@GetMapping with array parameters by application.yml
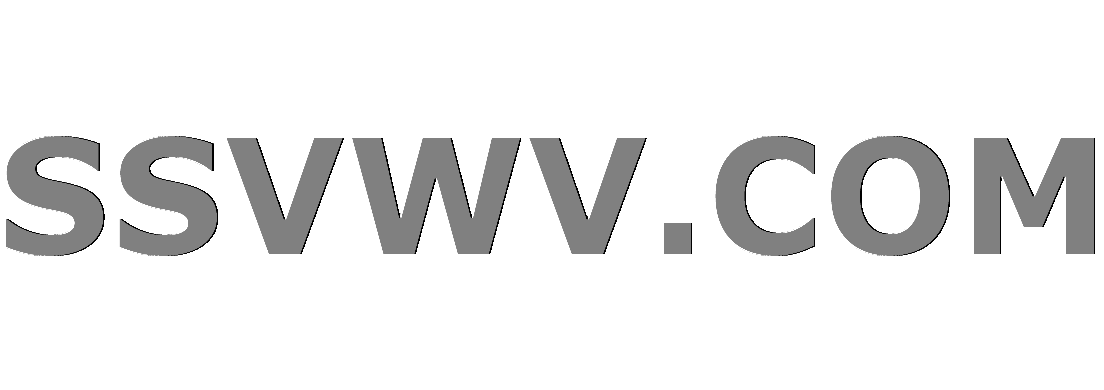
Multi tool use
I have developed this @GetMapping RestController and all works fine
@GetMapping(path = {"foo", "bar"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
now I want externalize the values inside the path array using my application.yml file,so I writed
url:
- foo
- bar
and I modified my code in order to use it, but it doesn't work in this two different ways
@GetMapping(path = "${url}")
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
@GetMapping(path = {"${url}"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
I don't understand if the application properties are not correctly formatted or I need to use the SpEL (https://docs.spring.io/spring/docs/3.0.x/reference/expressions.html.
I also want that the code is dynamic according to the application.yml properties, so if the url values increase or decrease the code must still work.
I'm using Springboot 1.5.13
java spring spring-boot spring-restcontroller
add a comment |
I have developed this @GetMapping RestController and all works fine
@GetMapping(path = {"foo", "bar"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
now I want externalize the values inside the path array using my application.yml file,so I writed
url:
- foo
- bar
and I modified my code in order to use it, but it doesn't work in this two different ways
@GetMapping(path = "${url}")
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
@GetMapping(path = {"${url}"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
I don't understand if the application properties are not correctly formatted or I need to use the SpEL (https://docs.spring.io/spring/docs/3.0.x/reference/expressions.html.
I also want that the code is dynamic according to the application.yml properties, so if the url values increase or decrease the code must still work.
I'm using Springboot 1.5.13
java spring spring-boot spring-restcontroller
You should try @ConfigurationProperties annotation to fetch yml configuration data.
– GauravRai1512
Nov 16 '18 at 11:33
@GauravRai1512 and after that? How I can use the fetched properties and inject it in the @GetMapping annotation? You cannot inject variable in the annotation, only constant
– Federico Gatti
Nov 16 '18 at 11:38
add a comment |
I have developed this @GetMapping RestController and all works fine
@GetMapping(path = {"foo", "bar"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
now I want externalize the values inside the path array using my application.yml file,so I writed
url:
- foo
- bar
and I modified my code in order to use it, but it doesn't work in this two different ways
@GetMapping(path = "${url}")
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
@GetMapping(path = {"${url}"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
I don't understand if the application properties are not correctly formatted or I need to use the SpEL (https://docs.spring.io/spring/docs/3.0.x/reference/expressions.html.
I also want that the code is dynamic according to the application.yml properties, so if the url values increase or decrease the code must still work.
