Modifying PagedList in Android Paging Architecture library
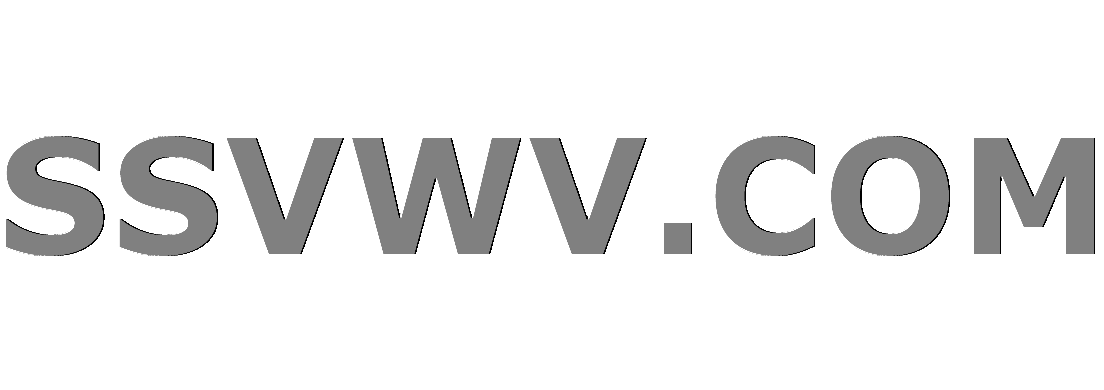
Multi tool use
up vote
3
down vote
favorite
I'm currently looking into incorporating the Paging Architecture library (version 2.1.0-beta01
at the time of writing) into my app. One components is a list which allows the user to delete individual items from it. This list is network-only and caching localy with Room does not make sense.
PagedList
is immutable and does not support modification. I have read that having a copy of the list which is than modified and returned as the new one is the way to go. The documentation states the same:
If you have more granular update signals, such as a network API signaling an update to a single item in the list, it's recommended to load data from the network into memory. Then present that data to the PagedList via a DataSource that wraps an in-memory snapshot. Each time the in-memory copy changes, invalidate the previous DataSource, and a new one wrapping the new state of the snapshot can be created.
I currently have the basic recommended implementation to show a simple list. My DataSource
looks like this:
class MyDataSource<SomeItem> : PageKeyedDataSource<Int, SomeItem>() {
override fun loadInitial(params: LoadInitialParams<Int>, callback: LoadInitialCallback<Int, SomeItem>) {
// Simple load from API and notification of `callback`.
}
override fun loadAfter(params: LoadParams<Int>, callback: LoadCallback<Int, SomeItem>) {
// Simple load from API and notification of `callback`.
}
override fun loadBefore(params: LoadParams<Int>, callback: LoadCallback<Int, SomeItem>) {
// Simple load from API and notification of `callback`.
}
}
How would a concrete implementation of an in-memory cache (without Room and without invalidating the entire dataset) as referenced in the documentation look like?


This question has an open bounty worth +50
reputation from rubengees ending in 6 days.
This question has not received enough attention.
The answer should contain a complete example for the solution, suggested by the documentation or a better approach.
add a comment |
up vote
3
down vote
favorite
I'm currently looking into incorporating the Paging Architecture library (version 2.1.0-beta01
at the time of writing) into my app. One components is a list which allows the user to delete individual items from it. This list is network-only and caching localy with Room does not make sense.
PagedList
is immutable and does not support modification. I have read that having a copy of the list which is than modified and returned as the new one is the way to go. The documentation states the same:
If you have more granular update signals, such as a network API signaling an update to a single item in the list, it's recommended to load data from the network into memory. Then present that data to the PagedList via a DataSource that wraps an in-memory snapshot. Each time the in-memory copy changes, invalidate the previous DataSource, and a new one wrapping the new state of the snapshot can be created.
I currently have the basic recommended implementation to show a simple list. My DataSource
looks like this:
class MyDataSource<SomeItem> : PageKeyedDataSource<Int, SomeItem>() {
override fun loadInitial(params: LoadInitialParams<Int>, callback: LoadInitialCallback<Int, SomeItem>) {
// Simple load from API and notification of `callback`.
}
override fun loadAfter(params: LoadParams<Int>, callback: LoadCallback<Int, SomeItem>) {
// Simple load from API and notification of `callback`.
}
override fun loadBefore(params: LoadParams<Int>, callback: LoadCallback<Int, SomeItem>) {
// Simple load from API and notification of `callback`.
}
}
How would a concrete implementation of an in-memory cache (without Room and without invalidating the entire dataset) as referenced in the documentation look like?


