Search for specific id in REST API
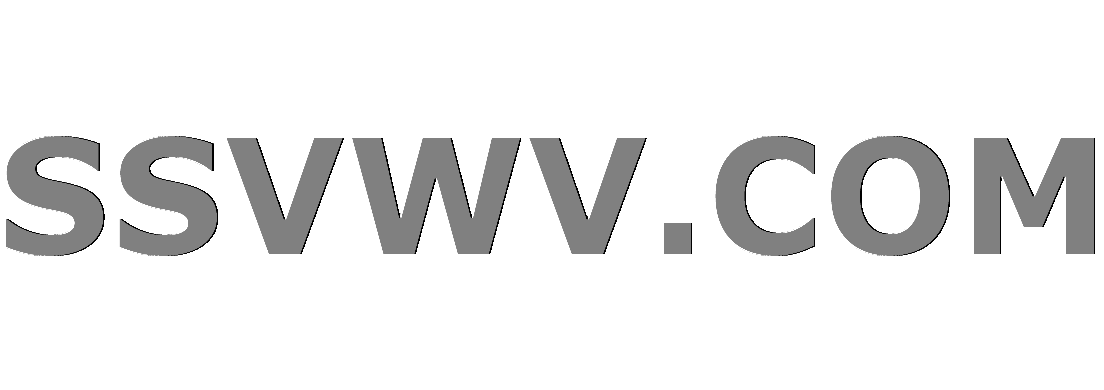
Multi tool use
I'm trying to create search in RESTful API to search for specific id and this is the code that I write:
<?php
header("Access-Control-Allow-Origin: *");
header("Content-Type: application/json; charset=UTF-8");
include_once 'dbconfig.php';
$database = new Database();
$db = $database->getConnection();
$channel = new Channel($db);
$keywords=isset($_GET["id"]) ? $_GET["id"] : "";
$stmt = $channel->searchChannel($keywords);
$num = $stmt->rowCount();
if($num>0){
$channel_arr["channel"]=array();
while ($row = $stmt->fetch(PDO::FETCH_ASSOC)){
extract($row);
$cat = $row['cat'];
$channel_item = array(
"id" => $id,
"title" => $title,
"cat" => $cat,
);
$stmt2 = $channel->searchChannels($cat);
array_push($channel_arr["channel"], $channel_item);
}
http_response_code(200);
echo json_encode($channel_arr);
}
else{
http_response_code(404);
echo json_encode(
array("message" => "No channels found.")
);
}
class Channel{
private $conn;
private $table_name = "esite_channels";
public $id;
public $title;
public $cat;
public function __construct($db){
$this->conn = $db;
}
function searchChannel($keywords){
$query = "SELECT
e.id, e.title, e.image, e.streamname, e.enable, e.cat, e.app
FROM
" . $this->table_name . " e
WHERE
e.id LIKE ?
";
$stmt = $this->conn->prepare($query);
$keywords=htmlspecialchars(strip_tags($keywords));
$keywords = "%{$keywords}%";
$stmt->bindParam(1, $keywords);
$stmt->execute();
return $stmt;
}
}
?>
it works fine while I search for id 20 with this link:
http://127.0.0.1/api/search.php?id=20
it bring data of that id like this:
[
{
channel: [
{
id: "20",
title: "title",
cat: "Sports",
}
]
}
]
but when change the id for 22 for example:
http://127.0.0.1/api/search.php?id=22
it bring me all items that contain 22 in the id like this:
[
{
channel: [
{
id: "22",
title: "BEIN SPORTS 3HD",
cat: "Sports",
},
{
id: "122",
title: "Fine Living HD",
cat: "Entertainment",
}
]
}
]
it bring 22 and 122 id while I want only the 22 and when I search for 122 I want it to bring me 122 only
how do this
php api
|
show 5 more comments
I'm trying to create search in RESTful API to search for specific id and this is the code that I write:
<?php
header("Access-Control-Allow-Origin: *");
header("Content-Type: application/json; charset=UTF-8");
include_once 'dbconfig.php';
$database = new Database();
$db = $database->getConnection();
$channel = new Channel($db);
$keywords=isset($_GET["id"]) ? $_GET["id"] : "";
$stmt = $channel->searchChannel($keywords);
$num = $stmt->rowCount();
if($num>0){
$channel_arr["channel"]=array();
while ($row = $stmt->fetch(PDO::FETCH_ASSOC)){
extract($row);
$cat = $row['cat'];
$channel_item = array(
"id" => $id,
"title" => $title,
"cat" => $cat,
);
$stmt2 = $channel->searchChannels($cat);
array_push($channel_arr["channel"], $channel_item);
}
http_response_code(200);
echo json_encode($channel_arr);
}
else{
http_response_code(404);
echo json_encode(
array("message" => "No channels found.")
