Most efficient way to get elements of specific XML nodes
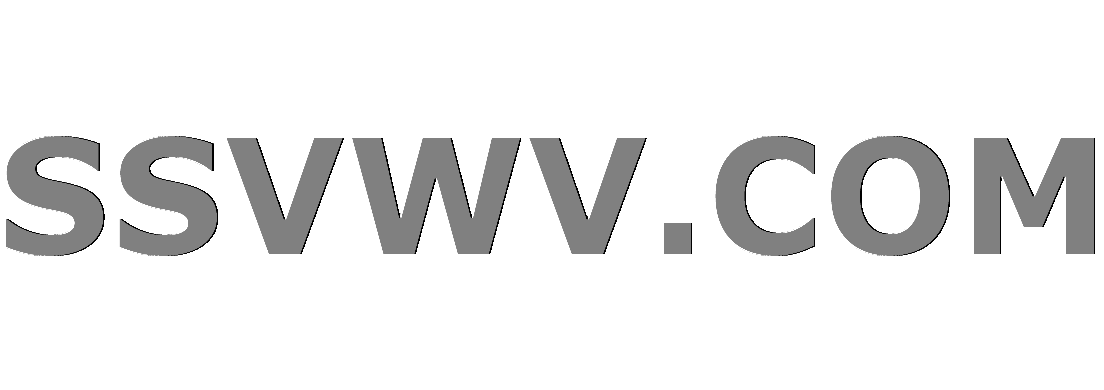
Multi tool use
What is the most efficiant way to get XML nodes of a specific parent each time?
Let's assume i have a XML that contains peoples' names, and each name node has favorite food's nodes and favorite drinks' node.
I have a system that let the user choose the people he want to get data for, and the system querying it by name and show it to the user after button click.
So is it better to split the main XML to xml for each person at the beginning of the program and then run just on the relevant person's xml, or maybe use doc.GetElementsByTagName("food")
and doc.GetElementsByTagName("drink")
by using some ifother conditions?
Thanks!!
XML example:
The user can choose "David" and get a list of his fave food and drinks, then choose John... etc.
<root>
<David>
<FAVE_FOOD>
<food value = "hamburger"/>
<food value = "banana"/>
</FAVE_FOOD>
<FAVE_Drinks>
<drink value = "water"/>
</FAVE_Drinks>
</David>
<John>
<FAVE_FOOD>
<food value = "icecream"/>
</FAVE_FOOD>
<FAVE_Drinks>
<drink value = "7up"/>
</FAVE_Drinks>
</John>
etc...
</root>
c# xml performance
add a comment |
What is the most efficiant way to get XML nodes of a specific parent each time?
Let's assume i have a XML that contains peoples' names, and each name node has favorite food's nodes and favorite drinks' node.
I have a system that let the user choose the people he want to get data for, and the system querying it by name and show it to the user after button click.
So is it better to split the main XML to xml for each person at the beginning of the program and then run just on the relevant person's xml, or maybe use doc.GetElementsByTagName("food")
and doc.GetElementsByTagName("drink")
by using some ifother conditions?
Thanks!!
XML example:
The user can choose "David" and get a list of his fave food and drinks, then choose John... etc.
<root>
<David>
<FAVE_FOOD>
<food value = "hamburger"/>
<food value = "banana"/>
</FAVE_FOOD>
<FAVE_Drinks>
<drink value = "water"/>
</FAVE_Drinks>
</David>
<John>
<FAVE_FOOD>
<food value = "icecream"/>
</FAVE_FOOD>
<FAVE_Drinks>
<drink value = "7up"/>
</FAVE_Drinks>
</John>
etc...
</root>
c# xml performance
1
Most efficient way is to store the xml tree in your own structure type, that uses a dictionary to store the root and it's children.
– Rango
Nov 14 '18 at 9:21
add a comment |
What is the most efficiant way to get XML nodes of a specific parent each time?
Let's assume i have a XML that contains peoples' names, and each name node has favorite food's nodes and favorite drinks' node.
I have a system that let the user choose the people he want to get data for, and the system querying it by name and show it to the user after button click.
So is it better to split the main XML to xml for each person at the beginning of the program and then run just on the relevant person's xml, or maybe use doc.GetElementsByTagName("food")
and doc.GetElementsByTagName("drink")
by using some ifother conditions?
Thanks!!
XML example:
The user can choose "David" and get a list of his fave food and drinks, then choose John... etc.
