Getting data from AWS Dynamodb through API Gateway using NodeJS Error
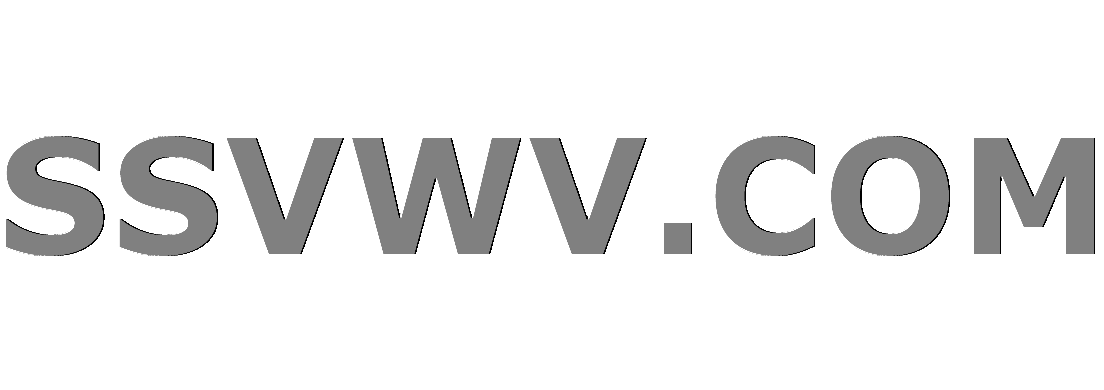
Multi tool use
up vote
0
down vote
favorite
I'm learning to build a API using Amazon Web Services' API Gateway, DynamoDB, and Lambda.
I've been following a video playlist tutorial on Youtube. So far, I've created the desired Lambda Function, DynamoDB Table, and also the API Gateway.
Now all I need to do is build a frontend that display my current items in the table and also the entry.
So far I made it to this part here.
In the video, he uses JavaScript to make an ajax request and fetch the items in the DynamoDB Table. I've written the same ajax request with the html.
<html>
<head>
<h1 style="color:rosybrown;">Latest guestbook entries:</h1>
</head>
<body>
<div id="entries">
</div>
<h1>New Entry</h1>
<form>
<label for="msg">Message</label>
<textarea id="msg"></textarea>
<button id="submitButton">Submit</button>
</form>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script type="text/javascript">
var API_URL = "someURL/prod/entries";
$(document).ready(function(){
$.ajax({
type: "GET",
url: API_URL,
success: function(data){
$("#entries").html("");
data.Items.forEach(function(guestbookItem){
$("#entries").append("<p>"+guestbookItem+"</p>");
})
}
});
});
</script>
</body>
So here I'm going to explain my purpose. The goal is the display the items in the DynamODB table that I have created while also accepting new entries. It has a date
and message
item.
The API Gateway has an /entries
resource and has two methods, GET
and POST
. /prod
means it was deployed on a production stage.
When invoking the URL for the GET
method. I receive this output
"{"Items": [{"date": 20180512, "message": "this is my 4th message"}, {"date": 20180513, "message": "I love AWS"}], "Count": 2, "ScannedCount": 2, "ResponseMetadata": {"RequestId": "SOME_REQUEST_ID", "HTTPStatusCode": 200, "HTTPHeaders": {"server": "Server", "date": "Mon, 14 May 2018 20:32:43 GMT", "content-type": "application/x-amz-json-1.0", "content-length": "160", "connection": "keep-alive", "x-amzn-requestid": "SOME_REQUEST_ID", "x-amz-crc32": "2401247050"}, "RetryAttempts": 0}}"
However, after running the index.html
file on my local host.
The entries did not appear. When I checked the console, it turns out I receive the following error
index.html:33 Uncaught TypeError: Cannot read property 'forEach' of undefined
at Object.success (index.html:33)
at u (jquery.min.js:2)
at Object.fireWith [as resolveWith] (jquery.min.js:2)
at k (jquery.min.js:2)
at XMLHttpRequest.<anonymous> (jquery.min.js:2)
I was not really sure what is going on. Items
does exist in the response. I've been stuck with this for days. If anybody could point out what I was missing, it would be greatly appreciated!
amazon-web-services amazon-dynamodb
|
show 3 more comments
up vote
0
down vote
favorite
I'm learning to build a API using Amazon Web Services' API Gateway, DynamoDB, and Lambda.
