415 Media not supported, calling post REST endpoint
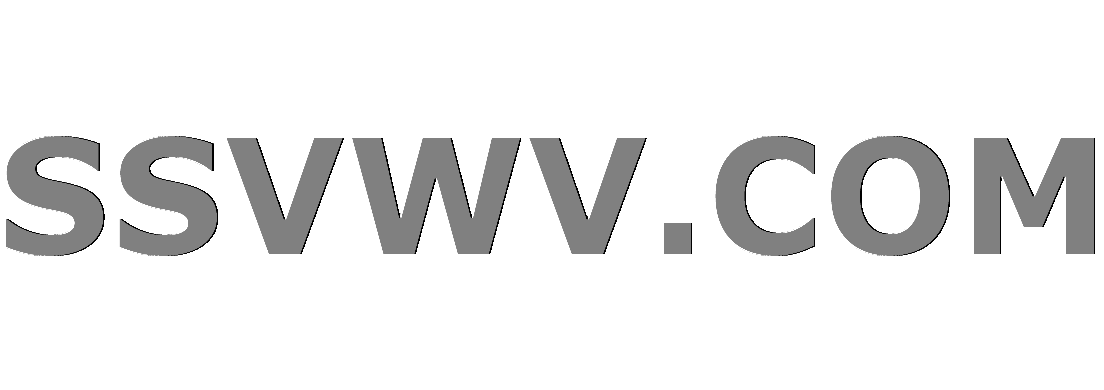
Multi tool use
up vote
2
down vote
favorite
I am trying to call a webapi from reactjs and I get this error:
415 Media not supported, calling post REST endpoint
[HttpPost]
[Route("")]
//[Route("api/SiteCollections/CreateModernSite")]
public async Task<IHttpActionResult> CreateModernSite([FromBody]NewSiteInformation model)
{
if (ModelState.IsValid)
{
AuthenticationManager auth = new AuthenticationManager();
auth.GetSharePointOnlineAuthenticatedContextTenant("url", "user", "password");
var tenant = await TenantHelper.GetTenantAsync();
using (var context = new OfficeDevPnP.Core.AuthenticationManager().GetSharePointOnlineAuthenticatedContextTenant("https://luisevalencia38.sharepoint.com/teamsite1/SitePages/Home.aspx", "luisevalencia38@luisevalencia38.onmicrosoft.com", "Vaz.717."))
{
var teamContext = await context.CreateSiteAsync(
new TeamSiteCollectionCreationInformation
{
Alias = model.Alias, // Mandatory
DisplayName = model.DisplayName, // Mandatory
Description = model.Description, // Optional
//Classification = Classification, // Optional
//IsPublic = IsPublic, // Optional, default true
}
);
teamContext.Load(teamContext.Web, _ => _.Url);
teamContext.ExecuteQueryRetry();
//204 with location and content set to created URL
return Created(teamContext.Web.Url, teamContext.Web.Url);
}
}
return BadRequest(ModelState);
}
My react code is this:
import React, { Component } from 'react';
import { Input} from 'antd';
import Form from '../../components/uielements/form';
import Button from '../../components/uielements/button';
import Notification from '../../components/notification';
import { adalApiFetch } from '../../adalConfig';
const FormItem = Form.Item;
class CreateSiteCollectionForm extends Component {
constructor(props) {
super(props);
this.state = {Alias:'',DisplayName:'', Description:''};
this.handleChangeAlias = this.handleChangeAlias.bind(this);
this.handleChangeDisplayName = this.handleChangeDisplayName.bind(this);
this.handleChangeDescription = this.handleChangeDescription.bind(this);
this.handleSubmit = this.handleSubmit.bind(this);
};
handleChangeAlias(event){
this.setState({Alias: event.target.value});
}
handleChangeDisplayName(event){
this.setState({DisplayName: event.target.value});
}
handleChangeDescription(event){
this.setState({Description: event.target.value});
}
handleSubmit(e){
e.preventDefault();
this.props.form.validateFieldsAndScroll((err, values) => {
if (!err) {
let data = new FormData();
//Append files to form data
//data.append(
const options = {
method: 'post',
body: JSON.stringify(
{
"Alias": this.state.Alias,
"DisplayName": this.state.DisplayName,
"Description": this.state.Description
}),
config: {
headers: {
'Content-Type': 'application/json; charset=utf-8'
}
}
};
adalApiFetch(fetch, "/SiteCollections", options)
.then(response =>{
if(response.status === 204){
Notification(
'success',
'Site collection created',
''
);
}else{
throw "error";
}
})
.catch(error => {
Notification(
'error',
'Site collection not created',
error
);
console.error(error);
});
}
});
}
render() {
const { getFieldDecorator } = this.props.form;
const formItemLayout = {
labelCol: {
xs: { span: 24 },
sm: { span: 6 },
},
wrapperCol: {
xs: { span: 24 },
sm: { span: 14 },
},
};
const tailFormItemLayout = {
wrapperCol: {
xs: {
span: 24,
offset: 0,
},
sm: {
span: 14,
offset: 6,
},
},
};
return (
<Form onSubmit={this.handleSubmit}>
<FormItem {...formItemLayout} label="Alias" hasFeedback>
{getFieldDecorator('Alias', {
