Node.js/Firebase: can't set headers after they're sent
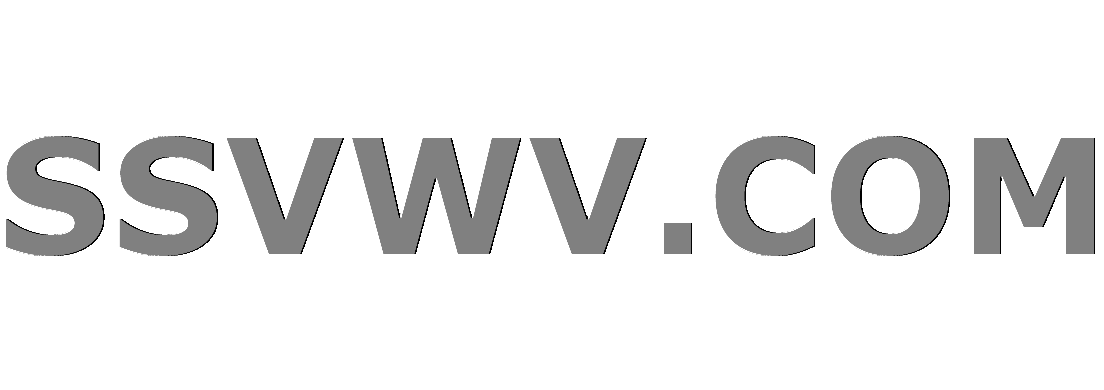
Multi tool use
up vote
0
down vote
favorite
We have an express.js/firebase project with an /api/progress POST route that takes a marker as a parameter and increments it if the scanned marker equals the current progress of the user in an array that stores a story order. For that, we have a GET route /api/progress that returns the user progress. We check if the number of the scanned marker equals the storyOrder[userProgress] (minus one because of array indices), user progress is obtained from the api/progress/?user="user" route.
When Number(marker) === storyOrder[response.data.data]-1
, we increment the progress in the firebase database by one. However, when the code is run, it sometimes produces the following error:
Error: Can't set headers after they are sent.
at validateHeader (_http_outgoing.js:491:11)
at ServerResponse.setHeader (_http_outgoing.js:498:3)
at ServerResponse.header (/Users/user/Documents/projects/project/node_modules/express/lib/response.js:767:10)
at ServerResponse.send (/Users/user/Documents/projects/project/node_modules/express/lib/response.js:170:12)
at ServerResponse.json (/Users/user/Documents/projects/project/node_modules/express/lib/response.js:267:15)
at userDB.on.data (file:///Users/user/Documents/projects/project/routes/api.mjs:104:15)
at /Users/user/Documents/projects/project/node_modules/@firebase/database/dist/index.node.cjs.js:4465:22
at exceptionGuard (/Users/user/Documents/projects/project/node_modules/@firebase/database/dist/index.node.cjs.js:691:9)
at EventList.raise (/Users/user/Documents/projects/project/node_modules/@firebase/database/dist/index.node.cjs.js:9727:17)
at EventQueue.raiseQueuedEventsMatchingPredicate_ (/Users/user/Documents/projects/project/node_modules/@firebase/database/dist/index.node.cjs.js:9681:41)
This is the code for the /api/progress GET and POST routes:
GET progress:
router.get('/progress', async (req, res, next) => {
let name = req.query.user || null;
console.log('Name: ',name);
let progress;
let dataList;
try {
const getData = async data => {
if (data.val()) {
let tmp = await data.val();
progress = parseInt(tmp[name].progressCounter);
console.log(progress);
// Return progress
res.json({ status: 200, data: progress });
} else {
res.json({ status: 500, err: 'No data! ' });
}
};
const errData = error => {
console.error('Something went wrong.');
console.error(error);
};
dataList = await userDB
.orderByKey()
.equalTo(name)
.on('value', getData, errData);
} catch (err) {
console.log('Error: ', err.message)
res.json({ status: 500, err: 'Error while getting progress' });
}
});
POST progress:
router.post('/progress', async (req, res, next) => {
const user = req.session.user;
const marker = req.body.marker;
if (marker!== undefined) {
const updateProgress = async progress => {
let updateProg = progress.data;
updateProg++;
await userDB.child(user).update({ progressCounter: updateProg });
res.json({ status: 200, marker: updateProg });
};
axios
.get(`http://localhost:5000/api/progress?user=${user}`)
.then(function(response) {
console.log(storyOrder[response.data.data]-1);
if (Number(marker) === storyOrder[response.data.data]-1) {
updateProgress(response.data);
} else {
res.status(304);
res.json({ status: 304 });
}
})
.catch(function(error) {
console.log(error);
});
}else{
res.json({status: 304})
}
});
node.js

New contributor
Alexander von Recum is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
