Make Bean depend on list of beans in springboot
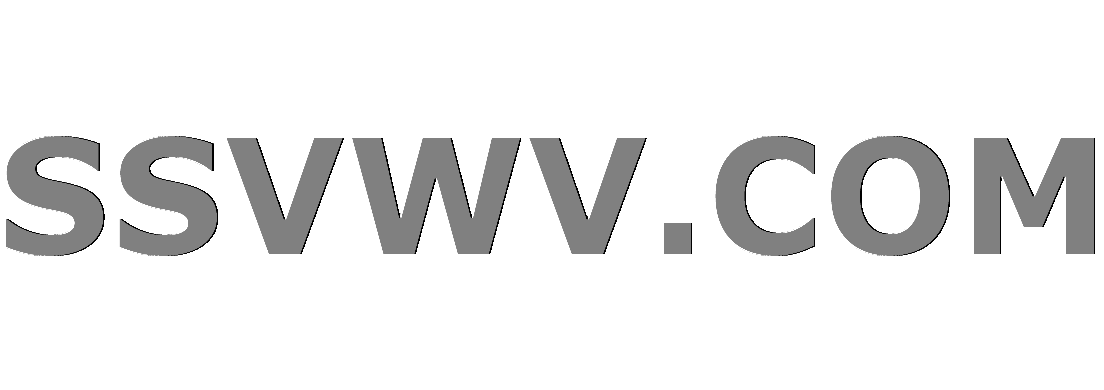
Multi tool use
I have following @Configuration
class, in which I am declaring a @Bean that depends on an @Autowired
list of beans. However, this list is not complete when I am accessing to it. All @Bean
definitions been executed, except the one defined in the same class.
@Configuration
public class MyConfig {
@Autowired
List<RequiredBean> requiredBeans;
@Bean(name="ProblemHere")
public CustomObject customObject() {
log.info(requiredBeans.size()); // 1 (debugging, I can see is the one defined in "AnotherConfigClass")
}
@Bean(name="reqBeanInsideClass")
public RequiredBean reqBean() {
// this method does not get executed
return new RequiredBean();
}
}
Having other classes like;
@Configuration
public class AnotherConfigClass {
@Bean(name="ThisOneGetsExecuted")
public RequiredBean reqBean() {
// this gets executed, and therefore, added to the list
return new RequiredBean();
}
}
Probably, the easiest solution would be to add @DependsOn("reqBeanInsideClass").
However:
- I wonder why it works for all
@Bean
s defined in different classes, but not in this one. - I'm not really sure that's exactly like that, and I'm afraid later on, another
@Bean
does not get executed
I guess the correct approach should be something like
@DependsOn(List<RequiredBean>) // Obviously this does not work
How should I solve this?
Update
I have copied the exact same class twice, in order to see what would happen, so now I have also:
@Configuration
public class MyConfig2 {
@Autowired
List<RequiredBean> requiredBeans;
@Bean(name="ProblemHere2")
public CustomObject customObject() {
log.info(requiredBeans.size());
}
@Bean(name="reqBeanInsideClass2")
public RequiredBean reqBean() {
// this method does not get executed
return new RequiredBean();
}
}
Amazingly, by doing this, both @Beans
methods (ProblemHere
& ProblemHere2
) are called before both reqBeanInsideClass
and reqBeanInsideClass2
methods.
For some reason I guess, Springboot is able to recognize @Beans
required for a class as long as they are defined in another class.
- Does this sound logic to anyone?
java spring spring-boot spring-config
add a comment |
I have following @Configuration
class, in which I am declaring a @Bean that depends on an @Autowired
list of beans. However, this list is not complete when I am accessing to it. All @Bean
definitions been executed, except the one defined in the same class.
@Configuration
public class MyConfig {
@Autowired
List<RequiredBean> requiredBeans;
@Bean(name="ProblemHere")
public CustomObject customObject() {
log.info(requiredBeans.size()); // 1 (debugging, I can see is the one defined in "AnotherConfigClass")
}
@Bean(name="reqBeanInsideClass")
public RequiredBean reqBean() {
// this method does not get executed
return new RequiredBean();
}
}
Having other classes like;
@Configuration
public class AnotherConfigClass {
@Bean(name="ThisOneGetsExecuted")
public RequiredBean reqBean() {
// this gets executed, and therefore, added to the list
return new RequiredBean();
}
}
Probably, the easiest solution would be to add @DependsOn("reqBeanInsideClass").