);
}
class Channel{
private $conn;
private $table_name = "esite_channels";
public $id;
public $title;
public $cat;
public function __construct($db){
$this->conn = $db;
}
function searchChannel($keywords){
$query = "SELECT
e.id, e.title, e.image, e.streamname, e.enable, e.cat, e.app
FROM
" . $this->table_name . " e
WHERE
e.id LIKE ?
";
$stmt = $this->conn->prepare($query);
$keywords=htmlspecialchars(strip_tags($keywords));
$keywords = "%{$keywords}%";
$stmt->bindParam(1, $keywords);
$stmt->execute();
return $stmt;
}
}
?>
it works fine while I search for id 20 with this link:
http://127.0.0.1/api/search.php?id=20
it bring data of that id like this:
[
{
channel: [
{
id: "20",
title: "title",
cat: "Sports",
}
]
}
]
but when change the id for 22 for example:
http://127.0.0.1/api/search.php?id=22
it bring me all items that contain 22 in the id like this:
[
{
channel: [
{
id: "22",
title: "BEIN SPORTS 3HD",
cat: "Sports",
},
{
id: "122",
title: "Fine Living HD",
cat: "Entertainment",
}
]
}
]
it bring 22 and 122 id while I want only the 22 and when I search for 122 I want it to bring me 122 only
how do this
php api
try thisWHERE e.id = ?
– Bhargav Chudasama
Nov 14 '18 at 10:11
why would you use LIKE when you donot want other related results. Try %{$keywords} or {$keywords}% or =. which best suits you.
– Rohan Kumar Shrestha
Nov 14 '18 at 10:14
when you want to retreive that only the ID 22, you must change your query in WHERE e.id LIKE ? to WHERE e.id = ?
– j3thamz
Nov 14 '18 at 10:14
I was writing the answer while you were commenting guys :) All are correct yes.
– Taha Paksu
Nov 14 '18 at 10:15
1
and use like this$keywords = "{$keywords}";
– Bhargav Chudasama
Nov 14 '18 at 10:17
|
show 5 more comments
I'm trying to create search in RESTful API to search for specific id and this is the code that I write:
<?php
header("Access-Control-Allow-Origin: *");
header("Content-Type: application/json; charset=UTF-8");
include_once 'dbconfig.php';
$database = new Database();
$db = $database->getConnection();
$channel = new Channel($db);
$keywords=isset($_GET["id"]) ? $_GET["id"] : "";
$stmt = $channel->searchChannel($keywords);
$num = $stmt->rowCount();
if($num>0){
$channel_arr["channel"]=array();
while ($row = $stmt->fetch(PDO::FETCH_ASSOC)){
extract($row);
$cat = $row['cat'];
$channel_item = array(
"id" => $id,
"title" => $title,
"cat" => $cat,
);
$stmt2 = $channel->searchChannels($cat);
array_push($channel_arr["channel"], $channel_item);
}
http_response_code(200);
echo json_encode($channel_arr);
}
else{
http_response_code(404);
echo json_encode(
array("message" => "No channels found.")
);
}
class Channel{
private $conn;
private $table_name = "esite_channels";
public $id;
public $title;
public $cat;
public function __construct($db){
$this->conn = $db;
}
function searchChannel($keywords){
$query = "SELECT
e.id, e.title, e.image, e.streamname, e.enable, e.cat, e.app
FROM
" . $this->table_name . " e
WHERE
e.id LIKE ?
