Angular 6 Forms Cannot find control error
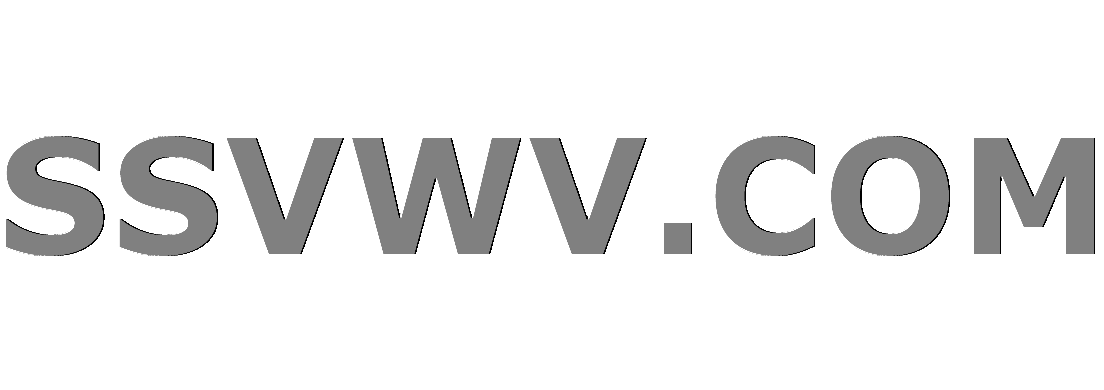
Multi tool use
I am trying to build form arrays in Angular. When the data didn't show up, I minimized the code to show behavior. I must use form arrays for the final code. Help me please get through this. I'm using Angular 6.
Why am I getting this error:
Error: Cannot find control with name: 'resultOptions'
// My component .ts file
import {FormControl, FormArray, FormBuilder, FormGroup} from '@angular/forms';
export class MyComponent implements OnInit, OnDestroy {
private resultForm: FormGroup;
private destroyed$: Subject<boolean> = new Subject();
resultData = [
{
text: 'My Text',
}];
constructor(private formBuilder: FormBuilder) {
this.resultForm = this.formBuilder.group({
childData: this.formBuilder.array()
});
this.setMainOptions();
}
setMainOptions() {
const control = <FormArray>this.resultForm.controls.childData;
this.resultData.forEach(x => {
control.push(this.formBuilder.group({
text: new FormControl()}))
})
}
ngOnInit() {
}
ngOnDestroy() {
this.destroyed$.complete();
}
}
// my html
<form [formGroup]="resultForm">
<div formArrayName="resultOptions">
<div *ngFor="let mainOption of resultForm.get('childData').controls; let i=index">
<div [formGroupName]="i">
<input [formControlName]="text" />
</div>
</div>
</div>
</form>
<pre>{{resultForm.value | json}}</pre>
Greatly appreciated!
angular angular-forms angular-formbuilder
add a comment |
I am trying to build form arrays in Angular. When the data didn't show up, I minimized the code to show behavior. I must use form arrays for the final code. Help me please get through this. I'm using Angular 6.
Why am I getting this error:
Error: Cannot find control with name: 'resultOptions'
// My component .ts file
import {FormControl, FormArray, FormBuilder, FormGroup} from '@angular/forms';
export class MyComponent implements OnInit, OnDestroy {
private resultForm: FormGroup;
private destroyed$: Subject<boolean> = new Subject();
resultData = [
{
text: 'My Text',
}];
constructor(private formBuilder: FormBuilder) {
this.resultForm = this.formBuilder.group({
childData: this.formBuilder.array()
});
this.setMainOptions();
}
setMainOptions() {
const control = <FormArray>this.resultForm.controls.childData;
this.resultData.forEach(x => {
control.push(this.formBuilder.group({
text: new FormControl()}))
})
}
ngOnInit() {
}
ngOnDestroy() {
this.destroyed$.complete();
}
}
// my html
<form [formGroup]="resultForm">
<div formArrayName="resultOptions">
<div *ngFor="let mainOption of resultForm.get('childData').controls; let i=index">
<div [formGroupName]="i">
<input [formControlName]="text" />
</div>
</div>
</div>
</form>
<pre>{{resultForm.value | json}}</pre>
Greatly appreciated!
angular angular-forms angular-formbuilder
1
can you post a minimal stackblitz ? That will help greatly
– Akber Iqbal
Nov 14 '18 at 6:12
1
Try usingchildData
instead ofresultOptions
inside HTML.