However:
- I wonder why it works for all
@Bean
s defined in different classes, but not in this one. - I'm not really sure that's exactly like that, and I'm afraid later on, another
@Bean
does not get executed
I guess the correct approach should be something like
@DependsOn(List<RequiredBean>) // Obviously this does not work
How should I solve this?
Update
I have copied the exact same class twice, in order to see what would happen, so now I have also:
@Configuration
public class MyConfig2 {
@Autowired
List<RequiredBean> requiredBeans;
@Bean(name="ProblemHere2")
public CustomObject customObject() {
log.info(requiredBeans.size());
}
@Bean(name="reqBeanInsideClass2")
public RequiredBean reqBean() {
// this method does not get executed
return new RequiredBean();
}
}
Amazingly, by doing this, both @Beans
methods (ProblemHere
& ProblemHere2
) are called before both reqBeanInsideClass
and reqBeanInsideClass2
methods.
For some reason I guess, Springboot is able to recognize @Beans
required for a class as long as they are defined in another class.
- Does this sound logic to anyone?
java spring spring-boot spring-config
🙋🏻♂️ you are declaring beans but no where adding to arraylist, even how does that list have size 1?
– Deadpool
Nov 16 '18 at 9:51
@Deadpool Probably because randomly other Beans get initialized first? I'm not sure, thats why I am asking which is the correct way to make this dependency
– Mayday
Nov 16 '18 at 9:53
add a comment |
I have following @Configuration
class, in which I am declaring a @Bean that depends on an @Autowired
list of beans. However, this list is not complete when I am accessing to it. All @Bean
definitions been executed, except the one defined in the same class.
@Configuration
public class MyConfig {
@Autowired
List<RequiredBean> requiredBeans;
@Bean(name="ProblemHere")
public CustomObject customObject() {
log.info(requiredBeans.size()); // 1 (debugging, I can see is the one defined in "AnotherConfigClass")
}
@Bean(name="reqBeanInsideClass")
public RequiredBean reqBean() {
// this method does not get executed
return new RequiredBean();
}
}
Having other classes like;
@Configuration
public class AnotherConfigClass {
@Bean(name="ThisOneGetsExecuted")
public RequiredBean reqBean() {
// this gets executed, and therefore, added to the list
return new RequiredBean();
}
}
Probably, the easiest solution would be to add @DependsOn("reqBeanInsideClass").
However:
- I wonder why it works for all
@Bean
s defined in different classes, but not in this one. - I'm not really sure that's exactly like that, and I'm afraid later on, another
@Bean
does not get executed
I guess the correct approach should be something like
@DependsOn(List<RequiredBean>) // Obviously this does not work
How should I solve this?
Update
I have copied the exact same class twice, in order to see what would happen, so now I have also:
@Configuration
public class MyConfig2 {
@Autowired
List<RequiredBean> requiredBeans;
@Bean(name="ProblemHere2")
public CustomObject customObject() {
log.info(requiredBeans.size());
}
@Bean(name="reqBeanInsideClass2")
public RequiredBean reqBean() {
// this method does not get executed
return new RequiredBean();
}
}
Amazingly, by doing this, both @Beans
methods (ProblemHere
& ProblemHere2
) are called before both reqBeanInsideClass
and reqBeanInsideClass2
methods.
For some reason I guess, Springboot is able to recognize @Beans
required for a class as long as they are defined in another class.
- Does this sound logic to anyone?
java spring spring-boot spring-config
I have following @Configuration
class, in which I am declaring a @Bean that depends on an @Autowired
list of beans. However, this list is not complete when I am accessing to it. All @Bean
definitions been executed, except the one defined in the same class.