";
$stmt = $this->conn->prepare($query);
$keywords=htmlspecialchars(strip_tags($keywords));
$keywords = "%{$keywords}%";
$stmt->bindParam(1, $keywords);
$stmt->execute();
return $stmt;
}
}
?>
it works fine while I search for id 20 with this link:
http://127.0.0.1/api/search.php?id=20
it bring data of that id like this:
[
{
channel: [
{
id: "20",
title: "title",
cat: "Sports",
}
]
}
]
but when change the id for 22 for example:
http://127.0.0.1/api/search.php?id=22
it bring me all items that contain 22 in the id like this:
[
{
channel: [
{
id: "22",
title: "BEIN SPORTS 3HD",
cat: "Sports",
},
{
id: "122",
title: "Fine Living HD",
cat: "Entertainment",
}
]
}
]
it bring 22 and 122 id while I want only the 22 and when I search for 122 I want it to bring me 122 only
how do this
php api
I'm trying to create search in RESTful API to search for specific id and this is the code that I write:
<?php
header("Access-Control-Allow-Origin: *");
header("Content-Type: application/json; charset=UTF-8");
include_once 'dbconfig.php';
$database = new Database();
$db = $database->getConnection();
$channel = new Channel($db);
$keywords=isset($_GET["id"]) ? $_GET["id"] : "";
$stmt = $channel->searchChannel($keywords);
$num = $stmt->rowCount();
if($num>0){
$channel_arr["channel"]=array();
while ($row = $stmt->fetch(PDO::FETCH_ASSOC)){
extract($row);
$cat = $row['cat'];
$channel_item = array(
"id" => $id,
"title" => $title,
"cat" => $cat,
);
$stmt2 = $channel->searchChannels($cat);
array_push($channel_arr["channel"], $channel_item);
}
http_response_code(200);
echo json_encode($channel_arr);
}
else{
http_response_code(404);
echo json_encode(
array("message" => "No channels found.")
);
}
class Channel{
private $conn;
private $table_name = "esite_channels";
public $id;
public $title;
public $cat;
public function __construct($db){
$this->conn = $db;
}
function searchChannel($keywords){
$query = "SELECT
e.id, e.title, e.image, e.streamname, e.enable, e.cat, e.app
FROM
" . $this->table_name . " e
WHERE
e.id LIKE ?
";
$stmt = $this->conn->prepare($query);
$keywords=htmlspecialchars(strip_tags($keywords));
$keywords = "%{$keywords}%";
$stmt->bindParam(1, $keywords);
$stmt->execute();
return $stmt;
}
}
?>
it works fine while I search for id 20 with this link:
http://127.0.0.1/api/search.php?id=20
it bring data of that id like this:
[
{
channel: [
{
id: "20",
title: "title",
cat: "Sports",
}
]
}
]
but when change the id for 22 for example:
http://127.0.0.1/api/search.php?id=22
it bring me all items that contain 22 in the id like this:
[
{
channel: [
{
id: "22",
title: "BEIN SPORTS 3HD",
cat: "Sports",
},
{
id: "122",
title: "Fine Living HD",
cat: "Entertainment",
}
]
}
]
it bring 22 and 122 id while I want only the 22 and when I search for 122 I want it to bring me 122 only
how do this
php api
php api
edited Nov 14 '18 at 10:12


Grokify
7,32822337
7,32822337
asked Nov 14 '18 at 10:08
GNDevsGNDevs
16810
16810
try thisWHERE e.id = ?
– Bhargav Chudasama
Nov 14 '18 at 10:11
why would you use LIKE when you donot want other related results. Try %{$keywords} or {$keywords}% or =. which best suits you.
– Rohan Kumar Shrestha
Nov 14 '18 at 10:14
when you want to retreive that only the ID 22, you must change your query in WHERE e.id LIKE ? to WHERE e.id = ?
– j3thamz
Nov 14 '18 at 10:14
I was writing the answer while you were commenting guys :) All are correct yes.
– Taha Paksu
Nov 14 '18 at 10:15
1
and use like this$keywords = "{$keywords}";
– Bhargav Chudasama
Nov 14 '18 at 10:17
|
show 5 more comments
try thisWHERE e.id = ?
– Bhargav Chudasama
Nov 14 '18 at 10:11
why would you use LIKE when you donot want other related results. Try %{$keywords} or {$keywords}% or =. which best suits you.
– Rohan Kumar Shrestha
Nov 14 '18 at 10:14
when you want to retreive that only the ID 22, you must change your query in WHERE e.id LIKE ? to WHERE e.id = ?
– j3thamz
Nov 14 '18 at 10:14
I was writing the answer while you were commenting guys :) All are correct yes.
– Taha Paksu
Nov 14 '18 at 10:15
1
and use like this$keywords = "{$keywords}";
– Bhargav Chudasama
Nov 14 '18 at 10:17
try this
WHERE e.id = ?
– Bhargav Chudasama
Nov 14 '18 at 10:11
try this
WHERE e.id = ?
– Bhargav Chudasama
Nov 14 '18 at 10:11
why would you use LIKE when you donot want other related results. Try %{$keywords} or {$keywords}% or =. which best suits you.
– Rohan Kumar Shrestha
Nov 14 '18 at 10:14
why would you use LIKE when you donot want other related results. Try %{$keywords} or {$keywords}% or =. which best suits you.
