when this.props is passed to the child component, it becomes undefined
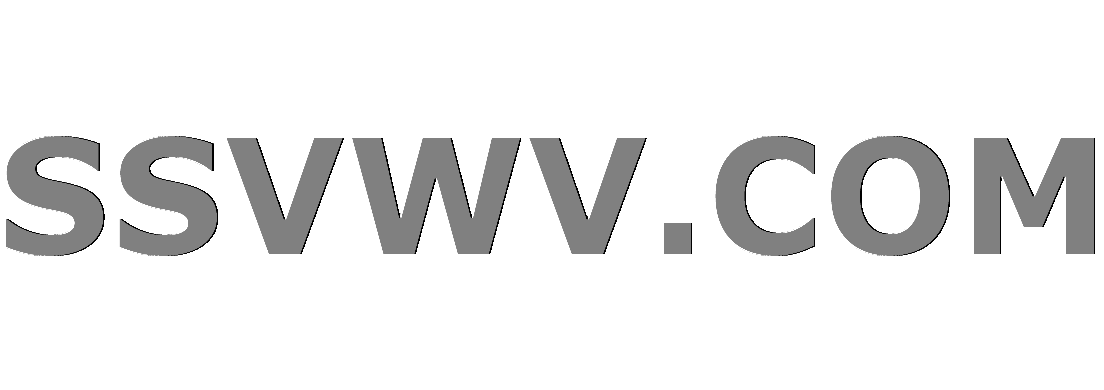
Multi tool use
My React project component structure is very simple: App >> SearchResults >> TrackList.
To test, I output the value of the data in each component.
In the App.js file, I used this.state to define an array of objects called searchResults.
class App extends React.Component {
constructor(props) {
super(props);
this.state = {
searchResults: [
{
name: 'name-1',
artist: 'artist-1',
alblum: 'alblum-1',
id: 101
},
{
name: 'name-2',
artist: 'artist-2',
alblum: 'alblum-2',
id: 202
}
]
}
}
render() {
console.log('Here is App.js', this.state.searchResults);
return (
<div>
<h1>Ja<span className="highlight">mmm</span>ing</h1>
<div className="App">
<SearchBar />
<div className="App-playlist">
<SearchResults searchResults={this.state.searchResults} />
<Playlist />
</div>
</div>
</div>
);
}
}
export default App;
In the SearchResults.js file, I used tracks={this.props.searchResults} to pass the data to the child component.
class SearchResults extends React.Component {
render() {
console.log('Here is SearchResults.js', this.props searchResults);
return (
<div className="SearchResults">
<h2>Results</h2>
<TrackList tracks={this.props.searchResults} />
</div>
);
}
}
export default SearchResults;
In the TrackList.js file, I output the data from parent component.
class TrackList extends React.Component {
render() {
console.log('Here is TrackList.js', this.props.tracks);
return (
<div className="TrackList">
{this.props.tracks.map(track => <Track key={track.id} track={track} />)}
</div>
);
}
}
export default TrackList
But, strange things happen, the console prints the data twice, showing the normal values the first time, and undefined the second time.
Because of this, I cannot use the.map() function for this data.
Why did this happen, and how to fix it?
console screenshot is here: Console
javascript reactjs
add a comment |
My React project component structure is very simple: App >> SearchResults >> TrackList.
To test, I output the value of the data in each component.
In the App.js file, I used this.state to define an array of objects called searchResults.
class App extends React.Component {
constructor(props) {
super(props);
this.state = {
searchResults: [
{
name: 'name-1',
artist: 'artist-1',
alblum: 'alblum-1',
id: 101
},
{
name: 'name-2',
artist: 'artist-2',
alblum: 'alblum-2',
id: 202
}
]
}
}
render() {
console.log('Here is App.js', this.state.searchResults);
return (
<div>
<h1>Ja<span className="highlight">mmm</span>ing</h1>
<div className="App">
<SearchBar />
<div className="App-playlist">
<SearchResults searchResults={this.state.searchResults} />
<Playlist />
</div>
</div>
</div>
);
}
}
export default App;
In the SearchResults.js file, I used tracks={this.props.searchResults} to pass the data to the child component.
