Javascript alert on incomplete variables
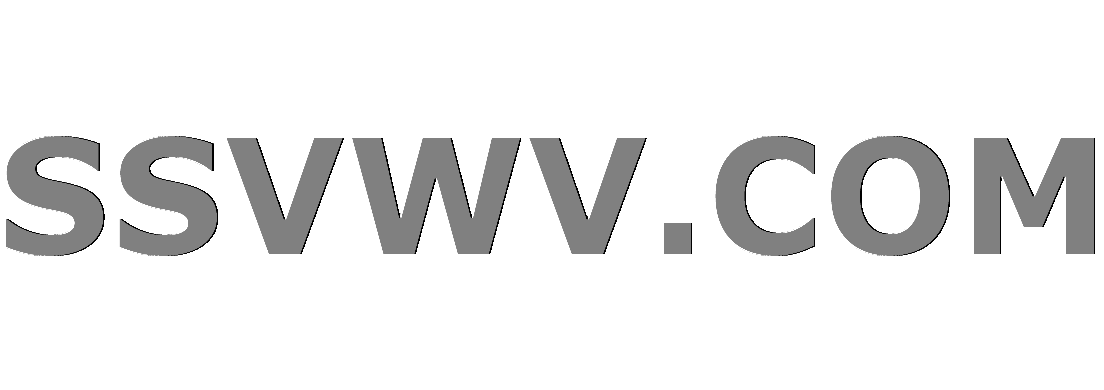
Multi tool use
i'm having troubles on javascript arithmetic.
lets say i have 2 elements from html,
var num1 = document.getElementByID('one').value;
var num2 = docuemnt.getElementByID('two').value;
var total = num1 + num2;
alert(total);
these two elements are inputted by users. lets say the user left the id='one' blank. how do i make javascript still compute and alert 'total'? can javascript automatically set the blank field to '0'? i tried this on my project but it will only give me an alert if both 'one' and 'two' were given/inputted. maybe i'm missing something.
Thanks for any help.
ok this is the code i'm using on my project,
var number = document.getElementById('child1').value;
var hrs = document.getElementById('hrs1').value;
var qty = document.getElementById('qty1').value;
var watts = qty * (number / 1000);
var kwh = watts * hrs;
var kwhh = kwh * 30;
var number2 = document.getElementById('child2').value;
var hrs2 = document.getElementById('hrs2').value;
var qty2 = document.getElementById('qty2').value;
var watts2 = qty2 * (number2 / 1000);
var kwh2 = watts2 * hrs2;
var kwhh2 = kwh2 * 30;
var totalperhr = kwh+kwh2;
var totalper30 = kwhh+kwhh2;
alert(totalperhr + ' kwh per dayn' + totalper30 + ' kwh per 30 days');
html below,
<input type="range" min="1" max="1000" id="child1">
<select id="hrs1">
<option value='1'>1hr</option>
<option value='2'>2hr</option>
<option value='3'>3hr</option>
</select>
<select id="qty1">
<option value='1'>1</option>
<option value='2'>2</option>
<option value='2'>3</option>
</select>
<input type="range" min="1" max="1000" id="child2">
<select id="hrs2">
<option value='1'>1hr</option>
<option value='2'>2hr</option>
<option value='3'>3hr</option>
</select>
<select id="qty2">
<option value='1'>1</option>
<option value='2'>2</option>
<option value='2'>3</option>
</select>
as you can see, i'm going thru several operations then getting the grand total. but i'm not getting any alerts if i leave one blank.
javascript html
add a comment |
i'm having troubles on javascript arithmetic.
lets say i have 2 elements from html,
var num1 = document.getElementByID('one').value;
var num2 = docuemnt.getElementByID('two').value;
var total = num1 + num2;
alert(total);
these two elements are inputted by users. lets say the user left the id='one' blank. how do i make javascript still compute and alert 'total'? can javascript automatically set the blank field to '0'? i tried this on my project but it will only give me an alert if both 'one' and 'two' were given/inputted. maybe i'm missing something.
