Set a django object property through a subquery
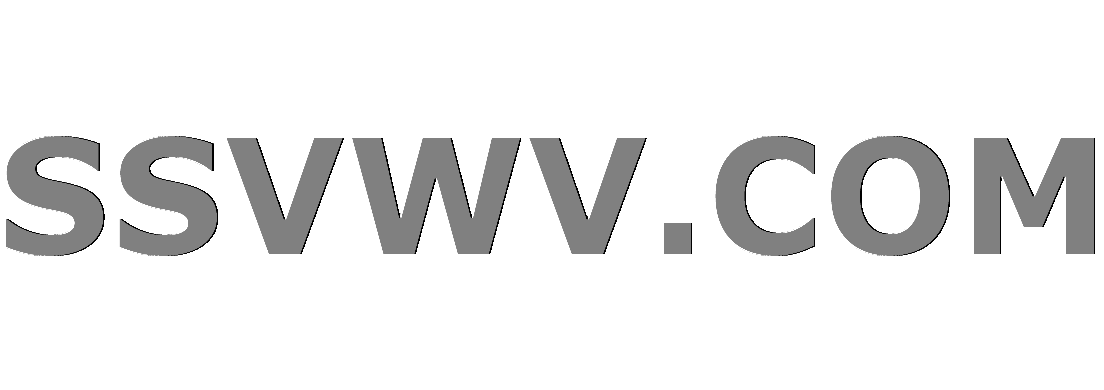
Multi tool use
I have an object of type Foo
with a ForeignKey to a Bar
. Bar
has a property called slug
.
I can do
myfoo.bar = Bar.objects.get(slug='123')
But this would add an extra query. Is it possible to have Django generate the assignment as a sub-query within the update?
i.e. generate SQL similar to:
UPDATE myfoo SET bar_id = (select id from bar where slug = '123')
django django-orm
add a comment |
I have an object of type Foo
with a ForeignKey to a Bar
. Bar
has a property called slug
.
I can do
myfoo.bar = Bar.objects.get(slug='123')
But this would add an extra query. Is it possible to have Django generate the assignment as a sub-query within the update?
i.e. generate SQL similar to:
UPDATE myfoo SET bar_id = (select id from bar where slug = '123')
django django-orm
add a comment |
I have an object of type Foo
with a ForeignKey to a Bar
. Bar
has a property called slug
.
I can do
myfoo.bar = Bar.objects.get(slug='123')
But this would add an extra query. Is it possible to have Django generate the assignment as a sub-query within the update?
i.e. generate SQL similar to:
UPDATE myfoo SET bar_id = (select id from bar where slug = '123')
django django-orm
I have an object of type Foo
with a ForeignKey to a Bar
. Bar
has a property called slug
.
I can do
myfoo.bar = Bar.objects.get(slug='123')
But this would add an extra query. Is it possible to have Django generate the assignment as a sub-query within the update?
i.e. generate SQL similar to:
UPDATE myfoo SET bar_id = (select id from bar where slug = '123')
django django-orm
django django-orm
edited Nov 14 '18 at 9:28


stellasia
2,16911126
2,16911126
asked Nov 14 '18 at 6:27
TordekTordek
7,03122861
7,03122861
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You can use a Django Subquery expression:
from django.db.models import Subquery
# create the "SELECT id FROM bar WHERE slug = '123' LIMIT 1" subquery
subquery = Bar.objects.filter(slug='123')[:1]
# update only the correct foo object with this subquery
Foo.objects.filter(pk=myfoo.pk).update(bar=Subquery(subquery))
# refresh the variable so that `myfoo.bar` is correct
myfoo.refresh_from_db()
Is it not possible to do it through simple assignment? I.e., I am setting several properties (including a set property) and I'd like to dofoo.a=1; foo.b=2; foo.c=[c,d,e]; foo.bar=Subquery(...)
. Though if I can doupdate(a=...,c=[...])
it could work.
– Tordek
Nov 14 '18 at 22:21
@Tordek Yes you have to useupdate
with multiple parameters. Otherwise, you will end up with a lot of queries.
– mistiru
Nov 15 '18 at 8:00
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53294276%2fset-a-django-object-property-through-a-subquery%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use a Django Subquery expression:
from django.db.models import Subquery
# create the "SELECT id FROM bar WHERE slug = '123' LIMIT 1" subquery
subquery = Bar.objects.filter(slug='123')[:1]
# update only the correct foo object with this subquery
Foo.objects.filter(pk=myfoo.pk).update(bar=Subquery(subquery))
# refresh the variable so that `myfoo.bar` is correct
myfoo.refresh_from_db()
Is it not possible to do it through simple assignment? I.e., I am setting several properties (including a set property) and I'd like to dofoo.a=1; foo.b=2; foo.c=[c,d,e]; foo.bar=Subquery(...)