class SearchResults extends React.Component {
render() {
console.log('Here is SearchResults.js', this.props searchResults);
return (
<div className="SearchResults">
<h2>Results</h2>
<TrackList tracks={this.props.searchResults} />
</div>
);
}
}
export default SearchResults;
In the TrackList.js file, I output the data from parent component.
class TrackList extends React.Component {
render() {
console.log('Here is TrackList.js', this.props.tracks);
return (
<div className="TrackList">
{this.props.tracks.map(track => <Track key={track.id} track={track} />)}
</div>
);
}
}
export default TrackList
But, strange things happen, the console prints the data twice, showing the normal values the first time, and undefined the second time.
Because of this, I cannot use the.map() function for this data.
Why did this happen, and how to fix it?
console screenshot is here: Console
javascript reactjs
By any chance, is the trackList component used somewhere else too??
– Shubham Khatri
Nov 14 '18 at 5:18
It's pretty tough to tell from your code what's going on. Can you post a codesandbox (or similar) link? From what I can tell, you shouldn't be having issues.
– Riley Steele Parsons
Nov 14 '18 at 5:20
@ShubhamKhatri My god!!! You're a genius. You know how long I've been testing this question, four hours!!! I did use <TrackList /> in another component and totally forgot about it
– Yanze
Nov 14 '18 at 5:30
@Yanze, no issues glad to have helped
– Shubham Khatri
Nov 14 '18 at 5:31
add a comment |
My React project component structure is very simple: App >> SearchResults >> TrackList.
To test, I output the value of the data in each component.
In the App.js file, I used this.state to define an array of objects called searchResults.
class App extends React.Component {
constructor(props) {
super(props);
this.state = {
searchResults: [
{
name: 'name-1',
artist: 'artist-1',
alblum: 'alblum-1',
id: 101
},
{
name: 'name-2',
artist: 'artist-2',
alblum: 'alblum-2',
id: 202
}
]
}
}
render() {
console.log('Here is App.js', this.state.searchResults);
return (
<div>
<h1>Ja<span className="highlight">mmm</span>ing</h1>
<div className="App">
<SearchBar />
<div className="App-playlist">
<SearchResults searchResults={this.state.searchResults} />
<Playlist />
</div>
</div>
</div>
);
}
}
export default App;
In the SearchResults.js file, I used tracks={this.props.searchResults} to pass the data to the child component.
class SearchResults extends React.Component {
render() {
console.log('Here is SearchResults.js', this.props searchResults);
return (
<div className="SearchResults">
<h2>Results</h2>
<TrackList tracks={this.props.searchResults} />
</div>
);
}
}
export default SearchResults;
In the TrackList.js file, I output the data from parent component.
class TrackList extends React.Component {
render() {
console.log('Here is TrackList.js', this.props.tracks);
return (
<div className="TrackList">
{this.props.tracks.map(track => <Track key={track.id} track={track} />)}
</div>
);
}
}
export default TrackList
But, strange things happen, the console prints the data twice, showing the normal values the first time, and undefined the second time.
Because of this, I cannot use the.map() function for this data.
Why did this happen, and how to fix it?
console screenshot is here: Console
javascript reactjs
My React project component structure is very simple: App >> SearchResults >> TrackList.
To test, I output the value of the data in each component.
In the App.js file, I used this.state to define an array of objects called searchResults.
class App extends React.Component {
constructor(props) {
super(props);
this.state = {
searchResults: [
{
name: 'name-1',
artist: 'artist-1',
alblum: 'alblum-1',
id: 101
},
{
name: 'name-2',
artist: 'artist-2',
alblum: 'alblum-2',
id: 202
}
]
}
}
render() {
console.log('Here is App.js', this.state.searchResults);
return (
<div>
<h1>Ja<span className="highlight">mmm</span>ing</h1>
<div className="App">
<SearchBar />
<div className="App-playlist">
<SearchResults searchResults={this.state.searchResults} />
<Playlist />
</div>
</div>
</div>
);
}
}
export default App;
In the SearchResults.js file, I used tracks={this.props.searchResults} to pass the data to the child component.
class SearchResults extends React.Component {
render() {
console.log('Here is SearchResults.js', this.props searchResults);
return (
<div className="SearchResults">
<h2>Results</h2>
<TrackList tracks={this.props.searchResults} />
</div>
);
}
}
export default SearchResults;
In the TrackList.js file, I output the data from parent component.
class TrackList extends React.Component {
render() {
console.log('Here is TrackList.js', this.props.tracks);
return (
<div className="TrackList">
{this.props.tracks.map(track => <Track key={track.id} track={track} />)}
</div>
);
}
}
export default TrackList
But, strange things happen, the console prints the data twice, showing the normal values the first time, and undefined the second time.
