Linq 'Unable to process the type 'Anonymous type', because it has no known mapping to the value layer.' in...
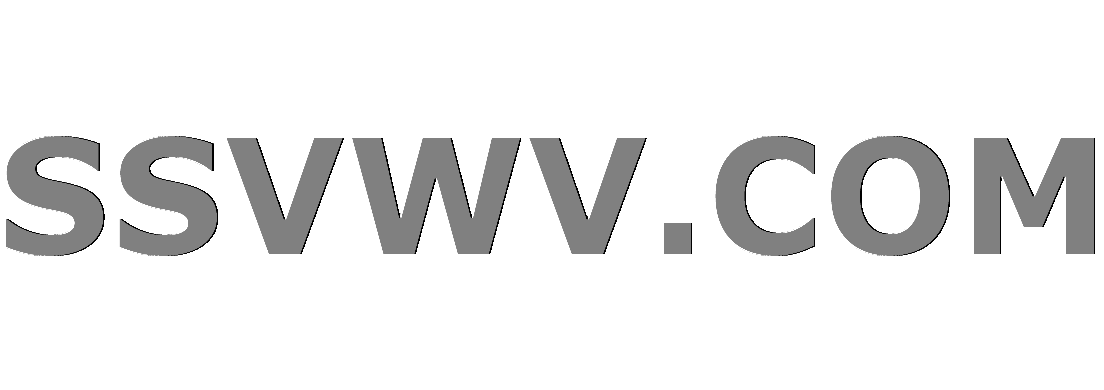
Multi tool use
I am trying to convert a SQL Command to Linq but gets the error 'Unable to process the type 'Anonymous type', because it has no known mapping to the value layer.'
SQL Code
SELECT ooe.id, parts.title, appro.totalAppro, allo.TotalAllotment, cost.total_cost, (CASE WHEN cost.total_cost IS NULL THEN (SELECT allo.TotalAllotment) ELSE (SELECT allo.TotalAllotment - cost.total_cost) END) AS unobligated
FROM pmTA_OoeGeneral AS ooe INNER JOIN
pmTA_Particulars AS parts ON ooe.particular_id = parts.id LEFT OUTER JOIN
pmTA_vwObligationRequestDetailsTotalCostByOoe AS cost ON ooe.id = cost.ooe_general_id
LEFT OUTER JOIN pmTA_vwTotalAllotmentGeneralFund AS allo ON ooe.id = allo.ooe_general_id
LEFT OUTER JOIN pmTA_vwTotalAppropriationGeneralFund AS appro ON ooe.id = appro.ooe_general_id
WHERE (ooe.year = @year) AND (ooe.project_category_id = @cat) and (ooe.type = @type) order by ooe.id
LINQ Code
var gvStatusGenOffices = (from a in db.iBudget_OoeGeneral
join b in db.iBudget_Particulars on a.ParticularID equals b.id
join c in obligationRequestDetailsTotalCostByOoe on a.id equals c.id
join d in totalAllotmentGeneralFund on a.id equals d.id
join e in totalAppropriationGeneralFund on a.id equals e.id
where a.YearID==year && a.ProjectID==cat && a.type==type
orderby a.id
select new
{
id = a.id,
title = b.title,
appro = e.totalAppro,
allo = d.totalAllo,
cost = c.totalCost,
unobligated = c.totalCost==null ? d.totalAllo : d.totalAllo - c.totalCost
}).ToList();
db.iBudget_OoeGeneral and db.iBudget_Particulars are model class in my project
obligationRequestDetailsTotalCostByOoe , totalAllotmentGeneralFund and totalAppropriationGeneralFund are variables with list values.
The question is what is causing this error? I tried looking the web for some answers but none was showing up.
UPDATE
var obligationRequestDetailsTotalCostByOoe = (from p in db.iBudget_ObligationRequestDetails
where p.ooe_general_id != null && p.is_approved == 1
group p by p.ooe_general_id into g
select g)
.AsEnumerable()
.Select(g => new {
id = g.Key,
totalCost = g.Sum(p => Convert.ToDouble(p.amount))
}).ToList();
Originally, obligationRequestDetailsTotalCostByOoe , totalAllotmentGeneralFund and totalAppropriationGeneralFund were Views in SQL Server. Since I was practicing how to code Linq, I didn't create a View and just made a var with list same with the original View Code in SQL.
c# linq
add a comment |
I am trying to convert a SQL Command to Linq but gets the error 'Unable to process the type 'Anonymous type', because it has no known mapping to the value layer.'