@Configuration
public class MyConfig {
@Autowired
List<RequiredBean> requiredBeans;
@Bean(name="ProblemHere")
public CustomObject customObject() {
log.info(requiredBeans.size()); // 1 (debugging, I can see is the one defined in "AnotherConfigClass")
}
@Bean(name="reqBeanInsideClass")
public RequiredBean reqBean() {
// this method does not get executed
return new RequiredBean();
}
}
Having other classes like;
@Configuration
public class AnotherConfigClass {
@Bean(name="ThisOneGetsExecuted")
public RequiredBean reqBean() {
// this gets executed, and therefore, added to the list
return new RequiredBean();
}
}
Probably, the easiest solution would be to add @DependsOn("reqBeanInsideClass").
However:
- I wonder why it works for all
@Bean
s defined in different classes, but not in this one. - I'm not really sure that's exactly like that, and I'm afraid later on, another
@Bean
does not get executed
I guess the correct approach should be something like
@DependsOn(List<RequiredBean>) // Obviously this does not work
How should I solve this?
Update
I have copied the exact same class twice, in order to see what would happen, so now I have also:
@Configuration
public class MyConfig2 {
@Autowired
List<RequiredBean> requiredBeans;
@Bean(name="ProblemHere2")
public CustomObject customObject() {
log.info(requiredBeans.size());
}
@Bean(name="reqBeanInsideClass2")
public RequiredBean reqBean() {
// this method does not get executed
return new RequiredBean();
}
}
Amazingly, by doing this, both @Beans
methods (ProblemHere
& ProblemHere2
) are called before both reqBeanInsideClass
and reqBeanInsideClass2
methods.
For some reason I guess, Springboot is able to recognize @Beans
required for a class as long as they are defined in another class.
- Does this sound logic to anyone?
java spring spring-boot spring-config
java spring spring-boot spring-config
edited Nov 16 '18 at 13:58
Mayday
asked Nov 16 '18 at 9:45
MaydayMayday
1,6271827
1,6271827
🙋🏻♂️ you are declaring beans but no where adding to arraylist, even how does that list have size 1?
– Deadpool
Nov 16 '18 at 9:51
@Deadpool Probably because randomly other Beans get initialized first? I'm not sure, thats why I am asking which is the correct way to make this dependency
– Mayday
Nov 16 '18 at 9:53
add a comment |
🙋🏻♂️ you are declaring beans but no where adding to arraylist, even how does that list have size 1?
– Deadpool
Nov 16 '18 at 9:51
@Deadpool Probably because randomly other Beans get initialized first? I'm not sure, thats why I am asking which is the correct way to make this dependency
– Mayday
Nov 16 '18 at 9:53
🙋🏻♂️ you are declaring beans but no where adding to arraylist, even how does that list have size 1?
– Deadpool
Nov 16 '18 at 9:51
🙋🏻♂️ you are declaring beans but no where adding to arraylist, even how does that list have size 1?
– Deadpool
Nov 16 '18 at 9:51
@Deadpool Probably because randomly other Beans get initialized first? I'm not sure, thats why I am asking which is the correct way to make this dependency
– Mayday
Nov 16 '18 at 9:53
@Deadpool Probably because randomly other Beans get initialized first? I'm not sure, thats why I am asking which is the correct way to make this dependency
– Mayday
Nov 16 '18 at 9:53
add a comment |
1 Answer
1
active
oldest
votes
Can you not utilize the array input for @DependsOn
rather than passing singular value, since it accepts String
? That would wait for all the beans that are explicitly declared in the array before initializing, though has to be defined manually.
@Configuration
public class MyConfig {
@Autowired
List<RequiredBean> requiredBeans;
@Bean(name="customObject")
@DependsOn({"reqBeanInsideClass", "thisOneGetsExecuted"})
public CustomObject customObject() {
log.info(requiredBeans.size());
}
@Bean(name="reqBeanInsideClass")
public RequiredBean reqBean() {
return new RequiredBean();
}
}
@Autowired
list of beans will be same as a single bean of same type, it will contain all beans with that type or with that superclass via springs injection, the problem is the ordering of bean initialization is not controlled properly, @DependsOn
with array bean input should resolve this!
