Display most liked post on a page php
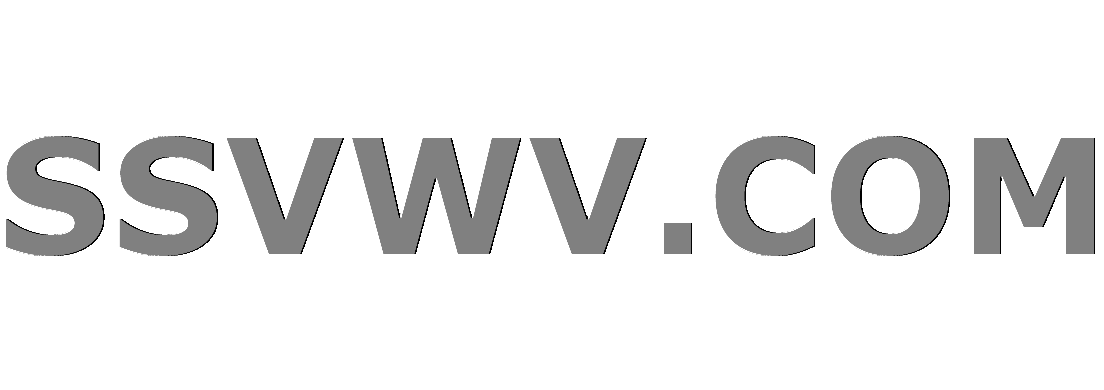
Multi tool use
I written a script where users can post without signing up or logging in and recieve likes. Sumbitted posts are being displayed on a page and post with most likes is first, which is great, but I created a Most Liked section where I would like to display post with most likes.
comment-like-unlike.php
?php
require_once ("db.php");
$memberId = 1;
$commentId = $_POST['comment_id'];
$likeOrUnlike = 0;
if($_POST['like_unlike'] == 1)
{
$likeOrUnlike = $_POST['like_unlike'];
}
$sql = "SELECT * FROM tbl_like_unlike WHERE comment_id=" . $commentId . " and member_id=" . $memberId;
$result = mysqli_query($conn, $sql);
$row = mysqli_fetch_array($result, MYSQLI_ASSOC);
if (! empty($row))
{
$query = "UPDATE tbl_like_unlike SET like_unlike = " . $likeOrUnlike . " WHERE comment_id=" . $commentId . " and member_id=" . $memberId;
} else
{
$query = "INSERT INTO tbl_like_unlike(member_id,comment_id,like_unlike) VALUES ('" . $memberId . "','" . $commentId . "','" . $likeOrUnlike . "')";
}
mysqli_query($conn, $query);
$totalLikes = "No ";
$likeQuery = "SELECT sum(like_unlike) AS likesCount FROM tbl_like_unlike WHERE comment_id=".$commentId;
$resultLikeQuery = mysqli_query($conn,$likeQuery);
$fetchLikes = mysqli_fetch_array($resultLikeQuery,MYSQLI_ASSOC);
if(isset($fetchLikes['likesCount'])) {
$totalLikes = $fetchLikes['likesCount'];
}
echo $totalLikes;
?>
comment-add.php
<?php
require_once ("db.php");
$commentId = isset($_POST['comment_id']) ? $_POST['comment_id'] : "";
$comment = isset($_POST['comment']) ? $_POST['comment'] : "";
$commentSenderName = isset($_POST['name']) ? $_POST['name'] : "";
$date = date('Y-m-d H:i:s');
$sql = "INSERT INTO tbl_comment(parent_comment_id,comment,comment_sender_name,date) VALUES ('" . $commentId . "','" . $comment . "','" . $commentSenderName . "','" . $date . "')";
$result = mysqli_query($conn, $sql);
if (! $result) {
$result = mysqli_error($conn);
}
echo $result;
?>
get-like-unlike.php
<?php
require_once ("db.php");
$commentId = $_POST['comment_id'];
$totalLikes = "No ";
$likeQuery = "SELECT sum(like_unlike) AS likesCount FROM tbl_like_unlike WHERE comment_id=".$commentId;
$resultLikeQuery = mysqli_query($conn,$likeQuery);
$fetchLikes = mysqli_fetch_array($resultLikeQuery,MYSQLI_ASSOC);
if(isset($fetchLikes['likesCount'])) {
$totalLikes = $fetchLikes['likesCount'];
}
echo $totalLikes;
?>
comment-list.php
<?php
require_once ("db.php");
$memberId = 1;
$sql = "SELECT tbl_comment.*,tbl_like_unlike.like_unlike FROM tbl_comment LEFT JOIN tbl_like_unlike ON tbl_comment.comment_id = tbl_like_unlike.comment_id AND member_id = " . $memberId . " ORDER BY tbl_like_unlike.like_unlike DESC, tbl_like_unlike.date DESC";
$result = mysqli_query($conn, $sql);
$record_set = array();
while ($row = mysqli_fetch_assoc($result)) {
array_push($record_set, $row);
}
mysqli_free_result($result);
mysqli_close($conn);
echo json_encode($record_set);
?>
index.php:
<div class="comment-form-container">
<form id="frm-comment">
<div class="input-row">
<input type="hidden" name="comment_id" id="commentId"
placeholder="Name" /> <input class="input-field"
type="text" name="name" id="name" placeholder="Name" />
</div>
<div class="input-row">
<textarea class="input-field" type="text" name="comment"
id="comment" placeholder="Add a Comment"> </textarea>
</div>
<div>
<font color="white"><input type="button" class="btn-submit" id="submitButton"
value="Publish" /></font>
</div>
</form>
</div>
<div id="output"></div>
<script>
var totalLikes = 0;
var totalUnlikes = 0;
function postReply(commentId) {
$('#commentId').val(commentId);
$("#name").focus();
}
$("#submitButton").click(function () {
$("#comment-message").css('display', 'none');
var str = $("#frm-comment").serialize();
$.ajax({
url: "comment-add.php",
data: str,
type: 'post',
success: function (response)
{
var result = eval('(' + response + ')');
if (response)
{
$("#comment-message").css('display', 'inline-block');
$("#name").val("");
$("#comment").val("");
$("#commentId").val("");
listComment();
} else
{
alert("Failed to add comments !");
return false;
}
}
});
});
$(document).ready(function () {
listComment();
});
function listComment() {
$.post("comment-list.php",
function (data) {
var data = JSON.parse(data);