rules: [
{
required: true,
message: 'Please input your alias',
}
]
})(<Input name="alias" id="alias" onChange={this.handleChangeAlias} />)}
</FormItem>
<FormItem {...formItemLayout} label="Display Name" hasFeedback>
{getFieldDecorator('displayname', {
rules: [
{
required: true,
message: 'Please input your display name',
}
]
})(<Input name="displayname" id="displayname" onChange={this.handleChangedisplayname} />)}
</FormItem>
<FormItem {...formItemLayout} label="Description" hasFeedback>
{getFieldDecorator('description', {
rules: [
{
required: true,
message: 'Please input your description',
}
],
})(<Input name="description" id="description" onChange={this.handleChangeDescription} />)}
</FormItem>
<FormItem {...tailFormItemLayout}>
<Button type="primary" htmlType="submit">
Create modern site
</Button>
</FormItem>
</Form>
);
}
}
const WrappedCreateSiteCollectionForm = Form.create()(CreateSiteCollectionForm);
export default WrappedCreateSiteCollectionForm;
How do I avoid: 415 Media not supported, when calling the endpoint? is my error on the client side code or on the webapi?
reactjs asp.net-web-api asp.net-web-api2
|
show 5 more comments
up vote
2
down vote
favorite
I am trying to call a webapi from reactjs and I get this error:
415 Media not supported, calling post REST endpoint
[HttpPost]
[Route("")]
//[Route("api/SiteCollections/CreateModernSite")]
public async Task<IHttpActionResult> CreateModernSite([FromBody]NewSiteInformation model)
{
if (ModelState.IsValid)
{
AuthenticationManager auth = new AuthenticationManager();
auth.GetSharePointOnlineAuthenticatedContextTenant("url", "user", "password");
var tenant = await TenantHelper.GetTenantAsync();
using (var context = new OfficeDevPnP.Core.AuthenticationManager().GetSharePointOnlineAuthenticatedContextTenant("https://luisevalencia38.sharepoint.com/teamsite1/SitePages/Home.aspx", "luisevalencia38@luisevalencia38.onmicrosoft.com", "Vaz.717."))
{
var teamContext = await context.CreateSiteAsync(
new TeamSiteCollectionCreationInformation
{
Alias = model.Alias, // Mandatory
DisplayName = model.DisplayName, // Mandatory
Description = model.Description, // Optional
//Classification = Classification, // Optional
//IsPublic = IsPublic, // Optional, default true
}
);
teamContext.Load(teamContext.Web, _ => _.Url);
teamContext.ExecuteQueryRetry();
//204 with location and content set to created URL
return Created(teamContext.Web.Url, teamContext.Web.Url);
}
}
return BadRequest(ModelState);
}
My react code is this:
import React, { Component } from 'react';
import { Input} from 'antd';
import Form from '../../components/uielements/form';
import Button from '../../components/uielements/button';
import Notification from '../../components/notification';
import { adalApiFetch } from '../../adalConfig';
const FormItem = Form.Item;
class CreateSiteCollectionForm extends Component {
constructor(props) {
super(props);
this.state = {Alias:'',DisplayName:'', Description:''};
this.handleChangeAlias = this.handleChangeAlias.bind(this);
this.handleChangeDisplayName = this.handleChangeDisplayName.bind(this);
this.handleChangeDescription = this.handleChangeDescription.bind(this);
this.handleSubmit = this.handleSubmit.bind(this);
};
handleChangeAlias(event){
this.setState({Alias: event.target.value});
}
handleChangeDisplayName(event){
this.setState({DisplayName: event.target.value});
}
handleChangeDescription(event){
this.setState({Description: event.target.value});
}
handleSubmit(e){
e.preventDefault();
this.props.form.validateFieldsAndScroll((err, values) => {
