count how many times a prime is dividing a number
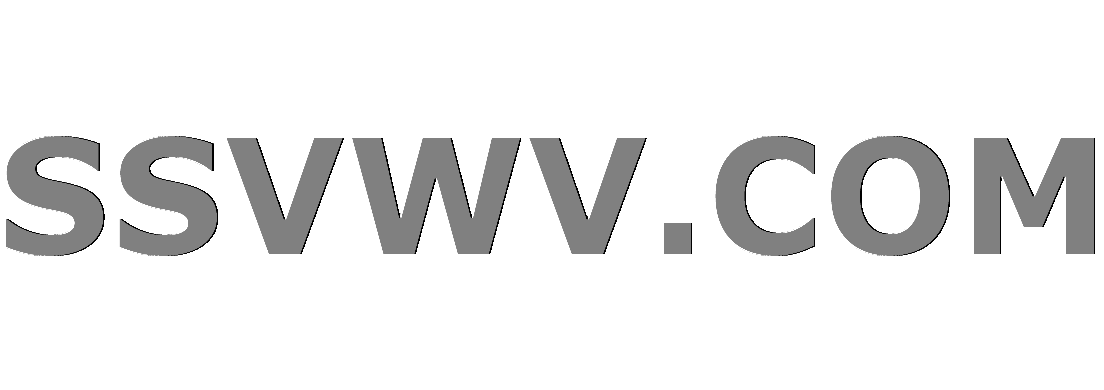
Multi tool use
up vote
0
down vote
favorite
I'm a beginner, my code finds all the primes that divide a given number but I want it to also print how many times the prime divides.
this is my code:
public static void main(){
Scanner myScanner = new Scanner (System.in) ;
int n = myScanner.nextInt();
int prime = 2 ;
int count = 0 ;
while ( prime <= n ){
if ( n%prime == 0 ) {
n = n/prime ;
System.out.println(prime + " " +count);
}
if ( n%prime !=0 ){
prime = prime + 1;
}
}
}
Hope you'll understand me, thanks!!
java primes divide
add a comment |
up vote
0
down vote
favorite
I'm a beginner, my code finds all the primes that divide a given number but I want it to also print how many times the prime divides.
this is my code:
public static void main(){
Scanner myScanner = new Scanner (System.in) ;
int n = myScanner.nextInt();
int prime = 2 ;
int count = 0 ;
while ( prime <= n ){
if ( n%prime == 0 ) {
n = n/prime ;
System.out.println(prime + " " +count);
}
if ( n%prime !=0 ){
prime = prime + 1;
}
}
}
Hope you'll understand me, thanks!!
java primes divide
2
you are not increasing your counter, add "count++;" before the print.
– dorony
Nov 10 at 21:54
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm a beginner, my code finds all the primes that divide a given number but I want it to also print how many times the prime divides.
this is my code:
public static void main(){
Scanner myScanner = new Scanner (System.in) ;
int n = myScanner.nextInt();
int prime = 2 ;
int count = 0 ;
while ( prime <= n ){
if ( n%prime == 0 ) {
n = n/prime ;
System.out.println(prime + " " +count);
}
if ( n%prime !=0 ){
prime = prime + 1;
}
}
}
Hope you'll understand me, thanks!!
java primes divide
I'm a beginner, my code finds all the primes that divide a given number but I want it to also print how many times the prime divides.
this is my code:
public static void main(){
Scanner myScanner = new Scanner (System.in) ;
int n = myScanner.nextInt();
int prime = 2 ;
int count = 0 ;
while ( prime <= n ){
if ( n%prime == 0 ) {
n = n/prime ;
System.out.println(prime + " " +count);
}
if ( n%prime !=0 ){
prime = prime + 1;
}
}
}
Hope you'll understand me, thanks!!
java primes divide
java primes divide
edited Nov 10 at 21:48


Stav Alfi
3,86474078
3,86474078
asked Nov 10 at 21:44
Sapirush Kalifa
6
6
2
you are not increasing your counter, add "count++;" before the print.
– dorony
Nov 10 at 21:54
add a comment |
2
you are not increasing your counter, add "count++;" before the print.
– dorony
Nov 10 at 21:54
2
2
you are not increasing your counter, add "count++;" before the print.
– dorony
Nov 10 at 21:54
you are not increasing your counter, add "count++;" before the print.
– dorony
Nov 10 at 21:54
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
You forgot to increase the counter:
System.out.println(prime + " " +(++count));
add a comment |
up vote
0
down vote
You need to increase the counter for every division that has a remainder 0
and you must exit the loop once the remainder is not 0
:
public static void main(String args) {
Scanner myScanner = new Scanner (System.in) ;
int n = myScanner.nextInt();
int prime = 2;
int count = 0;
while ( prime <= n ){
if ( n % prime == 0 ) {
n /= prime;
count++;
} else
break;
}
System.out.println(prime + " " +count);
}
it's not exectly what I want, lets say n=100, it prints: 2 1 2 2 5 3 5 5 I want it to print: 2 2 5 2
– Sapirush Kalifa
Nov 10 at 22:27
No it print 2 2. Copy the code and run it again
– forpas
Nov 10 at 22:28
@SapirushKalifa did you copy my code or something else?
– forpas
Nov 10 at 22:49
tried, didn't work
– Sapirush Kalifa
Nov 11 at 9:16
What didn't work? Don't you want to print 2 2 when you set number to 100?