Or
You can make CustomObject
bean @Lazy
, so it will be initialized only when it is used within the code after initialization is done. The bean must not be used within another non-lazy bean I think. Just call some logic where an @Autowired
CustomObject
is used, it should instantiate the bean at that moment, where the list will contain all possible RequiredBean
s
@Lazy
@Bean(name="customObject")
public CustomObject customObject() {
log.info(requiredBeans.size());
}
I could do that, but I would love to automatically just declare new @Beans of type RequiredBean in different parts of application, and not having to get worried about specifying them 1 by 1 every time I add a new one
– Mayday
Nov 16 '18 at 10:33
no, still not working. Probably because it is being required early on. (I do not really have control on when "CustomObject" gets executed, since it is related with JPA, to create datasources etc. Actually, "CustomObject" is just to make the sample easier to understand, but in my project is "LocalContainerEntityManagerFactoryBean") However, if I take the inside class Bean into another class, it always seems to work. I just can not understand why :/
– Mayday
Nov 16 '18 at 10:49
Oh, ifCustomObject
is a factory, then it has to be processed during the initialization, you have to utilize@DependsOn
with explicit beans, if possible you can try to put them all in a single class and put theCustomObject
at the bottom, the order is why yourRequiredBean
is not picked up I think. Or how about putting@Order
on your@Configuration
classes? Put a very high number on the one withCustomObject
bean, and any new one you add, use1, 2, 3, ...
, if you have access to those configuration source code of course, not sure if@Order
would work on@Configuration
though
– buræquete
Nov 16 '18 at 10:58
If I move thereqBeanInsideClass
and write it into anther config class, then it works :)
– Mayday
Nov 16 '18 at 11:57
1
Yes, I might need to go for that solution. It just sounded to me logic that springboot is able to inject properly a list of dependencies by having all the components scan defined. But maybe in future versions heh. Thank you very much for your time :)
– Mayday
Nov 16 '18 at 14:13
|
show 7 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53335191%2fmake-bean-depend-on-list-of-beans-in-springboot%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Can you not utilize the array input for @DependsOn
rather than passing singular value, since it accepts String
? That would wait for all the beans that are explicitly declared in the array before initializing, though has to be defined manually.
@Configuration
public class MyConfig {
@Autowired
List<RequiredBean> requiredBeans;
@Bean(name="customObject")
@DependsOn({"reqBeanInsideClass", "thisOneGetsExecuted"})
public CustomObject customObject() {
log.info(requiredBeans.size());
}
@Bean(name="reqBeanInsideClass")
public RequiredBean reqBean() {
return new RequiredBean();
}
}
@Autowired
list of beans will be same as a single bean of same type, it will contain all beans with that type or with that superclass via springs injection, the problem is the ordering of bean initialization is not controlled properly, @DependsOn
with array bean input should resolve this!
Or
You can make CustomObject
bean @Lazy
, so it will be initialized only when it is used within the code after initialization is done. The bean must not be used within another non-lazy bean I think. Just call some logic where an @Autowired
CustomObject
is used, it should instantiate the bean at that moment, where the list will contain all possible RequiredBean
s
@Lazy
@Bean(name="customObject")
public CustomObject customObject() {
log.info(requiredBeans.size());
}
I could do that, but I would love to automatically just declare new @Beans of type RequiredBean in different parts of application, and not having to get worried about specifying them 1 by 1 every time I add a new one
– Mayday
Nov 16 '18 at 10:33
no, still not working. Probably because it is being required early on. (I do not really have control on when "CustomObject" gets executed, since it is related with JPA, to create datasources etc. Actually, "CustomObject" is just to make the sample easier to understand, but in my project is "LocalContainerEntityManagerFactoryBean") However, if I take the inside class Bean into another class, it always seems to work. I just can not understand why :/
– Mayday
Nov 16 '18 at 10:49
Oh, ifCustomObject
is a factory, then it has to be processed during the initialization, you have to utilize@DependsOn
with explicit beans, if possible you can try to put them all in a single class and put theCustomObject
at the bottom, the order is why yourRequiredBean
is not picked up I think. Or how about putting@Order
on your@Configuration
classes? Put a very high number on the one withCustomObject
bean, and any new one you add, use1, 2, 3, ...
