How to make subplots in donut pie chart in matplotlib pyhon
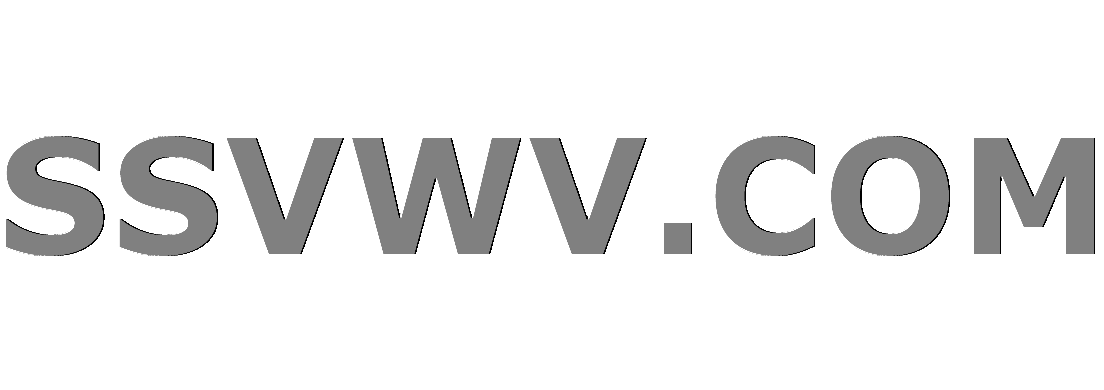
Multi tool use
How to make subplots in donut pie chart in matplotlib pyhon?
Below is the code I wrote,
import matplotlib.pyplot as plt
labels = 'Frogs', 'Hogs', 'Dogs'
sizes = [15, 30, 45]
colors = ['yellowgreen', 'gold', 'lightskyblue']
explode = (0, 0, 0) # explode a slice if required
subgroup_names = ['A.1', 'A.2', 'A.3', 'B.1', 'B.2', 'C.1']
subgroup_size = [13, 3, 5, 6, 5, 10]
a, b, c = [plt.cm.Blues, plt.cm.Reds, plt.cm.Greens]
plt.pie(sizes, explode=explode, labels=labels, pctdistance=0.45, labeldistance=0.65, colors=colors, autopct='%.2f%%', shadow=True)
centre_circle = plt.Circle((0, 0), 0.175, color='black', fc='white', linewidth=1.25)
plt.pie(subgroup_size, labels=subgroup_names, pctdistance=0.85, labeldistance=1.25, colors=[a(0.5), a(0.4), a(0.3), b(0.5), b(0.4), c(0.6)], autopct='%.2f%%', shadow=True)
centre_circle2 = plt.Circle((0, 0), 0.275, color='red', fc='white', linewidth=1.25)
fig = plt.gcf()
fig.gca().add_artist(centre_circle)
fig.gca().add_artist(centre_circle2)
plt.axis('equal')
plt.show()
The result shows like below picture,
But I hope the result like below image (have to display the percentage value). This is the result I hope come out(with percentage value):
python matplotlib pie-chart
add a comment |
How to make subplots in donut pie chart in matplotlib pyhon?
Below is the code I wrote,
import matplotlib.pyplot as plt
labels = 'Frogs', 'Hogs', 'Dogs'
sizes = [15, 30, 45]
colors = ['yellowgreen', 'gold', 'lightskyblue']
explode = (0, 0, 0) # explode a slice if required
subgroup_names = ['A.1', 'A.2', 'A.3', 'B.1', 'B.2', 'C.1']
subgroup_size = [13, 3, 5, 6, 5, 10]
a, b, c = [plt.cm.Blues, plt.cm.Reds, plt.cm.Greens]
plt.pie(sizes, explode=explode, labels=labels, pctdistance=0.45, labeldistance=0.65, colors=colors, autopct='%.2f%%', shadow=True)
centre_circle = plt.Circle((0, 0), 0.175, color='black', fc='white', linewidth=1.25)
plt.pie(subgroup_size, labels=subgroup_names, pctdistance=0.85, labeldistance=1.25, colors=[a(0.5), a(0.4), a(0.3), b(0.5), b(0.4), c(0.6)], autopct='%.2f%%', shadow=True)
centre_circle2 = plt.Circle((0, 0), 0.275, color='red', fc='white', linewidth=1.25)
fig = plt.gcf()
fig.gca().add_artist(centre_circle)
fig.gca().add_artist(centre_circle2)
plt.axis('equal')
plt.show()
The result shows like below picture,
But I hope the result like below image (have to display the percentage value). This is the result I hope come out(with percentage value):
python matplotlib pie-chart
There is an example for nested charts in the documentation. Can you apply it here and then ask for the remaining problem?
