Entity Framework Generic insert method is inserting already existing entity again along with the new entity
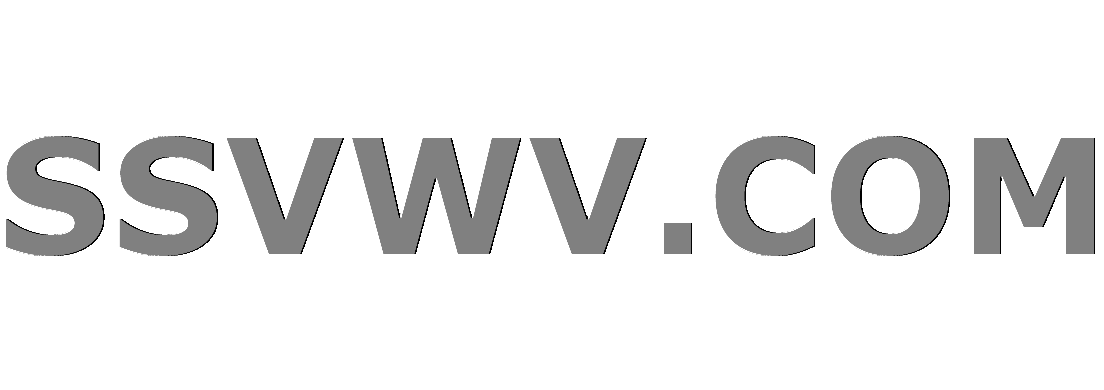
Multi tool use
I have following Insert method:
public static bool Insert<T>(T item) where T : class
{
using (ApplicationDbContext ctx = new ApplicationDbContext())
{
try
{
ctx.Set<T>().Add(item);
ctx.SaveChanges();
return true;
}
catch (Exception ex)
{
// ...
}
}
}
This works as expected but when i want to insert a new entity, which has a relation to a existing entity, EF is re-inserting this relational (already existing) entity again along with the new entity.
Details:
I have a entity Supplier which already exists in my database.
I want to insert a new entity Product which has this existing Supplier entity as relation so i retrieve this Supplier from the database and add it to this Product Entity. When i insert it using the generic method, it re-inserts this Supplier and obviously i don't want this behavior.
Am i doing something wrong here or is this by design and should i not use a generic insert function when having relational entities attached to my new entities?
Thank you for any suggestion or information!
Kind regards
EDIT:
Product Entity:
// ... non relational properties
public ICollection<Price> Prices { get; set; }
public ICollection<Supplier> Suppliers { get; set; }
public ICollection<Productnumber> ProductNumbers { get; set; }
Price Entity:
public Product Product { get; set; }
public Supplier Supplier { get; set; }
Supplier Entity:
public ICollection<Productnumber> ProductNumbers { get; set; }
public ICollection<Product> Products { get; set; }
public ICollection<Price> Prices { get; set; }
ProductNumber Entity:
public Supplier Supplier { get; set; }
public Product Product { get; set; }
How should i proceed to insert a new product? Is this possible having this structure and using a Generic insert?
c# entity-framework insert duplicates relational-database
add a comment |
I have following Insert method:
public static bool Insert<T>(T item) where T : class
{
using (ApplicationDbContext ctx = new ApplicationDbContext())
{
try
{
ctx.Set<T>().Add(item);
ctx.SaveChanges();
return true;
}
catch (Exception ex)
{
// ...
}
}
}
This works as expected but when i want to insert a new entity, which has a relation to a existing entity, EF is re-inserting this relational (already existing) entity again along with the new entity.
Details:
I have a entity Supplier which already exists in my database.
I want to insert a new entity Product which has this existing Supplier entity as relation so i retrieve this Supplier from the database and add it to this Product Entity. When i insert it using the generic method, it re-inserts this Supplier and obviously i don't want this behavior.
Am i doing something wrong here or is this by design and should i not use a generic insert function when having relational entities attached to my new entities?
Thank you for any suggestion or information!
