Function count calls
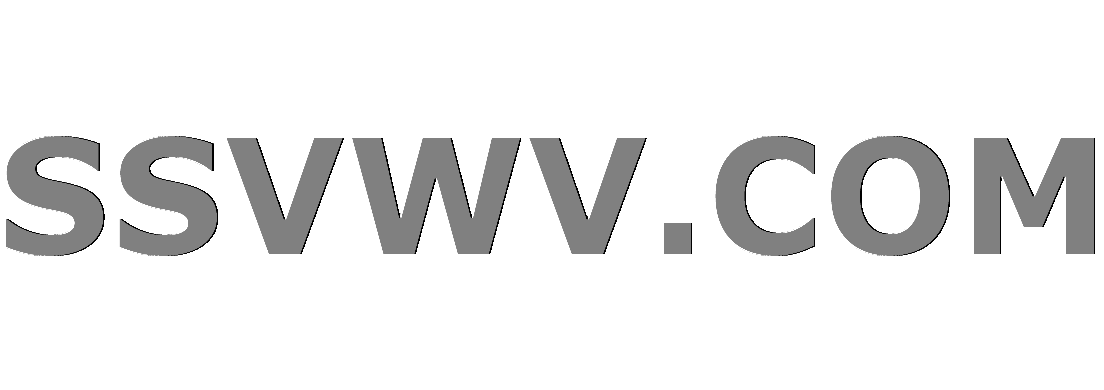
Multi tool use
up vote
5
down vote
favorite
I'm a beginner with JavaScript so please be patient =)
I am trying to write a function that counts the number of times it is called. What I have so far is a function with a counter that is incremented explicitly:
var increment = function () {
var i = 0;
this.inc = function () {i += 1;};
this.get = function () {return i;};
};
var ob = new increment();
ob.inc();
ob.inc();
alert(ob.get());
But I'm wondering how to call only ob();
, so the function could increment calls made to itself automatically. Is this possible and if so, how?
javascript function closures
add a comment |
up vote
5
down vote
favorite
I'm a beginner with JavaScript so please be patient =)
I am trying to write a function that counts the number of times it is called. What I have so far is a function with a counter that is incremented explicitly:
var increment = function () {
var i = 0;
this.inc = function () {i += 1;};
this.get = function () {return i;};
};
var ob = new increment();
ob.inc();
ob.inc();
alert(ob.get());
But I'm wondering how to call only ob();
, so the function could increment calls made to itself automatically. Is this possible and if so, how?
javascript function closures
add a comment |
up vote
5
down vote
favorite
up vote
5
down vote
favorite
I'm a beginner with JavaScript so please be patient =)
I am trying to write a function that counts the number of times it is called. What I have so far is a function with a counter that is incremented explicitly:
var increment = function () {
var i = 0;
this.inc = function () {i += 1;};
this.get = function () {return i;};
};
var ob = new increment();
ob.inc();
ob.inc();
alert(ob.get());
But I'm wondering how to call only ob();
, so the function could increment calls made to itself automatically. Is this possible and if so, how?
javascript function closures
I'm a beginner with JavaScript so please be patient =)
I am trying to write a function that counts the number of times it is called. What I have so far is a function with a counter that is incremented explicitly:
var increment = function () {
var i = 0;
this.inc = function () {i += 1;};
this.get = function () {return i;};
};
var ob = new increment();
ob.inc();
ob.inc();
alert(ob.get());
But I'm wondering how to call only ob();
, so the function could increment calls made to itself automatically. Is this possible and if so, how?
javascript function closures
javascript function closures
edited Aug 31 '11 at 6:45
Ray Toal
64.7k10118174
64.7k10118174
asked Aug 30 '11 at 12:26
Kamil
2612
2612
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
up vote
7
down vote
var increment = function() {
var i = 0;
return function() { return i += 1; };
};
var ob = increment();
2
+1 for throwing closures to a noob :)
– naveen
Aug 30 '11 at 12:36
1
+1. @naveen: The noob seems to be heading that way on his/her own. :)
– Shef
Aug 30 '11 at 12:38
@Shef: I said its good. Its a while before I realized there were closures. But then js was my secondary language. a tiny perk that has alertboxes :)
– naveen
Aug 30 '11 at 12:43
1
@naveen: I just agreed with you. :) I, too, was surprised to see the OP, a self-declared beginner, trying to learn closures.