– ImportanceOfBeingErnest
Nov 14 '18 at 10:10
add a comment |
How to make subplots in donut pie chart in matplotlib pyhon?
Below is the code I wrote,
import matplotlib.pyplot as plt
labels = 'Frogs', 'Hogs', 'Dogs'
sizes = [15, 30, 45]
colors = ['yellowgreen', 'gold', 'lightskyblue']
explode = (0, 0, 0) # explode a slice if required
subgroup_names = ['A.1', 'A.2', 'A.3', 'B.1', 'B.2', 'C.1']
subgroup_size = [13, 3, 5, 6, 5, 10]
a, b, c = [plt.cm.Blues, plt.cm.Reds, plt.cm.Greens]
plt.pie(sizes, explode=explode, labels=labels, pctdistance=0.45, labeldistance=0.65, colors=colors, autopct='%.2f%%', shadow=True)
centre_circle = plt.Circle((0, 0), 0.175, color='black', fc='white', linewidth=1.25)
plt.pie(subgroup_size, labels=subgroup_names, pctdistance=0.85, labeldistance=1.25, colors=[a(0.5), a(0.4), a(0.3), b(0.5), b(0.4), c(0.6)], autopct='%.2f%%', shadow=True)
centre_circle2 = plt.Circle((0, 0), 0.275, color='red', fc='white', linewidth=1.25)
fig = plt.gcf()
fig.gca().add_artist(centre_circle)
fig.gca().add_artist(centre_circle2)
plt.axis('equal')
plt.show()
The result shows like below picture,
But I hope the result like below image (have to display the percentage value). This is the result I hope come out(with percentage value):
python matplotlib pie-chart
How to make subplots in donut pie chart in matplotlib pyhon?
Below is the code I wrote,
import matplotlib.pyplot as plt
labels = 'Frogs', 'Hogs', 'Dogs'
sizes = [15, 30, 45]
colors = ['yellowgreen', 'gold', 'lightskyblue']
explode = (0, 0, 0) # explode a slice if required
subgroup_names = ['A.1', 'A.2', 'A.3', 'B.1', 'B.2', 'C.1']
subgroup_size = [13, 3, 5, 6, 5, 10]
a, b, c = [plt.cm.Blues, plt.cm.Reds, plt.cm.Greens]
plt.pie(sizes, explode=explode, labels=labels, pctdistance=0.45, labeldistance=0.65, colors=colors, autopct='%.2f%%', shadow=True)
centre_circle = plt.Circle((0, 0), 0.175, color='black', fc='white', linewidth=1.25)
plt.pie(subgroup_size, labels=subgroup_names, pctdistance=0.85, labeldistance=1.25, colors=[a(0.5), a(0.4), a(0.3), b(0.5), b(0.4), c(0.6)], autopct='%.2f%%', shadow=True)
centre_circle2 = plt.Circle((0, 0), 0.275, color='red', fc='white', linewidth=1.25)
fig = plt.gcf()
fig.gca().add_artist(centre_circle)
fig.gca().add_artist(centre_circle2)
plt.axis('equal')
plt.show()
The result shows like below picture,
But I hope the result like below image (have to display the percentage value). This is the result I hope come out(with percentage value):
python matplotlib pie-chart
python matplotlib pie-chart
edited Nov 14 '18 at 10:02


ImportanceOfBeingErnest
129k13138215
129k13138215
asked Nov 14 '18 at 4:53
newLearnernewLearner
133
133
There is an example for nested charts in the documentation. Can you apply it here and then ask for the remaining problem?