<root>
<David>
<FAVE_FOOD>
<food value = "hamburger"/>
<food value = "banana"/>
</FAVE_FOOD>
<FAVE_Drinks>
<drink value = "water"/>
</FAVE_Drinks>
</David>
<John>
<FAVE_FOOD>
<food value = "icecream"/>
</FAVE_FOOD>
<FAVE_Drinks>
<drink value = "7up"/>
</FAVE_Drinks>
</John>
etc...
</root>
c# xml performance
What is the most efficiant way to get XML nodes of a specific parent each time?
Let's assume i have a XML that contains peoples' names, and each name node has favorite food's nodes and favorite drinks' node.
I have a system that let the user choose the people he want to get data for, and the system querying it by name and show it to the user after button click.
So is it better to split the main XML to xml for each person at the beginning of the program and then run just on the relevant person's xml, or maybe use doc.GetElementsByTagName("food")
and doc.GetElementsByTagName("drink")
by using some ifother conditions?
Thanks!!
XML example:
The user can choose "David" and get a list of his fave food and drinks, then choose John... etc.
<root>
<David>
<FAVE_FOOD>
<food value = "hamburger"/>
<food value = "banana"/>
</FAVE_FOOD>
<FAVE_Drinks>
<drink value = "water"/>
</FAVE_Drinks>
</David>
<John>
<FAVE_FOOD>
<food value = "icecream"/>
</FAVE_FOOD>
<FAVE_Drinks>
<drink value = "7up"/>
</FAVE_Drinks>
</John>
etc...
</root>
c# xml performance
c# xml performance
asked Nov 14 '18 at 9:18


BenBen
671110
671110
1
Most efficient way is to store the xml tree in your own structure type, that uses a dictionary to store the root and it's children.
– Rango
Nov 14 '18 at 9:21
add a comment |
1
Most efficient way is to store the xml tree in your own structure type, that uses a dictionary to store the root and it's children.
– Rango
Nov 14 '18 at 9:21
1
1
Most efficient way is to store the xml tree in your own structure type, that uses a dictionary to store the root and it's children.
– Rango
Nov 14 '18 at 9:21
Most efficient way is to store the xml tree in your own structure type, that uses a dictionary to store the root and it's children.
– Rango
Nov 14 '18 at 9:21
add a comment |
2 Answers
2
active
oldest
votes
One way is to parse your XML with XDocument
.
Here i created a custom function GetUserData
. You need to pass username
like David
to this function and then this function can return you the list of food names and list of drink name.
Console app for demonstration purpose.
class Program
{
static void Main(string args)
{
XDocument doc = XDocument.Load(@"Your path to xml"); //<= Load xml
FoodData foodData = GetUserData(doc, "David"); //<= Pass "David" to function
foodData.FoodNames.ForEach(x => Console.WriteLine("Food: " + x)); //<= Print food name list
Console.WriteLine();
foodData.DrinkNames.ForEach(x => Console.WriteLine("Drink: " + x)); //<= Print drink name list
Console.ReadLine();
}
public static FoodData GetUserData(XDocument doc, string userName)
{
var data = doc.Root.Elements(userName).Elements();
FoodData foodData = new FoodData
{
FoodNames = data.Where(x => x.Name == "FAVE_FOOD").Descendants().Select(x => x.Attribute("value").Value).ToList(),
DrinkNames = data.Where(x => x.Name == "FAVE_Drinks").Descendants().Select(x => x.Attribute("value").Value).ToList()
};
return foodData;
}
}
public class FoodData
{
public List<string> FoodNames { get; set; }
public List<string> DrinkNames { get; set; }
}
Output:
Edit:
If you want to get all the food and drinks in one list (don't separate it to food list and drinks list).
Then simply get all food data and drink data into one list and return that list like
public static List<string> GetUserData(XDocument doc, string userName)
{
List<string> data = doc.Root.Elements(userName).Elements().Elements().Select(x => x.Attribute("value").Value).ToList();
return data;
}
And you can call above method like.
List<string> data = GetUserData(doc, "David");
Great idea, thanks for that! Is it efficient? I mean how long each name check operation should take (Let's say i have around 50-60 food and drinks values for each person).
