Iterating through array of object using pointer to array
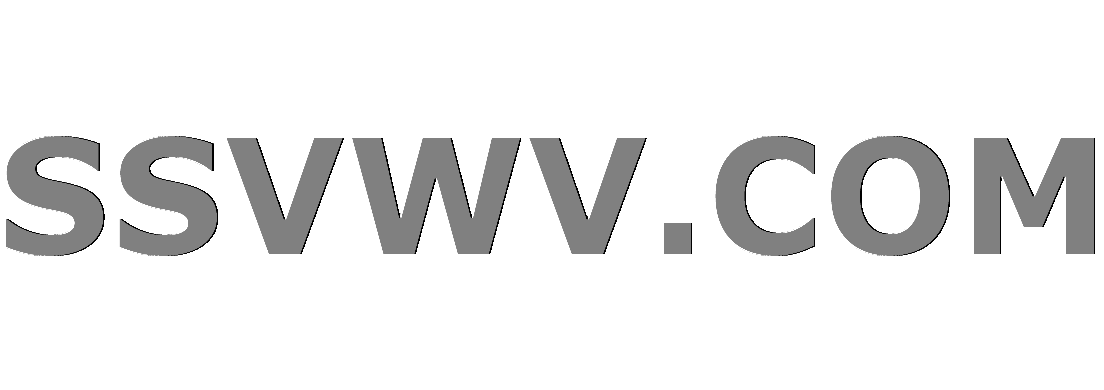
Multi tool use
I am new to C++ and working for an assignment, using C++98. I am trying to loop through an array of objects using pointer to array.
It is printing first point correctly, and after that some value.
Here are the code snippets:
struct Point { int x, y; };
int randomNumber(int low, int high ) {
int randomNumber = low + (rand())/ (RAND_MAX/(high-low));
cout << setprecision(2);
return randomNumber;
}
Point *generateCoordinates(int nodesPerBlock) {
Point cities[nodesPerBlock];
for (int i = 0; i < nodesPerBlock; i++) {
cities[i].x = randomNumber(1, 50);
cities[i].y = randomNumber(1, 50);
}
return cities;
}
int main() {
int nodesPerBlock = 5;
Point *points = generateCoordinates(nodesPerBlock);
for (int n = 0; n < (nodesPerBlock-2); n++) {
cout << "n: x=" << points[n].x << ", y=" << points[n].y << endl;
cout << "n+1: x=" << points[n+1].x << ", y=" << points[n+1].y << endl;
}
}
this prints:
n: x=1, y=4
n+1: x=18, y=11
n: x=2049417976, y=32767
n+1: x=2049417976, y=32767
n: x=2049417976, y=32767
n+1: x=2049417984, y=167804927
while the actual Points printed were:
Point : x=1, y=4.
Point : x=18, y=11.
Point : x=13, y=6.
Point : x=2, y=16.
Point : x=16, y=22.
referred this questions and this, but no success so far.
c++ arrays pointers c++98
add a comment |
I am new to C++ and working for an assignment, using C++98. I am trying to loop through an array of objects using pointer to array.
It is printing first point correctly, and after that some value.
Here are the code snippets:
struct Point { int x, y; };
int randomNumber(int low, int high ) {
int randomNumber = low + (rand())/ (RAND_MAX/(high-low));
cout << setprecision(2);
return randomNumber;
}
Point *generateCoordinates(int nodesPerBlock) {
Point cities[nodesPerBlock];
for (int i = 0; i < nodesPerBlock; i++) {
cities[i].x = randomNumber(1, 50);
cities[i].y = randomNumber(1, 50);
}
return cities;
}
int main() {
int nodesPerBlock = 5;
Point *points = generateCoordinates(nodesPerBlock);
for (int n = 0; n < (nodesPerBlock-2); n++) {
cout << "n: x=" << points[n].x << ", y=" << points[n].y << endl;
cout << "n+1: x=" << points[n+1].x << ", y=" << points[n+1].y << endl;
}
}
this prints:
n: x=1, y=4
n+1: x=18, y=11
n: x=2049417976, y=32767
n+1: x=2049417976, y=32767
n: x=2049417976, y=32767
n+1: x=2049417984, y=167804927
while the actual Points printed were:
Point : x=1, y=4.
