How to convert Java assignment expression to Kotlin
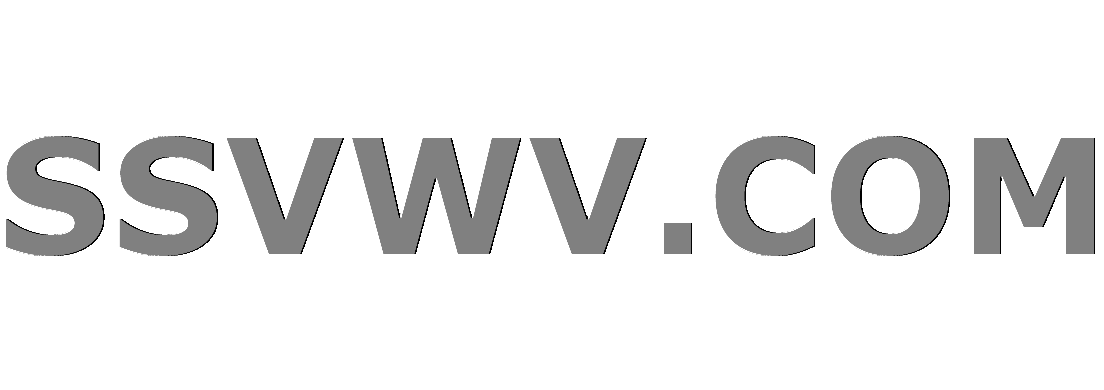
Multi tool use
Something in java like
int a = 1, b = 2, c = 1;
if ((a = b) !=c){
System.out.print(true);
}
now it should be converted to kotlin like
var a:Int? = 1
var b:Int? = 2
var c:Int? = 1
if ( (a = b) != c)
print(true)
but it's not correct.
Here is the error I get:
in " (a=b)" Error:(99, 9) Kotlin: Assignments are not expressions, and only expressions are allowed in this context
Actually the code above is just an example to clarify the problem. Here is my original code:
fun readFile(path: String): Unit {
var input: InputStream = FileInputStream(path)
var string: String = ""
var tmp: Int = -1
var bytes: ByteArray = ByteArray(1024)
while((tmp=input.read(bytes))!=-1) { }
}
java kotlin
add a comment |
Something in java like
int a = 1, b = 2, c = 1;
if ((a = b) !=c){
System.out.print(true);
}
now it should be converted to kotlin like
var a:Int? = 1
var b:Int? = 2
var c:Int? = 1
if ( (a = b) != c)
print(true)
but it's not correct.
Here is the error I get:
in " (a=b)" Error:(99, 9) Kotlin: Assignments are not expressions, and only expressions are allowed in this context
Actually the code above is just an example to clarify the problem. Here is my original code:
fun readFile(path: String): Unit {
var input: InputStream = FileInputStream(path)
var string: String = ""
var tmp: Int = -1
var bytes: ByteArray = ByteArray(1024)
while((tmp=input.read(bytes))!=-1) { }
}
java kotlin
What error (or errors) are you getting?
– Jay Elston
Apr 27 '16 at 2:44
in " (a=b)" Error:(99, 9) Kotlin: Assignments are not expressions, and only expressions are allowed in this context
– K.K Song
Apr 27 '16 at 3:01
add a comment |
Something in java like
int a = 1, b = 2, c = 1;
if ((a = b) !=c){
System.out.print(true);
}
now it should be converted to kotlin like
var a:Int? = 1
var b:Int? = 2
var c:Int? = 1
if ( (a = b) != c)
print(true)
but it's not correct.
Here is the error I get:
in " (a=b)" Error:(99, 9) Kotlin: Assignments are not expressions, and only expressions are allowed in this context
Actually the code above is just an example to clarify the problem. Here is my original code:
fun readFile(path: String): Unit {
var input: InputStream = FileInputStream(path)
var string: String = ""
var tmp: Int = -1
var bytes: ByteArray = ByteArray(1024)
while((tmp=input.read(bytes))!=-1) { }
}
java kotlin
Something in java like
int a = 1, b = 2, c = 1;
if ((a = b) !=c){
System.out.print(true);
}
now it should be converted to kotlin like
var a:Int? = 1
var b:Int? = 2
var c:Int? = 1
if ( (a = b) != c)
print(true)
but it's not correct.