– Ben
Nov 14 '18 at 12:32
let me check it with 100 foods and drinks. I'll update you in 5 min
– er-mfahhgk
Nov 14 '18 at 12:34
i'd check with 100 foods and 100 drinks forDavid
and it take only 2-4 miliseconds to fetch on my side :)
– er-mfahhgk
Nov 14 '18 at 12:39
I rewriteGetUserData
method to one more step to efficiency that we collect all the food data at once and then we apply filter to get food and drink names separately. plz view updated answer :)
– er-mfahhgk
Nov 14 '18 at 12:48
Thank you very much!!
– Ben
Nov 14 '18 at 13:04
|
show 4 more comments
I like using xml linq and putting into a dictionary with the key being the people names
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Xml;
using System.Xml.Linq;
namespace ConsoleApplication83
{
class Program
{
const string FILENAME = @"c:temptest.xml";
static void Main(string args)
{
XDocument doc = XDocument.Load(FILENAME);
Dictionary<string, object> dict = doc.Root.Elements()
.GroupBy(x => x.Name.LocalName, y => new
{
drinks = y.Descendants("drink").Select(z => (string)z.Attribute("value")).ToList(),
foods = y.Descendants("food").Select(z => (string)z.Attribute("value")).ToList()
})
.ToDictionary(x => x.Key, y => (object)y.FirstOrDefault());
}
}
}
Thanks for the reply!! And what will the value be.? object of what?
– Ben
Nov 14 '18 at 12:29
All the data put into a dictionary.
– jdweng
Nov 14 '18 at 12:46
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53296669%2fmost-efficient-way-to-get-elements-of-specific-xml-nodes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
One way is to parse your XML with XDocument
.
Here i created a custom function GetUserData
. You need to pass username
like David
to this function and then this function can return you the list of food names and list of drink name.
Console app for demonstration purpose.
class Program
{
static void Main(string args)
{
XDocument doc = XDocument.Load(@"Your path to xml"); //<= Load xml
FoodData foodData = GetUserData(doc, "David"); //<= Pass "David" to function
foodData.FoodNames.ForEach(x => Console.WriteLine("Food: " + x)); //<= Print food name list
Console.WriteLine();
foodData.DrinkNames.ForEach(x => Console.WriteLine("Drink: " + x)); //<= Print drink name list
Console.ReadLine();
}
public static FoodData GetUserData(XDocument doc, string userName)
{
var data = doc.Root.Elements(userName).Elements();
FoodData foodData = new FoodData
{
FoodNames = data.Where(x => x.Name == "FAVE_FOOD").Descendants().Select(x => x.Attribute("value").Value).ToList(),
DrinkNames = data.Where(x => x.Name == "FAVE_Drinks").Descendants().Select(x => x.Attribute("value").Value).ToList()
};
return foodData;
}
}
public class FoodData
{
public List<string> FoodNames { get; set; }
public List<string> DrinkNames { get; set; }
}
Output:
Edit:
If you want to get all the food and drinks in one list (don't separate it to food list and drinks list).
Then simply get all food data and drink data into one list and return that list like
public static List<string> GetUserData(XDocument doc, string userName)
{
List<string> data = doc.Root.Elements(userName).Elements().Elements().Select(x => x.Attribute("value").Value).ToList();
return data;
}
And you can call above method like.
List<string> data = GetUserData(doc, "David");
Great idea, thanks for that! Is it efficient? I mean how long each name check operation should take (Let's say i have around 50-60 food and drinks values for each person).
– Ben
Nov 14 '18 at 12:32
let me check it with 100 foods and drinks. I'll update you in 5 min
– er-mfahhgk
Nov 14 '18 at 12:34
i'd check with 100 foods and 100 drinks forDavid
and it take only 2-4 miliseconds to fetch on my side :)
– er-mfahhgk
Nov 14 '18 at 12:39
I rewriteGetUserData
method to one more step to efficiency that we collect all the food data at once and then we apply filter to get food and drink names separately. plz view updated answer :)
– er-mfahhgk
Nov 14 '18 at 12:48
Thank you very much!!
– Ben
Nov 14 '18 at 13:04
|
show 4 more comments
One way is to parse your XML with XDocument
.
Here i created a custom function GetUserData
. You need to pass username
like David
to this function and then this function can return you the list of food names and list of drink name.