Point : x=18, y=11.
Point : x=13, y=6.
Point : x=2, y=16.
Point : x=16, y=22.
referred this questions and this, but no success so far.
c++ arrays pointers c++98
Please see stackoverflow.com/questions/7769998/… but take the accepted answer with a grain of salt.
– paddy
Nov 15 '18 at 5:17
add a comment |
I am new to C++ and working for an assignment, using C++98. I am trying to loop through an array of objects using pointer to array.
It is printing first point correctly, and after that some value.
Here are the code snippets:
struct Point { int x, y; };
int randomNumber(int low, int high ) {
int randomNumber = low + (rand())/ (RAND_MAX/(high-low));
cout << setprecision(2);
return randomNumber;
}
Point *generateCoordinates(int nodesPerBlock) {
Point cities[nodesPerBlock];
for (int i = 0; i < nodesPerBlock; i++) {
cities[i].x = randomNumber(1, 50);
cities[i].y = randomNumber(1, 50);
}
return cities;
}
int main() {
int nodesPerBlock = 5;
Point *points = generateCoordinates(nodesPerBlock);
for (int n = 0; n < (nodesPerBlock-2); n++) {
cout << "n: x=" << points[n].x << ", y=" << points[n].y << endl;
cout << "n+1: x=" << points[n+1].x << ", y=" << points[n+1].y << endl;
}
}
this prints:
n: x=1, y=4
n+1: x=18, y=11
n: x=2049417976, y=32767
n+1: x=2049417976, y=32767
n: x=2049417976, y=32767
n+1: x=2049417984, y=167804927
while the actual Points printed were:
Point : x=1, y=4.
Point : x=18, y=11.
Point : x=13, y=6.
Point : x=2, y=16.
Point : x=16, y=22.
referred this questions and this, but no success so far.
c++ arrays pointers c++98
I am new to C++ and working for an assignment, using C++98. I am trying to loop through an array of objects using pointer to array.
It is printing first point correctly, and after that some value.
Here are the code snippets:
struct Point { int x, y; };
int randomNumber(int low, int high ) {
int randomNumber = low + (rand())/ (RAND_MAX/(high-low));
cout << setprecision(2);
return randomNumber;
}
Point *generateCoordinates(int nodesPerBlock) {
Point cities[nodesPerBlock];
for (int i = 0; i < nodesPerBlock; i++) {
cities[i].x = randomNumber(1, 50);
cities[i].y = randomNumber(1, 50);
}
return cities;
}
int main() {
int nodesPerBlock = 5;
Point *points = generateCoordinates(nodesPerBlock);
for (int n = 0; n < (nodesPerBlock-2); n++) {
cout << "n: x=" << points[n].x << ", y=" << points[n].y << endl;
cout << "n+1: x=" << points[n+1].x << ", y=" << points[n+1].y << endl;
}
}
this prints:
n: x=1, y=4
n+1: x=18, y=11
n: x=2049417976, y=32767
n+1: x=2049417976, y=32767
n: x=2049417976, y=32767
n+1: x=2049417984, y=167804927
while the actual Points printed were:
Point : x=1, y=4.
Point : x=18, y=11.
Point : x=13, y=6.
Point : x=2, y=16.
Point : x=16, y=22.
referred this questions and this, but no success so far.
c++ arrays pointers c++98
c++ arrays pointers c++98
edited Nov 15 '18 at 5:17
Nitin1706
asked Nov 15 '18 at 5:13


Nitin1706Nitin1706
14111
14111
Please see stackoverflow.com/questions/7769998/… but take the accepted answer with a grain of salt.
– paddy
Nov 15 '18 at 5:17
add a comment |
Please see stackoverflow.com/questions/7769998/… but take the accepted answer with a grain of salt.
– paddy
Nov 15 '18 at 5:17
Please see stackoverflow.com/questions/7769998/… but take the accepted answer with a grain of salt.
– paddy
Nov 15 '18 at 5:17
Please see stackoverflow.com/questions/7769998/… but take the accepted answer with a grain of salt.