Here is the error I get:
in " (a=b)" Error:(99, 9) Kotlin: Assignments are not expressions, and only expressions are allowed in this context
Actually the code above is just an example to clarify the problem. Here is my original code:
fun readFile(path: String): Unit {
var input: InputStream = FileInputStream(path)
var string: String = ""
var tmp: Int = -1
var bytes: ByteArray = ByteArray(1024)
while((tmp=input.read(bytes))!=-1) { }
}
java kotlin
java kotlin
edited Apr 27 '16 at 11:57


hotkey
65.2k14186205
65.2k14186205
asked Apr 27 '16 at 2:31
K.K SongK.K Song
12317
12317
What error (or errors) are you getting?
– Jay Elston
Apr 27 '16 at 2:44
in " (a=b)" Error:(99, 9) Kotlin: Assignments are not expressions, and only expressions are allowed in this context
– K.K Song
Apr 27 '16 at 3:01
add a comment |
What error (or errors) are you getting?
– Jay Elston
Apr 27 '16 at 2:44
in " (a=b)" Error:(99, 9) Kotlin: Assignments are not expressions, and only expressions are allowed in this context
– K.K Song
Apr 27 '16 at 3:01
What error (or errors) are you getting?
– Jay Elston
Apr 27 '16 at 2:44
What error (or errors) are you getting?
– Jay Elston
Apr 27 '16 at 2:44
in " (a=b)" Error:(99, 9) Kotlin: Assignments are not expressions, and only expressions are allowed in this context
– K.K Song
Apr 27 '16 at 3:01
in " (a=b)" Error:(99, 9) Kotlin: Assignments are not expressions, and only expressions are allowed in this context
– K.K Song
Apr 27 '16 at 3:01
add a comment |
6 Answers
6
active
oldest
votes
As @AndroidEx correctly stated, assignments are not expressions in Kotlin, unlike Java. The reason is that expressions with side effects are generally discouraged. See this discussion on a similar topic.
One solution is just to split the expression and move the assignment out of condition block:
a = b
if (a != c) { ... }
Another one is to use functions from stdlib like let
, which executes the lambda with the receiver as parameter and returns the lambda result. apply
and run
have similar semantics.
if (b.let { a = it; it != c }) { ... }
if (run { a = b; b != c }) { ... }
Thanks to inlining, this will be as efficient as plain code taken from the lambda.
Your example with InputStream
would look like
while (input.read(bytes).let { tmp = it; it != -1 }) { ... }
Also, consider readBytes
function for reading a ByteArray
from an InputStream
.
add a comment |
Assignments are not expressions in Kotlin, thus you'll need to do it outside:
var a: Int? = 1
var b: Int? = 2
var c: Int? = 1
a = b
if (a != c)
print(true)
For your other example with InputStream
you could do:
fun readFile(path: String) {
val input: InputStream = FileInputStream(path)
input.reader().forEachLine {
print(it)
}
}
im try to use i/o stream, so i have to put a=b in if() or while(), how should i do
– K.K Song
Apr 27 '16 at 3:18
sorry, here is my original codefun readFile(path: String): Unit { var input: InputStream = FileInputStream(path) var string: String = "" var tmp: Int = -1 var bytes: ByteArray = ByteArray(1024) while((tmp=input.read(bytes))!=-1){ } }
– K.K Song
Apr 27 '16 at 3:23
@K.KSong edited my answer
– AndroidEx
Apr 27 '16 at 3:27
1
Note:reader
andforEachLine
are not quite suitable for all the cases of an arbitrary byte stream, at least not with default (thus undefined) encoding which might not be single byte one and can fail at decoding the bytes.InputStream::readBytes
is better for that.
– hotkey
Apr 27 '16 at 11:37
Thank you, it worked for me!