– Vishw Patel
Nov 14 '18 at 6:13
1
change ` formArrayName="resultOptions"` to ` formArrayName="childData"`. The formArrayName must match what you pass it in the formGroup.
– pixelbits
Nov 14 '18 at 6:14
share yourhtml
view as well to see what is actually referenced there
– Farooq Khan
Nov 14 '18 at 6:18
add a comment |
I am trying to build form arrays in Angular. When the data didn't show up, I minimized the code to show behavior. I must use form arrays for the final code. Help me please get through this. I'm using Angular 6.
Why am I getting this error:
Error: Cannot find control with name: 'resultOptions'
// My component .ts file
import {FormControl, FormArray, FormBuilder, FormGroup} from '@angular/forms';
export class MyComponent implements OnInit, OnDestroy {
private resultForm: FormGroup;
private destroyed$: Subject<boolean> = new Subject();
resultData = [
{
text: 'My Text',
}];
constructor(private formBuilder: FormBuilder) {
this.resultForm = this.formBuilder.group({
childData: this.formBuilder.array()
});
this.setMainOptions();
}
setMainOptions() {
const control = <FormArray>this.resultForm.controls.childData;
this.resultData.forEach(x => {
control.push(this.formBuilder.group({
text: new FormControl()}))
})
}
ngOnInit() {
}
ngOnDestroy() {
this.destroyed$.complete();
}
}
// my html
<form [formGroup]="resultForm">
<div formArrayName="resultOptions">
<div *ngFor="let mainOption of resultForm.get('childData').controls; let i=index">
<div [formGroupName]="i">
<input [formControlName]="text" />
</div>
</div>
</div>
</form>
<pre>{{resultForm.value | json}}</pre>
Greatly appreciated!
angular angular-forms angular-formbuilder
I am trying to build form arrays in Angular. When the data didn't show up, I minimized the code to show behavior. I must use form arrays for the final code. Help me please get through this. I'm using Angular 6.
Why am I getting this error:
Error: Cannot find control with name: 'resultOptions'
// My component .ts file
import {FormControl, FormArray, FormBuilder, FormGroup} from '@angular/forms';
export class MyComponent implements OnInit, OnDestroy {
private resultForm: FormGroup;
private destroyed$: Subject<boolean> = new Subject();
resultData = [
{
text: 'My Text',
}];
constructor(private formBuilder: FormBuilder) {
this.resultForm = this.formBuilder.group({
childData: this.formBuilder.array()
});
this.setMainOptions();
}
setMainOptions() {
const control = <FormArray>this.resultForm.controls.childData;
this.resultData.forEach(x => {
control.push(this.formBuilder.group({
text: new FormControl()}))
})
}
ngOnInit() {
}
ngOnDestroy() {
this.destroyed$.complete();
}
}
// my html
<form [formGroup]="resultForm">
<div formArrayName="resultOptions">
<div *ngFor="let mainOption of resultForm.get('childData').controls; let i=index">
<div [formGroupName]="i">
<input [formControlName]="text" />
</div>
</div>
</div>
</form>
<pre>{{resultForm.value | json}}</pre>
Greatly appreciated!
angular angular-forms angular-formbuilder
angular angular-forms angular-formbuilder
asked Nov 14 '18 at 6:09
user1019042user1019042
90931954
90931954
1
can you post a minimal stackblitz ? That will help greatly
– Akber Iqbal
Nov 14 '18 at 6:12
1
Try usingchildData
instead ofresultOptions
inside HTML.
– Vishw Patel
Nov 14 '18 at 6:13
1
change ` formArrayName="resultOptions"` to ` formArrayName="childData"`. The formArrayName must match what you pass it in the formGroup.
– pixelbits
Nov 14 '18 at 6:14
share yourhtml
view as well to see what is actually referenced there
– Farooq Khan
Nov 14 '18 at 6:18
add a comment |
1
can you post a minimal stackblitz ? That will help greatly
– Akber Iqbal
Nov 14 '18 at 6:12
1
Try usingchildData
instead ofresultOptions
inside HTML.
– Vishw Patel
Nov 14 '18 at 6:13
1
change ` formArrayName="resultOptions"` to ` formArrayName="childData"`. The formArrayName must match what you pass it in the formGroup.