Console app for demonstration purpose.
class Program
{
static void Main(string args)
{
XDocument doc = XDocument.Load(@"Your path to xml"); //<= Load xml
FoodData foodData = GetUserData(doc, "David"); //<= Pass "David" to function
foodData.FoodNames.ForEach(x => Console.WriteLine("Food: " + x)); //<= Print food name list
Console.WriteLine();
foodData.DrinkNames.ForEach(x => Console.WriteLine("Drink: " + x)); //<= Print drink name list
Console.ReadLine();
}
public static FoodData GetUserData(XDocument doc, string userName)
{
var data = doc.Root.Elements(userName).Elements();
FoodData foodData = new FoodData
{
FoodNames = data.Where(x => x.Name == "FAVE_FOOD").Descendants().Select(x => x.Attribute("value").Value).ToList(),
DrinkNames = data.Where(x => x.Name == "FAVE_Drinks").Descendants().Select(x => x.Attribute("value").Value).ToList()
};
return foodData;
}
}
public class FoodData
{
public List<string> FoodNames { get; set; }
public List<string> DrinkNames { get; set; }
}
Output:
Edit:
If you want to get all the food and drinks in one list (don't separate it to food list and drinks list).
Then simply get all food data and drink data into one list and return that list like
public static List<string> GetUserData(XDocument doc, string userName)
{
List<string> data = doc.Root.Elements(userName).Elements().Elements().Select(x => x.Attribute("value").Value).ToList();
return data;
}
And you can call above method like.
List<string> data = GetUserData(doc, "David");
Great idea, thanks for that! Is it efficient? I mean how long each name check operation should take (Let's say i have around 50-60 food and drinks values for each person).
– Ben
Nov 14 '18 at 12:32
let me check it with 100 foods and drinks. I'll update you in 5 min
– er-mfahhgk
Nov 14 '18 at 12:34
i'd check with 100 foods and 100 drinks forDavid
and it take only 2-4 miliseconds to fetch on my side :)
– er-mfahhgk
Nov 14 '18 at 12:39
I rewriteGetUserData
method to one more step to efficiency that we collect all the food data at once and then we apply filter to get food and drink names separately. plz view updated answer :)
– er-mfahhgk
Nov 14 '18 at 12:48
Thank you very much!!
– Ben
Nov 14 '18 at 13:04
|
show 4 more comments
One way is to parse your XML with XDocument
.
Here i created a custom function GetUserData
. You need to pass username
like David
to this function and then this function can return you the list of food names and list of drink name.
Console app for demonstration purpose.
class Program
{
static void Main(string args)
{
XDocument doc = XDocument.Load(@"Your path to xml"); //<= Load xml
FoodData foodData = GetUserData(doc, "David"); //<= Pass "David" to function
foodData.FoodNames.ForEach(x => Console.WriteLine("Food: " + x)); //<= Print food name list
Console.WriteLine();
foodData.DrinkNames.ForEach(x => Console.WriteLine("Drink: " + x)); //<= Print drink name list
Console.ReadLine();
}
public static FoodData GetUserData(XDocument doc, string userName)
{
var data = doc.Root.Elements(userName).Elements();
FoodData foodData = new FoodData
{
FoodNames = data.Where(x => x.Name == "FAVE_FOOD").Descendants().Select(x => x.Attribute("value").Value).ToList(),
DrinkNames = data.Where(x => x.Name == "FAVE_Drinks").Descendants().Select(x => x.Attribute("value").Value).ToList()
};
return foodData;
}
}
public class FoodData
{
public List<string> FoodNames { get; set; }
public List<string> DrinkNames { get; set; }
}
Output:
Edit:
If you want to get all the food and drinks in one list (don't separate it to food list and drinks list).
Then simply get all food data and drink data into one list and return that list like
public static List<string> GetUserData(XDocument doc, string userName)
{
List<string> data = doc.Root.Elements(userName).Elements().Elements().Select(x => x.Attribute("value").Value).ToList();
return data;
}
And you can call above method like.
List<string> data = GetUserData(doc, "David");
One way is to parse your XML with XDocument
.
Here i created a custom function GetUserData
. You need to pass username
like David
to this function and then this function can return you the list of food names and list of drink name.