– hetsgandhi
Aug 2 '18 at 8:07
add a comment |
As pretty much everyone here has pointed out, assignments are not expressions in Kotlin. However, we can coerce the assignment into an expression using a function literal:
val reader = Files.newBufferedReader(path)
var line: String? = null
while ({ line = reader.readLine(); line }() != null) {
println(line);
}
add a comment |
Java: (a = b) != c
Kotlin: b.also { a = it } != c
Around OP's question:
Unlike the accepted answer, I suggest using Kotlin's also
function, instead of let
:
while (input.read(bytes).also { tmp = it } != -1) { ...
Because T.also
returns T
(it
) itself and then you can compare it with -1
. This is more similar to Java's assignment as a statement.
See "Return this
vs. other type" section, on this useful blog for details.
add a comment |
I think this may help you:
input.buffered(1024).reader().forEachLine {
fos.bufferedWriter().write(it)
}
add a comment |
the simple way in kotlin is
if (kotlin.run{ a=b; a != c}){ ... }
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f36879236%2fhow-to-convert-java-assignment-expression-to-kotlin%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
As @AndroidEx correctly stated, assignments are not expressions in Kotlin, unlike Java. The reason is that expressions with side effects are generally discouraged. See this discussion on a similar topic.
One solution is just to split the expression and move the assignment out of condition block:
a = b
if (a != c) { ... }
Another one is to use functions from stdlib like let
, which executes the lambda with the receiver as parameter and returns the lambda result. apply
and run
have similar semantics.
if (b.let { a = it; it != c }) { ... }
if (run { a = b; b != c }) { ... }
Thanks to inlining, this will be as efficient as plain code taken from the lambda.
Your example with InputStream
would look like
while (input.read(bytes).let { tmp = it; it != -1 }) { ... }
Also, consider readBytes
function for reading a ByteArray
from an InputStream
.
add a comment |
As @AndroidEx correctly stated, assignments are not expressions in Kotlin, unlike Java. The reason is that expressions with side effects are generally discouraged. See this discussion on a similar topic.
One solution is just to split the expression and move the assignment out of condition block:
a = b
if (a != c) { ... }
Another one is to use functions from stdlib like let
, which executes the lambda with the receiver as parameter and returns the lambda result. apply
and run
have similar semantics.
if (b.let { a = it; it != c }) { ... }
if (run { a = b; b != c }) { ... }
Thanks to inlining, this will be as efficient as plain code taken from the lambda.
Your example with InputStream
would look like
while (input.read(bytes).let { tmp = it; it != -1 }) { ... }
Also, consider readBytes
function for reading a ByteArray
from an InputStream
.
add a comment |
As @AndroidEx correctly stated, assignments are not expressions in Kotlin, unlike Java. The reason is that expressions with side effects are generally discouraged. See this discussion on a similar topic.
One solution is just to split the expression and move the assignment out of condition block:
a = b
if (a != c) { ... }
Another one is to use functions from stdlib like let
, which executes the lambda with the receiver as parameter and returns the lambda result. apply
and run
have similar semantics.
if (b.let { a = it; it != c }) { ... }
if (run { a = b; b != c }) { ... }
Thanks to inlining, this will be as efficient as plain code taken from the lambda.
Your example with InputStream
would look like
while (input.read(bytes).let { tmp = it; it != -1 }) { ... }
Also, consider readBytes
function for reading a ByteArray
from an InputStream
.
As @AndroidEx correctly stated, assignments are not expressions in Kotlin, unlike Java. The reason is that expressions with side effects are generally discouraged. See this discussion on a similar topic.
One solution is just to split the expression and move the assignment out of condition block:
a = b
if (a != c) { ... }
Another one is to use functions from stdlib like let
, which executes the lambda with the receiver as parameter and returns the lambda result. apply
and run
have similar semantics.
if (b.let { a = it; it != c }) { ... }
if (run { a = b; b != c }) { ... }
Thanks to inlining, this will be as efficient as plain code taken from the lambda.