We have an express.js/firebase project with an /api/progress POST route that takes a marker as a parameter and increments it if the scanned marker equals the current progress of the user in an array that stores a story order. For that, we have a GET route /api/progress that returns the user progress. We check if the number of the scanned marker equals the storyOrder[userProgress] (minus one because of array indices), user progress is obtained from the api/progress/?user="user" route.
When Number(marker) === storyOrder[response.data.data]-1
, we increment the progress in the firebase database by one. However, when the code is run, it sometimes produces the following error:
Error: Can't set headers after they are sent.
at validateHeader (_http_outgoing.js:491:11)
at ServerResponse.setHeader (_http_outgoing.js:498:3)
at ServerResponse.header (/Users/user/Documents/projects/project/node_modules/express/lib/response.js:767:10)
at ServerResponse.send (/Users/user/Documents/projects/project/node_modules/express/lib/response.js:170:12)
at ServerResponse.json (/Users/user/Documents/projects/project/node_modules/express/lib/response.js:267:15)
at userDB.on.data (file:///Users/user/Documents/projects/project/routes/api.mjs:104:15)
at /Users/user/Documents/projects/project/node_modules/@firebase/database/dist/index.node.cjs.js:4465:22
at exceptionGuard (/Users/user/Documents/projects/project/node_modules/@firebase/database/dist/index.node.cjs.js:691:9)
at EventList.raise (/Users/user/Documents/projects/project/node_modules/@firebase/database/dist/index.node.cjs.js:9727:17)
at EventQueue.raiseQueuedEventsMatchingPredicate_ (/Users/user/Documents/projects/project/node_modules/@firebase/database/dist/index.node.cjs.js:9681:41)
This is the code for the /api/progress GET and POST routes:
GET progress:
router.get('/progress', async (req, res, next) => {
let name = req.query.user || null;
console.log('Name: ',name);
let progress;
let dataList;
try {
const getData = async data => {
if (data.val()) {
let tmp = await data.val();
progress = parseInt(tmp[name].progressCounter);
console.log(progress);
// Return progress
res.json({ status: 200, data: progress });
} else {
res.json({ status: 500, err: 'No data! ' });
}
};
const errData = error => {
console.error('Something went wrong.');
console.error(error);
};
dataList = await userDB
.orderByKey()
.equalTo(name)
.on('value', getData, errData);
} catch (err) {
console.log('Error: ', err.message)
res.json({ status: 500, err: 'Error while getting progress' });
}
});
POST progress:
router.post('/progress', async (req, res, next) => {
const user = req.session.user;
const marker = req.body.marker;
if (marker!== undefined) {
const updateProgress = async progress => {
let updateProg = progress.data;
updateProg++;
await userDB.child(user).update({ progressCounter: updateProg });
res.json({ status: 200, marker: updateProg });
};
axios
.get(`http://localhost:5000/api/progress?user=${user}`)
.then(function(response) {
console.log(storyOrder[response.data.data]-1);
if (Number(marker) === storyOrder[response.data.data]-1) {
updateProgress(response.data);
} else {
res.status(304);
res.json({ status: 304 });
}
})
.catch(function(error) {
console.log(error);
});
}else{
res.json({status: 304})
}
});
node.js

New contributor
Alexander von Recum is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
We have an express.js/firebase project with an /api/progress POST route that takes a marker as a parameter and increments it if the scanned marker equals the current progress of the user in an array that stores a story order. For that, we have a GET route /api/progress that returns the user progress. We check if the number of the scanned marker equals the storyOrder[userProgress] (minus one because of array indices), user progress is obtained from the api/progress/?user="user" route.