, if you have access to those configuration source code of course, not sure if@Order
would work on@Configuration
though
– buræquete
Nov 16 '18 at 10:58
If I move thereqBeanInsideClass
and write it into anther config class, then it works :)
– Mayday
Nov 16 '18 at 11:57
1
Yes, I might need to go for that solution. It just sounded to me logic that springboot is able to inject properly a list of dependencies by having all the components scan defined. But maybe in future versions heh. Thank you very much for your time :)
– Mayday
Nov 16 '18 at 14:13
|
show 7 more comments
Can you not utilize the array input for @DependsOn
rather than passing singular value, since it accepts String
? That would wait for all the beans that are explicitly declared in the array before initializing, though has to be defined manually.
@Configuration
public class MyConfig {
@Autowired
List<RequiredBean> requiredBeans;
@Bean(name="customObject")
@DependsOn({"reqBeanInsideClass", "thisOneGetsExecuted"})
public CustomObject customObject() {
log.info(requiredBeans.size());
}
@Bean(name="reqBeanInsideClass")
public RequiredBean reqBean() {
return new RequiredBean();
}
}
@Autowired
list of beans will be same as a single bean of same type, it will contain all beans with that type or with that superclass via springs injection, the problem is the ordering of bean initialization is not controlled properly, @DependsOn
with array bean input should resolve this!
Or
You can make CustomObject
bean @Lazy
, so it will be initialized only when it is used within the code after initialization is done. The bean must not be used within another non-lazy bean I think. Just call some logic where an @Autowired
CustomObject
is used, it should instantiate the bean at that moment, where the list will contain all possible RequiredBean
s
@Lazy
@Bean(name="customObject")
public CustomObject customObject() {
log.info(requiredBeans.size());
}
I could do that, but I would love to automatically just declare new @Beans of type RequiredBean in different parts of application, and not having to get worried about specifying them 1 by 1 every time I add a new one
– Mayday
Nov 16 '18 at 10:33
no, still not working. Probably because it is being required early on. (I do not really have control on when "CustomObject" gets executed, since it is related with JPA, to create datasources etc. Actually, "CustomObject" is just to make the sample easier to understand, but in my project is "LocalContainerEntityManagerFactoryBean") However, if I take the inside class Bean into another class, it always seems to work. I just can not understand why :/
– Mayday
Nov 16 '18 at 10:49
Oh, ifCustomObject
is a factory, then it has to be processed during the initialization, you have to utilize@DependsOn
with explicit beans, if possible you can try to put them all in a single class and put theCustomObject
at the bottom, the order is why yourRequiredBean
is not picked up I think. Or how about putting@Order
on your@Configuration
classes? Put a very high number on the one withCustomObject
bean, and any new one you add, use1, 2, 3, ...
, if you have access to those configuration source code of course, not sure if@Order
would work on@Configuration
though
– buræquete
Nov 16 '18 at 10:58
If I move thereqBeanInsideClass
and write it into anther config class, then it works :)
– Mayday
Nov 16 '18 at 11:57
1
Yes, I might need to go for that solution. It just sounded to me logic that springboot is able to inject properly a list of dependencies by having all the components scan defined. But maybe in future versions heh. Thank you very much for your time :)
– Mayday
Nov 16 '18 at 14:13
|
show 7 more comments
Can you not utilize the array input for @DependsOn
rather than passing singular value, since it accepts String
? That would wait for all the beans that are explicitly declared in the array before initializing, though has to be defined manually.
@Configuration
public class MyConfig {
@Autowired
List<RequiredBean> requiredBeans;
@Bean(name="customObject")
@DependsOn({"reqBeanInsideClass", "thisOneGetsExecuted"})
public CustomObject customObject() {
log.info(requiredBeans.size());
}
@Bean(name="reqBeanInsideClass")
public RequiredBean reqBean() {
return new RequiredBean();
}
}
@Autowired
list of beans will be same as a single bean of same type, it will contain all beans with that type or with that superclass via springs injection, the problem is the ordering of bean initialization is not controlled properly, @DependsOn
with array bean input should resolve this!