Console app for demonstration purpose.
class Program
{
static void Main(string args)
{
XDocument doc = XDocument.Load(@"Your path to xml"); //<= Load xml
FoodData foodData = GetUserData(doc, "David"); //<= Pass "David" to function
foodData.FoodNames.ForEach(x => Console.WriteLine("Food: " + x)); //<= Print food name list
Console.WriteLine();
foodData.DrinkNames.ForEach(x => Console.WriteLine("Drink: " + x)); //<= Print drink name list
Console.ReadLine();
}
public static FoodData GetUserData(XDocument doc, string userName)
{
var data = doc.Root.Elements(userName).Elements();
FoodData foodData = new FoodData
{
FoodNames = data.Where(x => x.Name == "FAVE_FOOD").Descendants().Select(x => x.Attribute("value").Value).ToList(),
DrinkNames = data.Where(x => x.Name == "FAVE_Drinks").Descendants().Select(x => x.Attribute("value").Value).ToList()
};
return foodData;
}
}
public class FoodData
{
public List<string> FoodNames { get; set; }
public List<string> DrinkNames { get; set; }
}
Output:
Edit:
If you want to get all the food and drinks in one list (don't separate it to food list and drinks list).
Then simply get all food data and drink data into one list and return that list like
public static List<string> GetUserData(XDocument doc, string userName)
{
List<string> data = doc.Root.Elements(userName).Elements().Elements().Select(x => x.Attribute("value").Value).ToList();
return data;
}
And you can call above method like.
List<string> data = GetUserData(doc, "David");
edited Nov 15 '18 at 9:17
answered Nov 14 '18 at 10:24
er-mfahhgker-mfahhgk
5,5872616
5,5872616
Great idea, thanks for that! Is it efficient? I mean how long each name check operation should take (Let's say i have around 50-60 food and drinks values for each person).
– Ben
Nov 14 '18 at 12:32
let me check it with 100 foods and drinks. I'll update you in 5 min
– er-mfahhgk
Nov 14 '18 at 12:34
i'd check with 100 foods and 100 drinks forDavid
and it take only 2-4 miliseconds to fetch on my side :)
– er-mfahhgk
Nov 14 '18 at 12:39
I rewriteGetUserData
method to one more step to efficiency that we collect all the food data at once and then we apply filter to get food and drink names separately. plz view updated answer :)
– er-mfahhgk
Nov 14 '18 at 12:48
Thank you very much!!
– Ben
Nov 14 '18 at 13:04
|
show 4 more comments
Great idea, thanks for that! Is it efficient? I mean how long each name check operation should take (Let's say i have around 50-60 food and drinks values for each person).
– Ben
Nov 14 '18 at 12:32
let me check it with 100 foods and drinks. I'll update you in 5 min
– er-mfahhgk
Nov 14 '18 at 12:34
i'd check with 100 foods and 100 drinks forDavid
and it take only 2-4 miliseconds to fetch on my side :)
– er-mfahhgk
Nov 14 '18 at 12:39
I rewriteGetUserData
method to one more step to efficiency that we collect all the food data at once and then we apply filter to get food and drink names separately. plz view updated answer :)
– er-mfahhgk
Nov 14 '18 at 12:48
Thank you very much!!
– Ben
Nov 14 '18 at 13:04
Great idea, thanks for that! Is it efficient? I mean how long each name check operation should take (Let's say i have around 50-60 food and drinks values for each person).
– Ben
Nov 14 '18 at 12:32
Great idea, thanks for that! Is it efficient? I mean how long each name check operation should take (Let's say i have around 50-60 food and drinks values for each person).
– Ben
Nov 14 '18 at 12:32
let me check it with 100 foods and drinks. I'll update you in 5 min
– er-mfahhgk
Nov 14 '18 at 12:34
let me check it with 100 foods and drinks. I'll update you in 5 min
– er-mfahhgk
Nov 14 '18 at 12:34
i'd check with 100 foods and 100 drinks for
David
and it take only 2-4 miliseconds to fetch on my side :)– er-mfahhgk
Nov 14 '18 at 12:39
i'd check with 100 foods and 100 drinks for
David
and it take only 2-4 miliseconds to fetch on my side :)– er-mfahhgk
Nov 14 '18 at 12:39
I rewrite
GetUserData
method to one more step to efficiency that we collect all the food data at once and then we apply filter to get food and drink names separately. plz view updated answer :)– er-mfahhgk
Nov 14 '18 at 12:48
I rewrite
GetUserData
method to one more step to efficiency that we collect all the food data at once and then we apply filter to get food and drink names separately. plz view updated answer :)– er-mfahhgk
Nov 14 '18 at 12:48
Thank you very much!!
– Ben
Nov 14 '18 at 13:04
Thank you very much!!