var comments = "";
var replies = "";
var item = "";
var parent = -1;
var results = new Array();
var list = $("<ul class='outer-comment'>");
var item = $("<li>").html(comments);
for (var i = 0; (i < data.length); i++)
{
var commentId = data[i]['comment_id'];
parent = data[i]['parent_comment_id'];
var obj = getLikesUnlikes(commentId);
if (parent == "0")
{
if(data[i]['like_unlike'] >= 1)
{
like_icon = "<img src='like.png' id='unlike_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",-1)' />";
like_icon += "<img style='display:none;' src='unlike.png' id='like_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",1)' />";
}
else
{
like_icon = "<img style='display:none;' src='like.png' id='unlike_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",-1)' />";
like_icon += "<img src='unlike.png' id='like_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",1)' />";
}
comments = "
<div class='comment-row'>
<div class='comment-info'>
<span class='commet-row-label'>from</span>
<span class='posted-by'>" + data[i]['comment_sender_name'] + "</span>
<span class='commet-row-label'>at</span>
<span class='posted-at'>" + data[i]['date'] + "</span>
</div>
<div class='comment-text'>" + data[i]['comment'] + "</div>
<div>
<a class='btn-reply' onClick='postReply(" + commentId + ")'>Reply</a>
</div>
<div class='post-action'> " + like_icon + "
<span id='likes_" + commentId + "'> " + totalLikes + " likes </span>
</div>
</div>";
var item = $("<li>").html(comments);
list.append(item);
var reply_list = $('<ul>');
item.append(reply_list);
listReplies(commentId, data, reply_list);
}
}
$("#output").html(list);
});
}
function listReplies(commentId, data, list) {
for (var i = 0; (i < data.length); i++)
{
var obj = getLikesUnlikes(data[i].comment_id);
if (commentId == data[i].parent_comment_id)
{
if(data[i]['like_unlike'] >= 1)
{
like_icon = "<img src='like.png' id='unlike_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",-1)' />";
like_icon += "<img style='display:none;' src='unlike.png' id='like_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",1)' />";
}
else
{
like_icon = "<img style='display:none;' src='like.png' id='unlike_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",-1)' />";
like_icon += "<img src='unlike.png' id='like_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",1)' />";
}
var comments = "
<div class='comment-row'>
<div class='comment-info'>
<span class='commet-row-label'>from</span>
<span class='posted-by'>" + data[i]['comment_sender_name'] + "</span>
<span class='commet-row-label'>at</span>
<span class='posted-at'>" + data[i]['date'] + "</span>
</div>
<div class='comment-text'>" + data[i]['comment'] + "</div>
<div>
<a class='btn-reply' onClick='postReply(" + data[i]['comment_id'] + ")'>Reply</a>
</div>
<div class='post-action'> " + like_icon + "
<span id='likes_" + data[i]['comment_id'] + "'> " + totalLikes + " likes </span>
</div>
</div>";
var item = $("<li>").html(comments);
var reply_list = $('<ul>');
list.append(item);
item.append(reply_list);
listReplies(data[i].comment_id, data, reply_list);
}
}
}
function getLikesUnlikes(commentId)
{
$.ajax({
type: 'POST',
async: false,
url: 'get-like-unlike.php',
data: {comment_id: commentId},
success: function (data)
{
totalLikes = data;
}
});
}
function likeOrDislike(comment_id,like_unlike)
{
$.ajax({
url: 'comment-like-unlike.php',
async: false,
type: 'post',
data: {comment_id:comment_id,like_unlike:like_unlike},
dataType: 'json',
success: function (data) {
$("#likes_"+comment_id).text(data + " likes");
if (like_unlike == 1) {
$("#like_" + comment_id).css("display", "none");
$("#unlike_" + comment_id).show();
}
if (like_unlike == -1) {
$("#unlike_" + comment_id).css("display", "none");
$("#like_" + comment_id).show();
}
},
error: function (data) {
alert("error : " + JSON.stringify(data));
}
});
}
</script>
I will post picture of a website just you guys have a clue what Im talking about:
This is where I want most liked post:
javascript php html mysql
|
show 4 more comments
I written a script where users can post without signing up or logging in and recieve likes. Sumbitted posts are being displayed on a page and post with most likes is first, which is great, but I created a Most Liked section where I would like to display post with most likes.
