How do I replace variables in HTML with an object of variables from jQuery?
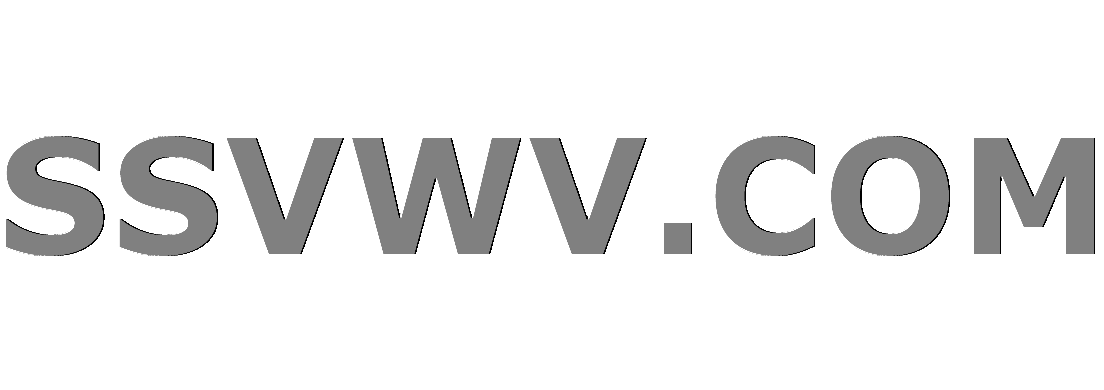
Multi tool use
I am trying to make a system for my web page that gets the content of a template found in the HTML and then replaces the appropriate variables with the corresponding values which is stored in an object. The problem is that nothing is being replaced. In this example, I am getting the contents of an RSS file that displays forum posts. I use ajax to get the contents, then iterate through each item. I assign the xml tag values to an object whose keys correspond to the variabls in the HTML template. For every post found, it should replace the HTML variable with the contents of the xml tag.
This is my Javascript:
$.ajax({
url: "rsstest.xml",
type: "GET",
dataType: 'xml',
crossDomain: true,
success: function(xml){
var news = $('#news-results');
news.empty();
if($(xml).find('item').length > 0){
$(xml).find('item').each(function(){
var temp_vars = {
news_title: $(this).find('title').text(),
news_body: $(this).find('description').text(),
news_date: $(this).find('pubDate').text(),
news_link: $(this).find('link').text()
}
var template = $('#news-template').contents().clone();
for(const variable in temp_vars){
template.text().replace("{"+variable+"}", temp_vars[variable])
}
news.append(template);
})
} else {
news.append($('<p></p>').text('There is no news to display at this time.'));
}
}
})
This is my HTML:
<h1>Announcements</h1>
<div id="news-results">
</div>
<div id="news-template" style="display: none;">
<div class="media">
<div class="media-body">
<h3>{news_title}</h3>
<p>{news_body}</p>
<div class="media-footer">
<div class="col-left">
<h4>Posted on {news_date}</h4>
</div>
<div class="col-right">
<a href="{news_link}" class="btn btn-default">Read More</a>
</div>
</div>
</div>
</div>
</div>
What am I doing wrong?
javascript jquery
|
show 1 more comment
I am trying to make a system for my web page that gets the content of a template found in the HTML and then replaces the appropriate variables with the corresponding values which is stored in an object. The problem is that nothing is being replaced. In this example, I am getting the contents of an RSS file that displays forum posts. I use ajax to get the contents, then iterate through each item. I assign the xml tag values to an object whose keys correspond to the variabls in the HTML template. For every post found, it should replace the HTML variable with the contents of the xml tag.
This is my Javascript:
$.ajax({
url: "rsstest.xml",
type: "GET",
dataType: 'xml',
crossDomain: true,
success: function(xml){
var news = $('#news-results');
news.empty();
if($(xml).find('item').length > 0){
$(xml).find('item').each(function(){
var temp_vars = {
news_title: $(this).find('title').text(),
news_body: $(this).find('description').text(),
news_date: $(this).find('pubDate').text(),
news_link: $(this).find('link').text()
}
var template = $('#news-template').contents().clone();
for(const variable in temp_vars){
template.text().replace("{"+variable+"}", temp_vars[variable])
}
news.append(template);
})
} else {
news.append($('<p></p>').text('There is no news to display at this time.'));
}
}
})
This is my HTML:
<h1>Announcements</h1>
<div id="news-results">
</div>
<div id="news-template" style="display: none;">
<div class="media">
<div class="media-body">
<h3>{news_title}</h3>
<p>{news_body}</p>
<div class="media-footer">
<div class="col-left">
<h4>Posted on {news_date}</h4>
</div>
<div class="col-right">
<a href="{news_link}" class="btn btn-default">Read More</a>
</div>
</div>
</div>
</div>
</div>
What am I doing wrong?
javascript jquery
Have you checked to see what's running? For instance, do you ever get to thevar temp_vars = {
line? Any errors?
