Post Requests in Python For JSON Objects
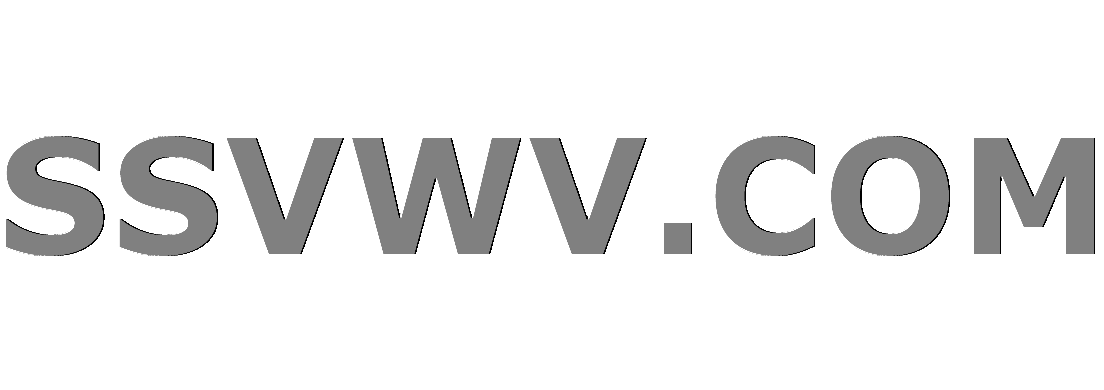
Multi tool use
I have a dataframe (df) in pandas which I have converted to JSON formot as:
json_obj = df.to_json(orient=records).
The json object looks like (say):
json_obj = [
{"a": "xxx", "b":"pqr", "c": 1},
{"a": "uuy", "b":"abc", "c": 3},
{"a": "yty", "b":"nnq", "c": 7}
]
Now when I send the data using an API URL (urlex (say)) (valid) as
import requests
r1 = requests.post('urlex', json = [
{"a": "xxx", "b":"pqr", "c": 1},
{"a":"uuy", "b":"abc", "c": 3},
{"a": "yty", "b":"nnq", "c": 7}
]
print (r1.status_code)
print(r1.content)
I get the response code 200 with **b'{"success":true}'**
However, when I do the same with
r1 = requests.post('urlex', json = json_obj ]
print (r1.status_code)
print(r1.content)
I get the response code 200 with **b'{"success":false}'**
What am I missing and what is the problem?
python json pandas api
add a comment |
I have a dataframe (df) in pandas which I have converted to JSON formot as:
json_obj = df.to_json(orient=records).
The json object looks like (say):
json_obj = [
{"a": "xxx", "b":"pqr", "c": 1},
{"a": "uuy", "b":"abc", "c": 3},
{"a": "yty", "b":"nnq", "c": 7}
]
Now when I send the data using an API URL (urlex (say)) (valid) as
import requests
r1 = requests.post('urlex', json = [
{"a": "xxx", "b":"pqr", "c": 1},
{"a":"uuy", "b":"abc", "c": 3},
{"a": "yty", "b":"nnq", "c": 7}
]
print (r1.status_code)
print(r1.content)
I get the response code 200 with **b'{"success":true}'**
However, when I do the same with
r1 = requests.post('urlex', json = json_obj ]
print (r1.status_code)
print(r1.content)
I get the response code 200 with **b'{"success":false}'**
What am I missing and what is the problem?
python json pandas api
add a comment |
I have a dataframe (df) in pandas which I have converted to JSON formot as:
json_obj = df.to_json(orient=records).
The json object looks like (say):
json_obj = [
{"a": "xxx", "b":"pqr", "c": 1},
{"a": "uuy", "b":"abc", "c": 3},
{"a": "yty", "b":"nnq", "c": 7}
]
Now when I send the data using an API URL (urlex (say)) (valid) as
import requests
r1 = requests.post('urlex', json = [
{"a": "xxx", "b":"pqr", "c": 1},
{"a":"uuy", "b":"abc", "c": 3},
{"a": "yty", "b":"nnq", "c": 7}
]
print (r1.status_code)
print(r1.content)
I get the response code 200 with **b'{"success":true}'**
However, when I do the same with
r1 = requests.post('urlex', json = json_obj ]
print (r1.status_code)
print(r1.content)
I get the response code 200 with **b'{"success":false}'**
What am I missing and what is the problem?
python json pandas api
I have a dataframe (df) in pandas which I have converted to JSON formot as:
json_obj = df.to_json(orient=records).