When Number(marker) === storyOrder[response.data.data]-1
, we increment the progress in the firebase database by one. However, when the code is run, it sometimes produces the following error:
Error: Can't set headers after they are sent.
at validateHeader (_http_outgoing.js:491:11)
at ServerResponse.setHeader (_http_outgoing.js:498:3)
at ServerResponse.header (/Users/user/Documents/projects/project/node_modules/express/lib/response.js:767:10)
at ServerResponse.send (/Users/user/Documents/projects/project/node_modules/express/lib/response.js:170:12)
at ServerResponse.json (/Users/user/Documents/projects/project/node_modules/express/lib/response.js:267:15)
at userDB.on.data (file:///Users/user/Documents/projects/project/routes/api.mjs:104:15)
at /Users/user/Documents/projects/project/node_modules/@firebase/database/dist/index.node.cjs.js:4465:22
at exceptionGuard (/Users/user/Documents/projects/project/node_modules/@firebase/database/dist/index.node.cjs.js:691:9)
at EventList.raise (/Users/user/Documents/projects/project/node_modules/@firebase/database/dist/index.node.cjs.js:9727:17)
at EventQueue.raiseQueuedEventsMatchingPredicate_ (/Users/user/Documents/projects/project/node_modules/@firebase/database/dist/index.node.cjs.js:9681:41)
This is the code for the /api/progress GET and POST routes:
GET progress:
router.get('/progress', async (req, res, next) => {
let name = req.query.user || null;
console.log('Name: ',name);
let progress;
let dataList;
try {
const getData = async data => {
if (data.val()) {
let tmp = await data.val();
progress = parseInt(tmp[name].progressCounter);
console.log(progress);
// Return progress
res.json({ status: 200, data: progress });
} else {
res.json({ status: 500, err: 'No data! ' });
}
};
const errData = error => {
console.error('Something went wrong.');
console.error(error);
};
dataList = await userDB
.orderByKey()
.equalTo(name)
.on('value', getData, errData);
} catch (err) {
console.log('Error: ', err.message)
res.json({ status: 500, err: 'Error while getting progress' });
}
});
POST progress:
router.post('/progress', async (req, res, next) => {
const user = req.session.user;
const marker = req.body.marker;
if (marker!== undefined) {
const updateProgress = async progress => {
let updateProg = progress.data;
updateProg++;
await userDB.child(user).update({ progressCounter: updateProg });
res.json({ status: 200, marker: updateProg });
};
axios
.get(`http://localhost:5000/api/progress?user=${user}`)
.then(function(response) {
console.log(storyOrder[response.data.data]-1);
if (Number(marker) === storyOrder[response.data.data]-1) {
updateProgress(response.data);
} else {
res.status(304);
res.json({ status: 304 });
}
})
.catch(function(error) {
console.log(error);
});
}else{
res.json({status: 304})
}
});
node.js

New contributor
Alexander von Recum is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
We have an express.js/firebase project with an /api/progress POST route that takes a marker as a parameter and increments it if the scanned marker equals the current progress of the user in an array that stores a story order. For that, we have a GET route /api/progress that returns the user progress. We check if the number of the scanned marker equals the storyOrder[userProgress] (minus one because of array indices), user progress is obtained from the api/progress/?user="user" route.