if (!err) {
let data = new FormData();
//Append files to form data
//data.append(
const options = {
method: 'post',
body: JSON.stringify(
{
"Alias": this.state.Alias,
"DisplayName": this.state.DisplayName,
"Description": this.state.Description
}),
config: {
headers: {
'Content-Type': 'application/json; charset=utf-8'
}
}
};
adalApiFetch(fetch, "/SiteCollections", options)
.then(response =>{
if(response.status === 204){
Notification(
'success',
'Site collection created',
''
);
}else{
throw "error";
}
})
.catch(error => {
Notification(
'error',
'Site collection not created',
error
);
console.error(error);
});
}
});
}
render() {
const { getFieldDecorator } = this.props.form;
const formItemLayout = {
labelCol: {
xs: { span: 24 },
sm: { span: 6 },
},
wrapperCol: {
xs: { span: 24 },
sm: { span: 14 },
},
};
const tailFormItemLayout = {
wrapperCol: {
xs: {
span: 24,
offset: 0,
},
sm: {
span: 14,
offset: 6,
},
},
};
return (
<Form onSubmit={this.handleSubmit}>
<FormItem {...formItemLayout} label="Alias" hasFeedback>
{getFieldDecorator('Alias', {
rules: [
{
required: true,
message: 'Please input your alias',
}
]
})(<Input name="alias" id="alias" onChange={this.handleChangeAlias} />)}
</FormItem>
<FormItem {...formItemLayout} label="Display Name" hasFeedback>
{getFieldDecorator('displayname', {
rules: [
{
required: true,
message: 'Please input your display name',
}
]
})(<Input name="displayname" id="displayname" onChange={this.handleChangedisplayname} />)}
</FormItem>
<FormItem {...formItemLayout} label="Description" hasFeedback>
{getFieldDecorator('description', {
rules: [
{
required: true,
message: 'Please input your description',
}
],
})(<Input name="description" id="description" onChange={this.handleChangeDescription} />)}
</FormItem>
<FormItem {...tailFormItemLayout}>
<Button type="primary" htmlType="submit">
Create modern site
</Button>
</FormItem>
</Form>
);
}
}
const WrappedCreateSiteCollectionForm = Form.create()(CreateSiteCollectionForm);
export default WrappedCreateSiteCollectionForm;
How do I avoid: 415 Media not supported, when calling the endpoint? is my error on the client side code or on the webapi?
reactjs asp.net-web-api asp.net-web-api2
updated, just in case it was not clear
– Luis Valencia
Oct 27 at 16:11
You have core web api in tags but all the code looks like web api2. was that a mistake?
– Nkosi
Oct 27 at 16:17
Check to see if any changes have been made to the Web Api Formatter when configuring it. (WebApiConfig)
– Nkosi
Oct 27 at 16:21
no, that file is the default one, I assumed .net api core is almost the same, so I tagged with it
– Luis Valencia
Oct 27 at 16:24
1
for some reason content type goes: text/plain;charset=UTF-8
– Luis Valencia
Nov 5 at 20:43
|
show 5 more comments
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I am trying to call a webapi from reactjs and I get this error:
415 Media not supported, calling post REST endpoint
[HttpPost]
[Route("")]
//[Route("api/SiteCollections/CreateModernSite")]
public async Task<IHttpActionResult> CreateModernSite([FromBody]NewSiteInformation model)
{
if (ModelState.IsValid)
{
AuthenticationManager auth = new AuthenticationManager();
auth.GetSharePointOnlineAuthenticatedContextTenant("url", "user", "password");
var tenant = await TenantHelper.GetTenantAsync();
using (var context = new OfficeDevPnP.Core.AuthenticationManager().GetSharePointOnlineAuthenticatedContextTenant("https://luisevalencia38.sharepoint.com/teamsite1/SitePages/Home.aspx", "luisevalencia38@luisevalencia38.onmicrosoft.com", "Vaz.717."))