– CertainPerformance
Nov 15 '18 at 3:59
I have added console.log(temp_vars[variable]) just inside the for loop and it logs correctly.
– ShoeLace1291
Nov 15 '18 at 4:01
template.text(template.text().replace("{"+variable+"}", temp_vars[variable]))
– ACD
Nov 15 '18 at 4:05
@ACD that makes sense! thanks!
– ShoeLace1291
Nov 15 '18 at 4:10
posting it as answer then :) glad to help
– ACD
Nov 15 '18 at 4:12
|
show 1 more comment
I am trying to make a system for my web page that gets the content of a template found in the HTML and then replaces the appropriate variables with the corresponding values which is stored in an object. The problem is that nothing is being replaced. In this example, I am getting the contents of an RSS file that displays forum posts. I use ajax to get the contents, then iterate through each item. I assign the xml tag values to an object whose keys correspond to the variabls in the HTML template. For every post found, it should replace the HTML variable with the contents of the xml tag.
This is my Javascript:
$.ajax({
url: "rsstest.xml",
type: "GET",
dataType: 'xml',
crossDomain: true,
success: function(xml){
var news = $('#news-results');
news.empty();
if($(xml).find('item').length > 0){
$(xml).find('item').each(function(){
var temp_vars = {
news_title: $(this).find('title').text(),
news_body: $(this).find('description').text(),
news_date: $(this).find('pubDate').text(),
news_link: $(this).find('link').text()
}
var template = $('#news-template').contents().clone();
for(const variable in temp_vars){
template.text().replace("{"+variable+"}", temp_vars[variable])
}
news.append(template);
})
} else {
news.append($('<p></p>').text('There is no news to display at this time.'));
}
}
})
This is my HTML:
<h1>Announcements</h1>
<div id="news-results">
</div>
<div id="news-template" style="display: none;">
<div class="media">
<div class="media-body">
<h3>{news_title}</h3>
<p>{news_body}</p>
<div class="media-footer">
<div class="col-left">
<h4>Posted on {news_date}</h4>
</div>
<div class="col-right">
<a href="{news_link}" class="btn btn-default">Read More</a>
</div>
</div>
</div>
</div>
</div>
What am I doing wrong?
javascript jquery
I am trying to make a system for my web page that gets the content of a template found in the HTML and then replaces the appropriate variables with the corresponding values which is stored in an object. The problem is that nothing is being replaced. In this example, I am getting the contents of an RSS file that displays forum posts. I use ajax to get the contents, then iterate through each item. I assign the xml tag values to an object whose keys correspond to the variabls in the HTML template. For every post found, it should replace the HTML variable with the contents of the xml tag.
This is my Javascript:
$.ajax({
url: "rsstest.xml",
type: "GET",
dataType: 'xml',
crossDomain: true,
success: function(xml){
var news = $('#news-results');
news.empty();
if($(xml).find('item').length > 0){
$(xml).find('item').each(function(){
var temp_vars = {
news_title: $(this).find('title').text(),
news_body: $(this).find('description').text(),
news_date: $(this).find('pubDate').text(),
news_link: $(this).find('link').text()
}
var template = $('#news-template').contents().clone();
for(const variable in temp_vars){
template.text().replace("{"+variable+"}", temp_vars[variable])
}
news.append(template);
})
} else {
news.append($('<p></p>').text('There is no news to display at this time.'));
}
}
})
This is my HTML:
<h1>Announcements</h1>
<div id="news-results">
</div>
<div id="news-template" style="display: none;">
<div class="media">
<div class="media-body">
<h3>{news_title}</h3>
<p>{news_body}</p>
<div class="media-footer">
<div class="col-left">
<h4>Posted on {news_date}</h4>
</div>
<div class="col-right">
<a href="{news_link}" class="btn btn-default">Read More</a>
</div>
</div>
</div>
</div>
</div>
What am I doing wrong?
javascript jquery
javascript jquery
asked Nov 15 '18 at 3:57
ShoeLace1291ShoeLace1291
1,64983155
1,64983155
Have you checked to see what's running? For instance, do you ever get to thevar temp_vars = {
line? Any errors?