– paddy
Nov 15 '18 at 5:17
add a comment |
2 Answers
2
active
oldest
votes
cities[nodesPerBlock]
is a local variable in generateCoordinates
function and it goes out of scope when the function exits.
You are returning an address to it and are accessing that address in main
. This is undefined behavior to do so.
You have to allocate cities
on the the heap using new
(since you are using C++98) and then return that address to main. Then you will be able to reliably access that address.
After your processing, do not forget to delete the memory you have allocated at the end of main
.
You can avoid memory allocation and deletion by changing your function to take an extra parameter which you can pass from main
. Then cities
can be an array of Point
s on the stack.
void generateCoordinates(Point cities, int nodesPerBlock);
Why not simplyvoid generateCoordinates(Point cities, int nodesPerBlock)
? Dynamic allocation is really not required here. And I'm pretty sure C++98 still hasstd::vector
, which should be the default advice given these days to anyone requiring dynamic contiguous memory on the heap.
– paddy
Nov 15 '18 at 5:21
I do not know what constraints the OP is under, whether he is permitted to usevector
or not. But it is better to have the function signature you suggest. Then there is no need for dynamic memory allocation. I will update the answer to that effect.
– P.W
Nov 15 '18 at 5:27
add a comment |
There are three mistakes in your code:
In
generateCoordinates
you declare an arraycities
with a size determined by the parameternodesPerBlock
. This is not allowed, because c++ forbids to declare an array of variable length. When you have an array with a variable size you have to explicitly allocate the memory. Though, the code may still compile because of a gnu extension (if you are using gcc), but still does not follows the standard.In
generateCoordinates
you return a pointer to a local variablecities
which is deallocated after you exit the function. This is why you get random number.You forgot to include the relevant headers.
A correct way to do the task could be to:
Allocate an array in
main()
Pass its pointer to
generateCoordinates
Also, do not forget to deallocate the array
Example of code:
#include <cstdlib>
#include <iomanip>
#include <iostream>
struct Point { int x, y; };
int randomNumber(int low, int high ) {
int randomNumber = low + (rand())/ (RAND_MAX/(high-low));
std::cout << std::setprecision(2);
return randomNumber;
}
void generateCoordinates(int nodesPerBlock, Point *cities) {
for (int i = 0; i < nodesPerBlock; i++) {
cities[i].x = randomNumber(1, 50);
cities[i].y = randomNumber(1, 50);
}
}
int main() {
int nodesPerBlock = 5;
Point *points = new Point[nodesPerBlock];
generateCoordinates(nodesPerBlock, points);
for (int n = 0; n < (nodesPerBlock-2); n++) {
std::cout << "n: x=" << points[n].x << ", y=" << points[n].y << std::endl;
std::cout << "n+1: x=" << points[n+1].x << ", y=" << points[n+1].y << std::endl;
}
delete points;
}
As a final comment: in the header part of a code you should avoid using namespace std
because, if you ever split the header into a separate file, every time you include it you will bring out all the names of std
into the global space, thus possibly colliding with names from other #include
.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53312832%2fiterating-through-array-of-object-using-pointer-to-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
cities[nodesPerBlock]
is a local variable in generateCoordinates
function and it goes out of scope when the function exits.
You are returning an address to it and are accessing that address in main
. This is undefined behavior to do so.
You have to allocate cities
on the the heap using new
(since you are using C++98) and then return that address to main. Then you will be able to reliably access that address.
After your processing, do not forget to delete the memory you have allocated at the end of main
.
You can avoid memory allocation and deletion by changing your function to take an extra parameter which you can pass from main
. Then cities
can be an array of Point
s on the stack.
void generateCoordinates(Point cities, int nodesPerBlock);
Why not simplyvoid generateCoordinates(Point cities, int nodesPerBlock)
? Dynamic allocation is really not required here. And I'm pretty sure C++98 still hasstd::vector
, which should be the default advice given these days to anyone requiring dynamic contiguous memory on the heap.
– paddy
Nov 15 '18 at 5:21
I do not know what constraints the OP is under, whether he is permitted to usevector
or not. But it is better to have the function signature you suggest. Then there is no need for dynamic memory allocation. I will update the answer to that effect.