Or
You can make CustomObject
bean @Lazy
, so it will be initialized only when it is used within the code after initialization is done. The bean must not be used within another non-lazy bean I think. Just call some logic where an @Autowired
CustomObject
is used, it should instantiate the bean at that moment, where the list will contain all possible RequiredBean
s
@Lazy
@Bean(name="customObject")
public CustomObject customObject() {
log.info(requiredBeans.size());
}
Can you not utilize the array input for @DependsOn
rather than passing singular value, since it accepts String
? That would wait for all the beans that are explicitly declared in the array before initializing, though has to be defined manually.
@Configuration
public class MyConfig {
@Autowired
List<RequiredBean> requiredBeans;
@Bean(name="customObject")
@DependsOn({"reqBeanInsideClass", "thisOneGetsExecuted"})
public CustomObject customObject() {
log.info(requiredBeans.size());
}
@Bean(name="reqBeanInsideClass")
public RequiredBean reqBean() {
return new RequiredBean();
}
}
@Autowired
list of beans will be same as a single bean of same type, it will contain all beans with that type or with that superclass via springs injection, the problem is the ordering of bean initialization is not controlled properly, @DependsOn
with array bean input should resolve this!
Or
You can make CustomObject
bean @Lazy
, so it will be initialized only when it is used within the code after initialization is done. The bean must not be used within another non-lazy bean I think. Just call some logic where an @Autowired
CustomObject
is used, it should instantiate the bean at that moment, where the list will contain all possible RequiredBean
s
@Lazy
@Bean(name="customObject")
public CustomObject customObject() {
log.info(requiredBeans.size());
}
edited Nov 16 '18 at 10:37
answered Nov 16 '18 at 10:15


buræqueteburæquete
5,47742150
5,47742150
I could do that, but I would love to automatically just declare new @Beans of type RequiredBean in different parts of application, and not having to get worried about specifying them 1 by 1 every time I add a new one
– Mayday
Nov 16 '18 at 10:33
no, still not working. Probably because it is being required early on. (I do not really have control on when "CustomObject" gets executed, since it is related with JPA, to create datasources etc. Actually, "CustomObject" is just to make the sample easier to understand, but in my project is "LocalContainerEntityManagerFactoryBean") However, if I take the inside class Bean into another class, it always seems to work. I just can not understand why :/
– Mayday
Nov 16 '18 at 10:49
Oh, ifCustomObject
is a factory, then it has to be processed during the initialization, you have to utilize@DependsOn
with explicit beans, if possible you can try to put them all in a single class and put theCustomObject
at the bottom, the order is why yourRequiredBean
is not picked up I think. Or how about putting@Order
on your@Configuration
classes? Put a very high number on the one withCustomObject
bean, and any new one you add, use1, 2, 3, ...
, if you have access to those configuration source code of course, not sure if@Order
would work on@Configuration
though
– buræquete
Nov 16 '18 at 10:58
If I move thereqBeanInsideClass
and write it into anther config class, then it works :)
– Mayday
Nov 16 '18 at 11:57
1
Yes, I might need to go for that solution. It just sounded to me logic that springboot is able to inject properly a list of dependencies by having all the components scan defined. But maybe in future versions heh. Thank you very much for your time :)
– Mayday
Nov 16 '18 at 14:13
|
show 7 more comments
I could do that, but I would love to automatically just declare new @Beans of type RequiredBean in different parts of application, and not having to get worried about specifying them 1 by 1 every time I add a new one
– Mayday
Nov 16 '18 at 10:33
no, still not working. Probably because it is being required early on. (I do not really have control on when "CustomObject" gets executed, since it is related with JPA, to create datasources etc. Actually, "CustomObject" is just to make the sample easier to understand, but in my project is "LocalContainerEntityManagerFactoryBean") However, if I take the inside class Bean into another class, it always seems to work. I just can not understand why :/
– Mayday
Nov 16 '18 at 10:49
Oh, ifCustomObject
is a factory, then it has to be processed during the initialization, you have to utilize@DependsOn
with explicit beans, if possible you can try to put them all in a single class and put theCustomObject
at the bottom, the order is why yourRequiredBean
is not picked up I think. Or how about putting@Order
on your@Configuration
classes? Put a very high number on the one withCustomObject
bean, and any new one you add, use1, 2, 3, ...