– CertainPerformance
Nov 15 '18 at 3:59
I have added console.log(temp_vars[variable]) just inside the for loop and it logs correctly.
– ShoeLace1291
Nov 15 '18 at 4:01
template.text(template.text().replace("{"+variable+"}", temp_vars[variable]))
– ACD
Nov 15 '18 at 4:05
@ACD that makes sense! thanks!
– ShoeLace1291
Nov 15 '18 at 4:10
posting it as answer then :) glad to help
– ACD
Nov 15 '18 at 4:12
|
show 1 more comment
Have you checked to see what's running? For instance, do you ever get to thevar temp_vars = {
line? Any errors?
– CertainPerformance
Nov 15 '18 at 3:59
I have added console.log(temp_vars[variable]) just inside the for loop and it logs correctly.
– ShoeLace1291
Nov 15 '18 at 4:01
template.text(template.text().replace("{"+variable+"}", temp_vars[variable]))
– ACD
Nov 15 '18 at 4:05
@ACD that makes sense! thanks!
– ShoeLace1291
Nov 15 '18 at 4:10
posting it as answer then :) glad to help
– ACD
Nov 15 '18 at 4:12
Have you checked to see what's running? For instance, do you ever get to the
var temp_vars = {
line? Any errors?– CertainPerformance
Nov 15 '18 at 3:59
Have you checked to see what's running? For instance, do you ever get to the
var temp_vars = {
line? Any errors?– CertainPerformance
Nov 15 '18 at 3:59
I have added console.log(temp_vars[variable]) just inside the for loop and it logs correctly.
– ShoeLace1291
Nov 15 '18 at 4:01
I have added console.log(temp_vars[variable]) just inside the for loop and it logs correctly.
– ShoeLace1291
Nov 15 '18 at 4:01
template.text(template.text().replace("{"+variable+"}", temp_vars[variable]))
– ACD
Nov 15 '18 at 4:05
template.text(template.text().replace("{"+variable+"}", temp_vars[variable]))
– ACD
Nov 15 '18 at 4:05
@ACD that makes sense! thanks!
– ShoeLace1291
Nov 15 '18 at 4:10
@ACD that makes sense! thanks!
– ShoeLace1291
Nov 15 '18 at 4:10
posting it as answer then :) glad to help
– ACD
Nov 15 '18 at 4:12
posting it as answer then :) glad to help
– ACD
Nov 15 '18 at 4:12
|
show 1 more comment
1 Answer
1
active
oldest
votes
Code below will only replace the text without assigning it.
template.html().replace("{"+variable+"}", temp_vars[variable])
You have to assign after replacing the text to update the actual value.
template.html(template.html().replace("{"+variable+"}", temp_vars[variable]))
2
You're right, but can you explain a bit, so as to make the answer more useful to others?
– CertainPerformance
Nov 15 '18 at 4:12
template.text(value) would wipe out the html. Similarly getting the text() would ignore the html
– charlietfl
Nov 15 '18 at 4:19
yeah sorry acd but i jumped the gun. what charlietfl said is happening.
– ShoeLace1291
Nov 15 '18 at 4:20
obviously it will. why not use .html instead of text?
– ACD
Nov 15 '18 at 4:23
That would make more sense
– charlietfl
Nov 15 '18 at 4:25
|
show 11 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53312227%2fhow-do-i-replace-variables-in-html-with-an-object-of-variables-from-jquery%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Code below will only replace the text without assigning it.
template.html().replace("{"+variable+"}", temp_vars[variable])
You have to assign after replacing the text to update the actual value.
template.html(template.html().replace("{"+variable+"}", temp_vars[variable]))
2
You're right, but can you explain a bit, so as to make the answer more useful to others?
– CertainPerformance
Nov 15 '18 at 4:12
template.text(value) would wipe out the html. Similarly getting the text() would ignore the html
– charlietfl
Nov 15 '18 at 4:19
yeah sorry acd but i jumped the gun. what charlietfl said is happening.
– ShoeLace1291
Nov 15 '18 at 4:20
obviously it will. why not use .html instead of text?