– P.W
Nov 15 '18 at 5:27
add a comment |
cities[nodesPerBlock]
is a local variable in generateCoordinates
function and it goes out of scope when the function exits.
You are returning an address to it and are accessing that address in main
. This is undefined behavior to do so.
You have to allocate cities
on the the heap using new
(since you are using C++98) and then return that address to main. Then you will be able to reliably access that address.
After your processing, do not forget to delete the memory you have allocated at the end of main
.
You can avoid memory allocation and deletion by changing your function to take an extra parameter which you can pass from main
. Then cities
can be an array of Point
s on the stack.
void generateCoordinates(Point cities, int nodesPerBlock);
Why not simplyvoid generateCoordinates(Point cities, int nodesPerBlock)
? Dynamic allocation is really not required here. And I'm pretty sure C++98 still hasstd::vector
, which should be the default advice given these days to anyone requiring dynamic contiguous memory on the heap.
– paddy
Nov 15 '18 at 5:21
I do not know what constraints the OP is under, whether he is permitted to usevector
or not. But it is better to have the function signature you suggest. Then there is no need for dynamic memory allocation. I will update the answer to that effect.
– P.W
Nov 15 '18 at 5:27
add a comment |
cities[nodesPerBlock]
is a local variable in generateCoordinates
function and it goes out of scope when the function exits.
You are returning an address to it and are accessing that address in main
. This is undefined behavior to do so.
You have to allocate cities
on the the heap using new
(since you are using C++98) and then return that address to main. Then you will be able to reliably access that address.
After your processing, do not forget to delete the memory you have allocated at the end of main
.
You can avoid memory allocation and deletion by changing your function to take an extra parameter which you can pass from main
. Then cities
can be an array of Point
s on the stack.
void generateCoordinates(Point cities, int nodesPerBlock);
cities[nodesPerBlock]
is a local variable in generateCoordinates
function and it goes out of scope when the function exits.
You are returning an address to it and are accessing that address in main
. This is undefined behavior to do so.
You have to allocate cities
on the the heap using new
(since you are using C++98) and then return that address to main. Then you will be able to reliably access that address.
After your processing, do not forget to delete the memory you have allocated at the end of main
.
You can avoid memory allocation and deletion by changing your function to take an extra parameter which you can pass from main
. Then cities
can be an array of Point
s on the stack.
void generateCoordinates(Point cities, int nodesPerBlock);
edited Nov 15 '18 at 5:27
answered Nov 15 '18 at 5:16
P.WP.W
15.1k31453
15.1k31453
Why not simplyvoid generateCoordinates(Point cities, int nodesPerBlock)
? Dynamic allocation is really not required here. And I'm pretty sure C++98 still hasstd::vector
, which should be the default advice given these days to anyone requiring dynamic contiguous memory on the heap.
– paddy
Nov 15 '18 at 5:21
I do not know what constraints the OP is under, whether he is permitted to usevector
or not. But it is better to have the function signature you suggest. Then there is no need for dynamic memory allocation. I will update the answer to that effect.
– P.W
Nov 15 '18 at 5:27
add a comment |
Why not simplyvoid generateCoordinates(Point cities, int nodesPerBlock)
? Dynamic allocation is really not required here. And I'm pretty sure C++98 still hasstd::vector
, which should be the default advice given these days to anyone requiring dynamic contiguous memory on the heap.
– paddy
Nov 15 '18 at 5:21
I do not know what constraints the OP is under, whether he is permitted to usevector
or not. But it is better to have the function signature you suggest. Then there is no need for dynamic memory allocation. I will update the answer to that effect.