When Number(marker) === storyOrder[response.data.data]-1
, we increment the progress in the firebase database by one. However, when the code is run, it sometimes produces the following error:
Error: Can't set headers after they are sent.
at validateHeader (_http_outgoing.js:491:11)
at ServerResponse.setHeader (_http_outgoing.js:498:3)
at ServerResponse.header (/Users/user/Documents/projects/project/node_modules/express/lib/response.js:767:10)
at ServerResponse.send (/Users/user/Documents/projects/project/node_modules/express/lib/response.js:170:12)
at ServerResponse.json (/Users/user/Documents/projects/project/node_modules/express/lib/response.js:267:15)
at userDB.on.data (file:///Users/user/Documents/projects/project/routes/api.mjs:104:15)
at /Users/user/Documents/projects/project/node_modules/@firebase/database/dist/index.node.cjs.js:4465:22
at exceptionGuard (/Users/user/Documents/projects/project/node_modules/@firebase/database/dist/index.node.cjs.js:691:9)
at EventList.raise (/Users/user/Documents/projects/project/node_modules/@firebase/database/dist/index.node.cjs.js:9727:17)
at EventQueue.raiseQueuedEventsMatchingPredicate_ (/Users/user/Documents/projects/project/node_modules/@firebase/database/dist/index.node.cjs.js:9681:41)
This is the code for the /api/progress GET and POST routes:
GET progress:
router.get('/progress', async (req, res, next) => {
let name = req.query.user || null;
console.log('Name: ',name);
let progress;
let dataList;
try {
const getData = async data => {
if (data.val()) {
let tmp = await data.val();
progress = parseInt(tmp[name].progressCounter);
console.log(progress);
// Return progress
res.json({ status: 200, data: progress });
} else {
res.json({ status: 500, err: 'No data! ' });
}
};
const errData = error => {
console.error('Something went wrong.');
console.error(error);
};
dataList = await userDB
.orderByKey()
.equalTo(name)
.on('value', getData, errData);
} catch (err) {
console.log('Error: ', err.message)
res.json({ status: 500, err: 'Error while getting progress' });
}
});
POST progress:
router.post('/progress', async (req, res, next) => {
const user = req.session.user;
const marker = req.body.marker;
if (marker!== undefined) {
const updateProgress = async progress => {
let updateProg = progress.data;
updateProg++;
await userDB.child(user).update({ progressCounter: updateProg });
res.json({ status: 200, marker: updateProg });
};
axios
.get(`http://localhost:5000/api/progress?user=${user}`)
.then(function(response) {
console.log(storyOrder[response.data.data]-1);
if (Number(marker) === storyOrder[response.data.data]-1) {
updateProgress(response.data);
} else {
res.status(304);
res.json({ status: 304 });
}
})
.catch(function(error) {
console.log(error);
});
}else{
res.json({status: 304})
}
});
node.js

node.js

New contributor
Alexander von Recum is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Alexander von Recum is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Alexander von Recum is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked yesterday


Alexander von Recum
31
31
New contributor
Alexander von Recum is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Alexander von Recum is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Alexander von Recum is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
You are getting that error because, your calling res.send() in the middle of your function and the code continues
This piece of code is called
const getData = async data => {
if (data.val()) {
let tmp = await data.val();
progress = parseInt(tmp[name].progressCounter);
console.log(progress);
// Return progress
res.json({ status: 200, data: progress });
} else {
res.json({ status: 500, err: 'No data! ' });
}
since an error occurs, is called
res.json({ status: 500, err: 'No data! ' });
And then this
dataList = await userDB
.orderByKey()
.equalTo(name)
.on('value', getData, errData);
} catch (err) {
console.log('Error: ', err.message)
res.json({ status: 500, err: 'Error while getting progress' });
}
That will call again the first piece of code with a new res.json, but since the response was already sent, you get that error
Thank you very much! This solved the problem.