Thanks for any help.
ok this is the code i'm using on my project,
var number = document.getElementById('child1').value;
var hrs = document.getElementById('hrs1').value;
var qty = document.getElementById('qty1').value;
var watts = qty * (number / 1000);
var kwh = watts * hrs;
var kwhh = kwh * 30;
var number2 = document.getElementById('child2').value;
var hrs2 = document.getElementById('hrs2').value;
var qty2 = document.getElementById('qty2').value;
var watts2 = qty2 * (number2 / 1000);
var kwh2 = watts2 * hrs2;
var kwhh2 = kwh2 * 30;
var totalperhr = kwh+kwh2;
var totalper30 = kwhh+kwhh2;
alert(totalperhr + ' kwh per dayn' + totalper30 + ' kwh per 30 days');
html below,
<input type="range" min="1" max="1000" id="child1">
<select id="hrs1">
<option value='1'>1hr</option>
<option value='2'>2hr</option>
<option value='3'>3hr</option>
</select>
<select id="qty1">
<option value='1'>1</option>
<option value='2'>2</option>
<option value='2'>3</option>
</select>
<input type="range" min="1" max="1000" id="child2">
<select id="hrs2">
<option value='1'>1hr</option>
<option value='2'>2hr</option>
<option value='3'>3hr</option>
</select>
<select id="qty2">
<option value='1'>1</option>
<option value='2'>2</option>
<option value='2'>3</option>
</select>
as you can see, i'm going thru several operations then getting the grand total. but i'm not getting any alerts if i leave one blank.
javascript html
2
Sounds odd, it shouldn't throw an error - if the value is empty, it will default to the empty string, which is perfectly concatenatable
– CertainPerformance
Nov 14 '18 at 6:15
FYI,<input>
value
properties are always strings, even<input type="number">
– Phil
Nov 14 '18 at 6:19
add a comment |
i'm having troubles on javascript arithmetic.
lets say i have 2 elements from html,
var num1 = document.getElementByID('one').value;
var num2 = docuemnt.getElementByID('two').value;
var total = num1 + num2;
alert(total);
these two elements are inputted by users. lets say the user left the id='one' blank. how do i make javascript still compute and alert 'total'? can javascript automatically set the blank field to '0'? i tried this on my project but it will only give me an alert if both 'one' and 'two' were given/inputted. maybe i'm missing something.
Thanks for any help.
ok this is the code i'm using on my project,
var number = document.getElementById('child1').value;
var hrs = document.getElementById('hrs1').value;
var qty = document.getElementById('qty1').value;
var watts = qty * (number / 1000);
var kwh = watts * hrs;
var kwhh = kwh * 30;
var number2 = document.getElementById('child2').value;
var hrs2 = document.getElementById('hrs2').value;
var qty2 = document.getElementById('qty2').value;
var watts2 = qty2 * (number2 / 1000);
var kwh2 = watts2 * hrs2;
var kwhh2 = kwh2 * 30;
var totalperhr = kwh+kwh2;
var totalper30 = kwhh+kwhh2;
alert(totalperhr + ' kwh per dayn' + totalper30 + ' kwh per 30 days');
html below,
<input type="range" min="1" max="1000" id="child1">
<select id="hrs1">
<option value='1'>1hr</option>
<option value='2'>2hr</option>
<option value='3'>3hr</option>
</select>
<select id="qty1">
<option value='1'>1</option>
<option value='2'>2</option>
<option value='2'>3</option>
</select>
<input type="range" min="1" max="1000" id="child2">
<select id="hrs2">
<option value='1'>1hr</option>
<option value='2'>2hr</option>
<option value='3'>3hr</option>
</select>
<select id="qty2">
<option value='1'>1</option>
<option value='2'>2</option>
<option value='2'>3</option>
</select>
as you can see, i'm going thru several operations then getting the grand total. but i'm not getting any alerts if i leave one blank.
javascript html
i'm having troubles on javascript arithmetic.
lets say i have 2 elements from html,
var num1 = document.getElementByID('one').value;
var num2 = docuemnt.getElementByID('two').value;
var total = num1 + num2;
alert(total);
these two elements are inputted by users. lets say the user left the id='one' blank. how do i make javascript still compute and alert 'total'? can javascript automatically set the blank field to '0'? i tried this on my project but it will only give me an alert if both 'one' and 'two' were given/inputted. maybe i'm missing something.
