How to override or perform min/max in python on your own class?
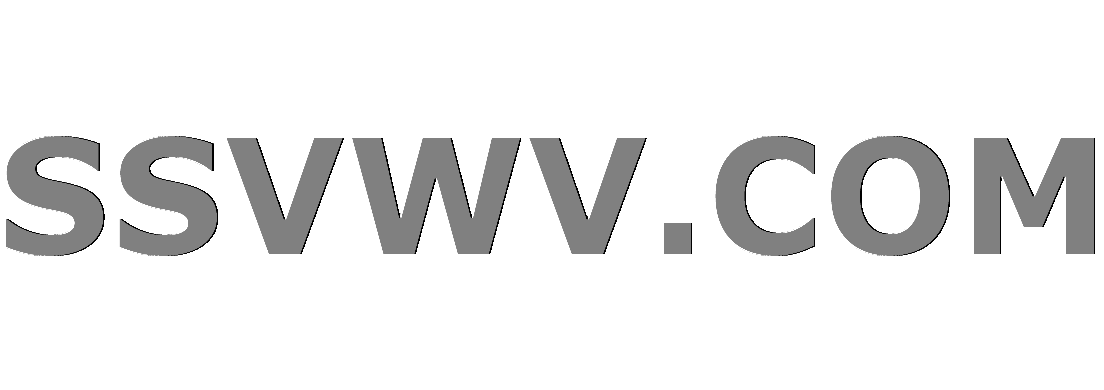
Multi tool use
Not sure the best way to title this question, but how can I override or perform min(a, b)
or max(a, b)
on objects of a class i made? I can override the gt
and lt like below but I would like to override the min or max so that I'll be able to use something like max(a, b, c ,d)
. The class will have multiple property as well, but I think 2 for this example is sufficient.
class MyClass:
def __init__(self, item1, item2):
self.item1 = item1
self.item2 = item2
def __gt__(self, other):
if isinstance(other, MyClass):
if self.item1 > other.item1:
return True
elif self.item1 <= other.item1:
return False
elif self.item2 > other.item2:
return True
elif self.item2 <= other.item2:
return False
def __lt__(self, other):
if isinstance(other, MyClass):
if self.item1 < other.item1:
return True
elif self.item1 >= other.item1:
return False
elif self.item2 < other.item2:
return True
elif self.item2 >= other.item2:
return False
Ex:
a = MyClass(2,3)
b = MyClass(3,3)
print(a > b)
# False
I tried overriding __cmp__
but that doesnt seem to work.
Would like to be able to do max(a, b)
and return b
object
python max min
|
show 3 more comments
Not sure the best way to title this question, but how can I override or perform min(a, b)
or max(a, b)
on objects of a class i made? I can override the gt
and lt like below but I would like to override the min or max so that I'll be able to use something like max(a, b, c ,d)
. The class will have multiple property as well, but I think 2 for this example is sufficient.
class MyClass:
def __init__(self, item1, item2):
self.item1 = item1
self.item2 = item2
def __gt__(self, other):
if isinstance(other, MyClass):
if self.item1 > other.item1:
return True
elif self.item1 <= other.item1:
return False
elif self.item2 > other.item2:
return True
elif self.item2 <= other.item2:
return False
def __lt__(self, other):
if isinstance(other, MyClass):
if self.item1 < other.item1:
return True
elif self.item1 >= other.item1:
return False
elif self.item2 < other.item2:
return True
elif self.item2 >= other.item2:
return False
Ex:
a = MyClass(2,3)
b = MyClass(3,3)
print(a > b)
# False
I tried overriding __cmp__
but that doesnt seem to work.
Would like to be able to do max(a, b)
and return b
object
python max min
You can already callmax(a, b, c, ...)
with an arbitrary number of arguments, and it'll return whichever is largest, by comparing them to each other. It uses__gt__
and__lt__
to do this, to the best of my knowledge. You can't overridemax()
itself, unfortunately, because it's a built-in method and isn't bound to a specific class (though you could easily write your own method to do the same thing if you wanted). Is there a specific thing you wantmax()
to do here that it doesn't do already, and if so could you give a clearer example?
– Green Cloak Guy
Nov 14 '18 at 3:29
(1) You are already returningb
viamax(a, b)
.max(a, b) is b
– Brad Solomon
Nov 14 '18 at 3:31
(2) Considerfunctools.total_ordering
– Brad Solomon
Nov 14 '18 at 3:31
(3)max(a, b, c ,d)
is also already valid. What specifically is wrong with what you've written now?
– Brad Solomon
Nov 14 '18 at 3:32
1
Don't forget thereturn NotImplemented
for when the other object isn't an instance of the expected class.