Kind regards
EDIT:
Product Entity:
// ... non relational properties
public ICollection<Price> Prices { get; set; }
public ICollection<Supplier> Suppliers { get; set; }
public ICollection<Productnumber> ProductNumbers { get; set; }
Price Entity:
public Product Product { get; set; }
public Supplier Supplier { get; set; }
Supplier Entity:
public ICollection<Productnumber> ProductNumbers { get; set; }
public ICollection<Product> Products { get; set; }
public ICollection<Price> Prices { get; set; }
ProductNumber Entity:
public Supplier Supplier { get; set; }
public Product Product { get; set; }
How should i proceed to insert a new product? Is this possible having this structure and using a Generic insert?
c# entity-framework insert duplicates relational-database
add a comment |
I have following Insert method:
public static bool Insert<T>(T item) where T : class
{
using (ApplicationDbContext ctx = new ApplicationDbContext())
{
try
{
ctx.Set<T>().Add(item);
ctx.SaveChanges();
return true;
}
catch (Exception ex)
{
// ...
}
}
}
This works as expected but when i want to insert a new entity, which has a relation to a existing entity, EF is re-inserting this relational (already existing) entity again along with the new entity.
Details:
I have a entity Supplier which already exists in my database.
I want to insert a new entity Product which has this existing Supplier entity as relation so i retrieve this Supplier from the database and add it to this Product Entity. When i insert it using the generic method, it re-inserts this Supplier and obviously i don't want this behavior.
Am i doing something wrong here or is this by design and should i not use a generic insert function when having relational entities attached to my new entities?
Thank you for any suggestion or information!
Kind regards
EDIT:
Product Entity:
// ... non relational properties
public ICollection<Price> Prices { get; set; }
public ICollection<Supplier> Suppliers { get; set; }
public ICollection<Productnumber> ProductNumbers { get; set; }
Price Entity:
public Product Product { get; set; }
public Supplier Supplier { get; set; }
Supplier Entity:
public ICollection<Productnumber> ProductNumbers { get; set; }
public ICollection<Product> Products { get; set; }
public ICollection<Price> Prices { get; set; }
ProductNumber Entity:
public Supplier Supplier { get; set; }
public Product Product { get; set; }
How should i proceed to insert a new product? Is this possible having this structure and using a Generic insert?
c# entity-framework insert duplicates relational-database
I have following Insert method:
public static bool Insert<T>(T item) where T : class
{
using (ApplicationDbContext ctx = new ApplicationDbContext())
{
try
{
ctx.Set<T>().Add(item);
ctx.SaveChanges();
return true;
}
catch (Exception ex)
{
// ...
}
}
}
This works as expected but when i want to insert a new entity, which has a relation to a existing entity, EF is re-inserting this relational (already existing) entity again along with the new entity.
Details:
I have a entity Supplier which already exists in my database.
I want to insert a new entity Product which has this existing Supplier entity as relation so i retrieve this Supplier from the database and add it to this Product Entity. When i insert it using the generic method, it re-inserts this Supplier and obviously i don't want this behavior.
Am i doing something wrong here or is this by design and should i not use a generic insert function when having relational entities attached to my new entities?
Thank you for any suggestion or information!
Kind regards
EDIT:
Product Entity:
// ... non relational properties
public ICollection<Price> Prices { get; set; }
public ICollection<Supplier> Suppliers { get; set; }
public ICollection<Productnumber> ProductNumbers { get; set; }
Price Entity:
public Product Product { get; set; }
public Supplier Supplier { get; set; }
Supplier Entity:
public ICollection<Productnumber> ProductNumbers { get; set; }
public ICollection<Product> Products { get; set; }
public ICollection<Price> Prices { get; set; }
ProductNumber Entity:
public Supplier Supplier { get; set; }
public Product Product { get; set; }
How should i proceed to insert a new product? Is this possible having this structure and using a Generic insert?
c# entity-framework insert duplicates relational-database
c# entity-framework insert duplicates relational-database
edited Nov 14 '18 at 12:54
Dimitri
asked Nov 14 '18 at 9:26


DimitriDimitri
170313
170313
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
If you want to add a new product to an existing Supplier you need to do as following:
1- Retrieve the supplier entity, let's call it sup
2- Add the new Product to it,
sup.Product = new Product{properties
...}
3- Update the supplier entity,
ctx.Entry(sup).State = EntityState.Modified;
ctx.SaveChanges();
You were adding a new supplier entity each time you were using the bool Insert<T> method
and this why you got unexpected behaviour, so just update the existing entry instead.