– Alexander von Recum
8 hours ago
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
You are getting that error because, your calling res.send() in the middle of your function and the code continues
This piece of code is called
const getData = async data => {
if (data.val()) {
let tmp = await data.val();
progress = parseInt(tmp[name].progressCounter);
console.log(progress);
// Return progress
res.json({ status: 200, data: progress });
} else {
res.json({ status: 500, err: 'No data! ' });
}
since an error occurs, is called
res.json({ status: 500, err: 'No data! ' });
And then this
dataList = await userDB
.orderByKey()
.equalTo(name)
.on('value', getData, errData);
} catch (err) {
console.log('Error: ', err.message)
res.json({ status: 500, err: 'Error while getting progress' });
}
That will call again the first piece of code with a new res.json, but since the response was already sent, you get that error
Thank you very much! This solved the problem.
– Alexander von Recum
8 hours ago
add a comment |
up vote
0
down vote
accepted
You are getting that error because, your calling res.send() in the middle of your function and the code continues
This piece of code is called
const getData = async data => {
if (data.val()) {
let tmp = await data.val();
progress = parseInt(tmp[name].progressCounter);
console.log(progress);
// Return progress
res.json({ status: 200, data: progress });
} else {
res.json({ status: 500, err: 'No data! ' });
}
since an error occurs, is called
res.json({ status: 500, err: 'No data! ' });
And then this
dataList = await userDB
.orderByKey()
.equalTo(name)
.on('value', getData, errData);
} catch (err) {
console.log('Error: ', err.message)
res.json({ status: 500, err: 'Error while getting progress' });
}
That will call again the first piece of code with a new res.json, but since the response was already sent, you get that error
Thank you very much! This solved the problem.
– Alexander von Recum
8 hours ago
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
You are getting that error because, your calling res.send() in the middle of your function and the code continues
This piece of code is called
const getData = async data => {
if (data.val()) {
let tmp = await data.val();
progress = parseInt(tmp[name].progressCounter);
console.log(progress);
// Return progress
res.json({ status: 200, data: progress });
} else {
res.json({ status: 500, err: 'No data! ' });
}
since an error occurs, is called
res.json({ status: 500, err: 'No data! ' });
And then this
dataList = await userDB
.orderByKey()
.equalTo(name)
.on('value', getData, errData);
} catch (err) {
console.log('Error: ', err.message)
res.json({ status: 500, err: 'Error while getting progress' });
}
That will call again the first piece of code with a new res.json, but since the response was already sent, you get that error
You are getting that error because, your calling res.send() in the middle of your function and the code continues
This piece of code is called
const getData = async data => {
if (data.val()) {
let tmp = await data.val();
progress = parseInt(tmp[name].progressCounter);
console.log(progress);
// Return progress
res.json({ status: 200, data: progress });
} else {
res.json({ status: 500, err: 'No data! ' });
}
since an error occurs, is called
res.json({ status: 500, err: 'No data! ' });
And then this
dataList = await userDB
.orderByKey()
.equalTo(name)
.on('value', getData, errData);
} catch (err) {
console.log('Error: ', err.message)
res.json({ status: 500, err: 'Error while getting progress' });
}
That will call again the first piece of code with a new res.json, but since the response was already sent, you get that error
answered yesterday


Pedro Silva
35910
35910
Thank you very much! This solved the problem.
– Alexander von Recum
8 hours ago
add a comment |
Thank you very much! This solved the problem.
– Alexander von Recum
8 hours ago
Thank you very much! This solved the problem.
– Alexander von Recum
8 hours ago
Thank you very much! This solved the problem.
– Alexander von Recum
8 hours ago
add a comment |
Alexander von Recum is a new contributor. Be nice, and check out our Code of Conduct.
Alexander von Recum is a new contributor. Be nice, and check out our Code of Conduct.
Alexander von Recum is a new contributor. Be nice, and check out our Code of Conduct.
Alexander von Recum is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53238436%2fnode-js-firebase-cant-set-headers-after-theyre-sent%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
aiidfP08bjtlv1IPAhF27,WUyGPi