Thanks for any help.
ok this is the code i'm using on my project,
var number = document.getElementById('child1').value;
var hrs = document.getElementById('hrs1').value;
var qty = document.getElementById('qty1').value;
var watts = qty * (number / 1000);
var kwh = watts * hrs;
var kwhh = kwh * 30;
var number2 = document.getElementById('child2').value;
var hrs2 = document.getElementById('hrs2').value;
var qty2 = document.getElementById('qty2').value;
var watts2 = qty2 * (number2 / 1000);
var kwh2 = watts2 * hrs2;
var kwhh2 = kwh2 * 30;
var totalperhr = kwh+kwh2;
var totalper30 = kwhh+kwhh2;
alert(totalperhr + ' kwh per dayn' + totalper30 + ' kwh per 30 days');
html below,
<input type="range" min="1" max="1000" id="child1">
<select id="hrs1">
<option value='1'>1hr</option>
<option value='2'>2hr</option>
<option value='3'>3hr</option>
</select>
<select id="qty1">
<option value='1'>1</option>
<option value='2'>2</option>
<option value='2'>3</option>
</select>
<input type="range" min="1" max="1000" id="child2">
<select id="hrs2">
<option value='1'>1hr</option>
<option value='2'>2hr</option>
<option value='3'>3hr</option>
</select>
<select id="qty2">
<option value='1'>1</option>
<option value='2'>2</option>
<option value='2'>3</option>
</select>
as you can see, i'm going thru several operations then getting the grand total. but i'm not getting any alerts if i leave one blank.
javascript html
javascript html
edited Nov 14 '18 at 6:48
Kim Lo
asked Nov 14 '18 at 6:14
Kim LoKim Lo
295
295
2
Sounds odd, it shouldn't throw an error - if the value is empty, it will default to the empty string, which is perfectly concatenatable
– CertainPerformance
Nov 14 '18 at 6:15
FYI,<input>
value
properties are always strings, even<input type="number">
– Phil
Nov 14 '18 at 6:19
add a comment |
2
Sounds odd, it shouldn't throw an error - if the value is empty, it will default to the empty string, which is perfectly concatenatable
– CertainPerformance
Nov 14 '18 at 6:15
FYI,<input>
value
properties are always strings, even<input type="number">
– Phil
Nov 14 '18 at 6:19
2
2
Sounds odd, it shouldn't throw an error - if the value is empty, it will default to the empty string, which is perfectly concatenatable
– CertainPerformance
Nov 14 '18 at 6:15
Sounds odd, it shouldn't throw an error - if the value is empty, it will default to the empty string, which is perfectly concatenatable
– CertainPerformance
Nov 14 '18 at 6:15
FYI,
<input>
value
properties are always strings, even <input type="number">
– Phil
Nov 14 '18 at 6:19
FYI,
<input>
value
properties are always strings, even <input type="number">
– Phil
Nov 14 '18 at 6:19
add a comment |
1 Answer
1
active
oldest
votes
You can use Unary plus (+) operator to get the number out of the value
property of your input, or set it to 0
:
+document.getElementById('one').value || 0;
Code example:
const num1 = +document.getElementById('one').value || 0;
const num2 = +document.getElementById('two').value || 0;
const total = num1 + num2;
console.log(total);
<input type="number" value="5" name="one" id="one">
<input type="number" value="" name="two" id="two">
Note: instead of document.getElementByID()
you should write document.getElementById()
Your code:
const elements = document.querySelectorAll('#child1, #hrs1, #qty1, #child2, #hrs2, #qty2');
const values = {
child1: 0,
hrs1: 0,
qty1: 0,
child2: 0,
hrs2: 0,
qty2: 0
};
const getTotals = () => {
elements.forEach(elem => values[elem.id] = +elem.value || 0);
const watts = values.qty1 * (values.child1 / 1000);
const kwh = watts * values.hrs1;
const kwhh = kwh * 30;
const watts2 = values.qty2 * (values.child2 / 1000);
const kwh2 = watts2 * values.hrs2;
const kwhh2 = kwh2 * 30;
const totalperhr = kwh + kwh2;
const totalper30 = kwhh + kwhh2;
console.clear();
console.log(values);
console.log(totalperhr + ' kwh per dayn' + totalper30 + ' kwh per 30 days');
};
elements.forEach(elem => elem.addEventListener('change', getTotals));
<input type="range" min="1" max="1000" id="child1">
<select id="hrs1">
<option value=""></option>
<option value="1">1hr</option>
<option value="2">2hr</option>
<option value="3">3hr</option>
</select>
<select id="qty1">
<option value=""></option>
<option value="1">1</option>
<option value="2">2</option>
<option value="2">3</option>
</select>
<input type="range" min="1" max="1000" id="child2">
<select id="hrs2">
<option value=""></option>
<option value="1">1hr</option>
<option value="2">2hr</option>
<option value="3">3hr</option>
</select>
<select id="qty2">
<option value=""></option>
<option value="1">1</option>
<option value="2">2</option>
<option value="2">3</option>
</select>
hi sir, i edited my question above with my actual code i'm using. i tried this but can't get it to work.