– pixelbits
Nov 14 '18 at 6:14
share yourhtml
view as well to see what is actually referenced there
– Farooq Khan
Nov 14 '18 at 6:18
1
1
can you post a minimal stackblitz ? That will help greatly
– Akber Iqbal
Nov 14 '18 at 6:12
can you post a minimal stackblitz ? That will help greatly
– Akber Iqbal
Nov 14 '18 at 6:12
1
1
Try using
childData
instead of resultOptions
inside HTML.– Vishw Patel
Nov 14 '18 at 6:13
Try using
childData
instead of resultOptions
inside HTML.– Vishw Patel
Nov 14 '18 at 6:13
1
1
change ` formArrayName="resultOptions"` to ` formArrayName="childData"`. The formArrayName must match what you pass it in the formGroup.
– pixelbits
Nov 14 '18 at 6:14
change ` formArrayName="resultOptions"` to ` formArrayName="childData"`. The formArrayName must match what you pass it in the formGroup.
– pixelbits
Nov 14 '18 at 6:14
share your
html
view as well to see what is actually referenced there– Farooq Khan
Nov 14 '18 at 6:18
share your
html
view as well to see what is actually referenced there– Farooq Khan
Nov 14 '18 at 6:18
add a comment |
1 Answer
1
active
oldest
votes
i tried to reproduce your error with stackblitz and found that you have to modify the code to accomplish what you want, with my best understanding. please check the link the code now not throwing any error in console.
The changed code as follows:
/// Component
import { Component, OnInit, OnDestroy } from '@angular/core';
import {FormControl, FormArray, FormBuilder, FormGroup} from '@angular/forms';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent implements OnInit, OnDestroy {
private resultForm: FormGroup;
// private destroyed$: Subject<boolean> = new Subject();
resultData = [
{
text: 'My Text 1',
},
{
text: 'My Text 2',
},
{
text: 'My Text 3',
},
{
text: 'My Text 4',
}];
constructor(private formBuilder: FormBuilder) {
this.resultForm = this.formBuilder.group({
childData: this.formBuilder.array()
});
this.setMainOptions();
}
setMainOptions() {
const control = <FormArray>this.resultForm.controls.childData;
this.resultData.forEach(x => {
control.push( new FormControl(x.text))
})
}
ngOnInit() {
}
ngOnDestroy() {
// this.destroyed$.complete();
}
}
\ HTML
<form [formGroup]="resultForm">
<div formArrayName="childData">
<ng-container>
<div *ngFor="let mainOption of resultForm.get('childData').controls; let i=index">
<input formControlName='{{i}}' />
</div>
</ng-container>
</div>
</form>
{{resultForm.get('childData').controls.length}}
<pre>{{resultForm.value | json}}</pre>
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53294106%2fangular-6-forms-cannot-find-control-error%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
i tried to reproduce your error with stackblitz and found that you have to modify the code to accomplish what you want, with my best understanding. please check the link the code now not throwing any error in console.
The changed code as follows:
/// Component
import { Component, OnInit, OnDestroy } from '@angular/core';
import {FormControl, FormArray, FormBuilder, FormGroup} from '@angular/forms';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent implements OnInit, OnDestroy {
private resultForm: FormGroup;
// private destroyed$: Subject<boolean> = new Subject();
resultData = [
{
text: 'My Text 1',
},
{
text: 'My Text 2',
},
{
text: 'My Text 3',
},
{
text: 'My Text 4',
}];
constructor(private formBuilder: FormBuilder) {
this.resultForm = this.formBuilder.group({
childData: this.formBuilder.array()
});
this.setMainOptions();
}
setMainOptions() {
const control = <FormArray>this.resultForm.controls.childData;
this.resultData.forEach(x => {
control.push( new FormControl(x.text))
})
}
ngOnInit() {
}
ngOnDestroy() {
// this.destroyed$.complete();
}
}
\ HTML
<form [formGroup]="resultForm">
<div formArrayName="childData">
<ng-container>
<div *ngFor="let mainOption of resultForm.get('childData').controls; let i=index">
<input formControlName='{{i}}' />
</div>
</ng-container>
</div>
</form>
{{resultForm.get('childData').controls.length}}
<pre>{{resultForm.value | json}}</pre>
add a comment |
i tried to reproduce your error with stackblitz and found that you have to modify the code to accomplish what you want, with my best understanding. please check the link the code now not throwing any error in console.