Because of this, I cannot use the.map() function for this data.
Why did this happen, and how to fix it?
console screenshot is here: Console
javascript reactjs
javascript reactjs
asked Nov 14 '18 at 5:12
YanzeYanze
1
1
By any chance, is the trackList component used somewhere else too??
– Shubham Khatri
Nov 14 '18 at 5:18
It's pretty tough to tell from your code what's going on. Can you post a codesandbox (or similar) link? From what I can tell, you shouldn't be having issues.
– Riley Steele Parsons
Nov 14 '18 at 5:20
@ShubhamKhatri My god!!! You're a genius. You know how long I've been testing this question, four hours!!! I did use <TrackList /> in another component and totally forgot about it
– Yanze
Nov 14 '18 at 5:30
@Yanze, no issues glad to have helped
– Shubham Khatri
Nov 14 '18 at 5:31
add a comment |
By any chance, is the trackList component used somewhere else too??
– Shubham Khatri
Nov 14 '18 at 5:18
It's pretty tough to tell from your code what's going on. Can you post a codesandbox (or similar) link? From what I can tell, you shouldn't be having issues.
– Riley Steele Parsons
Nov 14 '18 at 5:20
@ShubhamKhatri My god!!! You're a genius. You know how long I've been testing this question, four hours!!! I did use <TrackList /> in another component and totally forgot about it
– Yanze
Nov 14 '18 at 5:30
@Yanze, no issues glad to have helped
– Shubham Khatri
Nov 14 '18 at 5:31
By any chance, is the trackList component used somewhere else too??
– Shubham Khatri
Nov 14 '18 at 5:18
By any chance, is the trackList component used somewhere else too??
– Shubham Khatri
Nov 14 '18 at 5:18
It's pretty tough to tell from your code what's going on. Can you post a codesandbox (or similar) link? From what I can tell, you shouldn't be having issues.
– Riley Steele Parsons
Nov 14 '18 at 5:20
It's pretty tough to tell from your code what's going on. Can you post a codesandbox (or similar) link? From what I can tell, you shouldn't be having issues.
– Riley Steele Parsons
Nov 14 '18 at 5:20
@ShubhamKhatri My god!!! You're a genius. You know how long I've been testing this question, four hours!!! I did use <TrackList /> in another component and totally forgot about it
– Yanze
Nov 14 '18 at 5:30
@ShubhamKhatri My god!!! You're a genius. You know how long I've been testing this question, four hours!!! I did use <TrackList /> in another component and totally forgot about it
– Yanze
Nov 14 '18 at 5:30
@Yanze, no issues glad to have helped
– Shubham Khatri
Nov 14 '18 at 5:31
@Yanze, no issues glad to have helped
– Shubham Khatri
Nov 14 '18 at 5:31
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53293557%2fwhen-this-props-is-passed-to-the-child-component-it-becomes-undefined%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53293557%2fwhen-this-props-is-passed-to-the-child-component-it-becomes-undefined%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DdQoNmeEIC
By any chance, is the trackList component used somewhere else too??
– Shubham Khatri
Nov 14 '18 at 5:18
It's pretty tough to tell from your code what's going on. Can you post a codesandbox (or similar) link? From what I can tell, you shouldn't be having issues.
– Riley Steele Parsons
Nov 14 '18 at 5:20
@ShubhamKhatri My god!!! You're a genius. You know how long I've been testing this question, four hours!!! I did use <TrackList /> in another component and totally forgot about it
– Yanze
Nov 14 '18 at 5:30
@Yanze, no issues glad to have helped
– Shubham Khatri
Nov 14 '18 at 5:31