I'm using Springboot 1.5.13
java spring spring-boot spring-restcontroller
I have developed this @GetMapping RestController and all works fine
@GetMapping(path = {"foo", "bar"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
now I want externalize the values inside the path array using my application.yml file,so I writed
url:
- foo
- bar
and I modified my code in order to use it, but it doesn't work in this two different ways
@GetMapping(path = "${url}")
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
@GetMapping(path = {"${url}"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
I don't understand if the application properties are not correctly formatted or I need to use the SpEL (https://docs.spring.io/spring/docs/3.0.x/reference/expressions.html.
I also want that the code is dynamic according to the application.yml properties, so if the url values increase or decrease the code must still work.
I'm using Springboot 1.5.13
java spring spring-boot spring-restcontroller
java spring spring-boot spring-restcontroller
edited Nov 16 '18 at 13:02
Federico Gatti
asked Nov 16 '18 at 11:30


Federico GattiFederico Gatti
152114
152114
You should try @ConfigurationProperties annotation to fetch yml configuration data.
– GauravRai1512
Nov 16 '18 at 11:33
@GauravRai1512 and after that? How I can use the fetched properties and inject it in the @GetMapping annotation? You cannot inject variable in the annotation, only constant
– Federico Gatti
Nov 16 '18 at 11:38
add a comment |
You should try @ConfigurationProperties annotation to fetch yml configuration data.
– GauravRai1512
Nov 16 '18 at 11:33
@GauravRai1512 and after that? How I can use the fetched properties and inject it in the @GetMapping annotation? You cannot inject variable in the annotation, only constant
– Federico Gatti
Nov 16 '18 at 11:38
You should try @ConfigurationProperties annotation to fetch yml configuration data.
– GauravRai1512
Nov 16 '18 at 11:33
You should try @ConfigurationProperties annotation to fetch yml configuration data.
– GauravRai1512
Nov 16 '18 at 11:33
@GauravRai1512 and after that? How I can use the fetched properties and inject it in the @GetMapping annotation? You cannot inject variable in the annotation, only constant
– Federico Gatti
Nov 16 '18 at 11:38
@GauravRai1512 and after that? How I can use the fetched properties and inject it in the @GetMapping annotation? You cannot inject variable in the annotation, only constant
– Federico Gatti
Nov 16 '18 at 11:38
add a comment |
2 Answers
2
active
oldest
votes
You can use in your controller
@GetMapping(path = "${url[0]}")
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
@GetMapping(path = {"${url[1]}"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
Or you can do in this way:
@GetMapping(path = {"${url[0]}","${url[1]}"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
I think this is helpful
Thank you, your second solutions work fine with a specific number of url in the properties, I'm searching for a dynamic solution for any number of values inside the array
– Federico Gatti
Nov 16 '18 at 11:55
add a comment |
You can't bind YAML
list to array or list here. For more information see: @Value and @ConfigurationProperties behave differently when binding to arrays
However, you can achieve this by specifying regular expression in yml file like:
url: '{var:foo|bar}'
And then you can use it directly in your controller:
@GetMapping(path = "${url}")
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
Your solution doesn't work because you have only defined a PathVariable that is called foo|bar so the endpoint is matched by all the call like (localhost/anytypeofrequest)
– Federico Gatti
Nov 19 '18 at 9:39
@FedericoGatti Thanks, missed it. Edited answer.
– Sukhpal Singh
Nov 19 '18 at 10:55
Thank you, in this way is possible to define a PathVaribale only for one of the two urls? Something likeurl: '{var:foo/{foobar}|bar}'
– Federico Gatti
Nov 19 '18 at 11:55
@FedericoGattivar
is name of your@PathVariable
. I think this is what you need.