– Rohan Kumar Shrestha
Nov 14 '18 at 10:14
when you want to retreive that only the ID 22, you must change your query in WHERE e.id LIKE ? to WHERE e.id = ?
– j3thamz
Nov 14 '18 at 10:14
when you want to retreive that only the ID 22, you must change your query in WHERE e.id LIKE ? to WHERE e.id = ?
– j3thamz
Nov 14 '18 at 10:14
I was writing the answer while you were commenting guys :) All are correct yes.
– Taha Paksu
Nov 14 '18 at 10:15
I was writing the answer while you were commenting guys :) All are correct yes.
– Taha Paksu
Nov 14 '18 at 10:15
1
1
and use like this
$keywords = "{$keywords}";
– Bhargav Chudasama
Nov 14 '18 at 10:17
and use like this
$keywords = "{$keywords}";
– Bhargav Chudasama
Nov 14 '18 at 10:17
|
show 5 more comments
1 Answer
1
active
oldest
votes
Change e.id LIKE ?
to e.id = ?
in your query. And remove this line:
$keywords = "%{$keywords}%";
Because when you use LIKE
with "%keyword%"
structure, LIKE
operator searches for the records containing that value. You can also use the LIKE
operator without the wildcards (e.id LIKE '22'
), you can also achieve what you want. But the direct search is better.
$query = "SELECT
e.id, e.title, e.image, e.streamname, e.enable, e.cat, e.app
FROM
" . $this->table_name . " e
WHERE
e.id = ?
";
$stmt = $this->conn->prepare($query);
$keywords=htmlspecialchars(strip_tags($keywords));
$stmt->bindParam(1, $keywords);
I already tried this but it bring me the same result
– GNDevs
Nov 14 '18 at 10:16
Updated the answer.
– Taha Paksu
Nov 14 '18 at 10:20
thanks it works after I change this $keywords = "%{$keywords}%"; to this $keywords = "{$keywords}";
– GNDevs
Nov 14 '18 at 10:24
1
That's like$keywords = $keywords
. No need for that.
– Taha Paksu
Nov 14 '18 at 10:24
you are right..
– GNDevs
Nov 14 '18 at 10:26
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53297637%2fsearch-for-specific-id-in-rest-api%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Change e.id LIKE ?
to e.id = ?
in your query. And remove this line:
$keywords = "%{$keywords}%";
Because when you use LIKE
with "%keyword%"
structure, LIKE
operator searches for the records containing that value. You can also use the LIKE
operator without the wildcards (e.id LIKE '22'
), you can also achieve what you want. But the direct search is better.
$query = "SELECT
e.id, e.title, e.image, e.streamname, e.enable, e.cat, e.app
FROM
" . $this->table_name . " e
WHERE
e.id = ?
";
$stmt = $this->conn->prepare($query);
$keywords=htmlspecialchars(strip_tags($keywords));
$stmt->bindParam(1, $keywords);
I already tried this but it bring me the same result
– GNDevs
Nov 14 '18 at 10:16
Updated the answer.
– Taha Paksu
Nov 14 '18 at 10:20
thanks it works after I change this $keywords = "%{$keywords}%"; to this $keywords = "{$keywords}";
– GNDevs
Nov 14 '18 at 10:24
1
That's like$keywords = $keywords
. No need for that.
– Taha Paksu
Nov 14 '18 at 10:24
you are right..
– GNDevs
Nov 14 '18 at 10:26
add a comment |
Change e.id LIKE ?
to e.id = ?
in your query. And remove this line:
$keywords = "%{$keywords}%";
Because when you use LIKE
with "%keyword%"
structure, LIKE
operator searches for the records containing that value. You can also use the LIKE
operator without the wildcards (e.id LIKE '22'
), you can also achieve what you want. But the direct search is better.
$query = "SELECT
e.id, e.title, e.image, e.streamname, e.enable, e.cat, e.app
FROM
" . $this->table_name . " e
WHERE
e.id = ?
";
$stmt = $this->conn->prepare($query);
$keywords=htmlspecialchars(strip_tags($keywords));
$stmt->bindParam(1, $keywords);
I already tried this but it bring me the same result
– GNDevs
Nov 14 '18 at 10:16
Updated the answer.
– Taha Paksu
Nov 14 '18 at 10:20
thanks it works after I change this $keywords = "%{$keywords}%"; to this $keywords = "{$keywords}";
– GNDevs
Nov 14 '18 at 10:24
1
That's like$keywords = $keywords
. No need for that.