– Kim Lo
Nov 14 '18 at 6:38
Can you also add thehtml
code please?
– Yosvel Quintero
Nov 14 '18 at 6:39
-1, the answer is misleading. As @CertainPerformance has pointed out "if the value is empty, it will default to the empty string, which is perfectly concatenatable". I. e.,console.log(42 + '');
returns42
. This snippet will also work after removing the unary operator and the|| 0
.
– David
Nov 14 '18 at 6:44
1
@David that is a not good comment. I will never recommend to anybody that uses astring
in a matematic operation.. Because if there is a number what will happen?:console.log(42 + '0');
return420
– Yosvel Quintero
Nov 14 '18 at 6:48
1
@YosvelQuintero - That's completely correct. I didn't say that your answer is wrong. I said that is misleading because clearly that's not the OP problem. At least, not the only one.
– David
Nov 14 '18 at 6:49
|
show 3 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53294151%2fjavascript-alert-on-incomplete-variables%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use Unary plus (+) operator to get the number out of the value
property of your input, or set it to 0
:
+document.getElementById('one').value || 0;
Code example:
const num1 = +document.getElementById('one').value || 0;
const num2 = +document.getElementById('two').value || 0;
const total = num1 + num2;
console.log(total);
<input type="number" value="5" name="one" id="one">
<input type="number" value="" name="two" id="two">
Note: instead of document.getElementByID()
you should write document.getElementById()
Your code:
const elements = document.querySelectorAll('#child1, #hrs1, #qty1, #child2, #hrs2, #qty2');
const values = {
child1: 0,
hrs1: 0,
qty1: 0,
child2: 0,
hrs2: 0,
qty2: 0
};
const getTotals = () => {
elements.forEach(elem => values[elem.id] = +elem.value || 0);
const watts = values.qty1 * (values.child1 / 1000);
const kwh = watts * values.hrs1;
const kwhh = kwh * 30;
const watts2 = values.qty2 * (values.child2 / 1000);
const kwh2 = watts2 * values.hrs2;
const kwhh2 = kwh2 * 30;
const totalperhr = kwh + kwh2;
const totalper30 = kwhh + kwhh2;
console.clear();
console.log(values);
console.log(totalperhr + ' kwh per dayn' + totalper30 + ' kwh per 30 days');
};
elements.forEach(elem => elem.addEventListener('change', getTotals));
<input type="range" min="1" max="1000" id="child1">
<select id="hrs1">
<option value=""></option>
<option value="1">1hr</option>
<option value="2">2hr</option>
<option value="3">3hr</option>
</select>
<select id="qty1">
<option value=""></option>
<option value="1">1</option>
<option value="2">2</option>
<option value="2">3</option>
</select>
<input type="range" min="1" max="1000" id="child2">
<select id="hrs2">
<option value=""></option>
<option value="1">1hr</option>
<option value="2">2hr</option>
<option value="3">3hr</option>
</select>
<select id="qty2">
<option value=""></option>
<option value="1">1</option>
<option value="2">2</option>
<option value="2">3</option>
</select>
hi sir, i edited my question above with my actual code i'm using. i tried this but can't get it to work.