I've been following a video playlist tutorial on Youtube. So far, I've created the desired Lambda Function, DynamoDB Table, and also the API Gateway.
Now all I need to do is build a frontend that display my current items in the table and also the entry.
So far I made it to this part here.
In the video, he uses JavaScript to make an ajax request and fetch the items in the DynamoDB Table. I've written the same ajax request with the html.
<html>
<head>
<h1 style="color:rosybrown;">Latest guestbook entries:</h1>
</head>
<body>
<div id="entries">
</div>
<h1>New Entry</h1>
<form>
<label for="msg">Message</label>
<textarea id="msg"></textarea>
<button id="submitButton">Submit</button>
</form>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script type="text/javascript">
var API_URL = "someURL/prod/entries";
$(document).ready(function(){
$.ajax({
type: "GET",
url: API_URL,
success: function(data){
$("#entries").html("");
data.Items.forEach(function(guestbookItem){
$("#entries").append("<p>"+guestbookItem+"</p>");
})
}
});
});
</script>
</body>
So here I'm going to explain my purpose. The goal is the display the items in the DynamODB table that I have created while also accepting new entries. It has a date
and message
item.
The API Gateway has an /entries
resource and has two methods, GET
and POST
. /prod
means it was deployed on a production stage.
When invoking the URL for the GET
method. I receive this output
"{"Items": [{"date": 20180512, "message": "this is my 4th message"}, {"date": 20180513, "message": "I love AWS"}], "Count": 2, "ScannedCount": 2, "ResponseMetadata": {"RequestId": "SOME_REQUEST_ID", "HTTPStatusCode": 200, "HTTPHeaders": {"server": "Server", "date": "Mon, 14 May 2018 20:32:43 GMT", "content-type": "application/x-amz-json-1.0", "content-length": "160", "connection": "keep-alive", "x-amzn-requestid": "SOME_REQUEST_ID", "x-amz-crc32": "2401247050"}, "RetryAttempts": 0}}"
However, after running the index.html
file on my local host.
The entries did not appear. When I checked the console, it turns out I receive the following error
index.html:33 Uncaught TypeError: Cannot read property 'forEach' of undefined
at Object.success (index.html:33)
at u (jquery.min.js:2)
at Object.fireWith [as resolveWith] (jquery.min.js:2)
at k (jquery.min.js:2)
at XMLHttpRequest.<anonymous> (jquery.min.js:2)
I was not really sure what is going on. Items
does exist in the response. I've been stuck with this for days. If anybody could point out what I was missing, it would be greatly appreciated!
amazon-web-services amazon-dynamodb
could you console.log(data) in your success function please? It's been a while since I used jQuery.
– Mrk Fldig
May 14 at 22:15
Certainly! it outputs the same as what I get when I invoked the URL for theGET
method :) `
– Imperator123
May 14 at 22:48
hrm try console.log(JSON.parse(data.Items)) ? It looks like you're getting a string back, you need to turn that into a JS object. although I thought JQ did that.
– Mrk Fldig
May 14 at 22:49
So actually you wanna do var theItems = JSON.parse(data.Items); theItems.forEach.....
– Mrk Fldig
May 14 at 22:52
1
Okay, i was able to solve it. Doingvar theItems = JSON.parse(data);
actually solved it
– Imperator123
May 14 at 23:17
|
show 3 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm learning to build a API using Amazon Web Services' API Gateway, DynamoDB, and Lambda.
I've been following a video playlist tutorial on Youtube. So far, I've created the desired Lambda Function, DynamoDB Table, and also the API Gateway.
Now all I need to do is build a frontend that display my current items in the table and also the entry.
So far I made it to this part here.
In the video, he uses JavaScript to make an ajax request and fetch the items in the DynamoDB Table. I've written the same ajax request with the html.
<html>
<head>
<h1 style="color:rosybrown;">Latest guestbook entries:</h1>
</head>
<body>
<div id="entries">
</div>
<h1>New Entry</h1>
<form>
<label for="msg">Message</label>
<textarea id="msg"></textarea>
<button id="submitButton">Submit</button>
</form>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script type="text/javascript">
var API_URL = "someURL/prod/entries";
$(document).ready(function(){
$.ajax({
type: "GET",
url: API_URL,
success: function(data){
$("#entries").html("");
data.Items.forEach(function(guestbookItem){
$("#entries").append("<p>"+guestbookItem+"</p>");
})
}
});
});
</script>
</body>
So here I'm going to explain my purpose. The goal is the display the items in the DynamODB table that I have created while also accepting new entries. It has a date
and message
item.