comment-like-unlike.php
?php
require_once ("db.php");
$memberId = 1;
$commentId = $_POST['comment_id'];
$likeOrUnlike = 0;
if($_POST['like_unlike'] == 1)
{
$likeOrUnlike = $_POST['like_unlike'];
}
$sql = "SELECT * FROM tbl_like_unlike WHERE comment_id=" . $commentId . " and member_id=" . $memberId;
$result = mysqli_query($conn, $sql);
$row = mysqli_fetch_array($result, MYSQLI_ASSOC);
if (! empty($row))
{
$query = "UPDATE tbl_like_unlike SET like_unlike = " . $likeOrUnlike . " WHERE comment_id=" . $commentId . " and member_id=" . $memberId;
} else
{
$query = "INSERT INTO tbl_like_unlike(member_id,comment_id,like_unlike) VALUES ('" . $memberId . "','" . $commentId . "','" . $likeOrUnlike . "')";
}
mysqli_query($conn, $query);
$totalLikes = "No ";
$likeQuery = "SELECT sum(like_unlike) AS likesCount FROM tbl_like_unlike WHERE comment_id=".$commentId;
$resultLikeQuery = mysqli_query($conn,$likeQuery);
$fetchLikes = mysqli_fetch_array($resultLikeQuery,MYSQLI_ASSOC);
if(isset($fetchLikes['likesCount'])) {
$totalLikes = $fetchLikes['likesCount'];
}
echo $totalLikes;
?>
comment-add.php
<?php
require_once ("db.php");
$commentId = isset($_POST['comment_id']) ? $_POST['comment_id'] : "";
$comment = isset($_POST['comment']) ? $_POST['comment'] : "";
$commentSenderName = isset($_POST['name']) ? $_POST['name'] : "";
$date = date('Y-m-d H:i:s');
$sql = "INSERT INTO tbl_comment(parent_comment_id,comment,comment_sender_name,date) VALUES ('" . $commentId . "','" . $comment . "','" . $commentSenderName . "','" . $date . "')";
$result = mysqli_query($conn, $sql);
if (! $result) {
$result = mysqli_error($conn);
}
echo $result;
?>
get-like-unlike.php
<?php
require_once ("db.php");
$commentId = $_POST['comment_id'];
$totalLikes = "No ";
$likeQuery = "SELECT sum(like_unlike) AS likesCount FROM tbl_like_unlike WHERE comment_id=".$commentId;
$resultLikeQuery = mysqli_query($conn,$likeQuery);
$fetchLikes = mysqli_fetch_array($resultLikeQuery,MYSQLI_ASSOC);
if(isset($fetchLikes['likesCount'])) {
$totalLikes = $fetchLikes['likesCount'];
}
echo $totalLikes;
?>
comment-list.php
<?php
require_once ("db.php");
$memberId = 1;
$sql = "SELECT tbl_comment.*,tbl_like_unlike.like_unlike FROM tbl_comment LEFT JOIN tbl_like_unlike ON tbl_comment.comment_id = tbl_like_unlike.comment_id AND member_id = " . $memberId . " ORDER BY tbl_like_unlike.like_unlike DESC, tbl_like_unlike.date DESC";
$result = mysqli_query($conn, $sql);
$record_set = array();
while ($row = mysqli_fetch_assoc($result)) {
array_push($record_set, $row);
}
mysqli_free_result($result);
mysqli_close($conn);
echo json_encode($record_set);
?>
index.php:
<div class="comment-form-container">
<form id="frm-comment">
<div class="input-row">
<input type="hidden" name="comment_id" id="commentId"
placeholder="Name" /> <input class="input-field"
type="text" name="name" id="name" placeholder="Name" />
</div>
<div class="input-row">
<textarea class="input-field" type="text" name="comment"
id="comment" placeholder="Add a Comment"> </textarea>
</div>
<div>
<font color="white"><input type="button" class="btn-submit" id="submitButton"
value="Publish" /></font>
</div>
</form>
</div>
<div id="output"></div>
<script>
var totalLikes = 0;
var totalUnlikes = 0;
function postReply(commentId) {
$('#commentId').val(commentId);
$("#name").focus();
}
$("#submitButton").click(function () {
$("#comment-message").css('display', 'none');
var str = $("#frm-comment").serialize();
$.ajax({
url: "comment-add.php",
data: str,
type: 'post',
success: function (response)
{
var result = eval('(' + response + ')');
if (response)
{
$("#comment-message").css('display', 'inline-block');
$("#name").val("");
$("#comment").val("");
$("#commentId").val("");
listComment();
} else
{
alert("Failed to add comments !");
return false;
}
}
});
});
$(document).ready(function () {
listComment();
});
function listComment() {
$.post("comment-list.php",
function (data) {
var data = JSON.parse(data);
var comments = "";
var replies = "";
var item = "";
var parent = -1;
var results = new Array();
var list = $("<ul class='outer-comment'>");
var item = $("<li>").html(comments);
for (var i = 0; (i < data.length); i++)
{
var commentId = data[i]['comment_id'];
parent = data[i]['parent_comment_id'];
var obj = getLikesUnlikes(commentId);
if (parent == "0")
{
if(data[i]['like_unlike'] >= 1)
{
like_icon = "<img src='like.png' id='unlike_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",-1)' />";
like_icon += "<img style='display:none;' src='unlike.png' id='like_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",1)' />";
}
else
{
like_icon = "<img style='display:none;' src='like.png' id='unlike_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",-1)' />";
like_icon += "<img src='unlike.png' id='like_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",1)' />";
}
comments = "
<div class='comment-row'>
<div class='comment-info'>
<span class='commet-row-label'>from</span>
<span class='posted-by'>" + data[i]['comment_sender_name'] + "</span>
<span class='commet-row-label'>at</span>
<span class='posted-at'>" + data[i]['date'] + "</span>
</div>
<div class='comment-text'>" + data[i]['comment'] + "</div>
<div>
<a class='btn-reply' onClick='postReply(" + commentId + ")'>Reply</a>
</div>
<div class='post-action'> " + like_icon + "
<span id='likes_" + commentId + "'> " + totalLikes + " likes </span>
</div>
</div>";
var item = $("<li>").html(comments);
list.append(item);
var reply_list = $('<ul>');
item.append(reply_list);
listReplies(commentId, data, reply_list);
}
}
$("#output").html(list);
});
}
function listReplies(commentId, data, list) {
for (var i = 0; (i < data.length); i++)
{
var obj = getLikesUnlikes(data[i].comment_id);
if (commentId == data[i].parent_comment_id)
{
if(data[i]['like_unlike'] >= 1)
{