– ImportanceOfBeingErnest
Nov 14 '18 at 10:10
add a comment |
There is an example for nested charts in the documentation. Can you apply it here and then ask for the remaining problem?
– ImportanceOfBeingErnest
Nov 14 '18 at 10:10
There is an example for nested charts in the documentation. Can you apply it here and then ask for the remaining problem?
– ImportanceOfBeingErnest
Nov 14 '18 at 10:10
There is an example for nested charts in the documentation. Can you apply it here and then ask for the remaining problem?
– ImportanceOfBeingErnest
Nov 14 '18 at 10:10
add a comment |
1 Answer
1
active
oldest
votes
Matplotlib documentation has very nice examples for almost everything you can dream of. Please see here for a nested pie chart.
Following the comment on link-only answers, the keyword radius is what you are looking for in your call to pie(). It takes in a float which determines the absolute radius of your pie. Drawing nested pies thereby requires multiple calls to pie(), each with a different value of radius. Please see an example below
import matplotlib.pyplot as plt
plt.pie([1, 2, 3], radius=1,
colors=['xkcd:cerulean', 'xkcd:bright red', 'xkcd:grass green'],
labels=['Group A', 'Group B', 'Group C'], autopct='%.2f%%',
pctdistance=0.85, shadow=True,
wedgeprops=dict(width=0.3, edgecolor='white'))
plt.pie([0.3, 0.7, 0.4, 1.5, 0.1, 3], radius=0.7,
colors=['xkcd:sky blue', 'xkcd:light blue', 'xkcd:reddish pink',
'xkcd:salmon pink', 'xkcd:baby pink', 'xkcd:apple green'],
wedgeprops=dict(width=0.3, edgecolor='white'),
labels=['A.1', 'A.2', 'B.1', 'B.2', 'B.3', 'C.1'], autopct='%.2f%%',
pctdistance=0.8, labeldistance=0.4, shadow=True)
plt.axis('equal')
plt.show()
which returns the following plot
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53293382%2fhow-to-make-subplots-in-donut-pie-chart-in-matplotlib-pyhon%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Matplotlib documentation has very nice examples for almost everything you can dream of. Please see here for a nested pie chart.
Following the comment on link-only answers, the keyword radius is what you are looking for in your call to pie(). It takes in a float which determines the absolute radius of your pie. Drawing nested pies thereby requires multiple calls to pie(), each with a different value of radius. Please see an example below
import matplotlib.pyplot as plt
plt.pie([1, 2, 3], radius=1,
colors=['xkcd:cerulean', 'xkcd:bright red', 'xkcd:grass green'],
labels=['Group A', 'Group B', 'Group C'], autopct='%.2f%%',
pctdistance=0.85, shadow=True,
wedgeprops=dict(width=0.3, edgecolor='white'))
plt.pie([0.3, 0.7, 0.4, 1.5, 0.1, 3], radius=0.7,
colors=['xkcd:sky blue', 'xkcd:light blue', 'xkcd:reddish pink',
'xkcd:salmon pink', 'xkcd:baby pink', 'xkcd:apple green'],
wedgeprops=dict(width=0.3, edgecolor='white'),
labels=['A.1', 'A.2', 'B.1', 'B.2', 'B.3', 'C.1'], autopct='%.2f%%',
pctdistance=0.8, labeldistance=0.4, shadow=True)
plt.axis('equal')
plt.show()
which returns the following plot
add a comment |
Matplotlib documentation has very nice examples for almost everything you can dream of. Please see here for a nested pie chart.