The API Gateway has an /entries
resource and has two methods, GET
and POST
. /prod
means it was deployed on a production stage.
When invoking the URL for the GET
method. I receive this output
"{"Items": [{"date": 20180512, "message": "this is my 4th message"}, {"date": 20180513, "message": "I love AWS"}], "Count": 2, "ScannedCount": 2, "ResponseMetadata": {"RequestId": "SOME_REQUEST_ID", "HTTPStatusCode": 200, "HTTPHeaders": {"server": "Server", "date": "Mon, 14 May 2018 20:32:43 GMT", "content-type": "application/x-amz-json-1.0", "content-length": "160", "connection": "keep-alive", "x-amzn-requestid": "SOME_REQUEST_ID", "x-amz-crc32": "2401247050"}, "RetryAttempts": 0}}"
However, after running the index.html
file on my local host.
The entries did not appear. When I checked the console, it turns out I receive the following error
index.html:33 Uncaught TypeError: Cannot read property 'forEach' of undefined
at Object.success (index.html:33)
at u (jquery.min.js:2)
at Object.fireWith [as resolveWith] (jquery.min.js:2)
at k (jquery.min.js:2)
at XMLHttpRequest.<anonymous> (jquery.min.js:2)
I was not really sure what is going on. Items
does exist in the response. I've been stuck with this for days. If anybody could point out what I was missing, it would be greatly appreciated!
amazon-web-services amazon-dynamodb
I'm learning to build a API using Amazon Web Services' API Gateway, DynamoDB, and Lambda.
I've been following a video playlist tutorial on Youtube. So far, I've created the desired Lambda Function, DynamoDB Table, and also the API Gateway.
Now all I need to do is build a frontend that display my current items in the table and also the entry.
So far I made it to this part here.
In the video, he uses JavaScript to make an ajax request and fetch the items in the DynamoDB Table. I've written the same ajax request with the html.
<html>
<head>
<h1 style="color:rosybrown;">Latest guestbook entries:</h1>
</head>
<body>
<div id="entries">
</div>
<h1>New Entry</h1>
<form>
<label for="msg">Message</label>
<textarea id="msg"></textarea>
<button id="submitButton">Submit</button>
</form>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script type="text/javascript">
var API_URL = "someURL/prod/entries";
$(document).ready(function(){
$.ajax({
type: "GET",
url: API_URL,
success: function(data){
$("#entries").html("");
data.Items.forEach(function(guestbookItem){
$("#entries").append("<p>"+guestbookItem+"</p>");
})
}
});
});
</script>
</body>
So here I'm going to explain my purpose. The goal is the display the items in the DynamODB table that I have created while also accepting new entries. It has a date
and message
item.
The API Gateway has an /entries
resource and has two methods, GET
and POST
. /prod
means it was deployed on a production stage.
When invoking the URL for the GET
method. I receive this output
"{"Items": [{"date": 20180512, "message": "this is my 4th message"}, {"date": 20180513, "message": "I love AWS"}], "Count": 2, "ScannedCount": 2, "ResponseMetadata": {"RequestId": "SOME_REQUEST_ID", "HTTPStatusCode": 200, "HTTPHeaders": {"server": "Server", "date": "Mon, 14 May 2018 20:32:43 GMT", "content-type": "application/x-amz-json-1.0", "content-length": "160", "connection": "keep-alive", "x-amzn-requestid": "SOME_REQUEST_ID", "x-amz-crc32": "2401247050"}, "RetryAttempts": 0}}"
However, after running the index.html
file on my local host.
The entries did not appear. When I checked the console, it turns out I receive the following error
index.html:33 Uncaught TypeError: Cannot read property 'forEach' of undefined
at Object.success (index.html:33)
at u (jquery.min.js:2)
at Object.fireWith [as resolveWith] (jquery.min.js:2)
at k (jquery.min.js:2)
at XMLHttpRequest.<anonymous> (jquery.min.js:2)
I was not really sure what is going on. Items
does exist in the response. I've been stuck with this for days. If anybody could point out what I was missing, it would be greatly appreciated!
amazon-web-services amazon-dynamodb
amazon-web-services amazon-dynamodb
asked May 14 at 22:10
Imperator123
581312
581312
could you console.log(data) in your success function please? It's been a while since I used jQuery.