– user2357112
Nov 14 '18 at 5:32
|
show 3 more comments
Not sure the best way to title this question, but how can I override or perform min(a, b)
or max(a, b)
on objects of a class i made? I can override the gt
and lt like below but I would like to override the min or max so that I'll be able to use something like max(a, b, c ,d)
. The class will have multiple property as well, but I think 2 for this example is sufficient.
class MyClass:
def __init__(self, item1, item2):
self.item1 = item1
self.item2 = item2
def __gt__(self, other):
if isinstance(other, MyClass):
if self.item1 > other.item1:
return True
elif self.item1 <= other.item1:
return False
elif self.item2 > other.item2:
return True
elif self.item2 <= other.item2:
return False
def __lt__(self, other):
if isinstance(other, MyClass):
if self.item1 < other.item1:
return True
elif self.item1 >= other.item1:
return False
elif self.item2 < other.item2:
return True
elif self.item2 >= other.item2:
return False
Ex:
a = MyClass(2,3)
b = MyClass(3,3)
print(a > b)
# False
I tried overriding __cmp__
but that doesnt seem to work.
Would like to be able to do max(a, b)
and return b
object
python max min
Not sure the best way to title this question, but how can I override or perform min(a, b)
or max(a, b)
on objects of a class i made? I can override the gt
and lt like below but I would like to override the min or max so that I'll be able to use something like max(a, b, c ,d)
. The class will have multiple property as well, but I think 2 for this example is sufficient.
class MyClass:
def __init__(self, item1, item2):
self.item1 = item1
self.item2 = item2
def __gt__(self, other):
if isinstance(other, MyClass):
if self.item1 > other.item1:
return True
elif self.item1 <= other.item1:
return False
elif self.item2 > other.item2:
return True
elif self.item2 <= other.item2:
return False
def __lt__(self, other):
if isinstance(other, MyClass):
if self.item1 < other.item1:
return True
elif self.item1 >= other.item1:
return False
elif self.item2 < other.item2:
return True
elif self.item2 >= other.item2:
return False
Ex:
a = MyClass(2,3)
b = MyClass(3,3)
print(a > b)
# False
I tried overriding __cmp__
but that doesnt seem to work.
Would like to be able to do max(a, b)
and return b
object
python max min
python max min
edited Nov 14 '18 at 3:29


Aqueous Carlos
301313
301313
asked Nov 14 '18 at 3:24
user1179317user1179317
607818
607818
You can already callmax(a, b, c, ...)
with an arbitrary number of arguments, and it'll return whichever is largest, by comparing them to each other. It uses__gt__
and__lt__
to do this, to the best of my knowledge. You can't overridemax()
itself, unfortunately, because it's a built-in method and isn't bound to a specific class (though you could easily write your own method to do the same thing if you wanted). Is there a specific thing you wantmax()
to do here that it doesn't do already, and if so could you give a clearer example?
– Green Cloak Guy
Nov 14 '18 at 3:29
(1) You are already returningb
viamax(a, b)
.max(a, b) is b
– Brad Solomon
Nov 14 '18 at 3:31
(2) Considerfunctools.total_ordering
– Brad Solomon
Nov 14 '18 at 3:31
(3)max(a, b, c ,d)
is also already valid. What specifically is wrong with what you've written now?
– Brad Solomon
Nov 14 '18 at 3:32
1
Don't forget thereturn NotImplemented
for when the other object isn't an instance of the expected class.
– user2357112
Nov 14 '18 at 5:32
|
show 3 more comments
You can already callmax(a, b, c, ...)
with an arbitrary number of arguments, and it'll return whichever is largest, by comparing them to each other. It uses__gt__
and__lt__
to do this, to the best of my knowledge. You can't overridemax()
itself, unfortunately, because it's a built-in method and isn't bound to a specific class (though you could easily write your own method to do the same thing if you wanted). Is there a specific thing you wantmax()
to do here that it doesn't do already, and if so could you give a clearer example?
– Green Cloak Guy
Nov 14 '18 at 3:29
(1) You are already returningb
viamax(a, b)
.max(a, b) is b
– Brad Solomon
Nov 14 '18 at 3:31
(2) Considerfunctools.total_ordering
– Brad Solomon
Nov 14 '18 at 3:31
(3)max(a, b, c ,d)
is also already valid. What specifically is wrong with what you've written now?
– Brad Solomon
Nov 14 '18 at 3:32
1
Don't forget thereturn NotImplemented
for when the other object isn't an instance of the expected class.
– user2357112
Nov 14 '18 at 5:32
You can already call
max(a, b, c, ...)
with an arbitrary number of arguments, and it'll return whichever is largest, by comparing them to each other. It uses __gt__
and __lt__
to do this, to the best of my knowledge. You can't override max()
itself, unfortunately, because it's a built-in method and isn't bound to a specific class (though you could easily write your own method to do the same thing if you wanted). Is there a specific thing you want max()
to do here that it doesn't do already, and if so could you give a clearer example?– Green Cloak Guy
Nov 14 '18 at 3:29
You can already call
max(a, b, c, ...)