. Though if I can doupdate(a=...,c=[...])
it could work.
– Tordek
Nov 14 '18 at 22:21
@Tordek Yes you have to useupdate
with multiple parameters. Otherwise, you will end up with a lot of queries.
– mistiru
Nov 15 '18 at 8:00
add a comment |
You can use a Django Subquery expression:
from django.db.models import Subquery
# create the "SELECT id FROM bar WHERE slug = '123' LIMIT 1" subquery
subquery = Bar.objects.filter(slug='123')[:1]
# update only the correct foo object with this subquery
Foo.objects.filter(pk=myfoo.pk).update(bar=Subquery(subquery))
# refresh the variable so that `myfoo.bar` is correct
myfoo.refresh_from_db()
Is it not possible to do it through simple assignment? I.e., I am setting several properties (including a set property) and I'd like to dofoo.a=1; foo.b=2; foo.c=[c,d,e]; foo.bar=Subquery(...)
. Though if I can doupdate(a=...,c=[...])
it could work.
– Tordek
Nov 14 '18 at 22:21
@Tordek Yes you have to useupdate
with multiple parameters. Otherwise, you will end up with a lot of queries.
– mistiru
Nov 15 '18 at 8:00
add a comment |
You can use a Django Subquery expression:
from django.db.models import Subquery
# create the "SELECT id FROM bar WHERE slug = '123' LIMIT 1" subquery
subquery = Bar.objects.filter(slug='123')[:1]
# update only the correct foo object with this subquery
Foo.objects.filter(pk=myfoo.pk).update(bar=Subquery(subquery))
# refresh the variable so that `myfoo.bar` is correct
myfoo.refresh_from_db()
You can use a Django Subquery expression:
from django.db.models import Subquery
# create the "SELECT id FROM bar WHERE slug = '123' LIMIT 1" subquery
subquery = Bar.objects.filter(slug='123')[:1]
# update only the correct foo object with this subquery
Foo.objects.filter(pk=myfoo.pk).update(bar=Subquery(subquery))
# refresh the variable so that `myfoo.bar` is correct
myfoo.refresh_from_db()
answered Nov 14 '18 at 9:51


mistirumistiru
410112
410112
Is it not possible to do it through simple assignment? I.e., I am setting several properties (including a set property) and I'd like to dofoo.a=1; foo.b=2; foo.c=[c,d,e]; foo.bar=Subquery(...)
. Though if I can doupdate(a=...,c=[...])
it could work.
– Tordek
Nov 14 '18 at 22:21
@Tordek Yes you have to useupdate
with multiple parameters. Otherwise, you will end up with a lot of queries.
– mistiru
Nov 15 '18 at 8:00
add a comment |
Is it not possible to do it through simple assignment? I.e., I am setting several properties (including a set property) and I'd like to dofoo.a=1; foo.b=2; foo.c=[c,d,e]; foo.bar=Subquery(...)
. Though if I can doupdate(a=...,c=[...])
it could work.
– Tordek
Nov 14 '18 at 22:21
@Tordek Yes you have to useupdate
with multiple parameters. Otherwise, you will end up with a lot of queries.
– mistiru
Nov 15 '18 at 8:00
Is it not possible to do it through simple assignment? I.e., I am setting several properties (including a set property) and I'd like to do
foo.a=1; foo.b=2; foo.c=[c,d,e]; foo.bar=Subquery(...)
. Though if I can do update(a=...,c=[...])
it could work.– Tordek
Nov 14 '18 at 22:21
Is it not possible to do it through simple assignment? I.e., I am setting several properties (including a set property) and I'd like to do
foo.a=1; foo.b=2; foo.c=[c,d,e]; foo.bar=Subquery(...)
. Though if I can do update(a=...,c=[...])
it could work.– Tordek
Nov 14 '18 at 22:21
@Tordek Yes you have to use
update
with multiple parameters. Otherwise, you will end up with a lot of queries.– mistiru
Nov 15 '18 at 8:00
@Tordek Yes you have to use
update
with multiple parameters. Otherwise, you will end up with a lot of queries.– mistiru
Nov 15 '18 at 8:00
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53294276%2fset-a-django-object-property-through-a-subquery%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
caDnyX,MpSZ