SQL Code
SELECT ooe.id, parts.title, appro.totalAppro, allo.TotalAllotment, cost.total_cost, (CASE WHEN cost.total_cost IS NULL THEN (SELECT allo.TotalAllotment) ELSE (SELECT allo.TotalAllotment - cost.total_cost) END) AS unobligated
FROM pmTA_OoeGeneral AS ooe INNER JOIN
pmTA_Particulars AS parts ON ooe.particular_id = parts.id LEFT OUTER JOIN
pmTA_vwObligationRequestDetailsTotalCostByOoe AS cost ON ooe.id = cost.ooe_general_id
LEFT OUTER JOIN pmTA_vwTotalAllotmentGeneralFund AS allo ON ooe.id = allo.ooe_general_id
LEFT OUTER JOIN pmTA_vwTotalAppropriationGeneralFund AS appro ON ooe.id = appro.ooe_general_id
WHERE (ooe.year = @year) AND (ooe.project_category_id = @cat) and (ooe.type = @type) order by ooe.id
LINQ Code
var gvStatusGenOffices = (from a in db.iBudget_OoeGeneral
join b in db.iBudget_Particulars on a.ParticularID equals b.id
join c in obligationRequestDetailsTotalCostByOoe on a.id equals c.id
join d in totalAllotmentGeneralFund on a.id equals d.id
join e in totalAppropriationGeneralFund on a.id equals e.id
where a.YearID==year && a.ProjectID==cat && a.type==type
orderby a.id
select new
{
id = a.id,
title = b.title,
appro = e.totalAppro,
allo = d.totalAllo,
cost = c.totalCost,
unobligated = c.totalCost==null ? d.totalAllo : d.totalAllo - c.totalCost
}).ToList();
db.iBudget_OoeGeneral and db.iBudget_Particulars are model class in my project
obligationRequestDetailsTotalCostByOoe , totalAllotmentGeneralFund and totalAppropriationGeneralFund are variables with list values.
The question is what is causing this error? I tried looking the web for some answers but none was showing up.
UPDATE
var obligationRequestDetailsTotalCostByOoe = (from p in db.iBudget_ObligationRequestDetails
where p.ooe_general_id != null && p.is_approved == 1
group p by p.ooe_general_id into g
select g)
.AsEnumerable()
.Select(g => new {
id = g.Key,
totalCost = g.Sum(p => Convert.ToDouble(p.amount))
}).ToList();
Originally, obligationRequestDetailsTotalCostByOoe , totalAllotmentGeneralFund and totalAppropriationGeneralFund were Views in SQL Server. Since I was practicing how to code Linq, I didn't create a View and just made a var with list same with the original View Code in SQL.
c# linq
Can you show how are you creating e.g.obligationRequestDetailsTotalCostByOoe
?
– arekzyla
Apr 19 '18 at 7:42
Isdb.iBudget_OoeGeneral
andobligationRequestDetailsTotalCostByOne
in the same context ?
– Florian
Apr 19 '18 at 7:44
@arekzyla I have included the code for obligationRequestDetailsTotalCostByOoe . As you can see, this code is only a variable with stored data
– Mace Munch
Apr 20 '18 at 0:47
@Florian db.iBudget_OoeGeneral is taken from the model while the obligationRequestDetailsTotalCostByOoe is a var for the linq code I made
– Mace Munch
Apr 20 '18 at 0:48
add a comment |
I am trying to convert a SQL Command to Linq but gets the error 'Unable to process the type 'Anonymous type', because it has no known mapping to the value layer.'