like_icon = "<img src='like.png' id='unlike_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",-1)' />";
like_icon += "<img style='display:none;' src='unlike.png' id='like_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",1)' />";
}
else
{
like_icon = "<img style='display:none;' src='like.png' id='unlike_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",-1)' />";
like_icon += "<img src='unlike.png' id='like_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",1)' />";
}
var comments = "
<div class='comment-row'>
<div class='comment-info'>
<span class='commet-row-label'>from</span>
<span class='posted-by'>" + data[i]['comment_sender_name'] + "</span>
<span class='commet-row-label'>at</span>
<span class='posted-at'>" + data[i]['date'] + "</span>
</div>
<div class='comment-text'>" + data[i]['comment'] + "</div>
<div>
<a class='btn-reply' onClick='postReply(" + data[i]['comment_id'] + ")'>Reply</a>
</div>
<div class='post-action'> " + like_icon + "
<span id='likes_" + data[i]['comment_id'] + "'> " + totalLikes + " likes </span>
</div>
</div>";
var item = $("<li>").html(comments);
var reply_list = $('<ul>');
list.append(item);
item.append(reply_list);
listReplies(data[i].comment_id, data, reply_list);
}
}
}
function getLikesUnlikes(commentId)
{
$.ajax({
type: 'POST',
async: false,
url: 'get-like-unlike.php',
data: {comment_id: commentId},
success: function (data)
{
totalLikes = data;
}
});
}
function likeOrDislike(comment_id,like_unlike)
{
$.ajax({
url: 'comment-like-unlike.php',
async: false,
type: 'post',
data: {comment_id:comment_id,like_unlike:like_unlike},
dataType: 'json',
success: function (data) {
$("#likes_"+comment_id).text(data + " likes");
if (like_unlike == 1) {
$("#like_" + comment_id).css("display", "none");
$("#unlike_" + comment_id).show();
}
if (like_unlike == -1) {
$("#unlike_" + comment_id).css("display", "none");
$("#like_" + comment_id).show();
}
},
error: function (data) {
alert("error : " + JSON.stringify(data));
}
});
}
</script>
I will post picture of a website just you guys have a clue what Im talking about:
This is where I want most liked post:
javascript php html mysql
1
I'm curious; didn't you ask this already and gotten an answer? Or is this question different?
– Funk Forty Niner
Nov 16 '18 at 0:37
If you want to count, why are you usingSUM()
instead ofCOUNT()
?
– Funk Forty Niner
Nov 16 '18 at 0:38
It is, I need to show most liked post in a desired section, as you can see in image, Ive got an answer how to sort them by most liked, but not how to display it in a page in a specific section
– First Name
Nov 16 '18 at 0:39
OK. Not my downvote btw. So, for my 2nd comment about SUM/COUNT. UsingCOUNT()
would be easier I find. Note: I suggest you use a prepared statement since your code is open to injection.
– Funk Forty Niner
Nov 16 '18 at 0:43
1
Wanting to have the most liked, usingCOUNT(col)
would probably be your best bet. I'm thinking out loud here of course, but if that isn't the problem here, then I can't see / understand what isn't working the way you want it to. What results are you getting now as opposed to the desired results?
– Funk Forty Niner
Nov 16 '18 at 0:45
|
show 4 more comments
I written a script where users can post without signing up or logging in and recieve likes. Sumbitted posts are being displayed on a page and post with most likes is first, which is great, but I created a Most Liked section where I would like to display post with most likes.
comment-like-unlike.php
?php
require_once ("db.php");
$memberId = 1;
$commentId = $_POST['comment_id'];
$likeOrUnlike = 0;
if($_POST['like_unlike'] == 1)
{
$likeOrUnlike = $_POST['like_unlike'];
}
$sql = "SELECT * FROM tbl_like_unlike WHERE comment_id=" . $commentId . " and member_id=" . $memberId;
$result = mysqli_query($conn, $sql);
$row = mysqli_fetch_array($result, MYSQLI_ASSOC);
if (! empty($row))
{
$query = "UPDATE tbl_like_unlike SET like_unlike = " . $likeOrUnlike . " WHERE comment_id=" . $commentId . " and member_id=" . $memberId;
} else
{
$query = "INSERT INTO tbl_like_unlike(member_id,comment_id,like_unlike) VALUES ('" . $memberId . "','" . $commentId . "','" . $likeOrUnlike . "')";
}
mysqli_query($conn, $query);
$totalLikes = "No ";
$likeQuery = "SELECT sum(like_unlike) AS likesCount FROM tbl_like_unlike WHERE comment_id=".$commentId;
$resultLikeQuery = mysqli_query($conn,$likeQuery);
$fetchLikes = mysqli_fetch_array($resultLikeQuery,MYSQLI_ASSOC);
if(isset($fetchLikes['likesCount'])) {
$totalLikes = $fetchLikes['likesCount'];
}
echo $totalLikes;
?>
comment-add.php
<?php
require_once ("db.php");
$commentId = isset($_POST['comment_id']) ? $_POST['comment_id'] : "";
$comment = isset($_POST['comment']) ? $_POST['comment'] : "";
$commentSenderName = isset($_POST['name']) ? $_POST['name'] : "";
$date = date('Y-m-d H:i:s');
$sql = "INSERT INTO tbl_comment(parent_comment_id,comment,comment_sender_name,date) VALUES ('" . $commentId . "','" . $comment . "','" . $commentSenderName . "','" . $date . "')";
$result = mysqli_query($conn, $sql);
if (! $result) {
$result = mysqli_error($conn);
}
echo $result;
?>
get-like-unlike.php
<?php
require_once ("db.php");
$commentId = $_POST['comment_id'];
$totalLikes = "No ";
$likeQuery = "SELECT sum(like_unlike) AS likesCount FROM tbl_like_unlike WHERE comment_id=".$commentId;
$resultLikeQuery = mysqli_query($conn,$likeQuery);
$fetchLikes = mysqli_fetch_array($resultLikeQuery,MYSQLI_ASSOC);
if(isset($fetchLikes['likesCount'])) {
$totalLikes = $fetchLikes['likesCount'];
}
echo $totalLikes;
?>
comment-list.php
<?php
require_once ("db.php");
$memberId = 1;
$sql = "SELECT tbl_comment.*,tbl_like_unlike.like_unlike FROM tbl_comment LEFT JOIN tbl_like_unlike ON tbl_comment.comment_id = tbl_like_unlike.comment_id AND member_id = " . $memberId . " ORDER BY tbl_like_unlike.like_unlike DESC, tbl_like_unlike.date DESC";