Your example with InputStream
would look like
while (input.read(bytes).let { tmp = it; it != -1 }) { ... }
Also, consider readBytes
function for reading a ByteArray
from an InputStream
.
edited Apr 27 '16 at 15:26
answered Apr 27 '16 at 10:50


hotkeyhotkey
65.2k14186205
65.2k14186205
add a comment |
add a comment |
Assignments are not expressions in Kotlin, thus you'll need to do it outside:
var a: Int? = 1
var b: Int? = 2
var c: Int? = 1
a = b
if (a != c)
print(true)
For your other example with InputStream
you could do:
fun readFile(path: String) {
val input: InputStream = FileInputStream(path)
input.reader().forEachLine {
print(it)
}
}
im try to use i/o stream, so i have to put a=b in if() or while(), how should i do
– K.K Song
Apr 27 '16 at 3:18
sorry, here is my original codefun readFile(path: String): Unit { var input: InputStream = FileInputStream(path) var string: String = "" var tmp: Int = -1 var bytes: ByteArray = ByteArray(1024) while((tmp=input.read(bytes))!=-1){ } }
– K.K Song
Apr 27 '16 at 3:23
@K.KSong edited my answer
– AndroidEx
Apr 27 '16 at 3:27
1
Note:reader
andforEachLine
are not quite suitable for all the cases of an arbitrary byte stream, at least not with default (thus undefined) encoding which might not be single byte one and can fail at decoding the bytes.InputStream::readBytes
is better for that.
– hotkey
Apr 27 '16 at 11:37
Thank you, it worked for me!
– hetsgandhi
Aug 2 '18 at 8:07
add a comment |
Assignments are not expressions in Kotlin, thus you'll need to do it outside:
var a: Int? = 1
var b: Int? = 2
var c: Int? = 1
a = b
if (a != c)
print(true)
For your other example with InputStream
you could do:
fun readFile(path: String) {
val input: InputStream = FileInputStream(path)
input.reader().forEachLine {
print(it)
}
}
im try to use i/o stream, so i have to put a=b in if() or while(), how should i do
– K.K Song
Apr 27 '16 at 3:18
sorry, here is my original codefun readFile(path: String): Unit { var input: InputStream = FileInputStream(path) var string: String = "" var tmp: Int = -1 var bytes: ByteArray = ByteArray(1024) while((tmp=input.read(bytes))!=-1){ } }
– K.K Song
Apr 27 '16 at 3:23
@K.KSong edited my answer
– AndroidEx
Apr 27 '16 at 3:27
1
Note:reader
andforEachLine
are not quite suitable for all the cases of an arbitrary byte stream, at least not with default (thus undefined) encoding which might not be single byte one and can fail at decoding the bytes.InputStream::readBytes
is better for that.
– hotkey
Apr 27 '16 at 11:37
Thank you, it worked for me!
– hetsgandhi
Aug 2 '18 at 8:07
add a comment |
Assignments are not expressions in Kotlin, thus you'll need to do it outside:
var a: Int? = 1
var b: Int? = 2
var c: Int? = 1
a = b
if (a != c)
print(true)
For your other example with InputStream
you could do:
fun readFile(path: String) {
val input: InputStream = FileInputStream(path)
input.reader().forEachLine {
print(it)
}
}
Assignments are not expressions in Kotlin, thus you'll need to do it outside:
var a: Int? = 1
var b: Int? = 2
var c: Int? = 1
a = b
if (a != c)
print(true)
For your other example with InputStream
you could do:
fun readFile(path: String) {
val input: InputStream = FileInputStream(path)
input.reader().forEachLine {
print(it)
}
}
edited May 23 '17 at 10:30
Community♦
11
11
answered Apr 27 '16 at 3:01
AndroidExAndroidEx
10.1k64249
10.1k64249
im try to use i/o stream, so i have to put a=b in if() or while(), how should i do
– K.K Song
Apr 27 '16 at 3:18
sorry, here is my original codefun readFile(path: String): Unit { var input: InputStream = FileInputStream(path) var string: String = "" var tmp: Int = -1 var bytes: ByteArray = ByteArray(1024) while((tmp=input.read(bytes))!=-1){ } }
– K.K Song
Apr 27 '16 at 3:23
@K.KSong edited my answer
– AndroidEx
Apr 27 '16 at 3:27
1
Note:reader
andforEachLine
are not quite suitable for all the cases of an arbitrary byte stream, at least not with default (thus undefined) encoding which might not be single byte one and can fail at decoding the bytes.InputStream::readBytes
is better for that.