SQL Code
SELECT ooe.id, parts.title, appro.totalAppro, allo.TotalAllotment, cost.total_cost, (CASE WHEN cost.total_cost IS NULL THEN (SELECT allo.TotalAllotment) ELSE (SELECT allo.TotalAllotment - cost.total_cost) END) AS unobligated
FROM pmTA_OoeGeneral AS ooe INNER JOIN
pmTA_Particulars AS parts ON ooe.particular_id = parts.id LEFT OUTER JOIN
pmTA_vwObligationRequestDetailsTotalCostByOoe AS cost ON ooe.id = cost.ooe_general_id
LEFT OUTER JOIN pmTA_vwTotalAllotmentGeneralFund AS allo ON ooe.id = allo.ooe_general_id
LEFT OUTER JOIN pmTA_vwTotalAppropriationGeneralFund AS appro ON ooe.id = appro.ooe_general_id
WHERE (ooe.year = @year) AND (ooe.project_category_id = @cat) and (ooe.type = @type) order by ooe.id
LINQ Code
var gvStatusGenOffices = (from a in db.iBudget_OoeGeneral
join b in db.iBudget_Particulars on a.ParticularID equals b.id
join c in obligationRequestDetailsTotalCostByOoe on a.id equals c.id
join d in totalAllotmentGeneralFund on a.id equals d.id
join e in totalAppropriationGeneralFund on a.id equals e.id
where a.YearID==year && a.ProjectID==cat && a.type==type
orderby a.id
select new
{
id = a.id,
title = b.title,
appro = e.totalAppro,
allo = d.totalAllo,
cost = c.totalCost,
unobligated = c.totalCost==null ? d.totalAllo : d.totalAllo - c.totalCost
}).ToList();
db.iBudget_OoeGeneral and db.iBudget_Particulars are model class in my project
obligationRequestDetailsTotalCostByOoe , totalAllotmentGeneralFund and totalAppropriationGeneralFund are variables with list values.
The question is what is causing this error? I tried looking the web for some answers but none was showing up.
UPDATE
var obligationRequestDetailsTotalCostByOoe = (from p in db.iBudget_ObligationRequestDetails
where p.ooe_general_id != null && p.is_approved == 1
group p by p.ooe_general_id into g
select g)
.AsEnumerable()
.Select(g => new {
id = g.Key,
totalCost = g.Sum(p => Convert.ToDouble(p.amount))
}).ToList();
Originally, obligationRequestDetailsTotalCostByOoe , totalAllotmentGeneralFund and totalAppropriationGeneralFund were Views in SQL Server. Since I was practicing how to code Linq, I didn't create a View and just made a var with list same with the original View Code in SQL.
c# linq
I am trying to convert a SQL Command to Linq but gets the error 'Unable to process the type 'Anonymous type', because it has no known mapping to the value layer.'
SQL Code
SELECT ooe.id, parts.title, appro.totalAppro, allo.TotalAllotment, cost.total_cost, (CASE WHEN cost.total_cost IS NULL THEN (SELECT allo.TotalAllotment) ELSE (SELECT allo.TotalAllotment - cost.total_cost) END) AS unobligated
FROM pmTA_OoeGeneral AS ooe INNER JOIN
pmTA_Particulars AS parts ON ooe.particular_id = parts.id LEFT OUTER JOIN
pmTA_vwObligationRequestDetailsTotalCostByOoe AS cost ON ooe.id = cost.ooe_general_id
LEFT OUTER JOIN pmTA_vwTotalAllotmentGeneralFund AS allo ON ooe.id = allo.ooe_general_id
LEFT OUTER JOIN pmTA_vwTotalAppropriationGeneralFund AS appro ON ooe.id = appro.ooe_general_id
WHERE (ooe.year = @year) AND (ooe.project_category_id = @cat) and (ooe.type = @type) order by ooe.id
LINQ Code
var gvStatusGenOffices = (from a in db.iBudget_OoeGeneral
join b in db.iBudget_Particulars on a.ParticularID equals b.id
join c in obligationRequestDetailsTotalCostByOoe on a.id equals c.id
join d in totalAllotmentGeneralFund on a.id equals d.id
join e in totalAppropriationGeneralFund on a.id equals e.id
where a.YearID==year && a.ProjectID==cat && a.type==type
orderby a.id
select new
{
id = a.id,
title = b.title,
appro = e.totalAppro,
allo = d.totalAllo,
cost = c.totalCost,
unobligated = c.totalCost==null ? d.totalAllo : d.totalAllo - c.totalCost
}).ToList();
db.iBudget_OoeGeneral and db.iBudget_Particulars are model class in my project
obligationRequestDetailsTotalCostByOoe , totalAllotmentGeneralFund and totalAppropriationGeneralFund are variables with list values.
The question is what is causing this error? I tried looking the web for some answers but none was showing up.