$result = mysqli_query($conn, $sql);
$record_set = array();
while ($row = mysqli_fetch_assoc($result)) {
array_push($record_set, $row);
}
mysqli_free_result($result);
mysqli_close($conn);
echo json_encode($record_set);
?>
index.php:
<div class="comment-form-container">
<form id="frm-comment">
<div class="input-row">
<input type="hidden" name="comment_id" id="commentId"
placeholder="Name" /> <input class="input-field"
type="text" name="name" id="name" placeholder="Name" />
</div>
<div class="input-row">
<textarea class="input-field" type="text" name="comment"
id="comment" placeholder="Add a Comment"> </textarea>
</div>
<div>
<font color="white"><input type="button" class="btn-submit" id="submitButton"
value="Publish" /></font>
</div>
</form>
</div>
<div id="output"></div>
<script>
var totalLikes = 0;
var totalUnlikes = 0;
function postReply(commentId) {
$('#commentId').val(commentId);
$("#name").focus();
}
$("#submitButton").click(function () {
$("#comment-message").css('display', 'none');
var str = $("#frm-comment").serialize();
$.ajax({
url: "comment-add.php",
data: str,
type: 'post',
success: function (response)
{
var result = eval('(' + response + ')');
if (response)
{
$("#comment-message").css('display', 'inline-block');
$("#name").val("");
$("#comment").val("");
$("#commentId").val("");
listComment();
} else
{
alert("Failed to add comments !");
return false;
}
}
});
});
$(document).ready(function () {
listComment();
});
function listComment() {
$.post("comment-list.php",
function (data) {
var data = JSON.parse(data);
var comments = "";
var replies = "";
var item = "";
var parent = -1;
var results = new Array();
var list = $("<ul class='outer-comment'>");
var item = $("<li>").html(comments);
for (var i = 0; (i < data.length); i++)
{
var commentId = data[i]['comment_id'];
parent = data[i]['parent_comment_id'];
var obj = getLikesUnlikes(commentId);
if (parent == "0")
{
if(data[i]['like_unlike'] >= 1)
{
like_icon = "<img src='like.png' id='unlike_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",-1)' />";
like_icon += "<img style='display:none;' src='unlike.png' id='like_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",1)' />";
}
else
{
like_icon = "<img style='display:none;' src='like.png' id='unlike_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",-1)' />";
like_icon += "<img src='unlike.png' id='like_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",1)' />";
}
comments = "
<div class='comment-row'>
<div class='comment-info'>
<span class='commet-row-label'>from</span>
<span class='posted-by'>" + data[i]['comment_sender_name'] + "</span>
<span class='commet-row-label'>at</span>
<span class='posted-at'>" + data[i]['date'] + "</span>
</div>
<div class='comment-text'>" + data[i]['comment'] + "</div>
<div>
<a class='btn-reply' onClick='postReply(" + commentId + ")'>Reply</a>
</div>
<div class='post-action'> " + like_icon + "
<span id='likes_" + commentId + "'> " + totalLikes + " likes </span>
</div>
</div>";
var item = $("<li>").html(comments);
list.append(item);
var reply_list = $('<ul>');
item.append(reply_list);
listReplies(commentId, data, reply_list);
}
}
$("#output").html(list);
});
}
function listReplies(commentId, data, list) {
for (var i = 0; (i < data.length); i++)
{
var obj = getLikesUnlikes(data[i].comment_id);
if (commentId == data[i].parent_comment_id)
{
if(data[i]['like_unlike'] >= 1)
{
like_icon = "<img src='like.png' id='unlike_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",-1)' />";
like_icon += "<img style='display:none;' src='unlike.png' id='like_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",1)' />";
}
else
{
like_icon = "<img style='display:none;' src='like.png' id='unlike_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",-1)' />";
like_icon += "<img src='unlike.png' id='like_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",1)' />";
}
var comments = "
<div class='comment-row'>
<div class='comment-info'>
<span class='commet-row-label'>from</span>
<span class='posted-by'>" + data[i]['comment_sender_name'] + "</span>
<span class='commet-row-label'>at</span>
<span class='posted-at'>" + data[i]['date'] + "</span>
</div>
<div class='comment-text'>" + data[i]['comment'] + "</div>
<div>
<a class='btn-reply' onClick='postReply(" + data[i]['comment_id'] + ")'>Reply</a>
</div>
<div class='post-action'> " + like_icon + "
<span id='likes_" + data[i]['comment_id'] + "'> " + totalLikes + " likes </span>
</div>
</div>";
var item = $("<li>").html(comments);
var reply_list = $('<ul>');
list.append(item);
item.append(reply_list);
listReplies(data[i].comment_id, data, reply_list);
}
}
}
function getLikesUnlikes(commentId)
{
$.ajax({
type: 'POST',
async: false,
url: 'get-like-unlike.php',
data: {comment_id: commentId},
success: function (data)
{
totalLikes = data;
}
});
}
function likeOrDislike(comment_id,like_unlike)
{
$.ajax({
url: 'comment-like-unlike.php',
async: false,
type: 'post',
data: {comment_id:comment_id,like_unlike:like_unlike},
dataType: 'json',
success: function (data) {
$("#likes_"+comment_id).text(data + " likes");
if (like_unlike == 1) {
$("#like_" + comment_id).css("display", "none");
$("#unlike_" + comment_id).show();
}
if (like_unlike == -1) {
$("#unlike_" + comment_id).css("display", "none");
$("#like_" + comment_id).show();
}
},
error: function (data) {
alert("error : " + JSON.stringify(data));
}
});
}
</script>
I will post picture of a website just you guys have a clue what Im talking about:
This is where I want most liked post:
javascript php html mysql
I written a script where users can post without signing up or logging in and recieve likes. Sumbitted posts are being displayed on a page and post with most likes is first, which is great, but I created a Most Liked section where I would like to display post with most likes.