The changed code as follows:
/// Component
import { Component, OnInit, OnDestroy } from '@angular/core';
import {FormControl, FormArray, FormBuilder, FormGroup} from '@angular/forms';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent implements OnInit, OnDestroy {
private resultForm: FormGroup;
// private destroyed$: Subject<boolean> = new Subject();
resultData = [
{
text: 'My Text 1',
},
{
text: 'My Text 2',
},
{
text: 'My Text 3',
},
{
text: 'My Text 4',
}];
constructor(private formBuilder: FormBuilder) {
this.resultForm = this.formBuilder.group({
childData: this.formBuilder.array()
});
this.setMainOptions();
}
setMainOptions() {
const control = <FormArray>this.resultForm.controls.childData;
this.resultData.forEach(x => {
control.push( new FormControl(x.text))
})
}
ngOnInit() {
}
ngOnDestroy() {
// this.destroyed$.complete();
}
}
\ HTML
<form [formGroup]="resultForm">
<div formArrayName="childData">
<ng-container>
<div *ngFor="let mainOption of resultForm.get('childData').controls; let i=index">
<input formControlName='{{i}}' />
</div>
</ng-container>
</div>
</form>
{{resultForm.get('childData').controls.length}}
<pre>{{resultForm.value | json}}</pre>
add a comment |
i tried to reproduce your error with stackblitz and found that you have to modify the code to accomplish what you want, with my best understanding. please check the link the code now not throwing any error in console.
The changed code as follows:
/// Component
import { Component, OnInit, OnDestroy } from '@angular/core';
import {FormControl, FormArray, FormBuilder, FormGroup} from '@angular/forms';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent implements OnInit, OnDestroy {
private resultForm: FormGroup;
// private destroyed$: Subject<boolean> = new Subject();
resultData = [
{
text: 'My Text 1',
},
{
text: 'My Text 2',
},
{
text: 'My Text 3',
},
{
text: 'My Text 4',
}];
constructor(private formBuilder: FormBuilder) {
this.resultForm = this.formBuilder.group({
childData: this.formBuilder.array()
});
this.setMainOptions();
}
setMainOptions() {
const control = <FormArray>this.resultForm.controls.childData;
this.resultData.forEach(x => {
control.push( new FormControl(x.text))
})
}
ngOnInit() {
}
ngOnDestroy() {
// this.destroyed$.complete();
}
}
\ HTML
<form [formGroup]="resultForm">
<div formArrayName="childData">
<ng-container>
<div *ngFor="let mainOption of resultForm.get('childData').controls; let i=index">
<input formControlName='{{i}}' />
</div>
</ng-container>
</div>
</form>
{{resultForm.get('childData').controls.length}}
<pre>{{resultForm.value | json}}</pre>
i tried to reproduce your error with stackblitz and found that you have to modify the code to accomplish what you want, with my best understanding. please check the link the code now not throwing any error in console.
The changed code as follows:
/// Component
import { Component, OnInit, OnDestroy } from '@angular/core';
import {FormControl, FormArray, FormBuilder, FormGroup} from '@angular/forms';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent implements OnInit, OnDestroy {
private resultForm: FormGroup;
// private destroyed$: Subject<boolean> = new Subject();
resultData = [
{
text: 'My Text 1',
},
{
text: 'My Text 2',
},
{
text: 'My Text 3',
},
{
text: 'My Text 4',
}];
constructor(private formBuilder: FormBuilder) {
this.resultForm = this.formBuilder.group({
childData: this.formBuilder.array()
});
this.setMainOptions();
}
setMainOptions() {
const control = <FormArray>this.resultForm.controls.childData;
this.resultData.forEach(x => {
control.push( new FormControl(x.text))
})
}
ngOnInit() {
}
ngOnDestroy() {
// this.destroyed$.complete();
}
}
\ HTML
<form [formGroup]="resultForm">
<div formArrayName="childData">
<ng-container>
<div *ngFor="let mainOption of resultForm.get('childData').controls; let i=index">
<input formControlName='{{i}}' />
</div>
</ng-container>
</div>
</form>
{{resultForm.get('childData').controls.length}}
<pre>{{resultForm.value | json}}</pre>
edited Nov 17 '18 at 20:41
answered Nov 14 '18 at 6:58


Kedar9444Kedar9444
38328
38328
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53294106%2fangular-6-forms-cannot-find-control-error%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
d1IgWqhV,17,PXekdb gyfLuU 3vsZ0SS9,cbehX4uamT8 EN2Z9UPuS7R5Jnf0
1
can you post a minimal stackblitz ? That will help greatly
– Akber Iqbal
Nov 14 '18 at 6:12
1
Try using
childData
instead ofresultOptions
inside HTML.– Vishw Patel
Nov 14 '18 at 6:13
1
change ` formArrayName="resultOptions"` to ` formArrayName="childData"`. The formArrayName must match what you pass it in the formGroup.
– pixelbits
Nov 14 '18 at 6:14
share your
html
view as well to see what is actually referenced there– Farooq Khan
Nov 14 '18 at 6:18