– Kim Lo
Nov 14 '18 at 6:38
Can you also add thehtml
code please?
– Yosvel Quintero
Nov 14 '18 at 6:39
-1, the answer is misleading. As @CertainPerformance has pointed out "if the value is empty, it will default to the empty string, which is perfectly concatenatable". I. e.,console.log(42 + '');
returns42
. This snippet will also work after removing the unary operator and the|| 0
.
– David
Nov 14 '18 at 6:44
1
@David that is a not good comment. I will never recommend to anybody that uses astring
in a matematic operation.. Because if there is a number what will happen?:console.log(42 + '0');
return420
– Yosvel Quintero
Nov 14 '18 at 6:48
1
@YosvelQuintero - That's completely correct. I didn't say that your answer is wrong. I said that is misleading because clearly that's not the OP problem. At least, not the only one.
– David
Nov 14 '18 at 6:49
|
show 3 more comments
You can use Unary plus (+) operator to get the number out of the value
property of your input, or set it to 0
:
+document.getElementById('one').value || 0;
Code example:
const num1 = +document.getElementById('one').value || 0;
const num2 = +document.getElementById('two').value || 0;
const total = num1 + num2;
console.log(total);
<input type="number" value="5" name="one" id="one">
<input type="number" value="" name="two" id="two">
Note: instead of document.getElementByID()
you should write document.getElementById()
Your code:
const elements = document.querySelectorAll('#child1, #hrs1, #qty1, #child2, #hrs2, #qty2');
const values = {
child1: 0,
hrs1: 0,
qty1: 0,
child2: 0,
hrs2: 0,
qty2: 0
};
const getTotals = () => {
elements.forEach(elem => values[elem.id] = +elem.value || 0);
const watts = values.qty1 * (values.child1 / 1000);
const kwh = watts * values.hrs1;
const kwhh = kwh * 30;
const watts2 = values.qty2 * (values.child2 / 1000);
const kwh2 = watts2 * values.hrs2;
const kwhh2 = kwh2 * 30;
const totalperhr = kwh + kwh2;
const totalper30 = kwhh + kwhh2;
console.clear();
console.log(values);
console.log(totalperhr + ' kwh per dayn' + totalper30 + ' kwh per 30 days');
};
elements.forEach(elem => elem.addEventListener('change', getTotals));
<input type="range" min="1" max="1000" id="child1">
<select id="hrs1">
<option value=""></option>
<option value="1">1hr</option>
<option value="2">2hr</option>
<option value="3">3hr</option>
</select>
<select id="qty1">
<option value=""></option>
<option value="1">1</option>
<option value="2">2</option>
<option value="2">3</option>
</select>
<input type="range" min="1" max="1000" id="child2">
<select id="hrs2">
<option value=""></option>
<option value="1">1hr</option>
<option value="2">2hr</option>
<option value="3">3hr</option>
</select>
<select id="qty2">
<option value=""></option>
<option value="1">1</option>
<option value="2">2</option>
<option value="2">3</option>
</select>
hi sir, i edited my question above with my actual code i'm using. i tried this but can't get it to work.
– Kim Lo
Nov 14 '18 at 6:38
Can you also add thehtml
code please?
– Yosvel Quintero
Nov 14 '18 at 6:39
-1, the answer is misleading. As @CertainPerformance has pointed out "if the value is empty, it will default to the empty string, which is perfectly concatenatable". I. e.,console.log(42 + '');
returns42
. This snippet will also work after removing the unary operator and the|| 0
.
– David
Nov 14 '18 at 6:44
1
@David that is a not good comment. I will never recommend to anybody that uses astring
in a matematic operation.. Because if there is a number what will happen?:console.log(42 + '0');
return420
– Yosvel Quintero
Nov 14 '18 at 6:48
1
@YosvelQuintero - That's completely correct. I didn't say that your answer is wrong. I said that is misleading because clearly that's not the OP problem. At least, not the only one.