Hope this helps,
Oh my, this makes sense. But then again, if i have 2 relational entities for my Product (Supplier and Contract lets say). Would this still work, and not inserting a duplicate Contract? Seems strange to insert a new Product by updating a supplier :) Will try this in a moment and post my feedback. Thank you for your suggestion @Karoui Haithem!
– Dimitri
Nov 14 '18 at 10:42
1
yep, indeed, it works that way and its full of sense, if you want to link newEntityA to ExistingEntityB you retrive B and create a related A to it and then update B, same if you have A related to B to C ...
– Karoui Haithem
Nov 14 '18 at 10:51
So i have done what you described, when creating a new Product, i retrieve the supplier from database (which was selected in the UI to attach to this new product). I assign properties and so on to my new product and then i add this new product to the Suppliers.Products list property and save it. This indeed creates my new Product Entity but still is duplicating my supplier... Maybe it is another relational entity causing this? I will update my OP with my Entity details.(Thank you so far!)
– Dimitri
Nov 14 '18 at 11:15
1
put the code which does what you just described, i am not sure but i think that you missed updating the entity (ctx.Entry(yourEntity).State = EntityState.Modified;)
– Karoui Haithem
Nov 14 '18 at 11:20
1
you can post your last comment in a new question and link it here then i will post an answer showing how you can use IRepository pattern with generics to handle basic CRUD operation with EF
– Karoui Haithem
Nov 14 '18 at 16:51
|
show 2 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53296818%2fentity-framework-generic-insert-method-is-inserting-already-existing-entity-agai%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you want to add a new product to an existing Supplier you need to do as following:
1- Retrieve the supplier entity, let's call it sup
2- Add the new Product to it,
sup.Product = new Product{properties
...}
3- Update the supplier entity,
ctx.Entry(sup).State = EntityState.Modified;
ctx.SaveChanges();
You were adding a new supplier entity each time you were using the bool Insert<T> method
and this why you got unexpected behaviour, so just update the existing entry instead.
Hope this helps,
Oh my, this makes sense. But then again, if i have 2 relational entities for my Product (Supplier and Contract lets say). Would this still work, and not inserting a duplicate Contract? Seems strange to insert a new Product by updating a supplier :) Will try this in a moment and post my feedback. Thank you for your suggestion @Karoui Haithem!
– Dimitri
Nov 14 '18 at 10:42
1
yep, indeed, it works that way and its full of sense, if you want to link newEntityA to ExistingEntityB you retrive B and create a related A to it and then update B, same if you have A related to B to C ...
– Karoui Haithem
Nov 14 '18 at 10:51
So i have done what you described, when creating a new Product, i retrieve the supplier from database (which was selected in the UI to attach to this new product). I assign properties and so on to my new product and then i add this new product to the Suppliers.Products list property and save it. This indeed creates my new Product Entity but still is duplicating my supplier... Maybe it is another relational entity causing this? I will update my OP with my Entity details.(Thank you so far!)
– Dimitri
Nov 14 '18 at 11:15
1
put the code which does what you just described, i am not sure but i think that you missed updating the entity (ctx.Entry(yourEntity).State = EntityState.Modified;)
– Karoui Haithem
Nov 14 '18 at 11:20
1
you can post your last comment in a new question and link it here then i will post an answer showing how you can use IRepository pattern with generics to handle basic CRUD operation with EF
– Karoui Haithem
Nov 14 '18 at 16:51
|
show 2 more comments
If you want to add a new product to an existing Supplier you need to do as following:
1- Retrieve the supplier entity, let's call it sup
2- Add the new Product to it,
sup.Product = new Product{properties
...}
3- Update the supplier entity,
ctx.Entry(sup).State = EntityState.Modified;
ctx.SaveChanges();
You were adding a new supplier entity each time you were using the bool Insert<T> method
and this why you got unexpected behaviour, so just update the existing entry instead.