{
var teamContext = await context.CreateSiteAsync(
new TeamSiteCollectionCreationInformation
{
Alias = model.Alias, // Mandatory
DisplayName = model.DisplayName, // Mandatory
Description = model.Description, // Optional
//Classification = Classification, // Optional
//IsPublic = IsPublic, // Optional, default true
}
);
teamContext.Load(teamContext.Web, _ => _.Url);
teamContext.ExecuteQueryRetry();
//204 with location and content set to created URL
return Created(teamContext.Web.Url, teamContext.Web.Url);
}
}
return BadRequest(ModelState);
}
My react code is this:
import React, { Component } from 'react';
import { Input} from 'antd';
import Form from '../../components/uielements/form';
import Button from '../../components/uielements/button';
import Notification from '../../components/notification';
import { adalApiFetch } from '../../adalConfig';
const FormItem = Form.Item;
class CreateSiteCollectionForm extends Component {
constructor(props) {
super(props);
this.state = {Alias:'',DisplayName:'', Description:''};
this.handleChangeAlias = this.handleChangeAlias.bind(this);
this.handleChangeDisplayName = this.handleChangeDisplayName.bind(this);
this.handleChangeDescription = this.handleChangeDescription.bind(this);
this.handleSubmit = this.handleSubmit.bind(this);
};
handleChangeAlias(event){
this.setState({Alias: event.target.value});
}
handleChangeDisplayName(event){
this.setState({DisplayName: event.target.value});
}
handleChangeDescription(event){
this.setState({Description: event.target.value});
}
handleSubmit(e){
e.preventDefault();
this.props.form.validateFieldsAndScroll((err, values) => {
if (!err) {
let data = new FormData();
//Append files to form data
//data.append(
const options = {
method: 'post',
body: JSON.stringify(
{
"Alias": this.state.Alias,
"DisplayName": this.state.DisplayName,
"Description": this.state.Description
}),
config: {
headers: {
'Content-Type': 'application/json; charset=utf-8'
}
}
};
adalApiFetch(fetch, "/SiteCollections", options)
.then(response =>{
if(response.status === 204){
Notification(
'success',
'Site collection created',
''
);
}else{
throw "error";
}
})
.catch(error => {
Notification(
'error',
'Site collection not created',
error
);
console.error(error);
});
}
});
}
render() {
const { getFieldDecorator } = this.props.form;
const formItemLayout = {
labelCol: {
xs: { span: 24 },
sm: { span: 6 },
},
wrapperCol: {
xs: { span: 24 },
sm: { span: 14 },
},
};
const tailFormItemLayout = {
wrapperCol: {
xs: {
span: 24,
offset: 0,
},
sm: {
span: 14,
offset: 6,
},
},
};
return (
<Form onSubmit={this.handleSubmit}>
<FormItem {...formItemLayout} label="Alias" hasFeedback>
{getFieldDecorator('Alias', {
rules: [
{
required: true,
message: 'Please input your alias',
}
]
})(<Input name="alias" id="alias" onChange={this.handleChangeAlias} />)}
</FormItem>
<FormItem {...formItemLayout} label="Display Name" hasFeedback>
{getFieldDecorator('displayname', {
rules: [
{
required: true,
message: 'Please input your display name',
}
]
})(<Input name="displayname" id="displayname" onChange={this.handleChangedisplayname} />)}
</FormItem>
<FormItem {...formItemLayout} label="Description" hasFeedback>
{getFieldDecorator('description', {
rules: [
{
required: true,
message: 'Please input your description',
}
],
})(<Input name="description" id="description" onChange={this.handleChangeDescription} />)}
</FormItem>
<FormItem {...tailFormItemLayout}>
<Button type="primary" htmlType="submit">
Create modern site
</Button>
</FormItem>
</Form>
);
}
}
const WrappedCreateSiteCollectionForm = Form.create()(CreateSiteCollectionForm);
export default WrappedCreateSiteCollectionForm;
How do I avoid: 415 Media not supported, when calling the endpoint? is my error on the client side code or on the webapi?
reactjs asp.net-web-api asp.net-web-api2
I am trying to call a webapi from reactjs and I get this error:
415 Media not supported, calling post REST endpoint
[HttpPost]
[Route("")]
//[Route("api/SiteCollections/CreateModernSite")]
public async Task<IHttpActionResult> CreateModernSite([FromBody]NewSiteInformation model)
{
if (ModelState.IsValid)
{
AuthenticationManager auth = new AuthenticationManager();
auth.GetSharePointOnlineAuthenticatedContextTenant("url", "user", "password");
var tenant = await TenantHelper.GetTenantAsync();
using (var context = new OfficeDevPnP.Core.AuthenticationManager().GetSharePointOnlineAuthenticatedContextTenant("https://luisevalencia38.sharepoint.com/teamsite1/SitePages/Home.aspx", "luisevalencia38@luisevalencia38.onmicrosoft.com", "Vaz.717."))