– P.W
Nov 15 '18 at 5:27
Why not simply
void generateCoordinates(Point cities, int nodesPerBlock)
? Dynamic allocation is really not required here. And I'm pretty sure C++98 still has std::vector
, which should be the default advice given these days to anyone requiring dynamic contiguous memory on the heap.– paddy
Nov 15 '18 at 5:21
Why not simply
void generateCoordinates(Point cities, int nodesPerBlock)
? Dynamic allocation is really not required here. And I'm pretty sure C++98 still has std::vector
, which should be the default advice given these days to anyone requiring dynamic contiguous memory on the heap.– paddy
Nov 15 '18 at 5:21
I do not know what constraints the OP is under, whether he is permitted to use
vector
or not. But it is better to have the function signature you suggest. Then there is no need for dynamic memory allocation. I will update the answer to that effect.– P.W
Nov 15 '18 at 5:27
I do not know what constraints the OP is under, whether he is permitted to use
vector
or not. But it is better to have the function signature you suggest. Then there is no need for dynamic memory allocation. I will update the answer to that effect.– P.W
Nov 15 '18 at 5:27
add a comment |
There are three mistakes in your code:
In
generateCoordinates
you declare an arraycities
with a size determined by the parameternodesPerBlock
. This is not allowed, because c++ forbids to declare an array of variable length. When you have an array with a variable size you have to explicitly allocate the memory. Though, the code may still compile because of a gnu extension (if you are using gcc), but still does not follows the standard.In
generateCoordinates
you return a pointer to a local variablecities
which is deallocated after you exit the function. This is why you get random number.You forgot to include the relevant headers.
A correct way to do the task could be to:
Allocate an array in
main()
Pass its pointer to
generateCoordinates
Also, do not forget to deallocate the array
Example of code:
#include <cstdlib>
#include <iomanip>
#include <iostream>
struct Point { int x, y; };
int randomNumber(int low, int high ) {
int randomNumber = low + (rand())/ (RAND_MAX/(high-low));
std::cout << std::setprecision(2);
return randomNumber;
}
void generateCoordinates(int nodesPerBlock, Point *cities) {
for (int i = 0; i < nodesPerBlock; i++) {
cities[i].x = randomNumber(1, 50);
cities[i].y = randomNumber(1, 50);
}
}
int main() {
int nodesPerBlock = 5;
Point *points = new Point[nodesPerBlock];
generateCoordinates(nodesPerBlock, points);
for (int n = 0; n < (nodesPerBlock-2); n++) {
std::cout << "n: x=" << points[n].x << ", y=" << points[n].y << std::endl;
std::cout << "n+1: x=" << points[n+1].x << ", y=" << points[n+1].y << std::endl;
}
delete points;
}
As a final comment: in the header part of a code you should avoid using namespace std
because, if you ever split the header into a separate file, every time you include it you will bring out all the names of std
into the global space, thus possibly colliding with names from other #include
.
add a comment |
There are three mistakes in your code:
In
generateCoordinates
you declare an arraycities
with a size determined by the parameternodesPerBlock
. This is not allowed, because c++ forbids to declare an array of variable length. When you have an array with a variable size you have to explicitly allocate the memory. Though, the code may still compile because of a gnu extension (if you are using gcc), but still does not follows the standard.In
generateCoordinates
you return a pointer to a local variablecities
which is deallocated after you exit the function. This is why you get random number.You forgot to include the relevant headers.
A correct way to do the task could be to:
Allocate an array in
main()
Pass its pointer to
generateCoordinates
Also, do not forget to deallocate the array
Example of code:
#include <cstdlib>
#include <iomanip>
#include <iostream>
struct Point { int x, y; };
int randomNumber(int low, int high ) {
int randomNumber = low + (rand())/ (RAND_MAX/(high-low));
std::cout << std::setprecision(2);
return randomNumber;
}
void generateCoordinates(int nodesPerBlock, Point *cities) {
for (int i = 0; i < nodesPerBlock; i++) {
cities[i].x = randomNumber(1, 50);
cities[i].y = randomNumber(1, 50);
}
}
int main() {
int nodesPerBlock = 5;
Point *points = new Point[nodesPerBlock];
generateCoordinates(nodesPerBlock, points);
for (int n = 0; n < (nodesPerBlock-2); n++) {
std::cout << "n: x=" << points[n].x << ", y=" << points[n].y << std::endl;
std::cout << "n+1: x=" << points[n+1].x << ", y=" << points[n+1].y << std::endl;
}
delete points;
}
As a final comment: in the header part of a code you should avoid using namespace std
because, if you ever split the header into a separate file, every time you include it you will bring out all the names of std
into the global space, thus possibly colliding with names from other #include
.