– Taha Paksu
Nov 14 '18 at 10:24
you are right..
– GNDevs
Nov 14 '18 at 10:26
add a comment |
Change e.id LIKE ?
to e.id = ?
in your query. And remove this line:
$keywords = "%{$keywords}%";
Because when you use LIKE
with "%keyword%"
structure, LIKE
operator searches for the records containing that value. You can also use the LIKE
operator without the wildcards (e.id LIKE '22'
), you can also achieve what you want. But the direct search is better.
$query = "SELECT
e.id, e.title, e.image, e.streamname, e.enable, e.cat, e.app
FROM
" . $this->table_name . " e
WHERE
e.id = ?
";
$stmt = $this->conn->prepare($query);
$keywords=htmlspecialchars(strip_tags($keywords));
$stmt->bindParam(1, $keywords);
Change e.id LIKE ?
to e.id = ?
in your query. And remove this line:
$keywords = "%{$keywords}%";
Because when you use LIKE
with "%keyword%"
structure, LIKE
operator searches for the records containing that value. You can also use the LIKE
operator without the wildcards (e.id LIKE '22'
), you can also achieve what you want. But the direct search is better.
$query = "SELECT
e.id, e.title, e.image, e.streamname, e.enable, e.cat, e.app
FROM
" . $this->table_name . " e
WHERE
e.id = ?
";
$stmt = $this->conn->prepare($query);
$keywords=htmlspecialchars(strip_tags($keywords));
$stmt->bindParam(1, $keywords);
edited Nov 14 '18 at 10:17
answered Nov 14 '18 at 10:14


Taha PaksuTaha Paksu
11.4k12857
11.4k12857
I already tried this but it bring me the same result
– GNDevs
Nov 14 '18 at 10:16
Updated the answer.
– Taha Paksu
Nov 14 '18 at 10:20
thanks it works after I change this $keywords = "%{$keywords}%"; to this $keywords = "{$keywords}";
– GNDevs
Nov 14 '18 at 10:24
1
That's like$keywords = $keywords
. No need for that.
– Taha Paksu
Nov 14 '18 at 10:24
you are right..
– GNDevs
Nov 14 '18 at 10:26
add a comment |
I already tried this but it bring me the same result
– GNDevs
Nov 14 '18 at 10:16
Updated the answer.
– Taha Paksu
Nov 14 '18 at 10:20
thanks it works after I change this $keywords = "%{$keywords}%"; to this $keywords = "{$keywords}";
– GNDevs
Nov 14 '18 at 10:24
1
That's like$keywords = $keywords
. No need for that.
– Taha Paksu
Nov 14 '18 at 10:24
you are right..
– GNDevs
Nov 14 '18 at 10:26
I already tried this but it bring me the same result
– GNDevs
Nov 14 '18 at 10:16
I already tried this but it bring me the same result
– GNDevs
Nov 14 '18 at 10:16
Updated the answer.
– Taha Paksu
Nov 14 '18 at 10:20
Updated the answer.
– Taha Paksu
Nov 14 '18 at 10:20
thanks it works after I change this $keywords = "%{$keywords}%"; to this $keywords = "{$keywords}";
– GNDevs
Nov 14 '18 at 10:24
thanks it works after I change this $keywords = "%{$keywords}%"; to this $keywords = "{$keywords}";
– GNDevs
Nov 14 '18 at 10:24
1
1
That's like
$keywords = $keywords
. No need for that.– Taha Paksu
Nov 14 '18 at 10:24
That's like
$keywords = $keywords
. No need for that.– Taha Paksu
Nov 14 '18 at 10:24
you are right..
– GNDevs
Nov 14 '18 at 10:26
you are right..
– GNDevs
Nov 14 '18 at 10:26
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53297637%2fsearch-for-specific-id-in-rest-api%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
efRibFW34 6Nj
try this
WHERE e.id = ?
– Bhargav Chudasama
Nov 14 '18 at 10:11
why would you use LIKE when you donot want other related results. Try %{$keywords} or {$keywords}% or =. which best suits you.
– Rohan Kumar Shrestha
Nov 14 '18 at 10:14
when you want to retreive that only the ID 22, you must change your query in WHERE e.id LIKE ? to WHERE e.id = ?
– j3thamz
Nov 14 '18 at 10:14
I was writing the answer while you were commenting guys :) All are correct yes.
– Taha Paksu
Nov 14 '18 at 10:15
1
and use like this
$keywords = "{$keywords}";
– Bhargav Chudasama
Nov 14 '18 at 10:17