{
var teamContext = await context.CreateSiteAsync(
new TeamSiteCollectionCreationInformation
{
Alias = model.Alias, // Mandatory
DisplayName = model.DisplayName, // Mandatory
Description = model.Description, // Optional
//Classification = Classification, // Optional
//IsPublic = IsPublic, // Optional, default true
}
);
teamContext.Load(teamContext.Web, _ => _.Url);
teamContext.ExecuteQueryRetry();
//204 with location and content set to created URL
return Created(teamContext.Web.Url, teamContext.Web.Url);
}
}
return BadRequest(ModelState);
}
My react code is this:
import React, { Component } from 'react';
import { Input} from 'antd';
import Form from '../../components/uielements/form';
import Button from '../../components/uielements/button';
import Notification from '../../components/notification';
import { adalApiFetch } from '../../adalConfig';
const FormItem = Form.Item;
class CreateSiteCollectionForm extends Component {
constructor(props) {
super(props);
this.state = {Alias:'',DisplayName:'', Description:''};
this.handleChangeAlias = this.handleChangeAlias.bind(this);
this.handleChangeDisplayName = this.handleChangeDisplayName.bind(this);
this.handleChangeDescription = this.handleChangeDescription.bind(this);
this.handleSubmit = this.handleSubmit.bind(this);
};
handleChangeAlias(event){
this.setState({Alias: event.target.value});
}
handleChangeDisplayName(event){
this.setState({DisplayName: event.target.value});
}
handleChangeDescription(event){
this.setState({Description: event.target.value});
}
handleSubmit(e){
e.preventDefault();
this.props.form.validateFieldsAndScroll((err, values) => {
if (!err) {
let data = new FormData();
//Append files to form data
//data.append(
const options = {
method: 'post',
body: JSON.stringify(
{
"Alias": this.state.Alias,
"DisplayName": this.state.DisplayName,
"Description": this.state.Description
}),
config: {
headers: {
'Content-Type': 'application/json; charset=utf-8'
}
}
};
adalApiFetch(fetch, "/SiteCollections", options)
.then(response =>{
if(response.status === 204){
Notification(
'success',
'Site collection created',
''
);
}else{
throw "error";
}
})
.catch(error => {
Notification(
'error',
'Site collection not created',
error
);
console.error(error);
});
}
});
}
render() {
const { getFieldDecorator } = this.props.form;
const formItemLayout = {
labelCol: {
xs: { span: 24 },
sm: { span: 6 },
},
wrapperCol: {
xs: { span: 24 },
sm: { span: 14 },
},
};
const tailFormItemLayout = {
wrapperCol: {
xs: {
span: 24,
offset: 0,
},
sm: {
span: 14,
offset: 6,
},
},
};
return (
<Form onSubmit={this.handleSubmit}>
<FormItem {...formItemLayout} label="Alias" hasFeedback>
{getFieldDecorator('Alias', {
rules: [
{
required: true,
message: 'Please input your alias',
}
]
})(<Input name="alias" id="alias" onChange={this.handleChangeAlias} />)}
</FormItem>
<FormItem {...formItemLayout} label="Display Name" hasFeedback>
{getFieldDecorator('displayname', {
rules: [
{
required: true,
message: 'Please input your display name',
}
]
})(<Input name="displayname" id="displayname" onChange={this.handleChangedisplayname} />)}
</FormItem>
<FormItem {...formItemLayout} label="Description" hasFeedback>
{getFieldDecorator('description', {
rules: [
{
required: true,
message: 'Please input your description',
}
],
})(<Input name="description" id="description" onChange={this.handleChangeDescription} />)}
</FormItem>
<FormItem {...tailFormItemLayout}>
<Button type="primary" htmlType="submit">
Create modern site
</Button>
</FormItem>
</Form>
);
}
}
const WrappedCreateSiteCollectionForm = Form.create()(CreateSiteCollectionForm);
export default WrappedCreateSiteCollectionForm;
How do I avoid: 415 Media not supported, when calling the endpoint? is my error on the client side code or on the webapi?
reactjs asp.net-web-api asp.net-web-api2
reactjs asp.net-web-api asp.net-web-api2
edited Oct 29 at 3:05


Tao Zhou
4,19721027
4,19721027
asked Oct 27 at 15:52
Luis Valencia
8,78144163294
8,78144163294
updated, just in case it was not clear
– Luis Valencia
Oct 27 at 16:11
You have core web api in tags but all the code looks like web api2. was that a mistake?
