKey = String.format("%04d", currentFrame);
sprite.setRegion(textureAtlas.findRegion(currentAtlasKey));
}
}
,0,1/30.0f);
}
@Override
public void dispose() {
batch.dispose();
textureAtlas.dispose();
}
@Override
public void render() {
Gdx.gl.glClearColor(0, 0, 0, 1);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
batch.begin();
sprite.draw(batch);
batch.end();
}
@Override
public void resize(int width, int height) {
}
@Override
public void pause() {
}
@Override
public void resume() {
}
(if the code is hard to read I use exactly the same code that is in the tutorial linked below)
My package explorer looks like this :
imgur link
And it returns me :
Exception in thread "LWJGL Application" com.badlogic.gdx.utils.GdxRuntimeException: File not found: spritesheet.atlas (Internal)
at com.badlogic.gdx.files.FileHandle.read(FileHandle.java:136)
at com.badlogic.gdx.graphics.g2d.TextureAtlas$TextureAtlasData.(TextureAtlas.java:103)
at com.badlogic.gdx.graphics.g2d.TextureAtlas.(TextureAtlas.java:231)
at com.badlogic.gdx.graphics.g2d.TextureAtlas.(TextureAtlas.java:226)
at com.badlogic.gdx.graphics.g2d.TextureAtlas.(TextureAtlas.java:216)
at com.mygdx.game.MyGdxGame.create(MyGdxGame.java:23)
at com.badlogic.gdx.backends.lwjgl.LwjglApplication.mainLoop(LwjglApplication.java:149)
at com.badlogic.gdx.backends.lwjgl.LwjglApplication$1.run(LwjglApplication.java:126)
I tried some trick like Project>Clean, refreshing, close and re-open Eclipse and even recreated a project. Any idea ?
java eclipse libgdx
java eclipse libgdx
asked Nov 10 at 19:07
SamHel
65
65
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
maybe you haven't linked the asset folder, if so,
try right click on my-gdx-game-desktop, in properties dialog select Java Build Path, click link source button, then navigate to the assets folder.
if you're already set it, try readd with this step, restart eclipse if needed
Thank's for your answer, wasn't that in my case. But I know it can be a reason why it doesn't work indeed.
– SamHel
Nov 11 at 10:11
add a comment |
up vote
0
down vote
accepted
Okay, I found the trick. For those which encounter the same issue (working on Eclipse but it's pretty the same whatever the IDE is) there is one thread on stack overflow already existing which give additional solutions.
For me I had to set-up the "working directory" (for the desktop main for exemple). To do this go on Run>Run configuration>Arguments and at working directory's section there is Default and Other. Tick Other's box and me I had to write ${workspace_loc:my-gdx-game-core/assets} but I think it works like ${workspace_loc:[name-of-your-core-directory]/assets}. Hope it helped.
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
maybe you haven't linked the asset folder, if so,
try right click on my-gdx-game-desktop, in properties dialog select Java Build Path, click link source button, then navigate to the assets folder.
if you're already set it, try readd with this step, restart eclipse if needed
Thank's for your answer, wasn't that in my case. But I know it can be a reason why it doesn't work indeed.
– SamHel
Nov 11 at 10:11
add a comment |
up vote
0
down vote
maybe you haven't linked the asset folder, if so,
try right click on my-gdx-game-desktop, in properties dialog select Java Build Path, click link source button, then navigate to the assets folder.
if you're already set it, try readd with this step, restart eclipse if needed
Thank's for your answer, wasn't that in my case. But I know it can be a reason why it doesn't work indeed.
– SamHel
Nov 11 at 10:11
add a comment |
up vote
0
down vote
up vote
0
down vote
maybe you haven't linked the asset folder, if so,
try right click on my-gdx-game-desktop, in properties dialog select Java Build Path, click link source button, then navigate to the assets folder.
if you're already set it, try readd with this step, restart eclipse if needed
maybe you haven't linked the asset folder, if so,
try right click on my-gdx-game-desktop, in properties dialog select Java Build Path, click link source button, then navigate to the assets folder.
if you're already set it, try readd with this step, restart eclipse if needed
answered Nov 11 at 3:48


merecoin
1615
1615
Thank's for your answer, wasn't that in my case. But I know it can be a reason why it doesn't work indeed.
– SamHel
Nov 11 at 10:11
add a comment |
Thank's for your answer, wasn't that in my case. But I know it can be a reason why it doesn't work indeed.
– SamHel
Nov 11 at 10:11
Thank's for your answer, wasn't that in my case. But I know it can be a reason why it doesn't work indeed.
– SamHel
Nov 11 at 10:11
Thank's for your answer, wasn't that in my case. But I know it can be a reason why it doesn't work indeed.
– SamHel
Nov 11 at 10:11
add a comment |
up vote
0
down vote
accepted
Okay, I found the trick. For those which encounter the same issue (working on Eclipse but it's pretty the same whatever the IDE is) there is one thread on stack overflow already existing which give additional solutions.
For me I had to set-up the "working directory" (for the desktop main for exemple). To do this go on Run>Run configuration>Arguments and at working directory's section there is Default and Other. Tick Other's box and me I had to write ${workspace_loc:my-gdx-game-core/assets} but I think it works like ${workspace_loc:[name-of-your-core-directory]/assets}. Hope it helped.
add a comment |
up vote
0
down vote
accepted
Okay, I found the trick. For those which encounter the same issue (working on Eclipse but it's pretty the same whatever the IDE is) there is one thread on stack overflow already existing which give additional solutions.
For me I had to set-up the "working directory" (for the desktop main for exemple). To do this go on Run>Run configuration>Arguments and at working directory's section there is Default and Other. Tick Other's box and me I had to write ${workspace_loc:my-gdx-game-core/assets} but I think it works like ${workspace_loc:[name-of-your-core-directory]/assets}. Hope it helped.
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Okay, I found the trick. For those which encounter the same issue (working on Eclipse but it's pretty the same whatever the IDE is) there is one thread on stack overflow already existing which give additional solutions.
For me I had to set-up the "working directory" (for the desktop main for exemple). To do this go on Run>Run configuration>Arguments and at working directory's section there is Default and Other. Tick Other's box and me I had to write ${workspace_loc:my-gdx-game-core/assets} but I think it works like ${workspace_loc:[name-of-your-core-directory]/assets}. Hope it helped.
Okay, I found the trick. For those which encounter the same issue (working on Eclipse but it's pretty the same whatever the IDE is) there is one thread on stack overflow already existing which give additional solutions.
For me I had to set-up the "working directory" (for the desktop main for exemple). To do this go on Run>Run configuration>Arguments and at working directory's section there is Default and Other. Tick Other's box and me I had to write ${workspace_loc:my-gdx-game-core/assets} but I think it works like ${workspace_loc:[name-of-your-core-directory]/assets}. Hope it helped.
answered Nov 11 at 10:10
SamHel
65
65
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53242459%2flibgdx-gdx-files-internal-file-not-found%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9UQnzSAl6t,834cN,4l,M,JTcvXGQK,lS27tDfeJ,j,qkZKnd8c,f QGxIcsftDa,UFFU omGw0UwM2aR8mqTa1ZNjlBJa