with an arbitrary number of arguments, and it'll return whichever is largest, by comparing them to each other. It uses __gt__
and __lt__
to do this, to the best of my knowledge. You can't override max()
itself, unfortunately, because it's a built-in method and isn't bound to a specific class (though you could easily write your own method to do the same thing if you wanted). Is there a specific thing you want max()
to do here that it doesn't do already, and if so could you give a clearer example?– Green Cloak Guy
Nov 14 '18 at 3:29
(1) You are already returning
b
via max(a, b)
. max(a, b) is b
– Brad Solomon
Nov 14 '18 at 3:31
(1) You are already returning
b
via max(a, b)
. max(a, b) is b
– Brad Solomon
Nov 14 '18 at 3:31
(2) Consider
functools.total_ordering
– Brad Solomon
Nov 14 '18 at 3:31
(2) Consider
functools.total_ordering
– Brad Solomon
Nov 14 '18 at 3:31
(3)
max(a, b, c ,d)
is also already valid. What specifically is wrong with what you've written now?– Brad Solomon
Nov 14 '18 at 3:32
(3)
max(a, b, c ,d)
is also already valid. What specifically is wrong with what you've written now?– Brad Solomon
Nov 14 '18 at 3:32
1
1
Don't forget the
return NotImplemented
for when the other object isn't an instance of the expected class.– user2357112
Nov 14 '18 at 5:32
Don't forget the
return NotImplemented
for when the other object isn't an instance of the expected class.– user2357112
Nov 14 '18 at 5:32
|
show 3 more comments
1 Answer
1
active
oldest
votes
Just override the comparison magic methods
class A(object):
def __init__(self, value):
self.value = value
def __lt__(self, other):
return self.value < other.value
def __lt__(self, other):
return self.value <= other.value
def __eq__(self, other):
return self.value == other.value
def __ne__(self, other):
return self.value != other.value
def __gt__(self, other):
return self.value > other.value
def __ge__(self, other):
return self.value < other.value
def __str__(self):
return str(self.value)
a = A(10)
b = A(20)
min(a, b)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53292737%2fhow-to-override-or-perform-min-max-in-python-on-your-own-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Just override the comparison magic methods
class A(object):
def __init__(self, value):
self.value = value
def __lt__(self, other):
return self.value < other.value
def __lt__(self, other):
return self.value <= other.value
def __eq__(self, other):
return self.value == other.value
def __ne__(self, other):
return self.value != other.value
def __gt__(self, other):
return self.value > other.value
def __ge__(self, other):
return self.value < other.value
def __str__(self):
return str(self.value)
a = A(10)
b = A(20)
min(a, b)
add a comment |
Just override the comparison magic methods
class A(object):
def __init__(self, value):
self.value = value
def __lt__(self, other):
return self.value < other.value
def __lt__(self, other):
return self.value <= other.value
def __eq__(self, other):
return self.value == other.value
def __ne__(self, other):
return self.value != other.value
def __gt__(self, other):
return self.value > other.value
def __ge__(self, other):
return self.value < other.value
def __str__(self):
return str(self.value)
a = A(10)
b = A(20)
min(a, b)
add a comment |
Just override the comparison magic methods
class A(object):
def __init__(self, value):
self.value = value
def __lt__(self, other):
return self.value < other.value
def __lt__(self, other):
return self.value <= other.value
def __eq__(self, other):
return self.value == other.value
def __ne__(self, other):
return self.value != other.value
def __gt__(self, other):
return self.value > other.value
def __ge__(self, other):
return self.value < other.value
def __str__(self):
return str(self.value)
a = A(10)
b = A(20)
min(a, b)
Just override the comparison magic methods
class A(object):
def __init__(self, value):
self.value = value
def __lt__(self, other):
return self.value < other.value
def __lt__(self, other):
return self.value <= other.value
def __eq__(self, other):
return self.value == other.value
def __ne__(self, other):
return self.value != other.value
def __gt__(self, other):
return self.value > other.value
def __ge__(self, other):
return self.value < other.value
def __str__(self):
return str(self.value)
a = A(10)
b = A(20)
min(a, b)
edited Nov 14 '18 at 5:31
answered Nov 14 '18 at 5:25
Muruganandhan PeramagounderMuruganandhan Peramagounder
11
11
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53292737%2fhow-to-override-or-perform-min-max-in-python-on-your-own-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
32U3Zmfpyd2m7,wkK Oson8IkcvPO 2MGu6 6Y0DIufMNhx48ag,X 0RTJY4ko1J2,V8ZCINjReA7tNQQ,Wut XrmZ8180xf
You can already call
max(a, b, c, ...)
with an arbitrary number of arguments, and it'll return whichever is largest, by comparing them to each other. It uses__gt__
and__lt__
to do this, to the best of my knowledge. You can't overridemax()
itself, unfortunately, because it's a built-in method and isn't bound to a specific class (though you could easily write your own method to do the same thing if you wanted). Is there a specific thing you wantmax()
to do here that it doesn't do already, and if so could you give a clearer example?– Green Cloak Guy
Nov 14 '18 at 3:29
(1) You are already returning
b
viamax(a, b)
.max(a, b) is b
– Brad Solomon
Nov 14 '18 at 3:31
(2) Consider
functools.total_ordering
– Brad Solomon
Nov 14 '18 at 3:31
(3)
max(a, b, c ,d)
is also already valid. What specifically is wrong with what you've written now?– Brad Solomon
Nov 14 '18 at 3:32
1
Don't forget the
return NotImplemented
for when the other object isn't an instance of the expected class.– user2357112
Nov 14 '18 at 5:32