– Nkosi
Oct 27 at 16:17
Check to see if any changes have been made to the Web Api Formatter when configuring it. (WebApiConfig)
– Nkosi
Oct 27 at 16:21
no, that file is the default one, I assumed .net api core is almost the same, so I tagged with it
– Luis Valencia
Oct 27 at 16:24
1
for some reason content type goes: text/plain;charset=UTF-8
– Luis Valencia
Nov 5 at 20:43
|
show 5 more comments
updated, just in case it was not clear
– Luis Valencia
Oct 27 at 16:11
You have core web api in tags but all the code looks like web api2. was that a mistake?
– Nkosi
Oct 27 at 16:17
Check to see if any changes have been made to the Web Api Formatter when configuring it. (WebApiConfig)
– Nkosi
Oct 27 at 16:21
no, that file is the default one, I assumed .net api core is almost the same, so I tagged with it
– Luis Valencia
Oct 27 at 16:24
1
for some reason content type goes: text/plain;charset=UTF-8
– Luis Valencia
Nov 5 at 20:43
updated, just in case it was not clear
– Luis Valencia
Oct 27 at 16:11
updated, just in case it was not clear
– Luis Valencia
Oct 27 at 16:11
You have core web api in tags but all the code looks like web api2. was that a mistake?
– Nkosi
Oct 27 at 16:17
You have core web api in tags but all the code looks like web api2. was that a mistake?
– Nkosi
Oct 27 at 16:17
Check to see if any changes have been made to the Web Api Formatter when configuring it. (WebApiConfig)
– Nkosi
Oct 27 at 16:21
Check to see if any changes have been made to the Web Api Formatter when configuring it. (WebApiConfig)
– Nkosi
Oct 27 at 16:21
no, that file is the default one, I assumed .net api core is almost the same, so I tagged with it
– Luis Valencia
Oct 27 at 16:24
no, that file is the default one, I assumed .net api core is almost the same, so I tagged with it
– Luis Valencia
Oct 27 at 16:24
1
1
for some reason content type goes: text/plain;charset=UTF-8
– Luis Valencia
Nov 5 at 20:43
for some reason content type goes: text/plain;charset=UTF-8
– Luis Valencia
Nov 5 at 20:43
|
show 5 more comments
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
Try to use this one
const options = {
method: 'post',
mode: "cors",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify(
{
"Alias": this.state.Alias,
"DisplayName": this.state.DisplayName,
"Description": this.state.Description
})
};
Also see this question, it seems that overriding the Content-Type
request header is not allowed for no-cors
requests.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
Try to use this one
const options = {
method: 'post',
mode: "cors",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify(
{
"Alias": this.state.Alias,
"DisplayName": this.state.DisplayName,
"Description": this.state.Description
})
};
Also see this question, it seems that overriding the Content-Type
request header is not allowed for no-cors
requests.
add a comment |
up vote
1
down vote
accepted
Try to use this one
const options = {
method: 'post',
mode: "cors",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify(
{
"Alias": this.state.Alias,
"DisplayName": this.state.DisplayName,
"Description": this.state.Description
})
};
Also see this question, it seems that overriding the Content-Type
request header is not allowed for no-cors
requests.
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
Try to use this one
const options = {
method: 'post',
mode: "cors",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify(
{
"Alias": this.state.Alias,
"DisplayName": this.state.DisplayName,
"Description": this.state.Description
})
};
Also see this question, it seems that overriding the Content-Type
request header is not allowed for no-cors
requests.
Try to use this one
const options = {
method: 'post',
mode: "cors",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify(
{
"Alias": this.state.Alias,
"DisplayName": this.state.DisplayName,
"Description": this.state.Description
})
};
Also see this question, it seems that overriding the Content-Type
request header is not allowed for no-cors
requests.
edited Nov 7 at 19:53
answered Nov 7 at 19:47


Roman Marusyk
11.6k123268
11.6k123268
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53023680%2f415-media-not-supported-calling-post-rest-endpoint%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LaG K3,X,coYHL4VXrKLiTE,sD2 Ofsx
updated, just in case it was not clear
– Luis Valencia
Oct 27 at 16:11
You have core web api in tags but all the code looks like web api2. was that a mistake?
– Nkosi
Oct 27 at 16:17
Check to see if any changes have been made to the Web Api Formatter when configuring it. (WebApiConfig)
– Nkosi
Oct 27 at 16:21
no, that file is the default one, I assumed .net api core is almost the same, so I tagged with it
– Luis Valencia
Oct 27 at 16:24
1
for some reason content type goes: text/plain;charset=UTF-8
– Luis Valencia
Nov 5 at 20:43