comment-like-unlike.php
?php
require_once ("db.php");
$memberId = 1;
$commentId = $_POST['comment_id'];
$likeOrUnlike = 0;
if($_POST['like_unlike'] == 1)
{
$likeOrUnlike = $_POST['like_unlike'];
}
$sql = "SELECT * FROM tbl_like_unlike WHERE comment_id=" . $commentId . " and member_id=" . $memberId;
$result = mysqli_query($conn, $sql);
$row = mysqli_fetch_array($result, MYSQLI_ASSOC);
if (! empty($row))
{
$query = "UPDATE tbl_like_unlike SET like_unlike = " . $likeOrUnlike . " WHERE comment_id=" . $commentId . " and member_id=" . $memberId;
} else
{
$query = "INSERT INTO tbl_like_unlike(member_id,comment_id,like_unlike) VALUES ('" . $memberId . "','" . $commentId . "','" . $likeOrUnlike . "')";
}
mysqli_query($conn, $query);
$totalLikes = "No ";
$likeQuery = "SELECT sum(like_unlike) AS likesCount FROM tbl_like_unlike WHERE comment_id=".$commentId;
$resultLikeQuery = mysqli_query($conn,$likeQuery);
$fetchLikes = mysqli_fetch_array($resultLikeQuery,MYSQLI_ASSOC);
if(isset($fetchLikes['likesCount'])) {
$totalLikes = $fetchLikes['likesCount'];
}
echo $totalLikes;
?>
comment-add.php
<?php
require_once ("db.php");
$commentId = isset($_POST['comment_id']) ? $_POST['comment_id'] : "";
$comment = isset($_POST['comment']) ? $_POST['comment'] : "";
$commentSenderName = isset($_POST['name']) ? $_POST['name'] : "";
$date = date('Y-m-d H:i:s');
$sql = "INSERT INTO tbl_comment(parent_comment_id,comment,comment_sender_name,date) VALUES ('" . $commentId . "','" . $comment . "','" . $commentSenderName . "','" . $date . "')";
$result = mysqli_query($conn, $sql);
if (! $result) {
$result = mysqli_error($conn);
}
echo $result;
?>
get-like-unlike.php
<?php
require_once ("db.php");
$commentId = $_POST['comment_id'];
$totalLikes = "No ";
$likeQuery = "SELECT sum(like_unlike) AS likesCount FROM tbl_like_unlike WHERE comment_id=".$commentId;
$resultLikeQuery = mysqli_query($conn,$likeQuery);
$fetchLikes = mysqli_fetch_array($resultLikeQuery,MYSQLI_ASSOC);
if(isset($fetchLikes['likesCount'])) {
$totalLikes = $fetchLikes['likesCount'];
}
echo $totalLikes;
?>
comment-list.php
<?php
require_once ("db.php");
$memberId = 1;
$sql = "SELECT tbl_comment.*,tbl_like_unlike.like_unlike FROM tbl_comment LEFT JOIN tbl_like_unlike ON tbl_comment.comment_id = tbl_like_unlike.comment_id AND member_id = " . $memberId . " ORDER BY tbl_like_unlike.like_unlike DESC, tbl_like_unlike.date DESC";
$result = mysqli_query($conn, $sql);
$record_set = array();
while ($row = mysqli_fetch_assoc($result)) {
array_push($record_set, $row);
}
mysqli_free_result($result);
mysqli_close($conn);
echo json_encode($record_set);
?>
index.php:
<div class="comment-form-container">
<form id="frm-comment">
<div class="input-row">
<input type="hidden" name="comment_id" id="commentId"
placeholder="Name" /> <input class="input-field"
type="text" name="name" id="name" placeholder="Name" />
</div>
<div class="input-row">
<textarea class="input-field" type="text" name="comment"
id="comment" placeholder="Add a Comment"> </textarea>
</div>
<div>
<font color="white"><input type="button" class="btn-submit" id="submitButton"
value="Publish" /></font>
</div>
</form>
</div>
<div id="output"></div>
<script>
var totalLikes = 0;
var totalUnlikes = 0;
function postReply(commentId) {
$('#commentId').val(commentId);
$("#name").focus();
}
$("#submitButton").click(function () {
$("#comment-message").css('display', 'none');
var str = $("#frm-comment").serialize();
$.ajax({
url: "comment-add.php",
data: str,
type: 'post',
success: function (response)
{
var result = eval('(' + response + ')');
if (response)
{
$("#comment-message").css('display', 'inline-block');
$("#name").val("");
$("#comment").val("");
$("#commentId").val("");
listComment();
} else
{
alert("Failed to add comments !");
return false;
}
}
});
});
$(document).ready(function () {
listComment();
});
function listComment() {
$.post("comment-list.php",
function (data) {
var data = JSON.parse(data);
var comments = "";
var replies = "";
var item = "";
var parent = -1;
var results = new Array();
var list = $("<ul class='outer-comment'>");
var item = $("<li>").html(comments);
for (var i = 0; (i < data.length); i++)
{
var commentId = data[i]['comment_id'];
parent = data[i]['parent_comment_id'];
var obj = getLikesUnlikes(commentId);
if (parent == "0")
{
if(data[i]['like_unlike'] >= 1)
{
like_icon = "<img src='like.png' id='unlike_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",-1)' />";
like_icon += "<img style='display:none;' src='unlike.png' id='like_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",1)' />";