– Shef
Aug 30 '11 at 12:50
2
@Vaibhav: The increment function itself doesn't increment anything. It returns a function that does.ob
holds that function.
– Marcelo Cantos
Apr 14 '15 at 9:48
|
show 4 more comments
up vote
0
down vote
ob = function (){
++ob.i || (ob.i=1);
return ob.i;
}
add a comment |
up vote
0
down vote
Wrap a counter to any function:
/**
* Wrap a counter to a function
* Count how many times a function is called
* @param {Function} fn Function to count
* @param {Number} count Counter, default to 1
*/
function addCounterToFn(fn, count = 1) {
return function () {
fn.apply(null, arguments);
return count++;
}
}
See https://jsfiddle.net/n50eszwm/
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
7
down vote
var increment = function() {
var i = 0;
return function() { return i += 1; };
};
var ob = increment();
2
+1 for throwing closures to a noob :)
– naveen
Aug 30 '11 at 12:36
1
+1. @naveen: The noob seems to be heading that way on his/her own. :)
– Shef
Aug 30 '11 at 12:38
@Shef: I said its good. Its a while before I realized there were closures. But then js was my secondary language. a tiny perk that has alertboxes :)
– naveen
Aug 30 '11 at 12:43
1
@naveen: I just agreed with you. :) I, too, was surprised to see the OP, a self-declared beginner, trying to learn closures.
– Shef
Aug 30 '11 at 12:50
2
@Vaibhav: The increment function itself doesn't increment anything. It returns a function that does.ob
holds that function.
– Marcelo Cantos
Apr 14 '15 at 9:48
|
show 4 more comments
up vote
7
down vote
var increment = function() {
var i = 0;
return function() { return i += 1; };
};
var ob = increment();
2
+1 for throwing closures to a noob :)
– naveen
Aug 30 '11 at 12:36
1
+1. @naveen: The noob seems to be heading that way on his/her own. :)
– Shef
Aug 30 '11 at 12:38
@Shef: I said its good. Its a while before I realized there were closures. But then js was my secondary language. a tiny perk that has alertboxes :)
– naveen
Aug 30 '11 at 12:43
1
@naveen: I just agreed with you. :) I, too, was surprised to see the OP, a self-declared beginner, trying to learn closures.
– Shef
Aug 30 '11 at 12:50
2
@Vaibhav: The increment function itself doesn't increment anything. It returns a function that does.ob
holds that function.
– Marcelo Cantos
Apr 14 '15 at 9:48
|
show 4 more comments
up vote
7
down vote
up vote
7
down vote
var increment = function() {
var i = 0;
return function() { return i += 1; };
};
var ob = increment();
var increment = function() {
var i = 0;
return function() { return i += 1; };
};
var ob = increment();
answered Aug 30 '11 at 12:30
Marcelo Cantos
142k31279326
142k31279326
2
+1 for throwing closures to a noob :)
– naveen
Aug 30 '11 at 12:36
1
+1. @naveen: The noob seems to be heading that way on his/her own. :)
– Shef
Aug 30 '11 at 12:38
@Shef: I said its good. Its a while before I realized there were closures. But then js was my secondary language. a tiny perk that has alertboxes :)
– naveen
Aug 30 '11 at 12:43
1
@naveen: I just agreed with you. :) I, too, was surprised to see the OP, a self-declared beginner, trying to learn closures.
– Shef
Aug 30 '11 at 12:50
2
@Vaibhav: The increment function itself doesn't increment anything. It returns a function that does.ob
holds that function.
– Marcelo Cantos
Apr 14 '15 at 9:48
|
show 4 more comments
2
+1 for throwing closures to a noob :)
– naveen
Aug 30 '11 at 12:36
1
+1. @naveen: The noob seems to be heading that way on his/her own. :)
– Shef
Aug 30 '11 at 12:38
@Shef: I said its good. Its a while before I realized there were closures. But then js was my secondary language. a tiny perk that has alertboxes :)
– naveen
Aug 30 '11 at 12:43
1
@naveen: I just agreed with you. :) I, too, was surprised to see the OP, a self-declared beginner, trying to learn closures.