– ACD
Nov 15 '18 at 4:23
That would make more sense
– charlietfl
Nov 15 '18 at 4:25
|
show 11 more comments
Code below will only replace the text without assigning it.
template.html().replace("{"+variable+"}", temp_vars[variable])
You have to assign after replacing the text to update the actual value.
template.html(template.html().replace("{"+variable+"}", temp_vars[variable]))
2
You're right, but can you explain a bit, so as to make the answer more useful to others?
– CertainPerformance
Nov 15 '18 at 4:12
template.text(value) would wipe out the html. Similarly getting the text() would ignore the html
– charlietfl
Nov 15 '18 at 4:19
yeah sorry acd but i jumped the gun. what charlietfl said is happening.
– ShoeLace1291
Nov 15 '18 at 4:20
obviously it will. why not use .html instead of text?
– ACD
Nov 15 '18 at 4:23
That would make more sense
– charlietfl
Nov 15 '18 at 4:25
|
show 11 more comments
Code below will only replace the text without assigning it.
template.html().replace("{"+variable+"}", temp_vars[variable])
You have to assign after replacing the text to update the actual value.
template.html(template.html().replace("{"+variable+"}", temp_vars[variable]))
Code below will only replace the text without assigning it.
template.html().replace("{"+variable+"}", temp_vars[variable])
You have to assign after replacing the text to update the actual value.
template.html(template.html().replace("{"+variable+"}", temp_vars[variable]))
edited Nov 15 '18 at 4:23
answered Nov 15 '18 at 4:11


ACDACD
8941112
8941112
2
You're right, but can you explain a bit, so as to make the answer more useful to others?
– CertainPerformance
Nov 15 '18 at 4:12
template.text(value) would wipe out the html. Similarly getting the text() would ignore the html
– charlietfl
Nov 15 '18 at 4:19
yeah sorry acd but i jumped the gun. what charlietfl said is happening.
– ShoeLace1291
Nov 15 '18 at 4:20
obviously it will. why not use .html instead of text?
– ACD
Nov 15 '18 at 4:23
That would make more sense
– charlietfl
Nov 15 '18 at 4:25
|
show 11 more comments
2
You're right, but can you explain a bit, so as to make the answer more useful to others?
– CertainPerformance
Nov 15 '18 at 4:12
template.text(value) would wipe out the html. Similarly getting the text() would ignore the html
– charlietfl
Nov 15 '18 at 4:19
yeah sorry acd but i jumped the gun. what charlietfl said is happening.
– ShoeLace1291
Nov 15 '18 at 4:20
obviously it will. why not use .html instead of text?
– ACD
Nov 15 '18 at 4:23
That would make more sense
– charlietfl
Nov 15 '18 at 4:25
2
2
You're right, but can you explain a bit, so as to make the answer more useful to others?
– CertainPerformance
Nov 15 '18 at 4:12
You're right, but can you explain a bit, so as to make the answer more useful to others?
– CertainPerformance
Nov 15 '18 at 4:12
template.text(value) would wipe out the html. Similarly getting the text() would ignore the html
– charlietfl
Nov 15 '18 at 4:19
template.text(value) would wipe out the html. Similarly getting the text() would ignore the html
– charlietfl
Nov 15 '18 at 4:19
yeah sorry acd but i jumped the gun. what charlietfl said is happening.
– ShoeLace1291
Nov 15 '18 at 4:20
yeah sorry acd but i jumped the gun. what charlietfl said is happening.
– ShoeLace1291
Nov 15 '18 at 4:20
obviously it will. why not use .html instead of text?
– ACD
Nov 15 '18 at 4:23
obviously it will. why not use .html instead of text?
– ACD
Nov 15 '18 at 4:23
That would make more sense
– charlietfl
Nov 15 '18 at 4:25
That would make more sense
– charlietfl
Nov 15 '18 at 4:25
|
show 11 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53312227%2fhow-do-i-replace-variables-in-html-with-an-object-of-variables-from-jquery%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
QBC5 RQEF Bp
Have you checked to see what's running? For instance, do you ever get to the
var temp_vars = {
line? Any errors?– CertainPerformance
Nov 15 '18 at 3:59
I have added console.log(temp_vars[variable]) just inside the for loop and it logs correctly.
– ShoeLace1291
Nov 15 '18 at 4:01
template.text(template.text().replace("{"+variable+"}", temp_vars[variable]))
– ACD
Nov 15 '18 at 4:05
@ACD that makes sense! thanks!
– ShoeLace1291
Nov 15 '18 at 4:10
posting it as answer then :) glad to help
– ACD
Nov 15 '18 at 4:12