}
else
{
like_icon = "<img style='display:none;' src='like.png' id='unlike_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",-1)' />";
like_icon += "<img src='unlike.png' id='like_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",1)' />";
}
comments = "
<div class='comment-row'>
<div class='comment-info'>
<span class='commet-row-label'>from</span>
<span class='posted-by'>" + data[i]['comment_sender_name'] + "</span>
<span class='commet-row-label'>at</span>
<span class='posted-at'>" + data[i]['date'] + "</span>
</div>
<div class='comment-text'>" + data[i]['comment'] + "</div>
<div>
<a class='btn-reply' onClick='postReply(" + commentId + ")'>Reply</a>
</div>
<div class='post-action'> " + like_icon + "
<span id='likes_" + commentId + "'> " + totalLikes + " likes </span>
</div>
</div>";
var item = $("<li>").html(comments);
list.append(item);
var reply_list = $('<ul>');
item.append(reply_list);
listReplies(commentId, data, reply_list);
}
}
$("#output").html(list);
});
}
function listReplies(commentId, data, list) {
for (var i = 0; (i < data.length); i++)
{
var obj = getLikesUnlikes(data[i].comment_id);
if (commentId == data[i].parent_comment_id)
{
if(data[i]['like_unlike'] >= 1)
{
like_icon = "<img src='like.png' id='unlike_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",-1)' />";
like_icon += "<img style='display:none;' src='unlike.png' id='like_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",1)' />";
}
else
{
like_icon = "<img style='display:none;' src='like.png' id='unlike_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",-1)' />";
like_icon += "<img src='unlike.png' id='like_" + data[i]['comment_id'] + "' class='like-unlike' onClick='likeOrDislike(" + data[i]['comment_id'] + ",1)' />";
}
var comments = "
<div class='comment-row'>
<div class='comment-info'>
<span class='commet-row-label'>from</span>
<span class='posted-by'>" + data[i]['comment_sender_name'] + "</span>
<span class='commet-row-label'>at</span>
<span class='posted-at'>" + data[i]['date'] + "</span>
</div>
<div class='comment-text'>" + data[i]['comment'] + "</div>
<div>
<a class='btn-reply' onClick='postReply(" + data[i]['comment_id'] + ")'>Reply</a>
</div>
<div class='post-action'> " + like_icon + "
<span id='likes_" + data[i]['comment_id'] + "'> " + totalLikes + " likes </span>
</div>
</div>";
var item = $("<li>").html(comments);
var reply_list = $('<ul>');
list.append(item);
item.append(reply_list);
listReplies(data[i].comment_id, data, reply_list);
}
}
}
function getLikesUnlikes(commentId)
{
$.ajax({
type: 'POST',
async: false,
url: 'get-like-unlike.php',
data: {comment_id: commentId},
success: function (data)
{
totalLikes = data;
}
});
}
function likeOrDislike(comment_id,like_unlike)
{
$.ajax({
url: 'comment-like-unlike.php',
async: false,
type: 'post',
data: {comment_id:comment_id,like_unlike:like_unlike},
dataType: 'json',
success: function (data) {
$("#likes_"+comment_id).text(data + " likes");
if (like_unlike == 1) {
$("#like_" + comment_id).css("display", "none");
$("#unlike_" + comment_id).show();
}
if (like_unlike == -1) {
$("#unlike_" + comment_id).css("display", "none");
$("#like_" + comment_id).show();
}
},
error: function (data) {
alert("error : " + JSON.stringify(data));
}
});
}
</script>
I will post picture of a website just you guys have a clue what Im talking about:
This is where I want most liked post:
javascript php html mysql
javascript php html mysql
edited Nov 16 '18 at 0:37


Funk Forty Niner
1
1
asked Nov 16 '18 at 0:30


First NameFirst Name
869
869
1
I'm curious; didn't you ask this already and gotten an answer? Or is this question different?
– Funk Forty Niner
Nov 16 '18 at 0:37
If you want to count, why are you usingSUM()
instead ofCOUNT()
?
– Funk Forty Niner
Nov 16 '18 at 0:38
It is, I need to show most liked post in a desired section, as you can see in image, Ive got an answer how to sort them by most liked, but not how to display it in a page in a specific section
– First Name
Nov 16 '18 at 0:39
OK. Not my downvote btw. So, for my 2nd comment about SUM/COUNT. UsingCOUNT()
would be easier I find. Note: I suggest you use a prepared statement since your code is open to injection.
– Funk Forty Niner
Nov 16 '18 at 0:43
1
Wanting to have the most liked, usingCOUNT(col)
would probably be your best bet. I'm thinking out loud here of course, but if that isn't the problem here, then I can't see / understand what isn't working the way you want it to. What results are you getting now as opposed to the desired results?