– hotkey
Apr 27 '16 at 11:37
Thank you, it worked for me!
– hetsgandhi
Aug 2 '18 at 8:07
add a comment |
im try to use i/o stream, so i have to put a=b in if() or while(), how should i do
– K.K Song
Apr 27 '16 at 3:18
sorry, here is my original codefun readFile(path: String): Unit { var input: InputStream = FileInputStream(path) var string: String = "" var tmp: Int = -1 var bytes: ByteArray = ByteArray(1024) while((tmp=input.read(bytes))!=-1){ } }
– K.K Song
Apr 27 '16 at 3:23
@K.KSong edited my answer
– AndroidEx
Apr 27 '16 at 3:27
1
Note:reader
andforEachLine
are not quite suitable for all the cases of an arbitrary byte stream, at least not with default (thus undefined) encoding which might not be single byte one and can fail at decoding the bytes.InputStream::readBytes
is better for that.
– hotkey
Apr 27 '16 at 11:37
Thank you, it worked for me!
– hetsgandhi
Aug 2 '18 at 8:07
im try to use i/o stream, so i have to put a=b in if() or while(), how should i do
– K.K Song
Apr 27 '16 at 3:18
im try to use i/o stream, so i have to put a=b in if() or while(), how should i do
– K.K Song
Apr 27 '16 at 3:18
sorry, here is my original code
fun readFile(path: String): Unit { var input: InputStream = FileInputStream(path) var string: String = "" var tmp: Int = -1 var bytes: ByteArray = ByteArray(1024) while((tmp=input.read(bytes))!=-1){ } }
– K.K Song
Apr 27 '16 at 3:23
sorry, here is my original code
fun readFile(path: String): Unit { var input: InputStream = FileInputStream(path) var string: String = "" var tmp: Int = -1 var bytes: ByteArray = ByteArray(1024) while((tmp=input.read(bytes))!=-1){ } }
– K.K Song
Apr 27 '16 at 3:23
@K.KSong edited my answer
– AndroidEx
Apr 27 '16 at 3:27
@K.KSong edited my answer
– AndroidEx
Apr 27 '16 at 3:27
1
1
Note:
reader
and forEachLine
are not quite suitable for all the cases of an arbitrary byte stream, at least not with default (thus undefined) encoding which might not be single byte one and can fail at decoding the bytes. InputStream::readBytes
is better for that.– hotkey
Apr 27 '16 at 11:37
Note:
reader
and forEachLine
are not quite suitable for all the cases of an arbitrary byte stream, at least not with default (thus undefined) encoding which might not be single byte one and can fail at decoding the bytes. InputStream::readBytes
is better for that.– hotkey
Apr 27 '16 at 11:37
Thank you, it worked for me!
– hetsgandhi
Aug 2 '18 at 8:07
Thank you, it worked for me!