UPDATE
var obligationRequestDetailsTotalCostByOoe = (from p in db.iBudget_ObligationRequestDetails
where p.ooe_general_id != null && p.is_approved == 1
group p by p.ooe_general_id into g
select g)
.AsEnumerable()
.Select(g => new {
id = g.Key,
totalCost = g.Sum(p => Convert.ToDouble(p.amount))
}).ToList();
Originally, obligationRequestDetailsTotalCostByOoe , totalAllotmentGeneralFund and totalAppropriationGeneralFund were Views in SQL Server. Since I was practicing how to code Linq, I didn't create a View and just made a var with list same with the original View Code in SQL.
c# linq
c# linq
edited Apr 20 '18 at 1:42
Mace Munch
asked Apr 19 '18 at 7:16


Mace MunchMace Munch
206
206
Can you show how are you creating e.g.obligationRequestDetailsTotalCostByOoe
?
– arekzyla
Apr 19 '18 at 7:42
Isdb.iBudget_OoeGeneral
andobligationRequestDetailsTotalCostByOne
in the same context ?
– Florian
Apr 19 '18 at 7:44
@arekzyla I have included the code for obligationRequestDetailsTotalCostByOoe . As you can see, this code is only a variable with stored data
– Mace Munch
Apr 20 '18 at 0:47
@Florian db.iBudget_OoeGeneral is taken from the model while the obligationRequestDetailsTotalCostByOoe is a var for the linq code I made
– Mace Munch
Apr 20 '18 at 0:48
add a comment |
Can you show how are you creating e.g.obligationRequestDetailsTotalCostByOoe
?
– arekzyla
Apr 19 '18 at 7:42
Isdb.iBudget_OoeGeneral
andobligationRequestDetailsTotalCostByOne
in the same context ?
– Florian
Apr 19 '18 at 7:44
@arekzyla I have included the code for obligationRequestDetailsTotalCostByOoe . As you can see, this code is only a variable with stored data
– Mace Munch
Apr 20 '18 at 0:47
@Florian db.iBudget_OoeGeneral is taken from the model while the obligationRequestDetailsTotalCostByOoe is a var for the linq code I made
– Mace Munch
Apr 20 '18 at 0:48
Can you show how are you creating e.g.
obligationRequestDetailsTotalCostByOoe
?– arekzyla
Apr 19 '18 at 7:42
Can you show how are you creating e.g.
obligationRequestDetailsTotalCostByOoe
?– arekzyla
Apr 19 '18 at 7:42
Is
db.iBudget_OoeGeneral
and obligationRequestDetailsTotalCostByOne
in the same context ?– Florian
Apr 19 '18 at 7:44
Is
db.iBudget_OoeGeneral
and obligationRequestDetailsTotalCostByOne
in the same context ?– Florian
Apr 19 '18 at 7:44
@arekzyla I have included the code for obligationRequestDetailsTotalCostByOoe . As you can see, this code is only a variable with stored data
– Mace Munch
Apr 20 '18 at 0:47
@arekzyla I have included the code for obligationRequestDetailsTotalCostByOoe . As you can see, this code is only a variable with stored data
– Mace Munch
Apr 20 '18 at 0:47
@Florian db.iBudget_OoeGeneral is taken from the model while the obligationRequestDetailsTotalCostByOoe is a var for the linq code I made
– Mace Munch
Apr 20 '18 at 0:48
@Florian db.iBudget_OoeGeneral is taken from the model while the obligationRequestDetailsTotalCostByOoe is a var for the linq code I made
– Mace Munch
Apr 20 '18 at 0:48
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49914949%2flinq-unable-to-process-the-type-anonymous-type-because-it-has-no-known-mappi%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49914949%2flinq-unable-to-process-the-type-anonymous-type-because-it-has-no-known-mappi%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UPH 9k,Ht,za,AXMJGtPc,1UpKO5,5Z5kZjFJHk,mx8oEM VVkQTaPmfbht3isHmejridwOiKkgHg6qYr
Can you show how are you creating e.g.
obligationRequestDetailsTotalCostByOoe
?– arekzyla
Apr 19 '18 at 7:42
Is
db.iBudget_OoeGeneral
andobligationRequestDetailsTotalCostByOne
in the same context ?– Florian
Apr 19 '18 at 7:44
@arekzyla I have included the code for obligationRequestDetailsTotalCostByOoe . As you can see, this code is only a variable with stored data
– Mace Munch
Apr 20 '18 at 0:47
@Florian db.iBudget_OoeGeneral is taken from the model while the obligationRequestDetailsTotalCostByOoe is a var for the linq code I made
– Mace Munch
Apr 20 '18 at 0:48