, if you have access to those configuration source code of course, not sure if@Order
would work on@Configuration
though
– buræquete
Nov 16 '18 at 10:58
If I move thereqBeanInsideClass
and write it into anther config class, then it works :)
– Mayday
Nov 16 '18 at 11:57
1
Yes, I might need to go for that solution. It just sounded to me logic that springboot is able to inject properly a list of dependencies by having all the components scan defined. But maybe in future versions heh. Thank you very much for your time :)
– Mayday
Nov 16 '18 at 14:13
I could do that, but I would love to automatically just declare new @Beans of type RequiredBean in different parts of application, and not having to get worried about specifying them 1 by 1 every time I add a new one
– Mayday
Nov 16 '18 at 10:33
I could do that, but I would love to automatically just declare new @Beans of type RequiredBean in different parts of application, and not having to get worried about specifying them 1 by 1 every time I add a new one
– Mayday
Nov 16 '18 at 10:33
no, still not working. Probably because it is being required early on. (I do not really have control on when "CustomObject" gets executed, since it is related with JPA, to create datasources etc. Actually, "CustomObject" is just to make the sample easier to understand, but in my project is "LocalContainerEntityManagerFactoryBean") However, if I take the inside class Bean into another class, it always seems to work. I just can not understand why :/
– Mayday
Nov 16 '18 at 10:49
no, still not working. Probably because it is being required early on. (I do not really have control on when "CustomObject" gets executed, since it is related with JPA, to create datasources etc. Actually, "CustomObject" is just to make the sample easier to understand, but in my project is "LocalContainerEntityManagerFactoryBean") However, if I take the inside class Bean into another class, it always seems to work. I just can not understand why :/
– Mayday
Nov 16 '18 at 10:49
Oh, if
CustomObject
is a factory, then it has to be processed during the initialization, you have to utilize @DependsOn
with explicit beans, if possible you can try to put them all in a single class and put the CustomObject
at the bottom, the order is why your RequiredBean
is not picked up I think. Or how about putting @Order
on your @Configuration
classes? Put a very high number on the one with CustomObject
bean, and any new one you add, use 1, 2, 3, ...
, if you have access to those configuration source code of course, not sure if @Order
would work on @Configuration
though– buræquete
Nov 16 '18 at 10:58
Oh, if
CustomObject
is a factory, then it has to be processed during the initialization, you have to utilize @DependsOn
with explicit beans, if possible you can try to put them all in a single class and put the CustomObject
at the bottom, the order is why your RequiredBean
is not picked up I think. Or how about putting @Order
on your @Configuration
classes? Put a very high number on the one with CustomObject
bean, and any new one you add, use 1, 2, 3, ...
, if you have access to those configuration source code of course, not sure if @Order
would work on @Configuration
though– buræquete
Nov 16 '18 at 10:58
If I move the
reqBeanInsideClass
and write it into anther config class, then it works :)– Mayday
Nov 16 '18 at 11:57
If I move the
reqBeanInsideClass
and write it into anther config class, then it works :)– Mayday
Nov 16 '18 at 11:57
1
1
Yes, I might need to go for that solution. It just sounded to me logic that springboot is able to inject properly a list of dependencies by having all the components scan defined. But maybe in future versions heh. Thank you very much for your time :)
– Mayday
Nov 16 '18 at 14:13
Yes, I might need to go for that solution. It just sounded to me logic that springboot is able to inject properly a list of dependencies by having all the components scan defined. But maybe in future versions heh. Thank you very much for your time :)
– Mayday
Nov 16 '18 at 14:13
|
show 7 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53335191%2fmake-bean-depend-on-list-of-beans-in-springboot%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LJq0GEsO9YpmvCEJHQyXjG l6GGQBCv8Wkr3UZwrI ZHWFQhWYVr2MW480YDi5rn,etQ
🙋🏻♂️ you are declaring beans but no where adding to arraylist, even how does that list have size 1?
– Deadpool
Nov 16 '18 at 9:51
@Deadpool Probably because randomly other Beans get initialized first? I'm not sure, thats why I am asking which is the correct way to make this dependency
– Mayday
Nov 16 '18 at 9:53