– Mrk Fldig
May 14 at 22:15
Certainly! it outputs the same as what I get when I invoked the URL for theGET
method :) `
– Imperator123
May 14 at 22:48
hrm try console.log(JSON.parse(data.Items)) ? It looks like you're getting a string back, you need to turn that into a JS object. although I thought JQ did that.
– Mrk Fldig
May 14 at 22:49
So actually you wanna do var theItems = JSON.parse(data.Items); theItems.forEach.....
– Mrk Fldig
May 14 at 22:52
1
Okay, i was able to solve it. Doingvar theItems = JSON.parse(data);
actually solved it
– Imperator123
May 14 at 23:17
|
show 3 more comments
could you console.log(data) in your success function please? It's been a while since I used jQuery.
– Mrk Fldig
May 14 at 22:15
Certainly! it outputs the same as what I get when I invoked the URL for theGET
method :) `
– Imperator123
May 14 at 22:48
hrm try console.log(JSON.parse(data.Items)) ? It looks like you're getting a string back, you need to turn that into a JS object. although I thought JQ did that.
– Mrk Fldig
May 14 at 22:49
So actually you wanna do var theItems = JSON.parse(data.Items); theItems.forEach.....
– Mrk Fldig
May 14 at 22:52
1
Okay, i was able to solve it. Doingvar theItems = JSON.parse(data);
actually solved it
– Imperator123
May 14 at 23:17
could you console.log(data) in your success function please? It's been a while since I used jQuery.
– Mrk Fldig
May 14 at 22:15
could you console.log(data) in your success function please? It's been a while since I used jQuery.
– Mrk Fldig
May 14 at 22:15
Certainly! it outputs the same as what I get when I invoked the URL for the
GET
method :) `– Imperator123
May 14 at 22:48
Certainly! it outputs the same as what I get when I invoked the URL for the
GET
method :) `– Imperator123
May 14 at 22:48
hrm try console.log(JSON.parse(data.Items)) ? It looks like you're getting a string back, you need to turn that into a JS object. although I thought JQ did that.
– Mrk Fldig
May 14 at 22:49
hrm try console.log(JSON.parse(data.Items)) ? It looks like you're getting a string back, you need to turn that into a JS object. although I thought JQ did that.
– Mrk Fldig
May 14 at 22:49
So actually you wanna do var theItems = JSON.parse(data.Items); theItems.forEach.....
– Mrk Fldig
May 14 at 22:52
So actually you wanna do var theItems = JSON.parse(data.Items); theItems.forEach.....
– Mrk Fldig
May 14 at 22:52
1
1
Okay, i was able to solve it. Doing
var theItems = JSON.parse(data);
actually solved it– Imperator123
May 14 at 23:17
Okay, i was able to solve it. Doing
var theItems = JSON.parse(data);
actually solved it– Imperator123
May 14 at 23:17
|
show 3 more comments
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f50339717%2fgetting-data-from-aws-dynamodb-through-api-gateway-using-nodejs-error%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
d2hSfO Z,g86R 8Gfp,eXX4,GmbVzvankJ5jd Va,SBQVaYWhz6Fk4w0eZiXjOO36z0kDAaM S0YM c,0B8 9chrpsVH,nZ5Asv4A4MLUkLTIu
could you console.log(data) in your success function please? It's been a while since I used jQuery.
– Mrk Fldig
May 14 at 22:15
Certainly! it outputs the same as what I get when I invoked the URL for the
GET
method :) `– Imperator123
May 14 at 22:48
hrm try console.log(JSON.parse(data.Items)) ? It looks like you're getting a string back, you need to turn that into a JS object. although I thought JQ did that.
– Mrk Fldig
May 14 at 22:49
So actually you wanna do var theItems = JSON.parse(data.Items); theItems.forEach.....
– Mrk Fldig
May 14 at 22:52
1
Okay, i was able to solve it. Doing
var theItems = JSON.parse(data);
actually solved it– Imperator123
May 14 at 23:17