This question has an open bounty worth +50
reputation from rubengees ending in 6 days.
This question has not received enough attention.
The answer should contain a complete example for the solution, suggested by the documentation or a better approach.
add a comment |
up vote
3
down vote
favorite
up vote
3
down vote
favorite
I'm currently looking into incorporating the Paging Architecture library (version 2.1.0-beta01
at the time of writing) into my app. One components is a list which allows the user to delete individual items from it. This list is network-only and caching localy with Room does not make sense.
PagedList
is immutable and does not support modification. I have read that having a copy of the list which is than modified and returned as the new one is the way to go. The documentation states the same:
If you have more granular update signals, such as a network API signaling an update to a single item in the list, it's recommended to load data from the network into memory. Then present that data to the PagedList via a DataSource that wraps an in-memory snapshot. Each time the in-memory copy changes, invalidate the previous DataSource, and a new one wrapping the new state of the snapshot can be created.
I currently have the basic recommended implementation to show a simple list. My DataSource
looks like this:
class MyDataSource<SomeItem> : PageKeyedDataSource<Int, SomeItem>() {
override fun loadInitial(params: LoadInitialParams<Int>, callback: LoadInitialCallback<Int, SomeItem>) {
// Simple load from API and notification of `callback`.
}
override fun loadAfter(params: LoadParams<Int>, callback: LoadCallback<Int, SomeItem>) {
// Simple load from API and notification of `callback`.
}
override fun loadBefore(params: LoadParams<Int>, callback: LoadCallback<Int, SomeItem>) {
// Simple load from API and notification of `callback`.
}
}
How would a concrete implementation of an in-memory cache (without Room and without invalidating the entire dataset) as referenced in the documentation look like?


I'm currently looking into incorporating the Paging Architecture library (version 2.1.0-beta01
at the time of writing) into my app. One components is a list which allows the user to delete individual items from it. This list is network-only and caching localy with Room does not make sense.
PagedList
is immutable and does not support modification. I have read that having a copy of the list which is than modified and returned as the new one is the way to go. The documentation states the same:
If you have more granular update signals, such as a network API signaling an update to a single item in the list, it's recommended to load data from the network into memory. Then present that data to the PagedList via a DataSource that wraps an in-memory snapshot. Each time the in-memory copy changes, invalidate the previous DataSource, and a new one wrapping the new state of the snapshot can be created.
I currently have the basic recommended implementation to show a simple list. My DataSource
looks like this:
class MyDataSource<SomeItem> : PageKeyedDataSource<Int, SomeItem>() {
override fun loadInitial(params: LoadInitialParams<Int>, callback: LoadInitialCallback<Int, SomeItem>) {
// Simple load from API and notification of `callback`.
}
override fun loadAfter(params: LoadParams<Int>, callback: LoadCallback<Int, SomeItem>) {
// Simple load from API and notification of `callback`.
}
override fun loadBefore(params: LoadParams<Int>, callback: LoadCallback<Int, SomeItem>) {
// Simple load from API and notification of `callback`.
}
}
How would a concrete implementation of an in-memory cache (without Room and without invalidating the entire dataset) as referenced in the documentation look like?




edited 23 hours ago
Martin Zeitler
11.5k33559
11.5k33559
asked Nov 10 at 21:43


rubengees
1,2401921
1,2401921
This question has an open bounty worth +50
reputation from rubengees ending in 6 days.
This question has not received enough attention.
The answer should contain a complete example for the solution, suggested by the documentation or a better approach.
This question has an open bounty worth +50
reputation from rubengees ending in 6 days.
This question has not received enough attention.
The answer should contain a complete example for the solution, suggested by the documentation or a better approach.
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53243704%2fmodifying-pagedlist-in-android-paging-architecture-library%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UfEwX 31NH3pmLwbp6CYS