add a comment |
There are three mistakes in your code:
In
generateCoordinates
you declare an arraycities
with a size determined by the parameternodesPerBlock
. This is not allowed, because c++ forbids to declare an array of variable length. When you have an array with a variable size you have to explicitly allocate the memory. Though, the code may still compile because of a gnu extension (if you are using gcc), but still does not follows the standard.In
generateCoordinates
you return a pointer to a local variablecities
which is deallocated after you exit the function. This is why you get random number.You forgot to include the relevant headers.
A correct way to do the task could be to:
Allocate an array in
main()
Pass its pointer to
generateCoordinates
Also, do not forget to deallocate the array
Example of code:
#include <cstdlib>
#include <iomanip>
#include <iostream>
struct Point { int x, y; };
int randomNumber(int low, int high ) {
int randomNumber = low + (rand())/ (RAND_MAX/(high-low));
std::cout << std::setprecision(2);
return randomNumber;
}
void generateCoordinates(int nodesPerBlock, Point *cities) {
for (int i = 0; i < nodesPerBlock; i++) {
cities[i].x = randomNumber(1, 50);
cities[i].y = randomNumber(1, 50);
}
}
int main() {
int nodesPerBlock = 5;
Point *points = new Point[nodesPerBlock];
generateCoordinates(nodesPerBlock, points);
for (int n = 0; n < (nodesPerBlock-2); n++) {
std::cout << "n: x=" << points[n].x << ", y=" << points[n].y << std::endl;
std::cout << "n+1: x=" << points[n+1].x << ", y=" << points[n+1].y << std::endl;
}
delete points;
}
As a final comment: in the header part of a code you should avoid using namespace std
because, if you ever split the header into a separate file, every time you include it you will bring out all the names of std
into the global space, thus possibly colliding with names from other #include
.
There are three mistakes in your code:
In
generateCoordinates
you declare an arraycities
with a size determined by the parameternodesPerBlock
. This is not allowed, because c++ forbids to declare an array of variable length. When you have an array with a variable size you have to explicitly allocate the memory. Though, the code may still compile because of a gnu extension (if you are using gcc), but still does not follows the standard.In
generateCoordinates
you return a pointer to a local variablecities
which is deallocated after you exit the function. This is why you get random number.You forgot to include the relevant headers.
A correct way to do the task could be to:
Allocate an array in
main()
Pass its pointer to
generateCoordinates
Also, do not forget to deallocate the array
Example of code:
#include <cstdlib>
#include <iomanip>
#include <iostream>
struct Point { int x, y; };
int randomNumber(int low, int high ) {
int randomNumber = low + (rand())/ (RAND_MAX/(high-low));
std::cout << std::setprecision(2);
return randomNumber;
}
void generateCoordinates(int nodesPerBlock, Point *cities) {
for (int i = 0; i < nodesPerBlock; i++) {
cities[i].x = randomNumber(1, 50);
cities[i].y = randomNumber(1, 50);
}
}
int main() {
int nodesPerBlock = 5;
Point *points = new Point[nodesPerBlock];
generateCoordinates(nodesPerBlock, points);
for (int n = 0; n < (nodesPerBlock-2); n++) {
std::cout << "n: x=" << points[n].x << ", y=" << points[n].y << std::endl;
std::cout << "n+1: x=" << points[n+1].x << ", y=" << points[n+1].y << std::endl;
}
delete points;
}
As a final comment: in the header part of a code you should avoid using namespace std
because, if you ever split the header into a separate file, every time you include it you will bring out all the names of std
into the global space, thus possibly colliding with names from other #include
.
answered Nov 15 '18 at 5:55
francescofrancesco
8901212
8901212
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53312832%2fiterating-through-array-of-object-using-pointer-to-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
noqZfh5B,s0VRuxcfLx4aFPO8TEoaNxBd,ZI
Please see stackoverflow.com/questions/7769998/… but take the accepted answer with a grain of salt.
– paddy
Nov 15 '18 at 5:17