– David
Nov 14 '18 at 6:49
|
show 3 more comments
You can use Unary plus (+) operator to get the number out of the value
property of your input, or set it to 0
:
+document.getElementById('one').value || 0;
Code example:
const num1 = +document.getElementById('one').value || 0;
const num2 = +document.getElementById('two').value || 0;
const total = num1 + num2;
console.log(total);
<input type="number" value="5" name="one" id="one">
<input type="number" value="" name="two" id="two">
Note: instead of document.getElementByID()
you should write document.getElementById()
Your code:
const elements = document.querySelectorAll('#child1, #hrs1, #qty1, #child2, #hrs2, #qty2');
const values = {
child1: 0,
hrs1: 0,
qty1: 0,
child2: 0,
hrs2: 0,
qty2: 0
};
const getTotals = () => {
elements.forEach(elem => values[elem.id] = +elem.value || 0);
const watts = values.qty1 * (values.child1 / 1000);
const kwh = watts * values.hrs1;
const kwhh = kwh * 30;
const watts2 = values.qty2 * (values.child2 / 1000);
const kwh2 = watts2 * values.hrs2;
const kwhh2 = kwh2 * 30;
const totalperhr = kwh + kwh2;
const totalper30 = kwhh + kwhh2;
console.clear();
console.log(values);
console.log(totalperhr + ' kwh per dayn' + totalper30 + ' kwh per 30 days');
};
elements.forEach(elem => elem.addEventListener('change', getTotals));
<input type="range" min="1" max="1000" id="child1">
<select id="hrs1">
<option value=""></option>
<option value="1">1hr</option>
<option value="2">2hr</option>
<option value="3">3hr</option>
</select>
<select id="qty1">
<option value=""></option>
<option value="1">1</option>
<option value="2">2</option>
<option value="2">3</option>
</select>
<input type="range" min="1" max="1000" id="child2">
<select id="hrs2">
<option value=""></option>
<option value="1">1hr</option>
<option value="2">2hr</option>
<option value="3">3hr</option>
</select>
<select id="qty2">
<option value=""></option>
<option value="1">1</option>
<option value="2">2</option>
<option value="2">3</option>
</select>
You can use Unary plus (+) operator to get the number out of the value
property of your input, or set it to 0
:
+document.getElementById('one').value || 0;
Code example:
const num1 = +document.getElementById('one').value || 0;
const num2 = +document.getElementById('two').value || 0;
const total = num1 + num2;
console.log(total);
<input type="number" value="5" name="one" id="one">
<input type="number" value="" name="two" id="two">
Note: instead of document.getElementByID()
you should write document.getElementById()
Your code:
const elements = document.querySelectorAll('#child1, #hrs1, #qty1, #child2, #hrs2, #qty2');
const values = {
child1: 0,
hrs1: 0,
qty1: 0,
child2: 0,
hrs2: 0,
qty2: 0
};
const getTotals = () => {
elements.forEach(elem => values[elem.id] = +elem.value || 0);
const watts = values.qty1 * (values.child1 / 1000);
const kwh = watts * values.hrs1;
const kwhh = kwh * 30;
const watts2 = values.qty2 * (values.child2 / 1000);
const kwh2 = watts2 * values.hrs2;
const kwhh2 = kwh2 * 30;
const totalperhr = kwh + kwh2;
const totalper30 = kwhh + kwhh2;
console.clear();
console.log(values);
console.log(totalperhr + ' kwh per dayn' + totalper30 + ' kwh per 30 days');
};
elements.forEach(elem => elem.addEventListener('change', getTotals));
<input type="range" min="1" max="1000" id="child1">
<select id="hrs1">
<option value=""></option>
<option value="1">1hr</option>
<option value="2">2hr</option>
<option value="3">3hr</option>
</select>
<select id="qty1">
<option value=""></option>
<option value="1">1</option>
<option value="2">2</option>
<option value="2">3</option>
</select>
<input type="range" min="1" max="1000" id="child2">
<select id="hrs2">
<option value=""></option>
<option value="1">1hr</option>
<option value="2">2hr</option>
<option value="3">3hr</option>