– hetsgandhi
Aug 2 '18 at 8:07
add a comment |
As pretty much everyone here has pointed out, assignments are not expressions in Kotlin. However, we can coerce the assignment into an expression using a function literal:
val reader = Files.newBufferedReader(path)
var line: String? = null
while ({ line = reader.readLine(); line }() != null) {
println(line);
}
add a comment |
As pretty much everyone here has pointed out, assignments are not expressions in Kotlin. However, we can coerce the assignment into an expression using a function literal:
val reader = Files.newBufferedReader(path)
var line: String? = null
while ({ line = reader.readLine(); line }() != null) {
println(line);
}
add a comment |
As pretty much everyone here has pointed out, assignments are not expressions in Kotlin. However, we can coerce the assignment into an expression using a function literal:
val reader = Files.newBufferedReader(path)
var line: String? = null
while ({ line = reader.readLine(); line }() != null) {
println(line);
}
As pretty much everyone here has pointed out, assignments are not expressions in Kotlin. However, we can coerce the assignment into an expression using a function literal:
val reader = Files.newBufferedReader(path)
var line: String? = null
while ({ line = reader.readLine(); line }() != null) {
println(line);
}
answered Jul 11 '16 at 5:15
w.brianw.brian
7,25374280
7,25374280
add a comment |
add a comment |
Java: (a = b) != c
Kotlin: b.also { a = it } != c
Around OP's question:
Unlike the accepted answer, I suggest using Kotlin's also
function, instead of let
:
while (input.read(bytes).also { tmp = it } != -1) { ...
Because T.also
returns T
(it
) itself and then you can compare it with -1
. This is more similar to Java's assignment as a statement.
See "Return this
vs. other type" section, on this useful blog for details.
add a comment |
Java: (a = b) != c
Kotlin: b.also { a = it } != c
Around OP's question:
Unlike the accepted answer, I suggest using Kotlin's also
function, instead of let
:
while (input.read(bytes).also { tmp = it } != -1) { ...
Because T.also
returns T
(it
) itself and then you can compare it with -1
. This is more similar to Java's assignment as a statement.
See "Return this
vs. other type" section, on this useful blog for details.
add a comment |
Java: (a = b) != c
Kotlin: b.also { a = it } != c
Around OP's question:
Unlike the accepted answer, I suggest using Kotlin's also
function, instead of let
:
while (input.read(bytes).also { tmp = it } != -1) { ...
Because T.also
returns T
(it
) itself and then you can compare it with -1
. This is more similar to Java's assignment as a statement.
See "Return this
vs. other type" section, on this useful blog for details.
Java: (a = b) != c
Kotlin: b.also { a = it } != c
Around OP's question:
Unlike the accepted answer, I suggest using Kotlin's also
function, instead of let
:
while (input.read(bytes).also { tmp = it } != -1) { ...
Because T.also
returns T
(it
) itself and then you can compare it with -1
. This is more similar to Java's assignment as a statement.
See "Return this
vs. other type" section, on this useful blog for details.
edited Mar 11 at 4:37
answered Nov 15 '18 at 22:46


Mir-IsmailiMir-Ismaili
2,4791740
2,4791740
add a comment |
add a comment |
I think this may help you:
input.buffered(1024).reader().forEachLine {
fos.bufferedWriter().write(it)
}
add a comment |
I think this may help you:
input.buffered(1024).reader().forEachLine {
fos.bufferedWriter().write(it)
}
add a comment |
I think this may help you:
input.buffered(1024).reader().forEachLine {
fos.bufferedWriter().write(it)
}
I think this may help you:
input.buffered(1024).reader().forEachLine {
fos.bufferedWriter().write(it)
}
answered Jul 14 '17 at 10:52
user2944157user2944157
12
12
add a comment |
add a comment |
the simple way in kotlin is
if (kotlin.run{ a=b; a != c}){ ... }
add a comment |
the simple way in kotlin is
if (kotlin.run{ a=b; a != c}){ ... }
add a comment |
the simple way in kotlin is
if (kotlin.run{ a=b; a != c}){ ... }
the simple way in kotlin is
if (kotlin.run{ a=b; a != c}){ ... }
answered Mar 21 '18 at 13:39


Ara HakobyanAra Hakobyan
864416
864416
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f36879236%2fhow-to-convert-java-assignment-expression-to-kotlin%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
enwVBJqBdEqDN0eu8ARq2TxnoWYfAMAj,I,gvc 25udNMn0V o,VE9c
What error (or errors) are you getting?
– Jay Elston
Apr 27 '16 at 2:44
in " (a=b)" Error:(99, 9) Kotlin: Assignments are not expressions, and only expressions are allowed in this context
– K.K Song
Apr 27 '16 at 3:01