– Shef
Aug 30 '11 at 12:50
2
@Vaibhav: The increment function itself doesn't increment anything. It returns a function that does.ob
holds that function.
– Marcelo Cantos
Apr 14 '15 at 9:48
2
2
+1 for throwing closures to a noob :)
– naveen
Aug 30 '11 at 12:36
+1 for throwing closures to a noob :)
– naveen
Aug 30 '11 at 12:36
1
1
+1. @naveen: The noob seems to be heading that way on his/her own. :)
– Shef
Aug 30 '11 at 12:38
+1. @naveen: The noob seems to be heading that way on his/her own. :)
– Shef
Aug 30 '11 at 12:38
@Shef: I said its good. Its a while before I realized there were closures. But then js was my secondary language. a tiny perk that has alertboxes :)
– naveen
Aug 30 '11 at 12:43
@Shef: I said its good. Its a while before I realized there were closures. But then js was my secondary language. a tiny perk that has alertboxes :)
– naveen
Aug 30 '11 at 12:43
1
1
@naveen: I just agreed with you. :) I, too, was surprised to see the OP, a self-declared beginner, trying to learn closures.
– Shef
Aug 30 '11 at 12:50
@naveen: I just agreed with you. :) I, too, was surprised to see the OP, a self-declared beginner, trying to learn closures.
– Shef
Aug 30 '11 at 12:50
2
2
@Vaibhav: The increment function itself doesn't increment anything. It returns a function that does.
ob
holds that function.– Marcelo Cantos
Apr 14 '15 at 9:48
@Vaibhav: The increment function itself doesn't increment anything. It returns a function that does.
ob
holds that function.– Marcelo Cantos
Apr 14 '15 at 9:48
|
show 4 more comments
up vote
0
down vote
ob = function (){
++ob.i || (ob.i=1);
return ob.i;
}
add a comment |
up vote
0
down vote
ob = function (){
++ob.i || (ob.i=1);
return ob.i;
}
add a comment |
up vote
0
down vote
up vote
0
down vote
ob = function (){
++ob.i || (ob.i=1);
return ob.i;
}
ob = function (){
++ob.i || (ob.i=1);
return ob.i;
}
edited Mar 14 '17 at 21:23
answered Mar 14 '17 at 20:53
aljgom
1,146812
1,146812
add a comment |
add a comment |
up vote
0
down vote
Wrap a counter to any function:
/**
* Wrap a counter to a function
* Count how many times a function is called
* @param {Function} fn Function to count
* @param {Number} count Counter, default to 1
*/
function addCounterToFn(fn, count = 1) {
return function () {
fn.apply(null, arguments);
return count++;
}
}
See https://jsfiddle.net/n50eszwm/
add a comment |
up vote
0
down vote
Wrap a counter to any function:
/**
* Wrap a counter to a function
* Count how many times a function is called
* @param {Function} fn Function to count
* @param {Number} count Counter, default to 1
*/
function addCounterToFn(fn, count = 1) {
return function () {
fn.apply(null, arguments);
return count++;
}
}
See https://jsfiddle.net/n50eszwm/
add a comment |
up vote
0
down vote
up vote
0
down vote
Wrap a counter to any function:
/**
* Wrap a counter to a function
* Count how many times a function is called
* @param {Function} fn Function to count
* @param {Number} count Counter, default to 1
*/
function addCounterToFn(fn, count = 1) {
return function () {
fn.apply(null, arguments);
return count++;
}
}
See https://jsfiddle.net/n50eszwm/
Wrap a counter to any function:
/**
* Wrap a counter to a function
* Count how many times a function is called
* @param {Function} fn Function to count
* @param {Number} count Counter, default to 1
*/
function addCounterToFn(fn, count = 1) {
return function () {
fn.apply(null, arguments);
return count++;
}
}
See https://jsfiddle.net/n50eszwm/
answered Nov 10 at 23:46


pldg
412417
412417
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f7243101%2ffunction-count-calls%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
FgiW,E0ytpL pQKwpw5,Pi P10oDZhyfNXe xa6M