The json object looks like (say):
json_obj = [
{"a": "xxx", "b":"pqr", "c": 1},
{"a": "uuy", "b":"abc", "c": 3},
{"a": "yty", "b":"nnq", "c": 7}
]
Now when I send the data using an API URL (urlex (say)) (valid) as
import requests
r1 = requests.post('urlex', json = [
{"a": "xxx", "b":"pqr", "c": 1},
{"a":"uuy", "b":"abc", "c": 3},
{"a": "yty", "b":"nnq", "c": 7}
]
print (r1.status_code)
print(r1.content)
I get the response code 200 with **b'{"success":true}'**
However, when I do the same with
r1 = requests.post('urlex', json = json_obj ]
print (r1.status_code)
print(r1.content)
I get the response code 200 with **b'{"success":false}'**
What am I missing and what is the problem?
python json pandas api
python json pandas api
edited Nov 16 '18 at 2:23


nyedidikeke
2,82642135
2,82642135
asked Nov 15 '18 at 23:13
StanStan
467
467
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
I think your problem is that pd.DataFrame.to_json
returns a string:
data = pd.DataFrame({'a': [1, 2]})
type(data.to_json())
str
but the json
keyword argument of requests.post
expects a python object. If you want to submit a son string, use the data=
argument instead:
# this submits a jsonified string
response = requests.post('http://localhost:8888', json=data.to_json())
print(response.request.body.decode('utf-8'))
"{"a":{"0":1,"1":2}}"
# this submits the actual json object
response = requests.post(
'http://localhost:8888',
data=data.to_json().encode('utf-8'),
headers={'Content-Type': 'application/json'}
)
print(response.request.body.decode('utf-8')
{"a":{"0":1,"1":2}}
I am not sure whether encoding is necessary.
I have been able to use json.loads(json_obj), which worked...
– Stan
Nov 16 '18 at 0:03
add a comment |
You can take advantage of the simplejson
or json
packages:
import simplejson as json
response = requests.post('http://localhost:8888', data=json.loads(df.to_json(orient='records')),
headers={'Content-Type': 'application/json'}
)
Additionally, if you didn't want to orient as records you can use to_dict
instead of to_json
response = requests.post('http://localhost:8888', data=df.to_dict(),
headers={'Content-Type': 'application/json'}
)
The reason this works is because the data
argument in the requests
package accepts a string.
I have been able to use json.loads(json_obj), which worked...
– Stan
Nov 16 '18 at 0:04
@Stan yes, that's an alternative that fixes the problem as well
– Matthias Ossadnik
Nov 16 '18 at 0:04
Thank you !! I will try your one too.. Always better to have many ways
– Stan
Nov 16 '18 at 0:06
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53329189%2fpost-requests-in-python-for-json-objects%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think your problem is that pd.DataFrame.to_json
returns a string:
data = pd.DataFrame({'a': [1, 2]})
type(data.to_json())
str
but the json
keyword argument of requests.post
expects a python object. If you want to submit a son string, use the data=
argument instead:
# this submits a jsonified string
response = requests.post('http://localhost:8888', json=data.to_json())
print(response.request.body.decode('utf-8'))
"{"a":{"0":1,"1":2}}"
# this submits the actual json object
response = requests.post(
'http://localhost:8888',
data=data.to_json().encode('utf-8'),
headers={'Content-Type': 'application/json'}
)
print(response.request.body.decode('utf-8')
{"a":{"0":1,"1":2}}
I am not sure whether encoding is necessary.
I have been able to use json.loads(json_obj), which worked...
– Stan
Nov 16 '18 at 0:03
add a comment |
I think your problem is that pd.DataFrame.to_json
returns a string:
data = pd.DataFrame({'a': [1, 2]})
type(data.to_json())
str
but the json
keyword argument of requests.post
expects a python object. If you want to submit a son string, use the data=
argument instead:
# this submits a jsonified string
response = requests.post('http://localhost:8888', json=data.to_json())
print(response.request.body.decode('utf-8'))
"{"a":{"0":1,"1":2}}"
# this submits the actual json object
response = requests.post(
'http://localhost:8888',
data=data.to_json().encode('utf-8'),
headers={'Content-Type': 'application/json'}
)
print(response.request.body.decode('utf-8')
{"a":{"0":1,"1":2}}
I am not sure whether encoding is necessary.
I have been able to use json.loads(json_obj), which worked...
– Stan
Nov 16 '18 at 0:03
add a comment |
I think your problem is that pd.DataFrame.to_json
returns a string:
data = pd.DataFrame({'a': [1, 2]})
type(data.to_json())
str
but the json
keyword argument of requests.post
expects a python object. If you want to submit a son string, use the data=
argument instead:
# this submits a jsonified string
response = requests.post('http://localhost:8888', json=data.to_json())
print(response.request.body.decode('utf-8'))
"{"a":{"0":1,"1":2}}"
# this submits the actual json object
response = requests.post(
'http://localhost:8888',
data=data.to_json().encode('utf-8'),
headers={'Content-Type': 'application/json'}
)
print(response.request.body.decode('utf-8')
{"a":{"0":1,"1":2}}
I am not sure whether encoding is necessary.