Hope this helps,
Oh my, this makes sense. But then again, if i have 2 relational entities for my Product (Supplier and Contract lets say). Would this still work, and not inserting a duplicate Contract? Seems strange to insert a new Product by updating a supplier :) Will try this in a moment and post my feedback. Thank you for your suggestion @Karoui Haithem!
– Dimitri
Nov 14 '18 at 10:42
1
yep, indeed, it works that way and its full of sense, if you want to link newEntityA to ExistingEntityB you retrive B and create a related A to it and then update B, same if you have A related to B to C ...
– Karoui Haithem
Nov 14 '18 at 10:51
So i have done what you described, when creating a new Product, i retrieve the supplier from database (which was selected in the UI to attach to this new product). I assign properties and so on to my new product and then i add this new product to the Suppliers.Products list property and save it. This indeed creates my new Product Entity but still is duplicating my supplier... Maybe it is another relational entity causing this? I will update my OP with my Entity details.(Thank you so far!)
– Dimitri
Nov 14 '18 at 11:15
1
put the code which does what you just described, i am not sure but i think that you missed updating the entity (ctx.Entry(yourEntity).State = EntityState.Modified;)
– Karoui Haithem
Nov 14 '18 at 11:20
1
you can post your last comment in a new question and link it here then i will post an answer showing how you can use IRepository pattern with generics to handle basic CRUD operation with EF
– Karoui Haithem
Nov 14 '18 at 16:51
|
show 2 more comments
If you want to add a new product to an existing Supplier you need to do as following:
1- Retrieve the supplier entity, let's call it sup
2- Add the new Product to it,
sup.Product = new Product{properties
...}
3- Update the supplier entity,
ctx.Entry(sup).State = EntityState.Modified;
ctx.SaveChanges();
You were adding a new supplier entity each time you were using the bool Insert<T> method
and this why you got unexpected behaviour, so just update the existing entry instead.
Hope this helps,
If you want to add a new product to an existing Supplier you need to do as following:
1- Retrieve the supplier entity, let's call it sup
2- Add the new Product to it,
sup.Product = new Product{properties
...}
3- Update the supplier entity,
ctx.Entry(sup).State = EntityState.Modified;
ctx.SaveChanges();
You were adding a new supplier entity each time you were using the bool Insert<T> method
and this why you got unexpected behaviour, so just update the existing entry instead.
Hope this helps,
answered Nov 14 '18 at 10:28


Karoui HaithemKaroui Haithem
555421
555421
Oh my, this makes sense. But then again, if i have 2 relational entities for my Product (Supplier and Contract lets say). Would this still work, and not inserting a duplicate Contract? Seems strange to insert a new Product by updating a supplier :) Will try this in a moment and post my feedback. Thank you for your suggestion @Karoui Haithem!
– Dimitri
Nov 14 '18 at 10:42
1
yep, indeed, it works that way and its full of sense, if you want to link newEntityA to ExistingEntityB you retrive B and create a related A to it and then update B, same if you have A related to B to C ...
– Karoui Haithem
Nov 14 '18 at 10:51
So i have done what you described, when creating a new Product, i retrieve the supplier from database (which was selected in the UI to attach to this new product). I assign properties and so on to my new product and then i add this new product to the Suppliers.Products list property and save it. This indeed creates my new Product Entity but still is duplicating my supplier... Maybe it is another relational entity causing this? I will update my OP with my Entity details.(Thank you so far!)
– Dimitri
Nov 14 '18 at 11:15
1
put the code which does what you just described, i am not sure but i think that you missed updating the entity (ctx.Entry(yourEntity).State = EntityState.Modified;)
– Karoui Haithem
Nov 14 '18 at 11:20
1
you can post your last comment in a new question and link it here then i will post an answer showing how you can use IRepository pattern with generics to handle basic CRUD operation with EF
– Karoui Haithem
Nov 14 '18 at 16:51
|
show 2 more comments
Oh my, this makes sense. But then again, if i have 2 relational entities for my Product (Supplier and Contract lets say). Would this still work, and not inserting a duplicate Contract? Seems strange to insert a new Product by updating a supplier :) Will try this in a moment and post my feedback. Thank you for your suggestion @Karoui Haithem!