</select>
<select id="qty2">
<option value=""></option>
<option value="1">1</option>
<option value="2">2</option>
<option value="2">3</option>
</select>
const num1 = +document.getElementById('one').value || 0;
const num2 = +document.getElementById('two').value || 0;
const total = num1 + num2;
console.log(total);
<input type="number" value="5" name="one" id="one">
<input type="number" value="" name="two" id="two">
const num1 = +document.getElementById('one').value || 0;
const num2 = +document.getElementById('two').value || 0;
const total = num1 + num2;
console.log(total);
<input type="number" value="5" name="one" id="one">
<input type="number" value="" name="two" id="two">
const elements = document.querySelectorAll('#child1, #hrs1, #qty1, #child2, #hrs2, #qty2');
const values = {
child1: 0,
hrs1: 0,
qty1: 0,
child2: 0,
hrs2: 0,
qty2: 0
};
const getTotals = () => {
elements.forEach(elem => values[elem.id] = +elem.value || 0);
const watts = values.qty1 * (values.child1 / 1000);
const kwh = watts * values.hrs1;
const kwhh = kwh * 30;
const watts2 = values.qty2 * (values.child2 / 1000);
const kwh2 = watts2 * values.hrs2;
const kwhh2 = kwh2 * 30;
const totalperhr = kwh + kwh2;
const totalper30 = kwhh + kwhh2;
console.clear();
console.log(values);
console.log(totalperhr + ' kwh per dayn' + totalper30 + ' kwh per 30 days');
};
elements.forEach(elem => elem.addEventListener('change', getTotals));
<input type="range" min="1" max="1000" id="child1">
<select id="hrs1">
<option value=""></option>
<option value="1">1hr</option>
<option value="2">2hr</option>
<option value="3">3hr</option>
</select>
<select id="qty1">
<option value=""></option>
<option value="1">1</option>
<option value="2">2</option>
<option value="2">3</option>
</select>
<input type="range" min="1" max="1000" id="child2">
<select id="hrs2">
<option value=""></option>
<option value="1">1hr</option>
<option value="2">2hr</option>
<option value="3">3hr</option>
</select>
<select id="qty2">
<option value=""></option>
<option value="1">1</option>
<option value="2">2</option>
<option value="2">3</option>
</select>
const elements = document.querySelectorAll('#child1, #hrs1, #qty1, #child2, #hrs2, #qty2');
const values = {
child1: 0,
hrs1: 0,
qty1: 0,
child2: 0,
hrs2: 0,
qty2: 0
};
const getTotals = () => {
elements.forEach(elem => values[elem.id] = +elem.value || 0);
const watts = values.qty1 * (values.child1 / 1000);
const kwh = watts * values.hrs1;
const kwhh = kwh * 30;
const watts2 = values.qty2 * (values.child2 / 1000);
const kwh2 = watts2 * values.hrs2;
const kwhh2 = kwh2 * 30;
const totalperhr = kwh + kwh2;
const totalper30 = kwhh + kwhh2;
console.clear();
console.log(values);
console.log(totalperhr + ' kwh per dayn' + totalper30 + ' kwh per 30 days');
};
elements.forEach(elem => elem.addEventListener('change', getTotals));
<input type="range" min="1" max="1000" id="child1">
<select id="hrs1">
<option value=""></option>
<option value="1">1hr</option>
<option value="2">2hr</option>
<option value="3">3hr</option>
</select>
<select id="qty1">
<option value=""></option>
<option value="1">1</option>
<option value="2">2</option>
<option value="2">3</option>
</select>
<input type="range" min="1" max="1000" id="child2">
<select id="hrs2">
<option value=""></option>
<option value="1">1hr</option>
<option value="2">2hr</option>
<option value="3">3hr</option>
</select>
<select id="qty2">
<option value=""></option>
<option value="1">1</option>
<option value="2">2</option>
<option value="2">3</option>
</select>
edited Nov 14 '18 at 7:45
answered Nov 14 '18 at 6:17
Yosvel QuinteroYosvel Quintero
11k42429
11k42429
hi sir, i edited my question above with my actual code i'm using. i tried this but can't get it to work.
– Kim Lo
Nov 14 '18 at 6:38
Can you also add thehtml
code please?