Following the comment on link-only answers, the keyword radius is what you are looking for in your call to pie(). It takes in a float which determines the absolute radius of your pie. Drawing nested pies thereby requires multiple calls to pie(), each with a different value of radius. Please see an example below
import matplotlib.pyplot as plt
plt.pie([1, 2, 3], radius=1,
colors=['xkcd:cerulean', 'xkcd:bright red', 'xkcd:grass green'],
labels=['Group A', 'Group B', 'Group C'], autopct='%.2f%%',
pctdistance=0.85, shadow=True,
wedgeprops=dict(width=0.3, edgecolor='white'))
plt.pie([0.3, 0.7, 0.4, 1.5, 0.1, 3], radius=0.7,
colors=['xkcd:sky blue', 'xkcd:light blue', 'xkcd:reddish pink',
'xkcd:salmon pink', 'xkcd:baby pink', 'xkcd:apple green'],
wedgeprops=dict(width=0.3, edgecolor='white'),
labels=['A.1', 'A.2', 'B.1', 'B.2', 'B.3', 'C.1'], autopct='%.2f%%',
pctdistance=0.8, labeldistance=0.4, shadow=True)
plt.axis('equal')
plt.show()
which returns the following plot
add a comment |
Matplotlib documentation has very nice examples for almost everything you can dream of. Please see here for a nested pie chart.
Following the comment on link-only answers, the keyword radius is what you are looking for in your call to pie(). It takes in a float which determines the absolute radius of your pie. Drawing nested pies thereby requires multiple calls to pie(), each with a different value of radius. Please see an example below
import matplotlib.pyplot as plt
plt.pie([1, 2, 3], radius=1,
colors=['xkcd:cerulean', 'xkcd:bright red', 'xkcd:grass green'],
labels=['Group A', 'Group B', 'Group C'], autopct='%.2f%%',
pctdistance=0.85, shadow=True,
wedgeprops=dict(width=0.3, edgecolor='white'))
plt.pie([0.3, 0.7, 0.4, 1.5, 0.1, 3], radius=0.7,
colors=['xkcd:sky blue', 'xkcd:light blue', 'xkcd:reddish pink',
'xkcd:salmon pink', 'xkcd:baby pink', 'xkcd:apple green'],
wedgeprops=dict(width=0.3, edgecolor='white'),
labels=['A.1', 'A.2', 'B.1', 'B.2', 'B.3', 'C.1'], autopct='%.2f%%',
pctdistance=0.8, labeldistance=0.4, shadow=True)
plt.axis('equal')
plt.show()
which returns the following plot
Matplotlib documentation has very nice examples for almost everything you can dream of. Please see here for a nested pie chart.
Following the comment on link-only answers, the keyword radius is what you are looking for in your call to pie(). It takes in a float which determines the absolute radius of your pie. Drawing nested pies thereby requires multiple calls to pie(), each with a different value of radius. Please see an example below
import matplotlib.pyplot as plt
plt.pie([1, 2, 3], radius=1,
colors=['xkcd:cerulean', 'xkcd:bright red', 'xkcd:grass green'],
labels=['Group A', 'Group B', 'Group C'], autopct='%.2f%%',
pctdistance=0.85, shadow=True,
wedgeprops=dict(width=0.3, edgecolor='white'))
plt.pie([0.3, 0.7, 0.4, 1.5, 0.1, 3], radius=0.7,
colors=['xkcd:sky blue', 'xkcd:light blue', 'xkcd:reddish pink',
'xkcd:salmon pink', 'xkcd:baby pink', 'xkcd:apple green'],
wedgeprops=dict(width=0.3, edgecolor='white'),
labels=['A.1', 'A.2', 'B.1', 'B.2', 'B.3', 'C.1'], autopct='%.2f%%',
pctdistance=0.8, labeldistance=0.4, shadow=True)
plt.axis('equal')
plt.show()
which returns the following plot
edited Nov 15 '18 at 10:11
answered Nov 14 '18 at 5:03
Patol75Patol75
6236
6236
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53293382%2fhow-to-make-subplots-in-donut-pie-chart-in-matplotlib-pyhon%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
aHaKuWUu9JD 9Yr0dsZ18HxpyBHad2x,Y4wKGIO C,LMZwKJ0lY
There is an example for nested charts in the documentation. Can you apply it here and then ask for the remaining problem?
– ImportanceOfBeingErnest
Nov 14 '18 at 10:10