– forpas
Nov 11 at 9:17
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
You forgot to increase the counter:
System.out.println(prime + " " +(++count));
add a comment |
up vote
0
down vote
You forgot to increase the counter:
System.out.println(prime + " " +(++count));
add a comment |
up vote
0
down vote
up vote
0
down vote
You forgot to increase the counter:
System.out.println(prime + " " +(++count));
You forgot to increase the counter:
System.out.println(prime + " " +(++count));
answered Nov 10 at 21:58
HaroldSer
1,2762615
1,2762615
add a comment |
add a comment |
up vote
0
down vote
You need to increase the counter for every division that has a remainder 0
and you must exit the loop once the remainder is not 0
:
public static void main(String args) {
Scanner myScanner = new Scanner (System.in) ;
int n = myScanner.nextInt();
int prime = 2;
int count = 0;
while ( prime <= n ){
if ( n % prime == 0 ) {
n /= prime;
count++;
} else
break;
}
System.out.println(prime + " " +count);
}
it's not exectly what I want, lets say n=100, it prints: 2 1 2 2 5 3 5 5 I want it to print: 2 2 5 2
– Sapirush Kalifa
Nov 10 at 22:27
No it print 2 2. Copy the code and run it again
– forpas
Nov 10 at 22:28
@SapirushKalifa did you copy my code or something else?
– forpas
Nov 10 at 22:49
tried, didn't work
– Sapirush Kalifa
Nov 11 at 9:16
What didn't work? Don't you want to print 2 2 when you set number to 100?
– forpas
Nov 11 at 9:17
add a comment |
up vote
0
down vote
You need to increase the counter for every division that has a remainder 0
and you must exit the loop once the remainder is not 0
:
public static void main(String args) {
Scanner myScanner = new Scanner (System.in) ;
int n = myScanner.nextInt();
int prime = 2;
int count = 0;
while ( prime <= n ){
if ( n % prime == 0 ) {
n /= prime;
count++;
} else
break;
}
System.out.println(prime + " " +count);
}
it's not exectly what I want, lets say n=100, it prints: 2 1 2 2 5 3 5 5 I want it to print: 2 2 5 2
– Sapirush Kalifa
Nov 10 at 22:27
No it print 2 2. Copy the code and run it again
– forpas
Nov 10 at 22:28
@SapirushKalifa did you copy my code or something else?
– forpas
Nov 10 at 22:49
tried, didn't work
– Sapirush Kalifa
Nov 11 at 9:16
What didn't work? Don't you want to print 2 2 when you set number to 100?
– forpas
Nov 11 at 9:17
add a comment |
up vote
0
down vote
up vote
0
down vote
You need to increase the counter for every division that has a remainder 0
and you must exit the loop once the remainder is not 0
:
public static void main(String args) {
Scanner myScanner = new Scanner (System.in) ;
int n = myScanner.nextInt();
int prime = 2;
int count = 0;
while ( prime <= n ){
if ( n % prime == 0 ) {
n /= prime;
count++;
} else
break;
}
System.out.println(prime + " " +count);
}
You need to increase the counter for every division that has a remainder 0
and you must exit the loop once the remainder is not 0
:
public static void main(String args) {
Scanner myScanner = new Scanner (System.in) ;
int n = myScanner.nextInt();
int prime = 2;
int count = 0;
while ( prime <= n ){
if ( n % prime == 0 ) {
n /= prime;
count++;
} else
break;
}
System.out.println(prime + " " +count);
}
edited Nov 10 at 22:15
answered Nov 10 at 22:09
forpas
3,4021214
3,4021214
it's not exectly what I want, lets say n=100, it prints: 2 1 2 2 5 3 5 5 I want it to print: 2 2 5 2
– Sapirush Kalifa
Nov 10 at 22:27
No it print 2 2. Copy the code and run it again
– forpas
Nov 10 at 22:28
@SapirushKalifa did you copy my code or something else?
– forpas
Nov 10 at 22:49
tried, didn't work
– Sapirush Kalifa
Nov 11 at 9:16
What didn't work? Don't you want to print 2 2 when you set number to 100?
– forpas
Nov 11 at 9:17
add a comment |
it's not exectly what I want, lets say n=100, it prints: 2 1 2 2 5 3 5 5 I want it to print: 2 2 5 2
– Sapirush Kalifa
Nov 10 at 22:27
No it print 2 2. Copy the code and run it again
– forpas
Nov 10 at 22:28
@SapirushKalifa did you copy my code or something else?
– forpas
Nov 10 at 22:49
tried, didn't work
– Sapirush Kalifa
Nov 11 at 9:16
What didn't work? Don't you want to print 2 2 when you set number to 100?
– forpas
Nov 11 at 9:17
it's not exectly what I want, lets say n=100, it prints: 2 1 2 2 5 3 5 5 I want it to print: 2 2 5 2
– Sapirush Kalifa
Nov 10 at 22:27
it's not exectly what I want, lets say n=100, it prints: 2 1 2 2 5 3 5 5 I want it to print: 2 2 5 2
– Sapirush Kalifa
Nov 10 at 22:27
No it print 2 2. Copy the code and run it again
– forpas
Nov 10 at 22:28
No it print 2 2. Copy the code and run it again
– forpas
Nov 10 at 22:28
@SapirushKalifa did you copy my code or something else?
– forpas
Nov 10 at 22:49
@SapirushKalifa did you copy my code or something else?
– forpas
Nov 10 at 22:49
tried, didn't work
– Sapirush Kalifa
Nov 11 at 9:16
tried, didn't work
– Sapirush Kalifa
Nov 11 at 9:16
What didn't work? Don't you want to print 2 2 when you set number to 100?
– forpas
Nov 11 at 9:17
What didn't work? Don't you want to print 2 2 when you set number to 100?
– forpas
Nov 11 at 9:17
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53243710%2fcount-how-many-times-a-prime-is-dividing-a-number%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
FA9 Oxs1,FN0MsWpfjL5H9d,S1D S,XT8GihIj aBgjM tu,5iFWs
2
you are not increasing your counter, add "count++;" before the print.
– dorony
Nov 10 at 21:54