I think your problem is that pd.DataFrame.to_json
returns a string:
data = pd.DataFrame({'a': [1, 2]})
type(data.to_json())
str
but the json
keyword argument of requests.post
expects a python object. If you want to submit a son string, use the data=
argument instead:
# this submits a jsonified string
response = requests.post('http://localhost:8888', json=data.to_json())
print(response.request.body.decode('utf-8'))
"{"a":{"0":1,"1":2}}"
# this submits the actual json object
response = requests.post(
'http://localhost:8888',
data=data.to_json().encode('utf-8'),
headers={'Content-Type': 'application/json'}
)
print(response.request.body.decode('utf-8')
{"a":{"0":1,"1":2}}
I am not sure whether encoding is necessary.
answered Nov 15 '18 at 23:49
Matthias OssadnikMatthias Ossadnik
60937
60937
I have been able to use json.loads(json_obj), which worked...
– Stan
Nov 16 '18 at 0:03
add a comment |
I have been able to use json.loads(json_obj), which worked...
– Stan
Nov 16 '18 at 0:03
I have been able to use json.loads(json_obj), which worked...
– Stan
Nov 16 '18 at 0:03
I have been able to use json.loads(json_obj), which worked...
– Stan
Nov 16 '18 at 0:03
add a comment |
You can take advantage of the simplejson
or json
packages:
import simplejson as json
response = requests.post('http://localhost:8888', data=json.loads(df.to_json(orient='records')),
headers={'Content-Type': 'application/json'}
)
Additionally, if you didn't want to orient as records you can use to_dict
instead of to_json
response = requests.post('http://localhost:8888', data=df.to_dict(),
headers={'Content-Type': 'application/json'}
)
The reason this works is because the data
argument in the requests
package accepts a string.
I have been able to use json.loads(json_obj), which worked...
– Stan
Nov 16 '18 at 0:04
@Stan yes, that's an alternative that fixes the problem as well
– Matthias Ossadnik
Nov 16 '18 at 0:04
Thank you !! I will try your one too.. Always better to have many ways
– Stan
Nov 16 '18 at 0:06
add a comment |
You can take advantage of the simplejson
or json
packages:
import simplejson as json
response = requests.post('http://localhost:8888', data=json.loads(df.to_json(orient='records')),
headers={'Content-Type': 'application/json'}
)
Additionally, if you didn't want to orient as records you can use to_dict
instead of to_json
response = requests.post('http://localhost:8888', data=df.to_dict(),
headers={'Content-Type': 'application/json'}
)
The reason this works is because the data
argument in the requests
package accepts a string.
I have been able to use json.loads(json_obj), which worked...
– Stan
Nov 16 '18 at 0:04
@Stan yes, that's an alternative that fixes the problem as well
– Matthias Ossadnik
Nov 16 '18 at 0:04
Thank you !! I will try your one too.. Always better to have many ways
– Stan
Nov 16 '18 at 0:06
add a comment |
You can take advantage of the simplejson
or json
packages:
import simplejson as json
response = requests.post('http://localhost:8888', data=json.loads(df.to_json(orient='records')),
headers={'Content-Type': 'application/json'}
)
Additionally, if you didn't want to orient as records you can use to_dict
instead of to_json
response = requests.post('http://localhost:8888', data=df.to_dict(),
headers={'Content-Type': 'application/json'}
)
The reason this works is because the data
argument in the requests
package accepts a string.
You can take advantage of the simplejson
or json
packages:
import simplejson as json
response = requests.post('http://localhost:8888', data=json.loads(df.to_json(orient='records')),
headers={'Content-Type': 'application/json'}
)
Additionally, if you didn't want to orient as records you can use to_dict
instead of to_json
response = requests.post('http://localhost:8888', data=df.to_dict(),
headers={'Content-Type': 'application/json'}
)
The reason this works is because the data
argument in the requests
package accepts a string.
answered Nov 15 '18 at 23:56
quantikquantik
531821
531821
I have been able to use json.loads(json_obj), which worked...
– Stan
Nov 16 '18 at 0:04
@Stan yes, that's an alternative that fixes the problem as well
– Matthias Ossadnik
Nov 16 '18 at 0:04
Thank you !! I will try your one too.. Always better to have many ways
– Stan
Nov 16 '18 at 0:06
add a comment |
I have been able to use json.loads(json_obj), which worked...
– Stan
Nov 16 '18 at 0:04
@Stan yes, that's an alternative that fixes the problem as well
– Matthias Ossadnik
Nov 16 '18 at 0:04
Thank you !! I will try your one too.. Always better to have many ways
– Stan
Nov 16 '18 at 0:06
I have been able to use json.loads(json_obj), which worked...
– Stan
Nov 16 '18 at 0:04
I have been able to use json.loads(json_obj), which worked...
– Stan
Nov 16 '18 at 0:04
@Stan yes, that's an alternative that fixes the problem as well
– Matthias Ossadnik
Nov 16 '18 at 0:04
@Stan yes, that's an alternative that fixes the problem as well
– Matthias Ossadnik
Nov 16 '18 at 0:04
Thank you !! I will try your one too.. Always better to have many ways
– Stan
Nov 16 '18 at 0:06
Thank you !! I will try your one too.. Always better to have many ways
– Stan
Nov 16 '18 at 0:06
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53329189%2fpost-requests-in-python-for-json-objects%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9,RZoK 6,Z,CT KduKPa4VFbRLa9 Yqb,BoVH UrZ5qsnHFroWY