– Sukhpal Singh
Nov 19 '18 at 12:21
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53336985%2fgetmapping-with-array-parameters-by-application-yml%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use in your controller
@GetMapping(path = "${url[0]}")
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
@GetMapping(path = {"${url[1]}"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
Or you can do in this way:
@GetMapping(path = {"${url[0]}","${url[1]}"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
I think this is helpful
Thank you, your second solutions work fine with a specific number of url in the properties, I'm searching for a dynamic solution for any number of values inside the array
– Federico Gatti
Nov 16 '18 at 11:55
add a comment |
You can use in your controller
@GetMapping(path = "${url[0]}")
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
@GetMapping(path = {"${url[1]}"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
Or you can do in this way:
@GetMapping(path = {"${url[0]}","${url[1]}"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
I think this is helpful
Thank you, your second solutions work fine with a specific number of url in the properties, I'm searching for a dynamic solution for any number of values inside the array
– Federico Gatti
Nov 16 '18 at 11:55
add a comment |
You can use in your controller
@GetMapping(path = "${url[0]}")
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
@GetMapping(path = {"${url[1]}"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
Or you can do in this way:
@GetMapping(path = {"${url[0]}","${url[1]}"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
I think this is helpful
You can use in your controller
@GetMapping(path = "${url[0]}")
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
@GetMapping(path = {"${url[1]}"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
Or you can do in this way:
@GetMapping(path = {"${url[0]}","${url[1]}"})
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
I think this is helpful
answered Nov 16 '18 at 11:39


Mykhailo MoskuraMykhailo Moskura
877214
877214
Thank you, your second solutions work fine with a specific number of url in the properties, I'm searching for a dynamic solution for any number of values inside the array
– Federico Gatti
Nov 16 '18 at 11:55
add a comment |
Thank you, your second solutions work fine with a specific number of url in the properties, I'm searching for a dynamic solution for any number of values inside the array
– Federico Gatti
Nov 16 '18 at 11:55
Thank you, your second solutions work fine with a specific number of url in the properties, I'm searching for a dynamic solution for any number of values inside the array
– Federico Gatti
Nov 16 '18 at 11:55
Thank you, your second solutions work fine with a specific number of url in the properties, I'm searching for a dynamic solution for any number of values inside the array
– Federico Gatti
Nov 16 '18 at 11:55
add a comment |
You can't bind YAML
list to array or list here. For more information see: @Value and @ConfigurationProperties behave differently when binding to arrays
However, you can achieve this by specifying regular expression in yml file like:
url: '{var:foo|bar}'
And then you can use it directly in your controller:
@GetMapping(path = "${url}")
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
Your solution doesn't work because you have only defined a PathVariable that is called foo|bar so the endpoint is matched by all the call like (localhost/anytypeofrequest)
– Federico Gatti
Nov 19 '18 at 9:39
@FedericoGatti Thanks, missed it. Edited answer.
– Sukhpal Singh
Nov 19 '18 at 10:55
Thank you, in this way is possible to define a PathVaribale only for one of the two urls? Something likeurl: '{var:foo/{foobar}|bar}'
– Federico Gatti
Nov 19 '18 at 11:55
@FedericoGattivar
is name of your@PathVariable
. I think this is what you need.
– Sukhpal Singh
Nov 19 '18 at 12:21
add a comment |
You can't bind YAML
list to array or list here. For more information see: @Value and @ConfigurationProperties behave differently when binding to arrays
However, you can achieve this by specifying regular expression in yml file like:
url: '{var:foo|bar}'
And then you can use it directly in your controller:
@GetMapping(path = "${url}")
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
Your solution doesn't work because you have only defined a PathVariable that is called foo|bar so the endpoint is matched by all the call like (localhost/anytypeofrequest)
– Federico Gatti
Nov 19 '18 at 9:39
@FedericoGatti Thanks, missed it. Edited answer.
– Sukhpal Singh
Nov 19 '18 at 10:55
Thank you, in this way is possible to define a PathVaribale only for one of the two urls? Something likeurl: '{var:foo/{foobar}|bar}'
– Federico Gatti
Nov 19 '18 at 11:55
@FedericoGattivar
is name of your@PathVariable
. I think this is what you need.