– Ben
Nov 14 '18 at 13:04
|
show 4 more comments
I like using xml linq and putting into a dictionary with the key being the people names
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Xml;
using System.Xml.Linq;
namespace ConsoleApplication83
{
class Program
{
const string FILENAME = @"c:temptest.xml";
static void Main(string args)
{
XDocument doc = XDocument.Load(FILENAME);
Dictionary<string, object> dict = doc.Root.Elements()
.GroupBy(x => x.Name.LocalName, y => new
{
drinks = y.Descendants("drink").Select(z => (string)z.Attribute("value")).ToList(),
foods = y.Descendants("food").Select(z => (string)z.Attribute("value")).ToList()
})
.ToDictionary(x => x.Key, y => (object)y.FirstOrDefault());
}
}
}
Thanks for the reply!! And what will the value be.? object of what?
– Ben
Nov 14 '18 at 12:29
All the data put into a dictionary.
– jdweng
Nov 14 '18 at 12:46
add a comment |
I like using xml linq and putting into a dictionary with the key being the people names
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Xml;
using System.Xml.Linq;
namespace ConsoleApplication83
{
class Program
{
const string FILENAME = @"c:temptest.xml";
static void Main(string args)
{
XDocument doc = XDocument.Load(FILENAME);
Dictionary<string, object> dict = doc.Root.Elements()
.GroupBy(x => x.Name.LocalName, y => new
{
drinks = y.Descendants("drink").Select(z => (string)z.Attribute("value")).ToList(),
foods = y.Descendants("food").Select(z => (string)z.Attribute("value")).ToList()
})
.ToDictionary(x => x.Key, y => (object)y.FirstOrDefault());
}
}
}
Thanks for the reply!! And what will the value be.? object of what?
– Ben
Nov 14 '18 at 12:29
All the data put into a dictionary.
– jdweng
Nov 14 '18 at 12:46
add a comment |
I like using xml linq and putting into a dictionary with the key being the people names
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Xml;
using System.Xml.Linq;
namespace ConsoleApplication83
{
class Program
{
const string FILENAME = @"c:temptest.xml";
static void Main(string args)
{
XDocument doc = XDocument.Load(FILENAME);
Dictionary<string, object> dict = doc.Root.Elements()
.GroupBy(x => x.Name.LocalName, y => new
{
drinks = y.Descendants("drink").Select(z => (string)z.Attribute("value")).ToList(),
foods = y.Descendants("food").Select(z => (string)z.Attribute("value")).ToList()
})
.ToDictionary(x => x.Key, y => (object)y.FirstOrDefault());
}
}
}
I like using xml linq and putting into a dictionary with the key being the people names
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Xml;
using System.Xml.Linq;
namespace ConsoleApplication83
{
class Program
{
const string FILENAME = @"c:temptest.xml";
static void Main(string args)
{
XDocument doc = XDocument.Load(FILENAME);
Dictionary<string, object> dict = doc.Root.Elements()
.GroupBy(x => x.Name.LocalName, y => new
{
drinks = y.Descendants("drink").Select(z => (string)z.Attribute("value")).ToList(),
foods = y.Descendants("food").Select(z => (string)z.Attribute("value")).ToList()
})
.ToDictionary(x => x.Key, y => (object)y.FirstOrDefault());
}
}
}
answered Nov 14 '18 at 10:51
jdwengjdweng
17.2k2717
17.2k2717
Thanks for the reply!! And what will the value be.? object of what?
– Ben
Nov 14 '18 at 12:29
All the data put into a dictionary.
– jdweng
Nov 14 '18 at 12:46
add a comment |
Thanks for the reply!! And what will the value be.? object of what?
– Ben
Nov 14 '18 at 12:29
All the data put into a dictionary.
– jdweng
Nov 14 '18 at 12:46
Thanks for the reply!! And what will the value be.? object of what?
– Ben
Nov 14 '18 at 12:29
Thanks for the reply!! And what will the value be.? object of what?
– Ben
Nov 14 '18 at 12:29
All the data put into a dictionary.
– jdweng
Nov 14 '18 at 12:46
All the data put into a dictionary.
– jdweng
Nov 14 '18 at 12:46
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53296669%2fmost-efficient-way-to-get-elements-of-specific-xml-nodes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Gddmuyguoc,d
1
Most efficient way is to store the xml tree in your own structure type, that uses a dictionary to store the root and it's children.
– Rango
Nov 14 '18 at 9:21