– Funk Forty Niner
Nov 16 '18 at 0:45
|
show 4 more comments
1
I'm curious; didn't you ask this already and gotten an answer? Or is this question different?
– Funk Forty Niner
Nov 16 '18 at 0:37
If you want to count, why are you usingSUM()
instead ofCOUNT()
?
– Funk Forty Niner
Nov 16 '18 at 0:38
It is, I need to show most liked post in a desired section, as you can see in image, Ive got an answer how to sort them by most liked, but not how to display it in a page in a specific section
– First Name
Nov 16 '18 at 0:39
OK. Not my downvote btw. So, for my 2nd comment about SUM/COUNT. UsingCOUNT()
would be easier I find. Note: I suggest you use a prepared statement since your code is open to injection.
– Funk Forty Niner
Nov 16 '18 at 0:43
1
Wanting to have the most liked, usingCOUNT(col)
would probably be your best bet. I'm thinking out loud here of course, but if that isn't the problem here, then I can't see / understand what isn't working the way you want it to. What results are you getting now as opposed to the desired results?
– Funk Forty Niner
Nov 16 '18 at 0:45
1
1
I'm curious; didn't you ask this already and gotten an answer? Or is this question different?
– Funk Forty Niner
Nov 16 '18 at 0:37
I'm curious; didn't you ask this already and gotten an answer? Or is this question different?
– Funk Forty Niner
Nov 16 '18 at 0:37
If you want to count, why are you using
SUM()
instead of COUNT()
?– Funk Forty Niner
Nov 16 '18 at 0:38
If you want to count, why are you using
SUM()
instead of COUNT()
?– Funk Forty Niner
Nov 16 '18 at 0:38
It is, I need to show most liked post in a desired section, as you can see in image, Ive got an answer how to sort them by most liked, but not how to display it in a page in a specific section
– First Name
Nov 16 '18 at 0:39
It is, I need to show most liked post in a desired section, as you can see in image, Ive got an answer how to sort them by most liked, but not how to display it in a page in a specific section
– First Name
Nov 16 '18 at 0:39
OK. Not my downvote btw. So, for my 2nd comment about SUM/COUNT. Using
COUNT()
would be easier I find. Note: I suggest you use a prepared statement since your code is open to injection.– Funk Forty Niner
Nov 16 '18 at 0:43
OK. Not my downvote btw. So, for my 2nd comment about SUM/COUNT. Using
COUNT()
would be easier I find. Note: I suggest you use a prepared statement since your code is open to injection.– Funk Forty Niner
Nov 16 '18 at 0:43
1
1
Wanting to have the most liked, using
COUNT(col)
would probably be your best bet. I'm thinking out loud here of course, but if that isn't the problem here, then I can't see / understand what isn't working the way you want it to. What results are you getting now as opposed to the desired results?– Funk Forty Niner
Nov 16 '18 at 0:45
Wanting to have the most liked, using
COUNT(col)
would probably be your best bet. I'm thinking out loud here of course, but if that isn't the problem here, then I can't see / understand what isn't working the way you want it to. What results are you getting now as opposed to the desired results?– Funk Forty Niner
Nov 16 '18 at 0:45
|
show 4 more comments
1 Answer
1
active
oldest
votes
SELECT posts from your_table ORDER BY likes DESC
add LIMIT 1 to this query and you have a result
– Jbadminton
Nov 16 '18 at 7:47
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53329766%2fdisplay-most-liked-post-on-a-page-php%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
SELECT posts from your_table ORDER BY likes DESC
add LIMIT 1 to this query and you have a result
– Jbadminton
Nov 16 '18 at 7:47
add a comment |
SELECT posts from your_table ORDER BY likes DESC
add LIMIT 1 to this query and you have a result
– Jbadminton
Nov 16 '18 at 7:47
add a comment |
SELECT posts from your_table ORDER BY likes DESC
SELECT posts from your_table ORDER BY likes DESC
answered Nov 16 '18 at 7:42
kimo26kimo26
806
806
add LIMIT 1 to this query and you have a result
– Jbadminton
Nov 16 '18 at 7:47
add a comment |
add LIMIT 1 to this query and you have a result
– Jbadminton
Nov 16 '18 at 7:47
add LIMIT 1 to this query and you have a result
– Jbadminton
Nov 16 '18 at 7:47
add LIMIT 1 to this query and you have a result
– Jbadminton
Nov 16 '18 at 7:47
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53329766%2fdisplay-most-liked-post-on-a-page-php%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
iFLDWKBrw5A,4eEYYb,Q
1
I'm curious; didn't you ask this already and gotten an answer? Or is this question different?
– Funk Forty Niner
Nov 16 '18 at 0:37
If you want to count, why are you using
SUM()
instead ofCOUNT()
?– Funk Forty Niner
Nov 16 '18 at 0:38
It is, I need to show most liked post in a desired section, as you can see in image, Ive got an answer how to sort them by most liked, but not how to display it in a page in a specific section
– First Name
Nov 16 '18 at 0:39
OK. Not my downvote btw. So, for my 2nd comment about SUM/COUNT. Using
COUNT()
would be easier I find. Note: I suggest you use a prepared statement since your code is open to injection.– Funk Forty Niner
Nov 16 '18 at 0:43
1
Wanting to have the most liked, using
COUNT(col)
would probably be your best bet. I'm thinking out loud here of course, but if that isn't the problem here, then I can't see / understand what isn't working the way you want it to. What results are you getting now as opposed to the desired results?– Funk Forty Niner
Nov 16 '18 at 0:45