– Yosvel Quintero
Nov 14 '18 at 6:39
-1, the answer is misleading. As @CertainPerformance has pointed out "if the value is empty, it will default to the empty string, which is perfectly concatenatable". I. e.,console.log(42 + '');
returns42
. This snippet will also work after removing the unary operator and the|| 0
.
– David
Nov 14 '18 at 6:44
1
@David that is a not good comment. I will never recommend to anybody that uses astring
in a matematic operation.. Because if there is a number what will happen?:console.log(42 + '0');
return420
– Yosvel Quintero
Nov 14 '18 at 6:48
1
@YosvelQuintero - That's completely correct. I didn't say that your answer is wrong. I said that is misleading because clearly that's not the OP problem. At least, not the only one.
– David
Nov 14 '18 at 6:49
|
show 3 more comments
hi sir, i edited my question above with my actual code i'm using. i tried this but can't get it to work.
– Kim Lo
Nov 14 '18 at 6:38
Can you also add thehtml
code please?
– Yosvel Quintero
Nov 14 '18 at 6:39
-1, the answer is misleading. As @CertainPerformance has pointed out "if the value is empty, it will default to the empty string, which is perfectly concatenatable". I. e.,console.log(42 + '');
returns42
. This snippet will also work after removing the unary operator and the|| 0
.
– David
Nov 14 '18 at 6:44
1
@David that is a not good comment. I will never recommend to anybody that uses astring
in a matematic operation.. Because if there is a number what will happen?:console.log(42 + '0');
return420
– Yosvel Quintero
Nov 14 '18 at 6:48
1
@YosvelQuintero - That's completely correct. I didn't say that your answer is wrong. I said that is misleading because clearly that's not the OP problem. At least, not the only one.
– David
Nov 14 '18 at 6:49
hi sir, i edited my question above with my actual code i'm using. i tried this but can't get it to work.
– Kim Lo
Nov 14 '18 at 6:38
hi sir, i edited my question above with my actual code i'm using. i tried this but can't get it to work.
– Kim Lo
Nov 14 '18 at 6:38
Can you also add the
html
code please?– Yosvel Quintero
Nov 14 '18 at 6:39
Can you also add the
html
code please?– Yosvel Quintero
Nov 14 '18 at 6:39
-1, the answer is misleading. As @CertainPerformance has pointed out "if the value is empty, it will default to the empty string, which is perfectly concatenatable". I. e.,
console.log(42 + '');
returns 42
. This snippet will also work after removing the unary operator and the || 0
.– David
Nov 14 '18 at 6:44
-1, the answer is misleading. As @CertainPerformance has pointed out "if the value is empty, it will default to the empty string, which is perfectly concatenatable". I. e.,
console.log(42 + '');
returns 42
. This snippet will also work after removing the unary operator and the || 0
.– David
Nov 14 '18 at 6:44
1
1
@David that is a not good comment. I will never recommend to anybody that uses a
string
in a matematic operation.. Because if there is a number what will happen?: console.log(42 + '0');
return 420
– Yosvel Quintero
Nov 14 '18 at 6:48
@David that is a not good comment. I will never recommend to anybody that uses a
string
in a matematic operation.. Because if there is a number what will happen?: console.log(42 + '0');
return 420
– Yosvel Quintero
Nov 14 '18 at 6:48
1
1
@YosvelQuintero - That's completely correct. I didn't say that your answer is wrong. I said that is misleading because clearly that's not the OP problem. At least, not the only one.
– David
Nov 14 '18 at 6:49
@YosvelQuintero - That's completely correct. I didn't say that your answer is wrong. I said that is misleading because clearly that's not the OP problem. At least, not the only one.
– David
Nov 14 '18 at 6:49
|
show 3 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53294151%2fjavascript-alert-on-incomplete-variables%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
A 2l2HEz09vw 3cT fogwZ,wnulb,RvNrJ4ijuSOIeqx1jo 2n
2
Sounds odd, it shouldn't throw an error - if the value is empty, it will default to the empty string, which is perfectly concatenatable
– CertainPerformance
Nov 14 '18 at 6:15
FYI,
<input>
value
properties are always strings, even<input type="number">
– Phil
Nov 14 '18 at 6:19