– Sukhpal Singh
Nov 19 '18 at 12:21
add a comment |
You can't bind YAML
list to array or list here. For more information see: @Value and @ConfigurationProperties behave differently when binding to arrays
However, you can achieve this by specifying regular expression in yml file like:
url: '{var:foo|bar}'
And then you can use it directly in your controller:
@GetMapping(path = "${url}")
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
You can't bind YAML
list to array or list here. For more information see: @Value and @ConfigurationProperties behave differently when binding to arrays
However, you can achieve this by specifying regular expression in yml file like:
url: '{var:foo|bar}'
And then you can use it directly in your controller:
@GetMapping(path = "${url}")
public ResponseEntity<String> foobar() {
return ResponseEntity.ok("foobar");
}
edited Nov 19 '18 at 10:54
answered Nov 18 '18 at 13:48


Sukhpal SinghSukhpal Singh
1,039212
1,039212
Your solution doesn't work because you have only defined a PathVariable that is called foo|bar so the endpoint is matched by all the call like (localhost/anytypeofrequest)
– Federico Gatti
Nov 19 '18 at 9:39
@FedericoGatti Thanks, missed it. Edited answer.
– Sukhpal Singh
Nov 19 '18 at 10:55
Thank you, in this way is possible to define a PathVaribale only for one of the two urls? Something likeurl: '{var:foo/{foobar}|bar}'
– Federico Gatti
Nov 19 '18 at 11:55
@FedericoGattivar
is name of your@PathVariable
. I think this is what you need.
– Sukhpal Singh
Nov 19 '18 at 12:21
add a comment |
Your solution doesn't work because you have only defined a PathVariable that is called foo|bar so the endpoint is matched by all the call like (localhost/anytypeofrequest)
– Federico Gatti
Nov 19 '18 at 9:39
@FedericoGatti Thanks, missed it. Edited answer.
– Sukhpal Singh
Nov 19 '18 at 10:55
Thank you, in this way is possible to define a PathVaribale only for one of the two urls? Something likeurl: '{var:foo/{foobar}|bar}'
– Federico Gatti
Nov 19 '18 at 11:55
@FedericoGattivar
is name of your@PathVariable
. I think this is what you need.
– Sukhpal Singh
Nov 19 '18 at 12:21
Your solution doesn't work because you have only defined a PathVariable that is called foo|bar so the endpoint is matched by all the call like (localhost/anytypeofrequest)
– Federico Gatti
Nov 19 '18 at 9:39
Your solution doesn't work because you have only defined a PathVariable that is called foo|bar so the endpoint is matched by all the call like (localhost/anytypeofrequest)
– Federico Gatti
Nov 19 '18 at 9:39
@FedericoGatti Thanks, missed it. Edited answer.
– Sukhpal Singh
Nov 19 '18 at 10:55
@FedericoGatti Thanks, missed it. Edited answer.
– Sukhpal Singh
Nov 19 '18 at 10:55
Thank you, in this way is possible to define a PathVaribale only for one of the two urls? Something like
url: '{var:foo/{foobar}|bar}'
– Federico Gatti
Nov 19 '18 at 11:55
Thank you, in this way is possible to define a PathVaribale only for one of the two urls? Something like
url: '{var:foo/{foobar}|bar}'
– Federico Gatti
Nov 19 '18 at 11:55
@FedericoGatti
var
is name of your @PathVariable
. I think this is what you need.– Sukhpal Singh
Nov 19 '18 at 12:21
@FedericoGatti
var
is name of your @PathVariable
. I think this is what you need.– Sukhpal Singh
Nov 19 '18 at 12:21
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53336985%2fgetmapping-with-array-parameters-by-application-yml%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XIXRneEi 9IMib1 YGKM09eO Xfw7
You should try @ConfigurationProperties annotation to fetch yml configuration data.
– GauravRai1512
Nov 16 '18 at 11:33
@GauravRai1512 and after that? How I can use the fetched properties and inject it in the @GetMapping annotation? You cannot inject variable in the annotation, only constant
– Federico Gatti
Nov 16 '18 at 11:38