– Dimitri
Nov 14 '18 at 10:42
1
yep, indeed, it works that way and its full of sense, if you want to link newEntityA to ExistingEntityB you retrive B and create a related A to it and then update B, same if you have A related to B to C ...
– Karoui Haithem
Nov 14 '18 at 10:51
So i have done what you described, when creating a new Product, i retrieve the supplier from database (which was selected in the UI to attach to this new product). I assign properties and so on to my new product and then i add this new product to the Suppliers.Products list property and save it. This indeed creates my new Product Entity but still is duplicating my supplier... Maybe it is another relational entity causing this? I will update my OP with my Entity details.(Thank you so far!)
– Dimitri
Nov 14 '18 at 11:15
1
put the code which does what you just described, i am not sure but i think that you missed updating the entity (ctx.Entry(yourEntity).State = EntityState.Modified;)
– Karoui Haithem
Nov 14 '18 at 11:20
1
you can post your last comment in a new question and link it here then i will post an answer showing how you can use IRepository pattern with generics to handle basic CRUD operation with EF
– Karoui Haithem
Nov 14 '18 at 16:51
Oh my, this makes sense. But then again, if i have 2 relational entities for my Product (Supplier and Contract lets say). Would this still work, and not inserting a duplicate Contract? Seems strange to insert a new Product by updating a supplier :) Will try this in a moment and post my feedback. Thank you for your suggestion @Karoui Haithem!
– Dimitri
Nov 14 '18 at 10:42
Oh my, this makes sense. But then again, if i have 2 relational entities for my Product (Supplier and Contract lets say). Would this still work, and not inserting a duplicate Contract? Seems strange to insert a new Product by updating a supplier :) Will try this in a moment and post my feedback. Thank you for your suggestion @Karoui Haithem!
– Dimitri
Nov 14 '18 at 10:42
1
1
yep, indeed, it works that way and its full of sense, if you want to link newEntityA to ExistingEntityB you retrive B and create a related A to it and then update B, same if you have A related to B to C ...
– Karoui Haithem
Nov 14 '18 at 10:51
yep, indeed, it works that way and its full of sense, if you want to link newEntityA to ExistingEntityB you retrive B and create a related A to it and then update B, same if you have A related to B to C ...
– Karoui Haithem
Nov 14 '18 at 10:51
So i have done what you described, when creating a new Product, i retrieve the supplier from database (which was selected in the UI to attach to this new product). I assign properties and so on to my new product and then i add this new product to the Suppliers.Products list property and save it. This indeed creates my new Product Entity but still is duplicating my supplier... Maybe it is another relational entity causing this? I will update my OP with my Entity details.(Thank you so far!)
– Dimitri
Nov 14 '18 at 11:15
So i have done what you described, when creating a new Product, i retrieve the supplier from database (which was selected in the UI to attach to this new product). I assign properties and so on to my new product and then i add this new product to the Suppliers.Products list property and save it. This indeed creates my new Product Entity but still is duplicating my supplier... Maybe it is another relational entity causing this? I will update my OP with my Entity details.(Thank you so far!)
– Dimitri
Nov 14 '18 at 11:15
1
1
put the code which does what you just described, i am not sure but i think that you missed updating the entity (ctx.Entry(yourEntity).State = EntityState.Modified;)
– Karoui Haithem
Nov 14 '18 at 11:20
put the code which does what you just described, i am not sure but i think that you missed updating the entity (ctx.Entry(yourEntity).State = EntityState.Modified;)
– Karoui Haithem
Nov 14 '18 at 11:20
1
1
you can post your last comment in a new question and link it here then i will post an answer showing how you can use IRepository pattern with generics to handle basic CRUD operation with EF
– Karoui Haithem
Nov 14 '18 at 16:51
you can post your last comment in a new question and link it here then i will post an answer showing how you can use IRepository pattern with generics to handle basic CRUD operation with EF
– Karoui Haithem
Nov 14 '18 at 16:51
|
show 2 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53296818%2fentity-framework-generic-insert-method-is-inserting-already-existing-entity-agai%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
y1Ac,ggZSvCfj3TZxrDF8mKzO3Vw9bL2m1YvkKGD2PUpWJQmps1f PDyNBdu,zM 0nOV 6T VEP